STM32756G_EVAL BSP User Manual
|
stm32756g_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32756G-EVAL and STM32746G-EVAL 00009 * evaluation board(MB1167) from STMicroelectronics. 00010 * 00011 @verbatim 00012 This driver requires the stm32756g_eval_io.c/.h files to manage the 00013 IO module resources mapped on the MFX IO expander. 00014 These resources are mainly LEDs, Joystick push buttons, SD detect pin, 00015 USB OTG power switch/over current drive pins, Camera plug pin, Audio 00016 INT pin 00017 The use of the above eval resources is conditioned by the "USE_IOEXPANDER" 00018 preprocessor define which is enabled by default for the STM327x6G-EVAL 00019 boards Rev A. However for Rev B boards these resources are disabled by default 00020 (except LED1 and LED2) and to be able to use them, user must add "USE_IOEXPANDER" 00021 define in the compiler preprocessor configuration (or any header file that 00022 is processed before stm32756g_eval.h). 00023 On the STM327x6G-EVAL RevB LED1 and LED2 are directly mapped on GPIO pins, 00024 to avoid the unnecessary overhead of code brought by the use of MFX IO 00025 expander when no further evaluation board resources are needed by the 00026 application/example. 00027 For precise details on the use of the MFX IO expander, you can refer to 00028 the description provided within the stm32756g_eval_io.c file header 00029 @endverbatim 00030 ****************************************************************************** 00031 * @attention 00032 * 00033 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00034 * 00035 * Redistribution and use in source and binary forms, with or without modification, 00036 * are permitted provided that the following conditions are met: 00037 * 1. Redistributions of source code must retain the above copyright notice, 00038 * this list of conditions and the following disclaimer. 00039 * 2. Redistributions in binary form must reproduce the above copyright notice, 00040 * this list of conditions and the following disclaimer in the documentation 00041 * and/or other materials provided with the distribution. 00042 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00043 * may be used to endorse or promote products derived from this software 00044 * without specific prior written permission. 00045 * 00046 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00047 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00048 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00049 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00050 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00051 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00052 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00053 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00054 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00055 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00056 * 00057 ****************************************************************************** 00058 */ 00059 00060 /* Includes ------------------------------------------------------------------*/ 00061 #include "stm32756g_eval.h" 00062 #if defined(USE_IOEXPANDER) 00063 #include "stm32756g_eval_io.h" 00064 #endif /* USE_IOEXPANDER */ 00065 00066 /** @addtogroup BSP 00067 * @{ 00068 */ 00069 00070 /** @defgroup STM32756G_EVAL EVAL 00071 * @{ 00072 */ 00073 00074 /** @defgroup STM32756G_EVAL_LOW_LEVEL STM32756G-EVAL LOW LEVEL 00075 * @{ 00076 */ 00077 00078 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_TypesDefinitions STM32756G-EVAL LOW LEVEL Private Types Definitions 00079 * @{ 00080 */ 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Defines STM32756G-EVAL LOW LEVEL Private Defines 00086 * @{ 00087 */ 00088 /** 00089 * @brief STM32756G EVAL BSP Driver version number V2.0.0 00090 */ 00091 #define __STM32756G_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00092 #define __STM32756G_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00093 #define __STM32756G_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00094 #define __STM32756G_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00095 #define __STM32756G_EVAL_BSP_VERSION ((__STM32756G_EVAL_BSP_VERSION_MAIN << 24)\ 00096 |(__STM32756G_EVAL_BSP_VERSION_SUB1 << 16)\ 00097 |(__STM32756G_EVAL_BSP_VERSION_SUB2 << 8 )\ 00098 |(__STM32756G_EVAL_BSP_VERSION_RC)) 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Macros STM32756G-EVAL LOW LEVEL Private Macros 00104 * @{ 00105 */ 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Variables STM32756G-EVAL LOW LEVEL Private Variables 00111 * @{ 00112 */ 00113 00114 #if defined(USE_IOEXPANDER) 00115 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00116 LED2_PIN, 00117 LED3_PIN, 00118 LED4_PIN}; 00119 #else 00120 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00121 LED3_PIN}; 00122 #endif /* USE_IOEXPANDER */ 00123 00124 00125 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00126 TAMPER_BUTTON_GPIO_PORT, 00127 KEY_BUTTON_GPIO_PORT}; 00128 00129 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00130 TAMPER_BUTTON_PIN, 00131 KEY_BUTTON_PIN}; 00132 00133 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00134 TAMPER_BUTTON_EXTI_IRQn, 00135 KEY_BUTTON_EXTI_IRQn}; 00136 00137 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00138 00139 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00140 00141 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00142 00143 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00144 00145 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00146 00147 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00148 00149 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00150 00151 static I2C_HandleTypeDef hEvalI2c; 00152 static ADC_HandleTypeDef hEvalADC; 00153 /** 00154 * @} 00155 */ 00156 00157 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM32756G_EVAL LOW LEVEL Private Function Prototypes 00158 * @{ 00159 */ 00160 static void I2Cx_MspInit(void); 00161 static void I2Cx_Init(void); 00162 #if defined(USE_IOEXPANDER) 00163 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00164 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00165 #endif /* USE_IOEXPANDER */ 00166 00167 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00168 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00169 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00170 static void I2Cx_Error(uint8_t Addr); 00171 00172 #if defined(USE_IOEXPANDER) 00173 /* IOExpander IO functions */ 00174 void IOE_Init(void); 00175 void IOE_ITConfig(void); 00176 void IOE_Delay(uint32_t Delay); 00177 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00178 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00179 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00180 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00181 00182 /* MFX IO functions */ 00183 void MFX_IO_Init(void); 00184 void MFX_IO_DeInit(void); 00185 void MFX_IO_ITConfig(void); 00186 void MFX_IO_Delay(uint32_t Delay); 00187 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00188 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00189 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00190 void MFX_IO_Wakeup(void); 00191 void MFX_IO_EnableWakeupPin(void); 00192 #endif /* USE_IOEXPANDER */ 00193 00194 /* AUDIO IO functions */ 00195 void AUDIO_IO_Init(void); 00196 void AUDIO_IO_DeInit(void); 00197 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00198 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00199 void AUDIO_IO_Delay(uint32_t Delay); 00200 00201 /* CAMERA IO functions */ 00202 void CAMERA_IO_Init(void); 00203 void CAMERA_Delay(uint32_t Delay); 00204 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00205 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg); 00206 00207 /* I2C EEPROM IO function */ 00208 void EEPROM_IO_Init(void); 00209 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00210 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00211 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00212 /** 00213 * @} 00214 */ 00215 00216 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Functions STM32756G_EVAL LOW LEVEL Private Functions 00217 * @{ 00218 */ 00219 00220 /** 00221 * @brief This method returns the STM32756G EVAL BSP Driver revision 00222 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00223 */ 00224 uint32_t BSP_GetVersion(void) 00225 { 00226 return __STM32756G_EVAL_BSP_VERSION; 00227 } 00228 00229 /** 00230 * @brief Configures LED on GPIO and/or on MFX. 00231 * @param Led: LED to be configured. 00232 * This parameter can be one of the following values: 00233 * @arg LED1 00234 * @arg LED2 00235 * @arg LED3 00236 * @arg LED4 00237 * @retval None 00238 */ 00239 void BSP_LED_Init(Led_TypeDef Led) 00240 { 00241 #if !defined(USE_STM32756G_EVAL_REVA) 00242 /* On RevB and above evaluation boards, LED1 and LED3 are connected to GPIOs */ 00243 /* To use LED1 on RevB board, ensure that JP24 is in position 2-3, potentiometer is then no more usable */ 00244 /* To use LED3 on RevB board, ensure that JP23 is in position 2-3, camera is then no more usable */ 00245 GPIO_InitTypeDef gpio_init_structure; 00246 GPIO_TypeDef* gpio_led; 00247 00248 if ((Led == LED1) || (Led == LED3)) 00249 { 00250 if (Led == LED1) 00251 { 00252 gpio_led = LED1_GPIO_PORT; 00253 /* Enable the GPIO_LED clock */ 00254 LED1_GPIO_CLK_ENABLE(); 00255 } 00256 else 00257 { 00258 gpio_led = LED3_GPIO_PORT; 00259 /* Enable the GPIO_LED clock */ 00260 LED3_GPIO_CLK_ENABLE(); 00261 } 00262 00263 /* Configure the GPIO_LED pin */ 00264 gpio_init_structure.Pin = GPIO_PIN[Led]; 00265 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00266 gpio_init_structure.Pull = GPIO_PULLUP; 00267 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00268 00269 HAL_GPIO_Init(gpio_led, &gpio_init_structure); 00270 00271 /* By default, turn off LED */ 00272 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00273 } 00274 else 00275 { 00276 #endif /* !USE_STM32756G_EVAL_REVA */ 00277 00278 #if defined(USE_IOEXPANDER) 00279 /* On RevA eval board, all LEDs are connected to MFX */ 00280 /* On RevB and above eval board, LED2 and LED4 are connected to MFX */ 00281 BSP_IO_Init(); /* Initialize MFX */ 00282 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OUTPUT_PP_PU); 00283 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00284 #endif /* USE_IOEXPANDER */ 00285 00286 #if !defined(USE_STM32756G_EVAL_REVA) 00287 } 00288 #endif /* !USE_STM32756G_EVAL_REVA */ 00289 } 00290 00291 00292 /** 00293 * @brief DeInit LEDs. 00294 * @param Led: LED to be configured. 00295 * This parameter can be one of the following values: 00296 * @arg LED1 00297 * @arg LED2 00298 * @arg LED3 00299 * @arg LED4 00300 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00301 * @retval None 00302 */ 00303 void BSP_LED_DeInit(Led_TypeDef Led) 00304 { 00305 #if !defined(USE_STM32756G_EVAL_REVA) 00306 GPIO_InitTypeDef gpio_init_structure; 00307 GPIO_TypeDef* gpio_led; 00308 00309 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00310 if ((Led == LED1) || (Led == LED3)) 00311 { 00312 if (Led == LED1) 00313 { 00314 gpio_led = LED1_GPIO_PORT; 00315 } 00316 else 00317 { 00318 gpio_led = LED3_GPIO_PORT; 00319 } 00320 /* Turn off LED */ 00321 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00322 /* Configure the GPIO_LED pin */ 00323 gpio_init_structure.Pin = GPIO_PIN[Led]; 00324 HAL_GPIO_DeInit(gpio_led, gpio_init_structure.Pin); 00325 } 00326 else 00327 { 00328 #endif /* !USE_STM32756G_EVAL_REVA */ 00329 00330 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00331 /* GPIO_PIN[Led] depends on the board revision: */ 00332 /* - in case of RevA all leds are deinit */ 00333 /* - in case of RevB just led 2 and led4 are deinit */ 00334 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OFF); 00335 #endif /* USE_IOEXPANDER */ 00336 00337 #if !defined(USE_STM32756G_EVAL_REVA) 00338 } 00339 #endif /* !USE_STM32756G_EVAL_REVA */ 00340 } 00341 00342 /** 00343 * @brief Turns selected LED On. 00344 * @param Led: LED to be set on 00345 * This parameter can be one of the following values: 00346 * @arg LED1 00347 * @arg LED2 00348 * @arg LED3 00349 * @arg LED4 00350 * @retval None 00351 */ 00352 void BSP_LED_On(Led_TypeDef Led) 00353 { 00354 #if !defined(USE_STM32756G_EVAL_REVA) 00355 GPIO_TypeDef* gpio_led; 00356 00357 if ((Led == LED1) || (Led == LED3)) /* Switch On LED connected to GPIO */ 00358 { 00359 if (Led == LED1) 00360 { 00361 gpio_led = LED1_GPIO_PORT; 00362 } 00363 else 00364 { 00365 gpio_led = LED3_GPIO_PORT; 00366 } 00367 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00368 } 00369 else 00370 { 00371 #endif /* !USE_STM32756G_EVAL_REVA */ 00372 00373 #if defined(USE_IOEXPANDER) /* Switch On LED connected to MFX */ 00374 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_RESET); 00375 #endif /* USE_IOEXPANDER */ 00376 00377 #if !defined(USE_STM32756G_EVAL_REVA) 00378 } 00379 #endif /* !USE_STM32756G_EVAL_REVA */ 00380 } 00381 00382 /** 00383 * @brief Turns selected LED Off. 00384 * @param Led: LED to be set off 00385 * This parameter can be one of the following values: 00386 * @arg LED1 00387 * @arg LED2 00388 * @arg LED3 00389 * @arg LED4 00390 * @retval None 00391 */ 00392 void BSP_LED_Off(Led_TypeDef Led) 00393 { 00394 #if !defined(USE_STM32756G_EVAL_REVA) 00395 GPIO_TypeDef* gpio_led; 00396 00397 if ((Led == LED1) || (Led == LED3)) /* Switch Off LED connected to GPIO */ 00398 { 00399 if (Led == LED1) 00400 { 00401 gpio_led = LED1_GPIO_PORT; 00402 } 00403 else 00404 { 00405 gpio_led = LED3_GPIO_PORT; 00406 } 00407 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00408 } 00409 else 00410 { 00411 #endif /* !USE_STM32756G_EVAL_REVA */ 00412 00413 #if defined(USE_IOEXPANDER) /* Switch Off LED connected to MFX */ 00414 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00415 #endif /* USE_IOEXPANDER */ 00416 00417 #if !defined(USE_STM32756G_EVAL_REVA) 00418 } 00419 #endif /* !USE_STM32756G_EVAL_REVA */ 00420 00421 } 00422 00423 /** 00424 * @brief Toggles the selected LED. 00425 * @param Led: LED to be toggled 00426 * This parameter can be one of the following values: 00427 * @arg LED1 00428 * @arg LED2 00429 * @arg LED3 00430 * @arg LED4 00431 * @retval None 00432 */ 00433 void BSP_LED_Toggle(Led_TypeDef Led) 00434 { 00435 #if !defined(USE_STM32756G_EVAL_REVA) 00436 GPIO_TypeDef* gpio_led; 00437 00438 if ((Led == LED1) || (Led == LED3)) /* Toggle LED connected to GPIO */ 00439 { 00440 if (Led == LED1) 00441 { 00442 gpio_led = LED1_GPIO_PORT; 00443 } 00444 else 00445 { 00446 gpio_led = LED3_GPIO_PORT; 00447 } 00448 HAL_GPIO_TogglePin(gpio_led, GPIO_PIN[Led]); 00449 } 00450 else 00451 { 00452 #endif /* !USE_STM32756G_EVAL_REVA */ 00453 00454 #if defined(USE_IOEXPANDER) /* Toggle LED connected to MFX */ 00455 BSP_IO_TogglePin(GPIO_PIN[Led]); 00456 #endif /* USE_IOEXPANDER */ 00457 00458 #if !defined(USE_STM32756G_EVAL_REVA) 00459 } 00460 #endif /* !USE_STM32756G_EVAL_REVA */ 00461 } 00462 00463 /** 00464 * @brief Configures button GPIO and EXTI Line. 00465 * @param Button: Button to be configured 00466 * This parameter can be one of the following values: 00467 * @arg BUTTON_WAKEUP: Wakeup Push Button 00468 * @arg BUTTON_TAMPER: Tamper Push Button 00469 * @arg BUTTON_KEY: Key Push Button 00470 * @param ButtonMode: Button mode 00471 * This parameter can be one of the following values: 00472 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00473 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00474 * with interrupt generation capability 00475 * @note On STM32756G-EVAL evaluation board, the three buttons (Wakeup, Tamper 00476 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00477 * on the board serigraphy. 00478 * @retval None 00479 */ 00480 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00481 { 00482 GPIO_InitTypeDef gpio_init_structure; 00483 00484 /* Enable the BUTTON clock */ 00485 BUTTONx_GPIO_CLK_ENABLE(Button); 00486 00487 if(ButtonMode == BUTTON_MODE_GPIO) 00488 { 00489 /* Configure Button pin as input */ 00490 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00491 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00492 gpio_init_structure.Pull = GPIO_NOPULL; 00493 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00494 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00495 } 00496 00497 if(ButtonMode == BUTTON_MODE_EXTI) 00498 { 00499 /* Configure Button pin as input with External interrupt */ 00500 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00501 gpio_init_structure.Pull = GPIO_NOPULL; 00502 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00503 00504 if(Button != BUTTON_WAKEUP) 00505 { 00506 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00507 } 00508 else 00509 { 00510 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00511 } 00512 00513 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00514 00515 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00516 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00517 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00518 } 00519 } 00520 00521 /** 00522 * @brief Push Button DeInit. 00523 * @param Button: Button to be configured 00524 * This parameter can be one of the following values: 00525 * @arg BUTTON_WAKEUP: Wakeup Push Button 00526 * @arg BUTTON_TAMPER: Tamper Push Button 00527 * @arg BUTTON_KEY: Key Push Button 00528 * @note On STM32756G-EVAL evaluation board, the three buttons (Wakeup, Tamper 00529 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00530 * on the board serigraphy. 00531 * @note PB DeInit does not disable the GPIO clock 00532 * @retval None 00533 */ 00534 void BSP_PB_DeInit(Button_TypeDef Button) 00535 { 00536 GPIO_InitTypeDef gpio_init_structure; 00537 00538 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00539 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00540 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00541 } 00542 00543 00544 /** 00545 * @brief Returns the selected button state. 00546 * @param Button: Button to be checked 00547 * This parameter can be one of the following values: 00548 * @arg BUTTON_WAKEUP: Wakeup Push Button 00549 * @arg BUTTON_TAMPER: Tamper Push Button 00550 * @arg BUTTON_KEY: Key Push Button 00551 * @note On STM32756G-EVAL evaluation board, the three buttons (Wakeup, Tamper 00552 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00553 * on the board serigraphy. 00554 * @retval The Button GPIO pin value 00555 */ 00556 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00557 { 00558 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00559 } 00560 00561 /** 00562 * @brief Configures COM port. 00563 * @param COM: COM port to be configured. 00564 * This parameter can be one of the following values: 00565 * @arg COM1 00566 * @arg COM2 00567 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00568 * configuration information for the specified USART peripheral. 00569 * @retval None 00570 */ 00571 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00572 { 00573 GPIO_InitTypeDef gpio_init_structure; 00574 00575 /* Enable GPIO clock */ 00576 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00577 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00578 00579 /* Enable USART clock */ 00580 EVAL_COMx_CLK_ENABLE(COM); 00581 00582 /* Configure USART Tx as alternate function */ 00583 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00584 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00585 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00586 gpio_init_structure.Pull = GPIO_PULLUP; 00587 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00588 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00589 00590 /* Configure USART Rx as alternate function */ 00591 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00592 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00593 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00594 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00595 00596 /* USART configuration */ 00597 huart->Instance = COM_USART[COM]; 00598 HAL_UART_Init(huart); 00599 } 00600 00601 /** 00602 * @brief DeInit COM port. 00603 * @param COM: COM port to be configured. 00604 * This parameter can be one of the following values: 00605 * @arg COM1 00606 * @arg COM2 00607 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00608 * configuration information for the specified USART peripheral. 00609 * @retval None 00610 */ 00611 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00612 { 00613 /* USART configuration */ 00614 huart->Instance = COM_USART[COM]; 00615 HAL_UART_DeInit(huart); 00616 00617 /* Enable USART clock */ 00618 EVAL_COMx_CLK_DISABLE(COM); 00619 00620 /* DeInit GPIO pins can be done in the application 00621 (by surcharging this __weak function) */ 00622 00623 /* GPIO pins clock, DMA clock can be shut down in the application 00624 by surcharging this __weak function */ 00625 } 00626 00627 /** 00628 * @brief Init Potentiometer. 00629 * @retval None 00630 */ 00631 void BSP_POTENTIOMETER_Init(void) 00632 { 00633 GPIO_InitTypeDef GPIO_InitStruct; 00634 ADC_ChannelConfTypeDef ADC_Config; 00635 00636 /* ADC an GPIO Periph clock enable */ 00637 ADCx_CLK_ENABLE(); 00638 ADCx_CHANNEL_GPIO_CLK_ENABLE(); 00639 00640 /* ADC Channel GPIO pin configuration */ 00641 GPIO_InitStruct.Pin = ADCx_CHANNEL_PIN; 00642 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00643 GPIO_InitStruct.Pull = GPIO_NOPULL; 00644 HAL_GPIO_Init(ADCx_CHANNEL_GPIO_PORT, &GPIO_InitStruct); 00645 00646 /* Configure the ADC peripheral */ 00647 hEvalADC.Instance = ADCx; 00648 00649 HAL_ADC_DeInit(&hEvalADC); 00650 00651 hEvalADC.Init.ClockPrescaler = ADC_CLOCKPRESCALER_PCLK_DIV4; /* Asynchronous clock mode, input ADC clock not divided */ 00652 hEvalADC.Init.Resolution = ADC_RESOLUTION_12B; /* 12-bit resolution for converted data */ 00653 hEvalADC.Init.DataAlign = ADC_DATAALIGN_RIGHT; /* Right-alignment for converted data */ 00654 hEvalADC.Init.ScanConvMode = DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 00655 hEvalADC.Init.EOCSelection = DISABLE; /* EOC flag picked-up to indicate conversion end */ 00656 hEvalADC.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00657 hEvalADC.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 00658 hEvalADC.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00659 hEvalADC.Init.NbrOfDiscConversion = 0; /* Parameter discarded because sequencer is disabled */ 00660 hEvalADC.Init.ExternalTrigConv = ADC_EXTERNALTRIGCONV_T1_CC1; /* Software start to trig the 1st conversion manually, without external event */ 00661 hEvalADC.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because software trigger chosen */ 00662 hEvalADC.Init.DMAContinuousRequests = DISABLE; /* DMA one-shot mode selected */ 00663 00664 00665 HAL_ADC_Init(&hEvalADC); 00666 00667 /* Configure ADC regular channel */ 00668 ADC_Config.Channel = ADCx_CHANNEL; /* Sampled channel number */ 00669 ADC_Config.Rank = 1; /* Rank of sampled channel number ADCx_CHANNEL */ 00670 ADC_Config.SamplingTime = ADC_SAMPLETIME_3CYCLES; /* Sampling time (number of clock cycles unit) */ 00671 ADC_Config.Offset = 0; /* Parameter discarded because offset correction is disabled */ 00672 00673 HAL_ADC_ConfigChannel(&hEvalADC, &ADC_Config); 00674 } 00675 00676 /** 00677 * @brief Get Potentiometer level in 12 bits. 00678 * @retval Potentiometer level(0..0xFFF) / 0xFFFFFFFF : Error 00679 */ 00680 uint32_t BSP_POTENTIOMETER_GetLevel(void) 00681 { 00682 if(HAL_ADC_Start(&hEvalADC) == HAL_OK) 00683 { 00684 if(HAL_ADC_PollForConversion(&hEvalADC, ADCx_POLL_TIMEOUT)== HAL_OK) 00685 { 00686 return (HAL_ADC_GetValue(&hEvalADC)); 00687 } 00688 } 00689 return 0xFFFFFFFF; 00690 } 00691 00692 #if defined(USE_IOEXPANDER) 00693 /** 00694 * @brief Configures joystick GPIO and EXTI modes. 00695 * @param JoyMode: Button mode. 00696 * This parameter can be one of the following values: 00697 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00698 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00699 * with interrupt generation capability 00700 * @retval IO_OK: if all initializations are OK. Other value if error. 00701 */ 00702 uint8_t BSP_JOY_Init(JOYMode_TypeDef JoyMode) 00703 { 00704 uint8_t ret = 0; 00705 00706 /* Initialize the IO functionalities */ 00707 ret = BSP_IO_Init(); 00708 00709 /* Configure joystick pins in IT mode */ 00710 if(JoyMode == JOY_MODE_EXTI) 00711 { 00712 /* Configure IO interrupt acquisition mode */ 00713 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00714 } 00715 else 00716 { 00717 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00718 } 00719 00720 return ret; 00721 } 00722 00723 00724 /** 00725 * @brief DeInit joystick GPIOs. 00726 * @note JOY DeInit does not disable the MFX, just set the MFX pins in Off mode 00727 * @retval None. 00728 */ 00729 void BSP_JOY_DeInit() 00730 { 00731 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00732 } 00733 00734 /** 00735 * @brief Returns the current joystick status. 00736 * @retval Code of the joystick key pressed 00737 * This code can be one of the following values: 00738 * @arg JOY_NONE 00739 * @arg JOY_SEL 00740 * @arg JOY_DOWN 00741 * @arg JOY_LEFT 00742 * @arg JOY_RIGHT 00743 * @arg JOY_UP 00744 */ 00745 JOYState_TypeDef BSP_JOY_GetState(void) 00746 { 00747 uint16_t pin_status = 0; 00748 00749 /* Read the status joystick pins */ 00750 pin_status = BSP_IO_ReadPin(JOY_ALL_PINS); 00751 00752 /* Check the pressed keys */ 00753 if((pin_status & JOY_NONE_PIN) == JOY_NONE) 00754 { 00755 return(JOYState_TypeDef) JOY_NONE; 00756 } 00757 else if(!(pin_status & JOY_SEL_PIN)) 00758 { 00759 return(JOYState_TypeDef) JOY_SEL; 00760 } 00761 else if(!(pin_status & JOY_DOWN_PIN)) 00762 { 00763 return(JOYState_TypeDef) JOY_DOWN; 00764 } 00765 else if(!(pin_status & JOY_LEFT_PIN)) 00766 { 00767 return(JOYState_TypeDef) JOY_LEFT; 00768 } 00769 else if(!(pin_status & JOY_RIGHT_PIN)) 00770 { 00771 return(JOYState_TypeDef) JOY_RIGHT; 00772 } 00773 else if(!(pin_status & JOY_UP_PIN)) 00774 { 00775 return(JOYState_TypeDef) JOY_UP; 00776 } 00777 else 00778 { 00779 return(JOYState_TypeDef) JOY_NONE; 00780 } 00781 } 00782 00783 /** 00784 * @brief Check TS3510 touch screen presence 00785 * @retval Return 0 if TS3510 is detected, return 1 if not detected 00786 */ 00787 uint8_t BSP_TS3510_IsDetected(void) 00788 { 00789 HAL_StatusTypeDef status = HAL_OK; 00790 uint32_t error = 0; 00791 uint8_t a_buffer; 00792 00793 uint8_t tmp_buffer[2] = {0x81, 0x08}; 00794 00795 /* Prepare for LCD read data */ 00796 IOE_WriteMultiple(TS3510_I2C_ADDRESS, 0x8A, tmp_buffer, 2); 00797 00798 status = HAL_I2C_Mem_Read(&hEvalI2c, TS3510_I2C_ADDRESS, 0x8A, I2C_MEMADD_SIZE_8BIT, &a_buffer, 1, 1000); 00799 00800 /* Check the communication status */ 00801 if(status != HAL_OK) 00802 { 00803 error = (uint32_t)HAL_I2C_GetError(&hEvalI2c); 00804 00805 /* I2C error occurred */ 00806 I2Cx_Error(TS3510_I2C_ADDRESS); 00807 00808 if(error == HAL_I2C_ERROR_AF) 00809 { 00810 return 1; 00811 } 00812 } 00813 return 0; 00814 } 00815 #endif /* USE_IOEXPANDER */ 00816 00817 /******************************************************************************* 00818 BUS OPERATIONS 00819 *******************************************************************************/ 00820 00821 /******************************* I2C Routines *********************************/ 00822 /** 00823 * @brief Initializes I2C MSP. 00824 * @retval None 00825 */ 00826 static void I2Cx_MspInit(void) 00827 { 00828 GPIO_InitTypeDef gpio_init_structure; 00829 00830 /*** Configure the GPIOs ***/ 00831 /* Enable GPIO clock */ 00832 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00833 00834 /* Configure I2C Tx as alternate function */ 00835 gpio_init_structure.Pin = EVAL_I2Cx_SCL_PIN; 00836 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00837 gpio_init_structure.Pull = GPIO_NOPULL; 00838 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00839 gpio_init_structure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00840 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00841 00842 /* Configure I2C Rx as alternate function */ 00843 gpio_init_structure.Pin = EVAL_I2Cx_SDA_PIN; 00844 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00845 00846 /*** Configure the I2C peripheral ***/ 00847 /* Enable I2C clock */ 00848 EVAL_I2Cx_CLK_ENABLE(); 00849 00850 /* Force the I2C peripheral clock reset */ 00851 EVAL_I2Cx_FORCE_RESET(); 00852 00853 /* Release the I2C peripheral clock reset */ 00854 EVAL_I2Cx_RELEASE_RESET(); 00855 00856 /* Enable and set I2Cx Interrupt to a lower priority */ 00857 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x0F, 0); 00858 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00859 00860 /* Enable and set I2Cx Interrupt to a lower priority */ 00861 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x0F, 0); 00862 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00863 } 00864 00865 /** 00866 * @brief Initializes I2C HAL. 00867 * @retval None 00868 */ 00869 static void I2Cx_Init(void) 00870 { 00871 if(HAL_I2C_GetState(&hEvalI2c) == HAL_I2C_STATE_RESET) 00872 { 00873 hEvalI2c.Instance = EVAL_I2Cx; 00874 hEvalI2c.Init.Timing = EVAL_I2Cx_TIMING; 00875 hEvalI2c.Init.OwnAddress1 = 0; 00876 hEvalI2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00877 hEvalI2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00878 hEvalI2c.Init.OwnAddress2 = 0; 00879 hEvalI2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00880 hEvalI2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00881 00882 /* Init the I2C */ 00883 I2Cx_MspInit(); 00884 HAL_I2C_Init(&hEvalI2c); 00885 } 00886 } 00887 00888 00889 #if defined(USE_IOEXPANDER) 00890 /** 00891 * @brief Writes a single data. 00892 * @param Addr: I2C address 00893 * @param Reg: Register address 00894 * @param Value: Data to be written 00895 * @retval None 00896 */ 00897 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00898 { 00899 HAL_StatusTypeDef status = HAL_OK; 00900 00901 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00902 00903 /* Check the communication status */ 00904 if(status != HAL_OK) 00905 { 00906 /* Execute user timeout callback */ 00907 I2Cx_Error(Addr); 00908 } 00909 } 00910 00911 /** 00912 * @brief Reads a single data. 00913 * @param Addr: I2C address 00914 * @param Reg: Register address 00915 * @retval Read data 00916 */ 00917 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00918 { 00919 HAL_StatusTypeDef status = HAL_OK; 00920 uint8_t Value = 0; 00921 00922 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00923 00924 /* Check the communication status */ 00925 if(status != HAL_OK) 00926 { 00927 /* Execute user timeout callback */ 00928 I2Cx_Error(Addr); 00929 } 00930 return Value; 00931 } 00932 #endif /* USE_IOEXPANDER */ 00933 00934 /** 00935 * @brief Reads multiple data. 00936 * @param Addr: I2C address 00937 * @param Reg: Reg address 00938 * @param MemAddress: Internal memory address 00939 * @param Buffer: Pointer to data buffer 00940 * @param Length: Length of the data 00941 * @retval Number of read data 00942 */ 00943 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00944 { 00945 HAL_StatusTypeDef status = HAL_OK; 00946 00947 if(Addr == EXC7200_I2C_ADDRESS) 00948 { 00949 status = HAL_I2C_Master_Receive(&hEvalI2c, Addr, Buffer, Length, 1000); 00950 } 00951 else 00952 { 00953 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00954 } 00955 00956 /* Check the communication status */ 00957 if(status != HAL_OK) 00958 { 00959 /* I2C error occurred */ 00960 I2Cx_Error(Addr); 00961 } 00962 return status; 00963 } 00964 00965 /** 00966 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00967 * @param Addr: Device address on BUS Bus. 00968 * @param Reg: The target register address to write 00969 * @param MemAddress: Internal memory address 00970 * @param Buffer: The target register value to be written 00971 * @param Length: buffer size to be written 00972 * @retval HAL status 00973 */ 00974 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00975 { 00976 HAL_StatusTypeDef status = HAL_OK; 00977 00978 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00979 00980 /* Check the communication status */ 00981 if(status != HAL_OK) 00982 { 00983 /* Re-Initiaize the I2C Bus */ 00984 I2Cx_Error(Addr); 00985 } 00986 return status; 00987 } 00988 00989 /** 00990 * @brief Checks if target device is ready for communication. 00991 * @note This function is used with Memory devices 00992 * @param DevAddress: Target device address 00993 * @param Trials: Number of trials 00994 * @retval HAL status 00995 */ 00996 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00997 { 00998 return (HAL_I2C_IsDeviceReady(&hEvalI2c, DevAddress, Trials, 1000)); 00999 } 01000 01001 /** 01002 * @brief Manages error callback by re-initializing I2C. 01003 * @param Addr: I2C Address 01004 * @retval None 01005 */ 01006 static void I2Cx_Error(uint8_t Addr) 01007 { 01008 /* De-initialize the I2C communication bus */ 01009 HAL_I2C_DeInit(&hEvalI2c); 01010 01011 /* Re-Initialize the I2C communication bus */ 01012 I2Cx_Init(); 01013 } 01014 01015 /******************************************************************************* 01016 LINK OPERATIONS 01017 *******************************************************************************/ 01018 01019 /********************************* LINK IOE ***********************************/ 01020 #if defined(USE_IOEXPANDER) 01021 /** 01022 * @brief Initializes IOE low level. 01023 * @retval None 01024 */ 01025 void IOE_Init(void) 01026 { 01027 I2Cx_Init(); 01028 } 01029 01030 /** 01031 * @brief Configures IOE low level interrupt. 01032 * @retval None 01033 */ 01034 void IOE_ITConfig(void) 01035 { 01036 /* STMPE811 IO expander IT config done by BSP_TS_ITConfig function */ 01037 } 01038 01039 /** 01040 * @brief IOE writes single data. 01041 * @param Addr: I2C address 01042 * @param Reg: Register address 01043 * @param Value: Data to be written 01044 * @retval None 01045 */ 01046 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01047 { 01048 I2Cx_Write(Addr, Reg, Value); 01049 } 01050 01051 /** 01052 * @brief IOE reads single data. 01053 * @param Addr: I2C address 01054 * @param Reg: Register address 01055 * @retval Read data 01056 */ 01057 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 01058 { 01059 return I2Cx_Read(Addr, Reg); 01060 } 01061 01062 /** 01063 * @brief IOE reads multiple data. 01064 * @param Addr: I2C address 01065 * @param Reg: Register address 01066 * @param Buffer: Pointer to data buffer 01067 * @param Length: Length of the data 01068 * @retval Number of read data 01069 */ 01070 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01071 { 01072 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01073 } 01074 01075 /** 01076 * @brief IOE writes multiple data. 01077 * @param Addr: I2C address 01078 * @param Reg: Register address 01079 * @param Buffer: Pointer to data buffer 01080 * @param Length: Length of the data 01081 * @retval None 01082 */ 01083 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01084 { 01085 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01086 } 01087 01088 /** 01089 * @brief IOE delay 01090 * @param Delay: Delay in ms 01091 * @retval None 01092 */ 01093 void IOE_Delay(uint32_t Delay) 01094 { 01095 HAL_Delay(Delay); 01096 } 01097 #endif /* USE_IOEXPANDER */ 01098 01099 /********************************* LINK MFX ***********************************/ 01100 01101 #if defined(USE_IOEXPANDER) 01102 /** 01103 * @brief Initializes MFX low level. 01104 * @retval None 01105 */ 01106 void MFX_IO_Init(void) 01107 { 01108 I2Cx_Init(); 01109 } 01110 01111 /** 01112 * @brief DeInitializes MFX low level. 01113 * @retval None 01114 */ 01115 void MFX_IO_DeInit(void) 01116 { 01117 } 01118 01119 /** 01120 * @brief Configures MFX low level interrupt. 01121 * @retval None 01122 */ 01123 void MFX_IO_ITConfig(void) 01124 { 01125 static uint8_t mfx_io_it_enabled = 0; 01126 GPIO_InitTypeDef gpio_init_structure; 01127 01128 if(mfx_io_it_enabled == 0) 01129 { 01130 mfx_io_it_enabled = 1; 01131 /* Enable the GPIO EXTI clock */ 01132 __HAL_RCC_GPIOI_CLK_ENABLE(); 01133 __HAL_RCC_SYSCFG_CLK_ENABLE(); 01134 01135 gpio_init_structure.Pin = GPIO_PIN_8; 01136 gpio_init_structure.Pull = GPIO_NOPULL; 01137 gpio_init_structure.Speed = GPIO_SPEED_LOW; 01138 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 01139 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 01140 01141 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 01142 HAL_NVIC_SetPriority((IRQn_Type)(EXTI9_5_IRQn), 0x0F, 0x0F); 01143 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI9_5_IRQn)); 01144 } 01145 } 01146 01147 /** 01148 * @brief MFX writes single data. 01149 * @param Addr: I2C address 01150 * @param Reg: Register address 01151 * @param Value: Data to be written 01152 * @retval None 01153 */ 01154 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01155 { 01156 I2Cx_Write((uint8_t) Addr, Reg, Value); 01157 } 01158 01159 /** 01160 * @brief MFX reads single data. 01161 * @param Addr: I2C address 01162 * @param Reg: Register address 01163 * @retval Read data 01164 */ 01165 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01166 { 01167 return I2Cx_Read((uint8_t) Addr, Reg); 01168 } 01169 01170 /** 01171 * @brief MFX reads multiple data. 01172 * @param Addr: I2C address 01173 * @param Reg: Register address 01174 * @param Buffer: Pointer to data buffer 01175 * @param Length: Length of the data 01176 * @retval Number of read data 01177 */ 01178 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01179 { 01180 return I2Cx_ReadMultiple((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01181 } 01182 01183 /** 01184 * @brief MFX delay 01185 * @param Delay: Delay in ms 01186 * @retval None 01187 */ 01188 void MFX_IO_Delay(uint32_t Delay) 01189 { 01190 HAL_Delay(Delay); 01191 } 01192 01193 /** 01194 * @brief Used by Lx family but requested for MFX component compatibility. 01195 * @retval None 01196 */ 01197 void MFX_IO_Wakeup(void) 01198 { 01199 } 01200 01201 /** 01202 * @brief Used by Lx family but requested for MXF component compatibility. 01203 * @retval None 01204 */ 01205 void MFX_IO_EnableWakeupPin(void) 01206 { 01207 } 01208 01209 #endif /* USE_IOEXPANDER */ 01210 01211 /********************************* LINK AUDIO *********************************/ 01212 01213 /** 01214 * @brief Initializes Audio low level. 01215 * @retval None 01216 */ 01217 void AUDIO_IO_Init(void) 01218 { 01219 I2Cx_Init(); 01220 } 01221 01222 /** 01223 * @brief Deinitializes Audio low level. 01224 * @retval None 01225 */ 01226 void AUDIO_IO_DeInit(void) 01227 { 01228 } 01229 01230 /** 01231 * @brief Writes a single data. 01232 * @param Addr: I2C address 01233 * @param Reg: Reg address 01234 * @param Value: Data to be written 01235 * @retval None 01236 */ 01237 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01238 { 01239 uint16_t tmp = Value; 01240 01241 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01242 01243 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01244 01245 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01246 } 01247 01248 /** 01249 * @brief Reads a single data. 01250 * @param Addr: I2C address 01251 * @param Reg: Reg address 01252 * @retval Data to be read 01253 */ 01254 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01255 { 01256 uint16_t read_value = 0, tmp = 0; 01257 01258 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01259 01260 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01261 01262 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01263 01264 read_value = tmp; 01265 01266 return read_value; 01267 } 01268 01269 /** 01270 * @brief AUDIO Codec delay 01271 * @param Delay: Delay in ms 01272 * @retval None 01273 */ 01274 void AUDIO_IO_Delay(uint32_t Delay) 01275 { 01276 HAL_Delay(Delay); 01277 } 01278 01279 /********************************* LINK CAMERA ********************************/ 01280 01281 /** 01282 * @brief Initializes Camera low level. 01283 * @retval None 01284 */ 01285 void CAMERA_IO_Init(void) 01286 { 01287 I2Cx_Init(); 01288 } 01289 01290 /** 01291 * @brief Camera writes single data. 01292 * @param Addr: I2C address 01293 * @param Reg: Register address 01294 * @param Value: Data to be written 01295 * @retval None 01296 */ 01297 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01298 { 01299 uint16_t tmp = Value; 01300 /* For S5K5CAG sensor, 16 bits accesses are used */ 01301 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01302 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01303 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01304 } 01305 01306 /** 01307 * @brief Camera reads single data. 01308 * @param Addr: I2C address 01309 * @param Reg: Register address 01310 * @retval Read data 01311 */ 01312 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg) 01313 { 01314 uint16_t read_value = 0, tmp = 0; 01315 /* For S5K5CAG sensor, 16 bits accesses are used */ 01316 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01317 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01318 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01319 read_value = tmp; 01320 return read_value; 01321 } 01322 01323 /** 01324 * @brief Camera delay 01325 * @param Delay: Delay in ms 01326 * @retval None 01327 */ 01328 void CAMERA_Delay(uint32_t Delay) 01329 { 01330 HAL_Delay(Delay); 01331 } 01332 01333 /******************************** LINK I2C EEPROM *****************************/ 01334 01335 /** 01336 * @brief Initializes peripherals used by the I2C EEPROM driver. 01337 * @retval None 01338 */ 01339 void EEPROM_IO_Init(void) 01340 { 01341 I2Cx_Init(); 01342 } 01343 01344 /** 01345 * @brief Write data to I2C EEPROM driver in using DMA channel. 01346 * @param DevAddress: Target device address 01347 * @param MemAddress: Internal memory address 01348 * @param pBuffer: Pointer to data buffer 01349 * @param BufferSize: Amount of data to be sent 01350 * @retval HAL status 01351 */ 01352 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01353 { 01354 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01355 } 01356 01357 /** 01358 * @brief Read data from I2C EEPROM driver in using DMA channel. 01359 * @param DevAddress: Target device address 01360 * @param MemAddress: Internal memory address 01361 * @param pBuffer: Pointer to data buffer 01362 * @param BufferSize: Amount of data to be read 01363 * @retval HAL status 01364 */ 01365 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01366 { 01367 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01368 } 01369 01370 /** 01371 * @brief Checks if target device is ready for communication. 01372 * @note This function is used with Memory devices 01373 * @param DevAddress: Target device address 01374 * @param Trials: Number of trials 01375 * @retval HAL status 01376 */ 01377 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01378 { 01379 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01380 } 01381 01382 /** 01383 * @} 01384 */ 01385 01386 /** 01387 * @} 01388 */ 01389 01390 /** 01391 * @} 01392 */ 01393 01394 /** 01395 * @} 01396 */ 01397 01398 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 19:47:41 for STM32756G_EVAL BSP User Manual by
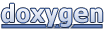