STM32756G_EVAL BSP User Manual
|
stm32756g_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32756G-EVAL and STM32746G-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive directly an LCD TFT using the LTDC controller. 00013 - This driver selects dynamically the mounted LCD, AMPIRE 640x480 LCD mounted 00014 on MB1063 or AMPIRE 480x272 LCD mounted on MB1046 daughter board, 00015 and uses the adequate timing and setting for the specified LCD using 00016 device ID of the STMPE811 mounted on MB1046 daughter board. 00017 00018 Driver description: 00019 ------------------ 00020 + Initialization steps: 00021 o Initialize the LCD using the BSP_LCD_Init() function. 00022 o Apply the Layer configuration using the BSP_LCD_LayerDefaultInit() function. 00023 o Select the LCD layer to be used using the BSP_LCD_SelectLayer() function. 00024 o Enable the LCD display using the BSP_LCD_DisplayOn() function. 00025 00026 + Options 00027 o Configure and enable the colour keying functionality using the 00028 BSP_LCD_SetColorKeying() function. 00029 o Modify in the fly the transparency and/or the frame buffer address 00030 using the following functions: 00031 - BSP_LCD_SetTransparency() 00032 - BSP_LCD_SetLayerAddress() 00033 00034 + Display on LCD 00035 o Clear the whole LCD using BSP_LCD_Clear() function or only one specified string 00036 line using the BSP_LCD_ClearStringLine() function. 00037 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00038 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00039 o Display a string line on the specified position (x,y in pixel) and align mode 00040 using the BSP_LCD_DisplayStringAtLine() function. 00041 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00042 on LCD using the available set of functions. 00043 @endverbatim 00044 ****************************************************************************** 00045 * @attention 00046 * 00047 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00048 * 00049 * Redistribution and use in source and binary forms, with or without modification, 00050 * are permitted provided that the following conditions are met: 00051 * 1. Redistributions of source code must retain the above copyright notice, 00052 * this list of conditions and the following disclaimer. 00053 * 2. Redistributions in binary form must reproduce the above copyright notice, 00054 * this list of conditions and the following disclaimer in the documentation 00055 * and/or other materials provided with the distribution. 00056 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00057 * may be used to endorse or promote products derived from this software 00058 * without specific prior written permission. 00059 * 00060 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00061 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00062 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00063 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00064 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00065 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00066 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00067 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00068 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00069 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00070 * 00071 ****************************************************************************** 00072 */ 00073 00074 /* Includes ------------------------------------------------------------------*/ 00075 #include "stm32756g_eval_lcd.h" 00076 #include "../../../Utilities/Fonts/fonts.h" 00077 #include "../../../Utilities/Fonts/font24.c" 00078 #include "../../../Utilities/Fonts/font20.c" 00079 #include "../../../Utilities/Fonts/font16.c" 00080 #include "../../../Utilities/Fonts/font12.c" 00081 #include "../../../Utilities/Fonts/font8.c" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32756G_EVAL 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM32756G_EVAL_LCD STM32756G_EVAL LCD 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM32756G_EVAL_LCD_Private_TypesDefinitions LCD Private TypesDefinitions 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32756G_EVAL_LCD_Private_Defines LCD Private Defines 00103 * @{ 00104 */ 00105 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00106 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32756G_EVAL_LCD_Private_Macros LCD Private Macros 00112 * @{ 00113 */ 00114 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00115 /** 00116 * @} 00117 */ 00118 00119 /** @defgroup STM32756G_EVAL_LCD_Private_Variables LCD Private Variables 00120 * @{ 00121 */ 00122 LTDC_HandleTypeDef hLtdcEval; 00123 static DMA2D_HandleTypeDef hDma2dEval; 00124 static uint32_t PCLK_profile = LCD_MAX_PCLK; 00125 /* Default LCD configuration with LCD Layer 1 */ 00126 static uint32_t ActiveLayer = 0; 00127 static LCD_DrawPropTypeDef DrawProp[MAX_LAYER_NUMBER]; 00128 /** 00129 * @} 00130 */ 00131 00132 /** @defgroup STM32756G_EVAL_LCD_Private_FunctionPrototypes LCD Private FunctionPrototypes 00133 * @{ 00134 */ 00135 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00136 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00137 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00138 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00139 /** 00140 * @} 00141 */ 00142 00143 /** @defgroup STM32756G_EVAL_LCD_Private_Functions LCD Private Functions 00144 * @{ 00145 */ 00146 00147 /** 00148 * @brief Initializes the LCD. 00149 * @retval LCD state 00150 */ 00151 uint8_t BSP_LCD_Init(void) 00152 { 00153 return (BSP_LCD_InitEx(LCD_MAX_PCLK)); 00154 } 00155 00156 /** 00157 * @brief Initializes the LCD. 00158 * @param PclkConfig : pixel clock profile 00159 * @retval LCD state 00160 */ 00161 uint8_t BSP_LCD_InitEx(uint32_t PclkConfig) 00162 { 00163 PCLK_profile = PclkConfig; 00164 /* Select the used LCD */ 00165 /* The AMPIRE 480x272 does not contain an ID register then we check the availability 00166 of AMPIRE 480x640 LCD using device ID of the STMPE811 mounted on MB1046 daughter board */ 00167 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00168 { 00169 /* The AMPIRE LCD 480x272 is selected */ 00170 /* Timing Configuration */ 00171 hLtdcEval.Init.HorizontalSync = (AMPIRE480272_HSYNC - 1); 00172 hLtdcEval.Init.VerticalSync = (AMPIRE480272_VSYNC - 1); 00173 hLtdcEval.Init.AccumulatedHBP = (AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00174 hLtdcEval.Init.AccumulatedVBP = (AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00175 hLtdcEval.Init.AccumulatedActiveH = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00176 hLtdcEval.Init.AccumulatedActiveW = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00177 hLtdcEval.Init.TotalHeigh = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP + AMPIRE480272_VFP - 1); 00178 hLtdcEval.Init.TotalWidth = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP + AMPIRE480272_HFP - 1); 00179 00180 /* Initialize the LCD pixel width and pixel height */ 00181 hLtdcEval.LayerCfg->ImageWidth = AMPIRE480272_WIDTH; 00182 hLtdcEval.LayerCfg->ImageHeight = AMPIRE480272_HEIGHT; 00183 } 00184 else 00185 { 00186 /* The LCD AMPIRE 640x480 is selected */ 00187 /* Timing configuration */ 00188 hLtdcEval.Init.HorizontalSync = (AMPIRE640480_HSYNC - 1); 00189 hLtdcEval.Init.VerticalSync = (AMPIRE640480_VSYNC - 1); 00190 hLtdcEval.Init.AccumulatedHBP = (AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00191 hLtdcEval.Init.AccumulatedVBP = (AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00192 hLtdcEval.Init.AccumulatedActiveH = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00193 hLtdcEval.Init.AccumulatedActiveW = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00194 hLtdcEval.Init.TotalHeigh = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP + AMPIRE640480_VFP - 1); 00195 hLtdcEval.Init.TotalWidth = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP + AMPIRE640480_HFP - 1); 00196 00197 /* Initialize the LCD pixel width and pixel height */ 00198 hLtdcEval.LayerCfg->ImageWidth = AMPIRE640480_WIDTH; 00199 hLtdcEval.LayerCfg->ImageHeight = AMPIRE640480_HEIGHT; 00200 } 00201 00202 /* Background value */ 00203 hLtdcEval.Init.Backcolor.Blue = 0; 00204 hLtdcEval.Init.Backcolor.Green = 0; 00205 hLtdcEval.Init.Backcolor.Red = 0; 00206 00207 /* Polarity */ 00208 hLtdcEval.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00209 hLtdcEval.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00210 hLtdcEval.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00211 hLtdcEval.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00212 hLtdcEval.Instance = LTDC; 00213 00214 /* LCD clock configuration */ 00215 BSP_LCD_ClockConfig(&hLtdcEval, &PCLK_profile); 00216 00217 if(HAL_LTDC_GetState(&hLtdcEval) == HAL_LTDC_STATE_RESET) 00218 { 00219 /* Initialize the LCD Msp: this __weak function can be rewritten by the application */ 00220 BSP_LCD_MspInit(&hLtdcEval, NULL); 00221 } 00222 HAL_LTDC_Init(&hLtdcEval); 00223 00224 #if !defined(DATA_IN_ExtSDRAM) 00225 /* When DATA_IN_ExtSDRAM define is enabled, the SDRAM will be configured in SystemInit() 00226 function (from system_stm32f7xx.c) before branch to main() routine. In such case, there 00227 is no need to reconfigure the SDRAM within the LCD driver, since it's already initialized. 00228 Otherwise the SDRAM must be configured. */ 00229 BSP_SDRAM_Init(); 00230 #endif 00231 00232 /* Initialize the font */ 00233 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00234 00235 return LCD_OK; 00236 } 00237 00238 /** 00239 * @brief DeInitializes the LCD. 00240 * @retval LCD state 00241 */ 00242 uint8_t BSP_LCD_DeInit(void) 00243 { 00244 /* Initialize the hltdc_eval Instance parameter */ 00245 hLtdcEval.Instance = LTDC; 00246 00247 /* Disable LTDC block */ 00248 __HAL_LTDC_DISABLE(&hLtdcEval); 00249 00250 /* DeInit the LTDC */ 00251 HAL_LTDC_DeInit(&hLtdcEval); 00252 00253 /* DeInit the LTDC MSP : this __weak function can be rewritten by the application */ 00254 BSP_LCD_MspDeInit(&hLtdcEval, NULL); 00255 00256 return LCD_OK; 00257 } 00258 00259 /** 00260 * @brief Gets the LCD X size. 00261 * @retval Used LCD X size 00262 */ 00263 uint32_t BSP_LCD_GetXSize(void) 00264 { 00265 return hLtdcEval.LayerCfg[ActiveLayer].ImageWidth; 00266 } 00267 00268 /** 00269 * @brief Gets the LCD Y size. 00270 * @retval Used LCD Y size 00271 */ 00272 uint32_t BSP_LCD_GetYSize(void) 00273 { 00274 return hLtdcEval.LayerCfg[ActiveLayer].ImageHeight; 00275 } 00276 00277 /** 00278 * @brief Set the LCD X size. 00279 * @param imageWidthPixels : image width in pixels unit 00280 * @retval None 00281 */ 00282 void BSP_LCD_SetXSize(uint32_t imageWidthPixels) 00283 { 00284 hLtdcEval.LayerCfg[ActiveLayer].ImageWidth = imageWidthPixels; 00285 } 00286 00287 /** 00288 * @brief Set the LCD Y size. 00289 * @param imageHeightPixels : image height in lines unit 00290 * @retval None 00291 */ 00292 void BSP_LCD_SetYSize(uint32_t imageHeightPixels) 00293 { 00294 hLtdcEval.LayerCfg[ActiveLayer].ImageHeight = imageHeightPixels; 00295 } 00296 00297 /** 00298 * @brief Initializes the LCD layers. 00299 * @param LayerIndex: Layer foreground or background 00300 * @param FB_Address: Layer frame buffer 00301 * @retval None 00302 */ 00303 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00304 { 00305 LCD_LayerCfgTypeDef layer_cfg; 00306 00307 /* Layer Init */ 00308 layer_cfg.WindowX0 = 0; 00309 layer_cfg.WindowX1 = BSP_LCD_GetXSize(); 00310 layer_cfg.WindowY0 = 0; 00311 layer_cfg.WindowY1 = BSP_LCD_GetYSize(); 00312 layer_cfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00313 layer_cfg.FBStartAdress = FB_Address; 00314 layer_cfg.Alpha = 255; 00315 layer_cfg.Alpha0 = 0; 00316 layer_cfg.Backcolor.Blue = 0; 00317 layer_cfg.Backcolor.Green = 0; 00318 layer_cfg.Backcolor.Red = 0; 00319 layer_cfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00320 layer_cfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00321 layer_cfg.ImageWidth = BSP_LCD_GetXSize(); 00322 layer_cfg.ImageHeight = BSP_LCD_GetYSize(); 00323 00324 HAL_LTDC_ConfigLayer(&hLtdcEval, &layer_cfg, LayerIndex); 00325 00326 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00327 DrawProp[LayerIndex].pFont = &Font24; 00328 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00329 } 00330 00331 /** 00332 * @brief Selects the LCD Layer. 00333 * @param LayerIndex: Layer foreground or background 00334 * @retval None 00335 */ 00336 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00337 { 00338 ActiveLayer = LayerIndex; 00339 } 00340 00341 /** 00342 * @brief Sets an LCD Layer visible 00343 * @param LayerIndex: Visible Layer 00344 * @param State: New state of the specified layer 00345 * This parameter can be one of the following values: 00346 * @arg ENABLE 00347 * @arg DISABLE 00348 * @retval None 00349 */ 00350 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00351 { 00352 if(State == ENABLE) 00353 { 00354 __HAL_LTDC_LAYER_ENABLE(&hLtdcEval, LayerIndex); 00355 } 00356 else 00357 { 00358 __HAL_LTDC_LAYER_DISABLE(&hLtdcEval, LayerIndex); 00359 } 00360 __HAL_LTDC_RELOAD_CONFIG(&hLtdcEval); 00361 } 00362 00363 /** 00364 * @brief Sets an LCD Layer visible without reloading. 00365 * @param LayerIndex: Visible Layer 00366 * @param State: New state of the specified layer 00367 * This parameter can be one of the following values: 00368 * @arg ENABLE 00369 * @arg DISABLE 00370 * @retval None 00371 */ 00372 void BSP_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State) 00373 { 00374 if(State == ENABLE) 00375 { 00376 __HAL_LTDC_LAYER_ENABLE(&hLtdcEval, LayerIndex); 00377 } 00378 else 00379 { 00380 __HAL_LTDC_LAYER_DISABLE(&hLtdcEval, LayerIndex); 00381 } 00382 /* Do not Sets the Reload */ 00383 } 00384 00385 /** 00386 * @brief Configures the transparency. 00387 * @param LayerIndex: Layer foreground or background. 00388 * @param Transparency: Transparency 00389 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00390 * @retval None 00391 */ 00392 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00393 { 00394 HAL_LTDC_SetAlpha(&hLtdcEval, Transparency, LayerIndex); 00395 } 00396 00397 /** 00398 * @brief Configures the transparency without reloading. 00399 * @param LayerIndex: Layer foreground or background. 00400 * @param Transparency: Transparency 00401 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00402 * @retval None 00403 */ 00404 void BSP_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency) 00405 { 00406 HAL_LTDC_SetAlpha_NoReload(&hLtdcEval, Transparency, LayerIndex); 00407 } 00408 00409 /** 00410 * @brief Sets an LCD layer frame buffer address. 00411 * @param LayerIndex: Layer foreground or background 00412 * @param Address: New LCD frame buffer value 00413 * @retval None 00414 */ 00415 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00416 { 00417 HAL_LTDC_SetAddress(&hLtdcEval, Address, LayerIndex); 00418 } 00419 00420 /** 00421 * @brief Sets an LCD layer frame buffer address without reloading. 00422 * @param LayerIndex: Layer foreground or background 00423 * @param Address: New LCD frame buffer value 00424 * @retval None 00425 */ 00426 void BSP_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address) 00427 { 00428 HAL_LTDC_SetAddress_NoReload(&hLtdcEval, Address, LayerIndex); 00429 } 00430 00431 /** 00432 * @brief Sets display window. 00433 * @param LayerIndex: Layer index 00434 * @param Xpos: LCD X position 00435 * @param Ypos: LCD Y position 00436 * @param Width: LCD window width 00437 * @param Height: LCD window height 00438 * @retval None 00439 */ 00440 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00441 { 00442 /* Reconfigure the layer size */ 00443 HAL_LTDC_SetWindowSize(&hLtdcEval, Width, Height, LayerIndex); 00444 00445 /* Reconfigure the layer position */ 00446 HAL_LTDC_SetWindowPosition(&hLtdcEval, Xpos, Ypos, LayerIndex); 00447 } 00448 00449 /** 00450 * @brief Sets display window without reloading. 00451 * @param LayerIndex: Layer index 00452 * @param Xpos: LCD X position 00453 * @param Ypos: LCD Y position 00454 * @param Width: LCD window width 00455 * @param Height: LCD window height 00456 * @retval None 00457 */ 00458 void BSP_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00459 { 00460 /* Reconfigure the layer size */ 00461 HAL_LTDC_SetWindowSize_NoReload(&hLtdcEval, Width, Height, LayerIndex); 00462 00463 /* Reconfigure the layer position */ 00464 HAL_LTDC_SetWindowPosition_NoReload(&hLtdcEval, Xpos, Ypos, LayerIndex); 00465 } 00466 00467 /** 00468 * @brief Configures and sets the color keying. 00469 * @param LayerIndex: Layer foreground or background 00470 * @param RGBValue: Color reference 00471 * @retval None 00472 */ 00473 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00474 { 00475 /* Configure and Enable the color Keying for LCD Layer */ 00476 HAL_LTDC_ConfigColorKeying(&hLtdcEval, RGBValue, LayerIndex); 00477 HAL_LTDC_EnableColorKeying(&hLtdcEval, LayerIndex); 00478 } 00479 00480 /** 00481 * @brief Configures and sets the color keying without reloading. 00482 * @param LayerIndex: Layer foreground or background 00483 * @param RGBValue: Color reference 00484 * @retval None 00485 */ 00486 void BSP_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue) 00487 { 00488 /* Configure and Enable the color Keying for LCD Layer */ 00489 HAL_LTDC_ConfigColorKeying_NoReload(&hLtdcEval, RGBValue, LayerIndex); 00490 HAL_LTDC_EnableColorKeying_NoReload(&hLtdcEval, LayerIndex); 00491 } 00492 00493 /** 00494 * @brief Disables the color keying. 00495 * @param LayerIndex: Layer foreground or background 00496 * @retval None 00497 */ 00498 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00499 { 00500 /* Disable the color Keying for LCD Layer */ 00501 HAL_LTDC_DisableColorKeying(&hLtdcEval, LayerIndex); 00502 } 00503 00504 /** 00505 * @brief Disables the color keying without reloading. 00506 * @param LayerIndex: Layer foreground or background 00507 * @retval None 00508 */ 00509 void BSP_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex) 00510 { 00511 /* Disable the color Keying for LCD Layer */ 00512 HAL_LTDC_DisableColorKeying_NoReload(&hLtdcEval, LayerIndex); 00513 } 00514 00515 /** 00516 * @brief Disables the color keying without reloading. 00517 * @param ReloadType: can be one of the following values 00518 * - LCD_RELOAD_IMMEDIATE 00519 * - LCD_RELOAD_VERTICAL_BLANKING 00520 * @retval None 00521 */ 00522 void BSP_LCD_Relaod(uint32_t ReloadType) 00523 { 00524 HAL_LTDC_Reload (&hLtdcEval, ReloadType); 00525 } 00526 00527 /** 00528 * @brief Sets the LCD text color. 00529 * @param Color: Text color code ARGB(8-8-8-8) 00530 * @retval None 00531 */ 00532 void BSP_LCD_SetTextColor(uint32_t Color) 00533 { 00534 DrawProp[ActiveLayer].TextColor = Color; 00535 } 00536 00537 /** 00538 * @brief Gets the LCD text color. 00539 * @retval Used text color. 00540 */ 00541 uint32_t BSP_LCD_GetTextColor(void) 00542 { 00543 return DrawProp[ActiveLayer].TextColor; 00544 } 00545 00546 /** 00547 * @brief Sets the LCD background color. 00548 * @param Color: Layer background color code ARGB(8-8-8-8) 00549 * @retval None 00550 */ 00551 void BSP_LCD_SetBackColor(uint32_t Color) 00552 { 00553 DrawProp[ActiveLayer].BackColor = Color; 00554 } 00555 00556 /** 00557 * @brief Gets the LCD background color. 00558 * @retval Used background color 00559 */ 00560 uint32_t BSP_LCD_GetBackColor(void) 00561 { 00562 return DrawProp[ActiveLayer].BackColor; 00563 } 00564 00565 /** 00566 * @brief Sets the LCD text font. 00567 * @param fonts: Layer font to be used 00568 * @retval None 00569 */ 00570 void BSP_LCD_SetFont(sFONT *fonts) 00571 { 00572 DrawProp[ActiveLayer].pFont = fonts; 00573 } 00574 00575 /** 00576 * @brief Gets the LCD text font. 00577 * @retval Used layer font 00578 */ 00579 sFONT *BSP_LCD_GetFont(void) 00580 { 00581 return DrawProp[ActiveLayer].pFont; 00582 } 00583 00584 /** 00585 * @brief Reads an LCD pixel. 00586 * @param Xpos: X position 00587 * @param Ypos: Y position 00588 * @retval RGB pixel color 00589 */ 00590 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00591 { 00592 uint32_t ret = 0; 00593 00594 if(hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00595 { 00596 /* Read data value from SDRAM memory */ 00597 ret = *(__IO uint32_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00598 } 00599 else if(hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00600 { 00601 /* Read data value from SDRAM memory */ 00602 ret = (*(__IO uint32_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00603 } 00604 else if((hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00605 (hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00606 (hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00607 { 00608 /* Read data value from SDRAM memory */ 00609 ret = *(__IO uint16_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00610 } 00611 else 00612 { 00613 /* Read data value from SDRAM memory */ 00614 ret = *(__IO uint8_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00615 } 00616 00617 return ret; 00618 } 00619 00620 /** 00621 * @brief Clears the hole LCD. 00622 * @param Color: Color of the background 00623 * @retval None 00624 */ 00625 void BSP_LCD_Clear(uint32_t Color) 00626 { 00627 /* Clear the LCD */ 00628 LL_FillBuffer(ActiveLayer, (uint32_t *)(hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00629 } 00630 00631 /** 00632 * @brief Clears the selected line. 00633 * @param Line: Line to be cleared 00634 * @retval None 00635 */ 00636 void BSP_LCD_ClearStringLine(uint32_t Line) 00637 { 00638 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00639 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00640 00641 /* Draw rectangle with background color */ 00642 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00643 00644 DrawProp[ActiveLayer].TextColor = color_backup; 00645 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00646 } 00647 00648 /** 00649 * @brief Displays one character. 00650 * @param Xpos: Start column address 00651 * @param Ypos: Line where to display the character shape. 00652 * @param Ascii: Character ascii code 00653 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00654 * @retval None 00655 */ 00656 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00657 { 00658 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00659 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00660 } 00661 00662 /** 00663 * @brief Displays characters on the LCD. 00664 * @param Xpos: X position (in pixel) 00665 * @param Ypos: Y position (in pixel) 00666 * @param Text: Pointer to string to display on LCD 00667 * @param Mode: Display mode 00668 * This parameter can be one of the following values: 00669 * @arg CENTER_MODE 00670 * @arg RIGHT_MODE 00671 * @arg LEFT_MODE 00672 * @retval None 00673 */ 00674 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00675 { 00676 uint16_t ref_column = 1, i = 0; 00677 uint32_t size = 0, xsize = 0; 00678 uint8_t *ptr = Text; 00679 00680 /* Get the text size */ 00681 while (*ptr++) size ++ ; 00682 00683 /* Characters number per line */ 00684 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00685 00686 switch (Mode) 00687 { 00688 case CENTER_MODE: 00689 { 00690 ref_column = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00691 break; 00692 } 00693 case LEFT_MODE: 00694 { 00695 ref_column = Xpos; 00696 break; 00697 } 00698 case RIGHT_MODE: 00699 { 00700 ref_column = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00701 break; 00702 } 00703 default: 00704 { 00705 ref_column = Xpos; 00706 break; 00707 } 00708 } 00709 00710 /* Check that the Start column is located in the screen */ 00711 if ((ref_column < 1) || (ref_column >= 0x8000)) 00712 { 00713 ref_column = 1; 00714 } 00715 00716 /* Send the string character by character on LCD */ 00717 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00718 { 00719 /* Display one character on LCD */ 00720 BSP_LCD_DisplayChar(ref_column, Ypos, *Text); 00721 /* Decrement the column position by 16 */ 00722 ref_column += DrawProp[ActiveLayer].pFont->Width; 00723 /* Point on the next character */ 00724 Text++; 00725 i++; 00726 } 00727 } 00728 00729 /** 00730 * @brief Displays a maximum of 60 characters on the LCD. 00731 * @param Line: Line where to display the character shape 00732 * @param ptr: Pointer to string to display on LCD 00733 * @retval None 00734 */ 00735 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00736 { 00737 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00738 } 00739 00740 /** 00741 * @brief Draws an horizontal line. 00742 * @param Xpos: X position 00743 * @param Ypos: Y position 00744 * @param Length: Line length 00745 * @retval None 00746 */ 00747 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00748 { 00749 uint32_t Xaddress = 0; 00750 00751 /* Get the line address */ 00752 Xaddress = (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00753 00754 /* Write line */ 00755 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00756 } 00757 00758 /** 00759 * @brief Draws a vertical line. 00760 * @param Xpos: X position 00761 * @param Ypos: Y position 00762 * @param Length: Line length 00763 * @retval None 00764 */ 00765 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00766 { 00767 uint32_t Xaddress = 0; 00768 00769 /* Get the line address */ 00770 Xaddress = (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00771 00772 /* Write line */ 00773 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00774 } 00775 00776 /** 00777 * @brief Draws an uni-line (between two points). 00778 * @param x1: Point 1 X position 00779 * @param y1: Point 1 Y position 00780 * @param x2: Point 2 X position 00781 * @param y2: Point 2 Y position 00782 * @retval None 00783 */ 00784 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00785 { 00786 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00787 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 00788 curpixel = 0; 00789 00790 deltax = ABS(x2 - x1); /* The difference between the x's */ 00791 deltay = ABS(y2 - y1); /* The difference between the y's */ 00792 x = x1; /* Start x off at the first pixel */ 00793 y = y1; /* Start y off at the first pixel */ 00794 00795 if (x2 >= x1) /* The x-values are increasing */ 00796 { 00797 xinc1 = 1; 00798 xinc2 = 1; 00799 } 00800 else /* The x-values are decreasing */ 00801 { 00802 xinc1 = -1; 00803 xinc2 = -1; 00804 } 00805 00806 if (y2 >= y1) /* The y-values are increasing */ 00807 { 00808 yinc1 = 1; 00809 yinc2 = 1; 00810 } 00811 else /* The y-values are decreasing */ 00812 { 00813 yinc1 = -1; 00814 yinc2 = -1; 00815 } 00816 00817 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00818 { 00819 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00820 yinc2 = 0; /* Don't change the y for every iteration */ 00821 den = deltax; 00822 num = deltax / 2; 00823 num_add = deltay; 00824 num_pixels = deltax; /* There are more x-values than y-values */ 00825 } 00826 else /* There is at least one y-value for every x-value */ 00827 { 00828 xinc2 = 0; /* Don't change the x for every iteration */ 00829 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00830 den = deltay; 00831 num = deltay / 2; 00832 num_add = deltax; 00833 num_pixels = deltay; /* There are more y-values than x-values */ 00834 } 00835 00836 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 00837 { 00838 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00839 num += num_add; /* Increase the numerator by the top of the fraction */ 00840 if (num >= den) /* Check if numerator >= denominator */ 00841 { 00842 num -= den; /* Calculate the new numerator value */ 00843 x += xinc1; /* Change the x as appropriate */ 00844 y += yinc1; /* Change the y as appropriate */ 00845 } 00846 x += xinc2; /* Change the x as appropriate */ 00847 y += yinc2; /* Change the y as appropriate */ 00848 } 00849 } 00850 00851 /** 00852 * @brief Draws a rectangle. 00853 * @param Xpos: X position 00854 * @param Ypos: Y position 00855 * @param Width: Rectangle width 00856 * @param Height: Rectangle height 00857 * @retval None 00858 */ 00859 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00860 { 00861 /* Draw horizontal lines */ 00862 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00863 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00864 00865 /* Draw vertical lines */ 00866 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00867 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00868 } 00869 00870 /** 00871 * @brief Draws a circle. 00872 * @param Xpos: X position 00873 * @param Ypos: Y position 00874 * @param Radius: Circle radius 00875 * @retval None 00876 */ 00877 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00878 { 00879 int32_t decision; /* Decision Variable */ 00880 uint32_t current_x; /* Current X Value */ 00881 uint32_t current_y; /* Current Y Value */ 00882 00883 decision = 3 - (Radius << 1); 00884 current_x = 0; 00885 current_y = Radius; 00886 00887 while (current_x <= current_y) 00888 { 00889 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00890 00891 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00892 00893 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00894 00895 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00896 00897 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00898 00899 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00900 00901 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00902 00903 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00904 00905 if (decision < 0) 00906 { 00907 decision += (current_x << 2) + 6; 00908 } 00909 else 00910 { 00911 decision += ((current_x - current_y) << 2) + 10; 00912 current_y--; 00913 } 00914 current_x++; 00915 } 00916 } 00917 00918 /** 00919 * @brief Draws an poly-line (between many points). 00920 * @param Points: Pointer to the points array 00921 * @param PointCount: Number of points 00922 * @retval None 00923 */ 00924 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00925 { 00926 int16_t x = 0, y = 0; 00927 00928 if(PointCount < 2) 00929 { 00930 return; 00931 } 00932 00933 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00934 00935 while(--PointCount) 00936 { 00937 x = Points->X; 00938 y = Points->Y; 00939 Points++; 00940 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00941 } 00942 } 00943 00944 /** 00945 * @brief Draws an ellipse on LCD. 00946 * @param Xpos: X position 00947 * @param Ypos: Y position 00948 * @param XRadius: Ellipse X radius 00949 * @param YRadius: Ellipse Y radius 00950 * @retval None 00951 */ 00952 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00953 { 00954 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00955 float k = 0, rad1 = 0, rad2 = 0; 00956 00957 rad1 = XRadius; 00958 rad2 = YRadius; 00959 00960 k = (float)(rad2/rad1); 00961 00962 do { 00963 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00964 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00965 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00966 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00967 00968 e2 = err; 00969 if (e2 <= x) { 00970 err += ++x*2+1; 00971 if (-y == x && e2 <= y) e2 = 0; 00972 } 00973 if (e2 > y) err += ++y*2+1; 00974 } 00975 while (y <= 0); 00976 } 00977 00978 /** 00979 * @brief Draws a pixel on LCD. 00980 * @param Xpos: X position 00981 * @param Ypos: Y position 00982 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 00983 * @retval None 00984 */ 00985 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 00986 { 00987 /* Write data value to all SDRAM memory */ 00988 *(__IO uint32_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 00989 } 00990 00991 /** 00992 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00993 * @param Xpos: Bmp X position in the LCD 00994 * @param Ypos: Bmp Y position in the LCD 00995 * @param pbmp: Pointer to Bmp picture address in the internal Flash 00996 * @retval None 00997 */ 00998 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 00999 { 01000 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 01001 uint32_t address; 01002 uint32_t input_color_mode = 0; 01003 01004 /* Get bitmap data address offset */ 01005 index = *(__IO uint16_t *) (pbmp + 10); 01006 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 01007 01008 /* Read bitmap width */ 01009 width = *(uint16_t *) (pbmp + 18); 01010 width |= (*(uint16_t *) (pbmp + 20)) << 16; 01011 01012 /* Read bitmap height */ 01013 height = *(uint16_t *) (pbmp + 22); 01014 height |= (*(uint16_t *) (pbmp + 24)) << 16; 01015 01016 /* Read bit/pixel */ 01017 bit_pixel = *(uint16_t *) (pbmp + 28); 01018 01019 /* Set the address */ 01020 address = hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Ypos) + Xpos)*(4)); 01021 01022 /* Get the layer pixel format */ 01023 if ((bit_pixel/8) == 4) 01024 { 01025 input_color_mode = DMA2D_INPUT_ARGB8888; 01026 } 01027 else if ((bit_pixel/8) == 2) 01028 { 01029 input_color_mode = DMA2D_INPUT_RGB565; 01030 } 01031 else 01032 { 01033 input_color_mode = DMA2D_INPUT_RGB888; 01034 } 01035 01036 /* Bypass the bitmap header */ 01037 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 01038 01039 /* Convert picture to ARGB8888 pixel format */ 01040 for(index=0; index < height; index++) 01041 { 01042 /* Pixel format conversion */ 01043 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)address, width, input_color_mode); 01044 01045 /* Increment the source and destination buffers */ 01046 address+= (BSP_LCD_GetXSize()*4); 01047 pbmp -= width*(bit_pixel/8); 01048 } 01049 } 01050 01051 /** 01052 * @brief Draws a full rectangle. 01053 * @param Xpos: X position 01054 * @param Ypos: Y position 01055 * @param Width: Rectangle width 01056 * @param Height: Rectangle height 01057 * @retval None 01058 */ 01059 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01060 { 01061 uint32_t x_address = 0; 01062 01063 /* Set the text color */ 01064 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01065 01066 /* Get the rectangle start address */ 01067 x_address = (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 01068 01069 /* Fill the rectangle */ 01070 LL_FillBuffer(ActiveLayer, (uint32_t *)x_address, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 01071 } 01072 01073 /** 01074 * @brief Draws a full circle. 01075 * @param Xpos: X position 01076 * @param Ypos: Y position 01077 * @param Radius: Circle radius 01078 * @retval None 01079 */ 01080 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 01081 { 01082 int32_t decision; /* Decision Variable */ 01083 uint32_t current_x; /* Current X Value */ 01084 uint32_t current_y; /* Current Y Value */ 01085 01086 decision = 3 - (Radius << 1); 01087 01088 current_x = 0; 01089 current_y = Radius; 01090 01091 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01092 01093 while (current_x <= current_y) 01094 { 01095 if(current_y > 0) 01096 { 01097 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 01098 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 01099 } 01100 01101 if(current_x > 0) 01102 { 01103 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 01104 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 01105 } 01106 if (decision < 0) 01107 { 01108 decision += (current_x << 2) + 6; 01109 } 01110 else 01111 { 01112 decision += ((current_x - current_y) << 2) + 10; 01113 current_y--; 01114 } 01115 current_x++; 01116 } 01117 01118 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01119 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 01120 } 01121 01122 /** 01123 * @brief Draws a full poly-line (between many points). 01124 * @param Points: Pointer to the points array 01125 * @param PointCount: Number of points 01126 * @retval None 01127 */ 01128 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01129 { 01130 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 01131 uint16_t image_left = 0, image_right = 0, image_top = 0, image_bottom = 0; 01132 01133 image_left = image_right = Points->X; 01134 image_top= image_bottom = Points->Y; 01135 01136 for(counter = 1; counter < PointCount; counter++) 01137 { 01138 pixelX = POLY_X(counter); 01139 if(pixelX < image_left) 01140 { 01141 image_left = pixelX; 01142 } 01143 if(pixelX > image_right) 01144 { 01145 image_right = pixelX; 01146 } 01147 01148 pixelY = POLY_Y(counter); 01149 if(pixelY < image_top) 01150 { 01151 image_top = pixelY; 01152 } 01153 if(pixelY > image_bottom) 01154 { 01155 image_bottom = pixelY; 01156 } 01157 } 01158 01159 if(PointCount < 2) 01160 { 01161 return; 01162 } 01163 01164 X_center = (image_left + image_right)/2; 01165 Y_center = (image_bottom + image_top)/2; 01166 01167 X_first = Points->X; 01168 Y_first = Points->Y; 01169 01170 while(--PointCount) 01171 { 01172 X = Points->X; 01173 Y = Points->Y; 01174 Points++; 01175 X2 = Points->X; 01176 Y2 = Points->Y; 01177 01178 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 01179 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 01180 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 01181 } 01182 01183 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 01184 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 01185 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 01186 } 01187 01188 /** 01189 * @brief Draws a full ellipse. 01190 * @param Xpos: X position 01191 * @param Ypos: Y position 01192 * @param XRadius: Ellipse X radius 01193 * @param YRadius: Ellipse Y radius 01194 * @retval None 01195 */ 01196 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01197 { 01198 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01199 float k = 0, rad1 = 0, rad2 = 0; 01200 01201 rad1 = XRadius; 01202 rad2 = YRadius; 01203 01204 k = (float)(rad2/rad1); 01205 01206 do 01207 { 01208 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 01209 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 01210 01211 e2 = err; 01212 if (e2 <= x) 01213 { 01214 err += ++x*2+1; 01215 if (-y == x && e2 <= y) e2 = 0; 01216 } 01217 if (e2 > y) err += ++y*2+1; 01218 } 01219 while (y <= 0); 01220 } 01221 01222 /** 01223 * @brief Enables the display. 01224 * @retval None 01225 */ 01226 void BSP_LCD_DisplayOn(void) 01227 { 01228 /* Display On */ 01229 __HAL_LTDC_ENABLE(&hLtdcEval); 01230 } 01231 01232 /** 01233 * @brief Disables the display. 01234 * @retval None 01235 */ 01236 void BSP_LCD_DisplayOff(void) 01237 { 01238 /* Display Off */ 01239 __HAL_LTDC_DISABLE(&hLtdcEval); 01240 } 01241 01242 /** 01243 * @brief Initializes the LTDC MSP. 01244 * @param hltdc: LTDC handle 01245 * @param Params 01246 * @retval None 01247 */ 01248 __weak void BSP_LCD_MspInit(LTDC_HandleTypeDef *hltdc, void *Params) 01249 { 01250 GPIO_InitTypeDef gpio_init_structure; 01251 01252 /* Enable the LTDC and DMA2D clocks */ 01253 __HAL_RCC_LTDC_CLK_ENABLE(); 01254 __HAL_RCC_DMA2D_CLK_ENABLE(); 01255 01256 /* Enable GPIOs clock */ 01257 __HAL_RCC_GPIOI_CLK_ENABLE(); 01258 __HAL_RCC_GPIOJ_CLK_ENABLE(); 01259 __HAL_RCC_GPIOK_CLK_ENABLE(); 01260 01261 /*** LTDC Pins configuration ***/ 01262 /* GPIOI configuration */ 01263 gpio_init_structure.Pin = GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01264 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01265 gpio_init_structure.Pull = GPIO_NOPULL; 01266 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01267 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01268 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 01269 01270 /* GPIOJ configuration */ 01271 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01272 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01273 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01274 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01275 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01276 gpio_init_structure.Pull = GPIO_NOPULL; 01277 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01278 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01279 HAL_GPIO_Init(GPIOJ, &gpio_init_structure); 01280 01281 /* GPIOK configuration */ 01282 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01283 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01284 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01285 gpio_init_structure.Pull = GPIO_NOPULL; 01286 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01287 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01288 HAL_GPIO_Init(GPIOK, &gpio_init_structure); 01289 } 01290 01291 /** 01292 * @brief DeInitializes BSP_LCD MSP. 01293 * @param hltdc: LTDC handle 01294 * @param Params 01295 * @retval None 01296 */ 01297 __weak void BSP_LCD_MspDeInit(LTDC_HandleTypeDef *hltdc, void *Params) 01298 { 01299 GPIO_InitTypeDef gpio_init_structure; 01300 01301 /* Disable LTDC block */ 01302 __HAL_LTDC_DISABLE(hltdc); 01303 01304 /* LTDC Pins deactivation */ 01305 /* GPIOI deactivation */ 01306 gpio_init_structure.Pin = GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01307 HAL_GPIO_DeInit(GPIOI, gpio_init_structure.Pin); 01308 /* GPIOJ deactivation */ 01309 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01310 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01311 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01312 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01313 HAL_GPIO_DeInit(GPIOJ, gpio_init_structure.Pin); 01314 /* GPIOK deactivation */ 01315 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01316 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01317 HAL_GPIO_DeInit(GPIOK, gpio_init_structure.Pin); 01318 01319 /* Disable LTDC clock */ 01320 __HAL_RCC_LTDC_CLK_DISABLE(); 01321 01322 /* GPIO pins clock can be shut down in the application 01323 by surcharging this __weak function */ 01324 } 01325 01326 /** 01327 * @brief Clock Config. 01328 * @param hltdc: LTDC handle 01329 * @param Params 01330 * @note This API is called by BSP_LCD_Init() 01331 * Being __weak it can be overwritten by the application 01332 * @retval None 01333 */ 01334 __weak void BSP_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params) 01335 { 01336 static RCC_PeriphCLKInitTypeDef periph_clk_init_struct; 01337 01338 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 01339 { 01340 /* AMPIRE480272 LCD clock configuration */ 01341 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01342 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 192 Mhz */ 01343 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 192/5 = 38.4 Mhz */ 01344 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_4 = 38.4/4 = 9.6Mhz */ 01345 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01346 periph_clk_init_struct.PLLSAI.PLLSAIN = 192; 01347 periph_clk_init_struct.PLLSAI.PLLSAIR = AMPIRE480272_FREQUENCY_DIVIDER; 01348 periph_clk_init_struct.PLLSAIDivR = RCC_PLLSAIDIVR_4; 01349 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01350 } 01351 else 01352 { 01353 /* The programmed LTDC pixel clock depends on the vertical refresh rate of the panel 60Hz => 25.16MHz and 01354 the LCD/SDRAM bandwidth affected by the several access on the bus and the number of used layers. 01355 */ 01356 if(*(uint32_t *)Params == LCD_MAX_PCLK) 01357 { 01358 /* In case of single layer the bandwidth is arround 160MBytesPerSec ==> theorical PCLK of 40MHz */ 01359 /* AMPIRE640480 typical PCLK is 25.16 MHz so the PLLSAI is configured to provide this clock */ 01360 /* AMPIRE640480 LCD clock configuration */ 01361 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01362 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 151 Mhz */ 01363 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 151/3 = 50.3 Mhz */ 01364 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_2 = 50.3/2 = 25.16 Mhz */ 01365 periph_clk_init_struct.PLLSAI.PLLSAIN = 151; 01366 } 01367 else 01368 { 01369 /* In case of double layers the bandwidth is arround 80MBytesPerSec => 20MHz (<25,16MHz) */ 01370 /* so the PLLSAI is configured to provide this clock */ 01371 /* AMPIRE640480 LCD clock configuration */ 01372 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01373 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 120 Mhz */ 01374 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 120/3 = 40 Mhz */ 01375 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_2 = 40/2 = 20 Mhz */ 01376 periph_clk_init_struct.PLLSAI.PLLSAIN = 120; 01377 } 01378 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01379 periph_clk_init_struct.PLLSAI.PLLSAIR = 3; 01380 periph_clk_init_struct.PLLSAIDivR = RCC_PLLSAIDIVR_2; 01381 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01382 } 01383 } 01384 01385 01386 /******************************************************************************* 01387 Static Functions 01388 *******************************************************************************/ 01389 01390 /** 01391 * @brief Draws a character on LCD. 01392 * @param Xpos: Line where to display the character shape 01393 * @param Ypos: Start column address 01394 * @param c: Pointer to the character data 01395 * @retval None 01396 */ 01397 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01398 { 01399 uint32_t i = 0, j = 0; 01400 uint16_t height, width; 01401 uint8_t offset; 01402 uint8_t *pchar; 01403 uint32_t line; 01404 01405 height = DrawProp[ActiveLayer].pFont->Height; 01406 width = DrawProp[ActiveLayer].pFont->Width; 01407 01408 offset = 8 *((width + 7)/8) - width ; 01409 01410 for(i = 0; i < height; i++) 01411 { 01412 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01413 01414 switch(((width + 7)/8)) 01415 { 01416 01417 case 1: 01418 line = pchar[0]; 01419 break; 01420 01421 case 2: 01422 line = (pchar[0]<< 8) | pchar[1]; 01423 break; 01424 01425 case 3: 01426 default: 01427 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01428 break; 01429 } 01430 01431 for (j = 0; j < width; j++) 01432 { 01433 if(line & (1 << (width- j + offset- 1))) 01434 { 01435 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01436 } 01437 else 01438 { 01439 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01440 } 01441 } 01442 Ypos++; 01443 } 01444 } 01445 01446 /** 01447 * @brief Fills a triangle (between 3 points). 01448 * @param x1: Point 1 X position 01449 * @param y1: Point 1 Y position 01450 * @param x2: Point 2 X position 01451 * @param y2: Point 2 Y position 01452 * @param x3: Point 3 X position 01453 * @param y3: Point 3 Y position 01454 * @retval None 01455 */ 01456 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01457 { 01458 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01459 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 01460 curpixel = 0; 01461 01462 deltax = ABS(x2 - x1); /* The difference between the x's */ 01463 deltay = ABS(y2 - y1); /* The difference between the y's */ 01464 x = x1; /* Start x off at the first pixel */ 01465 y = y1; /* Start y off at the first pixel */ 01466 01467 if (x2 >= x1) /* The x-values are increasing */ 01468 { 01469 xinc1 = 1; 01470 xinc2 = 1; 01471 } 01472 else /* The x-values are decreasing */ 01473 { 01474 xinc1 = -1; 01475 xinc2 = -1; 01476 } 01477 01478 if (y2 >= y1) /* The y-values are increasing */ 01479 { 01480 yinc1 = 1; 01481 yinc2 = 1; 01482 } 01483 else /* The y-values are decreasing */ 01484 { 01485 yinc1 = -1; 01486 yinc2 = -1; 01487 } 01488 01489 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01490 { 01491 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01492 yinc2 = 0; /* Don't change the y for every iteration */ 01493 den = deltax; 01494 num = deltax / 2; 01495 num_add = deltay; 01496 num_pixels = deltax; /* There are more x-values than y-values */ 01497 } 01498 else /* There is at least one y-value for every x-value */ 01499 { 01500 xinc2 = 0; /* Don't change the x for every iteration */ 01501 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01502 den = deltay; 01503 num = deltay / 2; 01504 num_add = deltax; 01505 num_pixels = deltay; /* There are more y-values than x-values */ 01506 } 01507 01508 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 01509 { 01510 BSP_LCD_DrawLine(x, y, x3, y3); 01511 01512 num += num_add; /* Increase the numerator by the top of the fraction */ 01513 if (num >= den) /* Check if numerator >= denominator */ 01514 { 01515 num -= den; /* Calculate the new numerator value */ 01516 x += xinc1; /* Change the x as appropriate */ 01517 y += yinc1; /* Change the y as appropriate */ 01518 } 01519 x += xinc2; /* Change the x as appropriate */ 01520 y += yinc2; /* Change the y as appropriate */ 01521 } 01522 } 01523 01524 /** 01525 * @brief Fills a buffer. 01526 * @param LayerIndex: Layer index 01527 * @param pDst: Pointer to destination buffer 01528 * @param xSize: Buffer width 01529 * @param ySize: Buffer height 01530 * @param OffLine: Offset 01531 * @param ColorIndex: Color index 01532 * @retval None 01533 */ 01534 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01535 { 01536 /* Register to memory mode with ARGB8888 as color Mode */ 01537 hDma2dEval.Init.Mode = DMA2D_R2M; 01538 hDma2dEval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01539 hDma2dEval.Init.OutputOffset = OffLine; 01540 01541 hDma2dEval.Instance = DMA2D; 01542 01543 /* DMA2D Initialization */ 01544 if(HAL_DMA2D_Init(&hDma2dEval) == HAL_OK) 01545 { 01546 if(HAL_DMA2D_ConfigLayer(&hDma2dEval, LayerIndex) == HAL_OK) 01547 { 01548 if (HAL_DMA2D_Start(&hDma2dEval, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01549 { 01550 /* Polling For DMA transfer */ 01551 HAL_DMA2D_PollForTransfer(&hDma2dEval, 10); 01552 } 01553 } 01554 } 01555 } 01556 01557 /** 01558 * @brief Converts a line to an ARGB8888 pixel format. 01559 * @param pSrc: Pointer to source buffer 01560 * @param pDst: Output color 01561 * @param xSize: Buffer width 01562 * @param ColorMode: Input color mode 01563 * @retval None 01564 */ 01565 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01566 { 01567 /* Configure the DMA2D Mode, Color Mode and output offset */ 01568 hDma2dEval.Init.Mode = DMA2D_M2M_PFC; 01569 hDma2dEval.Init.ColorMode = DMA2D_OUTPUT_ARGB8888; 01570 hDma2dEval.Init.OutputOffset = 0; 01571 01572 /* Foreground Configuration */ 01573 hDma2dEval.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01574 hDma2dEval.LayerCfg[1].InputAlpha = 0xFF; 01575 hDma2dEval.LayerCfg[1].InputColorMode = ColorMode; 01576 hDma2dEval.LayerCfg[1].InputOffset = 0; 01577 01578 hDma2dEval.Instance = DMA2D; 01579 01580 /* DMA2D Initialization */ 01581 if(HAL_DMA2D_Init(&hDma2dEval) == HAL_OK) 01582 { 01583 if(HAL_DMA2D_ConfigLayer(&hDma2dEval, 1) == HAL_OK) 01584 { 01585 if (HAL_DMA2D_Start(&hDma2dEval, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01586 { 01587 /* Polling For DMA transfer */ 01588 HAL_DMA2D_PollForTransfer(&hDma2dEval, 10); 01589 } 01590 } 01591 } 01592 } 01593 01594 /** 01595 * @} 01596 */ 01597 01598 /** 01599 * @} 01600 */ 01601 01602 /** 01603 * @} 01604 */ 01605 01606 /** 01607 * @} 01608 */ 01609 01610 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 19:47:41 for STM32756G_EVAL BSP User Manual by
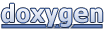