STM32373C_EVAL BSP User Manual
|
stm32373c_eval_eeprom.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32373c_eval_eeprom.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage a M24LR64 00006 * or M24M01 I2C EEPROM memory. 00007 * 00008 * =================================================================== 00009 * Notes: 00010 * - This driver is intended for STM32F37x families devices only. 00011 * - The I2C EEPROM memory (M24LR64) is available on separate daughter 00012 * board ANT7-M24LR-A, which is provided with the STM32F373C 00013 * EVAL board. 00014 * To use this driver with M24LR64, you have to connect 00015 * the ANT7-M24LR-A to CN1 connector of STM32F373C EVAL board. 00016 * Jumpers JP4 and JP5 connected on 1-2 (left side) 00017 * 00018 * - The I2C EEPROM memory (M24M01) is mounted on the STM32F373C 00019 * EVAL board. 00020 * Jumpers JP4 and JP5 connected on 2-3 (right side) 00021 * =================================================================== 00022 * 00023 * It implements a high level communication layer for read and write 00024 * from/to this memory. The needed STM32F37x hardware resources (I2C and 00025 * GPIO) are defined in stm32373c_eval.h file, and the initialization is 00026 * performed in EEPROM_IO_Init() function declared in stm32373c_eval.c 00027 * file. 00028 * You can easily tailor this driver to any other development board, 00029 * by just adapting the defines for hardware resources and 00030 * EEPROM_IO_Init() function. 00031 * 00032 * @note In this driver, basic read and write functions 00033 * (BSP_EEPROM_ReadBuffer() and BSP_EEPROM_WriteBuffer()) 00034 * use Polling mode to perform the data transfer to/from EEPROM memories. 00035 * +-----------------------------------------------------------------+ 00036 * | Pin assignment for M24M01 EEPROM | 00037 * +---------------------------------------+-----------+-------------+ 00038 * | STM32F37x I2C Pins | sEE | Pin | 00039 * +---------------------------------------+-----------+-------------+ 00040 * | . | NC | 1 | 00041 * | . | E1(VDD) | 2 (3.3V) | 00042 * | . | E2(GND) | 3 (0V) | 00043 * | . | VSS | 4 (0V) | 00044 * | sEE_I2C_SDA_PIN/ SDA | SDA | 5 | 00045 * | sEE_I2C_SCL_PIN/ SCL | SCL | 6 | 00046 * | . | /WC(VSS)| 7 (0V) | 00047 * | . | VDD | 8 (3.3V) | 00048 * +---------------------------------------+-----------+-------------+ 00049 * +-----------------------------------------------------------------+ 00050 * | Pin assignment for M24LR64 EEPROM | 00051 * +---------------------------------------+-----------+-------------+ 00052 * | STM32F37x I2C Pins | sEE | Pin | 00053 * +---------------------------------------+-----------+-------------+ 00054 * | . | E0(GND) | 1 (0V) | 00055 * | . | AC0 | 2 | 00056 * | . | AC1 | 3 | 00057 * | . | VSS | 4 (0V) | 00058 * | sEE_I2C_SDA_PIN/ SDA | SDA | 5 | 00059 * | sEE_I2C_SCL_PIN/ SCL | SCL | 6 | 00060 * | . | E1(GND) | 7 (0V) | 00061 * | . | VDD | 8 (3.3V) | 00062 * +---------------------------------------+-----------+-------------+ 00063 * 00064 ****************************************************************************** 00065 * @attention 00066 * 00067 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00068 * 00069 * Redistribution and use in source and binary forms, with or without modification, 00070 * are permitted provided that the following conditions are met: 00071 * 1. Redistributions of source code must retain the above copyright notice, 00072 * this list of conditions and the following disclaimer. 00073 * 2. Redistributions in binary form must reproduce the above copyright notice, 00074 * this list of conditions and the following disclaimer in the documentation 00075 * and/or other materials provided with the distribution. 00076 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00077 * may be used to endorse or promote products derived from this software 00078 * without specific prior written permission. 00079 * 00080 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00081 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00082 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00083 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00084 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00085 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00086 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00087 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00088 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00089 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00090 * 00091 ****************************************************************************** 00092 */ 00093 /* Includes ------------------------------------------------------------------*/ 00094 #include "stm32373c_eval_eeprom.h" 00095 00096 /** @addtogroup BSP 00097 * @{ 00098 */ 00099 00100 /** @addtogroup STM32373C_EVAL 00101 * @{ 00102 */ 00103 00104 /** @addtogroup STM32373C_EVAL_EEPROM 00105 * @brief This file includes the I2C EEPROM driver 00106 * of STM32373C-EVAL board. 00107 * @{ 00108 */ 00109 00110 /** @addtogroup STM32373C_EVAL_EEPROM_Private_Variables 00111 * @{ 00112 */ 00113 00114 /** @defgroup Private_Types Private_Types 00115 * @{ 00116 */ 00117 /** 00118 * @} 00119 */ 00120 00121 /** @addtogroup STM32373C_EVAL_EEPROM_Private_Functions 00122 * @{ 00123 */ 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup STM32373C_EVAL_EEPROM_Private_Types Private Types 00129 * @{ 00130 */ 00131 00132 /** @defgroup Private_Variables Private_Variables 00133 * @{ 00134 */ 00135 __IO uint16_t EEPROMAddress = 0; 00136 __IO uint16_t EEPROMPageSize = 0; 00137 /** 00138 * @} 00139 */ 00140 00141 00142 /** @defgroup Private_Function_Prototypes Private_Function_Prototypes 00143 * @{ 00144 */ 00145 static uint32_t EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite); 00146 /** 00147 * @} 00148 */ 00149 00150 /** @addtogroup STM32373C_EVAL_EEPROM_Exported_Functions 00151 * @{ 00152 */ 00153 00154 /** 00155 * @brief Initializes peripherals used by the I2C EEPROM driver. 00156 * 00157 * @note There are 2 different versions of EEPROM (A01 & A02). 00158 * Then try to connect on 1st one (EEPROM_I2C_ADDRESS_A01) 00159 * and if problem, check the 2nd one (EEPROM_I2C_ADDRESS_A02) 00160 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00161 * different from EEPROM_OK (0) 00162 */ 00163 uint32_t BSP_EEPROM_Init(void) 00164 { 00165 EEPROM_IO_Init(); 00166 00167 /*Select the EEPROM address for M24LR64 A01 and check if OK*/ 00168 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A01; 00169 EEPROMPageSize = EEPROM_PAGESIZE_M24LR64; 00170 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00171 { 00172 /*Select the EEPROM address for M24LR64 A02 and check if OK*/ 00173 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A02; 00174 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00175 { 00176 /*Select the EEPROM address for M24M01 A01 and check if OK*/ 00177 EEPROMAddress = EEPROM_ADDRESS_M24M01_A01; 00178 EEPROMPageSize = EEPROM_PAGESIZE_M24M01; 00179 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00180 { 00181 /*Select the EEPROM address for M24M01 A02 and check if OK*/ 00182 EEPROMAddress = EEPROM_ADDRESS_M24M01_A02; 00183 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00184 { 00185 return EEPROM_FAIL; 00186 } 00187 } 00188 } 00189 } 00190 00191 return EEPROM_OK; 00192 } 00193 00194 /** 00195 * @brief Reads a block of data from the EEPROM. 00196 * @param pBuffer pointer to the buffer that receives the data read from 00197 * the EEPROM. 00198 * @param ReadAddr EEPROM's internal address to start reading from. 00199 * @param NumByteToRead pointer to the variable holding number of bytes to 00200 * be read from the EEPROM. 00201 * 00202 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00203 * data are read from the EEPROM. Application should monitor this 00204 * variable in order know when the transfer is complete. 00205 * 00206 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00207 * different from EEPROM_OK (0) or the timeout user callback. 00208 */ 00209 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00210 { 00211 uint32_t buffersize = *NumByteToRead; 00212 00213 if (EEPROM_IO_ReadData(EEPROMAddress, ReadAddr, pBuffer, buffersize) != HAL_OK) 00214 { 00215 return EEPROM_FAIL; 00216 } 00217 00218 /* If all operations OK, return EEPROM_OK (0) */ 00219 return EEPROM_OK; 00220 } 00221 00222 /** 00223 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00224 * 00225 * @note The number of bytes (combined to write start address) must not 00226 * cross the EEPROM page boundary. This function can only write into 00227 * the boundaries of an EEPROM page. 00228 * This function doesn't check on boundaries condition (in this driver 00229 * the function EEPROM_WriteBuffer() which calls EEPROM_WritePage() is 00230 * responsible of checking on Page boundaries). 00231 * 00232 * @param pBuffer pointer to the buffer containing the data to be written to 00233 * the EEPROM. 00234 * @param WriteAddr EEPROM's internal address to write to. 00235 * @param NumByteToWrite pointer to the variable holding number of bytes to 00236 * be written into the EEPROM. 00237 * 00238 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00239 * data are written to the EEPROM. Application should monitor this 00240 * variable in order know when the transfer is complete. 00241 * 00242 * @note This function just configure the communication and enable the DMA 00243 * channel to transfer data. Meanwhile, the user application may perform 00244 * other tasks in parallel. 00245 * 00246 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00247 * different from EEPROM_OK (0) or the timeout user callback. 00248 */ 00249 static uint32_t EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite) 00250 { 00251 uint32_t buffersize = *NumByteToWrite; 00252 00253 if (EEPROM_IO_WriteData(EEPROMAddress, WriteAddr, pBuffer, buffersize) != HAL_OK) 00254 { 00255 return EEPROM_FAIL; 00256 } 00257 00258 /* Wait for EEPROM Standby state */ 00259 if (BSP_EEPROM_WaitEepromStandbyState() != EEPROM_OK) 00260 { 00261 return EEPROM_FAIL; 00262 } 00263 00264 /* If all operations OK, return EEPROM_OK (0) */ 00265 return EEPROM_OK; 00266 } 00267 00268 /** 00269 * @brief Writes buffer of data to the I2C EEPROM. 00270 * @param pBuffer pointer to the buffer containing the data to be written 00271 * to the EEPROM. 00272 * @param WriteAddr EEPROM's internal address to write to. 00273 * @param NumByteToWrite number of bytes to write to the EEPROM. 00274 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00275 * different from EEPROM_OK (0) or the timeout user callback. 00276 */ 00277 uint32_t BSP_EEPROM_WriteBuffer(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t NumByteToWrite) 00278 { 00279 uint16_t numofpage = 0, numofsingle = 0, count = 0; 00280 uint16_t addr = 0; 00281 uint32_t dataindex = 0; 00282 uint32_t status = EEPROM_OK; 00283 00284 addr = WriteAddr % EEPROMPageSize; 00285 count = EEPROMPageSize - addr; 00286 numofpage = NumByteToWrite / EEPROMPageSize; 00287 numofsingle = NumByteToWrite % EEPROMPageSize; 00288 00289 /*!< If WriteAddr is EEPROM_PAGESIZE aligned */ 00290 if(addr == 0) 00291 { 00292 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00293 if(numofpage == 0) 00294 { 00295 /* Store the number of data to be written */ 00296 dataindex = numofsingle; 00297 /* Start writing data */ 00298 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00299 if (status != EEPROM_OK) 00300 { 00301 return status; 00302 } 00303 } 00304 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00305 else 00306 { 00307 while(numofpage--) 00308 { 00309 /* Store the number of data to be written */ 00310 dataindex = EEPROMPageSize; 00311 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00312 if (status != EEPROM_OK) 00313 { 00314 return status; 00315 } 00316 00317 WriteAddr += EEPROMPageSize; 00318 pBuffer += EEPROMPageSize; 00319 } 00320 00321 if(numofsingle!=0) 00322 { 00323 /* Store the number of data to be written */ 00324 dataindex = numofsingle; 00325 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00326 if (status != EEPROM_OK) 00327 { 00328 return status; 00329 } 00330 } 00331 } 00332 } 00333 /*!< If WriteAddr is not EEPROM_PAGESIZE aligned */ 00334 else 00335 { 00336 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00337 if(numofpage== 0) 00338 { 00339 /*!< If the number of data to be written is more than the remaining space 00340 in the current page: */ 00341 if (NumByteToWrite > count) 00342 { 00343 /* Store the number of data to be written */ 00344 dataindex = count; 00345 /*!< Write the data contained in same page */ 00346 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00347 if (status != EEPROM_OK) 00348 { 00349 return status; 00350 } 00351 00352 /* Store the number of data to be written */ 00353 dataindex = (NumByteToWrite - count); 00354 /*!< Write the remaining data in the following page */ 00355 status = EEPROM_WritePage((uint8_t*)(pBuffer + count), (WriteAddr + count), (uint32_t*)(&dataindex)); 00356 if (status != EEPROM_OK) 00357 { 00358 return status; 00359 } 00360 } 00361 else 00362 { 00363 /* Store the number of data to be written */ 00364 dataindex = numofsingle; 00365 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00366 if (status != EEPROM_OK) 00367 { 00368 return status; 00369 } 00370 } 00371 } 00372 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00373 else 00374 { 00375 NumByteToWrite -= count; 00376 numofpage = NumByteToWrite / EEPROMPageSize; 00377 numofsingle = NumByteToWrite % EEPROMPageSize; 00378 00379 if(count != 0) 00380 { 00381 /* Store the number of data to be written */ 00382 dataindex = count; 00383 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00384 if (status != EEPROM_OK) 00385 { 00386 return status; 00387 } 00388 WriteAddr += count; 00389 pBuffer += count; 00390 } 00391 00392 while(numofpage--) 00393 { 00394 /* Store the number of data to be written */ 00395 dataindex = EEPROMPageSize; 00396 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00397 if (status != EEPROM_OK) 00398 { 00399 return status; 00400 } 00401 WriteAddr += EEPROMPageSize; 00402 pBuffer += EEPROMPageSize; 00403 } 00404 if(numofsingle != 0) 00405 { 00406 /* Store the number of data to be written */ 00407 dataindex = numofsingle; 00408 status = EEPROM_WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00409 if (status != EEPROM_OK) 00410 { 00411 return status; 00412 } 00413 } 00414 } 00415 } 00416 00417 /* If all operations OK, return EEPROM_OK (0) */ 00418 return EEPROM_OK; 00419 } 00420 00421 /** 00422 * @brief Wait for EEPROM Standby state. 00423 * 00424 * @note This function allows to wait and check that EEPROM has finished the 00425 * last operation. It is mostly used after Write operation: after receiving 00426 * the buffer to be written, the EEPROM may need additional time to actually 00427 * perform the write operation. During this time, it doesn't answer to 00428 * I2C packets addressed to it. Once the write operation is complete 00429 * the EEPROM responds to its address. 00430 * 00431 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00432 * different from EEPROM_OK (0) or the timeout user callback. 00433 */ 00434 uint32_t BSP_EEPROM_WaitEepromStandbyState(void) 00435 { 00436 /* Check if the maximum allowed number of trials has bee reached */ 00437 if (EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00438 { 00439 /* If the maximum number of trials has been reached, exit the function */ 00440 BSP_EEPROM_TIMEOUT_UserCallback(); 00441 return EEPROM_TIMEOUT; 00442 } 00443 return EEPROM_OK; 00444 } 00445 00446 /** 00447 * @brief Basic management of the timeout situation. 00448 * @retval None. 00449 */ 00450 __weak void BSP_EEPROM_TIMEOUT_UserCallback(void) 00451 { 00452 } 00453 00454 /** 00455 * @} 00456 */ 00457 00458 /** 00459 * @} 00460 */ 00461 00462 /** 00463 * @} 00464 */ 00465 00466 /** 00467 * @} 00468 */ 00469 00470 /** 00471 * @} 00472 */ 00473 00474 /** 00475 * @} 00476 */ 00477 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 11:20:44 for STM32373C_EVAL BSP User Manual by
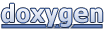