STM32F0xx_Nucleo BSP User Manual
|
stm32f0xx_nucleo.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f0xx_nucleo.c 00004 * @author MCD Application Team 00005 * @version V1.1.4 00006 * @date 04-November-2016 00007 * @brief This file provides set of firmware functions to manage: 00008 * - LEDs and push-button available on STM32F0XX-Nucleo Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "stm32f0xx_nucleo.h" 00044 00045 /** @addtogroup BSP 00046 * @{ 00047 */ 00048 00049 /** @addtogroup STM32F0XX_NUCLEO 00050 * @{ 00051 */ 00052 00053 /** @defgroup STM32F0XX_NUCLEO_Private_Defines Private Defines 00054 * @{ 00055 */ 00056 00057 /** 00058 * @brief STM32F0XX NUCLEO BSP Driver version number V1.1.4 00059 */ 00060 #define __STM32F0XX_NUCLEO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00061 #define __STM32F0XX_NUCLEO_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00062 #define __STM32F0XX_NUCLEO_BSP_VERSION_SUB2 (0x04) /*!< [15:8] sub2 version */ 00063 #define __STM32F0XX_NUCLEO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00064 #define __STM32F0XX_NUCLEO_BSP_VERSION ((__STM32F0XX_NUCLEO_BSP_VERSION_MAIN << 24)\ 00065 |(__STM32F0XX_NUCLEO_BSP_VERSION_SUB1 << 16)\ 00066 |(__STM32F0XX_NUCLEO_BSP_VERSION_SUB2 << 8 )\ 00067 |(__STM32F0XX_NUCLEO_BSP_VERSION_RC)) 00068 00069 /** 00070 * @brief LINK SD Card 00071 */ 00072 #define SD_DUMMY_BYTE 0xFF 00073 #define SD_NO_RESPONSE_EXPECTED 0x80 00074 00075 /** 00076 * @} 00077 */ 00078 00079 /** @defgroup STM32F0XX_NUCLEO_Private_Variables Private Variables 00080 * @{ 00081 */ 00082 GPIO_TypeDef* LED_PORT[LEDn] = {LED2_GPIO_PORT}; 00083 const uint16_t LED_PIN[LEDn] = {LED2_PIN}; 00084 00085 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00086 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00087 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn }; 00088 00089 /** 00090 * @brief BUS variables 00091 */ 00092 00093 #ifdef HAL_SPI_MODULE_ENABLED 00094 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00095 static SPI_HandleTypeDef hnucleo_Spi; 00096 #endif /* HAL_SPI_MODULE_ENABLED */ 00097 00098 #ifdef HAL_ADC_MODULE_ENABLED 00099 static ADC_HandleTypeDef hnucleo_Adc; 00100 /* ADC channel configuration structure declaration */ 00101 static ADC_ChannelConfTypeDef sConfig; 00102 #endif /* HAL_ADC_MODULE_ENABLED */ 00103 /** 00104 * @} 00105 */ 00106 00107 /** @defgroup STM32F0XX_NUCLEO_Private_Functions Private Functions 00108 * @{ 00109 */ 00110 #ifdef HAL_SPI_MODULE_ENABLED 00111 static void SPIx_Init(void); 00112 static void SPIx_Write(uint8_t Value); 00113 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength); 00114 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth); 00115 static void SPIx_FlushFifo(void); 00116 static void SPIx_Error(void); 00117 static void SPIx_MspInit(void); 00118 00119 /* SD IO functions */ 00120 void SD_IO_Init(void); 00121 void SD_IO_CSState(uint8_t state); 00122 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00123 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength); 00124 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength); 00125 uint8_t SD_IO_WriteByte(uint8_t Data); 00126 uint8_t SD_IO_ReadByte(void); 00127 00128 /* LCD IO functions */ 00129 void LCD_IO_Init(void); 00130 void LCD_IO_WriteData(uint8_t Data); 00131 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00132 void LCD_IO_WriteReg(uint8_t LCDReg); 00133 void LCD_Delay(uint32_t delay); 00134 #endif /* HAL_SPI_MODULE_ENABLED */ 00135 00136 #ifdef HAL_ADC_MODULE_ENABLED 00137 static HAL_StatusTypeDef ADCx_Init(void); 00138 static void ADCx_DeInit(void); 00139 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00140 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc); 00141 #endif /* HAL_ADC_MODULE_ENABLED */ 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup STM32F0XX_NUCLEO_Exported_Functions Exported Functions 00147 * @{ 00148 */ 00149 00150 /** 00151 * @brief This method returns the STM32F0XX NUCLEO BSP Driver revision 00152 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00153 */ 00154 uint32_t BSP_GetVersion(void) 00155 { 00156 return __STM32F0XX_NUCLEO_BSP_VERSION; 00157 } 00158 00159 /** @addtogroup STM32F0XX_NUCLEO_LED_Functions 00160 * @{ 00161 */ 00162 00163 /** 00164 * @brief Configures LED GPIO. 00165 * @param Led: Led to be configured. 00166 * This parameter can be one of the following values: 00167 * @arg LED2 00168 * @retval None 00169 */ 00170 void BSP_LED_Init(Led_TypeDef Led) 00171 { 00172 GPIO_InitTypeDef gpioinitstruct; 00173 00174 /* Enable the GPIO_LED Clock */ 00175 LEDx_GPIO_CLK_ENABLE(Led); 00176 00177 /* Configure the GPIO_LED pin */ 00178 gpioinitstruct.Pin = LED_PIN[Led]; 00179 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00180 gpioinitstruct.Pull = GPIO_NOPULL; 00181 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00182 00183 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00184 } 00185 00186 /** 00187 * @brief DeInit LEDs. 00188 * @param Led: LED to be de-init. 00189 * This parameter can be one of the following values: 00190 * @arg LED2 00191 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00192 * @retval None 00193 */ 00194 void BSP_LED_DeInit(Led_TypeDef Led) 00195 { 00196 GPIO_InitTypeDef gpio_init_structure; 00197 00198 /* Turn off LED */ 00199 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00200 /* DeInit the GPIO_LED pin */ 00201 gpio_init_structure.Pin = LED_PIN[Led]; 00202 HAL_GPIO_DeInit(LED_PORT[Led], gpio_init_structure.Pin); 00203 } 00204 00205 /** 00206 * @brief Turns selected LED On. 00207 * @param Led: Specifies the Led to be set on. 00208 * This parameter can be one of following parameters: 00209 * @arg LED2 00210 * @retval None 00211 */ 00212 void BSP_LED_On(Led_TypeDef Led) 00213 { 00214 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00215 } 00216 00217 /** 00218 * @brief Turns selected LED Off. 00219 * @param Led: Specifies the Led to be set off. 00220 * This parameter can be one of following parameters: 00221 * @arg LED2 00222 * @retval None 00223 */ 00224 void BSP_LED_Off(Led_TypeDef Led) 00225 { 00226 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00227 } 00228 00229 /** 00230 * @brief Toggles the selected LED. 00231 * @param Led: Specifies the Led to be toggled. 00232 * This parameter can be one of following parameters: 00233 * @arg LED2 00234 * @retval None 00235 */ 00236 void BSP_LED_Toggle(Led_TypeDef Led) 00237 { 00238 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00239 } 00240 00241 /** 00242 * @} 00243 */ 00244 00245 /** @defgroup STM32F0XX_NUCLEO_BUTTON_Functions BUTTON Functions 00246 * @{ 00247 */ 00248 00249 /** 00250 * @brief Configures Button GPIO and EXTI Line. 00251 * @param Button: Specifies the Button to be configured. 00252 * This parameter should be: BUTTON_USER 00253 * @param ButtonMode: Specifies Button mode. 00254 * This parameter can be one of following parameters: 00255 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00256 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00257 * generation capability 00258 * @retval None 00259 */ 00260 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00261 { 00262 GPIO_InitTypeDef gpioinitstruct; 00263 00264 /* Enable the BUTTON Clock */ 00265 BUTTONx_GPIO_CLK_ENABLE(Button); 00266 00267 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00268 gpioinitstruct.Pull = GPIO_NOPULL; 00269 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00270 00271 if(ButtonMode == BUTTON_MODE_GPIO) 00272 { 00273 /* Configure Button pin as input */ 00274 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00275 00276 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00277 } 00278 00279 if(ButtonMode == BUTTON_MODE_EXTI) 00280 { 00281 /* Configure Button pin as input with External interrupt */ 00282 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00283 00284 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00285 00286 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00287 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x03, 0x00); 00288 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00289 } 00290 } 00291 00292 /** 00293 * @brief Push Button DeInit. 00294 * @param Button: Button to be configured 00295 * This parameter should be: BUTTON_USER 00296 * @note PB DeInit does not disable the GPIO clock 00297 * @retval None 00298 */ 00299 void BSP_PB_DeInit(Button_TypeDef Button) 00300 { 00301 GPIO_InitTypeDef gpio_init_structure; 00302 00303 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00304 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00305 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00306 } 00307 00308 /** 00309 * @brief Returns the selected Button state. 00310 * @param Button: Specifies the Button to be checked. 00311 * This parameter should be: BUTTON_USER 00312 * @retval Button state. 00313 */ 00314 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00315 { 00316 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00317 } 00318 /** 00319 * @} 00320 */ 00321 00322 /** 00323 * @} 00324 */ 00325 00326 /** @addtogroup STM32F0XX_NUCLEO_Private_Functions 00327 * @{ 00328 */ 00329 00330 #ifdef HAL_SPI_MODULE_ENABLED 00331 /****************************************************************************** 00332 BUS OPERATIONS 00333 *******************************************************************************/ 00334 /** 00335 * @brief Initialize SPI MSP. 00336 * @retval None 00337 */ 00338 static void SPIx_MspInit(void) 00339 { 00340 GPIO_InitTypeDef gpioinitstruct = {0}; 00341 00342 /*** Configure the GPIOs ***/ 00343 /* Enable GPIO clock */ 00344 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00345 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00346 00347 /* Configure SPI SCK */ 00348 gpioinitstruct.Pin = NUCLEO_SPIx_SCK_PIN; 00349 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00350 gpioinitstruct.Pull = GPIO_PULLUP; 00351 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00352 gpioinitstruct.Alternate = NUCLEO_SPIx_SCK_AF; 00353 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00354 00355 /* Configure SPI MISO and MOSI */ 00356 gpioinitstruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00357 gpioinitstruct.Alternate = NUCLEO_SPIx_MISO_MOSI_AF; 00358 gpioinitstruct.Pull = GPIO_PULLDOWN; 00359 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00360 00361 gpioinitstruct.Pin = NUCLEO_SPIx_MISO_PIN; 00362 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00363 00364 /*** Configure the SPI peripheral ***/ 00365 /* Enable SPI clock */ 00366 NUCLEO_SPIx_CLK_ENABLE(); 00367 } 00368 00369 /** 00370 * @brief Initialize SPI HAL. 00371 * @retval None 00372 */ 00373 static void SPIx_Init(void) 00374 { 00375 if(HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00376 { 00377 /* SPI Config */ 00378 hnucleo_Spi.Instance = NUCLEO_SPIx; 00379 /* SPI baudrate is set to 12 MHz maximum (PCLK1/SPI_BaudRatePrescaler = 48/4 = 12 MHz) 00380 to verify these constraints: 00381 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00382 Since the provided driver doesn't use read capability from LCD, only constraint 00383 on write baudrate is considered. 00384 - SD card SPI interface max baudrate is 25MHz for write/read 00385 - PCLK1 max frequency is 48 MHz 00386 */ 00387 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_4; 00388 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00389 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00390 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00391 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00392 hnucleo_Spi.Init.CRCLength = SPI_CRC_LENGTH_DATASIZE; 00393 hnucleo_Spi.Init.CRCPolynomial = 7; 00394 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00395 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00396 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00397 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00398 hnucleo_Spi.Init.NSSPMode = SPI_NSS_PULSE_DISABLE; 00399 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00400 00401 SPIx_MspInit(); 00402 HAL_SPI_Init(&hnucleo_Spi); 00403 } 00404 } 00405 00406 /** 00407 * @brief SPI Write a byte to device 00408 * @param DataIn: value to be written 00409 * @param DataOut: read value 00410 * @param DataLength: value data length 00411 * @retval None 00412 */ 00413 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00414 { 00415 HAL_StatusTypeDef status = HAL_OK; 00416 00417 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) DataIn, DataOut, DataLength, SpixTimeout); 00418 00419 /* Check the communication status */ 00420 if(status != HAL_OK) 00421 { 00422 /* Execute user timeout callback */ 00423 SPIx_Error(); 00424 } 00425 } 00426 00427 /** 00428 * @brief SPI Write an amount of data to device 00429 * @param DataIn: value to be written 00430 * @param DataLength: number of bytes to write 00431 * @retval None 00432 */ 00433 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength) 00434 { 00435 HAL_StatusTypeDef status = HAL_OK; 00436 00437 status = HAL_SPI_Transmit(&hnucleo_Spi, DataIn, DataLength, SpixTimeout); 00438 00439 /* Check the communication status */ 00440 if(status != HAL_OK) 00441 { 00442 /* Execute user timeout callback */ 00443 SPIx_Error(); 00444 } 00445 } 00446 00447 /** 00448 * @brief SPI Write a byte to device 00449 * @param Value: value to be written 00450 * @retval None 00451 */ 00452 static void SPIx_Write(uint8_t Value) 00453 { 00454 HAL_StatusTypeDef status = HAL_OK; 00455 uint8_t data; 00456 00457 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00458 00459 /* Check the communication status */ 00460 if(status != HAL_OK) 00461 { 00462 /* Execute user timeout callback */ 00463 SPIx_Error(); 00464 } 00465 } 00466 00467 /** 00468 * @brief SPIx_FlushFifo 00469 * @retval None 00470 */ 00471 static void SPIx_FlushFifo(void) 00472 { 00473 00474 HAL_SPIEx_FlushRxFifo(&hnucleo_Spi); 00475 } 00476 00477 /** 00478 * @brief SPI error treatment function 00479 * @retval None 00480 */ 00481 static void SPIx_Error (void) 00482 { 00483 /* De-initialize the SPI communication BUS */ 00484 HAL_SPI_DeInit(&hnucleo_Spi); 00485 00486 /* Re-Initiaize the SPI communication BUS */ 00487 SPIx_Init(); 00488 } 00489 00490 /****************************************************************************** 00491 LINK OPERATIONS 00492 *******************************************************************************/ 00493 00494 /********************************* LINK SD ************************************/ 00495 /** 00496 * @brief Initialize the SD Card and put it into StandBy State (Ready for 00497 * data transfer). 00498 * @retval None 00499 */ 00500 void SD_IO_Init(void) 00501 { 00502 GPIO_InitTypeDef gpioinitstruct = {0}; 00503 uint8_t counter = 0; 00504 00505 /* SD_CS_GPIO Periph clock enable */ 00506 SD_CS_GPIO_CLK_ENABLE(); 00507 00508 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00509 gpioinitstruct.Pin = SD_CS_PIN; 00510 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00511 gpioinitstruct.Pull = GPIO_PULLUP; 00512 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00513 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00514 00515 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00516 gpioinitstruct.Pin = LCD_CS_PIN; 00517 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00518 gpioinitstruct.Pull = GPIO_NOPULL; 00519 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00520 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00521 LCD_CS_HIGH(); 00522 00523 /*------------Put SD in SPI mode--------------*/ 00524 /* SD SPI Config */ 00525 SPIx_Init(); 00526 00527 /* SD chip select high */ 00528 SD_CS_HIGH(); 00529 00530 /* Send dummy byte 0xFF, 10 times with CS high */ 00531 /* Rise CS and MOSI for 80 clocks cycles */ 00532 for (counter = 0; counter <= 9; counter++) 00533 { 00534 /* Send dummy byte 0xFF */ 00535 SD_IO_WriteByte(SD_DUMMY_BYTE); 00536 } 00537 } 00538 00539 /** 00540 * @brief Set the SD_CS pin. 00541 * @param val: pin value. 00542 * @retval None 00543 */ 00544 void SD_IO_CSState(uint8_t val) 00545 { 00546 if(val == 1) 00547 { 00548 SD_CS_HIGH(); 00549 } 00550 else 00551 { 00552 SD_CS_LOW(); 00553 } 00554 } 00555 00556 /** 00557 * @brief Write byte(s) on the SD 00558 * @param DataIn: Pointer to data buffer to write 00559 * @param DataOut: Pointer to data buffer for read data 00560 * @param DataLength: number of bytes to write 00561 * @retval None 00562 */ 00563 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00564 { 00565 /* Send the byte */ 00566 SPIx_WriteReadData(DataIn, DataOut, DataLength); 00567 } 00568 00569 /** 00570 * @brief Write a byte on the SD. 00571 * @param Data: byte to send. 00572 * @retval Data written 00573 */ 00574 uint8_t SD_IO_WriteByte(uint8_t Data) 00575 { 00576 uint8_t tmp; 00577 00578 /* Send the byte */ 00579 SPIx_WriteReadData(&Data,&tmp,1); 00580 return tmp; 00581 } 00582 00583 /** 00584 * @brief Write an amount of data on the SD. 00585 * @param DataOut: byte to send. 00586 * @param DataLength: number of bytes to write 00587 * @retval none 00588 */ 00589 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength) 00590 { 00591 /* Send the byte */ 00592 SD_IO_WriteReadData(DataOut, DataOut, DataLength); 00593 } 00594 00595 /** 00596 * @brief Write an amount of data on the SD. 00597 * @param Data: byte to send. 00598 * @param DataLength: number of bytes to write 00599 * @retval none 00600 */ 00601 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength) 00602 { 00603 /* Send the byte */ 00604 SPIx_WriteData((uint8_t *)Data, DataLength); 00605 SPIx_FlushFifo(); 00606 } 00607 00608 /********************************* LINK LCD ***********************************/ 00609 /** 00610 * @brief Initialize the LCD 00611 * @retval None 00612 */ 00613 void LCD_IO_Init(void) 00614 { 00615 GPIO_InitTypeDef gpioinitstruct; 00616 00617 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00618 LCD_CS_GPIO_CLK_ENABLE(); 00619 LCD_DC_GPIO_CLK_ENABLE(); 00620 00621 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00622 gpioinitstruct.Pin = LCD_CS_PIN; 00623 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00624 gpioinitstruct.Pull = GPIO_NOPULL; 00625 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00626 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00627 00628 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00629 gpioinitstruct.Pin = LCD_DC_PIN; 00630 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &gpioinitstruct); 00631 00632 /* LCD chip select high */ 00633 LCD_CS_HIGH(); 00634 00635 /* LCD SPI Config */ 00636 SPIx_Init(); 00637 } 00638 00639 /** 00640 * @brief Write command to select the LCD register. 00641 * @param LCDReg: Address of the selected register. 00642 * @retval None 00643 */ 00644 void LCD_IO_WriteReg(uint8_t LCDReg) 00645 { 00646 /* Reset LCD control line CS */ 00647 LCD_CS_LOW(); 00648 00649 /* Set LCD data/command line DC to Low */ 00650 LCD_DC_LOW(); 00651 00652 /* Send Command */ 00653 SPIx_Write(LCDReg); 00654 00655 /* Deselect : Chip Select high */ 00656 LCD_CS_HIGH(); 00657 } 00658 00659 /** 00660 * @brief Write register value. 00661 * @param pData Pointer on the register value 00662 * @param Size Size of byte to transmit to the register 00663 * @retval None 00664 */ 00665 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00666 { 00667 uint32_t counter = 0; 00668 00669 /* Reset LCD control line CS */ 00670 LCD_CS_LOW(); 00671 00672 /* Set LCD data/command line DC to High */ 00673 LCD_DC_HIGH(); 00674 00675 if (Size == 1) 00676 { 00677 /* Only 1 byte to be sent to LCD - general interface can be used */ 00678 /* Send Data */ 00679 SPIx_Write(*pData); 00680 } 00681 else 00682 { 00683 /* Several data should be sent in a raw */ 00684 /* Direct SPI accesses for optimization */ 00685 for (counter = Size; counter != 0; counter--) 00686 { 00687 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00688 { 00689 } 00690 /* Need to invert bytes for LCD*/ 00691 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *(pData+1); 00692 00693 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00694 { 00695 } 00696 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *pData; 00697 counter--; 00698 pData += 2; 00699 } 00700 00701 /* Wait until the bus is ready before releasing Chip select */ 00702 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 00703 { 00704 } 00705 } 00706 00707 /* Empty the Rx fifo */ 00708 SPIx_FlushFifo(); 00709 00710 /* Deselect : Chip Select high */ 00711 LCD_CS_HIGH(); 00712 } 00713 00714 /** 00715 * @brief Wait for loop in ms. 00716 * @param Delay in ms. 00717 * @retval None 00718 */ 00719 void LCD_Delay(uint32_t Delay) 00720 { 00721 HAL_Delay(Delay); 00722 } 00723 00724 #endif /* HAL_SPI_MODULE_ENABLED */ 00725 00726 #ifdef HAL_ADC_MODULE_ENABLED 00727 /******************************* LINK JOYSTICK ********************************/ 00728 /** 00729 * @brief Initialize ADC MSP. 00730 * @retval None 00731 */ 00732 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 00733 { 00734 GPIO_InitTypeDef gpioinitstruct; 00735 00736 /*** Configure the GPIOs ***/ 00737 /* Enable GPIO clock */ 00738 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 00739 00740 /* Configure ADC1 Channel8 as analog input */ 00741 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00742 gpioinitstruct.Mode = GPIO_MODE_ANALOG; 00743 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &gpioinitstruct); 00744 00745 /*** Configure the ADC peripheral ***/ 00746 /* Enable ADC clock */ 00747 NUCLEO_ADCx_CLK_ENABLE(); 00748 } 00749 00750 /** 00751 * @brief DeInitializes ADC MSP. 00752 * @note ADC DeInit does not disable the GPIO clock 00753 * @retval None 00754 */ 00755 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc) 00756 { 00757 GPIO_InitTypeDef gpioinitstruct; 00758 00759 /*** DeInit the ADC peripheral ***/ 00760 /* Disable ADC clock */ 00761 NUCLEO_ADCx_CLK_DISABLE(); 00762 00763 /* Configure the selected ADC Channel as analog input */ 00764 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00765 HAL_GPIO_DeInit(NUCLEO_ADCx_GPIO_PORT, gpioinitstruct.Pin); 00766 00767 /* Disable GPIO clock has to be done by the application*/ 00768 /* NUCLEO_ADCx_GPIO_CLK_DISABLE(); */ 00769 } 00770 00771 /** 00772 * @brief Initializes ADC HAL. 00773 * @retval None 00774 */ 00775 static HAL_StatusTypeDef ADCx_Init(void) 00776 { 00777 /* Set ADC instance */ 00778 hnucleo_Adc.Instance = NUCLEO_ADCx; 00779 00780 if(HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 00781 { 00782 /* ADC Config */ 00783 hnucleo_Adc.Init.ClockPrescaler = ADC_CLOCK_SYNC_PCLK_DIV4; /* ADC clock of STM32F0 must not exceed 14MHz */ 00784 hnucleo_Adc.Init.Resolution = ADC_RESOLUTION_12B; 00785 hnucleo_Adc.Init.DataAlign = ADC_DATAALIGN_RIGHT; 00786 hnucleo_Adc.Init.ScanConvMode = ADC_SCAN_DIRECTION_FORWARD; /* Sequencer will convert the number of channels configured below, successively from the lowest to the highest channel number */ 00787 hnucleo_Adc.Init.EOCSelection = ADC_EOC_SINGLE_CONV; 00788 hnucleo_Adc.Init.LowPowerAutoWait = DISABLE; 00789 hnucleo_Adc.Init.LowPowerAutoPowerOff = DISABLE; 00790 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00791 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00792 hnucleo_Adc.Init.ExternalTrigConv = ADC_SOFTWARE_START; /* Software start to trig the 1st conversion manually, without external event */ 00793 hnucleo_Adc.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because trig by software start */ 00794 hnucleo_Adc.Init.DMAContinuousRequests = DISABLE; 00795 hnucleo_Adc.Init.Overrun = ADC_OVR_DATA_OVERWRITTEN; 00796 hnucleo_Adc.Init.SamplingTimeCommon = ADC_SAMPLETIME_41CYCLES_5; 00797 00798 /* Initialize MSP related to ADC */ 00799 ADCx_MspInit(&hnucleo_Adc); 00800 00801 /* Initialize ADC */ 00802 if (HAL_ADC_Init(&hnucleo_Adc) != HAL_OK) 00803 { 00804 return HAL_ERROR; 00805 } 00806 00807 /* Run ADC calibration */ 00808 if (HAL_ADCEx_Calibration_Start(&hnucleo_Adc) != HAL_OK) 00809 { 00810 return HAL_ERROR; 00811 } 00812 } 00813 00814 return HAL_OK; 00815 } 00816 00817 /** 00818 * @brief Initializes ADC HAL. 00819 * @retval None 00820 */ 00821 static void ADCx_DeInit(void) 00822 { 00823 hnucleo_Adc.Instance = NUCLEO_ADCx; 00824 00825 HAL_ADC_DeInit(&hnucleo_Adc); 00826 ADCx_MspDeInit(&hnucleo_Adc); 00827 } 00828 00829 /******************************* LINK JOYSTICK ********************************/ 00830 00831 /** 00832 * @brief Configures joystick available on adafruit 1.8" TFT shield 00833 * managed through ADC to detect motion. 00834 * @retval Joystickstatus (0=> success, 1=> fail) 00835 */ 00836 uint8_t BSP_JOY_Init(void) 00837 { 00838 if (ADCx_Init() != HAL_OK) 00839 { 00840 return (uint8_t) HAL_ERROR; 00841 } 00842 00843 /* Select Channel 8 to be converted */ 00844 sConfig.Channel = ADC_CHANNEL_8; 00845 sConfig.Rank = ADC_RANK_CHANNEL_NUMBER; 00846 00847 /* Return Joystick initialization status */ 00848 return (uint8_t)HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00849 } 00850 00851 /** 00852 * @brief DeInit joystick GPIOs. 00853 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00854 * @retval None. 00855 */ 00856 void BSP_JOY_DeInit(void) 00857 { 00858 ADCx_DeInit(); 00859 } 00860 00861 /** 00862 * @brief Returns the Joystick key pressed. 00863 * @note To know which Joystick key is pressed we need to detect the voltage 00864 * level on each key output 00865 * - None : 3.3 V / 4095 00866 * - SEL : 1.055 V / 1308 00867 * - DOWN : 0.71 V / 88 00868 * - LEFT : 3.0 V / 3720 00869 * - RIGHT : 0.595 V / 737 00870 * - UP : 1.65 V / 2046 00871 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00872 */ 00873 JOYState_TypeDef BSP_JOY_GetState(void) 00874 { 00875 JOYState_TypeDef state; 00876 uint16_t KeyConvertedValue = 0; 00877 00878 /* Start the conversion process */ 00879 HAL_ADC_Start(&hnucleo_Adc); 00880 00881 /* Wait for the end of conversion */ 00882 if (HAL_ADC_PollForConversion(&hnucleo_Adc, 10) != HAL_TIMEOUT) 00883 { 00884 /* Get the converted value of regular channel */ 00885 KeyConvertedValue = HAL_ADC_GetValue(&hnucleo_Adc); 00886 } 00887 00888 if((KeyConvertedValue > 2010) && (KeyConvertedValue < 2090)) 00889 { 00890 state = JOY_UP; 00891 } 00892 else if((KeyConvertedValue > 680) && (KeyConvertedValue < 780)) 00893 { 00894 state = JOY_RIGHT; 00895 } 00896 else if((KeyConvertedValue > 1270) && (KeyConvertedValue < 1350)) 00897 { 00898 state = JOY_SEL; 00899 } 00900 else if((KeyConvertedValue > 50) && (KeyConvertedValue < 130)) 00901 { 00902 state = JOY_DOWN; 00903 } 00904 else if((KeyConvertedValue > 3680) && (KeyConvertedValue < 3760)) 00905 { 00906 state = JOY_LEFT; 00907 } 00908 else 00909 { 00910 state = JOY_NONE; 00911 } 00912 00913 /* Return the code of the Joystick key pressed */ 00914 return state; 00915 } 00916 #endif /* HAL_ADC_MODULE_ENABLED */ 00917 00918 /** 00919 * @} 00920 */ 00921 00922 /** 00923 * @} 00924 */ 00925 00926 /** 00927 * @} 00928 */ 00929 00930 /** 00931 * @} 00932 */ 00933 00934 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Oct 25 2016 18:35:27 for STM32F0xx_Nucleo BSP User Manual by
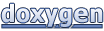