OSX MotionFX Software Library
|
osx_motion_fx.h
Go to the documentation of this file.
214 void osx_MotionFX_update(osxMFX_output *data_out, osxMFX_input *data_in, float eml_deltatime, float *eml_q_update);
223 void osx_MotionFX_propagate(osxMFX_output *data_out, osxMFX_input *data_in, float eml_deltatime);
Definition: osx_motion_fx.h:111
void osx_MotionFX_getCalibrationData(osxMFX_calibFactor *CalibrationData)
Get calibration data.
Definition: osx_motion_fx.h:82
void osx_MotionFX_enable_6X(osxMFX_Engine_State enable)
Enable or disable the 6 axes function (ACC + GYRO)
void osx_MotionFX_compass_init(void)
Initialize the compass calibration library.
Definition: osx_motion_fx.h:125
void osx_MotionFX_update(osxMFX_output *data_out, osxMFX_input *data_in, float eml_deltatime, float *eml_q_update)
Run the Kalman filter update.
void osx_MotionFX_setCalibrationData(osxMFX_calibFactor *CalibrationData)
Set calibration data.
osxMFX_Engine_State osx_MotionFX_getStatus_6X(void)
Get the status of the 6 axes library.
void osx_MotionFX_compass_forceReCalibration(void)
Force new calibration.
unsigned char osx_MotionFX_compass_isCalibrated(void)
Check if calibration is needed.
osxMFX_Engine_State osx_MotionFX_getStatus_9X(void)
Get the status of the 9 axes library.
Definition: osx_motion_fx.h:104
void osx_MotionFX_compass_saveMag(int mag_x, int mag_y, int mag_z)
Save magnetometer data ENU systems coordinate.
void osx_MotionFX_propagate(osxMFX_output *data_out, osxMFX_input *data_in, float eml_deltatime)
Run the Kalman filter propagate.
void osx_MotionFX_compass_saveAcc(int acc_x, int acc_y, int acc_z)
Save accelerometer data ENU systems coordinate.
void osx_MotionFX_enable_9X(osxMFX_Engine_State enable)
Enable or disable the 9 axes function (ACC + GYRO + MAG)
Generated on Tue Apr 5 2016 17:29:15 for OSX MotionFX Software Library by
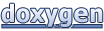