STM32F411E-Discovery BSP User Manual
|
stm32f411e_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f411e_discovery.c 00004 * @author MCD Application Team 00005 * @version V1.0.2 00006 * @date 31-January-2017 00007 * @brief This file provides set of firmware functions to manage LEDs and 00008 * push-button available on STM32F411-Discovery Kit from STMicroelectronics. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32f411e_discovery.h" 00041 00042 /** @defgroup BSP BSP 00043 * @{ 00044 */ 00045 00046 /** @defgroup STM32F411E_DISCOVERY STM32F411E discovery 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32F411E_discovery_LOW_LEVEL STM32F411E discovery LOW LEVEL 00051 * @brief This file provides set of firmware functions to manage Leds and push-button 00052 * available on STM32F411-Discovery Kit from STMicroelectronics. 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32F411E_discovery_LOW_LEVEL_Private_TypesDefinitions STM32F411E discovery LOW LEVEL Privat TypesDefinitions 00057 * @{ 00058 */ 00059 /** 00060 * @} 00061 */ 00062 00063 /** @defgroup STM32F411E_discovery_LOW_LEVEL_Private_Defines STM32F411E discovery LOW LEVEL Private Defines 00064 * @{ 00065 */ 00066 00067 /** 00068 * @brief STM32F411E DISCO BSP Driver version number V1.0.2 00069 */ 00070 #define __STM32F411E_DISCO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00071 #define __STM32F411E_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00072 #define __STM32F411E_DISCO_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00073 #define __STM32F411E_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00074 #define __STM32F411E_DISCO_BSP_VERSION ((__STM32F411E_DISCO_BSP_VERSION_MAIN << 24)\ 00075 |(__STM32F411E_DISCO_BSP_VERSION_SUB1 << 16)\ 00076 |(__STM32F411E_DISCO_BSP_VERSION_SUB2 << 8 )\ 00077 |(__STM32F411E_DISCO_BSP_VERSION_RC)) 00078 /** 00079 * @} 00080 */ 00081 00082 /** @defgroup STM32F411E_discovery_LOW_LEVEL_Private_Macros STM32F411E discovery LOW LEVEL Private Macros 00083 * @{ 00084 */ 00085 /** 00086 * @} 00087 */ 00088 00089 /** @defgroup STM32F411E_discovery_LOW_LEVEL_Private_Variables STM32F411E discovery LOW LEVEL Private Variables 00090 * @{ 00091 */ 00092 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED4_GPIO_PORT, 00093 LED3_GPIO_PORT, 00094 LED5_GPIO_PORT, 00095 LED6_GPIO_PORT}; 00096 00097 const uint16_t GPIO_PIN[LEDn] = {LED4_PIN, 00098 LED3_PIN, 00099 LED5_PIN, 00100 LED6_PIN}; 00101 00102 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {KEY_BUTTON_GPIO_PORT}; 00103 const uint16_t BUTTON_PIN[BUTTONn] = {KEY_BUTTON_PIN}; 00104 const uint8_t BUTTON_IRQn[BUTTONn] = {KEY_BUTTON_EXTI_IRQn}; 00105 00106 uint32_t I2cxTimeout = I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00107 uint32_t SpixTimeout = SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00108 00109 static I2C_HandleTypeDef I2cHandle; 00110 static SPI_HandleTypeDef SpiHandle; 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32F411E_discovery_LOW_LEVEL_Private_FunctionPrototypes STM32F411E discovery LOW LEVEL Private FunctionPrototypes 00116 * @{ 00117 */ 00118 /* I2Cx bus function */ 00119 static void I2Cx_Init(void); 00120 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value); 00121 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg); 00122 static void I2Cx_Error (void); 00123 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00124 00125 /* SPIx bus function */ 00126 static void SPIx_Init(void); 00127 static uint8_t SPIx_WriteRead(uint8_t byte); 00128 static void SPIx_Error (void); 00129 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00130 00131 /* Link function for GYRO peripheral */ 00132 void GYRO_IO_Init(void); 00133 void GYRO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite); 00134 void GYRO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead); 00135 00136 /* Link functions for AUDIO */ 00137 void AUDIO_IO_Init(void); 00138 void AUDIO_IO_DeInit(void); 00139 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00140 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00141 00142 /* Link function for COMPASS / ACCELERO peripheral */ 00143 void COMPASSACCELERO_IO_Init(void); 00144 void COMPASSACCELERO_IO_ITConfig(void); 00145 void COMPASSACCELERO_IO_Write(uint16_t DeviceAddr, uint8_t RegisterAddr, uint8_t Value); 00146 uint8_t COMPASSACCELERO_IO_Read(uint16_t DeviceAddr, uint8_t RegisterAddr); 00147 /** 00148 * @} 00149 */ 00150 00151 /** @defgroup STM32F411E_discovery_LOW_LEVEL_Private_Functions STM32F411E discovery LOW LEVEL Private Functions 00152 * @{ 00153 */ 00154 00155 /** 00156 * @brief This method returns the STM32F411 DISCO BSP Driver revision 00157 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00158 */ 00159 uint32_t BSP_GetVersion(void) 00160 { 00161 return __STM32F411E_DISCO_BSP_VERSION; 00162 } 00163 00164 /** 00165 * @brief Configures LED GPIO. 00166 * @param Led: Specifies the Led to be configured. 00167 * This parameter can be one of following parameters: 00168 * @arg LED4 00169 * @arg LED3 00170 * @arg LED5 00171 * @arg LED6 00172 */ 00173 void BSP_LED_Init(Led_TypeDef Led) 00174 { 00175 GPIO_InitTypeDef GPIO_InitStruct; 00176 00177 /* Enable the GPIO_LED Clock */ 00178 LEDx_GPIO_CLK_ENABLE(Led); 00179 00180 /* Configure the GPIO_LED pin */ 00181 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00182 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00183 GPIO_InitStruct.Pull = GPIO_PULLUP; 00184 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00185 00186 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00187 00188 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00189 } 00190 00191 /** 00192 * @brief Turns selected LED On. 00193 * @param Led: Specifies the Led to be set on. 00194 * This parameter can be one of following parameters: 00195 * @arg LED4 00196 * @arg LED3 00197 * @arg LED5 00198 * @arg LED6 00199 */ 00200 void BSP_LED_On(Led_TypeDef Led) 00201 { 00202 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00203 } 00204 00205 /** 00206 * @brief Turns selected LED Off. 00207 * @param Led: Specifies the Led to be set off. 00208 * This parameter can be one of following parameters: 00209 * @arg LED4 00210 * @arg LED3 00211 * @arg LED5 00212 * @arg LED6 00213 */ 00214 void BSP_LED_Off(Led_TypeDef Led) 00215 { 00216 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00217 } 00218 00219 /** 00220 * @brief Toggles the selected LED. 00221 * @param Led: Specifies the Led to be toggled. 00222 * This parameter can be one of following parameters: 00223 * @arg LED4 00224 * @arg LED3 00225 * @arg LED5 00226 * @arg LED6 00227 */ 00228 void BSP_LED_Toggle(Led_TypeDef Led) 00229 { 00230 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00231 } 00232 00233 /** 00234 * @brief Configures Button GPIO and EXTI Line. 00235 * @param Button: Specifies the Button to be configured. 00236 * This parameter should be: BUTTON_KEY 00237 * @param ButtonMode: Specifies Button mode. 00238 * This parameter can be one of following parameters: 00239 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00240 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00241 * generation capability 00242 */ 00243 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00244 { 00245 GPIO_InitTypeDef GPIO_InitStruct; 00246 00247 /* Enable the BUTTON Clock */ 00248 BUTTONx_GPIO_CLK_ENABLE(Button); 00249 00250 if(ButtonMode == BUTTON_MODE_GPIO) 00251 { 00252 /* Configure Button pin as input */ 00253 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00254 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00255 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00256 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00257 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00258 } 00259 00260 if(ButtonMode == BUTTON_MODE_EXTI) 00261 { 00262 /* Configure Button pin as input with External interrupt */ 00263 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00264 GPIO_InitStruct.Pull = GPIO_NOPULL; 00265 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00266 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00267 00268 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00269 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00270 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00271 } 00272 } 00273 00274 /** 00275 * @brief Returns the selected Button state. 00276 * @param Button: Specifies the Button to be checked. 00277 * This parameter should be: BUTTON_KEY 00278 * @retval The Button GPIO pin value. 00279 */ 00280 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00281 { 00282 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00283 } 00284 00285 /******************************************************************************* 00286 BUS OPERATIONS 00287 *******************************************************************************/ 00288 00289 /******************************* I2C Routines *********************************/ 00290 00291 /** 00292 * @brief I2Cx Bus initialization. 00293 */ 00294 static void I2Cx_Init(void) 00295 { 00296 if(HAL_I2C_GetState(&I2cHandle) == HAL_I2C_STATE_RESET) 00297 { 00298 I2cHandle.Instance = DISCOVERY_I2Cx; 00299 I2cHandle.Init.OwnAddress1 = 0x43; 00300 I2cHandle.Init.ClockSpeed = I2Cx_MAX_COMMUNICATION_FREQ; 00301 I2cHandle.Init.DutyCycle = I2C_DUTYCYCLE_2; 00302 I2cHandle.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00303 I2cHandle.Init.DualAddressMode = I2C_DUALADDRESS_DISABLED; 00304 I2cHandle.Init.OwnAddress2 = 0x00; 00305 I2cHandle.Init.GeneralCallMode = I2C_GENERALCALL_DISABLED; 00306 I2cHandle.Init.NoStretchMode = I2C_NOSTRETCH_DISABLED; 00307 00308 /* Init the I2C */ 00309 I2Cx_MspInit(&I2cHandle); 00310 HAL_I2C_Init(&I2cHandle); 00311 } 00312 } 00313 00314 /** 00315 * @brief Writes a value in a register of the device through BUS. 00316 * @param Addr: Device address on BUS Bus. 00317 * @param Reg: The target register address to write 00318 * @param Value: The target register value to be written 00319 */ 00320 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value) 00321 { 00322 HAL_StatusTypeDef status = HAL_OK; 00323 00324 status = HAL_I2C_Mem_Write(&I2cHandle, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00325 00326 /* Check the communication status */ 00327 if(status != HAL_OK) 00328 { 00329 /* Execute user timeout callback */ 00330 I2Cx_Error(); 00331 } 00332 } 00333 00334 /** 00335 * @brief Reads a register of the device through BUS. 00336 * @param Addr: Device address on BUS Bus. 00337 * @param Reg: The target register address to write 00338 * @retval Data read at register address 00339 */ 00340 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg) 00341 { 00342 HAL_StatusTypeDef status = HAL_OK; 00343 uint8_t value = 0; 00344 00345 status = HAL_I2C_Mem_Read(&I2cHandle, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &value, 1, I2cxTimeout); 00346 00347 /* Check the communication status */ 00348 if(status != HAL_OK) 00349 { 00350 /* Execute user timeout callback */ 00351 I2Cx_Error(); 00352 } 00353 return value; 00354 } 00355 00356 /** 00357 * @brief I2Cx error treatment function. 00358 */ 00359 static void I2Cx_Error(void) 00360 { 00361 /* De-initialize the I2C comunication BUS */ 00362 HAL_I2C_DeInit(&I2cHandle); 00363 00364 /* Re- Initiaize the I2C comunication BUS */ 00365 I2Cx_Init(); 00366 } 00367 00368 /** 00369 * @brief I2Cx MSP Init. 00370 * @param hi2c: I2C handle 00371 */ 00372 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00373 { 00374 GPIO_InitTypeDef GPIO_InitStructure; 00375 00376 /* Enable the I2C peripheral */ 00377 DISCOVERY_I2Cx_CLOCK_ENABLE(); 00378 00379 /* Enable SCK and SDA GPIO clocks */ 00380 DISCOVERY_I2Cx_GPIO_CLK_ENABLE(); 00381 00382 /* I2Cx SD1 & SCK pin configuration */ 00383 GPIO_InitStructure.Pin = DISCOVERY_I2Cx_SDA_PIN | DISCOVERY_I2Cx_SCL_PIN; 00384 GPIO_InitStructure.Mode = GPIO_MODE_AF_OD; 00385 GPIO_InitStructure.Pull = GPIO_NOPULL; 00386 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 00387 GPIO_InitStructure.Alternate = DISCOVERY_I2Cx_AF; 00388 00389 HAL_GPIO_Init(DISCOVERY_I2Cx_GPIO_PORT, &GPIO_InitStructure); 00390 00391 /* Force the I2C peripheral clock reset */ 00392 DISCOVERY_I2Cx_FORCE_RESET(); 00393 00394 /* Release the I2C peripheral clock reset */ 00395 DISCOVERY_I2Cx_RELEASE_RESET(); 00396 00397 /* Enable and set I2Cx Interrupt to the lowest priority */ 00398 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_EV_IRQn, 0x0F, 0); 00399 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_EV_IRQn); 00400 00401 /* Enable and set I2Cx Interrupt to the lowest priority */ 00402 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_ER_IRQn, 0x0F, 0); 00403 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_ER_IRQn); 00404 } 00405 00406 /******************************* SPI Routines**********************************/ 00407 00408 /** 00409 * @brief SPIx Bus initialization. 00410 */ 00411 static void SPIx_Init(void) 00412 { 00413 if(HAL_SPI_GetState(&SpiHandle) == HAL_SPI_STATE_RESET) 00414 { 00415 /* SPI Configuration */ 00416 SpiHandle.Instance = DISCOVERY_SPIx; 00417 /* SPI baudrate is set to 5.6 MHz (PCLK2/SPI_BaudRatePrescaler = 90/16 = 5.625 MHz) 00418 to verify these constraints: 00419 ILI9341 LCD SPI interface max baudrate is 10MHz for write and 6.66MHz for read 00420 L3GD20 SPI interface max baudrate is 10MHz for write/read 00421 PCLK2 frequency is set to 90 MHz 00422 */ 00423 SpiHandle.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00424 SpiHandle.Init.Direction = SPI_DIRECTION_2LINES; 00425 SpiHandle.Init.CLKPhase = SPI_PHASE_1EDGE; 00426 SpiHandle.Init.CLKPolarity = SPI_POLARITY_LOW; 00427 SpiHandle.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLED; 00428 SpiHandle.Init.CRCPolynomial = 7; 00429 SpiHandle.Init.DataSize = SPI_DATASIZE_8BIT; 00430 SpiHandle.Init.FirstBit = SPI_FIRSTBIT_MSB; 00431 SpiHandle.Init.NSS = SPI_NSS_SOFT; 00432 SpiHandle.Init.TIMode = SPI_TIMODE_DISABLED; 00433 SpiHandle.Init.Mode = SPI_MODE_MASTER; 00434 00435 SPIx_MspInit(&SpiHandle); 00436 HAL_SPI_Init(&SpiHandle); 00437 } 00438 } 00439 00440 /** 00441 * @brief Sends a Byte through the SPI interface and return the Byte received 00442 * from the SPI bus. 00443 * @param Byte: Byte send. 00444 * @retval The received byte value 00445 */ 00446 static uint8_t SPIx_WriteRead(uint8_t Byte) 00447 { 00448 uint8_t receivedbyte = 0; 00449 00450 /* Send a Byte through the SPI peripheral */ 00451 /* Read byte from the SPI bus */ 00452 if(HAL_SPI_TransmitReceive(&SpiHandle, (uint8_t*) &Byte, (uint8_t*) &receivedbyte, 1, SpixTimeout) != HAL_OK) 00453 { 00454 SPIx_Error(); 00455 } 00456 00457 return receivedbyte; 00458 } 00459 00460 /** 00461 * @brief SPIx error treatment function. 00462 */ 00463 static void SPIx_Error (void) 00464 { 00465 /* De-initialize the SPI comunication BUS */ 00466 HAL_SPI_DeInit(&SpiHandle); 00467 00468 /* Re-Initiaize the SPI comunication BUS */ 00469 SPIx_Init(); 00470 } 00471 00472 /** 00473 * @brief SPI MSP Init. 00474 * @param hspi: SPI handle 00475 */ 00476 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00477 { 00478 GPIO_InitTypeDef GPIO_InitStructure; 00479 00480 /* Enable SPIx clock */ 00481 DISCOVERY_SPIx_CLOCK_ENABLE(); 00482 00483 /* Enable SPIx GPIO clock */ 00484 DISCOVERY_SPIx_GPIO_CLK_ENABLE(); 00485 00486 /* Configure SPIx SCK, MOSI and MISO */ 00487 GPIO_InitStructure.Pin = (DISCOVERY_SPIx_SCK_PIN | DISCOVERY_SPIx_MOSI_PIN | DISCOVERY_SPIx_MISO_PIN); 00488 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00489 GPIO_InitStructure.Pull = GPIO_PULLDOWN; 00490 GPIO_InitStructure.Speed = GPIO_SPEED_MEDIUM; 00491 GPIO_InitStructure.Alternate = DISCOVERY_SPIx_AF; 00492 HAL_GPIO_Init(DISCOVERY_SPIx_GPIO_PORT, &GPIO_InitStructure); 00493 } 00494 00495 /******************************************************************************* 00496 LINK OPERATIONS 00497 *******************************************************************************/ 00498 00499 /********************************* LINK GYROSCOPE *****************************/ 00500 /** 00501 * @brief Configures the GYRO SPI interface. 00502 */ 00503 void GYRO_IO_Init(void) 00504 { 00505 GPIO_InitTypeDef GPIO_InitStructure; 00506 00507 /* Configure the Gyroscope Control pins ------------------------------------*/ 00508 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 00509 GYRO_CS_GPIO_CLK_ENABLE(); 00510 GPIO_InitStructure.Pin = GYRO_CS_PIN; 00511 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 00512 GPIO_InitStructure.Pull = GPIO_NOPULL; 00513 GPIO_InitStructure.Speed = GPIO_SPEED_MEDIUM; 00514 HAL_GPIO_Init(GYRO_CS_GPIO_PORT, &GPIO_InitStructure); 00515 00516 /* Deselect : Chip Select high */ 00517 GYRO_CS_HIGH(); 00518 00519 /* Enable INT1, INT2 GPIO clock and Configure GPIO PINs to detect Interrupts */ 00520 GYRO_INT_GPIO_CLK_ENABLE(); 00521 GPIO_InitStructure.Pin = GYRO_INT1_PIN | GYRO_INT2_PIN; 00522 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 00523 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 00524 GPIO_InitStructure.Pull= GPIO_NOPULL; 00525 HAL_GPIO_Init(GYRO_INT_GPIO_PORT, &GPIO_InitStructure); 00526 00527 SPIx_Init(); 00528 } 00529 00530 /** 00531 * @brief Writes one byte to the GYRO. 00532 * @param pBuffer: pointer to the buffer containing the data to be written to the GYRO. 00533 * @param WriteAddr : GYRO's internal address to write to. 00534 * @param NumByteToWrite: Number of bytes to write. 00535 */ 00536 void GYRO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) 00537 { 00538 /* Configure the MS bit: 00539 - When 0, the address will remain unchanged in multiple read/write commands. 00540 - When 1, the address will be auto incremented in multiple read/write commands. 00541 */ 00542 if(NumByteToWrite > 0x01) 00543 { 00544 WriteAddr |= (uint8_t)MULTIPLEBYTE_CMD; 00545 } 00546 /* Set chip select Low at the start of the transmission */ 00547 GYRO_CS_LOW(); 00548 00549 /* Send the Address of the indexed register */ 00550 SPIx_WriteRead(WriteAddr); 00551 00552 /* Send the data that will be written into the device (MSB First) */ 00553 while(NumByteToWrite >= 0x01) 00554 { 00555 SPIx_WriteRead(*pBuffer); 00556 NumByteToWrite--; 00557 pBuffer++; 00558 } 00559 00560 /* Set chip select High at the end of the transmission */ 00561 GYRO_CS_HIGH(); 00562 } 00563 00564 /** 00565 * @brief Reads a block of data from the GYRO. 00566 * @param pBuffer: pointer to the buffer that receives the data read from the GYRO. 00567 * @param ReadAddr: GYRO's internal address to read from. 00568 * @param NumByteToRead: Number of bytes to read from the GYRO. 00569 */ 00570 void GYRO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) 00571 { 00572 if(NumByteToRead > 0x01) 00573 { 00574 ReadAddr |= (uint8_t)(READWRITE_CMD | MULTIPLEBYTE_CMD); 00575 } 00576 else 00577 { 00578 ReadAddr |= (uint8_t)READWRITE_CMD; 00579 } 00580 00581 /* Set chip select Low at the start of the transmission */ 00582 GYRO_CS_LOW(); 00583 00584 /* Send the Address of the indexed register */ 00585 SPIx_WriteRead(ReadAddr); 00586 00587 /* Receive the data that will be read from the device (MSB First) */ 00588 while(NumByteToRead > 0x00) 00589 { 00590 /* Send dummy byte (0x00) to generate the SPI clock to GYRO (Slave device) */ 00591 *pBuffer = SPIx_WriteRead(DUMMY_BYTE); 00592 NumByteToRead--; 00593 pBuffer++; 00594 } 00595 00596 /* Set chip select High at the end of the transmission */ 00597 GYRO_CS_HIGH(); 00598 } 00599 00600 /********************************* LINK AUDIO *********************************/ 00601 00602 /** 00603 * @brief Initializes Audio low level. 00604 */ 00605 void AUDIO_IO_Init(void) 00606 { 00607 GPIO_InitTypeDef GPIO_InitStruct; 00608 00609 /* Enable Reset GPIO Clock */ 00610 AUDIO_RESET_GPIO_CLK_ENABLE(); 00611 00612 /* Audio reset pin configuration -------------------------------------------*/ 00613 GPIO_InitStruct.Pin = AUDIO_RESET_PIN; 00614 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00615 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00616 GPIO_InitStruct.Pull = GPIO_NOPULL; 00617 HAL_GPIO_Init(AUDIO_RESET_GPIO, &GPIO_InitStruct); 00618 00619 I2Cx_Init(); 00620 00621 /* Power Down the codec */ 00622 CODEC_AUDIO_POWER_OFF(); 00623 00624 /* Wait for a delay to insure registers erasing */ 00625 HAL_Delay(5); 00626 00627 /* Power on the codec */ 00628 CODEC_AUDIO_POWER_ON(); 00629 00630 /* Wait for a delay to insure registers erasing */ 00631 HAL_Delay(5); 00632 } 00633 00634 /** 00635 * @brief DeInitializes Audio low level. 00636 */ 00637 void AUDIO_IO_DeInit(void) 00638 { 00639 00640 } 00641 00642 /** 00643 * @brief Writes a single data. 00644 * @param Addr: I2C address 00645 * @param Reg: Reg address 00646 * @param Value: Data to be written 00647 */ 00648 void AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) 00649 { 00650 I2Cx_WriteData(Addr, Reg, Value); 00651 } 00652 00653 /** 00654 * @brief Reads a single data. 00655 * @param Addr: I2C address 00656 * @param Reg: Reg address 00657 * @retval Data to be read 00658 */ 00659 uint8_t AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) 00660 { 00661 return I2Cx_ReadData(Addr, Reg); 00662 } 00663 00664 /****************************** LINK ACCELEROMETER ****************************/ 00665 00666 /** 00667 * @brief Configures COMPASS / ACCELERO I2C interface. 00668 */ 00669 void COMPASSACCELERO_IO_Init(void) 00670 { 00671 GPIO_InitTypeDef GPIO_InitStructure; 00672 00673 /* Enable DRDY clock */ 00674 ACCELERO_DRDY_GPIO_CLK_ENABLE(); 00675 00676 /* MEMS DRDY pin configuration */ 00677 GPIO_InitStructure.Pin = ACCELERO_DRDY_PIN; 00678 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 00679 GPIO_InitStructure.Pull = GPIO_NOPULL; 00680 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 00681 HAL_GPIO_Init(ACCELERO_DRDY_GPIO_PORT, &GPIO_InitStructure); 00682 00683 I2Cx_Init(); 00684 } 00685 00686 /** 00687 * @brief Configures COMPASS / ACCELERO click IT. 00688 */ 00689 void COMPASSACCELERO_IO_ITConfig(void) 00690 { 00691 GPIO_InitTypeDef GPIO_InitStructure; 00692 00693 /* Enable INT1 and INT2 GPIO clock */ 00694 ACCELERO_INT_GPIO_CLK_ENABLE(); 00695 00696 /* Configure GPIO PINs to detect Interrupts */ 00697 GPIO_InitStructure.Pin = ACCELERO_INT1_PIN | ACCELERO_INT2_PIN; 00698 GPIO_InitStructure.Mode = GPIO_MODE_IT_RISING; 00699 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 00700 GPIO_InitStructure.Pull = GPIO_NOPULL; 00701 HAL_GPIO_Init(ACCELERO_INT_GPIO_PORT, &GPIO_InitStructure); 00702 00703 /* Enable and set COMPASS / ACCELERO Interrupt to the lowest priority */ 00704 HAL_NVIC_SetPriority(ACCELERO_INT1_EXTI_IRQn, 0x0F, 0x00); 00705 HAL_NVIC_EnableIRQ(ACCELERO_INT1_EXTI_IRQn); 00706 } 00707 00708 /** 00709 * @brief Writes one byte to the COMPASS / ACCELERO. 00710 * @param DeviceAddr: the slave address to be programmed 00711 * @param RegisterAddr: the COMPASS / ACCELERO register to be written 00712 * @param Value: Data to be written 00713 */ 00714 void COMPASSACCELERO_IO_Write(uint16_t DeviceAddr, uint8_t RegisterAddr, uint8_t Value) 00715 { 00716 /* Call I2Cx Read data bus function */ 00717 I2Cx_WriteData(DeviceAddr, RegisterAddr, Value); 00718 } 00719 00720 /** 00721 * @brief Reads a block of data from the COMPASS / ACCELERO. 00722 * @param DeviceAddr: the slave address to be programmed(ACC_I2C_ADDRESS or MAG_I2C_ADDRESS). 00723 * @param RegisterAddr: the COMPASS / ACCELERO internal address register to read from 00724 * @retval COMPASS / ACCELERO register value 00725 */ 00726 uint8_t COMPASSACCELERO_IO_Read(uint16_t DeviceAddr, uint8_t RegisterAddr) 00727 { 00728 /* Call I2Cx Read data bus function */ 00729 return I2Cx_ReadData(DeviceAddr, RegisterAddr); 00730 } 00731 00732 /** 00733 * @} 00734 */ 00735 00736 /** 00737 * @} 00738 */ 00739 00740 /** 00741 * @} 00742 */ 00743 00744 /** 00745 * @} 00746 */ 00747 00748 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 20 2017 11:38:17 for STM32F411E-Discovery BSP User Manual by
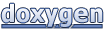