STM32756G_EVAL BSP User Manual
|
stm32756g_eval_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval_sd.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 22-May-2015 00007 * @brief This file includes the uSD card driver mounted on STM32756G-EVAL and 00008 * STM32746G-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the micro SD external card mounted on STM32756G-EVAL 00013 evaluation board. 00014 - This driver does not need a specific component driver for the micro SD device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the micro SD card using the BSP_SD_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 SDIO interface configuration to interface with the external micro SD. It 00023 also includes the micro SD initialization sequence. 00024 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00025 returns the detection status 00026 o If SD presence detection interrupt mode is desired, you must configure the 00027 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00028 is generated as an external interrupt whenever the micro SD card is 00029 plugged/unplugged in/from the evaluation board. The SD detection is managed by MFX, 00030 so the SD detection interrupt has to be treated by MFX_IRQOUT gpio pin IRQ handler. 00031 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00032 which is stored in the structure "HAL_SD_CardInfoTypedef". 00033 00034 + Micro SD card operations 00035 o The micro SD card can be accessed with read/write block(s) operations once 00036 it is ready for access. The access can be performed whether using the polling 00037 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00038 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00039 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00040 is complete, the SD interrupt is handled using the function BSP_SD_IRQHandler(), 00041 the DMA Tx/Rx transfer complete are handled using the functions 00042 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00043 are implemented by the user at application level. 00044 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00045 the number of blocks to erase. 00046 o The SD runtime status is returned when calling the function BSP_SD_GetStatus(). 00047 @endverbatim 00048 ****************************************************************************** 00049 * @attention 00050 * 00051 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00052 * 00053 * Redistribution and use in source and binary forms, with or without modification, 00054 * are permitted provided that the following conditions are met: 00055 * 1. Redistributions of source code must retain the above copyright notice, 00056 * this list of conditions and the following disclaimer. 00057 * 2. Redistributions in binary form must reproduce the above copyright notice, 00058 * this list of conditions and the following disclaimer in the documentation 00059 * and/or other materials provided with the distribution. 00060 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00061 * may be used to endorse or promote products derived from this software 00062 * without specific prior written permission. 00063 * 00064 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00065 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00066 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00067 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00068 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00069 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00070 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00071 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00072 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00073 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00074 * 00075 ****************************************************************************** 00076 */ 00077 00078 /* Includes ------------------------------------------------------------------*/ 00079 #include "stm32756g_eval_sd.h" 00080 00081 /** @addtogroup BSP 00082 * @{ 00083 */ 00084 00085 /** @addtogroup STM32756G_EVAL 00086 * @{ 00087 */ 00088 00089 /** @defgroup STM32756G_EVAL_SD 00090 * @{ 00091 */ 00092 00093 00094 /** @defgroup STM32756G_EVAL_SD_Private_TypesDefinitions 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32756G_EVAL_SD_Private_Defines 00102 * @{ 00103 */ 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM32756G_EVAL_SD_Private_Macros 00109 * @{ 00110 */ 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32756G_EVAL_SD_Private_Variables 00116 * @{ 00117 */ 00118 static SD_HandleTypeDef uSdHandle; 00119 static SD_CardInfo uSdCardInfo; 00120 static uint8_t UseExtiModeDetection = 0; 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32756G_EVAL_SD_Private_FunctionPrototypes 00127 * @{ 00128 */ 00129 /** 00130 * @} 00131 */ 00132 00133 /** @defgroup STM32756G_EVAL_SD_Private_Functions 00134 * @{ 00135 */ 00136 00137 /** 00138 * @brief Initializes the SD card device. 00139 * @retval SD status 00140 */ 00141 uint8_t BSP_SD_Init(void) 00142 { 00143 uint8_t sd_state = MSD_OK; 00144 00145 /* uSD device interface configuration */ 00146 uSdHandle.Instance = SDMMC1; 00147 00148 uSdHandle.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00149 uSdHandle.Init.ClockBypass = SDMMC_CLOCK_BYPASS_DISABLE; 00150 uSdHandle.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00151 uSdHandle.Init.BusWide = SDMMC_BUS_WIDE_1B; 00152 uSdHandle.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00153 uSdHandle.Init.ClockDiv = SDMMC_TRANSFER_CLK_DIV; 00154 00155 /* Initialize IO functionalities (MFX) used by SD detect pin */ 00156 BSP_IO_Init(); 00157 00158 /* Check if the SD card is plugged in the slot */ 00159 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT_PU); 00160 if(BSP_SD_IsDetected() != SD_PRESENT) 00161 { 00162 return MSD_ERROR_SD_NOT_PRESENT; 00163 } 00164 00165 /* Msp SD initialization */ 00166 BSP_SD_MspInit(&uSdHandle, NULL); 00167 00168 /* HAL SD initialization */ 00169 if(HAL_SD_Init(&uSdHandle, &uSdCardInfo) != SD_OK) 00170 { 00171 sd_state = MSD_ERROR; 00172 } 00173 00174 /* Configure SD Bus width */ 00175 if(sd_state == MSD_OK) 00176 { 00177 /* Enable wide operation */ 00178 if(HAL_SD_WideBusOperation_Config(&uSdHandle, SDMMC_BUS_WIDE_4B) != SD_OK) 00179 { 00180 sd_state = MSD_ERROR; 00181 } 00182 else 00183 { 00184 sd_state = MSD_OK; 00185 } 00186 } 00187 00188 return sd_state; 00189 } 00190 00191 /** 00192 * @brief DeInitializes the SD card device. 00193 * @retval SD status 00194 */ 00195 uint8_t BSP_SD_DeInit(void) 00196 { 00197 uint8_t sd_state = MSD_OK; 00198 00199 uSdHandle.Instance = SDMMC1; 00200 00201 /* Set back Mfx pin to INPUT mode in case it was in exti */ 00202 UseExtiModeDetection = 0; 00203 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_INPUT_PU); 00204 00205 /* HAL SD deinitialization */ 00206 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00207 { 00208 sd_state = MSD_ERROR; 00209 } 00210 00211 /* Msp SD deinitialization */ 00212 uSdHandle.Instance = SDMMC1; 00213 BSP_SD_MspDeInit(&uSdHandle, NULL); 00214 00215 return sd_state; 00216 } 00217 00218 /** 00219 * @brief Configures Interrupt mode for SD detection pin. 00220 * @retval Returns 0 00221 */ 00222 uint8_t BSP_SD_ITConfig(void) 00223 { 00224 /* Configure Interrupt mode for SD detection pin */ 00225 /* Note: disabling exti mode can be done calling SD_DeInit() */ 00226 UseExtiModeDetection = 1; 00227 BSP_SD_IsDetected(); 00228 00229 return 0; 00230 } 00231 00232 /** 00233 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00234 * @param None 00235 * @retval Returns if SD is detected or not 00236 */ 00237 uint8_t BSP_SD_IsDetected(void) 00238 { 00239 __IO uint8_t status = SD_PRESENT; 00240 00241 /* Check SD card detect pin */ 00242 if((BSP_IO_ReadPin(SD_DETECT_PIN)&SD_DETECT_PIN) != SD_DETECT_PIN) 00243 { 00244 if (UseExtiModeDetection) 00245 { 00246 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_RISING_EDGE_PU); 00247 } 00248 } 00249 else 00250 { 00251 status = SD_NOT_PRESENT; 00252 if (UseExtiModeDetection) 00253 { 00254 BSP_IO_ConfigPin(SD_DETECT_PIN, IO_MODE_IT_FALLING_EDGE_PU); 00255 } 00256 } 00257 return status; 00258 } 00259 00260 /** 00261 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00262 * @param pData: Pointer to the buffer that will contain the data to transmit 00263 * @param ReadAddr: Address from where data is to be read 00264 * @param BlockSize: SD card data block size, that should be 512 00265 * @param NumOfBlocks: Number of SD blocks to read 00266 * @retval SD status 00267 */ 00268 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00269 { 00270 if(HAL_SD_ReadBlocks(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00271 { 00272 return MSD_ERROR; 00273 } 00274 else 00275 { 00276 return MSD_OK; 00277 } 00278 } 00279 00280 /** 00281 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00282 * @param pData: Pointer to the buffer that will contain the data to transmit 00283 * @param WriteAddr: Address from where data is to be written 00284 * @param BlockSize: SD card data block size, that should be 512 00285 * @param NumOfBlocks: Number of SD blocks to write 00286 * @retval SD status 00287 */ 00288 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00289 { 00290 if(HAL_SD_WriteBlocks(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00291 { 00292 return MSD_ERROR; 00293 } 00294 else 00295 { 00296 return MSD_OK; 00297 } 00298 } 00299 00300 /** 00301 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00302 * @param pData: Pointer to the buffer that will contain the data to transmit 00303 * @param ReadAddr: Address from where data is to be read 00304 * @param BlockSize: SD card data block size, that should be 512 00305 * @param NumOfBlocks: Number of SD blocks to read 00306 * @retval SD status 00307 */ 00308 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00309 { 00310 uint8_t sd_state = MSD_OK; 00311 00312 /* Read block(s) in DMA transfer mode */ 00313 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, pData, ReadAddr, BlockSize, NumOfBlocks) != SD_OK) 00314 { 00315 sd_state = MSD_ERROR; 00316 } 00317 00318 /* Wait until transfer is complete */ 00319 if(sd_state == MSD_OK) 00320 { 00321 if(HAL_SD_CheckReadOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00322 { 00323 sd_state = MSD_ERROR; 00324 } 00325 else 00326 { 00327 sd_state = MSD_OK; 00328 } 00329 } 00330 00331 return sd_state; 00332 } 00333 00334 /** 00335 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00336 * @param pData: Pointer to the buffer that will contain the data to transmit 00337 * @param WriteAddr: Address from where data is to be written 00338 * @param BlockSize: SD card data block size, that should be 512 00339 * @param NumOfBlocks: Number of SD blocks to write 00340 * @retval SD status 00341 */ 00342 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks) 00343 { 00344 uint8_t sd_state = MSD_OK; 00345 00346 /* Write block(s) in DMA transfer mode */ 00347 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, pData, WriteAddr, BlockSize, NumOfBlocks) != SD_OK) 00348 { 00349 sd_state = MSD_ERROR; 00350 } 00351 00352 /* Wait until transfer is complete */ 00353 if(sd_state == MSD_OK) 00354 { 00355 if(HAL_SD_CheckWriteOperation(&uSdHandle, (uint32_t)SD_DATATIMEOUT) != SD_OK) 00356 { 00357 sd_state = MSD_ERROR; 00358 } 00359 else 00360 { 00361 sd_state = MSD_OK; 00362 } 00363 } 00364 00365 return sd_state; 00366 } 00367 00368 /** 00369 * @brief Erases the specified memory area of the given SD card. 00370 * @param StartAddr: Start byte address 00371 * @param EndAddr: End byte address 00372 * @retval SD status 00373 */ 00374 uint8_t BSP_SD_Erase(uint64_t StartAddr, uint64_t EndAddr) 00375 { 00376 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != SD_OK) 00377 { 00378 return MSD_ERROR; 00379 } 00380 else 00381 { 00382 return MSD_OK; 00383 } 00384 } 00385 00386 /** 00387 * @brief Initializes the SD MSP. 00388 * @param hsd: SD handle 00389 * @retval None 00390 */ 00391 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00392 { 00393 static DMA_HandleTypeDef dma_rx_handle; 00394 static DMA_HandleTypeDef dma_tx_handle; 00395 GPIO_InitTypeDef gpio_init_structure; 00396 00397 /* Camera has to be powered down as some signals use same GPIOs between 00398 * SD card and camera bus. Camera drives its signals to low impedance 00399 * when powered ON. So the camera is powered off to let its signals 00400 * in high impedance */ 00401 00402 /* Camera power down sequence */ 00403 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00404 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00405 /* De-assert the camera STANDBY pin (active high) */ 00406 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00407 /* Assert the camera RSTI pin (active low) */ 00408 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00409 00410 /* Enable SDIO clock */ 00411 __HAL_RCC_SDMMC1_CLK_ENABLE(); 00412 00413 /* Enable DMA2 clocks */ 00414 __DMAx_TxRx_CLK_ENABLE(); 00415 00416 /* Enable GPIOs clock */ 00417 __HAL_RCC_GPIOC_CLK_ENABLE(); 00418 __HAL_RCC_GPIOD_CLK_ENABLE(); 00419 00420 /* Common GPIO configuration */ 00421 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00422 gpio_init_structure.Pull = GPIO_PULLUP; 00423 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00424 gpio_init_structure.Alternate = GPIO_AF12_SDMMC1; 00425 00426 /* GPIOC configuration */ 00427 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00428 00429 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00430 00431 /* GPIOD configuration */ 00432 gpio_init_structure.Pin = GPIO_PIN_2; 00433 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00434 00435 /* NVIC configuration for SDIO interrupts */ 00436 HAL_NVIC_SetPriority(SDMMC1_IRQn, 5, 0); 00437 HAL_NVIC_EnableIRQ(SDMMC1_IRQn); 00438 00439 /* Configure DMA Rx parameters */ 00440 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00441 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00442 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00443 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00444 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00445 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00446 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00447 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00448 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00449 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00450 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00451 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00452 00453 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00454 00455 /* Associate the DMA handle */ 00456 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00457 00458 /* Deinitialize the stream for new transfer */ 00459 HAL_DMA_DeInit(&dma_rx_handle); 00460 00461 /* Configure the DMA stream */ 00462 HAL_DMA_Init(&dma_rx_handle); 00463 00464 /* Configure DMA Tx parameters */ 00465 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00466 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00467 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00468 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00469 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00470 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00471 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00472 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00473 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00474 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00475 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00476 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00477 00478 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00479 00480 /* Associate the DMA handle */ 00481 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00482 00483 /* Deinitialize the stream for new transfer */ 00484 HAL_DMA_DeInit(&dma_tx_handle); 00485 00486 /* Configure the DMA stream */ 00487 HAL_DMA_Init(&dma_tx_handle); 00488 00489 /* NVIC configuration for DMA transfer complete interrupt */ 00490 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 6, 0); 00491 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00492 00493 /* NVIC configuration for DMA transfer complete interrupt */ 00494 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 6, 0); 00495 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00496 } 00497 00498 /** 00499 * @brief DeInitializes the SD MSP. 00500 * @param hsd: SD handle 00501 * @retval None 00502 */ 00503 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00504 { 00505 static DMA_HandleTypeDef dma_rx_handle; 00506 static DMA_HandleTypeDef dma_tx_handle; 00507 00508 /* Disable NVIC for DMA transfer complete interrupts */ 00509 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00510 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00511 00512 /* Deinitialize the stream for new transfer */ 00513 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00514 HAL_DMA_DeInit(&dma_rx_handle); 00515 00516 /* Deinitialize the stream for new transfer */ 00517 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00518 HAL_DMA_DeInit(&dma_tx_handle); 00519 00520 /* Disable NVIC for SDIO interrupts */ 00521 HAL_NVIC_DisableIRQ(SDMMC1_IRQn); 00522 00523 /* DeInit GPIO pins can be done in the application 00524 (by surcharging this __weak function) */ 00525 00526 /* Disable SDMMC1 clock */ 00527 __HAL_RCC_SDMMC1_CLK_DISABLE(); 00528 00529 /* GPIO pins clock and DMA clocks can be shut down in the application 00530 by surcharging this __weak function */ 00531 } 00532 00533 /** 00534 * @brief Handles SD card interrupt request. 00535 * @retval None 00536 */ 00537 void BSP_SD_IRQHandler(void) 00538 { 00539 HAL_SD_IRQHandler(&uSdHandle); 00540 } 00541 00542 /** 00543 * @brief Handles SD DMA Tx transfer interrupt request. 00544 * @retval None 00545 */ 00546 void BSP_SD_DMA_Tx_IRQHandler(void) 00547 { 00548 HAL_DMA_IRQHandler(uSdHandle.hdmatx); 00549 } 00550 00551 /** 00552 * @brief Handles SD DMA Rx transfer interrupt request. 00553 * @retval None 00554 */ 00555 void BSP_SD_DMA_Rx_IRQHandler(void) 00556 { 00557 HAL_DMA_IRQHandler(uSdHandle.hdmarx); 00558 } 00559 00560 /** 00561 * @brief Gets the current SD card data status. 00562 * @retval Data transfer state. 00563 * This value can be one of the following values: 00564 * @arg SD_TRANSFER_OK: No data transfer is acting 00565 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00566 * @arg SD_TRANSFER_ERROR: Data transfer error 00567 */ 00568 HAL_SD_TransferStateTypedef BSP_SD_GetStatus(void) 00569 { 00570 return(HAL_SD_GetStatus(&uSdHandle)); 00571 } 00572 00573 /** 00574 * @brief Get SD information about specific SD card. 00575 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00576 * @retval None 00577 */ 00578 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypedef *CardInfo) 00579 { 00580 /* Get SD card Information */ 00581 HAL_SD_Get_CardInfo(&uSdHandle, CardInfo); 00582 } 00583 00584 /** 00585 * @} 00586 */ 00587 00588 /** 00589 * @} 00590 */ 00591 00592 /** 00593 * @} 00594 */ 00595 00596 /** 00597 * @} 00598 */ 00599 00600 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri May 22 2015 13:59:20 for STM32756G_EVAL BSP User Manual by
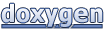