STM32F3-Discovery BSP User Manual
|
stm32f3_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f3_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage Leds and 00006 * push-button available on STM32F3-DISCOVERY Kit from STMicroelectronics. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32f3_discovery.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32F3_DISCOVERY 00045 * @brief This file provides set of firmware functions to manage Leds and push-button 00046 * available on STM32F3-Discovery Kit from STMicroelectronics. 00047 * @{ 00048 */ 00049 /** @addtogroup STM32F3_DISCOVERY_Common 00050 * @{ 00051 */ 00052 00053 /** @addtogroup STM32F3_DISCOVERY_Private_Constants 00054 * @{ 00055 */ 00056 00057 /** 00058 * @brief STM32F3 DISCOVERY BSP Driver version number V2.1.5 00059 */ 00060 #define __STM32F3_DISCO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00061 #define __STM32F3_DISCO_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00062 #define __STM32F3_DISCO_BSP_VERSION_SUB2 (0x05) /*!< [15:8] sub2 version */ 00063 #define __STM32F3_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00064 #define __STM32F3_DISCO_BSP_VERSION ((__STM32F3_DISCO_BSP_VERSION_MAIN << 24)\ 00065 |(__STM32F3_DISCO_BSP_VERSION_SUB1 << 16)\ 00066 |(__STM32F3_DISCO_BSP_VERSION_SUB2 << 8 )\ 00067 |(__STM32F3_DISCO_BSP_VERSION_RC)) 00068 /** 00069 * @} 00070 */ 00071 00072 /** @addtogroup STM32F3_DISCOVERY_Private_Variables 00073 * @{ 00074 */ 00075 /** 00076 * @brief LED variables 00077 */ 00078 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT, LED4_GPIO_PORT, LED5_GPIO_PORT, LED6_GPIO_PORT, 00079 LED7_GPIO_PORT, LED8_GPIO_PORT, LED9_GPIO_PORT, LED10_GPIO_PORT}; 00080 00081 const uint16_t LED_PIN[LEDn] = {LED3_PIN, LED4_PIN, LED5_PIN, LED6_PIN, 00082 LED7_PIN, LED8_PIN, LED9_PIN, LED10_PIN}; 00083 00084 /** 00085 * @brief BUTTON variables 00086 */ 00087 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00088 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00089 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00090 00091 /** 00092 * @brief BUS variables 00093 */ 00094 #ifdef HAL_SPI_MODULE_ENABLED 00095 uint32_t SpixTimeout = SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00096 static SPI_HandleTypeDef SpiHandle; 00097 #endif 00098 00099 #ifdef HAL_I2C_MODULE_ENABLED 00100 static I2C_HandleTypeDef I2cHandle; 00101 uint32_t I2cxTimeout = I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00102 #endif 00103 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM32F3_DISCOVERY_BUS Bus Operation functions 00109 * @{ 00110 */ 00111 #ifdef HAL_I2C_MODULE_ENABLED 00112 /* I2Cx bus function */ 00113 static void I2Cx_Init(void); 00114 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value); 00115 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg); 00116 static void I2Cx_Error (void); 00117 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00118 #endif 00119 00120 #ifdef HAL_SPI_MODULE_ENABLED 00121 /* SPIx bus function */ 00122 static void SPIx_Init(void); 00123 static uint8_t SPIx_WriteRead(uint8_t byte); 00124 static void SPIx_Error (void); 00125 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00126 #endif 00127 00128 #ifdef HAL_SPI_MODULE_ENABLED 00129 /* Link function for GYRO peripheral */ 00130 void GYRO_IO_Init(void); 00131 void GYRO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite); 00132 void GYRO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead); 00133 #endif 00134 00135 #ifdef HAL_I2C_MODULE_ENABLED 00136 /* Link function for COMPASS / ACCELEROMETER peripheral */ 00137 void COMPASSACCELERO_IO_Init(void); 00138 void COMPASSACCELERO_IO_ITConfig(void); 00139 void COMPASSACCELERO_IO_Write(uint16_t DeviceAddr, uint8_t RegisterAddr, uint8_t Value); 00140 uint8_t COMPASSACCELERO_IO_Read(uint16_t DeviceAddr, uint8_t RegisterAddr); 00141 #endif 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** @addtogroup STM32F3_DISCOVERY_Exported_Functions 00148 * @{ 00149 */ 00150 00151 /** 00152 * @brief This method returns the STM32F3-DISCOVERY BSP Driver revision 00153 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00154 */ 00155 uint32_t BSP_GetVersion(void) 00156 { 00157 return __STM32F3_DISCO_BSP_VERSION; 00158 } 00159 00160 /** 00161 * @brief Configures LED GPIO. 00162 * @param Led Specifies the Led to be configured. 00163 * This parameter can be one of following parameters: 00164 * @arg LED_RED 00165 * @arg LED_BLUE 00166 * @arg LED_ORANGE 00167 * @arg LED_GREEN 00168 * @arg LED_GREEN2 00169 * @arg LED_ORANGE2 00170 * @arg LED_BLUE2 00171 * @arg LED_RED2 00172 * @retval None 00173 */ 00174 void BSP_LED_Init(Led_TypeDef Led) 00175 { 00176 GPIO_InitTypeDef GPIO_InitStruct; 00177 00178 /* Enable the GPIO_LED Clock */ 00179 LEDx_GPIO_CLK_ENABLE(Led); 00180 00181 /* Configure the GPIO_LED pin */ 00182 GPIO_InitStruct.Pin = LED_PIN[Led]; 00183 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00184 GPIO_InitStruct.Pull = GPIO_PULLUP; 00185 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00186 00187 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00188 00189 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00190 } 00191 00192 /** 00193 * @brief Turns selected LED On. 00194 * @param Led Specifies the Led to be set on. 00195 * This parameter can be one of following parameters: 00196 * @arg LED_RED 00197 * @arg LED4 00198 * @arg LED5 00199 * @arg LED6 00200 * @arg LED7 00201 * @arg LED8 00202 * @arg LED9 00203 * @arg LED10 00204 * @retval None 00205 */ 00206 void BSP_LED_On(Led_TypeDef Led) 00207 { 00208 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00209 } 00210 00211 /** 00212 * @brief Turns selected LED Off. 00213 * @param Led Specifies the Led to be set off. 00214 * This parameter can be one of following parameters: 00215 * @arg LED_RED 00216 * @arg LED_BLUE 00217 * @arg LED_ORANGE 00218 * @arg LED_GREEN 00219 * @arg LED_GREEN2 00220 * @arg LED_ORANGE2 00221 * @arg LED_BLUE2 00222 * @arg LED_RED2 00223 * @retval None 00224 */ 00225 void BSP_LED_Off(Led_TypeDef Led) 00226 { 00227 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00228 } 00229 00230 /** 00231 * @brief Toggles the selected LED. 00232 * @param Led Specifies the Led to be toggled. 00233 * This parameter can be one of following parameters: 00234 * @arg LED_RED 00235 * @arg LED_BLUE 00236 * @arg LED_ORANGE 00237 * @arg LED_GREEN 00238 * @arg LED_GREEN2 00239 * @arg LED_ORANGE2 00240 * @arg LED_BLUE2 00241 * @arg LED_RED2 00242 * @retval None 00243 */ 00244 void BSP_LED_Toggle(Led_TypeDef Led) 00245 { 00246 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00247 } 00248 00249 00250 /** 00251 * @brief Configures Push Button GPIO and EXTI Line. 00252 * @param Button Specifies the Button to be configured. 00253 * This parameter should be: BUTTON_USER 00254 * @param ButtonMode Specifies Button mode. 00255 * This parameter can be one of following parameters: 00256 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00257 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00258 * generation capability 00259 * @retval None 00260 */ 00261 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00262 { 00263 GPIO_InitTypeDef GPIO_InitStruct; 00264 00265 /* Enable the BUTTON Clock */ 00266 BUTTONx_GPIO_CLK_ENABLE(Button); 00267 __HAL_RCC_SYSCFG_CLK_ENABLE(); 00268 00269 if (ButtonMode == BUTTON_MODE_GPIO) 00270 { 00271 /* Configure Button pin as input */ 00272 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00273 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00274 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00275 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00276 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00277 } 00278 00279 if (ButtonMode == BUTTON_MODE_EXTI) 00280 { 00281 /* Configure Button pin as input with External interrupt */ 00282 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00283 GPIO_InitStruct.Pull = GPIO_NOPULL; 00284 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00285 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00286 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00287 00288 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00289 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00290 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00291 } 00292 } 00293 00294 /** 00295 * @brief Returns the selected Push Button state. 00296 * @param Button Specifies the Button to be checked. 00297 * This parameter should be: BUTTON_USER 00298 * @retval The Button GPIO pin value. 00299 */ 00300 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00301 { 00302 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00303 } 00304 00305 /** 00306 * @} 00307 */ 00308 00309 /** @addtogroup STM32F3_DISCOVERY_BUS 00310 * @{ 00311 */ 00312 /****************************************************************************** 00313 BUS OPERATIONS 00314 *******************************************************************************/ 00315 #ifdef HAL_I2C_MODULE_ENABLED 00316 /******************************* I2C Routines**********************************/ 00317 00318 /** 00319 * @brief Discovery I2Cx MSP Initialization 00320 * @param hi2c I2C handle 00321 * @retval None 00322 */ 00323 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00324 { 00325 00326 GPIO_InitTypeDef GPIO_InitStructure; 00327 00328 /* Enable SCK and SDA GPIO clocks */ 00329 DISCOVERY_I2Cx_GPIO_CLK_ENABLE(); 00330 00331 /* I2Cx SD1 & SCK pin configuration */ 00332 GPIO_InitStructure.Pin = (DISCOVERY_I2Cx_SDA_PIN | DISCOVERY_I2Cx_SCL_PIN); 00333 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00334 GPIO_InitStructure.Pull = GPIO_PULLDOWN; 00335 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00336 GPIO_InitStructure.Alternate = DISCOVERY_I2Cx_AF; 00337 HAL_GPIO_Init(DISCOVERY_I2Cx_GPIO_PORT, &GPIO_InitStructure); 00338 00339 /* Enable the I2C clock */ 00340 DISCOVERY_I2Cx_CLK_ENABLE(); 00341 } 00342 00343 /** 00344 * @brief Discovery I2Cx Bus initialization 00345 * @retval None 00346 */ 00347 static void I2Cx_Init(void) 00348 { 00349 if(HAL_I2C_GetState(&I2cHandle) == HAL_I2C_STATE_RESET) 00350 { 00351 I2cHandle.Instance = DISCOVERY_I2Cx; 00352 I2cHandle.Init.OwnAddress1 = ACCELERO_I2C_ADDRESS; 00353 I2cHandle.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00354 I2cHandle.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00355 I2cHandle.Init.OwnAddress2 = 0; 00356 I2cHandle.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00357 I2cHandle.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00358 00359 /* Init the I2C */ 00360 I2Cx_MspInit(&I2cHandle); 00361 HAL_I2C_Init(&I2cHandle); 00362 } 00363 } 00364 00365 /** 00366 * @brief Write a value in a register of the device through BUS. 00367 * @param Addr Device address on BUS Bus. 00368 * @param Reg The target register address to write 00369 * @param Value The target register value to be written 00370 * @retval None 00371 */ 00372 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value) 00373 { 00374 HAL_StatusTypeDef status = HAL_OK; 00375 00376 status = HAL_I2C_Mem_Write(&I2cHandle, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00377 00378 /* Check the communication status */ 00379 if(status != HAL_OK) 00380 { 00381 /* Execute user timeout callback */ 00382 I2Cx_Error(); 00383 } 00384 } 00385 00386 /** 00387 * @brief Read a value in a register of the device through BUS. 00388 * @param Addr Device address on BUS Bus. 00389 * @param Reg The target register address to write 00390 * @retval Data read at register @ 00391 */ 00392 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg) 00393 { 00394 HAL_StatusTypeDef status = HAL_OK; 00395 uint8_t value = 0; 00396 00397 status = HAL_I2C_Mem_Read(&I2cHandle, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &value, 1, I2cxTimeout); 00398 00399 /* Check the communication status */ 00400 if(status != HAL_OK) 00401 { 00402 /* Execute user timeout callback */ 00403 I2Cx_Error(); 00404 00405 } 00406 return value; 00407 } 00408 00409 /** 00410 * @brief I2C3 error treatment function 00411 * @retval None 00412 */ 00413 static void I2Cx_Error (void) 00414 { 00415 /* De-initialize the I2C comunication BUS */ 00416 HAL_I2C_DeInit(&I2cHandle); 00417 00418 /* Re- Initiaize the I2C comunication BUS */ 00419 I2Cx_Init(); 00420 } 00421 #endif 00422 00423 00424 #ifdef HAL_SPI_MODULE_ENABLED 00425 /******************************* SPI Routines**********************************/ 00426 /** 00427 * @brief SPIx Bus initialization 00428 * @retval None 00429 */ 00430 static void SPIx_Init(void) 00431 { 00432 if(HAL_SPI_GetState(&SpiHandle) == HAL_SPI_STATE_RESET) 00433 { 00434 /* SPI Config */ 00435 SpiHandle.Instance = DISCOVERY_SPIx; 00436 /* SPI baudrate is set to 5.6 MHz (PCLK2/SPI_BaudRatePrescaler = 90/16 = 5.625 MHz) 00437 to verify these constraints: 00438 ILI9341 LCD SPI interface max baudrate is 10MHz for write and 6.66MHz for read 00439 l3gd20 SPI interface max baudrate is 10MHz for write/read 00440 PCLK2 frequency is set to 90 MHz 00441 */ 00442 SpiHandle.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_16; 00443 SpiHandle.Init.Direction = SPI_DIRECTION_2LINES; 00444 SpiHandle.Init.CLKPhase = SPI_PHASE_1EDGE; 00445 SpiHandle.Init.CLKPolarity = SPI_POLARITY_LOW; 00446 SpiHandle.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00447 SpiHandle.Init.CRCPolynomial = 7; 00448 SpiHandle.Init.DataSize = SPI_DATASIZE_8BIT; 00449 SpiHandle.Init.FirstBit = SPI_FIRSTBIT_MSB; 00450 SpiHandle.Init.NSS = SPI_NSS_SOFT; 00451 SpiHandle.Init.TIMode = SPI_TIMODE_DISABLE; 00452 SpiHandle.Init.Mode = SPI_MODE_MASTER; 00453 00454 SPIx_MspInit(&SpiHandle); 00455 HAL_SPI_Init(&SpiHandle); 00456 } 00457 } 00458 00459 /** 00460 * @brief Sends a Byte through the SPI interface and return the Byte received 00461 * from the SPI bus. 00462 * @param Byte Byte send. 00463 * @retval The received byte value 00464 */ 00465 static uint8_t SPIx_WriteRead(uint8_t Byte) 00466 { 00467 00468 uint8_t receivedbyte = 0; 00469 00470 /* Send a Byte through the SPI peripheral */ 00471 /* Read byte from the SPI bus */ 00472 if(HAL_SPI_TransmitReceive(&SpiHandle, (uint8_t*) &Byte, (uint8_t*) &receivedbyte, 1, SpixTimeout) != HAL_OK) 00473 { 00474 SPIx_Error(); 00475 } 00476 00477 return receivedbyte; 00478 } 00479 00480 00481 /** 00482 * @brief SPIx error treatment function 00483 * @retval None 00484 */ 00485 static void SPIx_Error (void) 00486 { 00487 /* De-initialize the SPI comunication BUS */ 00488 HAL_SPI_DeInit(&SpiHandle); 00489 00490 /* Re- Initiaize the SPI comunication BUS */ 00491 SPIx_Init(); 00492 } 00493 00494 00495 /** 00496 * @brief SPI MSP Init 00497 * @param hspi SPI handle 00498 * @retval None 00499 */ 00500 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00501 { 00502 GPIO_InitTypeDef GPIO_InitStructure; 00503 00504 /* Enable SPI1 clock */ 00505 DISCOVERY_SPIx_CLK_ENABLE(); 00506 00507 /* enable SPI1 gpio clock */ 00508 DISCOVERY_SPIx_GPIO_CLK_ENABLE(); 00509 00510 /* configure SPI1 SCK, MOSI and MISO */ 00511 GPIO_InitStructure.Pin = (DISCOVERY_SPIx_SCK_PIN | DISCOVERY_SPIx_MOSI_PIN | DISCOVERY_SPIx_MISO_PIN); 00512 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 00513 GPIO_InitStructure.Pull = GPIO_NOPULL; /* or GPIO_PULLDOWN */ 00514 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00515 GPIO_InitStructure.Alternate = DISCOVERY_SPIx_AF; 00516 HAL_GPIO_Init(DISCOVERY_SPIx_GPIO_PORT, &GPIO_InitStructure); 00517 } 00518 /** 00519 * @} 00520 */ 00521 00522 /** @defgroup STM32F3_DISCOVERY_LINK_OPERATIONS Link Operation functions 00523 * @{ 00524 */ 00525 00526 /****************************************************************************** 00527 LINK OPERATIONS 00528 *******************************************************************************/ 00529 00530 /********************************* LINK GYROSCOPE *****************************/ 00531 /** 00532 * @brief Configures the GYROSCOPE SPI interface. 00533 * @retval None 00534 */ 00535 void GYRO_IO_Init(void) 00536 { 00537 GPIO_InitTypeDef GPIO_InitStructure; 00538 00539 /* Configure the Gyroscope Control pins ------------------------------------------*/ 00540 /* Enable CS GPIO clock and Configure GPIO PIN for Gyroscope Chip select */ 00541 GYRO_CS_GPIO_CLK_ENABLE(); 00542 GPIO_InitStructure.Pin = GYRO_CS_PIN; 00543 GPIO_InitStructure.Mode = GPIO_MODE_OUTPUT_PP; 00544 GPIO_InitStructure.Pull = GPIO_NOPULL; 00545 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00546 HAL_GPIO_Init(GYRO_CS_GPIO_PORT, &GPIO_InitStructure); 00547 00548 /* Deselect : Chip Select high */ 00549 GYRO_CS_HIGH(); 00550 00551 /* Enable INT1, INT2 GPIO clock and Configure GPIO PINs to detect Interrupts */ 00552 GYRO_INT_GPIO_CLK_ENABLE(); 00553 GPIO_InitStructure.Pin = GYRO_INT1_PIN | GYRO_INT2_PIN; 00554 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 00555 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00556 GPIO_InitStructure.Pull= GPIO_NOPULL; 00557 HAL_GPIO_Init(GYRO_INT_GPIO_PORT, &GPIO_InitStructure); 00558 00559 SPIx_Init(); 00560 } 00561 00562 /** 00563 * @brief Writes one byte to the GYROSCOPE. 00564 * @param pBuffer pointer to the buffer containing the data to be written to the GYROSCOPE. 00565 * @param WriteAddr GYROSCOPE's internal address to write to. 00566 * @param NumByteToWrite Number of bytes to write. 00567 * @retval None 00568 */ 00569 void GYRO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) 00570 { 00571 /* Configure the MS bit: 00572 - When 0, the address will remain unchanged in multiple read/write commands. 00573 - When 1, the address will be auto incremented in multiple read/write commands. 00574 */ 00575 if(NumByteToWrite > 0x01) 00576 { 00577 WriteAddr |= (uint8_t)MULTIPLEBYTE_CMD; 00578 } 00579 /* Set chip select Low at the start of the transmission */ 00580 GYRO_CS_LOW(); 00581 00582 /* Send the Address of the indexed register */ 00583 SPIx_WriteRead(WriteAddr); 00584 00585 /* Send the data that will be written into the device (MSB First) */ 00586 while(NumByteToWrite >= 0x01) 00587 { 00588 SPIx_WriteRead(*pBuffer); 00589 NumByteToWrite--; 00590 pBuffer++; 00591 } 00592 00593 /* Set chip select High at the end of the transmission */ 00594 GYRO_CS_HIGH(); 00595 } 00596 00597 /** 00598 * @brief Reads a block of data from the GYROSCOPE. 00599 * @param pBuffer pointer to the buffer that receives the data read from the GYROSCOPE. 00600 * @param ReadAddr GYROSCOPE's internal address to read from. 00601 * @param NumByteToRead number of bytes to read from the GYROSCOPE. 00602 * @retval None 00603 */ 00604 void GYRO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) 00605 { 00606 if(NumByteToRead > 0x01) 00607 { 00608 ReadAddr |= (uint8_t)(READWRITE_CMD | MULTIPLEBYTE_CMD); 00609 } 00610 else 00611 { 00612 ReadAddr |= (uint8_t)READWRITE_CMD; 00613 } 00614 /* Set chip select Low at the start of the transmission */ 00615 GYRO_CS_LOW(); 00616 00617 /* Send the Address of the indexed register */ 00618 SPIx_WriteRead(ReadAddr); 00619 00620 /* Receive the data that will be read from the device (MSB First) */ 00621 while(NumByteToRead > 0x00) 00622 { 00623 /* Send dummy byte (0x00) to generate the SPI clock to GYROSCOPE (Slave device) */ 00624 *pBuffer = SPIx_WriteRead(DUMMY_BYTE); 00625 NumByteToRead--; 00626 pBuffer++; 00627 } 00628 00629 /* Set chip select High at the end of the transmission */ 00630 GYRO_CS_HIGH(); 00631 } 00632 #endif /* HAL_SPI_MODULE_ENABLED */ 00633 00634 #ifdef HAL_I2C_MODULE_ENABLED 00635 /********************************* LINK ACCELEROMETER *****************************/ 00636 /** 00637 * @brief Configures COMPASS / ACCELEROMETER I2C interface. 00638 * @retval None 00639 */ 00640 void COMPASSACCELERO_IO_Init(void) 00641 { 00642 GPIO_InitTypeDef GPIO_InitStructure; 00643 00644 /* Enable DRDY clock */ 00645 ACCELERO_DRDY_GPIO_CLK_ENABLE(); 00646 00647 /* Enable INT1 & INT2 GPIO clock */ 00648 ACCELERO_INT_GPIO_CLK_ENABLE(); 00649 00650 /* Mems DRDY pin configuration */ 00651 GPIO_InitStructure.Pin = ACCELERO_DRDY_PIN; 00652 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 00653 GPIO_InitStructure.Pull = GPIO_NOPULL; 00654 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00655 HAL_GPIO_Init(ACCELERO_DRDY_GPIO_PORT, &GPIO_InitStructure); 00656 00657 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00658 HAL_NVIC_SetPriority(ACCELERO_DRDY_EXTI_IRQn, 0x0F, 0x00); 00659 HAL_NVIC_EnableIRQ(ACCELERO_DRDY_EXTI_IRQn); 00660 00661 /* Configure GPIO PINs to detect Interrupts */ 00662 GPIO_InitStructure.Pin = ACCELERO_INT1_PIN | ACCELERO_INT2_PIN; 00663 GPIO_InitStructure.Mode = GPIO_MODE_INPUT; 00664 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00665 GPIO_InitStructure.Pull = GPIO_NOPULL; 00666 HAL_GPIO_Init(ACCELERO_INT_GPIO_PORT, &GPIO_InitStructure); 00667 00668 I2Cx_Init(); 00669 } 00670 00671 /** 00672 * @brief Configures COMPASS / ACCELERO click IT 00673 * @retval None 00674 */ 00675 void COMPASSACCELERO_IO_ITConfig(void) 00676 { 00677 GPIO_InitTypeDef GPIO_InitStructure; 00678 00679 /* Enable INT1 & INT2 GPIO clock */ 00680 ACCELERO_INT_GPIO_CLK_ENABLE(); 00681 00682 /* Configure GPIO PINs to detect Interrupts */ 00683 GPIO_InitStructure.Pin = ACCELERO_INT1_PIN | ACCELERO_INT2_PIN; 00684 GPIO_InitStructure.Mode = GPIO_MODE_IT_RISING; 00685 GPIO_InitStructure.Speed = GPIO_SPEED_FREQ_HIGH; 00686 GPIO_InitStructure.Pull = GPIO_NOPULL; 00687 HAL_GPIO_Init(ACCELERO_INT_GPIO_PORT, &GPIO_InitStructure); 00688 00689 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00690 HAL_NVIC_SetPriority(ACCELERO_INT1_EXTI_IRQn, 0x0F, 0x00); 00691 HAL_NVIC_EnableIRQ(ACCELERO_INT1_EXTI_IRQn); 00692 00693 } 00694 00695 /** 00696 * @brief Writes one byte to the COMPASS / ACCELEROMETER. 00697 * @param DeviceAddr specifies the slave address to be programmed. 00698 * @param RegisterAddr specifies the COMPASS / ACCELEROMETER register to be written. 00699 * @param Value Data to be written 00700 * @retval None 00701 */ 00702 void COMPASSACCELERO_IO_Write(uint16_t DeviceAddr, uint8_t RegisterAddr, uint8_t Value) 00703 { 00704 /* call I2Cx Read data bus function */ 00705 I2Cx_WriteData(DeviceAddr, RegisterAddr, Value); 00706 } 00707 00708 /** 00709 * @brief Reads a block of data from the COMPASS / ACCELEROMETER. 00710 * @param DeviceAddr specifies the slave address to be programmed(ACC_I2C_ADDRESS or MAG_I2C_ADDRESS). 00711 * @param RegisterAddr specifies the COMPASS / ACCELEROMETER internal address register to read from 00712 * @retval ACCELEROMETER register value 00713 */ 00714 uint8_t COMPASSACCELERO_IO_Read(uint16_t DeviceAddr, uint8_t RegisterAddr) 00715 { 00716 /* call I2Cx Read data bus function */ 00717 return I2Cx_ReadData(DeviceAddr, RegisterAddr); 00718 } 00719 #endif /* HAL_I2C_MODULE_ENABLED */ 00720 00721 00722 00723 /** 00724 * @} 00725 */ 00726 00727 /** 00728 * @} 00729 */ 00730 00731 /** 00732 * @} 00733 */ 00734 00735 /** 00736 * @} 00737 */ 00738 00739 /******************* (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:47:40 for STM32F3-Discovery BSP User Manual by
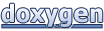