STM32412G-Discovery BSP User Manual
|
stm32412g_discovery_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32412g_discovery_sd.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 27-January-2017 00007 * @brief This file includes the uSD card driver mounted on STM32412G-DISCOVERY 00008 * board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the micro SD external card mounted on STM32412G-DISCOVERY 00044 board. 00045 - This driver does not need a specific component driver for the micro SD device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the micro SD card using the BSP_SD_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 SDIO interface configuration to interface with the external micro SD. It 00054 also includes the micro SD initialization sequence. 00055 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00056 returns the detection status 00057 o If SD presence detection interrupt mode is desired, you must configure the 00058 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00059 is generated as an external interrupt whenever the micro SD card is 00060 plugged/unplugged in/from the board. 00061 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00062 which is stored in the structure "HAL_SD_CardInfoTypeDef". 00063 00064 + Micro SD card operations 00065 o The micro SD card can be accessed with read/write block(s) operations once 00066 it is ready for access. The access can be performed whether using the polling 00067 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00068 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00069 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00070 is complete, the SD interrupt is handled using the function BSP_SD_IRQHandler(), 00071 the DMA Tx/Rx transfer complete are handled using the functions 00072 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00073 are implemented by the user at application level. 00074 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00075 the number of blocks to erase. 00076 o The SD runtime status is returned when calling the function BSP_SD_GetCardState(). 00077 00078 ------------------------------------------------------------------------------*/ 00079 00080 /* Includes ------------------------------------------------------------------*/ 00081 #include "stm32412g_discovery_sd.h" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32412G_DISCOVERY 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM32412G_DISCOVERY_SD STM32412G-DISCOVERY SD 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM32412G_DISCOVERY_SD_Private_Variables STM32412G Discovery Sd Private Variables 00096 * @{ 00097 */ 00098 SD_HandleTypeDef uSdHandle; 00099 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32412G_DISCOVERY_SD_Private_Functions STM32412G Discovery Sd Private Functions 00105 * @{ 00106 */ 00107 00108 /** 00109 * @brief Initializes the SD card device. 00110 * @retval SD status 00111 */ 00112 uint8_t BSP_SD_Init(void) 00113 { 00114 uint8_t sd_state = MSD_OK; 00115 00116 /* uSD device interface configuration */ 00117 uSdHandle.Instance = SDIO; 00118 00119 uSdHandle.Init.ClockEdge = SDIO_CLOCK_EDGE_RISING; 00120 uSdHandle.Init.ClockBypass = SDIO_CLOCK_BYPASS_DISABLE; 00121 uSdHandle.Init.ClockPowerSave = SDIO_CLOCK_POWER_SAVE_DISABLE; 00122 uSdHandle.Init.BusWide = SDIO_BUS_WIDE_1B; 00123 uSdHandle.Init.HardwareFlowControl = SDIO_HARDWARE_FLOW_CONTROL_ENABLE; 00124 uSdHandle.Init.ClockDiv = SDIO_TRANSFER_CLK_DIV; 00125 00126 /* Msp SD Detect pin initialization */ 00127 BSP_SD_Detect_MspInit(&uSdHandle, NULL); 00128 00129 /* Check if SD card is present */ 00130 if(BSP_SD_IsDetected() != SD_PRESENT) 00131 { 00132 return MSD_ERROR_SD_NOT_PRESENT; 00133 } 00134 00135 /* Msp SD initialization */ 00136 BSP_SD_MspInit(&uSdHandle, NULL); 00137 00138 /* HAL SD initialization */ 00139 if(HAL_SD_Init(&uSdHandle) != HAL_OK) 00140 { 00141 sd_state = MSD_ERROR; 00142 } 00143 00144 /* Configure SD Bus width */ 00145 if(sd_state == MSD_OK) 00146 { 00147 /* Enable wide operation */ 00148 if(HAL_SD_ConfigWideBusOperation(&uSdHandle, SDIO_BUS_WIDE_4B) != HAL_OK) 00149 { 00150 sd_state = MSD_ERROR; 00151 } 00152 else 00153 { 00154 sd_state = MSD_OK; 00155 } 00156 } 00157 00158 return sd_state; 00159 } 00160 00161 /** 00162 * @brief DeInitializes the SD card device. 00163 * @retval SD status 00164 */ 00165 uint8_t BSP_SD_DeInit(void) 00166 { 00167 uint8_t sd_state = MSD_OK; 00168 00169 uSdHandle.Instance = SDIO; 00170 00171 /* HAL SD deinitialization */ 00172 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00173 { 00174 sd_state = MSD_ERROR; 00175 } 00176 00177 /* Msp SD deinitialization */ 00178 uSdHandle.Instance = SDIO; 00179 BSP_SD_MspDeInit(&uSdHandle, NULL); 00180 00181 return sd_state; 00182 } 00183 00184 /** 00185 * @brief Configures Interrupt mode for SD detection pin. 00186 * @retval Returns MSD_OK 00187 */ 00188 uint8_t BSP_SD_ITConfig(void) 00189 { 00190 GPIO_InitTypeDef gpio_init_structure; 00191 00192 /* Configure Interrupt mode for SD detection pin */ 00193 gpio_init_structure.Pin = SD_DETECT_PIN; 00194 gpio_init_structure.Pull = GPIO_PULLUP; 00195 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00196 gpio_init_structure.Mode = GPIO_MODE_IT_RISING_FALLING; 00197 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00198 00199 /* Enable and set SD detect EXTI Interrupt to the lowest priority */ 00200 HAL_NVIC_SetPriority((IRQn_Type)(SD_DETECT_EXTI_IRQn), 0x0F, 0x00); 00201 HAL_NVIC_EnableIRQ((IRQn_Type)(SD_DETECT_EXTI_IRQn)); 00202 00203 return MSD_OK; 00204 } 00205 00206 /** 00207 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00208 * @retval Returns if SD is detected or not 00209 */ 00210 uint8_t BSP_SD_IsDetected(void) 00211 { 00212 __IO uint8_t status = SD_PRESENT; 00213 00214 /* Check SD card detect pin */ 00215 if (HAL_GPIO_ReadPin(SD_DETECT_GPIO_PORT, SD_DETECT_PIN) == GPIO_PIN_SET) 00216 { 00217 status = SD_NOT_PRESENT; 00218 } 00219 00220 return status; 00221 } 00222 00223 /** 00224 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00225 * @param pData: Pointer to the buffer that will contain the data to transmit 00226 * @param ReadAddr: Address from where data is to be read 00227 * @param NumOfBlocks: Number of SD blocks to read 00228 * @param Timeout: Timeout for read operation 00229 * @retval SD status 00230 */ 00231 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00232 { 00233 if(HAL_SD_ReadBlocks(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout) != HAL_OK) 00234 { 00235 return MSD_ERROR; 00236 } 00237 else 00238 { 00239 return MSD_OK; 00240 } 00241 } 00242 00243 /** 00244 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00245 * @param pData: Pointer to the buffer that will contain the data to transmit 00246 * @param WriteAddr: Address from where data is to be written 00247 * @param NumOfBlocks: Number of SD blocks to write 00248 * @param Timeout: Timeout for write operation 00249 * @retval SD status 00250 */ 00251 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00252 { 00253 if(HAL_SD_WriteBlocks(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout) != HAL_OK) 00254 { 00255 return MSD_ERROR; 00256 } 00257 else 00258 { 00259 return MSD_OK; 00260 } 00261 } 00262 00263 /** 00264 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00265 * @param pData: Pointer to the buffer that will contain the data to transmit 00266 * @param ReadAddr: Address from where data is to be read 00267 * @param NumOfBlocks: Number of SD blocks to read 00268 * @retval SD status 00269 */ 00270 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00271 { 00272 /* Read block(s) in DMA transfer mode */ 00273 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks) != HAL_OK) 00274 { 00275 return MSD_ERROR; 00276 } 00277 else 00278 { 00279 return MSD_OK; 00280 } 00281 } 00282 00283 /** 00284 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00285 * @param pData: Pointer to the buffer that will contain the data to transmit 00286 * @param WriteAddr: Address from where data is to be written 00287 * @param NumOfBlocks: Number of SD blocks to write 00288 * @retval SD status 00289 */ 00290 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00291 { 00292 /* Write block(s) in DMA transfer mode */ 00293 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks) != HAL_OK) 00294 { 00295 return MSD_ERROR; 00296 } 00297 else 00298 { 00299 return MSD_OK; 00300 } 00301 } 00302 00303 /** 00304 * @brief Erases the specified memory area of the given SD card. 00305 * @param StartAddr: Start byte address 00306 * @param EndAddr: End byte address 00307 * @retval SD status 00308 */ 00309 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr) 00310 { 00311 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != HAL_OK) 00312 { 00313 return MSD_ERROR; 00314 } 00315 else 00316 { 00317 return MSD_OK; 00318 } 00319 } 00320 00321 /** 00322 * @brief Initializes the SD MSP. 00323 * @param hsd: SD handle 00324 * @param Params : pointer on additional configuration parameters, can be NULL. 00325 * @retval None 00326 */ 00327 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00328 { 00329 static DMA_HandleTypeDef dma_rx_handle; 00330 static DMA_HandleTypeDef dma_tx_handle; 00331 GPIO_InitTypeDef gpio_init_structure; 00332 00333 /* Enable SDIO clock */ 00334 __HAL_RCC_SDIO_CLK_ENABLE(); 00335 00336 /* Enable DMA2 clocks */ 00337 __DMAx_TxRx_CLK_ENABLE(); 00338 00339 /* Enable GPIOs clock */ 00340 __HAL_RCC_GPIOC_CLK_ENABLE(); 00341 __HAL_RCC_GPIOD_CLK_ENABLE(); 00342 00343 /* Common GPIO configuration */ 00344 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00345 gpio_init_structure.Pull = GPIO_PULLUP; 00346 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00347 gpio_init_structure.Alternate = GPIO_AF12_SDIO; 00348 00349 /* GPIOC configuration */ 00350 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00351 00352 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00353 00354 /* GPIOD configuration */ 00355 gpio_init_structure.Pin = GPIO_PIN_2; 00356 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00357 00358 /* NVIC configuration for SDIO interrupts */ 00359 HAL_NVIC_SetPriority(SDIO_IRQn, 0x0E, 0); 00360 HAL_NVIC_EnableIRQ(SDIO_IRQn); 00361 00362 /* Configure DMA Rx parameters */ 00363 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00364 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00365 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00366 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00367 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00368 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00369 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00370 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00371 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00372 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00373 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00374 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00375 00376 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00377 00378 /* Associate the DMA handle */ 00379 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00380 00381 /* Deinitialize the stream for new transfer */ 00382 HAL_DMA_DeInit(&dma_rx_handle); 00383 00384 /* Configure the DMA stream */ 00385 HAL_DMA_Init(&dma_rx_handle); 00386 00387 /* Configure DMA Tx parameters */ 00388 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00389 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00390 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00391 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00392 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00393 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00394 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00395 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00396 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00397 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00398 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00399 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00400 00401 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00402 00403 /* Associate the DMA handle */ 00404 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00405 00406 /* Deinitialize the stream for new transfer */ 00407 HAL_DMA_DeInit(&dma_tx_handle); 00408 00409 /* Configure the DMA stream */ 00410 HAL_DMA_Init(&dma_tx_handle); 00411 00412 /* NVIC configuration for DMA transfer complete interrupt */ 00413 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 0x0F, 0); 00414 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00415 00416 /* NVIC configuration for DMA transfer complete interrupt */ 00417 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 0x0F, 0); 00418 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00419 } 00420 00421 /** 00422 * @brief Initializes the SD Detect pin MSP. 00423 * @param hsd: SD handle 00424 * @param Params : pointer on additional configuration parameters, can be NULL. 00425 * @retval None 00426 */ 00427 __weak void BSP_SD_Detect_MspInit(SD_HandleTypeDef *hsd, void *Params) 00428 { 00429 GPIO_InitTypeDef gpio_init_structure; 00430 00431 SD_DETECT_GPIO_CLK_ENABLE(); 00432 00433 /* GPIO configuration in input for uSD_Detect signal */ 00434 gpio_init_structure.Pin = SD_DETECT_PIN; 00435 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00436 gpio_init_structure.Pull = GPIO_PULLUP; 00437 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00438 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00439 } 00440 00441 /** 00442 * @brief DeInitializes the SD MSP. 00443 * @param hsd: SD handle 00444 * @param Params : pointer on additional configuration parameters, can be NULL. 00445 * @retval None 00446 */ 00447 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00448 { 00449 static DMA_HandleTypeDef dma_rx_handle; 00450 static DMA_HandleTypeDef dma_tx_handle; 00451 00452 /* Disable NVIC for DMA transfer complete interrupts */ 00453 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00454 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00455 00456 /* Deinitialize the stream for new transfer */ 00457 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00458 HAL_DMA_DeInit(&dma_rx_handle); 00459 00460 /* Deinitialize the stream for new transfer */ 00461 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00462 HAL_DMA_DeInit(&dma_tx_handle); 00463 00464 /* Disable NVIC for SDIO interrupts */ 00465 HAL_NVIC_DisableIRQ(SDIO_IRQn); 00466 00467 /* DeInit GPIO pins can be done in the application 00468 (by surcharging this __weak function) */ 00469 00470 /* Disable SDIO clock */ 00471 __HAL_RCC_SDIO_CLK_DISABLE(); 00472 00473 /* GPIO pins clock and DMA clocks can be shut down in the application 00474 by surcharging this __weak function */ 00475 } 00476 00477 /** 00478 * @brief Gets the current SD card data status. 00479 * @retval Data transfer state. 00480 * This value can be one of the following values: 00481 * @arg SD_TRANSFER_OK: No data transfer is acting 00482 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00483 */ 00484 uint8_t BSP_SD_GetCardState(void) 00485 { 00486 return((HAL_SD_GetCardState(&uSdHandle) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00487 } 00488 00489 00490 /** 00491 * @brief Get SD information about specific SD card. 00492 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00493 */ 00494 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypeDef *CardInfo) 00495 { 00496 /* Get SD card Information */ 00497 HAL_SD_GetCardInfo(&uSdHandle, CardInfo); 00498 } 00499 00500 /** 00501 * @brief SD Abort callbacks 00502 * @param hsd: SD handle 00503 */ 00504 void HAL_SD_AbortCallback(SD_HandleTypeDef *hsd) 00505 { 00506 BSP_SD_AbortCallback(); 00507 } 00508 00509 /** 00510 * @brief Tx Transfer completed callbacks 00511 * @param hsd: SD handle 00512 */ 00513 void HAL_SD_TxCpltCallback(SD_HandleTypeDef *hsd) 00514 { 00515 BSP_SD_WriteCpltCallback(); 00516 } 00517 00518 /** 00519 * @brief Rx Transfer completed callbacks 00520 * @param hsd: SD handle 00521 */ 00522 void HAL_SD_RxCpltCallback(SD_HandleTypeDef *hsd) 00523 { 00524 BSP_SD_ReadCpltCallback(); 00525 } 00526 00527 /** 00528 * @brief BSP SD Abort callbacks 00529 */ 00530 __weak void BSP_SD_AbortCallback(void) 00531 { 00532 00533 } 00534 00535 /** 00536 * @brief BSP Tx Transfer completed callbacks 00537 */ 00538 __weak void BSP_SD_WriteCpltCallback(void) 00539 { 00540 00541 } 00542 00543 /** 00544 * @brief BSP Rx Transfer completed callbacks 00545 */ 00546 __weak void BSP_SD_ReadCpltCallback(void) 00547 { 00548 00549 } 00550 00551 /** 00552 * @} 00553 */ 00554 00555 /** 00556 * @} 00557 */ 00558 00559 /** 00560 * @} 00561 */ 00562 00563 /** 00564 * @} 00565 */ 00566 00567 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 24 2017 11:28:05 for STM32412G-Discovery BSP User Manual by
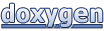