STM32F3-Discovery BSP User Manual
|
stm32f3_discovery_accelerometer.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f3_discovery_accelerometer.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the ACCELEROMETER 00006 * MEMS available on STM32F3-Discovery Kit. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32f3_discovery_accelerometer.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32F3_DISCOVERY 00045 * @{ 00046 */ 00047 00048 /** @defgroup STM32F3_DISCOVERY_ACCELEROMETER STM32F3-DISCOVERY ACCELEROMETER 00049 * @{ 00050 */ 00051 00052 /* Private typedef -----------------------------------------------------------*/ 00053 /** @defgroup STM32F3_DISCOVERY_ACCELEROMETER_Private_Types Private Types 00054 * @{ 00055 */ 00056 /** 00057 * @} 00058 */ 00059 00060 /* Private defines ------------------------------------------------------------*/ 00061 /** @defgroup STM32F3_DISCOVERY_ACCELEROMETER_Private_Constants Private Constants 00062 * @{ 00063 */ 00064 /** 00065 * @} 00066 */ 00067 00068 /* Private macros ------------------------------------------------------------*/ 00069 /** @defgroup STM32F3_DISCOVERY_ACCELEROMETER_Private_Macros Private Macros 00070 * @{ 00071 */ 00072 /** 00073 * @} 00074 */ 00075 00076 /* Private variables ---------------------------------------------------------*/ 00077 /** @defgroup STM32F3_DISCOVERY_ACCELEROMETER_Private_Variables Private Variables 00078 * @{ 00079 */ 00080 static ACCELERO_DrvTypeDef *AccelerometerDrv; 00081 00082 00083 /** 00084 * @} 00085 */ 00086 00087 /* Private function prototypes -----------------------------------------------*/ 00088 /** @addtogroup STM32F3_DISCOVERY_ACCELEROMETER_Private_FunctionPrototypes Private Functions 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /* Exported functions ---------------------------------------------------------*/ 00096 /** @addtogroup STM32F3_DISCOVERY_ACCELEROMETER_Exported_Functions 00097 * @{ 00098 */ 00099 uint8_t BSP_ACCELERO_Init(void) 00100 { 00101 uint8_t ret = ACCELERO_ERROR; 00102 uint16_t ctrl = 0x0000; 00103 ACCELERO_InitTypeDef LSM303DLHC_InitStructure; 00104 ACCELERO_FilterConfigTypeDef LSM303DLHC_FilterStructure; 00105 00106 if(Lsm303dlhcDrv.ReadID() == I_AM_LMS303DLHC) 00107 { 00108 /* Initialize the gyroscope driver structure */ 00109 AccelerometerDrv = &Lsm303dlhcDrv; 00110 00111 /* MEMS configuration ------------------------------------------------------*/ 00112 /* Fill the accelerometer structure */ 00113 LSM303DLHC_InitStructure.Power_Mode = LSM303DLHC_NORMAL_MODE; 00114 LSM303DLHC_InitStructure.AccOutput_DataRate = LSM303DLHC_ODR_50_HZ; 00115 LSM303DLHC_InitStructure.Axes_Enable= LSM303DLHC_AXES_ENABLE; 00116 LSM303DLHC_InitStructure.AccFull_Scale = LSM303DLHC_FULLSCALE_2G; 00117 LSM303DLHC_InitStructure.BlockData_Update = LSM303DLHC_BlockUpdate_Continous; 00118 LSM303DLHC_InitStructure.Endianness=LSM303DLHC_BLE_LSB; 00119 LSM303DLHC_InitStructure.High_Resolution=LSM303DLHC_HR_ENABLE; 00120 00121 /* Configure MEMS: data rate, power mode, full scale and axes */ 00122 ctrl |= (LSM303DLHC_InitStructure.Power_Mode | LSM303DLHC_InitStructure.AccOutput_DataRate | \ 00123 LSM303DLHC_InitStructure.Axes_Enable); 00124 00125 ctrl |= ((LSM303DLHC_InitStructure.BlockData_Update | LSM303DLHC_InitStructure.Endianness | \ 00126 LSM303DLHC_InitStructure.AccFull_Scale | LSM303DLHC_InitStructure.High_Resolution) << 8); 00127 00128 /* Configure the accelerometer main parameters */ 00129 AccelerometerDrv->Init(ctrl); 00130 00131 /* Fill the accelerometer LPF structure */ 00132 LSM303DLHC_FilterStructure.HighPassFilter_Mode_Selection =LSM303DLHC_HPM_NORMAL_MODE; 00133 LSM303DLHC_FilterStructure.HighPassFilter_CutOff_Frequency = LSM303DLHC_HPFCF_16; 00134 LSM303DLHC_FilterStructure.HighPassFilter_AOI1 = LSM303DLHC_HPF_AOI1_DISABLE; 00135 LSM303DLHC_FilterStructure.HighPassFilter_AOI2 = LSM303DLHC_HPF_AOI2_DISABLE; 00136 00137 /* Configure MEMS: mode, cutoff frquency, Filter status, Click, AOI1 and AOI2 */ 00138 ctrl = (uint8_t) (LSM303DLHC_FilterStructure.HighPassFilter_Mode_Selection |\ 00139 LSM303DLHC_FilterStructure.HighPassFilter_CutOff_Frequency|\ 00140 LSM303DLHC_FilterStructure.HighPassFilter_AOI1|\ 00141 LSM303DLHC_FilterStructure.HighPassFilter_AOI2); 00142 00143 /* Configure the accelerometer LPF main parameters */ 00144 AccelerometerDrv->FilterConfig(ctrl); 00145 00146 ret = ACCELERO_OK; 00147 } 00148 else 00149 { 00150 ret = ACCELERO_ERROR; 00151 } 00152 00153 return ret; 00154 } 00155 00156 /** 00157 * @brief Reboot memory content of ACCELEROMETER 00158 * @retval None 00159 */ 00160 void BSP_ACCELERO_Reset(void) 00161 { 00162 if(AccelerometerDrv->Reset != NULL) 00163 { 00164 AccelerometerDrv->Reset(); 00165 } 00166 } 00167 00168 /** 00169 * @brief Get XYZ acceleration 00170 * @param pDataXYZ Pointeur on 3 angular accelerations 00171 * pDataXYZ[0] = X axis, pDataXYZ[1] = Y axis, pDataXYZ[2] = Z axis 00172 * @retval None 00173 */ 00174 void BSP_ACCELERO_GetXYZ(int16_t *pDataXYZ) 00175 { 00176 if(AccelerometerDrv->GetXYZ!= NULL) 00177 { 00178 AccelerometerDrv->GetXYZ(pDataXYZ); 00179 } 00180 } 00181 00182 00183 /** 00184 * @} 00185 */ 00186 00187 /** 00188 * @} 00189 */ 00190 00191 /** 00192 * @} 00193 */ 00194 00195 /** 00196 * @} 00197 */ 00198 00199 00200 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:47:40 for STM32F3-Discovery BSP User Manual by
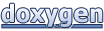