STM32746G-Discovery BSP User Manual
|
This file provides the Audio driver for the STM32746G-Discovery board. More...
#include "stm32746g_discovery_audio.h"
Go to the source code of this file.
Functions | |
static void | SAIx_Out_Init (uint32_t AudioFreq) |
Initializes the output Audio Codec audio interface (SAI). | |
static void | SAIx_Out_DeInit (void) |
Deinitializes the output Audio Codec audio interface (SAI). | |
static void | SAIx_In_Init (uint32_t SaiOutMode, uint32_t SlotActive, uint32_t AudioFreq) |
Initializes the input Audio Codec audio interface (SAI). | |
static void | SAIx_In_DeInit (void) |
Deinitializes the output Audio Codec audio interface (SAI). | |
uint8_t | BSP_AUDIO_OUT_Init (uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) |
Configures the audio peripherals. | |
uint8_t | BSP_AUDIO_OUT_Play (uint16_t *pBuffer, uint32_t Size) |
Starts playing audio stream from a data buffer for a determined size. | |
void | BSP_AUDIO_OUT_ChangeBuffer (uint16_t *pData, uint16_t Size) |
Sends n-Bytes on the SAI interface. | |
uint8_t | BSP_AUDIO_OUT_Pause (void) |
This function Pauses the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Resume (void) |
This function Resumes the audio file stream. | |
uint8_t | BSP_AUDIO_OUT_Stop (uint32_t Option) |
Stops audio playing and Power down the Audio Codec. | |
uint8_t | BSP_AUDIO_OUT_SetVolume (uint8_t Volume) |
Controls the current audio volume level. | |
uint8_t | BSP_AUDIO_OUT_SetMute (uint32_t Cmd) |
Enables or disables the MUTE mode by software. | |
uint8_t | BSP_AUDIO_OUT_SetOutputMode (uint8_t Output) |
Switch dynamically (while audio file is played) the output target (speaker or headphone). | |
void | BSP_AUDIO_OUT_SetFrequency (uint32_t AudioFreq) |
Updates the audio frequency. | |
void | BSP_AUDIO_OUT_SetAudioFrameSlot (uint32_t AudioFrameSlot) |
Updates the Audio frame slot configuration. | |
void | BSP_AUDIO_OUT_DeInit (void) |
Deinit the audio peripherals. | |
void | HAL_SAI_TxCpltCallback (SAI_HandleTypeDef *hsai) |
Tx Transfer completed callbacks. | |
void | HAL_SAI_TxHalfCpltCallback (SAI_HandleTypeDef *hsai) |
Tx Half Transfer completed callbacks. | |
void | HAL_SAI_ErrorCallback (SAI_HandleTypeDef *hsai) |
SAI error callbacks. | |
__weak void | BSP_AUDIO_OUT_TransferComplete_CallBack (void) |
Manages the DMA full Transfer complete event. | |
__weak void | BSP_AUDIO_OUT_HalfTransfer_CallBack (void) |
Manages the DMA Half Transfer complete event. | |
__weak void | BSP_AUDIO_OUT_Error_CallBack (void) |
Manages the DMA FIFO error event. | |
__weak void | BSP_AUDIO_OUT_MspInit (SAI_HandleTypeDef *hsai, void *Params) |
Initializes BSP_AUDIO_OUT MSP. | |
__weak void | BSP_AUDIO_OUT_MspDeInit (SAI_HandleTypeDef *hsai, void *Params) |
Deinitializes SAI MSP. | |
__weak void | BSP_AUDIO_OUT_ClockConfig (SAI_HandleTypeDef *hsai, uint32_t AudioFreq, void *Params) |
Clock Config. | |
uint8_t | BSP_AUDIO_IN_Init (uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr) |
Initializes wave recording. | |
uint8_t | BSP_AUDIO_IN_InitEx (uint16_t InputDevice, uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr) |
Initializes wave recording. | |
uint8_t | BSP_AUDIO_IN_OUT_Init (uint16_t InputDevice, uint16_t OutputDevice, uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr) |
Initializes wave recording and playback in parallel. | |
uint8_t | BSP_AUDIO_IN_Record (uint16_t *pbuf, uint32_t size) |
Starts audio recording. | |
uint8_t | BSP_AUDIO_IN_Stop (uint32_t Option) |
Stops audio recording. | |
uint8_t | BSP_AUDIO_IN_Pause (void) |
Pauses the audio file stream. | |
uint8_t | BSP_AUDIO_IN_Resume (void) |
Resumes the audio file stream. | |
uint8_t | BSP_AUDIO_IN_SetVolume (uint8_t Volume) |
Controls the audio in volume level. | |
void | BSP_AUDIO_IN_DeInit (void) |
Deinit the audio IN peripherals. | |
void | HAL_SAI_RxCpltCallback (SAI_HandleTypeDef *hsai) |
Rx Transfer completed callbacks. | |
void | HAL_SAI_RxHalfCpltCallback (SAI_HandleTypeDef *hsai) |
Rx Half Transfer completed callbacks. | |
__weak void | BSP_AUDIO_IN_TransferComplete_CallBack (void) |
User callback when record buffer is filled. | |
__weak void | BSP_AUDIO_IN_HalfTransfer_CallBack (void) |
Manages the DMA Half Transfer complete event. | |
__weak void | BSP_AUDIO_IN_Error_CallBack (void) |
Audio IN Error callback function. | |
__weak void | BSP_AUDIO_IN_MspInit (SAI_HandleTypeDef *hsai, void *Params) |
Initializes BSP_AUDIO_IN MSP. | |
__weak void | BSP_AUDIO_IN_MspDeInit (SAI_HandleTypeDef *hsai, void *Params) |
DeInitializes BSP_AUDIO_IN MSP. | |
Variables | |
AUDIO_DrvTypeDef * | audio_drv |
SAI_HandleTypeDef | haudio_out_sai = {0} |
SAI_HandleTypeDef | haudio_in_sai = {0} |
TIM_HandleTypeDef | haudio_tim |
uint16_t __IO | AudioInVolume = DEFAULT_AUDIO_IN_VOLUME |
Detailed Description
This file provides the Audio driver for the STM32746G-Discovery board.
- Attention:
© COPYRIGHT(c) 2016 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file stm32746g_discovery_audio.c.
Generated on Fri Dec 30 2016 16:31:33 for STM32746G-Discovery BSP User Manual by
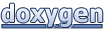