STM32746G-Discovery BSP User Manual
|
stm32746g_discovery_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32746g_discovery_camera.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file includes the driver for Camera modules mounted on 00008 * STM32746G-Discovery board. 00009 @verbatim 00010 How to use this driver: 00011 ------------------------ 00012 - This driver is used to drive the camera. 00013 - The OV9655 component driver MUST be included with this driver. 00014 00015 Driver description: 00016 ------------------- 00017 + Initialization steps: 00018 o Initialize the camera using the BSP_CAMERA_Init() function. 00019 o Start the camera capture/snapshot using the CAMERA_Start() function. 00020 o Suspend, resume or stop the camera capture using the following functions: 00021 - BSP_CAMERA_Suspend() 00022 - BSP_CAMERA_Resume() 00023 - BSP_CAMERA_Stop() 00024 00025 + Options 00026 o Increase or decrease on the fly the brightness and/or contrast 00027 using the following function: 00028 - BSP_CAMERA_ContrastBrightnessConfig 00029 o Add a special effect on the fly using the following functions: 00030 - BSP_CAMERA_BlackWhiteConfig() 00031 - BSP_CAMERA_ColorEffectConfig() 00032 @endverbatim 00033 ****************************************************************************** 00034 * @attention 00035 * 00036 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00037 * 00038 * Redistribution and use in source and binary forms, with or without modification, 00039 * are permitted provided that the following conditions are met: 00040 * 1. Redistributions of source code must retain the above copyright notice, 00041 * this list of conditions and the following disclaimer. 00042 * 2. Redistributions in binary form must reproduce the above copyright notice, 00043 * this list of conditions and the following disclaimer in the documentation 00044 * and/or other materials provided with the distribution. 00045 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00046 * may be used to endorse or promote products derived from this software 00047 * without specific prior written permission. 00048 * 00049 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00050 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00051 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00052 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00053 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00054 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00055 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00056 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00057 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00058 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00059 * 00060 ****************************************************************************** 00061 */ 00062 00063 /* Includes ------------------------------------------------------------------*/ 00064 #include "stm32746g_discovery_camera.h" 00065 #include "stm32746g_discovery.h" 00066 00067 /** @addtogroup BSP 00068 * @{ 00069 */ 00070 00071 /** @addtogroup STM32746G_DISCOVERY 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM32746G_DISCOVERY_CAMERA 00076 * @{ 00077 */ 00078 00079 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_TypesDefinitions STM32746G_DISCOVERY_CAMERA Private Types Definitions 00080 * @{ 00081 */ 00082 /** 00083 * @} 00084 */ 00085 00086 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_Defines STM32746G_DISCOVERY_CAMERA Private Defines 00087 * @{ 00088 */ 00089 #define CAMERA_VGA_RES_X 640 00090 #define CAMERA_VGA_RES_Y 480 00091 #define CAMERA_480x272_RES_X 480 00092 #define CAMERA_480x272_RES_Y 272 00093 #define CAMERA_QVGA_RES_X 320 00094 #define CAMERA_QVGA_RES_Y 240 00095 #define CAMERA_QQVGA_RES_X 160 00096 #define CAMERA_QQVGA_RES_Y 120 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_Macros STM32746G_DISCOVERY_CAMERA Private Macros 00102 * @{ 00103 */ 00104 /** 00105 * @} 00106 */ 00107 00108 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_Variables STM32746G_DISCOVERY_CAMERA Private Variables 00109 * @{ 00110 */ 00111 DCMI_HandleTypeDef hDcmiHandler; 00112 CAMERA_DrvTypeDef *camera_drv; 00113 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00114 static uint32_t CameraCurrentResolution; 00115 00116 /* Camera module I2C HW address */ 00117 static uint32_t CameraHwAddress; 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32746G_DISCOVERY_CAMERA_Private_FunctionPrototypes STM32746G_DISCOVERY_CAMERA Private Function Prototypes 00123 * @{ 00124 */ 00125 static uint32_t GetSize(uint32_t resolution); 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32746G_DISCOVERY_CAMERA_Exported_Functions STM32746G_DISCOVERY_CAMERA Exported Functions 00131 * @{ 00132 */ 00133 00134 /** 00135 * @brief Initializes the camera. 00136 * @param Resolution : camera sensor requested resolution (x, y) : standard resolution 00137 * naming QQVGA, QVGA, VGA ... 00138 * @retval Camera status 00139 */ 00140 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00141 { 00142 DCMI_HandleTypeDef *phdcmi; 00143 uint8_t status = CAMERA_ERROR; 00144 00145 /* Get the DCMI handle structure */ 00146 phdcmi = &hDcmiHandler; 00147 00148 /*** Configures the DCMI to interface with the camera module ***/ 00149 /* DCMI configuration */ 00150 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00151 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_LOW; 00152 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00153 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00154 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00155 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00156 phdcmi->Instance = DCMI; 00157 00158 /* Power up camera */ 00159 BSP_CAMERA_PwrUp(); 00160 00161 /* Read ID of Camera module via I2C */ 00162 if(ov9655_ReadID(CAMERA_I2C_ADDRESS) == OV9655_ID) 00163 { 00164 /* Initialize the camera driver structure */ 00165 camera_drv = &ov9655_drv; 00166 CameraHwAddress = CAMERA_I2C_ADDRESS; 00167 00168 /* DCMI Initialization */ 00169 BSP_CAMERA_MspInit(&hDcmiHandler, NULL); 00170 HAL_DCMI_Init(phdcmi); 00171 00172 /* Camera Module Initialization via I2C to the wanted 'Resolution' */ 00173 if (Resolution == CAMERA_R480x272) 00174 { /* For 480x272 resolution, the OV9655 sensor is set to VGA resolution 00175 * as OV9655 doesn't supports 480x272 resolution, 00176 * then DCMI is configured to output a 480x272 cropped window */ 00177 camera_drv->Init(CameraHwAddress, CAMERA_R640x480); 00178 HAL_DCMI_ConfigCROP(phdcmi, /* Crop in the middle of the VGA picture */ 00179 (CAMERA_VGA_RES_X - CAMERA_480x272_RES_X)/2, 00180 (CAMERA_VGA_RES_Y - CAMERA_480x272_RES_Y)/2, 00181 (CAMERA_480x272_RES_X * 2) - 1, 00182 CAMERA_480x272_RES_Y - 1); 00183 HAL_DCMI_EnableCROP(phdcmi); 00184 } 00185 else 00186 { 00187 camera_drv->Init(CameraHwAddress, Resolution); 00188 HAL_DCMI_DisableCROP(phdcmi); 00189 } 00190 00191 CameraCurrentResolution = Resolution; 00192 00193 /* Return CAMERA_OK status */ 00194 status = CAMERA_OK; 00195 } 00196 else 00197 { 00198 /* Return CAMERA_NOT_SUPPORTED status */ 00199 status = CAMERA_NOT_SUPPORTED; 00200 } 00201 00202 return status; 00203 } 00204 00205 /** 00206 * @brief DeInitializes the camera. 00207 * @retval Camera status 00208 */ 00209 uint8_t BSP_CAMERA_DeInit(void) 00210 { 00211 hDcmiHandler.Instance = DCMI; 00212 00213 HAL_DCMI_DeInit(&hDcmiHandler); 00214 BSP_CAMERA_MspDeInit(&hDcmiHandler, NULL); 00215 return CAMERA_OK; 00216 } 00217 00218 /** 00219 * @brief Starts the camera capture in continuous mode. 00220 * @param buff: pointer to the camera output buffer 00221 * @retval None 00222 */ 00223 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00224 { 00225 /* Start the camera capture */ 00226 HAL_DCMI_Start_DMA(&hDcmiHandler, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00227 } 00228 00229 /** 00230 * @brief Starts the camera capture in snapshot mode. 00231 * @param buff: pointer to the camera output buffer 00232 * @retval None 00233 */ 00234 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00235 { 00236 /* Start the camera capture */ 00237 HAL_DCMI_Start_DMA(&hDcmiHandler, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00238 } 00239 00240 /** 00241 * @brief Suspend the CAMERA capture 00242 * @retval None 00243 */ 00244 void BSP_CAMERA_Suspend(void) 00245 { 00246 /* Suspend the Camera Capture */ 00247 HAL_DCMI_Suspend(&hDcmiHandler); 00248 } 00249 00250 /** 00251 * @brief Resume the CAMERA capture 00252 * @retval None 00253 */ 00254 void BSP_CAMERA_Resume(void) 00255 { 00256 /* Start the Camera Capture */ 00257 HAL_DCMI_Resume(&hDcmiHandler); 00258 } 00259 00260 /** 00261 * @brief Stop the CAMERA capture 00262 * @retval Camera status 00263 */ 00264 uint8_t BSP_CAMERA_Stop(void) 00265 { 00266 uint8_t status = CAMERA_ERROR; 00267 00268 if(HAL_DCMI_Stop(&hDcmiHandler) == HAL_OK) 00269 { 00270 status = CAMERA_OK; 00271 } 00272 00273 /* Set Camera in Power Down */ 00274 BSP_CAMERA_PwrDown(); 00275 00276 return status; 00277 } 00278 00279 /** 00280 * @brief CANERA power up 00281 * @retval None 00282 */ 00283 void BSP_CAMERA_PwrUp(void) 00284 { 00285 GPIO_InitTypeDef gpio_init_structure; 00286 00287 /* Enable GPIO clock */ 00288 __HAL_RCC_GPIOH_CLK_ENABLE(); 00289 00290 /*** Configure the GPIO ***/ 00291 /* Configure DCMI GPIO as alternate function */ 00292 gpio_init_structure.Pin = GPIO_PIN_13; 00293 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00294 gpio_init_structure.Pull = GPIO_NOPULL; 00295 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00296 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00297 00298 /* De-assert the camera POWER_DOWN pin (active high) */ 00299 HAL_GPIO_WritePin(GPIOH, GPIO_PIN_13, GPIO_PIN_RESET); 00300 00301 HAL_Delay(3); /* POWER_DOWN de-asserted during 3ms */ 00302 } 00303 00304 /** 00305 * @brief CAMERA power down 00306 * @retval None 00307 */ 00308 void BSP_CAMERA_PwrDown(void) 00309 { 00310 GPIO_InitTypeDef gpio_init_structure; 00311 00312 /* Enable GPIO clock */ 00313 __HAL_RCC_GPIOH_CLK_ENABLE(); 00314 00315 /*** Configure the GPIO ***/ 00316 /* Configure DCMI GPIO as alternate function */ 00317 gpio_init_structure.Pin = GPIO_PIN_13; 00318 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00319 gpio_init_structure.Pull = GPIO_NOPULL; 00320 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00321 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00322 00323 /* Assert the camera POWER_DOWN pin (active high) */ 00324 HAL_GPIO_WritePin(GPIOH, GPIO_PIN_13, GPIO_PIN_SET); 00325 } 00326 00327 /** 00328 * @brief Configures the camera contrast and brightness. 00329 * @param contrast_level: Contrast level 00330 * This parameter can be one of the following values: 00331 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00332 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00333 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00334 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00335 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00336 * @param brightness_level: Contrast level 00337 * This parameter can be one of the following values: 00338 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00339 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00340 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00341 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00342 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00343 * @retval None 00344 */ 00345 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00346 { 00347 if(camera_drv->Config != NULL) 00348 { 00349 camera_drv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00350 } 00351 } 00352 00353 /** 00354 * @brief Configures the camera white balance. 00355 * @param Mode: black_white mode 00356 * This parameter can be one of the following values: 00357 * @arg CAMERA_BLACK_WHITE_BW 00358 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00359 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00360 * @arg CAMERA_BLACK_WHITE_NORMAL 00361 * @retval None 00362 */ 00363 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00364 { 00365 if(camera_drv->Config != NULL) 00366 { 00367 camera_drv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00368 } 00369 } 00370 00371 /** 00372 * @brief Configures the camera color effect. 00373 * @param Effect: Color effect 00374 * This parameter can be one of the following values: 00375 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00376 * @arg CAMERA_COLOR_EFFECT_BLUE 00377 * @arg CAMERA_COLOR_EFFECT_GREEN 00378 * @arg CAMERA_COLOR_EFFECT_RED 00379 * @retval None 00380 */ 00381 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00382 { 00383 if(camera_drv->Config != NULL) 00384 { 00385 camera_drv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00386 } 00387 } 00388 00389 /** 00390 * @brief Get the capture size in pixels unit. 00391 * @param resolution: the current resolution. 00392 * @retval capture size in pixels unit. 00393 */ 00394 static uint32_t GetSize(uint32_t resolution) 00395 { 00396 uint32_t size = 0; 00397 00398 /* Get capture size */ 00399 switch (resolution) 00400 { 00401 case CAMERA_R160x120: 00402 { 00403 size = 0x2580; 00404 } 00405 break; 00406 case CAMERA_R320x240: 00407 { 00408 size = 0x9600; 00409 } 00410 break; 00411 case CAMERA_R480x272: 00412 { 00413 size = 0xFF00; 00414 } 00415 break; 00416 case CAMERA_R640x480: 00417 { 00418 size = 0x25800; 00419 } 00420 break; 00421 default: 00422 { 00423 break; 00424 } 00425 } 00426 00427 return size; 00428 } 00429 00430 /** 00431 * @brief Initializes the DCMI MSP. 00432 * @param hdcmi: HDMI handle 00433 * @param Params 00434 * @retval None 00435 */ 00436 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00437 { 00438 static DMA_HandleTypeDef hdma_handler; 00439 GPIO_InitTypeDef gpio_init_structure; 00440 00441 /*** Enable peripherals and GPIO clocks ***/ 00442 /* Enable DCMI clock */ 00443 __HAL_RCC_DCMI_CLK_ENABLE(); 00444 00445 /* Enable DMA2 clock */ 00446 __HAL_RCC_DMA2_CLK_ENABLE(); 00447 00448 /* Enable GPIO clocks */ 00449 __HAL_RCC_GPIOA_CLK_ENABLE(); 00450 __HAL_RCC_GPIOD_CLK_ENABLE(); 00451 __HAL_RCC_GPIOE_CLK_ENABLE(); 00452 __HAL_RCC_GPIOG_CLK_ENABLE(); 00453 __HAL_RCC_GPIOH_CLK_ENABLE(); 00454 00455 /*** Configure the GPIO ***/ 00456 /* Configure DCMI GPIO as alternate function */ 00457 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_6; 00458 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00459 gpio_init_structure.Pull = GPIO_PULLUP; 00460 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00461 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00462 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00463 00464 gpio_init_structure.Pin = GPIO_PIN_3; 00465 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00466 gpio_init_structure.Pull = GPIO_PULLUP; 00467 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00468 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00469 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00470 00471 gpio_init_structure.Pin = GPIO_PIN_5 | GPIO_PIN_6; 00472 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00473 gpio_init_structure.Pull = GPIO_PULLUP; 00474 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00475 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00476 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00477 00478 gpio_init_structure.Pin = GPIO_PIN_9; 00479 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00480 gpio_init_structure.Pull = GPIO_PULLUP; 00481 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00482 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00483 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00484 00485 gpio_init_structure.Pin = GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00486 GPIO_PIN_12 | GPIO_PIN_14; 00487 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00488 gpio_init_structure.Pull = GPIO_PULLUP; 00489 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00490 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00491 HAL_GPIO_Init(GPIOH, &gpio_init_structure); 00492 00493 /*** Configure the DMA ***/ 00494 /* Set the parameters to be configured */ 00495 hdma_handler.Init.Channel = DMA_CHANNEL_1; 00496 hdma_handler.Init.Direction = DMA_PERIPH_TO_MEMORY; 00497 hdma_handler.Init.PeriphInc = DMA_PINC_DISABLE; 00498 hdma_handler.Init.MemInc = DMA_MINC_ENABLE; 00499 hdma_handler.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00500 hdma_handler.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00501 hdma_handler.Init.Mode = DMA_CIRCULAR; 00502 hdma_handler.Init.Priority = DMA_PRIORITY_HIGH; 00503 hdma_handler.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00504 hdma_handler.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00505 hdma_handler.Init.MemBurst = DMA_MBURST_SINGLE; 00506 hdma_handler.Init.PeriphBurst = DMA_PBURST_SINGLE; 00507 00508 hdma_handler.Instance = DMA2_Stream1; 00509 00510 /* Associate the initialized DMA handle to the DCMI handle */ 00511 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_handler); 00512 00513 /*** Configure the NVIC for DCMI and DMA ***/ 00514 /* NVIC configuration for DCMI transfer complete interrupt */ 00515 HAL_NVIC_SetPriority(DCMI_IRQn, 0x0F, 0); 00516 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00517 00518 /* NVIC configuration for DMA2D transfer complete interrupt */ 00519 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 0x0F, 0); 00520 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00521 00522 /* Configure the DMA stream */ 00523 HAL_DMA_Init(hdcmi->DMA_Handle); 00524 } 00525 00526 00527 /** 00528 * @brief DeInitializes the DCMI MSP. 00529 * @param hdcmi: HDMI handle 00530 * @param Params 00531 * @retval None 00532 */ 00533 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00534 { 00535 /* Disable NVIC for DCMI transfer complete interrupt */ 00536 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00537 00538 /* Disable NVIC for DMA2 transfer complete interrupt */ 00539 HAL_NVIC_DisableIRQ(DMA2_Stream1_IRQn); 00540 00541 /* Configure the DMA stream */ 00542 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00543 00544 /* Disable DCMI clock */ 00545 __HAL_RCC_DCMI_CLK_DISABLE(); 00546 00547 /* GPIO pins clock and DMA clock can be shut down in the application 00548 by surcharging this __weak function */ 00549 } 00550 00551 /** 00552 * @brief Line event callback 00553 * @param hdcmi: pointer to the DCMI handle 00554 * @retval None 00555 */ 00556 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00557 { 00558 BSP_CAMERA_LineEventCallback(); 00559 } 00560 00561 /** 00562 * @brief Line Event callback. 00563 * @retval None 00564 */ 00565 __weak void BSP_CAMERA_LineEventCallback(void) 00566 { 00567 /* NOTE : This function Should not be modified, when the callback is needed, 00568 the HAL_DCMI_LineEventCallback could be implemented in the user file 00569 */ 00570 } 00571 00572 /** 00573 * @brief VSYNC event callback 00574 * @param hdcmi: pointer to the DCMI handle 00575 * @retval None 00576 */ 00577 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00578 { 00579 BSP_CAMERA_VsyncEventCallback(); 00580 } 00581 00582 /** 00583 * @brief VSYNC Event callback. 00584 * @retval None 00585 */ 00586 __weak void BSP_CAMERA_VsyncEventCallback(void) 00587 { 00588 /* NOTE : This function Should not be modified, when the callback is needed, 00589 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00590 */ 00591 } 00592 00593 /** 00594 * @brief Frame event callback 00595 * @param hdcmi: pointer to the DCMI handle 00596 * @retval None 00597 */ 00598 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00599 { 00600 BSP_CAMERA_FrameEventCallback(); 00601 } 00602 00603 /** 00604 * @brief Frame Event callback. 00605 * @retval None 00606 */ 00607 __weak void BSP_CAMERA_FrameEventCallback(void) 00608 { 00609 /* NOTE : This function Should not be modified, when the callback is needed, 00610 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00611 */ 00612 } 00613 00614 /** 00615 * @brief Error callback 00616 * @param hdcmi: pointer to the DCMI handle 00617 * @retval None 00618 */ 00619 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00620 { 00621 BSP_CAMERA_ErrorCallback(); 00622 } 00623 00624 /** 00625 * @brief Error callback. 00626 * @retval None 00627 */ 00628 __weak void BSP_CAMERA_ErrorCallback(void) 00629 { 00630 /* NOTE : This function Should not be modified, when the callback is needed, 00631 the HAL_DCMI_ErrorCallback could be implemented in the user file 00632 */ 00633 } 00634 00635 /** 00636 * @} 00637 */ 00638 00639 /** 00640 * @} 00641 */ 00642 00643 /** 00644 * @} 00645 */ 00646 00647 /** 00648 * @} 00649 */ 00650 00651 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 16:31:33 for STM32746G-Discovery BSP User Manual by
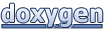