STM32F0xx_Nucleo BSP User Manual
|
stm32f0xx_nucleo.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f0xx_nucleo.h 00004 * @author MCD Application Team 00005 * @version V1.1.4 00006 * @date 04-November-2016 00007 * @brief This file contains definitions for: 00008 * - LEDs and push-button available on STM32F0XX-Nucleo Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 #ifndef __STM32F0XX_NUCLEO_H 00044 #define __STM32F0XX_NUCLEO_H 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32F0XX_NUCLEO STM32F0XX-NUCLEO 00055 * @{ 00056 */ 00057 00058 /* Includes ------------------------------------------------------------------*/ 00059 #include "stm32f0xx_hal.h" 00060 00061 00062 /** @defgroup STM32F0XX_NUCLEO_Exported_Types Exported Types 00063 * @{ 00064 */ 00065 typedef enum 00066 { 00067 LED2 = 0, 00068 LED_GREEN = LED2 00069 } Led_TypeDef; 00070 00071 typedef enum 00072 { 00073 BUTTON_USER = 0, 00074 /* Alias */ 00075 BUTTON_KEY = BUTTON_USER 00076 } Button_TypeDef; 00077 00078 typedef enum 00079 { 00080 BUTTON_MODE_GPIO = 0, 00081 BUTTON_MODE_EXTI = 1 00082 } ButtonMode_TypeDef; 00083 00084 typedef enum 00085 { 00086 JOY_NONE = 0, 00087 JOY_SEL = 1, 00088 JOY_DOWN = 2, 00089 JOY_LEFT = 3, 00090 JOY_RIGHT = 4, 00091 JOY_UP = 5 00092 } JOYState_TypeDef; 00093 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32F0XX_NUCLEO_Exported_Constants Exported Constants 00099 * @{ 00100 */ 00101 00102 /** 00103 * @brief Define for STM32F0XX_NUCLEO board 00104 */ 00105 #if !defined (USE_STM32F0XX_NUCLEO) 00106 #define USE_STM32F0XX_NUCLEO 00107 #endif 00108 00109 /** @defgroup STM32F0XX_NUCLEO_LED LED Constants 00110 * @{ 00111 */ 00112 #define LEDn 1 00113 00114 #define LED2_PIN GPIO_PIN_5 00115 #define LED2_GPIO_PORT GPIOA 00116 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00117 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00118 00119 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) LED2_GPIO_CLK_ENABLE();} while(0) 00120 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? LED2_GPIO_CLK_DISABLE() : 0) 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32F0XX_NUCLEO_BUTTON BUTTON Constants 00127 * @{ 00128 */ 00129 #define BUTTONn 1 00130 00131 /** 00132 * @brief User push-button 00133 */ 00134 #define USER_BUTTON_PIN GPIO_PIN_13 00135 #define USER_BUTTON_GPIO_PORT GPIOC 00136 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00137 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00138 #define USER_BUTTON_EXTI_LINE GPIO_PIN_13 00139 #define USER_BUTTON_EXTI_IRQn EXTI4_15_IRQn 00140 /* Aliases */ 00141 #define KEY_BUTTON_PIN USER_BUTTON_PIN 00142 #define KEY_BUTTON_GPIO_PORT USER_BUTTON_GPIO_PORT 00143 #define KEY_BUTTON_GPIO_CLK_ENABLE() USER_BUTTON_GPIO_CLK_ENABLE() 00144 #define KEY_BUTTON_GPIO_CLK_DISABLE() USER_BUTTON_GPIO_CLK_DISABLE() 00145 #define KEY_BUTTON_EXTI_LINE USER_BUTTON_EXTI_LINE 00146 #define KEY_BUTTON_EXTI_IRQn USER_BUTTON_EXTI_IRQn 00147 00148 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) USER_BUTTON_GPIO_CLK_ENABLE();} while(0) 00149 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? USER_BUTTON_GPIO_CLK_DISABLE() : 0) 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup STM32F0XX_NUCLEO_BUS BUS Constants 00155 * @{ 00156 */ 00157 /*###################### SPI1 ###################################*/ 00158 #define NUCLEO_SPIx SPI1 00159 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00160 00161 #define NUCLEO_SPIx_SCK_AF GPIO_AF0_SPI1 00162 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00163 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_5 00164 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00165 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00166 00167 #define NUCLEO_SPIx_MISO_MOSI_AF GPIO_AF0_SPI1 00168 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOA 00169 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00170 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00171 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_6 00172 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_7 00173 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00174 on accurate values, they just guarantee that the application will not remain 00175 stuck if the SPI communication is corrupted. 00176 You may modify these timeout values depending on CPU frequency and application 00177 conditions (interrupts routines ...). */ 00178 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00179 00180 00181 /** 00182 * @brief SD Control Lines management 00183 */ 00184 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00185 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00186 00187 /** 00188 * @brief LCD Control Lines management 00189 */ 00190 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00191 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00192 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00193 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00194 00195 /** 00196 * @brief SD Control Interface pins (shield D4) 00197 */ 00198 #define SD_CS_PIN GPIO_PIN_5 00199 #define SD_CS_GPIO_PORT GPIOB 00200 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00201 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00202 00203 /** 00204 * @brief LCD Control Interface pins (shield D10) 00205 */ 00206 #define LCD_CS_PIN GPIO_PIN_6 00207 #define LCD_CS_GPIO_PORT GPIOB 00208 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00209 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00210 00211 /** 00212 * @brief LCD Data/Command Interface pins 00213 */ 00214 #define LCD_DC_PIN GPIO_PIN_9 00215 #define LCD_DC_GPIO_PORT GPIOA 00216 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00217 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00218 00219 /*##################### ADC1 ###################################*/ 00220 /** 00221 * @brief ADC Interface pins 00222 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00223 */ 00224 #define NUCLEO_ADCx ADC1 00225 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC1_CLK_ENABLE() 00226 #define NUCLEO_ADCx_CLK_DISABLE() __HAL_RCC_ADC1_CLK_DISABLE() 00227 00228 #define NUCLEO_ADCx_GPIO_PORT GPIOB 00229 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_0 00230 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00231 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00232 00233 /** 00234 * @} 00235 */ 00236 00237 /** 00238 * @} 00239 */ 00240 00241 /** @defgroup STM32F0XX_NUCLEO_Exported_Functions Exported Functions 00242 * @{ 00243 */ 00244 uint32_t BSP_GetVersion(void); 00245 /** @defgroup STM32F0XX_NUCLEO_LED_Functions LED Functions 00246 * @{ 00247 */ 00248 void BSP_LED_Init(Led_TypeDef Led); 00249 void BSP_LED_DeInit(Led_TypeDef Led); 00250 void BSP_LED_On(Led_TypeDef Led); 00251 void BSP_LED_Off(Led_TypeDef Led); 00252 void BSP_LED_Toggle(Led_TypeDef Led); 00253 /** 00254 * @} 00255 */ 00256 00257 /** @addtogroup STM32F0XX_NUCLEO_BUTTON_Functions 00258 * @{ 00259 */ 00260 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00261 void BSP_PB_DeInit(Button_TypeDef Button); 00262 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00263 #if defined(HAL_ADC_MODULE_ENABLED) 00264 uint8_t BSP_JOY_Init(void); 00265 JOYState_TypeDef BSP_JOY_GetState(void); 00266 void BSP_JOY_DeInit(void); 00267 #endif /* HAL_ADC_MODULE_ENABLED */ 00268 00269 00270 /** 00271 * @} 00272 */ 00273 00274 /** 00275 * @} 00276 */ 00277 00278 /** 00279 * @} 00280 */ 00281 00282 /** 00283 * @} 00284 */ 00285 00286 #ifdef __cplusplus 00287 } 00288 #endif 00289 00290 #endif /* __STM32F0XX_NUCLEO_H */ 00291 00292 00293 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00294
Generated on Tue Oct 25 2016 18:35:27 for STM32F0xx_Nucleo BSP User Manual by
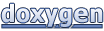