STM32756G_EVAL BSP User Manual
|
stm32756g_eval_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval_camera.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes the driver for Camera modules mounted on 00008 * STM32756G-EVAL and STM32746G-EVAL evaluation boards. 00009 @verbatim 00010 How to use this driver: 00011 ----------------------- 00012 - This driver is used to drive the camera. 00013 - The S5K5CAG component driver MUST be included with this driver. 00014 00015 Driver description: 00016 ------------------ 00017 + Initialization steps: 00018 o Initialize the camera using the BSP_CAMERA_Init() function. 00019 o Start the camera capture/snapshot using the CAMERA_Start() function. 00020 o Suspend, resume or stop the camera capture using the following functions: 00021 - BSP_CAMERA_Suspend() 00022 - BSP_CAMERA_Resume() 00023 - BSP_CAMERA_Stop() 00024 00025 + Options 00026 o Increase or decrease on the fly the brightness and/or contrast 00027 using the following function: 00028 - BSP_CAMERA_ContrastBrightnessConfig 00029 o Add a special effect on the fly using the following functions: 00030 - BSP_CAMERA_BlackWhiteConfig() 00031 - BSP_CAMERA_ColorEffectConfig() 00032 @endverbatim 00033 ****************************************************************************** 00034 * @attention 00035 * 00036 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00037 * 00038 * Redistribution and use in source and binary forms, with or without modification, 00039 * are permitted provided that the following conditions are met: 00040 * 1. Redistributions of source code must retain the above copyright notice, 00041 * this list of conditions and the following disclaimer. 00042 * 2. Redistributions in binary form must reproduce the above copyright notice, 00043 * this list of conditions and the following disclaimer in the documentation 00044 * and/or other materials provided with the distribution. 00045 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00046 * may be used to endorse or promote products derived from this software 00047 * without specific prior written permission. 00048 * 00049 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00050 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00051 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00052 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00053 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00054 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00055 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00056 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00057 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00058 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00059 * 00060 ****************************************************************************** 00061 */ 00062 00063 /* Includes ------------------------------------------------------------------*/ 00064 #include "stm32756g_eval_camera.h" 00065 00066 /** @addtogroup BSP 00067 * @{ 00068 */ 00069 00070 /** @addtogroup STM32756G_EVAL 00071 * @{ 00072 */ 00073 00074 /** @defgroup STM32756G_EVAL_CAMERA STM32756G_EVAL CAMERA 00075 * @{ 00076 */ 00077 00078 /** @defgroup STM32756G_EVAL_CAMERA_Private_TypesDefinitions CAMERA Private TypesDefinitions 00079 * @{ 00080 */ 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32756G_EVAL_CAMERA_Private_Defines CAMERA Private Defines 00086 * @{ 00087 */ 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM32756G_EVAL_CAMERA_Private_Macros CAMERA Private Macros 00093 * @{ 00094 */ 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM32756G_EVAL_CAMERA_Private_Variables CAMERA Private Variables 00100 * @{ 00101 */ 00102 DCMI_HandleTypeDef hDcmiEval; 00103 CAMERA_DrvTypeDef *camera_drv; 00104 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00105 static uint32_t CameraCurrentResolution; 00106 00107 /* Camera module I2C HW address */ 00108 static uint32_t CameraHwAddress; 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup STM32756G_EVAL_CAMERA_Private_FunctionsPrototypes CAMERA Private Functions Prototypes 00114 * @{ 00115 */ 00116 static uint32_t GetSize(uint32_t resolution); 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM32756G_EVAL_CAMERA_Private_Functions CAMERA Private Functions 00122 * @{ 00123 */ 00124 00125 /** 00126 * @brief Initializes the camera. 00127 * @param Resolution : camera sensor requested resolution (x, y) : standard resolution 00128 * naming QQVGA, QVGA, VGA ... 00129 * 00130 * @retval Camera status 00131 */ 00132 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00133 { 00134 DCMI_HandleTypeDef *phdcmi; 00135 uint8_t status = CAMERA_ERROR; 00136 00137 /* Get the DCMI handle structure */ 00138 phdcmi = &hDcmiEval; 00139 00140 /*** Configures the DCMI to interface with the camera module ***/ 00141 /* DCMI configuration */ 00142 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00143 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_HIGH; 00144 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00145 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00146 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00147 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00148 phdcmi->Instance = DCMI; 00149 00150 /* Configure IO functionalities for CAMERA detect pin */ 00151 BSP_IO_Init(); 00152 00153 /* Apply Camera Module hardware reset */ 00154 BSP_CAMERA_HwReset(); 00155 00156 /* Check if the CAMERA Module is plugged on board */ 00157 if(BSP_IO_ReadPin(CAM_PLUG_PIN) == BSP_IO_PIN_SET) 00158 { 00159 status = CAMERA_NOT_DETECTED; 00160 return status; /* Exit with error */ 00161 } 00162 00163 /* Read ID of Camera module via I2C */ 00164 if (s5k5cag_ReadID(CAMERA_I2C_ADDRESS) == S5K5CAG_ID) 00165 { 00166 /* Initialize the camera driver structure */ 00167 camera_drv = &s5k5cag_drv; 00168 CameraHwAddress = CAMERA_I2C_ADDRESS; 00169 00170 /* DCMI Initialization */ 00171 BSP_CAMERA_MspInit(&hDcmiEval, NULL); 00172 HAL_DCMI_Init(phdcmi); 00173 00174 /* Camera Module Initialization via I2C to the wanted 'Resolution' */ 00175 camera_drv->Init(CameraHwAddress, Resolution); 00176 00177 CameraCurrentResolution = Resolution; 00178 00179 /* Return CAMERA_OK status */ 00180 status = CAMERA_OK; 00181 } 00182 else 00183 { 00184 /* Return CAMERA_NOT_SUPPORTED status */ 00185 status = CAMERA_NOT_SUPPORTED; 00186 } 00187 00188 return status; 00189 } 00190 00191 /** 00192 * @brief DeInitializes the camera. 00193 * @retval Camera status 00194 */ 00195 uint8_t BSP_CAMERA_DeInit(void) 00196 { 00197 hDcmiEval.Instance = DCMI; 00198 00199 HAL_DCMI_DeInit(&hDcmiEval); 00200 BSP_CAMERA_MspDeInit(&hDcmiEval, NULL); 00201 return CAMERA_OK; 00202 } 00203 00204 /** 00205 * @brief Starts the camera capture in continuous mode. 00206 * @param buff: pointer to the camera output buffer 00207 * @retval None 00208 */ 00209 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00210 { 00211 /* Start the camera capture */ 00212 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00213 } 00214 00215 /** 00216 * @brief Starts the camera capture in snapshot mode. 00217 * @param buff: pointer to the camera output buffer 00218 * @retval None 00219 */ 00220 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00221 { 00222 /* Start the camera capture */ 00223 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00224 } 00225 00226 /** 00227 * @brief Suspend the CAMERA capture 00228 00229 * @retval None 00230 */ 00231 void BSP_CAMERA_Suspend(void) 00232 { 00233 /* Suspend the Camera Capture */ 00234 HAL_DCMI_Suspend(&hDcmiEval); 00235 } 00236 00237 /** 00238 * @brief Resume the CAMERA capture 00239 * @retval None 00240 */ 00241 void BSP_CAMERA_Resume(void) 00242 { 00243 /* Start the Camera Capture */ 00244 HAL_DCMI_Resume(&hDcmiEval); 00245 } 00246 00247 /** 00248 * @brief Stop the CAMERA capture 00249 * @retval Camera status 00250 */ 00251 uint8_t BSP_CAMERA_Stop(void) 00252 { 00253 uint8_t status = CAMERA_ERROR; 00254 00255 if(HAL_DCMI_Stop(&hDcmiEval) == HAL_OK) 00256 { 00257 status = CAMERA_OK; 00258 } 00259 00260 /* Set Camera in Power Down */ 00261 BSP_CAMERA_PwrDown(); 00262 00263 return status; 00264 } 00265 00266 /** 00267 * @brief CANERA hardware reset 00268 * @retval None 00269 */ 00270 void BSP_CAMERA_HwReset(void) 00271 { 00272 /* Camera sensor RESET sequence */ 00273 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00274 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00275 00276 /* Assert the camera STANDBY pin (active high) */ 00277 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_SET); 00278 00279 /* Assert the camera RSTI pin (active low) */ 00280 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00281 00282 HAL_Delay(100); /* RST and XSDN signals asserted during 100ms */ 00283 00284 /* De-assert the camera STANDBY pin (active high) */ 00285 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00286 00287 HAL_Delay(3); /* RST de-asserted and XSDN asserted during 3ms */ 00288 00289 /* De-assert the camera RSTI pin (active low) */ 00290 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_SET); 00291 00292 HAL_Delay(6); /* RST de-asserted during 3ms */ 00293 } 00294 00295 /** 00296 * @brief CAMERA power down 00297 * @retval None 00298 */ 00299 void BSP_CAMERA_PwrDown(void) 00300 { 00301 /* Camera power down sequence */ 00302 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00303 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00304 00305 /* De-assert the camera STANDBY pin (active high) */ 00306 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00307 00308 /* Assert the camera RSTI pin (active low) */ 00309 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00310 } 00311 00312 /** 00313 * @brief Configures the camera contrast and brightness. 00314 * @param contrast_level: Contrast level 00315 * This parameter can be one of the following values: 00316 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00317 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00318 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00319 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00320 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00321 * @param brightness_level: Contrast level 00322 * This parameter can be one of the following values: 00323 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00324 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00325 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00326 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00327 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00328 * @retval None 00329 */ 00330 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00331 { 00332 if(camera_drv->Config != NULL) 00333 { 00334 camera_drv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00335 } 00336 } 00337 00338 /** 00339 * @brief Configures the camera white balance. 00340 * @param Mode: black_white mode 00341 * This parameter can be one of the following values: 00342 * @arg CAMERA_BLACK_WHITE_BW 00343 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00344 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00345 * @arg CAMERA_BLACK_WHITE_NORMAL 00346 * @retval None 00347 */ 00348 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00349 { 00350 if(camera_drv->Config != NULL) 00351 { 00352 camera_drv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00353 } 00354 } 00355 00356 /** 00357 * @brief Configures the camera color effect. 00358 * @param Effect: Color effect 00359 * This parameter can be one of the following values: 00360 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00361 * @arg CAMERA_COLOR_EFFECT_BLUE 00362 * @arg CAMERA_COLOR_EFFECT_GREEN 00363 * @arg CAMERA_COLOR_EFFECT_RED 00364 * @retval None 00365 */ 00366 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00367 { 00368 if(camera_drv->Config != NULL) 00369 { 00370 camera_drv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00371 } 00372 } 00373 00374 /** 00375 * @brief Get the capture size in pixels unit. 00376 * @param resolution: the current resolution. 00377 * @retval capture size in pixels unit. 00378 */ 00379 static uint32_t GetSize(uint32_t resolution) 00380 { 00381 uint32_t size = 0; 00382 00383 /* Get capture size */ 00384 switch (resolution) 00385 { 00386 case CAMERA_R160x120: 00387 { 00388 size = 0x2580; 00389 } 00390 break; 00391 case CAMERA_R320x240: 00392 { 00393 size = 0x9600; 00394 } 00395 break; 00396 case CAMERA_R480x272: 00397 { 00398 size = 0xFF00; 00399 } 00400 break; 00401 case CAMERA_R640x480: 00402 { 00403 size = 0x25800; 00404 } 00405 break; 00406 default: 00407 { 00408 break; 00409 } 00410 } 00411 00412 return size; 00413 } 00414 00415 /** 00416 * @brief Initializes the DCMI MSP. 00417 * @param hdcmi: pointer to the DCMI handle 00418 * @param Params 00419 * @retval None 00420 */ 00421 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00422 { 00423 static DMA_HandleTypeDef hdma_eval; 00424 GPIO_InitTypeDef gpio_init_structure; 00425 00426 /*** Enable peripherals and GPIO clocks ***/ 00427 /* Enable DCMI clock */ 00428 __HAL_RCC_DCMI_CLK_ENABLE(); 00429 00430 /* Enable DMA2 clock */ 00431 __HAL_RCC_DMA2_CLK_ENABLE(); 00432 00433 /* Enable GPIO clocks */ 00434 __HAL_RCC_GPIOA_CLK_ENABLE(); 00435 __HAL_RCC_GPIOB_CLK_ENABLE(); 00436 __HAL_RCC_GPIOC_CLK_ENABLE(); 00437 __HAL_RCC_GPIOD_CLK_ENABLE(); 00438 __HAL_RCC_GPIOE_CLK_ENABLE(); 00439 00440 /*** Configure the GPIO ***/ 00441 /* Configure DCMI GPIO as alternate function */ 00442 /* On STM32756G-EVAL RevB, to use camera, ensure that JP23 is in position 1-2, 00443 * LED3 is then no more usable */ 00444 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_6; 00445 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00446 gpio_init_structure.Pull = GPIO_PULLUP; 00447 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00448 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00449 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00450 00451 gpio_init_structure.Pin = GPIO_PIN_7; 00452 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00453 gpio_init_structure.Pull = GPIO_PULLUP; 00454 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00455 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00456 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00457 00458 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00459 GPIO_PIN_9 | GPIO_PIN_11; 00460 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00461 gpio_init_structure.Pull = GPIO_PULLUP; 00462 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00463 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00464 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00465 00466 gpio_init_structure.Pin = GPIO_PIN_3 | GPIO_PIN_6; 00467 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00468 gpio_init_structure.Pull = GPIO_PULLUP; 00469 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00470 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00471 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00472 00473 gpio_init_structure.Pin = GPIO_PIN_5 | GPIO_PIN_6; 00474 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00475 gpio_init_structure.Pull = GPIO_PULLUP; 00476 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00477 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00478 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00479 00480 /*** Configure the DMA ***/ 00481 /* Set the parameters to be configured */ 00482 hdma_eval.Init.Channel = DMA_CHANNEL_1; 00483 hdma_eval.Init.Direction = DMA_PERIPH_TO_MEMORY; 00484 hdma_eval.Init.PeriphInc = DMA_PINC_DISABLE; 00485 hdma_eval.Init.MemInc = DMA_MINC_ENABLE; 00486 hdma_eval.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00487 hdma_eval.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00488 hdma_eval.Init.Mode = DMA_CIRCULAR; 00489 hdma_eval.Init.Priority = DMA_PRIORITY_HIGH; 00490 hdma_eval.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00491 hdma_eval.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00492 hdma_eval.Init.MemBurst = DMA_MBURST_SINGLE; 00493 hdma_eval.Init.PeriphBurst = DMA_PBURST_SINGLE; 00494 00495 hdma_eval.Instance = DMA2_Stream1; 00496 00497 /* Associate the initialized DMA handle to the DCMI handle */ 00498 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_eval); 00499 00500 /*** Configure the NVIC for DCMI and DMA ***/ 00501 /* NVIC configuration for DCMI transfer complete interrupt */ 00502 HAL_NVIC_SetPriority(DCMI_IRQn, 0x0F, 0); 00503 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00504 00505 /* NVIC configuration for DMA2D transfer complete interrupt */ 00506 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 0x0F, 0); 00507 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00508 00509 /* Configure the DMA stream */ 00510 HAL_DMA_Init(hdcmi->DMA_Handle); 00511 } 00512 00513 00514 /** 00515 * @brief DeInitializes the DCMI MSP. 00516 * @param hdcmi: pointer to the DCMI handle 00517 * @param Params 00518 * @retval None 00519 */ 00520 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00521 { 00522 /* Disable NVIC for DCMI transfer complete interrupt */ 00523 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00524 00525 /* Disable NVIC for DMA2 transfer complete interrupt */ 00526 HAL_NVIC_DisableIRQ(DMA2_Stream1_IRQn); 00527 00528 /* Configure the DMA stream */ 00529 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00530 00531 /* Disable DCMI clock */ 00532 __HAL_RCC_DCMI_CLK_DISABLE(); 00533 00534 /* GPIO pins clock and DMA clock can be shut down in the application 00535 by surcharging this __weak function */ 00536 } 00537 00538 /** 00539 * @brief Line event callback 00540 * @param hdcmi: pointer to the DCMI handle 00541 * @retval None 00542 */ 00543 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00544 { 00545 BSP_CAMERA_LineEventCallback(); 00546 } 00547 00548 /** 00549 * @brief Line Event callback. 00550 * @retval None 00551 */ 00552 __weak void BSP_CAMERA_LineEventCallback(void) 00553 { 00554 /* NOTE : This function Should not be modified, when the callback is needed, 00555 the HAL_DCMI_LineEventCallback could be implemented in the user file 00556 */ 00557 } 00558 00559 /** 00560 * @brief VSYNC event callback 00561 * @param hdcmi: pointer to the DCMI handle 00562 * @retval None 00563 */ 00564 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00565 { 00566 BSP_CAMERA_VsyncEventCallback(); 00567 } 00568 00569 /** 00570 * @brief VSYNC Event callback. 00571 * @retval None 00572 */ 00573 __weak void BSP_CAMERA_VsyncEventCallback(void) 00574 { 00575 /* NOTE : This function Should not be modified, when the callback is needed, 00576 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00577 */ 00578 } 00579 00580 /** 00581 * @brief Frame event callback 00582 * @param hdcmi: pointer to the DCMI handle 00583 * @retval None 00584 */ 00585 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00586 { 00587 BSP_CAMERA_FrameEventCallback(); 00588 } 00589 00590 /** 00591 * @brief Frame Event callback. 00592 * @retval None 00593 */ 00594 __weak void BSP_CAMERA_FrameEventCallback(void) 00595 { 00596 /* NOTE : This function Should not be modified, when the callback is needed, 00597 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00598 */ 00599 } 00600 00601 /** 00602 * @brief Error callback 00603 * @param hdcmi: pointer to the DCMI handle 00604 * @retval None 00605 */ 00606 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00607 { 00608 BSP_CAMERA_ErrorCallback(); 00609 } 00610 00611 /** 00612 * @brief Error callback. 00613 * @retval None 00614 */ 00615 __weak void BSP_CAMERA_ErrorCallback(void) 00616 { 00617 /* NOTE : This function Should not be modified, when the callback is needed, 00618 the HAL_DCMI_ErrorCallback could be implemented in the user file 00619 */ 00620 } 00621 00622 /** 00623 * @} 00624 */ 00625 00626 /** 00627 * @} 00628 */ 00629 00630 /** 00631 * @} 00632 */ 00633 00634 /** 00635 * @} 00636 */ 00637 00638 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 19:47:41 for STM32756G_EVAL BSP User Manual by
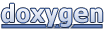