STM32F2xx_Nucleo_144 BSP User Manual
|
stm32f2xx_nucleo_144.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f2xx_nucleo_144.h 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 20-November-2015 00007 * @brief This file contains definitions for: 00008 * - LEDs and push-button available on STM32F2XX-Nucleo-144 Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 #ifndef __STM32F2XX_NUCLEO_144_H 00044 #define __STM32F2XX_NUCLEO_144_H 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /* Includes ------------------------------------------------------------------*/ 00051 #include "stm32f2xx_hal.h" 00052 00053 /* To be defined only if the board is provided with the related shield */ 00054 /* https://www.adafruit.com/products/802 */ 00055 #ifndef ADAFRUIT_TFT_JOY_SD_ID802 00056 #define ADAFRUIT_TFT_JOY_SD_ID802 00057 #endif 00058 00059 /** @addtogroup BSP 00060 * @{ 00061 */ 00062 00063 /** @addtogroup STM32F2XX_NUCLEO_144 00064 * @{ 00065 */ 00066 00067 /** @addtogroup STM32F2XX_NUCLEO_144_LOW_LEVEL 00068 * @{ 00069 */ 00070 00071 /** @defgroup STM32F2XX_NUCLEO_144_LOW_LEVEL_Exported_Types STM32F2XX NUCLEO 144 LOW LEVEL Exported Types 00072 * @{ 00073 */ 00074 typedef enum 00075 { 00076 LED1 = 0, 00077 LED_GREEN = LED1, 00078 LED2 = 1, 00079 LED_BLUE = LED2, 00080 LED3 = 2, 00081 LED_RED = LED3 00082 }Led_TypeDef; 00083 00084 typedef enum 00085 { 00086 BUTTON_USER = 0, 00087 /* Alias */ 00088 BUTTON_KEY = BUTTON_USER 00089 }Button_TypeDef; 00090 00091 typedef enum 00092 { 00093 BUTTON_MODE_GPIO = 0, 00094 BUTTON_MODE_EXTI = 1 00095 }ButtonMode_TypeDef; 00096 00097 typedef enum 00098 { 00099 JOY_NONE = 0, 00100 JOY_SEL = 1, 00101 JOY_DOWN = 2, 00102 JOY_LEFT = 3, 00103 JOY_RIGHT = 4, 00104 JOY_UP = 5 00105 }JOYState_TypeDef; 00106 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32F2XX_NUCLEO_144_LOW_LEVEL_Exported_Constants STM32F2XX NUCLEO 144 LOW LEVEL Exported Constants 00112 * @{ 00113 */ 00114 00115 /** 00116 * @brief Define for STM32F2XX_NUCLEO_144 board 00117 */ 00118 #if !defined (USE_STM32F2XX_NUCLEO_144) 00119 #define USE_STM32F2XX_NUCLEO_144 00120 #endif 00121 00122 /** @defgroup STM32F2XX_NUCLEO_144_LOW_LEVEL_LED STM32F2XX NUCLEO 144 LOW LEVEL LED 00123 * @{ 00124 */ 00125 #define LEDn 3 00126 00127 #define LED1_PIN GPIO_PIN_0 00128 #define LED1_GPIO_PORT GPIOB 00129 #define LED1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00130 #define LED1_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00131 00132 #define LED2_PIN GPIO_PIN_7 00133 #define LED2_GPIO_PORT GPIOB 00134 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00135 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00136 00137 #define LED3_PIN GPIO_PIN_14 00138 #define LED3_GPIO_PORT GPIOB 00139 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00140 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00141 00142 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) {__HAL_RCC_GPIOB_CLK_ENABLE();} else\ 00143 {__HAL_RCC_GPIOB_CLK_ENABLE(); }} while(0) 00144 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) do { if((__INDEX__) == 0) {__HAL_RCC_GPIOB_CLK_DISABLE();} else\ 00145 {__HAL_RCC_GPIOB_CLK_DISABLE(); }} while(0) 00146 /** 00147 * @} 00148 */ 00149 00150 /** @defgroup STM32F2XX_NUCLEO_144_LOW_LEVEL_BUTTON STM32F2XX NUCLEO 144 LOW LEVEL BUTTON 00151 * @{ 00152 */ 00153 #define BUTTONn 1 00154 00155 /** 00156 * @brief Key push-button 00157 */ 00158 #define USER_BUTTON_PIN GPIO_PIN_13 00159 #define USER_BUTTON_GPIO_PORT GPIOC 00160 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00161 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00162 #define USER_BUTTON_EXTI_LINE GPIO_PIN_13 00163 #define USER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00164 00165 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) USER_BUTTON_GPIO_CLK_ENABLE() 00166 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) USER_BUTTON_GPIO_CLK_DISABLE() 00167 00168 /* Aliases */ 00169 #define KEY_BUTTON_PIN USER_BUTTON_PIN 00170 #define KEY_BUTTON_GPIO_PORT USER_BUTTON_GPIO_PORT 00171 #define KEY_BUTTON_GPIO_CLK_ENABLE() USER_BUTTON_GPIO_CLK_ENABLE() 00172 #define KEY_BUTTON_GPIO_CLK_DISABLE() USER_BUTTON_GPIO_CLK_DISABLE() 00173 #define KEY_BUTTON_EXTI_LINE USER_BUTTON_EXTI_LINE 00174 #define KEY_BUTTON_EXTI_IRQn USER_BUTTON_EXTI_IRQn 00175 00176 00177 /** 00178 * @brief OTG_FS1 OVER_CURRENT and POWER_SWITCH Pins definition 00179 */ 00180 00181 00182 #define OTG_FS1_OVER_CURRENT_PIN GPIO_PIN_7 00183 #define OTG_FS1_OVER_CURRENT_PORT GPIOG 00184 #define OTG_FS1_OVER_CURRENT_PORT_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00185 00186 #define OTG_FS1_POWER_SWITCH_PIN GPIO_PIN_6 00187 #define OTG_FS1_POWER_SWITCH_PORT GPIOG 00188 #define OTG_FS1_POWER_SWITCH_PORT_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00189 00190 /** 00191 * @} 00192 */ 00193 00194 /** @defgroup STM32F2XX_NUCLEO_144_LOW_LEVEL_BUS STM32F2XX NUCLEO 144 LOW LEVEL BUS 00195 * @{ 00196 */ 00197 /*############################### SPI_A #######################################*/ 00198 #ifdef HAL_SPI_MODULE_ENABLED 00199 00200 #define NUCLEO_SPIx SPI1 00201 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00202 00203 #define NUCLEO_SPIx_SCK_AF GPIO_AF5_SPI1 00204 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00205 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_5 00206 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00207 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00208 00209 #define NUCLEO_SPIx_MISO_MOSI_AF GPIO_AF5_SPI1 00210 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOA 00211 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00212 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00213 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_6 00214 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_7 00215 /* Maximum Timeout values for flags waiting loops. These timeout are not based 00216 on accurate values, they just guarantee that the application will not remain 00217 stuck if the SPI communication is corrupted. 00218 You may modify these timeout values depending on CPU frequency and application 00219 conditions (interrupts routines ...). */ 00220 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00221 00222 #define NUCLEO_SPIx_CS_GPIO_PORT GPIOD 00223 #define NUCLEO_SPIx_CS_PIN GPIO_PIN_14 00224 #define NUCLEO_SPIx_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00225 #define NUCLEO_SPIx_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00226 00227 #define SPIx__CS_LOW() HAL_GPIO_WritePin(NUCLEO_SPIx_CS_GPIO_PORT, NUCLEO_SPIx_CS_PIN, GPIO_PIN_RESET) 00228 #define SPIx__CS_HIGH() HAL_GPIO_WritePin(NUCLEO_SPIx_CS_GPIO_PORT, NUCLEO_SPIx_CS_PIN, GPIO_PIN_SET) 00229 00230 /** 00231 * @brief SD Control Lines management 00232 */ 00233 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00234 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00235 00236 /** 00237 * @brief LCD Control Lines management 00238 */ 00239 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00240 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00241 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00242 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00243 00244 /** 00245 * @brief SD Control Interface pins (shield D4) 00246 */ 00247 #define SD_CS_PIN GPIO_PIN_14 00248 #define SD_CS_GPIO_PORT GPIOF 00249 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00250 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00251 00252 /** 00253 * @brief LCD Control Interface pins (shield D10) 00254 */ 00255 #define LCD_CS_PIN GPIO_PIN_14 00256 #define LCD_CS_GPIO_PORT GPIOD 00257 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00258 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00259 00260 /** 00261 * @brief LCD Data/Command Interface pins (shield D8) 00262 */ 00263 #define LCD_DC_PIN GPIO_PIN_12 00264 #define LCD_DC_GPIO_PORT GPIOF 00265 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00266 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00267 00268 #endif /* HAL_SPI_MODULE_ENABLED */ 00269 00270 /*################################ ADCx for Nucleo 144 board ######################################*/ 00271 /** 00272 * @brief ADCx Interface pins 00273 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00274 */ 00275 00276 #ifdef HAL_ADC_MODULE_ENABLED 00277 00278 #define NUCLEO_ADCx ADC3 00279 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC3_CLK_ENABLE() 00280 #define NUCLEO_ADCx_CLK_DISABLE() __HAL_RCC_ADC3_CLK_DISABLE() 00281 00282 #define NUCLEO_ADCx_CHANNEL ADC_CHANNEL_9 00283 00284 #define NUCLEO_ADCx_GPIO_PORT GPIOF 00285 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_3 00286 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00287 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00288 00289 #endif /* HAL_ADC_MODULE_ENABLED */ 00290 00291 /** 00292 * @} 00293 */ 00294 00295 /** 00296 * @} 00297 */ 00298 00299 /** @defgroup STM32F2XX_NUCLEO_144_LOW_LEVEL_Exported_Macros STM32F2XX NUCLEO 144 LOW LEVEL Exported Macros 00300 * @{ 00301 */ 00302 /** 00303 * @} 00304 */ 00305 00306 /** @defgroup STM32F2XX_NUCLEO_144_LOW_LEVEL_Exported_Functions STM32F2XX NUCLEO 144 LOW LEVEL Exported Functions 00307 * @{ 00308 */ 00309 uint32_t BSP_GetVersion(void); 00310 void BSP_LED_Init(Led_TypeDef Led); 00311 void BSP_LED_DeInit(Led_TypeDef Led); 00312 void BSP_LED_On(Led_TypeDef Led); 00313 void BSP_LED_Off(Led_TypeDef Led); 00314 void BSP_LED_Toggle(Led_TypeDef Led); 00315 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00316 void BSP_PB_DeInit(Button_TypeDef Button); 00317 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00318 #ifdef HAL_ADC_MODULE_ENABLED 00319 uint8_t BSP_JOY_Init(void); 00320 JOYState_TypeDef BSP_JOY_GetState(void); 00321 void BSP_JOY_DeInit(void); 00322 #endif /* HAL_ADC_MODULE_ENABLED */ 00323 00324 00325 /** 00326 * @} 00327 */ 00328 00329 /** 00330 * @} 00331 */ 00332 00333 /** 00334 * @} 00335 */ 00336 00337 /** 00338 * @} 00339 */ 00340 00341 #ifdef __cplusplus 00342 } 00343 #endif 00344 00345 #endif /* __STM32F2XX_NUCLEO_144_H */ 00346 00347 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 27 2017 11:11:58 for STM32F2xx_Nucleo_144 BSP User Manual by
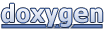