STM32756G_EVAL BSP User Manual
|
stm32756g_eval_nor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval_nor.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes a standard driver for the PC28F128M29EWLA NOR flash memory 00008 * device mounted on STM32756G-EVAL and STM32746G-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the PC28F128M29EWLA NOR flash external memory mounted 00013 on STM32756G-EVAL evaluation board. 00014 - This driver does not need a specific component driver for the NOR device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the NOR external memory using the BSP_NOR_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 FMC controller configuration to interface with the external NOR memory. 00023 00024 + NOR flash operations 00025 o NOR external memory can be accessed with read/write operations once it is 00026 initialized. 00027 Read/write operation can be performed with AHB access using the functions 00028 BSP_NOR_ReadData()/BSP_NOR_WriteData(). The BSP_NOR_WriteData() performs write operation 00029 of an amount of data by unit (halfword). You can also perform a program data 00030 operation of an amount of data using the function BSP_NOR_ProgramData(). 00031 o The function BSP_NOR_Read_ID() returns the chip IDs stored in the structure 00032 "NOR_IDTypeDef". (see the NOR IDs in the memory data sheet) 00033 o Perform erase block operation using the function BSP_NOR_Erase_Block() and by 00034 specifying the block address. You can perform an erase operation of the whole 00035 chip by calling the function BSP_NOR_Erase_Chip(). 00036 o After other operations, the function BSP_NOR_ReturnToReadMode() allows the NOR 00037 flash to return to read mode to perform read operations on it. 00038 @endverbatim 00039 ****************************************************************************** 00040 * @attention 00041 * 00042 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00043 * 00044 * Redistribution and use in source and binary forms, with or without modification, 00045 * are permitted provided that the following conditions are met: 00046 * 1. Redistributions of source code must retain the above copyright notice, 00047 * this list of conditions and the following disclaimer. 00048 * 2. Redistributions in binary form must reproduce the above copyright notice, 00049 * this list of conditions and the following disclaimer in the documentation 00050 * and/or other materials provided with the distribution. 00051 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00052 * may be used to endorse or promote products derived from this software 00053 * without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00056 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00057 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00059 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00060 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00061 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00062 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00063 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00064 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 * 00066 ****************************************************************************** 00067 */ 00068 00069 /* Includes ------------------------------------------------------------------*/ 00070 #include "stm32756g_eval_nor.h" 00071 00072 /** @addtogroup BSP 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32756G_EVAL 00077 * @{ 00078 */ 00079 00080 /** @defgroup STM32756G_EVAL_NOR STM32756G_EVAL NOR 00081 * @{ 00082 */ 00083 00084 /* Private typedef -----------------------------------------------------------*/ 00085 00086 /** @defgroup STM32756G_EVAL_NOR_Private_Types_Definitions NOR Private Types Definitions 00087 * @{ 00088 */ 00089 /** 00090 * @} 00091 */ 00092 /* Private define ------------------------------------------------------------*/ 00093 00094 /** @defgroup STM32756G_EVAL_NOR_Private_Defines NOR Private Defines 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 /* Private macro -------------------------------------------------------------*/ 00101 00102 /** @defgroup STM32756G_EVAL_NOR_Private_Macros NOR Private Macros 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 /* Private variables ---------------------------------------------------------*/ 00109 00110 /** @defgroup STM32756G_EVAL_NOR_Private_Variables NOR Private Variables 00111 * @{ 00112 */ 00113 static NOR_HandleTypeDef norHandle; 00114 static FMC_NORSRAM_TimingTypeDef Timing; 00115 00116 /** 00117 * @} 00118 */ 00119 00120 /* Private function prototypes -----------------------------------------------*/ 00121 00122 /** @defgroup STM32756G_EVAL_NOR_Private_Functions_Prototypes NOR Private Functions Prototypes 00123 * @{ 00124 */ 00125 /** 00126 * @} 00127 */ 00128 /* Private functions ---------------------------------------------------------*/ 00129 00130 /** @defgroup STM32756G_EVAL_NOR_Private_Functions NOR Private Functions 00131 * @{ 00132 */ 00133 00134 /** 00135 * @brief Initializes the NOR device. 00136 * @retval NOR memory status 00137 */ 00138 uint8_t BSP_NOR_Init(void) 00139 { 00140 static uint8_t nor_status = NOR_STATUS_ERROR; 00141 norHandle.Instance = FMC_NORSRAM_DEVICE; 00142 norHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00143 00144 /* NOR device configuration */ 00145 /* Timing configuration derived from system clock (up to 216Mhz) 00146 for 108Mhz as NOR clock frequency */ 00147 Timing.AddressSetupTime = 4; 00148 Timing.AddressHoldTime = 3; 00149 Timing.DataSetupTime = 8; 00150 Timing.BusTurnAroundDuration = 1; 00151 Timing.CLKDivision = 2; 00152 Timing.DataLatency = 2; 00153 Timing.AccessMode = FMC_ACCESS_MODE_A; 00154 00155 norHandle.Init.NSBank = FMC_NORSRAM_BANK1; 00156 norHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00157 norHandle.Init.MemoryType = FMC_MEMORY_TYPE_NOR; 00158 norHandle.Init.MemoryDataWidth = NOR_MEMORY_WIDTH; 00159 norHandle.Init.BurstAccessMode = NOR_BURSTACCESS; 00160 norHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00161 norHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00162 norHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00163 norHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_ENABLE; 00164 norHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00165 norHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_ENABLE; 00166 norHandle.Init.WriteBurst = NOR_WRITEBURST; 00167 norHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00168 00169 /* NOR controller initialization */ 00170 BSP_NOR_MspInit(&norHandle, NULL); /* __weak function can be rewritten by the application */ 00171 00172 if(HAL_NOR_Init(&norHandle, &Timing, &Timing) != HAL_OK) 00173 { 00174 nor_status = NOR_STATUS_ERROR; 00175 } 00176 else 00177 { 00178 nor_status = NOR_STATUS_OK; 00179 } 00180 return nor_status; 00181 } 00182 00183 /** 00184 * @brief DeInitializes the NOR device. 00185 * @retval NOR status 00186 */ 00187 uint8_t BSP_NOR_DeInit(void) 00188 { 00189 static uint8_t nor_status = NOR_ERROR; 00190 /* NOR device de-initialization */ 00191 norHandle.Instance = FMC_NORSRAM_DEVICE; 00192 norHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00193 00194 if(HAL_NOR_DeInit(&norHandle) != HAL_OK) 00195 { 00196 nor_status = NOR_STATUS_ERROR; 00197 } 00198 else 00199 { 00200 nor_status = NOR_STATUS_OK; 00201 } 00202 00203 /* NOR controller de-initialization */ 00204 BSP_NOR_MspDeInit(&norHandle, NULL); 00205 00206 return nor_status; 00207 } 00208 00209 /** 00210 * @brief Reads an amount of data from the NOR device. 00211 * @param uwStartAddress: Read start address 00212 * @param pData: Pointer to data to be read 00213 * @param uwDataSize: Size of data to read 00214 * @retval NOR memory status 00215 */ 00216 uint8_t BSP_NOR_ReadData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00217 { 00218 if(HAL_NOR_ReadBuffer(&norHandle, NOR_DEVICE_ADDR + uwStartAddress, pData, uwDataSize) != HAL_OK) 00219 { 00220 return NOR_STATUS_ERROR; 00221 } 00222 else 00223 { 00224 return NOR_STATUS_OK; 00225 } 00226 } 00227 00228 /** 00229 * @brief Returns the NOR memory to read mode. 00230 * @retval None 00231 */ 00232 void BSP_NOR_ReturnToReadMode(void) 00233 { 00234 HAL_NOR_ReturnToReadMode(&norHandle); 00235 } 00236 00237 /** 00238 * @brief Writes an amount of data to the NOR device. 00239 * @param uwStartAddress: Write start address 00240 * @param pData: Pointer to data to be written 00241 * @param uwDataSize: Size of data to write 00242 * @retval NOR memory status 00243 */ 00244 uint8_t BSP_NOR_WriteData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00245 { 00246 uint32_t index = uwDataSize; 00247 00248 while(index > 0) 00249 { 00250 /* Write data to NOR */ 00251 HAL_NOR_Program(&norHandle, (uint32_t *)(NOR_DEVICE_ADDR + uwStartAddress), pData); 00252 00253 /* Read NOR device status */ 00254 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00255 { 00256 return NOR_STATUS_ERROR; 00257 } 00258 00259 /* Update the counters */ 00260 index--; 00261 uwStartAddress += 2; 00262 pData++; 00263 } 00264 00265 return NOR_STATUS_OK; 00266 } 00267 00268 /** 00269 * @brief Programs an amount of data to the NOR device. 00270 * @param uwStartAddress: Write start address 00271 * @param pData: Pointer to data to be written 00272 * @param uwDataSize: Size of data to write 00273 * @retval NOR memory status 00274 */ 00275 uint8_t BSP_NOR_ProgramData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00276 { 00277 /* Send NOR program buffer operation */ 00278 HAL_NOR_ProgramBuffer(&norHandle, uwStartAddress, pData, uwDataSize); 00279 00280 /* Return the NOR memory status */ 00281 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00282 { 00283 return NOR_STATUS_ERROR; 00284 } 00285 else 00286 { 00287 return NOR_STATUS_OK; 00288 } 00289 } 00290 00291 /** 00292 * @brief Erases the specified block of the NOR device. 00293 * @param BlockAddress: Block address to erase 00294 * @retval NOR memory status 00295 */ 00296 uint8_t BSP_NOR_Erase_Block(uint32_t BlockAddress) 00297 { 00298 /* Send NOR erase block operation */ 00299 HAL_NOR_Erase_Block(&norHandle, BlockAddress, NOR_DEVICE_ADDR); 00300 00301 /* Return the NOR memory status */ 00302 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, BLOCKERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00303 { 00304 return NOR_STATUS_ERROR; 00305 } 00306 else 00307 { 00308 return NOR_STATUS_OK; 00309 } 00310 } 00311 00312 /** 00313 * @brief Erases the entire NOR chip. 00314 * @retval NOR memory status 00315 */ 00316 uint8_t BSP_NOR_Erase_Chip(void) 00317 { 00318 /* Send NOR Erase chip operation */ 00319 HAL_NOR_Erase_Chip(&norHandle, NOR_DEVICE_ADDR); 00320 00321 /* Return the NOR memory status */ 00322 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, CHIPERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00323 { 00324 return NOR_STATUS_ERROR; 00325 } 00326 else 00327 { 00328 return NOR_STATUS_OK; 00329 } 00330 } 00331 00332 /** 00333 * @brief Reads NOR flash IDs. 00334 * @param pNOR_ID : Pointer to NOR ID structure 00335 * @retval NOR memory status 00336 */ 00337 uint8_t BSP_NOR_Read_ID(NOR_IDTypeDef *pNOR_ID) 00338 { 00339 if(HAL_NOR_Read_ID(&norHandle, pNOR_ID) != HAL_OK) 00340 { 00341 return NOR_STATUS_ERROR; 00342 } 00343 else 00344 { 00345 return NOR_STATUS_OK; 00346 } 00347 } 00348 00349 /** 00350 * @brief Initializes the NOR MSP. 00351 * @param hnor: NOR handle 00352 * @param Params 00353 * @retval None 00354 */ 00355 __weak void BSP_NOR_MspInit(NOR_HandleTypeDef *hnor, void *Params) 00356 { 00357 GPIO_InitTypeDef gpio_init_structure; 00358 00359 /* Enable FMC clock */ 00360 __HAL_RCC_FMC_CLK_ENABLE(); 00361 00362 /* Enable GPIOs clock */ 00363 __HAL_RCC_GPIOD_CLK_ENABLE(); 00364 __HAL_RCC_GPIOE_CLK_ENABLE(); 00365 __HAL_RCC_GPIOF_CLK_ENABLE(); 00366 __HAL_RCC_GPIOG_CLK_ENABLE(); 00367 00368 /* Common GPIO configuration */ 00369 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00370 gpio_init_structure.Pull = GPIO_PULLUP; 00371 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00372 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00373 00374 /* GPIOD configuration */ 00375 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |\ 00376 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00377 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00378 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00379 00380 /* GPIOE configuration */ 00381 gpio_init_structure.Pin = GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |\ 00382 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00383 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00384 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00385 00386 /* GPIOF configuration */ 00387 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00388 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00389 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00390 00391 /* GPIOG configuration */ 00392 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00393 GPIO_PIN_5; 00394 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00395 } 00396 00397 /** 00398 * @brief DeInitializes NOR MSP. 00399 * @param hnor: NOR handle 00400 * @param Params 00401 * @retval None 00402 */ 00403 __weak void BSP_NOR_MspDeInit(NOR_HandleTypeDef *hnor, void *Params) 00404 { 00405 /* GPIO pins clock, FMC clock can be shut down in the application 00406 by surcharging this __weak function */ 00407 } 00408 00409 /** 00410 * @brief NOR BSP Wait for Ready/Busy signal. 00411 * @param hnor: Pointer to NOR handle 00412 * @param Timeout: Timeout duration 00413 * @retval None 00414 */ 00415 void HAL_NOR_MspWait(NOR_HandleTypeDef *hnor, uint32_t Timeout) 00416 { 00417 uint32_t timeout = Timeout; 00418 00419 /* Polling on Ready/Busy signal */ 00420 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_BUSY_STATE) && (timeout > 0)) 00421 { 00422 timeout--; 00423 } 00424 00425 timeout = Timeout; 00426 00427 /* Polling on Ready/Busy signal */ 00428 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_READY_STATE) && (timeout > 0)) 00429 { 00430 timeout--; 00431 } 00432 } 00433 00434 /** 00435 * @} 00436 */ 00437 00438 /** 00439 * @} 00440 */ 00441 00442 /** 00443 * @} 00444 */ 00445 00446 /** 00447 * @} 00448 */ 00449 00450 00451 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 19:47:41 for STM32756G_EVAL BSP User Manual by
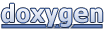