STM32412G-Discovery BSP User Manual
|
stm32412g_discovery_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32412g_discovery_audio.h 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 27-January-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32412g_discovery_audio.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32412G_DISCOVERY_AUDIO_H 00041 #define __STM32412G_DISCOVERY_AUDIO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include audio component Driver */ 00049 #include "../Components/wm8994/wm8994.h" 00050 #include "stm32412g_discovery.h" 00051 #include "../../../Middlewares/ST/STM32_Audio/Addons/PDM/pdm_filter.h" 00052 00053 /** @addtogroup BSP 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32412G_DISCOVERY 00058 * @{ 00059 */ 00060 00061 /** @addtogroup STM32412G_DISCOVERY_AUDIO 00062 * @{ 00063 */ 00064 00065 /** @defgroup STM32412G_DISCOVERY_AUDIO_Exported_Types STM32412G DISCOVERY Audio Exported Types 00066 * @{ 00067 */ 00068 typedef struct 00069 { 00070 uint32_t Frequency; /* Record Frequency */ 00071 uint32_t BitResolution; /* Record bit resolution */ 00072 uint32_t ChannelNbr; /* Record Channel Number */ 00073 uint16_t *pRecBuf; /* Pointer to record user buffer */ 00074 uint32_t RecSize; /* Size to record in mono, double size to record in stereo */ 00075 uint32_t InputDevice; /* Audio Input Device */ 00076 uint32_t MultiBuffMode; /* Multi buffer mode selection */ 00077 }AUDIOIN_ContextTypeDef; 00078 00079 /** 00080 * @} 00081 */ 00082 00083 /** @defgroup STM32412G_DISCOVERY_AUDIO_Exported_Constants STM32412G DISCOVERY Audio Exported Constants 00084 * @{ 00085 */ 00086 00087 /*------------------------------------------------------------------------------ 00088 AUDIO OUT CONFIGURATION 00089 ------------------------------------------------------------------------------*/ 00090 /* SPI Configuration defines */ 00091 #define AUDIO_OUT_I2Sx SPI3 00092 #define AUDIO_OUT_I2Sx_CLK_ENABLE() __HAL_RCC_SPI3_CLK_ENABLE() 00093 #define AUDIO_OUT_I2Sx_CLK_DISABLE() __HAL_RCC_SPI3_CLK_DISABLE() 00094 00095 #define AUDIO_OUT_I2Sx_MCK_PIN GPIO_PIN_7 00096 #define AUDIO_OUT_I2Sx_MCK_GPIO_PORT GPIOC 00097 #define AUDIO_OUT_I2Sx_MCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00098 #define AUDIO_OUT_I2Sx_MCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00099 #define AUDIO_OUT_I2Sx_MCK_AF GPIO_AF6_SPI3 00100 00101 #define AUDIO_OUT_I2Sx_SCK_PIN GPIO_PIN_12 00102 #define AUDIO_OUT_I2Sx_SCK_GPIO_PORT GPIOB 00103 #define AUDIO_OUT_I2Sx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00104 #define AUDIO_OUT_I2Sx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00105 #define AUDIO_OUT_I2Sx_SCK_AF GPIO_AF7_SPI3 00106 00107 #define AUDIO_OUT_I2Sx_WS_PIN GPIO_PIN_4 00108 #define AUDIO_OUT_I2Sx_WS_GPIO_PORT GPIOA 00109 #define AUDIO_OUT_I2Sx_WS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00110 #define AUDIO_OUT_I2Sx_WS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00111 #define AUDIO_OUT_I2Sx_WS_AF GPIO_AF6_SPI3 00112 00113 #define AUDIO_OUT_I2Sx_SD_PIN GPIO_PIN_5 00114 #define AUDIO_OUT_I2Sx_SD_GPIO_PORT GPIOB 00115 #define AUDIO_OUT_I2Sx_SD_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00116 #define AUDIO_OUT_I2Sx_SD_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00117 #define AUDIO_OUT_I2Sx_SD_AF GPIO_AF6_SPI3 00118 00119 /* I2S DMA Stream Tx definitions */ 00120 #define AUDIO_OUT_I2Sx_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00121 #define AUDIO_OUT_I2Sx_DMAx_CLK_DISABLE() __HAL_RCC_DMA1_CLK_DISABLE() 00122 #define AUDIO_OUT_I2Sx_DMAx_STREAM DMA1_Stream5 00123 #define AUDIO_OUT_I2Sx_DMAx_CHANNEL DMA_CHANNEL_0 00124 #define AUDIO_OUT_I2Sx_DMAx_IRQ DMA1_Stream5_IRQn 00125 #define AUDIO_OUT_I2Sx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00126 #define AUDIO_OUT_I2Sx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00127 #define DMA_MAX_SIZE 0xFFFF 00128 00129 #define AUDIO_OUT_I2Sx_DMAx_IRQHandler DMA1_Stream5_IRQHandler 00130 00131 /* Select the interrupt preemption priority for the DMA interrupt */ 00132 #define AUDIO_OUT_IRQ_PREPRIO ((uint32_t)0x0E) /* Select the preemption priority level(0 is the highest) */ 00133 00134 00135 /*------------------------------------------------------------------------------ 00136 AUDIO IN CONFIGURATION 00137 ------------------------------------------------------------------------------*/ 00138 /* DFSDM Configuration defines */ 00139 #define AUDIO_DFSDMx_MIC1_CHANNEL DFSDM1_Channel0 /* MP34DT01TR microphone on PCB top side */ 00140 #define AUDIO_DFSDMx_MIC2_CHANNEL DFSDM1_Channel3 /* MP34DT01TR microphone on PCB top side */ 00141 #define AUDIO_DFSDMx_MIC1_CHANNEL_FOR_FILTER DFSDM_CHANNEL_0 00142 #define AUDIO_DFSDMx_MIC2_CHANNEL_FOR_FILTER DFSDM_CHANNEL_3 00143 #define AUDIO_DFSDMx_MIC1_FILTER DFSDM1_Filter0 /* Common filter for MP34DT01TR microphone input */ 00144 #define AUDIO_DFSDMx_MIC2_FILTER DFSDM1_Filter1 /* Common filter for MP34DT01TR microphone input */ 00145 #define AUDIO_DFSDMx_CLK_ENABLE() __HAL_RCC_DFSDM1_CLK_ENABLE() 00146 #define AUDIO_DFSDMx_CKOUT_PIN GPIO_PIN_2 00147 #define AUDIO_DFSDMx_CKOUT_DMIC_GPIO_PORT GPIOC 00148 #define AUDIO_DFSDMx_CKOUT_DMIC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00149 #define AUDIO_DFSDMx_CKOUT_DMIC_AF GPIO_AF8_DFSDM1 00150 #define AUDIO_DFSDMx_DMIC_PIN GPIO_PIN_1 00151 #define AUDIO_DFSDMx_DMIC_GPIO_PORT GPIOB 00152 #define AUDIO_DFSDMx_DMIC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00153 #define AUDIO_DFSDMx_DMIC_AF GPIO_AF8_DFSDM1 00154 00155 /* DFSDM DMA MIC1 and MIC2 channels definitions */ 00156 #define AUDIO_DFSDMx_DMAx_CLK_ENABLE() __HAL_RCC_DMA2_CLK_ENABLE() 00157 #define AUDIO_DFSDMx_DMAx_MIC1_STREAM DMA2_Stream6 00158 #define AUDIO_DFSDMx_DMAx_MIC1_CHANNEL DMA_CHANNEL_3 00159 #define AUDIO_DFSDMx_DMAx_MIC2_STREAM DMA2_Stream4 00160 #define AUDIO_DFSDMx_DMAx_MIC2_CHANNEL DMA_CHANNEL_3 00161 #define AUDIO_DFSDMx_DMAx_MIC1_IRQ DMA2_Stream6_IRQn 00162 #define AUDIO_DFSDMx_DMAx_MIC2_IRQ DMA2_Stream4_IRQn 00163 #define AUDIO_DFSDMx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_WORD 00164 #define AUDIO_DFSDMx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_WORD 00165 00166 #define AUDIO_DFSDM_DMAx_MIC1_IRQHandler DMA2_Stream6_IRQHandler 00167 #define AUDIO_DFSDM_DMAx_MIC2_IRQHandler DMA2_Stream4_IRQHandler 00168 00169 /* Select the interrupt preemption priority and subpriority for the IT/DMA interrupt */ 00170 #define AUDIO_IN_IRQ_PREPRIO 0x0F /* Select the preemption priority level(0 is the highest) */ 00171 00172 /* HW defines for Analog mic configuration */ 00173 #define AUDIO_IN_I2Sx SPI3 00174 #define AUDIO_IN_I2Sx_CLK_ENABLE() __HAL_RCC_SPI3_CLK_ENABLE() 00175 #define AUDIO_IN_I2Sx_CLK_DISABLE() __HAL_RCC_SPI3_CLK_DISABLE() 00176 00177 #define AUDIO_IN_I2Sx_EXT_SD_PIN GPIO_PIN_4 00178 #define AUDIO_IN_I2Sx_EXT_SD_GPIO_PORT GPIOB 00179 #define AUDIO_IN_I2Sx_EXT_SD_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00180 #define AUDIO_IN_I2Sx_EXT_SD_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00181 #define AUDIO_IN_I2Sx_EXT_SD_AF GPIO_AF7_SPI3 00182 00183 #if 0 00184 #define AUDIO_IN_CODEC_INT_PIN GPIO_PIN_2 00185 #define AUDIO_IN_CODEC_INT_GPIO_PORT GPIOG 00186 #define AUDIO_IN_CODEC_INT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00187 #define AUDIO_IN_CODEC_INT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOG_CLK_DISABLE() 00188 #define AUDIO_IN_CODEC_INT_IRQ EXTI2_IRQn 00189 #endif 00190 00191 /* I2S DMA Stream Rx definitions */ 00192 #define AUDIO_IN_I2Sx_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00193 #define AUDIO_IN_I2Sx_DMAx_CLK_DISABLE() __HAL_RCC_DMA1_CLK_DISABLE() 00194 #define AUDIO_IN_I2Sx_DMAx_STREAM DMA1_Stream0 00195 #define AUDIO_IN_I2Sx_DMAx_CHANNEL DMA_CHANNEL_3 00196 #define AUDIO_IN_I2Sx_DMAx_IRQ DMA1_Stream0_IRQn 00197 #define AUDIO_IN_I2Sx_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00198 #define AUDIO_IN_I2Sx_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00199 00200 #define AUDIO_IN_I2Sx_DMAx_IRQHandler DMA1_Stream0_IRQHandler 00201 #define AUDIO_IN_I2Sx_DMAx_IRQ DMA1_Stream0_IRQn 00202 00203 /* Two channels are used: 00204 - one channel as input which is connected to I2S SCK in stereo mode 00205 - one channel as output which divides the frequency on the input 00206 */ 00207 00208 #define AUDIO_TIMx_CLK_ENABLE() __HAL_RCC_TIM4_CLK_ENABLE() 00209 #define AUDIO_TIMx_CLK_DISABLE() __HAL_RCC_TIM4_CLK_DISABLE() 00210 #define AUDIO_TIMx TIM4 00211 #define AUDIO_TIMx_IN_CHANNEL TIM_CHANNEL_1 00212 #define AUDIO_TIMx_OUT_CHANNEL TIM_CHANNEL_2 /* Select channel 2 as output */ 00213 #define AUDIO_TIMx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00214 #define AUDIO_TIMx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00215 #define AUDIO_TIMx_GPIO_PORT GPIOB 00216 #define AUDIO_TIMx_IN_GPIO_PIN GPIO_PIN_6 00217 #define AUDIO_TIMx_OUT_GPIO_PIN GPIO_PIN_7 00218 #define AUDIO_TIMx_AF GPIO_AF2_TIM4 00219 00220 /*------------------------------------------------------------------------------ 00221 CONFIGURATION: Audio Driver Configuration parameters 00222 ------------------------------------------------------------------------------*/ 00223 00224 #define AUDIODATA_SIZE 2 /* 16-bits audio data size */ 00225 00226 /* Audio status definition */ 00227 #define AUDIO_OK ((uint8_t)0) 00228 #define AUDIO_ERROR ((uint8_t)1) 00229 #define AUDIO_TIMEOUT ((uint8_t)2) 00230 00231 /* Audio out parameters */ 00232 #define DEFAULT_AUDIO_OUT_FREQ I2S_AUDIOFREQ_48K 00233 #define DEFAULT_AUDIO_OUT_BIT_RESOLUTION ((uint8_t)16) 00234 #define DEFAULT_AUDIO_OUT_CHANNEL_NBR ((uint8_t)2) /* Mono = 1, Stereo = 2 */ 00235 #define DEFAULT_AUDIO_OUT_VOLUME ((uint16_t)64) 00236 00237 /* Audio in parameters */ 00238 #define DEFAULT_AUDIO_IN_FREQ I2S_AUDIOFREQ_16K 00239 #define DEFAULT_AUDIO_IN_BIT_RESOLUTION ((uint8_t)16) 00240 #define DEFAULT_AUDIO_IN_CHANNEL_NBR ((uint8_t)2) /* Mono = 1, Stereo = 2 */ 00241 #define DEFAULT_AUDIO_IN_VOLUME ((uint16_t)64) 00242 00243 /*------------------------------------------------------------------------------ 00244 OUTPUT DEVICES definition 00245 ------------------------------------------------------------------------------*/ 00246 00247 /* Alias on existing output devices to adapt for 2 headphones output */ 00248 #define OUTPUT_DEVICE_HEADPHONE1 OUTPUT_DEVICE_HEADPHONE 00249 #define OUTPUT_DEVICE_HEADPHONE2 OUTPUT_DEVICE_SPEAKER /* Headphone2 is connected to Speaker output of the wm8994 */ 00250 00251 /*------------------------------------------------------------------------------ 00252 INPUT DEVICES definition 00253 ------------------------------------------------------------------------------*/ 00254 /* Analog microphone input from 3.5 audio jack connector */ 00255 #define INPUT_DEVICE_ANALOG_MIC ((uint32_t)0x00000001) 00256 /* MP34DT01TR digital microphone on PCB top side */ 00257 #define INPUT_DEVICE_DIGITAL_MIC1 ((uint32_t)0x00000010) 00258 #define INPUT_DEVICE_DIGITAL_MIC2 ((uint32_t)0x00000020) 00259 #define INPUT_DEVICE_DIGITAL_MIC ((uint32_t)(INPUT_DEVICE_DIGITAL_MIC1 | INPUT_DEVICE_DIGITAL_MIC2)) 00260 #define DFSDM_MIC_NUMBER (2) 00261 /** 00262 * @} 00263 */ 00264 00265 /** @defgroup STM32412G_DISCOVERY_AUDIO_Exported_Variables STM32412G DISCOVERY Audio Exported Variables 00266 * @{ 00267 */ 00268 extern __IO uint16_t AudioInVolume; 00269 /** 00270 * @} 00271 */ 00272 00273 /** @defgroup STM32412G_DISCOVERY_AUDIO_Exported_Macros STM32412G DISCOVERY Audio Exported Macros 00274 * @{ 00275 */ 00276 #define DMA_MAX(x) (((x) <= DMA_MAX_SIZE)? (x):DMA_MAX_SIZE) 00277 #define POS_VAL(VAL) (POSITION_VAL(VAL) - 4) 00278 /** 00279 * @} 00280 */ 00281 00282 /** @defgroup STM32412G_DISCOVERY_AUDIO_OUT_Exported_Functions STM32412G DISCOVERY AUDIO OUT Exported Functions 00283 * @{ 00284 */ 00285 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00286 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00287 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00288 uint8_t BSP_AUDIO_OUT_Pause(void); 00289 uint8_t BSP_AUDIO_OUT_Resume(void); 00290 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00291 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00292 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00293 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd); 00294 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00295 void BSP_AUDIO_OUT_DeInit(void); 00296 00297 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00298 /* This function is called when the requested data has been completely transferred.*/ 00299 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00300 00301 /* This function is called when half of the requested buffer has been transferred. */ 00302 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00303 00304 /* This function is called when an Interrupt due to transfer error on or peripheral 00305 error occurs. */ 00306 void BSP_AUDIO_OUT_Error_CallBack(void); 00307 00308 /* These function can be modified in case the current settings (e.g. DMA stream) 00309 need to be changed for specific application needs */ 00310 void BSP_AUDIO_OUT_ClockConfig(I2S_HandleTypeDef *hi2s, uint32_t AudioFreq, void *Params); 00311 void BSP_AUDIO_OUT_MspInit(I2S_HandleTypeDef *hi2s, void *Params); 00312 void BSP_AUDIO_OUT_MspDeInit(I2S_HandleTypeDef *hi2s, void *Params); 00313 00314 /** 00315 * @} 00316 */ 00317 00318 /** @defgroup STM32412G_DISCOVERY_AUDIO_IN_Exported_Functions STM32412G DISCOVERY AUDIO IN Exported Functions 00319 * @{ 00320 */ 00321 uint8_t BSP_AUDIO_IN_Init(uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00322 uint8_t BSP_AUDIO_IN_InitEx(uint32_t InputDevice, uint32_t AudioFreq, uint32_t BitRes, uint32_t ChnlNbr); 00323 uint8_t BSP_AUDIO_IN_ConfigMicDefault(uint32_t InputDevice); 00324 uint8_t BSP_AUDIO_IN_ConfigDigitalMic(uint32_t InputDevice, void *Params); 00325 uint8_t BSP_AUDIO_IN_AllocScratch (int32_t *pScratch, uint32_t size); 00326 uint8_t BSP_AUDIO_IN_Record(uint16_t *pBuf, uint32_t Size); 00327 uint8_t BSP_AUDIO_IN_RecordEx(uint32_t *pBuf, uint32_t Size); 00328 uint8_t BSP_AUDIO_IN_SetFrequency(uint32_t AudioFreq); 00329 uint8_t BSP_AUDIO_IN_Stop(void); 00330 uint8_t BSP_AUDIO_IN_StopEx(uint32_t InputDevice); 00331 uint8_t BSP_AUDIO_IN_Pause(void); 00332 uint8_t BSP_AUDIO_IN_PauseEx(uint32_t InputDevice); 00333 uint8_t BSP_AUDIO_IN_Resume(void); 00334 uint8_t BSP_AUDIO_IN_ResumeEx(uint32_t *pBuf, uint32_t InputDevice); 00335 uint8_t BSP_AUDIO_IN_SetVolume(uint8_t Volume); 00336 void BSP_AUDIO_IN_DeInit(void); 00337 00338 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00339 /* This function should be implemented by the user application. 00340 It is called into this driver when the current buffer is filled to prepare the next 00341 buffer pointer and its size. */ 00342 void BSP_AUDIO_IN_TransferComplete_CallBack(void); 00343 void BSP_AUDIO_IN_HalfTransfer_CallBack(void); 00344 void BSP_AUDIO_IN_TransferComplete_CallBackEx(uint32_t InputDevice); 00345 void BSP_AUDIO_IN_HalfTransfer_CallBackEx(uint32_t InputDevice); 00346 00347 /* This function is called when an Interrupt due to transfer error on or peripheral 00348 error occurs. */ 00349 void BSP_AUDIO_IN_Error_Callback(void); 00350 00351 /* These function can be modified in case the current settings (e.g. DMA stream) 00352 need to be changed for specific application needs */ 00353 uint8_t BSP_AUDIO_IN_ClockConfig(uint32_t AudioFreq, void *Params); 00354 void BSP_AUDIO_IN_MspInit(void *Params); 00355 void BSP_AUDIO_IN_MspDeInit(void *Params); 00356 00357 /** 00358 * @} 00359 */ 00360 00361 /** 00362 * @} 00363 */ 00364 00365 /** 00366 * @} 00367 */ 00368 00369 /** 00370 * @} 00371 */ 00372 00373 #ifdef __cplusplus 00374 } 00375 #endif 00376 00377 #endif /* __STM32412G_DISCOVERY_AUDIO_H */ 00378 00379 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jan 24 2017 11:28:05 for STM32412G-Discovery BSP User Manual by
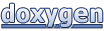