STM32F411E-Discovery BSP User Manual
|
stm32f411e_discovery_accelerometer.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f411e_discovery_accelerometer.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 31-January-2017 00007 * @brief This file provides a set of functions needed to manage the 00008 * MEMS accelerometer available on STM32F411E-Discovery Kit. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32f411e_discovery_accelerometer.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32F411E_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32F411E_DISCOVERY_ACCELEROMETER STM32F411E DISCOVERY ACCELEROMETER 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32F411E_DISCOVERY_ACCELEROMETER_Private_TypesDefinitions STM32F411E DISCOVERY ACCELEROMETER Private TypesDefinitions 00055 * @{ 00056 */ 00057 /** 00058 * @} 00059 */ 00060 00061 /** @defgroup STM32F411E_DISCOVERY_ACCELEROMETER_Private_Defines STM32F411E DISCOVERY ACCELEROMETER Private Defines 00062 * @{ 00063 */ 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup STM32F411E_DISCOVERY_ACCELEROMETER_Private_Macros STM32F411E DISCOVERY ACCELEROMETER Private Macros 00069 * @{ 00070 */ 00071 /** 00072 * @} 00073 */ 00074 00075 /** @defgroup STM32F411E_DISCOVERY_ACCELEROMETER_Private_Variables STM32F411E DISCOVERY ACCELEROMETER Private Variables 00076 * @{ 00077 */ 00078 static ACCELERO_DrvTypeDef *AccelerometerDrv; 00079 /** 00080 * @} 00081 */ 00082 00083 /** @defgroup STM32F411E_DISCOVERY_ACCELEROMETER_Private_FunctionPrototypes STM32F411E DISCOVERY ACCELEROMETER Private FunctionPrototypes 00084 * @{ 00085 */ 00086 /** 00087 * @} 00088 */ 00089 00090 /** @defgroup STM32F411E_DISCOVERY_ACCELEROMETER_Private_Functions STM32F411E DISCOVERY ACCELEROMETER Private Functions 00091 * @{ 00092 */ 00093 00094 /** 00095 * @brief Set Accelerometer Initialization. 00096 * @retval ACCELERO_OK if no problem during initialization 00097 */ 00098 uint8_t BSP_ACCELERO_Init(void) 00099 { 00100 uint8_t ret = ACCELERO_ERROR; 00101 uint16_t ctrl = 0x0000; 00102 ACCELERO_InitTypeDef LSM303DLHC_InitStructure; 00103 ACCELERO_FilterConfigTypeDef LSM303DLHC_FilterStructure = {0,0,0,0}; 00104 00105 if(Lsm303dlhcDrv.ReadID() == I_AM_LMS303DLHC) 00106 { 00107 /* Initialize the Accelerometer driver structure */ 00108 AccelerometerDrv = &Lsm303dlhcDrv; 00109 00110 /* MEMS configuration ----------------------------------------------------*/ 00111 /* Fill the Accelerometer structure */ 00112 LSM303DLHC_InitStructure.Power_Mode = LSM303DLHC_NORMAL_MODE; 00113 LSM303DLHC_InitStructure.AccOutput_DataRate = LSM303DLHC_ODR_50_HZ; 00114 LSM303DLHC_InitStructure.Axes_Enable = LSM303DLHC_AXES_ENABLE; 00115 LSM303DLHC_InitStructure.AccFull_Scale = LSM303DLHC_FULLSCALE_2G; 00116 LSM303DLHC_InitStructure.BlockData_Update = LSM303DLHC_BlockUpdate_Continous; 00117 LSM303DLHC_InitStructure.Endianness = LSM303DLHC_BLE_LSB; 00118 LSM303DLHC_InitStructure.High_Resolution = LSM303DLHC_HR_ENABLE; 00119 00120 /* Configure MEMS: data rate, power mode, full scale and axes */ 00121 ctrl |= (LSM303DLHC_InitStructure.Power_Mode | LSM303DLHC_InitStructure.AccOutput_DataRate | \ 00122 LSM303DLHC_InitStructure.Axes_Enable); 00123 00124 ctrl |= ((LSM303DLHC_InitStructure.BlockData_Update | LSM303DLHC_InitStructure.Endianness | \ 00125 LSM303DLHC_InitStructure.AccFull_Scale | LSM303DLHC_InitStructure.High_Resolution) << 8); 00126 00127 /* Configure the Accelerometer main parameters */ 00128 AccelerometerDrv->Init(ctrl); 00129 00130 /* Fill the Accelerometer LPF structure */ 00131 LSM303DLHC_FilterStructure.HighPassFilter_Mode_Selection =LSM303DLHC_HPM_NORMAL_MODE; 00132 LSM303DLHC_FilterStructure.HighPassFilter_CutOff_Frequency = LSM303DLHC_HPFCF_16; 00133 LSM303DLHC_FilterStructure.HighPassFilter_AOI1 = LSM303DLHC_HPF_AOI1_DISABLE; 00134 LSM303DLHC_FilterStructure.HighPassFilter_AOI2 = LSM303DLHC_HPF_AOI2_DISABLE; 00135 00136 /* Configure MEMS: mode, cutoff frquency, Filter status, Click, AOI1 and AOI2 */ 00137 ctrl = (uint8_t) (LSM303DLHC_FilterStructure.HighPassFilter_Mode_Selection |\ 00138 LSM303DLHC_FilterStructure.HighPassFilter_CutOff_Frequency|\ 00139 LSM303DLHC_FilterStructure.HighPassFilter_AOI1|\ 00140 LSM303DLHC_FilterStructure.HighPassFilter_AOI2); 00141 00142 /* Configure the Accelerometer LPF main parameters */ 00143 AccelerometerDrv->FilterConfig(ctrl); 00144 00145 ret = ACCELERO_OK; 00146 } 00147 else 00148 { 00149 ret = ACCELERO_ERROR; 00150 } 00151 00152 return ret; 00153 } 00154 00155 /** 00156 * @brief Reboot memory content of Accelerometer. 00157 */ 00158 void BSP_ACCELERO_Reset(void) 00159 { 00160 if(AccelerometerDrv->Reset != NULL) 00161 { 00162 AccelerometerDrv->Reset(); 00163 } 00164 } 00165 00166 /** 00167 * @brief Configure Accelerometer click IT. 00168 */ 00169 void BSP_ACCELERO_Click_ITConfig(void) 00170 { 00171 if(AccelerometerDrv->ConfigIT!= NULL) 00172 { 00173 AccelerometerDrv->ConfigIT(); 00174 } 00175 } 00176 00177 /** 00178 * @brief Get XYZ axes acceleration. 00179 * @param pDataXYZ: Pointer to 3 angular acceleration axes. 00180 * pDataXYZ[0] = X axis, pDataXYZ[1] = Y axis, pDataXYZ[2] = Z axis 00181 */ 00182 void BSP_ACCELERO_GetXYZ(int16_t *pDataXYZ) 00183 { 00184 int16_t SwitchXY = 0; 00185 00186 if(AccelerometerDrv->GetXYZ!= NULL) 00187 { 00188 AccelerometerDrv->GetXYZ(pDataXYZ); 00189 00190 /* Switch X and Y Axes in case of LSM303DLHC MEMS */ 00191 if(AccelerometerDrv == &Lsm303dlhcDrv) 00192 { 00193 SwitchXY = pDataXYZ[0]; 00194 pDataXYZ[0] = pDataXYZ[1]; 00195 00196 /* Invert Y Axis to be conpliant with LIS3DSH */ 00197 pDataXYZ[1] = -SwitchXY; 00198 } 00199 } 00200 } 00201 00202 /** 00203 * @} 00204 */ 00205 00206 /** 00207 * @} 00208 */ 00209 00210 /** 00211 * @} 00212 */ 00213 00214 /** 00215 * @} 00216 */ 00217 00218 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 20 2017 11:38:17 for STM32F411E-Discovery BSP User Manual by
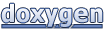