STM32746G-Discovery BSP User Manual
|
stm32746g_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32746g_discovery.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 30-December-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32746G-Discovery 00009 * board(MB1191) from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32746g_discovery.h" 00042 00043 /** @addtogroup BSP 00044 * @{ 00045 */ 00046 00047 /** @addtogroup STM32746G_DISCOVERY 00048 * @{ 00049 */ 00050 00051 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL STM32746G_DISCOVERY_LOW_LEVEL 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32746G_DISCOVERY_LOW_LEVEL Private Types Definitions 00056 * @{ 00057 */ 00058 /** 00059 * @} 00060 */ 00061 00062 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Defines STM32746G_DISCOVERY_LOW_LEVEL Private Defines 00063 * @{ 00064 */ 00065 /** 00066 * @brief STM32746G DISCOVERY BSP Driver version number V2.0.0 00067 */ 00068 #define __STM32746G_DISCO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00069 #define __STM32746G_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00070 #define __STM32746G_DISCO_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00071 #define __STM32746G_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00072 #define __STM32746G_DISCO_BSP_VERSION ((__STM32746G_DISCO_BSP_VERSION_MAIN << 24)\ 00073 |(__STM32746G_DISCO_BSP_VERSION_SUB1 << 16)\ 00074 |(__STM32746G_DISCO_BSP_VERSION_SUB2 << 8 )\ 00075 |(__STM32746G_DISCO_BSP_VERSION_RC)) 00076 /** 00077 * @} 00078 */ 00079 00080 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Macros STM32746G_DISCOVERY_LOW_LEVEL Private Macros 00081 * @{ 00082 */ 00083 /** 00084 * @} 00085 */ 00086 00087 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_Variables STM32746G_DISCOVERY_LOW_LEVEL Private Variables 00088 * @{ 00089 */ 00090 00091 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN}; 00092 00093 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00094 TAMPER_BUTTON_GPIO_PORT, 00095 KEY_BUTTON_GPIO_PORT}; 00096 00097 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00098 TAMPER_BUTTON_PIN, 00099 KEY_BUTTON_PIN}; 00100 00101 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00102 TAMPER_BUTTON_EXTI_IRQn, 00103 KEY_BUTTON_EXTI_IRQn}; 00104 00105 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00106 00107 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00108 00109 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00110 00111 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00112 00113 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00114 00115 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00116 00117 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00118 00119 static I2C_HandleTypeDef hI2cAudioHandler = {0}; 00120 static I2C_HandleTypeDef hI2cExtHandler = {0}; 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes STM32746G_DISCOVERY_LOW_LEVEL Private Function Prototypes 00127 * @{ 00128 */ 00129 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00130 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00131 00132 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00133 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00134 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials); 00135 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00136 00137 /* AUDIO IO functions */ 00138 void AUDIO_IO_Init(void); 00139 void AUDIO_IO_DeInit(void); 00140 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00141 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00142 void AUDIO_IO_Delay(uint32_t Delay); 00143 00144 /* TOUCHSCREEN IO functions */ 00145 void TS_IO_Init(void); 00146 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00147 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00148 void TS_IO_Delay(uint32_t Delay); 00149 00150 /* CAMERA IO functions */ 00151 void CAMERA_IO_Init(void); 00152 void CAMERA_Delay(uint32_t Delay); 00153 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00154 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg); 00155 00156 /* I2C EEPROM IO function */ 00157 void EEPROM_IO_Init(void); 00158 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00159 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00160 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00161 /** 00162 * @} 00163 */ 00164 00165 /** @defgroup STM32746G_DISCOVERY_LOW_LEVEL_Exported_Functions STM32746G_DISCOVERY_LOW_LEVELSTM32746G_DISCOVERY_LOW_LEVEL Exported Functions 00166 * @{ 00167 */ 00168 00169 /** 00170 * @brief This method returns the STM32746G DISCOVERY BSP Driver revision 00171 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00172 */ 00173 uint32_t BSP_GetVersion(void) 00174 { 00175 return __STM32746G_DISCO_BSP_VERSION; 00176 } 00177 00178 /** 00179 * @brief Configures LED on GPIO. 00180 * @param Led: LED to be configured. 00181 * This parameter can be one of the following values: 00182 * @arg LED1 00183 * @retval None 00184 */ 00185 void BSP_LED_Init(Led_TypeDef Led) 00186 { 00187 GPIO_InitTypeDef gpio_init_structure; 00188 GPIO_TypeDef* gpio_led; 00189 00190 if (Led == LED1) 00191 { 00192 gpio_led = LED1_GPIO_PORT; 00193 /* Enable the GPIO_LED clock */ 00194 LED1_GPIO_CLK_ENABLE(); 00195 00196 /* Configure the GPIO_LED pin */ 00197 gpio_init_structure.Pin = GPIO_PIN[Led]; 00198 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00199 gpio_init_structure.Pull = GPIO_PULLUP; 00200 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00201 00202 HAL_GPIO_Init(gpio_led, &gpio_init_structure); 00203 00204 /* By default, turn off LED */ 00205 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00206 } 00207 } 00208 00209 /** 00210 * @brief DeInit LEDs. 00211 * @param Led: LED to be configured. 00212 * This parameter can be one of the following values: 00213 * @arg LED1 00214 * @note Led DeInit does not disable the GPIO clock 00215 * @retval None 00216 */ 00217 void BSP_LED_DeInit(Led_TypeDef Led) 00218 { 00219 GPIO_InitTypeDef gpio_init_structure; 00220 GPIO_TypeDef* gpio_led; 00221 00222 if (Led == LED1) 00223 { 00224 gpio_led = LED1_GPIO_PORT; 00225 /* Turn off LED */ 00226 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00227 /* Configure the GPIO_LED pin */ 00228 gpio_init_structure.Pin = GPIO_PIN[Led]; 00229 HAL_GPIO_DeInit(gpio_led, gpio_init_structure.Pin); 00230 } 00231 } 00232 00233 /** 00234 * @brief Turns selected LED On. 00235 * @param Led: LED to be set on 00236 * This parameter can be one of the following values: 00237 * @arg LED1 00238 * @retval None 00239 */ 00240 void BSP_LED_On(Led_TypeDef Led) 00241 { 00242 GPIO_TypeDef* gpio_led; 00243 00244 if (Led == LED1) /* Switch On LED connected to GPIO */ 00245 { 00246 gpio_led = LED1_GPIO_PORT; 00247 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00248 } 00249 } 00250 00251 /** 00252 * @brief Turns selected LED Off. 00253 * @param Led: LED to be set off 00254 * This parameter can be one of the following values: 00255 * @arg LED1 00256 * @retval None 00257 */ 00258 void BSP_LED_Off(Led_TypeDef Led) 00259 { 00260 GPIO_TypeDef* gpio_led; 00261 00262 if (Led == LED1) /* Switch Off LED connected to GPIO */ 00263 { 00264 gpio_led = LED1_GPIO_PORT; 00265 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00266 } 00267 } 00268 00269 /** 00270 * @brief Toggles the selected LED. 00271 * @param Led: LED to be toggled 00272 * This parameter can be one of the following values: 00273 * @arg LED1 00274 * @retval None 00275 */ 00276 void BSP_LED_Toggle(Led_TypeDef Led) 00277 { 00278 GPIO_TypeDef* gpio_led; 00279 00280 if (Led == LED1) /* Toggle LED connected to GPIO */ 00281 { 00282 gpio_led = LED1_GPIO_PORT; 00283 HAL_GPIO_TogglePin(gpio_led, GPIO_PIN[Led]); 00284 } 00285 } 00286 00287 /** 00288 * @brief Configures button GPIO and EXTI Line. 00289 * @param Button: Button to be configured 00290 * This parameter can be one of the following values: 00291 * @arg BUTTON_WAKEUP: Wakeup Push Button 00292 * @arg BUTTON_TAMPER: Tamper Push Button 00293 * @arg BUTTON_KEY: Key Push Button 00294 * @param ButtonMode: Button mode 00295 * This parameter can be one of the following values: 00296 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00297 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00298 * with interrupt generation capability 00299 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00300 * are mapped on the same push button named "User" 00301 * on the board serigraphy. 00302 * @retval None 00303 */ 00304 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00305 { 00306 GPIO_InitTypeDef gpio_init_structure; 00307 00308 /* Enable the BUTTON clock */ 00309 BUTTONx_GPIO_CLK_ENABLE(Button); 00310 00311 if(ButtonMode == BUTTON_MODE_GPIO) 00312 { 00313 /* Configure Button pin as input */ 00314 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00315 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00316 gpio_init_structure.Pull = GPIO_NOPULL; 00317 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00318 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00319 } 00320 00321 if(ButtonMode == BUTTON_MODE_EXTI) 00322 { 00323 /* Configure Button pin as input with External interrupt */ 00324 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00325 gpio_init_structure.Pull = GPIO_NOPULL; 00326 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00327 00328 if(Button != BUTTON_WAKEUP) 00329 { 00330 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00331 } 00332 else 00333 { 00334 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00335 } 00336 00337 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00338 00339 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00340 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00341 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00342 } 00343 } 00344 00345 /** 00346 * @brief Push Button DeInit. 00347 * @param Button: Button to be configured 00348 * This parameter can be one of the following values: 00349 * @arg BUTTON_WAKEUP: Wakeup Push Button 00350 * @arg BUTTON_TAMPER: Tamper Push Button 00351 * @arg BUTTON_KEY: Key Push Button 00352 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00353 * are mapped on the same push button named "User" 00354 * on the board serigraphy. 00355 * @note PB DeInit does not disable the GPIO clock 00356 * @retval None 00357 */ 00358 void BSP_PB_DeInit(Button_TypeDef Button) 00359 { 00360 GPIO_InitTypeDef gpio_init_structure; 00361 00362 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00363 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00364 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00365 } 00366 00367 00368 /** 00369 * @brief Returns the selected button state. 00370 * @param Button: Button to be checked 00371 * This parameter can be one of the following values: 00372 * @arg BUTTON_WAKEUP: Wakeup Push Button 00373 * @arg BUTTON_TAMPER: Tamper Push Button 00374 * @arg BUTTON_KEY: Key Push Button 00375 * @note On STM32746G-Discovery board, the three buttons (Wakeup, Tamper and key buttons) 00376 * are mapped on the same push button named "User" 00377 * on the board serigraphy. 00378 * @retval The Button GPIO pin value 00379 */ 00380 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00381 { 00382 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00383 } 00384 00385 /** 00386 * @brief Configures COM port. 00387 * @param COM: COM port to be configured. 00388 * This parameter can be one of the following values: 00389 * @arg COM1 00390 * @arg COM2 00391 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00392 * configuration information for the specified USART peripheral. 00393 * @retval None 00394 */ 00395 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00396 { 00397 GPIO_InitTypeDef gpio_init_structure; 00398 00399 /* Enable GPIO clock */ 00400 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00401 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00402 00403 /* Enable USART clock */ 00404 DISCOVERY_COMx_CLK_ENABLE(COM); 00405 00406 /* Configure USART Tx as alternate function */ 00407 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00408 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00409 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00410 gpio_init_structure.Pull = GPIO_PULLUP; 00411 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00412 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00413 00414 /* Configure USART Rx as alternate function */ 00415 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00416 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00417 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00418 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00419 00420 /* USART configuration */ 00421 huart->Instance = COM_USART[COM]; 00422 HAL_UART_Init(huart); 00423 } 00424 00425 /** 00426 * @brief DeInit COM port. 00427 * @param COM: COM port to be configured. 00428 * This parameter can be one of the following values: 00429 * @arg COM1 00430 * @arg COM2 00431 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00432 * configuration information for the specified USART peripheral. 00433 * @retval None 00434 */ 00435 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00436 { 00437 /* USART configuration */ 00438 huart->Instance = COM_USART[COM]; 00439 HAL_UART_DeInit(huart); 00440 00441 /* Enable USART clock */ 00442 DISCOVERY_COMx_CLK_DISABLE(COM); 00443 00444 /* DeInit GPIO pins can be done in the application 00445 (by surcharging this __weak function) */ 00446 00447 /* GPIO pins clock, DMA clock can be shut down in the application 00448 by surcharging this __weak function */ 00449 } 00450 00451 /******************************************************************************* 00452 BUS OPERATIONS 00453 *******************************************************************************/ 00454 00455 /******************************* I2C Routines *********************************/ 00456 /** 00457 * @brief Initializes I2C MSP. 00458 * @param i2c_handler : I2C handler 00459 * @retval None 00460 */ 00461 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00462 { 00463 GPIO_InitTypeDef gpio_init_structure; 00464 00465 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00466 { 00467 /* AUDIO and LCD I2C MSP init */ 00468 00469 /*** Configure the GPIOs ***/ 00470 /* Enable GPIO clock */ 00471 DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00472 00473 /* Configure I2C Tx as alternate function */ 00474 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00475 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00476 gpio_init_structure.Pull = GPIO_NOPULL; 00477 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00478 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_SDA_AF; 00479 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00480 00481 /* Configure I2C Rx as alternate function */ 00482 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00483 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00484 00485 /*** Configure the I2C peripheral ***/ 00486 /* Enable I2C clock */ 00487 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00488 00489 /* Force the I2C peripheral clock reset */ 00490 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00491 00492 /* Release the I2C peripheral clock reset */ 00493 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00494 00495 /* Enable and set I2Cx Interrupt to a lower priority */ 00496 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0); 00497 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00498 00499 /* Enable and set I2Cx Interrupt to a lower priority */ 00500 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0); 00501 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00502 } 00503 else 00504 { 00505 /* External, camera and Arduino connector I2C MSP init */ 00506 00507 /*** Configure the GPIOs ***/ 00508 /* Enable GPIO clock */ 00509 DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00510 00511 /* Configure I2C Tx as alternate function */ 00512 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SCL_PIN; 00513 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00514 gpio_init_structure.Pull = GPIO_NOPULL; 00515 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00516 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SCL_SDA_AF; 00517 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00518 00519 /* Configure I2C Rx as alternate function */ 00520 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SDA_PIN; 00521 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00522 00523 /*** Configure the I2C peripheral ***/ 00524 /* Enable I2C clock */ 00525 DISCOVERY_EXT_I2Cx_CLK_ENABLE(); 00526 00527 /* Force the I2C peripheral clock reset */ 00528 DISCOVERY_EXT_I2Cx_FORCE_RESET(); 00529 00530 /* Release the I2C peripheral clock reset */ 00531 DISCOVERY_EXT_I2Cx_RELEASE_RESET(); 00532 00533 /* Enable and set I2Cx Interrupt to a lower priority */ 00534 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_EV_IRQn, 0x0F, 0); 00535 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_EV_IRQn); 00536 00537 /* Enable and set I2Cx Interrupt to a lower priority */ 00538 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_ER_IRQn, 0x0F, 0); 00539 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_ER_IRQn); 00540 } 00541 } 00542 00543 /** 00544 * @brief Initializes I2C HAL. 00545 * @param i2c_handler : I2C handler 00546 * @retval None 00547 */ 00548 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00549 { 00550 if(HAL_I2C_GetState(i2c_handler) == HAL_I2C_STATE_RESET) 00551 { 00552 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00553 { 00554 /* Audio and LCD I2C configuration */ 00555 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00556 } 00557 else 00558 { 00559 /* External, camera and Arduino connector I2C configuration */ 00560 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00561 } 00562 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00563 i2c_handler->Init.OwnAddress1 = 0; 00564 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00565 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00566 i2c_handler->Init.OwnAddress2 = 0; 00567 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00568 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00569 00570 /* Init the I2C */ 00571 I2Cx_MspInit(i2c_handler); 00572 HAL_I2C_Init(i2c_handler); 00573 } 00574 } 00575 00576 /** 00577 * @brief Reads multiple data. 00578 * @param i2c_handler : I2C handler 00579 * @param Addr: I2C address 00580 * @param Reg: Reg address 00581 * @param MemAddress: Memory address 00582 * @param Buffer: Pointer to data buffer 00583 * @param Length: Length of the data 00584 * @retval Number of read data 00585 */ 00586 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, 00587 uint8_t Addr, 00588 uint16_t Reg, 00589 uint16_t MemAddress, 00590 uint8_t *Buffer, 00591 uint16_t Length) 00592 { 00593 HAL_StatusTypeDef status = HAL_OK; 00594 00595 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00596 00597 /* Check the communication status */ 00598 if(status != HAL_OK) 00599 { 00600 /* I2C error occurred */ 00601 I2Cx_Error(i2c_handler, Addr); 00602 } 00603 return status; 00604 } 00605 00606 /** 00607 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00608 * @param i2c_handler : I2C handler 00609 * @param Addr: Device address on BUS Bus. 00610 * @param Reg: The target register address to write 00611 * @param MemAddress: Memory address 00612 * @param Buffer: The target register value to be written 00613 * @param Length: buffer size to be written 00614 * @retval HAL status 00615 */ 00616 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, 00617 uint8_t Addr, 00618 uint16_t Reg, 00619 uint16_t MemAddress, 00620 uint8_t *Buffer, 00621 uint16_t Length) 00622 { 00623 HAL_StatusTypeDef status = HAL_OK; 00624 00625 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00626 00627 /* Check the communication status */ 00628 if(status != HAL_OK) 00629 { 00630 /* Re-Initiaize the I2C Bus */ 00631 I2Cx_Error(i2c_handler, Addr); 00632 } 00633 return status; 00634 } 00635 00636 /** 00637 * @brief Checks if target device is ready for communication. 00638 * @note This function is used with Memory devices 00639 * @param i2c_handler : I2C handler 00640 * @param DevAddress: Target device address 00641 * @param Trials: Number of trials 00642 * @retval HAL status 00643 */ 00644 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) 00645 { 00646 return (HAL_I2C_IsDeviceReady(i2c_handler, DevAddress, Trials, 1000)); 00647 } 00648 00649 /** 00650 * @brief Manages error callback by re-initializing I2C. 00651 * @param i2c_handler : I2C handler 00652 * @param Addr: I2C Address 00653 * @retval None 00654 */ 00655 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00656 { 00657 /* De-initialize the I2C communication bus */ 00658 HAL_I2C_DeInit(i2c_handler); 00659 00660 /* Re-Initialize the I2C communication bus */ 00661 I2Cx_Init(i2c_handler); 00662 } 00663 00664 /******************************************************************************* 00665 LINK OPERATIONS 00666 *******************************************************************************/ 00667 00668 /********************************* LINK AUDIO *********************************/ 00669 00670 /** 00671 * @brief Initializes Audio low level. 00672 * @retval None 00673 */ 00674 void AUDIO_IO_Init(void) 00675 { 00676 I2Cx_Init(&hI2cAudioHandler); 00677 } 00678 00679 /** 00680 * @brief Deinitializes Audio low level. 00681 * @retval None 00682 */ 00683 void AUDIO_IO_DeInit(void) 00684 { 00685 } 00686 00687 /** 00688 * @brief Writes a single data. 00689 * @param Addr: I2C address 00690 * @param Reg: Reg address 00691 * @param Value: Data to be written 00692 * @retval None 00693 */ 00694 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00695 { 00696 uint16_t tmp = Value; 00697 00698 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00699 00700 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00701 00702 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00703 } 00704 00705 /** 00706 * @brief Reads a single data. 00707 * @param Addr: I2C address 00708 * @param Reg: Reg address 00709 * @retval Data to be read 00710 */ 00711 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00712 { 00713 uint16_t read_value = 0, tmp = 0; 00714 00715 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 00716 00717 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 00718 00719 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 00720 00721 read_value = tmp; 00722 00723 return read_value; 00724 } 00725 00726 /** 00727 * @brief AUDIO Codec delay 00728 * @param Delay: Delay in ms 00729 * @retval None 00730 */ 00731 void AUDIO_IO_Delay(uint32_t Delay) 00732 { 00733 HAL_Delay(Delay); 00734 } 00735 00736 /********************************* LINK CAMERA ********************************/ 00737 00738 /** 00739 * @brief Initializes Camera low level. 00740 * @retval None 00741 */ 00742 void CAMERA_IO_Init(void) 00743 { 00744 I2Cx_Init(&hI2cExtHandler); 00745 } 00746 00747 /** 00748 * @brief Camera writes single data. 00749 * @param Addr: I2C address 00750 * @param Reg: Register address 00751 * @param Value: Data to be written 00752 * @retval None 00753 */ 00754 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00755 { 00756 I2Cx_WriteMultiple(&hI2cExtHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00757 } 00758 00759 /** 00760 * @brief Camera reads single data. 00761 * @param Addr: I2C address 00762 * @param Reg: Register address 00763 * @retval Read data 00764 */ 00765 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg) 00766 { 00767 uint8_t read_value = 0; 00768 00769 I2Cx_ReadMultiple(&hI2cExtHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00770 00771 return read_value; 00772 } 00773 00774 /** 00775 * @brief Camera delay 00776 * @param Delay: Delay in ms 00777 * @retval None 00778 */ 00779 void CAMERA_Delay(uint32_t Delay) 00780 { 00781 HAL_Delay(Delay); 00782 } 00783 00784 /******************************** LINK I2C EEPROM *****************************/ 00785 00786 /** 00787 * @brief Initializes peripherals used by the I2C EEPROM driver. 00788 * @retval None 00789 */ 00790 void EEPROM_IO_Init(void) 00791 { 00792 I2Cx_Init(&hI2cExtHandler); 00793 } 00794 00795 /** 00796 * @brief Write data to I2C EEPROM driver in using DMA channel. 00797 * @param DevAddress: Target device address 00798 * @param MemAddress: Internal memory address 00799 * @param pBuffer: Pointer to data buffer 00800 * @param BufferSize: Amount of data to be sent 00801 * @retval HAL status 00802 */ 00803 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00804 { 00805 return (I2Cx_WriteMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00806 } 00807 00808 /** 00809 * @brief Read data from I2C EEPROM driver in using DMA channel. 00810 * @param DevAddress: Target device address 00811 * @param MemAddress: Internal memory address 00812 * @param pBuffer: Pointer to data buffer 00813 * @param BufferSize: Amount of data to be read 00814 * @retval HAL status 00815 */ 00816 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00817 { 00818 return (I2Cx_ReadMultiple(&hI2cExtHandler, DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00819 } 00820 00821 /** 00822 * @brief Checks if target device is ready for communication. 00823 * @note This function is used with Memory devices 00824 * @param DevAddress: Target device address 00825 * @param Trials: Number of trials 00826 * @retval HAL status 00827 */ 00828 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00829 { 00830 return (I2Cx_IsDeviceReady(&hI2cExtHandler, DevAddress, Trials)); 00831 } 00832 00833 /********************************* LINK TOUCHSCREEN *********************************/ 00834 00835 /** 00836 * @brief Initializes Touchscreen low level. 00837 * @retval None 00838 */ 00839 void TS_IO_Init(void) 00840 { 00841 I2Cx_Init(&hI2cAudioHandler); 00842 } 00843 00844 /** 00845 * @brief Writes a single data. 00846 * @param Addr: I2C address 00847 * @param Reg: Reg address 00848 * @param Value: Data to be written 00849 * @retval None 00850 */ 00851 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00852 { 00853 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00854 } 00855 00856 /** 00857 * @brief Reads a single data. 00858 * @param Addr: I2C address 00859 * @param Reg: Reg address 00860 * @retval Data to be read 00861 */ 00862 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00863 { 00864 uint8_t read_value = 0; 00865 00866 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00867 00868 return read_value; 00869 } 00870 00871 /** 00872 * @brief TS delay 00873 * @param Delay: Delay in ms 00874 * @retval None 00875 */ 00876 void TS_IO_Delay(uint32_t Delay) 00877 { 00878 HAL_Delay(Delay); 00879 } 00880 00881 /** 00882 * @} 00883 */ 00884 00885 /** 00886 * @} 00887 */ 00888 00889 /** 00890 * @} 00891 */ 00892 00893 /** 00894 * @} 00895 */ 00896 00897 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Dec 30 2016 16:31:33 for STM32746G-Discovery BSP User Manual by
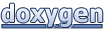