STM32746G-Discovery BSP User Manual
|
stm32746g_discovery_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32746g_discovery_lcd.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32746G-Discovery board. 00009 @verbatim 00010 1. How To use this driver: 00011 -------------------------- 00012 - This driver is used to drive directly an LCD TFT using the LTDC controller. 00013 - This driver uses timing and setting for RK043FN48H LCD. 00014 00015 2. Driver description: 00016 --------------------- 00017 + Initialization steps: 00018 o Initialize the LCD using the BSP_LCD_Init() function. 00019 o Apply the Layer configuration using the BSP_LCD_LayerDefaultInit() function. 00020 o Select the LCD layer to be used using the BSP_LCD_SelectLayer() function. 00021 o Enable the LCD display using the BSP_LCD_DisplayOn() function. 00022 00023 + Options 00024 o Configure and enable the color keying functionality using the 00025 BSP_LCD_SetColorKeying() function. 00026 o Modify in the fly the transparency and/or the frame buffer address 00027 using the following functions: 00028 - BSP_LCD_SetTransparency() 00029 - BSP_LCD_SetLayerAddress() 00030 00031 + Display on LCD 00032 o Clear the hole LCD using BSP_LCD_Clear() function or only one specified string 00033 line using the BSP_LCD_ClearStringLine() function. 00034 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00035 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00036 o Display a string line on the specified position (x,y in pixel) and align mode 00037 using the BSP_LCD_DisplayStringAtLine() function. 00038 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00039 on LCD using the available set of functions. 00040 @endverbatim 00041 ****************************************************************************** 00042 * @attention 00043 * 00044 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00045 * 00046 * Redistribution and use in source and binary forms, with or without modification, 00047 * are permitted provided that the following conditions are met: 00048 * 1. Redistributions of source code must retain the above copyright notice, 00049 * this list of conditions and the following disclaimer. 00050 * 2. Redistributions in binary form must reproduce the above copyright notice, 00051 * this list of conditions and the following disclaimer in the documentation 00052 * and/or other materials provided with the distribution. 00053 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00054 * may be used to endorse or promote products derived from this software 00055 * without specific prior written permission. 00056 * 00057 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00058 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00059 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00060 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00061 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00062 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00063 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00064 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00065 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00066 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00067 * 00068 ****************************************************************************** 00069 */ 00070 00071 /* Includes ------------------------------------------------------------------*/ 00072 #include "stm32746g_discovery_lcd.h" 00073 #include "../../../Utilities/Fonts/fonts.h" 00074 #include "../../../Utilities/Fonts/font24.c" 00075 #include "../../../Utilities/Fonts/font20.c" 00076 #include "../../../Utilities/Fonts/font16.c" 00077 #include "../../../Utilities/Fonts/font12.c" 00078 #include "../../../Utilities/Fonts/font8.c" 00079 00080 /** @addtogroup BSP 00081 * @{ 00082 */ 00083 00084 /** @addtogroup STM32746G_DISCOVERY 00085 * @{ 00086 */ 00087 00088 /** @addtogroup STM32746G_DISCOVERY_LCD 00089 * @{ 00090 */ 00091 00092 /** @defgroup STM32746G_DISCOVERY_LCD_Private_TypesDefinitions STM32746G_DISCOVERY_LCD Private Types Definitions 00093 * @{ 00094 */ 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM32746G_DISCOVERY_LCD_Private_Defines STM32746G_DISCOVERY LCD Private Defines 00100 * @{ 00101 */ 00102 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00103 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM32746G_DISCOVERY_LCD_Private_Macros STM32746G_DISCOVERY_LCD Private Macros 00109 * @{ 00110 */ 00111 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM32746G_DISCOVERY_LCD_Private_Variables STM32746G_DISCOVERY_LCD Private Variables 00117 * @{ 00118 */ 00119 LTDC_HandleTypeDef hLtdcHandler; 00120 static DMA2D_HandleTypeDef hDma2dHandler; 00121 00122 /* Default LCD configuration with LCD Layer 1 */ 00123 static uint32_t ActiveLayer = 0; 00124 static LCD_DrawPropTypeDef DrawProp[MAX_LAYER_NUMBER]; 00125 /** 00126 * @} 00127 */ 00128 00129 /** @defgroup STM32746G_DISCOVERY_LCD_Private_FunctionPrototypes STM32746G_DISCOVERY_LCD Private Function Prototypes 00130 * @{ 00131 */ 00132 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00133 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00134 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00135 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00136 /** 00137 * @} 00138 */ 00139 00140 /** @defgroup STM32746G_DISCOVERY_LCD_Exported_Functions STM32746G_DISCOVERY_LCD Exported Functions 00141 * @{ 00142 */ 00143 00144 /** 00145 * @brief Initializes the LCD. 00146 * @retval LCD state 00147 */ 00148 uint8_t BSP_LCD_Init(void) 00149 { 00150 /* Select the used LCD */ 00151 00152 /* The RK043FN48H LCD 480x272 is selected */ 00153 /* Timing Configuration */ 00154 hLtdcHandler.Init.HorizontalSync = (RK043FN48H_HSYNC - 1); 00155 hLtdcHandler.Init.VerticalSync = (RK043FN48H_VSYNC - 1); 00156 hLtdcHandler.Init.AccumulatedHBP = (RK043FN48H_HSYNC + RK043FN48H_HBP - 1); 00157 hLtdcHandler.Init.AccumulatedVBP = (RK043FN48H_VSYNC + RK043FN48H_VBP - 1); 00158 hLtdcHandler.Init.AccumulatedActiveH = (RK043FN48H_HEIGHT + RK043FN48H_VSYNC + RK043FN48H_VBP - 1); 00159 hLtdcHandler.Init.AccumulatedActiveW = (RK043FN48H_WIDTH + RK043FN48H_HSYNC + RK043FN48H_HBP - 1); 00160 hLtdcHandler.Init.TotalHeigh = (RK043FN48H_HEIGHT + RK043FN48H_VSYNC + RK043FN48H_VBP + RK043FN48H_VFP - 1); 00161 hLtdcHandler.Init.TotalWidth = (RK043FN48H_WIDTH + RK043FN48H_HSYNC + RK043FN48H_HBP + RK043FN48H_HFP - 1); 00162 00163 /* LCD clock configuration */ 00164 BSP_LCD_ClockConfig(&hLtdcHandler, NULL); 00165 00166 /* Initialize the LCD pixel width and pixel height */ 00167 hLtdcHandler.LayerCfg->ImageWidth = RK043FN48H_WIDTH; 00168 hLtdcHandler.LayerCfg->ImageHeight = RK043FN48H_HEIGHT; 00169 00170 /* Background value */ 00171 hLtdcHandler.Init.Backcolor.Blue = 0; 00172 hLtdcHandler.Init.Backcolor.Green = 0; 00173 hLtdcHandler.Init.Backcolor.Red = 0; 00174 00175 /* Polarity */ 00176 hLtdcHandler.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00177 hLtdcHandler.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00178 hLtdcHandler.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00179 hLtdcHandler.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00180 hLtdcHandler.Instance = LTDC; 00181 00182 if(HAL_LTDC_GetState(&hLtdcHandler) == HAL_LTDC_STATE_RESET) 00183 { 00184 /* Initialize the LCD Msp: this __weak function can be rewritten by the application */ 00185 BSP_LCD_MspInit(&hLtdcHandler, NULL); 00186 } 00187 HAL_LTDC_Init(&hLtdcHandler); 00188 00189 /* Assert display enable LCD_DISP pin */ 00190 HAL_GPIO_WritePin(LCD_DISP_GPIO_PORT, LCD_DISP_PIN, GPIO_PIN_SET); 00191 00192 /* Assert backlight LCD_BL_CTRL pin */ 00193 HAL_GPIO_WritePin(LCD_BL_CTRL_GPIO_PORT, LCD_BL_CTRL_PIN, GPIO_PIN_SET); 00194 00195 #if !defined(DATA_IN_ExtSDRAM) 00196 /* Initialize the SDRAM */ 00197 BSP_SDRAM_Init(); 00198 #endif 00199 00200 /* Initialize the font */ 00201 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00202 00203 return LCD_OK; 00204 } 00205 00206 /** 00207 * @brief DeInitializes the LCD. 00208 * @retval LCD state 00209 */ 00210 uint8_t BSP_LCD_DeInit(void) 00211 { 00212 /* Initialize the hLtdcHandler Instance parameter */ 00213 hLtdcHandler.Instance = LTDC; 00214 00215 /* Disable LTDC block */ 00216 __HAL_LTDC_DISABLE(&hLtdcHandler); 00217 00218 /* DeInit the LTDC */ 00219 HAL_LTDC_DeInit(&hLtdcHandler); 00220 00221 /* DeInit the LTDC MSP : this __weak function can be rewritten by the application */ 00222 BSP_LCD_MspDeInit(&hLtdcHandler, NULL); 00223 00224 return LCD_OK; 00225 } 00226 00227 /** 00228 * @brief Gets the LCD X size. 00229 * @retval Used LCD X size 00230 */ 00231 uint32_t BSP_LCD_GetXSize(void) 00232 { 00233 return hLtdcHandler.LayerCfg[ActiveLayer].ImageWidth; 00234 } 00235 00236 /** 00237 * @brief Gets the LCD Y size. 00238 * @retval Used LCD Y size 00239 */ 00240 uint32_t BSP_LCD_GetYSize(void) 00241 { 00242 return hLtdcHandler.LayerCfg[ActiveLayer].ImageHeight; 00243 } 00244 00245 /** 00246 * @brief Set the LCD X size. 00247 * @param imageWidthPixels : image width in pixels unit 00248 * @retval None 00249 */ 00250 void BSP_LCD_SetXSize(uint32_t imageWidthPixels) 00251 { 00252 hLtdcHandler.LayerCfg[ActiveLayer].ImageWidth = imageWidthPixels; 00253 } 00254 00255 /** 00256 * @brief Set the LCD Y size. 00257 * @param imageHeightPixels : image height in lines unit 00258 * @retval None 00259 */ 00260 void BSP_LCD_SetYSize(uint32_t imageHeightPixels) 00261 { 00262 hLtdcHandler.LayerCfg[ActiveLayer].ImageHeight = imageHeightPixels; 00263 } 00264 00265 /** 00266 * @brief Initializes the LCD layer in ARGB8888 format (32 bits per pixel). 00267 * @param LayerIndex: Layer foreground or background 00268 * @param FB_Address: Layer frame buffer 00269 * @retval None 00270 */ 00271 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00272 { 00273 LCD_LayerCfgTypeDef layer_cfg; 00274 00275 /* Layer Init */ 00276 layer_cfg.WindowX0 = 0; 00277 layer_cfg.WindowX1 = BSP_LCD_GetXSize(); 00278 layer_cfg.WindowY0 = 0; 00279 layer_cfg.WindowY1 = BSP_LCD_GetYSize(); 00280 layer_cfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00281 layer_cfg.FBStartAdress = FB_Address; 00282 layer_cfg.Alpha = 255; 00283 layer_cfg.Alpha0 = 0; 00284 layer_cfg.Backcolor.Blue = 0; 00285 layer_cfg.Backcolor.Green = 0; 00286 layer_cfg.Backcolor.Red = 0; 00287 layer_cfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00288 layer_cfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00289 layer_cfg.ImageWidth = BSP_LCD_GetXSize(); 00290 layer_cfg.ImageHeight = BSP_LCD_GetYSize(); 00291 00292 HAL_LTDC_ConfigLayer(&hLtdcHandler, &layer_cfg, LayerIndex); 00293 00294 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00295 DrawProp[LayerIndex].pFont = &Font24; 00296 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00297 } 00298 00299 /** 00300 * @brief Initializes the LCD layer in RGB565 format (16 bits per pixel). 00301 * @param LayerIndex: Layer foreground or background 00302 * @param FB_Address: Layer frame buffer 00303 * @retval None 00304 */ 00305 void BSP_LCD_LayerRgb565Init(uint16_t LayerIndex, uint32_t FB_Address) 00306 { 00307 LCD_LayerCfgTypeDef layer_cfg; 00308 00309 /* Layer Init */ 00310 layer_cfg.WindowX0 = 0; 00311 layer_cfg.WindowX1 = BSP_LCD_GetXSize(); 00312 layer_cfg.WindowY0 = 0; 00313 layer_cfg.WindowY1 = BSP_LCD_GetYSize(); 00314 layer_cfg.PixelFormat = LTDC_PIXEL_FORMAT_RGB565; 00315 layer_cfg.FBStartAdress = FB_Address; 00316 layer_cfg.Alpha = 255; 00317 layer_cfg.Alpha0 = 0; 00318 layer_cfg.Backcolor.Blue = 0; 00319 layer_cfg.Backcolor.Green = 0; 00320 layer_cfg.Backcolor.Red = 0; 00321 layer_cfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00322 layer_cfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00323 layer_cfg.ImageWidth = BSP_LCD_GetXSize(); 00324 layer_cfg.ImageHeight = BSP_LCD_GetYSize(); 00325 00326 HAL_LTDC_ConfigLayer(&hLtdcHandler, &layer_cfg, LayerIndex); 00327 00328 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00329 DrawProp[LayerIndex].pFont = &Font24; 00330 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00331 } 00332 00333 /** 00334 * @brief Selects the LCD Layer. 00335 * @param LayerIndex: Layer foreground or background 00336 * @retval None 00337 */ 00338 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00339 { 00340 ActiveLayer = LayerIndex; 00341 } 00342 00343 /** 00344 * @brief Sets an LCD Layer visible 00345 * @param LayerIndex: Visible Layer 00346 * @param State: New state of the specified layer 00347 * This parameter can be one of the following values: 00348 * @arg ENABLE 00349 * @arg DISABLE 00350 * @retval None 00351 */ 00352 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00353 { 00354 if(State == ENABLE) 00355 { 00356 __HAL_LTDC_LAYER_ENABLE(&hLtdcHandler, LayerIndex); 00357 } 00358 else 00359 { 00360 __HAL_LTDC_LAYER_DISABLE(&hLtdcHandler, LayerIndex); 00361 } 00362 __HAL_LTDC_RELOAD_CONFIG(&hLtdcHandler); 00363 } 00364 00365 /** 00366 * @brief Sets an LCD Layer visible without reloading. 00367 * @param LayerIndex: Visible Layer 00368 * @param State: New state of the specified layer 00369 * This parameter can be one of the following values: 00370 * @arg ENABLE 00371 * @arg DISABLE 00372 * @retval None 00373 */ 00374 void BSP_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State) 00375 { 00376 if(State == ENABLE) 00377 { 00378 __HAL_LTDC_LAYER_ENABLE(&hLtdcHandler, LayerIndex); 00379 } 00380 else 00381 { 00382 __HAL_LTDC_LAYER_DISABLE(&hLtdcHandler, LayerIndex); 00383 } 00384 /* Do not Sets the Reload */ 00385 } 00386 00387 /** 00388 * @brief Configures the transparency. 00389 * @param LayerIndex: Layer foreground or background. 00390 * @param Transparency: Transparency 00391 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00392 * @retval None 00393 */ 00394 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00395 { 00396 HAL_LTDC_SetAlpha(&hLtdcHandler, Transparency, LayerIndex); 00397 } 00398 00399 /** 00400 * @brief Configures the transparency without reloading. 00401 * @param LayerIndex: Layer foreground or background. 00402 * @param Transparency: Transparency 00403 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00404 * @retval None 00405 */ 00406 void BSP_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency) 00407 { 00408 HAL_LTDC_SetAlpha_NoReload(&hLtdcHandler, Transparency, LayerIndex); 00409 } 00410 00411 /** 00412 * @brief Sets an LCD layer frame buffer address. 00413 * @param LayerIndex: Layer foreground or background 00414 * @param Address: New LCD frame buffer value 00415 * @retval None 00416 */ 00417 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00418 { 00419 HAL_LTDC_SetAddress(&hLtdcHandler, Address, LayerIndex); 00420 } 00421 00422 /** 00423 * @brief Sets an LCD layer frame buffer address without reloading. 00424 * @param LayerIndex: Layer foreground or background 00425 * @param Address: New LCD frame buffer value 00426 * @retval None 00427 */ 00428 void BSP_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address) 00429 { 00430 HAL_LTDC_SetAddress_NoReload(&hLtdcHandler, Address, LayerIndex); 00431 } 00432 00433 /** 00434 * @brief Sets display window. 00435 * @param LayerIndex: Layer index 00436 * @param Xpos: LCD X position 00437 * @param Ypos: LCD Y position 00438 * @param Width: LCD window width 00439 * @param Height: LCD window height 00440 * @retval None 00441 */ 00442 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00443 { 00444 /* Reconfigure the layer size */ 00445 HAL_LTDC_SetWindowSize(&hLtdcHandler, Width, Height, LayerIndex); 00446 00447 /* Reconfigure the layer position */ 00448 HAL_LTDC_SetWindowPosition(&hLtdcHandler, Xpos, Ypos, LayerIndex); 00449 } 00450 00451 /** 00452 * @brief Sets display window without reloading. 00453 * @param LayerIndex: Layer index 00454 * @param Xpos: LCD X position 00455 * @param Ypos: LCD Y position 00456 * @param Width: LCD window width 00457 * @param Height: LCD window height 00458 * @retval None 00459 */ 00460 void BSP_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00461 { 00462 /* Reconfigure the layer size */ 00463 HAL_LTDC_SetWindowSize_NoReload(&hLtdcHandler, Width, Height, LayerIndex); 00464 00465 /* Reconfigure the layer position */ 00466 HAL_LTDC_SetWindowPosition_NoReload(&hLtdcHandler, Xpos, Ypos, LayerIndex); 00467 } 00468 00469 /** 00470 * @brief Configures and sets the color keying. 00471 * @param LayerIndex: Layer foreground or background 00472 * @param RGBValue: Color reference 00473 * @retval None 00474 */ 00475 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00476 { 00477 /* Configure and Enable the color Keying for LCD Layer */ 00478 HAL_LTDC_ConfigColorKeying(&hLtdcHandler, RGBValue, LayerIndex); 00479 HAL_LTDC_EnableColorKeying(&hLtdcHandler, LayerIndex); 00480 } 00481 00482 /** 00483 * @brief Configures and sets the color keying without reloading. 00484 * @param LayerIndex: Layer foreground or background 00485 * @param RGBValue: Color reference 00486 * @retval None 00487 */ 00488 void BSP_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue) 00489 { 00490 /* Configure and Enable the color Keying for LCD Layer */ 00491 HAL_LTDC_ConfigColorKeying_NoReload(&hLtdcHandler, RGBValue, LayerIndex); 00492 HAL_LTDC_EnableColorKeying_NoReload(&hLtdcHandler, LayerIndex); 00493 } 00494 00495 /** 00496 * @brief Disables the color keying. 00497 * @param LayerIndex: Layer foreground or background 00498 * @retval None 00499 */ 00500 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00501 { 00502 /* Disable the color Keying for LCD Layer */ 00503 HAL_LTDC_DisableColorKeying(&hLtdcHandler, LayerIndex); 00504 } 00505 00506 /** 00507 * @brief Disables the color keying without reloading. 00508 * @param LayerIndex: Layer foreground or background 00509 * @retval None 00510 */ 00511 void BSP_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex) 00512 { 00513 /* Disable the color Keying for LCD Layer */ 00514 HAL_LTDC_DisableColorKeying_NoReload(&hLtdcHandler, LayerIndex); 00515 } 00516 00517 /** 00518 * @brief Disables the color keying without reloading. 00519 * @param ReloadType: can be one of the following values 00520 * - LCD_RELOAD_IMMEDIATE 00521 * - LCD_RELOAD_VERTICAL_BLANKING 00522 * @retval None 00523 */ 00524 void BSP_LCD_Reload(uint32_t ReloadType) 00525 { 00526 HAL_LTDC_Reload (&hLtdcHandler, ReloadType); 00527 } 00528 00529 /** 00530 * @brief Sets the LCD text color. 00531 * @param Color: Text color code ARGB(8-8-8-8) 00532 * @retval None 00533 */ 00534 void BSP_LCD_SetTextColor(uint32_t Color) 00535 { 00536 DrawProp[ActiveLayer].TextColor = Color; 00537 } 00538 00539 /** 00540 * @brief Gets the LCD text color. 00541 * @retval Used text color. 00542 */ 00543 uint32_t BSP_LCD_GetTextColor(void) 00544 { 00545 return DrawProp[ActiveLayer].TextColor; 00546 } 00547 00548 /** 00549 * @brief Sets the LCD background color. 00550 * @param Color: Layer background color code ARGB(8-8-8-8) 00551 * @retval None 00552 */ 00553 void BSP_LCD_SetBackColor(uint32_t Color) 00554 { 00555 DrawProp[ActiveLayer].BackColor = Color; 00556 } 00557 00558 /** 00559 * @brief Gets the LCD background color. 00560 * @retval Used background colour 00561 */ 00562 uint32_t BSP_LCD_GetBackColor(void) 00563 { 00564 return DrawProp[ActiveLayer].BackColor; 00565 } 00566 00567 /** 00568 * @brief Sets the LCD text font. 00569 * @param fonts: Layer font to be used 00570 * @retval None 00571 */ 00572 void BSP_LCD_SetFont(sFONT *fonts) 00573 { 00574 DrawProp[ActiveLayer].pFont = fonts; 00575 } 00576 00577 /** 00578 * @brief Gets the LCD text font. 00579 * @retval Used layer font 00580 */ 00581 sFONT *BSP_LCD_GetFont(void) 00582 { 00583 return DrawProp[ActiveLayer].pFont; 00584 } 00585 00586 /** 00587 * @brief Reads an LCD pixel. 00588 * @param Xpos: X position 00589 * @param Ypos: Y position 00590 * @retval RGB pixel color 00591 */ 00592 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00593 { 00594 uint32_t ret = 0; 00595 00596 if(hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00597 { 00598 /* Read data value from SDRAM memory */ 00599 ret = *(__IO uint32_t*) (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00600 } 00601 else if(hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00602 { 00603 /* Read data value from SDRAM memory */ 00604 ret = (*(__IO uint32_t*) (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00605 } 00606 else if((hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00607 (hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00608 (hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00609 { 00610 /* Read data value from SDRAM memory */ 00611 ret = *(__IO uint16_t*) (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00612 } 00613 else 00614 { 00615 /* Read data value from SDRAM memory */ 00616 ret = *(__IO uint8_t*) (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00617 } 00618 00619 return ret; 00620 } 00621 00622 /** 00623 * @brief Clears the hole LCD. 00624 * @param Color: Color of the background 00625 * @retval None 00626 */ 00627 void BSP_LCD_Clear(uint32_t Color) 00628 { 00629 /* Clear the LCD */ 00630 LL_FillBuffer(ActiveLayer, (uint32_t *)(hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00631 } 00632 00633 /** 00634 * @brief Clears the selected line. 00635 * @param Line: Line to be cleared 00636 * @retval None 00637 */ 00638 void BSP_LCD_ClearStringLine(uint32_t Line) 00639 { 00640 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00641 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00642 00643 /* Draw rectangle with background color */ 00644 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00645 00646 DrawProp[ActiveLayer].TextColor = color_backup; 00647 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00648 } 00649 00650 /** 00651 * @brief Displays one character. 00652 * @param Xpos: Start column address 00653 * @param Ypos: Line where to display the character shape. 00654 * @param Ascii: Character ascii code 00655 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00656 * @retval None 00657 */ 00658 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00659 { 00660 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00661 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00662 } 00663 00664 /** 00665 * @brief Displays characters on the LCD. 00666 * @param Xpos: X position (in pixel) 00667 * @param Ypos: Y position (in pixel) 00668 * @param Text: Pointer to string to display on LCD 00669 * @param Mode: Display mode 00670 * This parameter can be one of the following values: 00671 * @arg CENTER_MODE 00672 * @arg RIGHT_MODE 00673 * @arg LEFT_MODE 00674 * @retval None 00675 */ 00676 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00677 { 00678 uint16_t ref_column = 1, i = 0; 00679 uint32_t size = 0, xsize = 0; 00680 uint8_t *ptr = Text; 00681 00682 /* Get the text size */ 00683 while (*ptr++) size ++ ; 00684 00685 /* Characters number per line */ 00686 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00687 00688 switch (Mode) 00689 { 00690 case CENTER_MODE: 00691 { 00692 ref_column = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00693 break; 00694 } 00695 case LEFT_MODE: 00696 { 00697 ref_column = Xpos; 00698 break; 00699 } 00700 case RIGHT_MODE: 00701 { 00702 ref_column = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00703 break; 00704 } 00705 default: 00706 { 00707 ref_column = Xpos; 00708 break; 00709 } 00710 } 00711 00712 /* Check that the Start column is located in the screen */ 00713 if ((ref_column < 1) || (ref_column >= 0x8000)) 00714 { 00715 ref_column = 1; 00716 } 00717 00718 /* Send the string character by character on LCD */ 00719 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00720 { 00721 /* Display one character on LCD */ 00722 BSP_LCD_DisplayChar(ref_column, Ypos, *Text); 00723 /* Decrement the column position by 16 */ 00724 ref_column += DrawProp[ActiveLayer].pFont->Width; 00725 /* Point on the next character */ 00726 Text++; 00727 i++; 00728 } 00729 } 00730 00731 /** 00732 * @brief Displays a maximum of 60 characters on the LCD. 00733 * @param Line: Line where to display the character shape 00734 * @param ptr: Pointer to string to display on LCD 00735 * @retval None 00736 */ 00737 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00738 { 00739 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00740 } 00741 00742 /** 00743 * @brief Draws an horizontal line. 00744 * @param Xpos: X position 00745 * @param Ypos: Y position 00746 * @param Length: Line length 00747 * @retval None 00748 */ 00749 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00750 { 00751 uint32_t Xaddress = 0; 00752 00753 /* Get the line address */ 00754 if(hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 00755 { /* RGB565 format */ 00756 Xaddress = (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 2*(BSP_LCD_GetXSize()*Ypos + Xpos); 00757 } 00758 else 00759 { /* ARGB8888 format */ 00760 Xaddress = (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00761 } 00762 00763 /* Write line */ 00764 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00765 } 00766 00767 /** 00768 * @brief Draws a vertical line. 00769 * @param Xpos: X position 00770 * @param Ypos: Y position 00771 * @param Length: Line length 00772 * @retval None 00773 */ 00774 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00775 { 00776 uint32_t Xaddress = 0; 00777 00778 /* Get the line address */ 00779 if(hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 00780 { /* RGB565 format */ 00781 Xaddress = (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 2*(BSP_LCD_GetXSize()*Ypos + Xpos); 00782 } 00783 else 00784 { /* ARGB8888 format */ 00785 Xaddress = (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00786 } 00787 00788 /* Write line */ 00789 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00790 } 00791 00792 /** 00793 * @brief Draws an uni-line (between two points). 00794 * @param x1: Point 1 X position 00795 * @param y1: Point 1 Y position 00796 * @param x2: Point 2 X position 00797 * @param y2: Point 2 Y position 00798 * @retval None 00799 */ 00800 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00801 { 00802 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00803 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 00804 curpixel = 0; 00805 00806 deltax = ABS(x2 - x1); /* The difference between the x's */ 00807 deltay = ABS(y2 - y1); /* The difference between the y's */ 00808 x = x1; /* Start x off at the first pixel */ 00809 y = y1; /* Start y off at the first pixel */ 00810 00811 if (x2 >= x1) /* The x-values are increasing */ 00812 { 00813 xinc1 = 1; 00814 xinc2 = 1; 00815 } 00816 else /* The x-values are decreasing */ 00817 { 00818 xinc1 = -1; 00819 xinc2 = -1; 00820 } 00821 00822 if (y2 >= y1) /* The y-values are increasing */ 00823 { 00824 yinc1 = 1; 00825 yinc2 = 1; 00826 } 00827 else /* The y-values are decreasing */ 00828 { 00829 yinc1 = -1; 00830 yinc2 = -1; 00831 } 00832 00833 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00834 { 00835 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00836 yinc2 = 0; /* Don't change the y for every iteration */ 00837 den = deltax; 00838 num = deltax / 2; 00839 num_add = deltay; 00840 num_pixels = deltax; /* There are more x-values than y-values */ 00841 } 00842 else /* There is at least one y-value for every x-value */ 00843 { 00844 xinc2 = 0; /* Don't change the x for every iteration */ 00845 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00846 den = deltay; 00847 num = deltay / 2; 00848 num_add = deltax; 00849 num_pixels = deltay; /* There are more y-values than x-values */ 00850 } 00851 00852 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 00853 { 00854 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00855 num += num_add; /* Increase the numerator by the top of the fraction */ 00856 if (num >= den) /* Check if numerator >= denominator */ 00857 { 00858 num -= den; /* Calculate the new numerator value */ 00859 x += xinc1; /* Change the x as appropriate */ 00860 y += yinc1; /* Change the y as appropriate */ 00861 } 00862 x += xinc2; /* Change the x as appropriate */ 00863 y += yinc2; /* Change the y as appropriate */ 00864 } 00865 } 00866 00867 /** 00868 * @brief Draws a rectangle. 00869 * @param Xpos: X position 00870 * @param Ypos: Y position 00871 * @param Width: Rectangle width 00872 * @param Height: Rectangle height 00873 * @retval None 00874 */ 00875 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00876 { 00877 /* Draw horizontal lines */ 00878 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00879 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00880 00881 /* Draw vertical lines */ 00882 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00883 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00884 } 00885 00886 /** 00887 * @brief Draws a circle. 00888 * @param Xpos: X position 00889 * @param Ypos: Y position 00890 * @param Radius: Circle radius 00891 * @retval None 00892 */ 00893 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00894 { 00895 int32_t decision; /* Decision Variable */ 00896 uint32_t current_x; /* Current X Value */ 00897 uint32_t current_y; /* Current Y Value */ 00898 00899 decision = 3 - (Radius << 1); 00900 current_x = 0; 00901 current_y = Radius; 00902 00903 while (current_x <= current_y) 00904 { 00905 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00906 00907 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00908 00909 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00910 00911 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00912 00913 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00914 00915 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00916 00917 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00918 00919 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00920 00921 if (decision < 0) 00922 { 00923 decision += (current_x << 2) + 6; 00924 } 00925 else 00926 { 00927 decision += ((current_x - current_y) << 2) + 10; 00928 current_y--; 00929 } 00930 current_x++; 00931 } 00932 } 00933 00934 /** 00935 * @brief Draws an poly-line (between many points). 00936 * @param Points: Pointer to the points array 00937 * @param PointCount: Number of points 00938 * @retval None 00939 */ 00940 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00941 { 00942 int16_t x = 0, y = 0; 00943 00944 if(PointCount < 2) 00945 { 00946 return; 00947 } 00948 00949 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00950 00951 while(--PointCount) 00952 { 00953 x = Points->X; 00954 y = Points->Y; 00955 Points++; 00956 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00957 } 00958 } 00959 00960 /** 00961 * @brief Draws an ellipse on LCD. 00962 * @param Xpos: X position 00963 * @param Ypos: Y position 00964 * @param XRadius: Ellipse X radius 00965 * @param YRadius: Ellipse Y radius 00966 * @retval None 00967 */ 00968 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00969 { 00970 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00971 float k = 0, rad1 = 0, rad2 = 0; 00972 00973 rad1 = XRadius; 00974 rad2 = YRadius; 00975 00976 k = (float)(rad2/rad1); 00977 00978 do { 00979 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00980 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00981 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00982 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00983 00984 e2 = err; 00985 if (e2 <= x) { 00986 err += ++x*2+1; 00987 if (-y == x && e2 <= y) e2 = 0; 00988 } 00989 if (e2 > y) err += ++y*2+1; 00990 } 00991 while (y <= 0); 00992 } 00993 00994 /** 00995 * @brief Draws a pixel on LCD. 00996 * @param Xpos: X position 00997 * @param Ypos: Y position 00998 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 00999 * @retval None 01000 */ 01001 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01002 { 01003 /* Write data value to all SDRAM memory */ 01004 if(hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 01005 { /* RGB565 format */ 01006 *(__IO uint16_t*) (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))) = (uint16_t)RGB_Code; 01007 } 01008 else 01009 { /* ARGB8888 format */ 01010 *(__IO uint32_t*) (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 01011 } 01012 } 01013 01014 /** 01015 * @brief Draws a bitmap picture loaded in the internal Flash in ARGB888 format (32 bits per pixel). 01016 * @param Xpos: Bmp X position in the LCD 01017 * @param Ypos: Bmp Y position in the LCD 01018 * @param pbmp: Pointer to Bmp picture address in the internal Flash 01019 * @retval None 01020 */ 01021 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 01022 { 01023 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 01024 uint32_t address; 01025 uint32_t input_color_mode = 0; 01026 01027 /* Get bitmap data address offset */ 01028 index = *(__IO uint16_t *) (pbmp + 10); 01029 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 01030 01031 /* Read bitmap width */ 01032 width = *(uint16_t *) (pbmp + 18); 01033 width |= (*(uint16_t *) (pbmp + 20)) << 16; 01034 01035 /* Read bitmap height */ 01036 height = *(uint16_t *) (pbmp + 22); 01037 height |= (*(uint16_t *) (pbmp + 24)) << 16; 01038 01039 /* Read bit/pixel */ 01040 bit_pixel = *(uint16_t *) (pbmp + 28); 01041 01042 /* Set the address */ 01043 address = hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Ypos) + Xpos)*(4)); 01044 01045 /* Get the layer pixel format */ 01046 if ((bit_pixel/8) == 4) 01047 { 01048 input_color_mode = CM_ARGB8888; 01049 } 01050 else if ((bit_pixel/8) == 2) 01051 { 01052 input_color_mode = CM_RGB565; 01053 } 01054 else 01055 { 01056 input_color_mode = CM_RGB888; 01057 } 01058 01059 /* Bypass the bitmap header */ 01060 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 01061 01062 /* Convert picture to ARGB8888 pixel format */ 01063 for(index=0; index < height; index++) 01064 { 01065 /* Pixel format conversion */ 01066 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)address, width, input_color_mode); 01067 01068 /* Increment the source and destination buffers */ 01069 address+= (BSP_LCD_GetXSize()*4); 01070 pbmp -= width*(bit_pixel/8); 01071 } 01072 } 01073 01074 /** 01075 * @brief Draws a full rectangle. 01076 * @param Xpos: X position 01077 * @param Ypos: Y position 01078 * @param Width: Rectangle width 01079 * @param Height: Rectangle height 01080 * @retval None 01081 */ 01082 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01083 { 01084 uint32_t x_address = 0; 01085 01086 /* Set the text color */ 01087 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01088 01089 /* Get the rectangle start address */ 01090 if(hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 01091 { /* RGB565 format */ 01092 x_address = (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 2*(BSP_LCD_GetXSize()*Ypos + Xpos); 01093 } 01094 else 01095 { /* ARGB8888 format */ 01096 x_address = (hLtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 01097 } 01098 /* Fill the rectangle */ 01099 LL_FillBuffer(ActiveLayer, (uint32_t *)x_address, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 01100 } 01101 01102 /** 01103 * @brief Draws a full circle. 01104 * @param Xpos: X position 01105 * @param Ypos: Y position 01106 * @param Radius: Circle radius 01107 * @retval None 01108 */ 01109 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 01110 { 01111 int32_t decision; /* Decision Variable */ 01112 uint32_t current_x; /* Current X Value */ 01113 uint32_t current_y; /* Current Y Value */ 01114 01115 decision = 3 - (Radius << 1); 01116 01117 current_x = 0; 01118 current_y = Radius; 01119 01120 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01121 01122 while (current_x <= current_y) 01123 { 01124 if(current_y > 0) 01125 { 01126 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 01127 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 01128 } 01129 01130 if(current_x > 0) 01131 { 01132 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 01133 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 01134 } 01135 if (decision < 0) 01136 { 01137 decision += (current_x << 2) + 6; 01138 } 01139 else 01140 { 01141 decision += ((current_x - current_y) << 2) + 10; 01142 current_y--; 01143 } 01144 current_x++; 01145 } 01146 01147 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01148 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 01149 } 01150 01151 /** 01152 * @brief Draws a full poly-line (between many points). 01153 * @param Points: Pointer to the points array 01154 * @param PointCount: Number of points 01155 * @retval None 01156 */ 01157 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01158 { 01159 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 01160 uint16_t image_left = 0, image_right = 0, image_top = 0, image_bottom = 0; 01161 01162 image_left = image_right = Points->X; 01163 image_top= image_bottom = Points->Y; 01164 01165 for(counter = 1; counter < PointCount; counter++) 01166 { 01167 pixelX = POLY_X(counter); 01168 if(pixelX < image_left) 01169 { 01170 image_left = pixelX; 01171 } 01172 if(pixelX > image_right) 01173 { 01174 image_right = pixelX; 01175 } 01176 01177 pixelY = POLY_Y(counter); 01178 if(pixelY < image_top) 01179 { 01180 image_top = pixelY; 01181 } 01182 if(pixelY > image_bottom) 01183 { 01184 image_bottom = pixelY; 01185 } 01186 } 01187 01188 if(PointCount < 2) 01189 { 01190 return; 01191 } 01192 01193 X_center = (image_left + image_right)/2; 01194 Y_center = (image_bottom + image_top)/2; 01195 01196 X_first = Points->X; 01197 Y_first = Points->Y; 01198 01199 while(--PointCount) 01200 { 01201 X = Points->X; 01202 Y = Points->Y; 01203 Points++; 01204 X2 = Points->X; 01205 Y2 = Points->Y; 01206 01207 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 01208 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 01209 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 01210 } 01211 01212 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 01213 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 01214 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 01215 } 01216 01217 /** 01218 * @brief Draws a full ellipse. 01219 * @param Xpos: X position 01220 * @param Ypos: Y position 01221 * @param XRadius: Ellipse X radius 01222 * @param YRadius: Ellipse Y radius 01223 * @retval None 01224 */ 01225 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01226 { 01227 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01228 float k = 0, rad1 = 0, rad2 = 0; 01229 01230 rad1 = XRadius; 01231 rad2 = YRadius; 01232 01233 k = (float)(rad2/rad1); 01234 01235 do 01236 { 01237 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 01238 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 01239 01240 e2 = err; 01241 if (e2 <= x) 01242 { 01243 err += ++x*2+1; 01244 if (-y == x && e2 <= y) e2 = 0; 01245 } 01246 if (e2 > y) err += ++y*2+1; 01247 } 01248 while (y <= 0); 01249 } 01250 01251 /** 01252 * @brief Enables the display. 01253 * @retval None 01254 */ 01255 void BSP_LCD_DisplayOn(void) 01256 { 01257 /* Display On */ 01258 __HAL_LTDC_ENABLE(&hLtdcHandler); 01259 HAL_GPIO_WritePin(LCD_DISP_GPIO_PORT, LCD_DISP_PIN, GPIO_PIN_SET); /* Assert LCD_DISP pin */ 01260 HAL_GPIO_WritePin(LCD_BL_CTRL_GPIO_PORT, LCD_BL_CTRL_PIN, GPIO_PIN_SET); /* Assert LCD_BL_CTRL pin */ 01261 } 01262 01263 /** 01264 * @brief Disables the display. 01265 * @retval None 01266 */ 01267 void BSP_LCD_DisplayOff(void) 01268 { 01269 /* Display Off */ 01270 __HAL_LTDC_DISABLE(&hLtdcHandler); 01271 HAL_GPIO_WritePin(LCD_DISP_GPIO_PORT, LCD_DISP_PIN, GPIO_PIN_RESET); /* De-assert LCD_DISP pin */ 01272 HAL_GPIO_WritePin(LCD_BL_CTRL_GPIO_PORT, LCD_BL_CTRL_PIN, GPIO_PIN_RESET);/* De-assert LCD_BL_CTRL pin */ 01273 } 01274 01275 /** 01276 * @brief Initializes the LTDC MSP. 01277 * @param hltdc: LTDC handle 01278 * @param Params 01279 * @retval None 01280 */ 01281 __weak void BSP_LCD_MspInit(LTDC_HandleTypeDef *hltdc, void *Params) 01282 { 01283 GPIO_InitTypeDef gpio_init_structure; 01284 01285 /* Enable the LTDC and DMA2D clocks */ 01286 __HAL_RCC_LTDC_CLK_ENABLE(); 01287 __HAL_RCC_DMA2D_CLK_ENABLE(); 01288 01289 /* Enable GPIOs clock */ 01290 __HAL_RCC_GPIOE_CLK_ENABLE(); 01291 __HAL_RCC_GPIOG_CLK_ENABLE(); 01292 __HAL_RCC_GPIOI_CLK_ENABLE(); 01293 __HAL_RCC_GPIOJ_CLK_ENABLE(); 01294 __HAL_RCC_GPIOK_CLK_ENABLE(); 01295 LCD_DISP_GPIO_CLK_ENABLE(); 01296 LCD_BL_CTRL_GPIO_CLK_ENABLE(); 01297 01298 /*** LTDC Pins configuration ***/ 01299 /* GPIOE configuration */ 01300 gpio_init_structure.Pin = GPIO_PIN_4; 01301 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01302 gpio_init_structure.Pull = GPIO_NOPULL; 01303 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01304 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01305 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 01306 01307 /* GPIOG configuration */ 01308 gpio_init_structure.Pin = GPIO_PIN_12; 01309 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01310 gpio_init_structure.Alternate = GPIO_AF9_LTDC; 01311 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 01312 01313 /* GPIOI LTDC alternate configuration */ 01314 gpio_init_structure.Pin = GPIO_PIN_9 | GPIO_PIN_10 | \ 01315 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01316 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01317 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01318 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 01319 01320 /* GPIOJ configuration */ 01321 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01322 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01323 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01324 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01325 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01326 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01327 HAL_GPIO_Init(GPIOJ, &gpio_init_structure); 01328 01329 /* GPIOK configuration */ 01330 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_4 | \ 01331 GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01332 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01333 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01334 HAL_GPIO_Init(GPIOK, &gpio_init_structure); 01335 01336 /* LCD_DISP GPIO configuration */ 01337 gpio_init_structure.Pin = LCD_DISP_PIN; /* LCD_DISP pin has to be manually controlled */ 01338 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 01339 HAL_GPIO_Init(LCD_DISP_GPIO_PORT, &gpio_init_structure); 01340 01341 /* LCD_BL_CTRL GPIO configuration */ 01342 gpio_init_structure.Pin = LCD_BL_CTRL_PIN; /* LCD_BL_CTRL pin has to be manually controlled */ 01343 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 01344 HAL_GPIO_Init(LCD_BL_CTRL_GPIO_PORT, &gpio_init_structure); 01345 } 01346 01347 /** 01348 * @brief DeInitializes BSP_LCD MSP. 01349 * @param hltdc: LTDC handle 01350 * @param Params 01351 * @retval None 01352 */ 01353 __weak void BSP_LCD_MspDeInit(LTDC_HandleTypeDef *hltdc, void *Params) 01354 { 01355 GPIO_InitTypeDef gpio_init_structure; 01356 01357 /* Disable LTDC block */ 01358 __HAL_LTDC_DISABLE(hltdc); 01359 01360 /* LTDC Pins deactivation */ 01361 01362 /* GPIOE deactivation */ 01363 gpio_init_structure.Pin = GPIO_PIN_4; 01364 HAL_GPIO_DeInit(GPIOE, gpio_init_structure.Pin); 01365 01366 /* GPIOG deactivation */ 01367 gpio_init_structure.Pin = GPIO_PIN_12; 01368 HAL_GPIO_DeInit(GPIOG, gpio_init_structure.Pin); 01369 01370 /* GPIOI deactivation */ 01371 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_12 | \ 01372 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01373 HAL_GPIO_DeInit(GPIOI, gpio_init_structure.Pin); 01374 01375 /* GPIOJ deactivation */ 01376 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01377 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01378 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01379 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01380 HAL_GPIO_DeInit(GPIOJ, gpio_init_structure.Pin); 01381 01382 /* GPIOK deactivation */ 01383 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_4 | \ 01384 GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01385 HAL_GPIO_DeInit(GPIOK, gpio_init_structure.Pin); 01386 01387 /* Disable LTDC clock */ 01388 __HAL_RCC_LTDC_CLK_DISABLE(); 01389 01390 /* GPIO pins clock can be shut down in the application 01391 by surcharging this __weak function */ 01392 } 01393 01394 /** 01395 * @brief Clock Config. 01396 * @param hltdc: LTDC handle 01397 * @param Params 01398 * @note This API is called by BSP_LCD_Init() 01399 * Being __weak it can be overwritten by the application 01400 * @retval None 01401 */ 01402 __weak void BSP_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params) 01403 { 01404 static RCC_PeriphCLKInitTypeDef periph_clk_init_struct; 01405 01406 /* RK043FN48H LCD clock configuration */ 01407 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01408 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 192 Mhz */ 01409 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 192/5 = 38.4 Mhz */ 01410 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_4 = 38.4/4 = 9.6Mhz */ 01411 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01412 periph_clk_init_struct.PLLSAI.PLLSAIN = 192; 01413 periph_clk_init_struct.PLLSAI.PLLSAIR = RK043FN48H_FREQUENCY_DIVIDER; 01414 periph_clk_init_struct.PLLSAIDivR = RCC_PLLSAIDIVR_4; 01415 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01416 } 01417 01418 01419 /******************************************************************************* 01420 Static Functions 01421 *******************************************************************************/ 01422 01423 /** 01424 * @brief Draws a character on LCD. 01425 * @param Xpos: Line where to display the character shape 01426 * @param Ypos: Start column address 01427 * @param c: Pointer to the character data 01428 * @retval None 01429 */ 01430 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01431 { 01432 uint32_t i = 0, j = 0; 01433 uint16_t height, width; 01434 uint8_t offset; 01435 uint8_t *pchar; 01436 uint32_t line; 01437 01438 height = DrawProp[ActiveLayer].pFont->Height; 01439 width = DrawProp[ActiveLayer].pFont->Width; 01440 01441 offset = 8 *((width + 7)/8) - width ; 01442 01443 for(i = 0; i < height; i++) 01444 { 01445 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01446 01447 switch(((width + 7)/8)) 01448 { 01449 01450 case 1: 01451 line = pchar[0]; 01452 break; 01453 01454 case 2: 01455 line = (pchar[0]<< 8) | pchar[1]; 01456 break; 01457 01458 case 3: 01459 default: 01460 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01461 break; 01462 } 01463 01464 for (j = 0; j < width; j++) 01465 { 01466 if(line & (1 << (width- j + offset- 1))) 01467 { 01468 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01469 } 01470 else 01471 { 01472 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01473 } 01474 } 01475 Ypos++; 01476 } 01477 } 01478 01479 /** 01480 * @brief Fills a triangle (between 3 points). 01481 * @param x1: Point 1 X position 01482 * @param y1: Point 1 Y position 01483 * @param x2: Point 2 X position 01484 * @param y2: Point 2 Y position 01485 * @param x3: Point 3 X position 01486 * @param y3: Point 3 Y position 01487 * @retval None 01488 */ 01489 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01490 { 01491 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01492 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 01493 curpixel = 0; 01494 01495 deltax = ABS(x2 - x1); /* The difference between the x's */ 01496 deltay = ABS(y2 - y1); /* The difference between the y's */ 01497 x = x1; /* Start x off at the first pixel */ 01498 y = y1; /* Start y off at the first pixel */ 01499 01500 if (x2 >= x1) /* The x-values are increasing */ 01501 { 01502 xinc1 = 1; 01503 xinc2 = 1; 01504 } 01505 else /* The x-values are decreasing */ 01506 { 01507 xinc1 = -1; 01508 xinc2 = -1; 01509 } 01510 01511 if (y2 >= y1) /* The y-values are increasing */ 01512 { 01513 yinc1 = 1; 01514 yinc2 = 1; 01515 } 01516 else /* The y-values are decreasing */ 01517 { 01518 yinc1 = -1; 01519 yinc2 = -1; 01520 } 01521 01522 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01523 { 01524 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01525 yinc2 = 0; /* Don't change the y for every iteration */ 01526 den = deltax; 01527 num = deltax / 2; 01528 num_add = deltay; 01529 num_pixels = deltax; /* There are more x-values than y-values */ 01530 } 01531 else /* There is at least one y-value for every x-value */ 01532 { 01533 xinc2 = 0; /* Don't change the x for every iteration */ 01534 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01535 den = deltay; 01536 num = deltay / 2; 01537 num_add = deltax; 01538 num_pixels = deltay; /* There are more y-values than x-values */ 01539 } 01540 01541 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 01542 { 01543 BSP_LCD_DrawLine(x, y, x3, y3); 01544 01545 num += num_add; /* Increase the numerator by the top of the fraction */ 01546 if (num >= den) /* Check if numerator >= denominator */ 01547 { 01548 num -= den; /* Calculate the new numerator value */ 01549 x += xinc1; /* Change the x as appropriate */ 01550 y += yinc1; /* Change the y as appropriate */ 01551 } 01552 x += xinc2; /* Change the x as appropriate */ 01553 y += yinc2; /* Change the y as appropriate */ 01554 } 01555 } 01556 01557 /** 01558 * @brief Fills a buffer. 01559 * @param LayerIndex: Layer index 01560 * @param pDst: Pointer to destination buffer 01561 * @param xSize: Buffer width 01562 * @param ySize: Buffer height 01563 * @param OffLine: Offset 01564 * @param ColorIndex: Color index 01565 * @retval None 01566 */ 01567 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01568 { 01569 /* Register to memory mode with ARGB8888 as color Mode */ 01570 hDma2dHandler.Init.Mode = DMA2D_R2M; 01571 if(hLtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) 01572 { /* RGB565 format */ 01573 hDma2dHandler.Init.ColorMode = DMA2D_RGB565; 01574 } 01575 else 01576 { /* ARGB8888 format */ 01577 hDma2dHandler.Init.ColorMode = DMA2D_ARGB8888; 01578 } 01579 hDma2dHandler.Init.OutputOffset = OffLine; 01580 01581 hDma2dHandler.Instance = DMA2D; 01582 01583 /* DMA2D Initialization */ 01584 if(HAL_DMA2D_Init(&hDma2dHandler) == HAL_OK) 01585 { 01586 if(HAL_DMA2D_ConfigLayer(&hDma2dHandler, LayerIndex) == HAL_OK) 01587 { 01588 if (HAL_DMA2D_Start(&hDma2dHandler, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01589 { 01590 /* Polling For DMA transfer */ 01591 HAL_DMA2D_PollForTransfer(&hDma2dHandler, 10); 01592 } 01593 } 01594 } 01595 } 01596 01597 /** 01598 * @brief Converts a line to an ARGB8888 pixel format. 01599 * @param pSrc: Pointer to source buffer 01600 * @param pDst: Output color 01601 * @param xSize: Buffer width 01602 * @param ColorMode: Input color mode 01603 * @retval None 01604 */ 01605 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01606 { 01607 /* Configure the DMA2D Mode, Color Mode and output offset */ 01608 hDma2dHandler.Init.Mode = DMA2D_M2M_PFC; 01609 hDma2dHandler.Init.ColorMode = DMA2D_ARGB8888; 01610 hDma2dHandler.Init.OutputOffset = 0; 01611 01612 /* Foreground Configuration */ 01613 hDma2dHandler.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01614 hDma2dHandler.LayerCfg[1].InputAlpha = 0xFF; 01615 hDma2dHandler.LayerCfg[1].InputColorMode = ColorMode; 01616 hDma2dHandler.LayerCfg[1].InputOffset = 0; 01617 01618 hDma2dHandler.Instance = DMA2D; 01619 01620 /* DMA2D Initialization */ 01621 if(HAL_DMA2D_Init(&hDma2dHandler) == HAL_OK) 01622 { 01623 if(HAL_DMA2D_ConfigLayer(&hDma2dHandler, 1) == HAL_OK) 01624 { 01625 if (HAL_DMA2D_Start(&hDma2dHandler, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01626 { 01627 /* Polling For DMA transfer */ 01628 HAL_DMA2D_PollForTransfer(&hDma2dHandler, 10); 01629 } 01630 } 01631 } 01632 } 01633 01634 /** 01635 * @} 01636 */ 01637 01638 /** 01639 * @} 01640 */ 01641 01642 /** 01643 * @} 01644 */ 01645 01646 /** 01647 * @} 01648 */ 01649 01650 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 16:31:33 for STM32746G-Discovery BSP User Manual by
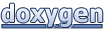