STM32F3-Discovery BSP User Manual
|
stm32f3_discovery_gyroscope.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f3_discovery_gyroscope.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the l3gd20 00006 * MEMS accelerometer available on STM32F3-Discovery Kit. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 /* Includes ------------------------------------------------------------------*/ 00037 #include "stm32f3_discovery_gyroscope.h" 00038 00039 /** @addtogroup BSP 00040 * @{ 00041 */ 00042 00043 /** @addtogroup STM32F3_DISCOVERY 00044 * @{ 00045 */ 00046 00047 /** @defgroup STM32F3_DISCOVERY_GYROSCOPE STM32F3-DISCOVERY GYROSCOPE 00048 * @{ 00049 */ 00050 00051 /* Private typedef -----------------------------------------------------------*/ 00052 /** @defgroup STM32F3_DISCOVERY_GYROSCOPE_Private_Types Private Types 00053 * @{ 00054 */ 00055 /** 00056 * @} 00057 */ 00058 00059 /* Private defines ------------------------------------------------------------*/ 00060 /** @defgroup STM32F3_DISCOVERY_GYROSCOPE_Private_Constants Private Constants 00061 * @{ 00062 */ 00063 /** 00064 * @} 00065 */ 00066 00067 /* Private macros ------------------------------------------------------------*/ 00068 /** @defgroup STM32F3_DISCOVERY_GYROSCOPE_Private_Macros Private Macros 00069 * @{ 00070 */ 00071 /** 00072 * @} 00073 */ 00074 00075 /* Private variables ---------------------------------------------------------*/ 00076 /** @defgroup STM32F3_DISCOVERY_GYROSCOPE_Private_Variables Private Variables 00077 * @{ 00078 */ 00079 static GYRO_DrvTypeDef *GyroscopeDrv; 00080 00081 /** 00082 * @} 00083 */ 00084 00085 /* Private function prototypes -----------------------------------------------*/ 00086 /** @defgroup STM32F3_DISCOVERY_GYROSCOPE_Private_FunctionPrototypes Private Functions 00087 * @{ 00088 */ 00089 /** 00090 * @} 00091 */ 00092 00093 /* Exported functions --------------------------------------------------------*/ 00094 /** @addtogroup STM32F3_DISCOVERY_GYROSCOPE_Exported_Functions 00095 * @{ 00096 */ 00097 00098 /** 00099 * @brief Set GYROSCOPE Initialization. 00100 * @retval GYRO_OK if no problem during initialization 00101 */ 00102 uint8_t BSP_GYRO_Init(void) 00103 { 00104 uint8_t ret = GYRO_ERROR; 00105 uint16_t ctrl = 0x0000; 00106 GYRO_InitTypeDef L3GD20_InitStructure; 00107 GYRO_FilterConfigTypeDef L3GD20_FilterStructure; 00108 00109 if((L3gd20Drv.ReadID() == I_AM_L3GD20) || (L3gd20Drv.ReadID() == I_AM_L3GD20_TR)) 00110 { 00111 /* Initialize the gyroscope driver structure */ 00112 GyroscopeDrv = &L3gd20Drv; 00113 00114 /* Configure Mems : data rate, power mode, full scale and axes */ 00115 L3GD20_InitStructure.Power_Mode = L3GD20_MODE_ACTIVE; 00116 L3GD20_InitStructure.Output_DataRate = L3GD20_OUTPUT_DATARATE_1; 00117 L3GD20_InitStructure.Axes_Enable = L3GD20_AXES_ENABLE; 00118 L3GD20_InitStructure.Band_Width = L3GD20_BANDWIDTH_4; 00119 L3GD20_InitStructure.BlockData_Update = L3GD20_BlockDataUpdate_Continous; 00120 L3GD20_InitStructure.Endianness = L3GD20_BLE_LSB; 00121 L3GD20_InitStructure.Full_Scale = L3GD20_FULLSCALE_500; 00122 00123 /* Configure MEMS: data rate, power mode, full scale and axes */ 00124 ctrl = (uint16_t) (L3GD20_InitStructure.Power_Mode | L3GD20_InitStructure.Output_DataRate | \ 00125 L3GD20_InitStructure.Axes_Enable | L3GD20_InitStructure.Band_Width); 00126 00127 ctrl |= (uint16_t) ((L3GD20_InitStructure.BlockData_Update | L3GD20_InitStructure.Endianness | \ 00128 L3GD20_InitStructure.Full_Scale) << 8); 00129 00130 /* L3gd20 Init */ 00131 GyroscopeDrv->Init(ctrl); 00132 00133 L3GD20_FilterStructure.HighPassFilter_Mode_Selection =L3GD20_HPM_NORMAL_MODE_RES; 00134 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency = L3GD20_HPFCF_0; 00135 00136 ctrl = (uint8_t) ((L3GD20_FilterStructure.HighPassFilter_Mode_Selection |\ 00137 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency)); 00138 00139 GyroscopeDrv->FilterConfig(ctrl) ; 00140 00141 GyroscopeDrv->FilterCmd(L3GD20_HIGHPASSFILTER_ENABLE); 00142 00143 ret = GYRO_OK; 00144 } 00145 else 00146 { 00147 ret = GYRO_ERROR; 00148 } 00149 00150 return ret; 00151 } 00152 00153 /** 00154 * @brief Read ID of Gyroscope component 00155 * @retval ID 00156 */ 00157 uint8_t BSP_GYRO_ReadID(void) 00158 { 00159 uint8_t id = 0x00; 00160 00161 if(GyroscopeDrv->ReadID != NULL) 00162 { 00163 id = GyroscopeDrv->ReadID(); 00164 } 00165 return id; 00166 } 00167 00168 /** 00169 * @brief Reboot memory content of GYROSCOPE 00170 * @retval None 00171 */ 00172 void BSP_GYRO_Reset(void) 00173 { 00174 if(GyroscopeDrv->Reset != NULL) 00175 { 00176 GyroscopeDrv->Reset(); 00177 } 00178 } 00179 00180 00181 /** 00182 * @brief Configure INT1 interrupt 00183 * @param pIntConfig pointer to a L3GD20_InterruptConfig_TypeDef 00184 * structure that contains the configuration setting for the L3GD20 Interrupt. 00185 * @retval None 00186 */ 00187 void BSP_GYRO_ITConfig(GYRO_InterruptConfigTypeDef *pIntConfig) 00188 { 00189 uint16_t interruptconfig = 0x0000; 00190 00191 if(GyroscopeDrv->ConfigIT != NULL) 00192 { 00193 /* Configure latch Interrupt request and axe interrupts */ 00194 interruptconfig |= ((uint8_t)(pIntConfig->Latch_Request| \ 00195 pIntConfig->Interrupt_Axes) << 8); 00196 00197 interruptconfig |= (uint8_t)(pIntConfig->Interrupt_ActiveEdge); 00198 00199 GyroscopeDrv->ConfigIT(interruptconfig); 00200 } 00201 } 00202 00203 /** 00204 * @brief Enable INT1 or INT2 interrupt 00205 * @param IntPin Interrupt pin 00206 * This parameter can be: 00207 * @arg L3GD20_INT1 00208 * @arg L3GD20_INT2 00209 * @retval None 00210 */ 00211 void BSP_GYRO_EnableIT(uint8_t IntPin) 00212 { 00213 if(GyroscopeDrv->EnableIT != NULL) 00214 { 00215 GyroscopeDrv->EnableIT(IntPin); 00216 } 00217 } 00218 00219 /** 00220 * @brief Disable INT1 or INT2 interrupt 00221 * @param IntPin Interrupt pin 00222 * This parameter can be: 00223 * @arg L3GD20_INT1 00224 * @arg L3GD20_INT2 00225 * @retval None 00226 */ 00227 void BSP_GYRO_DisableIT(uint8_t IntPin) 00228 { 00229 if(GyroscopeDrv->DisableIT != NULL) 00230 { 00231 GyroscopeDrv->DisableIT(IntPin); 00232 } 00233 } 00234 00235 /** 00236 * @brief Get XYZ angular acceleration 00237 * @param pfData pointer on floating array 00238 * @retval None 00239 */ 00240 void BSP_GYRO_GetXYZ(float* pfData) 00241 { 00242 if(GyroscopeDrv->GetXYZ!= NULL) 00243 { 00244 GyroscopeDrv->GetXYZ(pfData); 00245 } 00246 } 00247 00248 /** 00249 * @} 00250 */ 00251 00252 /** 00253 * @} 00254 */ 00255 00256 /** 00257 * @} 00258 */ 00259 00260 /** 00261 * @} 00262 */ 00263 00264 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:47:40 for STM32F3-Discovery BSP User Manual by
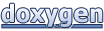