STM32746G-Discovery BSP User Manual
|
stm32746g_discovery_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32746g_discovery_sd.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes the uSD card driver mounted on STM32746G-Discovery 00008 * board. 00009 @verbatim 00010 1. How To use this driver: 00011 -------------------------- 00012 - This driver is used to drive the micro SD external card mounted on STM32746G-Discovery 00013 board. 00014 - This driver does not need a specific component driver for the micro SD device 00015 to be included with. 00016 00017 2. Driver description: 00018 --------------------- 00019 + Initialization steps: 00020 o Initialize the micro SD card using the BSP_SD_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 SDIO interface configuration to interface with the external micro SD. It 00023 also includes the micro SD initialization sequence. 00024 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00025 returns the detection status 00026 o If SD presence detection interrupt mode is desired, you must configure the 00027 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00028 is generated as an external interrupt whenever the micro SD card is 00029 plugged/unplugged in/from the board. 00030 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00031 which is stored in the structure "HAL_SD_CardInfoTypedef". 00032 00033 + Micro SD card operations 00034 o The micro SD card can be accessed with read/write block(s) operations once 00035 it is ready for access. The access can be performed whether using the polling 00036 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00037 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00038 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00039 is complete, the SD interrupt is handled using the function BSP_SD_IRQHandler(), 00040 the DMA Tx/Rx transfer complete are handled using the functions 00041 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00042 are implemented by the user at application level. 00043 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00044 the number of blocks to erase. 00045 o The SD runtime status is returned when calling the function BSP_SD_GetCardState(). 00046 00047 @endverbatim 00048 ****************************************************************************** 00049 * @attention 00050 * 00051 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00052 * 00053 * Redistribution and use in source and binary forms, with or without modification, 00054 * are permitted provided that the following conditions are met: 00055 * 1. Redistributions of source code must retain the above copyright notice, 00056 * this list of conditions and the following disclaimer. 00057 * 2. Redistributions in binary form must reproduce the above copyright notice, 00058 * this list of conditions and the following disclaimer in the documentation 00059 * and/or other materials provided with the distribution. 00060 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00061 * may be used to endorse or promote products derived from this software 00062 * without specific prior written permission. 00063 * 00064 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00065 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00066 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00067 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00068 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00069 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00070 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00071 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00072 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00073 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00074 * 00075 ****************************************************************************** 00076 */ 00077 00078 /* Includes ------------------------------------------------------------------*/ 00079 #include "stm32746g_discovery_sd.h" 00080 00081 /** @addtogroup BSP 00082 * @{ 00083 */ 00084 00085 /** @addtogroup STM32746G_DISCOVERY 00086 * @{ 00087 */ 00088 00089 /** @defgroup STM32746G_DISCOVERY_SD STM32746G_DISCOVERY_SD 00090 * @{ 00091 */ 00092 00093 00094 /** @defgroup STM32746G_DISCOVERY_SD_Private_TypesDefinitions STM32746G_DISCOVERY_SD Private Types Definitions 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32746G_DISCOVERY_SD_Private_Defines STM32746G_DISCOVERY_SD Private Defines 00102 * @{ 00103 */ 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM32746G_DISCOVERY_SD_Private_Macros STM32746G_DISCOVERY_SD Private Macros 00109 * @{ 00110 */ 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32746G_DISCOVERY_SD_Private_Variables STM32746G_DISCOVERY_SD Private Variables 00116 * @{ 00117 */ 00118 SD_HandleTypeDef uSdHandle; 00119 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM32746G_DISCOVERY_SD_Private_FunctionPrototypes STM32746G_DISCOVERY_SD Private Function Prototypes 00125 * @{ 00126 */ 00127 /** 00128 * @} 00129 */ 00130 00131 /** @defgroup STM32746G_DISCOVERY_SD_Exported_Functions STM32746G_DISCOVERY_SD Exported Functions 00132 * @{ 00133 */ 00134 00135 /** 00136 * @brief Initializes the SD card device. 00137 * @retval SD status 00138 */ 00139 uint8_t BSP_SD_Init(void) 00140 { 00141 uint8_t sd_state = MSD_OK; 00142 00143 /* uSD device interface configuration */ 00144 uSdHandle.Instance = SDMMC1; 00145 00146 uSdHandle.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00147 uSdHandle.Init.ClockBypass = SDMMC_CLOCK_BYPASS_DISABLE; 00148 uSdHandle.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00149 uSdHandle.Init.BusWide = SDMMC_BUS_WIDE_1B; 00150 uSdHandle.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00151 uSdHandle.Init.ClockDiv = SDMMC_TRANSFER_CLK_DIV; 00152 00153 /* Msp SD Detect pin initialization */ 00154 BSP_SD_Detect_MspInit(&uSdHandle, NULL); 00155 if(BSP_SD_IsDetected() != SD_PRESENT) /* Check if SD card is present */ 00156 { 00157 return MSD_ERROR_SD_NOT_PRESENT; 00158 } 00159 00160 /* Msp SD initialization */ 00161 BSP_SD_MspInit(&uSdHandle, NULL); 00162 00163 /* HAL SD initialization */ 00164 if(HAL_SD_Init(&uSdHandle) != HAL_OK) 00165 { 00166 sd_state = MSD_ERROR; 00167 } 00168 00169 /* Configure SD Bus width */ 00170 if(sd_state == MSD_OK) 00171 { 00172 /* Enable wide operation */ 00173 if(HAL_SD_ConfigWideBusOperation(&uSdHandle, SDMMC_BUS_WIDE_4B) != HAL_OK) 00174 { 00175 sd_state = MSD_ERROR; 00176 } 00177 else 00178 { 00179 sd_state = MSD_OK; 00180 } 00181 } 00182 00183 return sd_state; 00184 } 00185 00186 /** 00187 * @brief DeInitializes the SD card device. 00188 * @retval SD status 00189 */ 00190 uint8_t BSP_SD_DeInit(void) 00191 { 00192 uint8_t sd_state = MSD_OK; 00193 00194 uSdHandle.Instance = SDMMC1; 00195 00196 /* HAL SD deinitialization */ 00197 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00198 { 00199 sd_state = MSD_ERROR; 00200 } 00201 00202 /* Msp SD deinitialization */ 00203 uSdHandle.Instance = SDMMC1; 00204 BSP_SD_MspDeInit(&uSdHandle, NULL); 00205 00206 return sd_state; 00207 } 00208 00209 /** 00210 * @brief Configures Interrupt mode for SD detection pin. 00211 * @retval Returns MSD_OK 00212 */ 00213 uint8_t BSP_SD_ITConfig(void) 00214 { 00215 GPIO_InitTypeDef gpio_init_structure; 00216 00217 /* Configure Interrupt mode for SD detection pin */ 00218 gpio_init_structure.Pin = SD_DETECT_PIN; 00219 gpio_init_structure.Pull = GPIO_PULLUP; 00220 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00221 gpio_init_structure.Mode = GPIO_MODE_IT_RISING_FALLING; 00222 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00223 00224 /* Enable and set SD detect EXTI Interrupt to the lowest priority */ 00225 HAL_NVIC_SetPriority((IRQn_Type)(SD_DETECT_EXTI_IRQn), 0x0F, 0x00); 00226 HAL_NVIC_EnableIRQ((IRQn_Type)(SD_DETECT_EXTI_IRQn)); 00227 00228 return MSD_OK; 00229 } 00230 00231 /** 00232 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00233 * @retval Returns if SD is detected or not 00234 */ 00235 uint8_t BSP_SD_IsDetected(void) 00236 { 00237 __IO uint8_t status = SD_PRESENT; 00238 00239 /* Check SD card detect pin */ 00240 if (HAL_GPIO_ReadPin(SD_DETECT_GPIO_PORT, SD_DETECT_PIN) == GPIO_PIN_SET) 00241 { 00242 status = SD_NOT_PRESENT; 00243 } 00244 00245 return status; 00246 } 00247 00248 /** 00249 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00250 * @param pData: Pointer to the buffer that will contain the data to transmit 00251 * @param ReadAddr: Address from where data is to be read 00252 * @param NumOfBlocks: Number of SD blocks to read 00253 * @param Timeout: Timeout for read operation 00254 * @retval SD status 00255 */ 00256 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00257 { 00258 if(HAL_SD_ReadBlocks(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout) != HAL_OK) 00259 { 00260 return MSD_ERROR; 00261 } 00262 else 00263 { 00264 return MSD_OK; 00265 } 00266 } 00267 00268 /** 00269 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00270 * @param pData: Pointer to the buffer that will contain the data to transmit 00271 * @param WriteAddr: Address from where data is to be written 00272 * @param NumOfBlocks: Number of SD blocks to write 00273 * @param Timeout: Timeout for write operation 00274 * @retval SD status 00275 */ 00276 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00277 { 00278 if(HAL_SD_WriteBlocks(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout) != HAL_OK) 00279 { 00280 return MSD_ERROR; 00281 } 00282 else 00283 { 00284 return MSD_OK; 00285 } 00286 } 00287 00288 /** 00289 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00290 * @param pData: Pointer to the buffer that will contain the data to transmit 00291 * @param ReadAddr: Address from where data is to be read 00292 * @param NumOfBlocks: Number of SD blocks to read 00293 * @retval SD status 00294 */ 00295 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00296 { 00297 /* Read block(s) in DMA transfer mode */ 00298 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks) != HAL_OK) 00299 { 00300 return MSD_ERROR; 00301 } 00302 else 00303 { 00304 return MSD_OK; 00305 } 00306 } 00307 00308 /** 00309 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00310 * @param pData: Pointer to the buffer that will contain the data to transmit 00311 * @param WriteAddr: Address from where data is to be written 00312 * @param NumOfBlocks: Number of SD blocks to write 00313 * @retval SD status 00314 */ 00315 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00316 { 00317 /* Write block(s) in DMA transfer mode */ 00318 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks) != HAL_OK) 00319 { 00320 return MSD_ERROR; 00321 } 00322 else 00323 { 00324 return MSD_OK; 00325 } 00326 } 00327 00328 /** 00329 * @brief Erases the specified memory area of the given SD card. 00330 * @param StartAddr: Start byte address 00331 * @param EndAddr: End byte address 00332 * @retval SD status 00333 */ 00334 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr) 00335 { 00336 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != HAL_OK) 00337 { 00338 return MSD_ERROR; 00339 } 00340 else 00341 { 00342 return MSD_OK; 00343 } 00344 } 00345 00346 /** 00347 * @brief Initializes the SD MSP. 00348 * @param hsd: SD handle 00349 * @param Params 00350 * @retval None 00351 */ 00352 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00353 { 00354 static DMA_HandleTypeDef dma_rx_handle; 00355 static DMA_HandleTypeDef dma_tx_handle; 00356 GPIO_InitTypeDef gpio_init_structure; 00357 00358 /* Enable SDIO clock */ 00359 __HAL_RCC_SDMMC1_CLK_ENABLE(); 00360 00361 /* Enable DMA2 clocks */ 00362 __DMAx_TxRx_CLK_ENABLE(); 00363 00364 /* Enable GPIOs clock */ 00365 __HAL_RCC_GPIOC_CLK_ENABLE(); 00366 __HAL_RCC_GPIOD_CLK_ENABLE(); 00367 00368 /* Common GPIO configuration */ 00369 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00370 gpio_init_structure.Pull = GPIO_PULLUP; 00371 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00372 gpio_init_structure.Alternate = GPIO_AF12_SDMMC1; 00373 00374 /* GPIOC configuration */ 00375 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00376 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00377 00378 /* GPIOD configuration */ 00379 gpio_init_structure.Pin = GPIO_PIN_2; 00380 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00381 00382 /* NVIC configuration for SDIO interrupts */ 00383 HAL_NVIC_SetPriority(SDMMC1_IRQn, 0x0E, 0); 00384 HAL_NVIC_EnableIRQ(SDMMC1_IRQn); 00385 00386 /* Configure DMA Rx parameters */ 00387 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00388 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00389 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00390 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00391 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00392 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00393 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00394 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00395 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00396 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00397 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00398 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00399 00400 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00401 00402 /* Associate the DMA handle */ 00403 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00404 00405 /* Deinitialize the stream for new transfer */ 00406 HAL_DMA_DeInit(&dma_rx_handle); 00407 00408 /* Configure the DMA stream */ 00409 HAL_DMA_Init(&dma_rx_handle); 00410 00411 /* Configure DMA Tx parameters */ 00412 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00413 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00414 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00415 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00416 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00417 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00418 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00419 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00420 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00421 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00422 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00423 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00424 00425 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00426 00427 /* Associate the DMA handle */ 00428 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00429 00430 /* Deinitialize the stream for new transfer */ 00431 HAL_DMA_DeInit(&dma_tx_handle); 00432 00433 /* Configure the DMA stream */ 00434 HAL_DMA_Init(&dma_tx_handle); 00435 00436 /* NVIC configuration for DMA transfer complete interrupt */ 00437 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 0x0F, 0); 00438 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00439 00440 /* NVIC configuration for DMA transfer complete interrupt */ 00441 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 0x0F, 0); 00442 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00443 } 00444 00445 /** 00446 * @brief Initializes the SD Detect pin MSP. 00447 * @param hsd: SD handle 00448 * @param Params 00449 * @retval None 00450 */ 00451 __weak void BSP_SD_Detect_MspInit(SD_HandleTypeDef *hsd, void *Params) 00452 { 00453 GPIO_InitTypeDef gpio_init_structure; 00454 00455 SD_DETECT_GPIO_CLK_ENABLE(); 00456 00457 /* GPIO configuration in input for uSD_Detect signal */ 00458 gpio_init_structure.Pin = SD_DETECT_PIN; 00459 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00460 gpio_init_structure.Pull = GPIO_PULLUP; 00461 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00462 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00463 } 00464 00465 /** 00466 * @brief DeInitializes the SD MSP. 00467 * @param hsd: SD handle 00468 * @param Params 00469 * @retval None 00470 */ 00471 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00472 { 00473 static DMA_HandleTypeDef dma_rx_handle; 00474 static DMA_HandleTypeDef dma_tx_handle; 00475 00476 /* Disable NVIC for DMA transfer complete interrupts */ 00477 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00478 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00479 00480 /* Deinitialize the stream for new transfer */ 00481 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00482 HAL_DMA_DeInit(&dma_rx_handle); 00483 00484 /* Deinitialize the stream for new transfer */ 00485 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00486 HAL_DMA_DeInit(&dma_tx_handle); 00487 00488 /* Disable NVIC for SDIO interrupts */ 00489 HAL_NVIC_DisableIRQ(SDMMC1_IRQn); 00490 00491 /* DeInit GPIO pins can be done in the application 00492 (by surcharging this __weak function) */ 00493 00494 /* Disable SDMMC1 clock */ 00495 __HAL_RCC_SDMMC1_CLK_DISABLE(); 00496 00497 /* GPIO pins clock and DMA clocks can be shut down in the application 00498 by surcharging this __weak function */ 00499 } 00500 00501 /** 00502 * @brief Gets the current SD card data status. 00503 * @retval Data transfer state. 00504 * This value can be one of the following values: 00505 * @arg SD_TRANSFER_OK: No data transfer is acting 00506 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00507 */ 00508 uint8_t BSP_SD_GetCardState(void) 00509 { 00510 return((HAL_SD_GetCardState(&uSdHandle) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00511 } 00512 00513 00514 /** 00515 * @brief Get SD information about specific SD card. 00516 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00517 * @retval None 00518 */ 00519 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypeDef *CardInfo) 00520 { 00521 /* Get SD card Information */ 00522 HAL_SD_GetCardInfo(&uSdHandle, CardInfo); 00523 } 00524 00525 /** 00526 * @brief SD Abort callbacks 00527 * @param hsd: SD handle 00528 * @retval None 00529 */ 00530 void HAL_SD_AbortCallback(SD_HandleTypeDef *hsd) 00531 { 00532 BSP_SD_AbortCallback(); 00533 } 00534 00535 /** 00536 * @brief Tx Transfer completed callbacks 00537 * @param hsd: SD handle 00538 * @retval None 00539 */ 00540 void HAL_SD_TxCpltCallback(SD_HandleTypeDef *hsd) 00541 { 00542 BSP_SD_WriteCpltCallback(); 00543 } 00544 00545 /** 00546 * @brief Rx Transfer completed callbacks 00547 * @param hsd: SD handle 00548 * @retval None 00549 */ 00550 void HAL_SD_RxCpltCallback(SD_HandleTypeDef *hsd) 00551 { 00552 BSP_SD_ReadCpltCallback(); 00553 } 00554 00555 /** 00556 * @brief BSP SD Abort callbacks 00557 * @retval None 00558 */ 00559 __weak void BSP_SD_AbortCallback(void) 00560 { 00561 00562 } 00563 00564 /** 00565 * @brief BSP Tx Transfer completed callbacks 00566 * @retval None 00567 */ 00568 __weak void BSP_SD_WriteCpltCallback(void) 00569 { 00570 00571 } 00572 00573 /** 00574 * @brief BSP Rx Transfer completed callbacks 00575 * @retval None 00576 */ 00577 __weak void BSP_SD_ReadCpltCallback(void) 00578 { 00579 00580 } 00581 00582 /** 00583 * @} 00584 */ 00585 00586 /** 00587 * @} 00588 */ 00589 00590 /** 00591 * @} 00592 */ 00593 00594 /** 00595 * @} 00596 */ 00597 00598 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 16:31:33 for STM32746G-Discovery BSP User Manual by
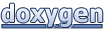