ODBJscript
javascript plugin
|
Go to the source code of this file.
Enumerations | |
enum | e_exceptmask { IGNORE_NONE = 0x00000000, IGNORE_ACCESS_KER32 = 0x00000001, IGNORE_INT3 = 0x00000002, IGNORE_TRAP = 0x00000004, IGNORE_ACCESS = 0x00000010, IGNORE_DIV_ZERO = 0x00000020, IGNORE_ILLEGAL_INST = 0x00000040, IGNORE_FPU_EXCEPT = 0x00000100, IGNORE_CUSTOM_EXCEPT = 0x00000200, IGNORE_MANUALLY = 0xFFFFFFFF, IGNORE_ALL = IGNORE_ACCESS_KER32|IGNORE_INT3|IGNORE_TRAP|IGNORE_ACCESS|IGNORE_DIV_ZERO|IGNORE_ILLEGAL_INST|IGNORE_FPU_EXCEPT|IGNORE_CUSTOM_EXCEPT } |
enum | e_memoryinfo { MEMORYBASE, MEMORYSIZE, MEMORYOWNER } |
enum | t_moduleinfo { MODULEBASE, MODULESIZE, CODEBASE, CODESIZE, ENTRY, NSECT, DATABASE, EDATATABLE, EDATASIZE, IDATATABLE, RESBASE, RESSIZE, RELOCTABLE, RELOCSIZE } |
enum | e_cmdinfo { COMMAND, CONDITION, DESTINATION, FINALDEST, SIZE, TYPE } |
enum | e_dumptype { DU_HEXTEXT = 0x01000L, DU_TEXT = 0x02000L, DU_UNICODE = 0x03000L, DU_INT = 0x04000L, DU_UINT = 0x05000L, DU_IHEX = 0x06000L, DU_FLOAT = 0x07000L, DU_ADDR = 0x08000L, DU_DISASM = 0x09000L, DU_HEXUNI = 0x0A000L, DU_ADRASC = 0x0B000L, DU_ADRUNI = 0x0C000L } |
enum | e_processinfo { HPROCESS = 20, PROCESSID, HMAINTHREAD, MAINTHREADID, MAINBASE, PROCESSNAME, EXEFILENAME, CURRENTDIR, SYSTEMDIR } |
enum | e_origin { CPUDASM, CPUDUMP, CPUSTACK } |
Functions | |
ulong | DD (ulong addr) |
read dword from address addr | |
ulong | DW (ulong addr) |
read dword from address addr | |
ulong | DB (ulong addr) |
read dword from address addr | |
ulong | SETOPTION (DWORD mask) |
sets/unsets exception to be ignored by ollydbg. | |
void | EVAL (void) |
void | MSGYN (void) |
void | MSG (void) |
void | REFRESH (bool onoff) |
sets on/off refreshing ollydbg MDI windows. | |
void | LOGBUF (ulong ip, size_t size, int nepl=32, char *separator="") |
logs size bytes of memory beginning from address ip. | |
void | LOG (...) |
logs any data to log window. | |
void | TICND (char *cond, ulong in0, ulong in1, ulong out0, ulong out1) |
Traces into calls until cond is true and/or on EIP in range [in0-in1] and/or out of range [out0-out1]. | |
void | BPGOTO (ulong addr, FUNC *proc) |
Installs a callback function that will be called each time breakpoint at address addr is hit. | |
void | EOB (FUNC *proc) |
installs a callbacks function that will be called every time a breakpoint is hit. | |
void | EOE (FUNC *proc) |
installs a callbacks function that will be called every time an exception occures. | |
void | COB () |
void | COE () |
void | BPX (char *procname, DWORD addr=0) |
set bp on every caller of label procname | |
void | BPD (char *procname) |
void | GBPM () |
ulong | FIND (ulong addr, char *what) |
search for a pattern of bytes starting from address addr | |
ulong | MOV (ulong addr, char *what, int n=lenght_of_what) |
writes n or all element of what to address addr | |
ulong | MOVS (ulong addr, char *str, int n=lenght_of_what) |
writes n or all characters of string str to address addr | |
ulong | FINDO (ulong addr, char *what, int blocklen, func callback,...) |
stands for find-do, it allows you to call a callback function eatch time the pattern is found. | |
ulong | PREOP (ulong addr) |
Get asm command line address just before specified address. | |
ulong | GCI (ulong addr, int info) |
stands for get command information | |
void | KEY (int vkcode, bool shift=false, bool ctrl=false, int origin=PM_DISASM) |
void | OPENDUMP (ulong addr, ulong base, ulong size, int type=Hex/ASCII) |
ulong | BUF (var) |
ulong | CLOSE (window) |
ulong | DBH (void) |
ulong | DBS (void) |
ulong | DPE (char *filename, ulong ep) |
ulong | ENDE (void) |
void | ENDSEARCH (void) |
void | EXEC (void) |
ulong | GAPI (ulong addr) |
ulong | GMA (char *name, int info) |
returns information about module named name | |
ulong | GMEXP (ulong moduleaddr, int info,[int num]) |
char * | GN (ulong addr) |
ulong | GREF ([int line]) |
ulong | GRO (ulong addr) |
char * | GSTR (ulong addr[, int len]) |
return string of length len from memory addr | |
uchar * | GSTRW (ulong addr[, int len]) |
return unicode string of length len from memory addr | |
ulong | LM (ulong addr, int size, char *filename) |
ulong | LOADLIB (char *dllname) |
ulong | MEMCPY (ulong dest, ulong src, int size) |
ulong | NAMES (ulong addr) |
ulong | OLLY (int info) |
void | PAUSE (void) |
void | POPA (void) |
void | PUSHA (void) |
void | STR (var) |
void | AI (void) |
void | AO (void) |
void | ERUN (void) |
void | ESTI (void) |
void | GO (ulong addr) |
void | RTR (void) |
void | RTU (void) |
void | RUN (void) |
void | STI (void) |
void | STO (void) |
void | TI (void) |
void | TO (void) |
void | TOCND (char *cond) |
Traces over calls until cond is true and/or on EIP in range [in0-in1] and/or out of range [out0-out1]. | |
void | CMT (char *comment) |
void | AN (ulong addr) |
void | BC (ulong addr) |
void | BD (ulong addr) |
void | BE (ulong addr) |
void | BP (ulong addr) |
void | BPCND (ulong addr, char *cond) |
void | BPLCND (ulong addr, char *cond, char *expr) |
void | BPWM (ulong addr, int size) |
void | BPHWS (ulong addr, char *mode="x", int size=1) |
void | BPHWC (ulong addr) |
void | BPL (ulong addr, char *expr) |
void | BPMC () |
void | BPRM (ulong addr, int size) |
void | GBPR () |
ulong | GMEMI (ulong addr, int info) |
ulong | GPA (char *proc, char *lib, bool bKeepInMem) |
ulong | REPL (ulong addr, char *find, char *repl, int len) |
ulong | WRT (char *file, char *data) |
ulong | WRTA (char *file, char *data[, char *separator]) |
ulong | OPCODE (ulong addr) |
ulong | FILL (ulong addr, int len, char *value) |
void | LBL (ulong addr, char *text) |
void | LC () |
void | OPENTRACE () |
void | TC () |
ulong | ALLOC (int size) |
ulong | ASK (char *question) |
void | BACKUP (ulong addr) |
ulong | DM (ulong addr, int size, file) |
ulong | DMA (ulong addr, int size, file) |
void | ESTEP (void) |
void | FREE (ulong addr) |
ulong | GFO (ulong addr) |
ulong | GPI (info) |
ulong | GSL ([int where]) |
ulong | LEN (char *str) |
ulong | POP () |
void | PUSH (ulong dw) |
char * | READSTR (char *str, int len) |
ulong | REV (ulong what) |
ulong | ROL (ulong op, ulong ulong count) |
ulong | ROR (ulong op, ulong count) |
ulong | XCHG (object *regdest, object *regsrc) |
exchanges values of 2 registers of same size | |
void | MOVB (object *objaddr, object *odjdata) |
batch mov | |
void | ASMB (object *objaddr, object *odjdata) |
batch assemble | |
ulong | TICK (void) |
returns value of GetTickCount() |
Enumeration Type Documentation
enum e_cmdinfo |
- Enumerator:
enum e_dumptype |
- Enumerator:
enum e_exceptmask |
ignore means check box of the exception
- Enumerator:
enum e_memoryinfo |
enum e_origin |
enum e_processinfo |
- Enumerator:
enum t_moduleinfo |
- Enumerator:
Function Documentation
void AI | ( | void | ) |
ulong ALLOC | ( | int | size | ) |
void AN | ( | ulong | addr | ) |
see ODBGScript documentation for details.
AN(eip.v);
void AO | ( | void | ) |
ulong ASK | ( | char * | question | ) |
see ODBGScript documentation for details.
// @todo give example
void ASMB | ( | object * | objaddr, |
object * | odjdata | ||
) |
batch assemble
- Parameters:
-
objaddr javascript array containning address odjdata javascript array of strings (assembly commands). Eatch element is assembled to corresponding address from objaddr.
asmb stands for batch assemble, i.e. you can assemble to a debuggee memory page tons of commands at once. As access of debuggee memory is a time consuming operation, this function can accelerate the process handreds of times as it reads the whole page, modifies it and then rewrite it back one time.
- See also:
- MOVB
function jsmain() { var my_array = [[], []]; // array compsed by 2 arrays // populate address object my_array[0].push(0x401000); my_array[0].push(0x401005); my_array[0].push(0x40100A); my_array[0].push(0x40100F); my_array[0].push(0x401014); my_array[0].push(0x401019); my_array[0].push(0x40101E); my_array[0].push(0x401023); my_array[0].push(0x401028); my_array[0].push(0x40102D); // populate data object my_array[1].push("call 501000"); my_array[1].push("call 502000"); my_array[1].push("call 503000"); my_array[1].push("call 504000"); my_array[1].push("call 505000"); my_array[1].push("call 506000"); my_array[1].push("call 507000"); my_array[1].push("call 508000"); my_array[1].push("call 509000"); my_array[1].push("call 50A000"); tc = TICK(); for (var i = 0; i < my_array[0].length; i++) ASM(my_array[0][i], my_array[1][i]); LOG("calling asm in loop took:", TICK() - tc, " ms"); tc = TICK(); ASMB(my_array[0], my_array[1]); LOG("calling asmb took:", TICK() - tc, " ms"); }
void BACKUP | ( | ulong | addr | ) |
see ODBGScript documentation for details.
BACKUP(0x40115C);
void BC | ( | ulong | addr | ) |
void BD | ( | ulong | addr | ) |
void BE | ( | ulong | addr | ) |
see ODBGScript documentation for details.
void BP | ( | ulong | addr | ) |
void BPCND | ( | ulong | addr, |
char * | cond | ||
) |
void BPD | ( | char * | procname | ) |
void BPGOTO | ( | ulong | addr, |
FUNC * | proc | ||
) |
Installs a callback function that will be called each time breakpoint at address addr is hit.
- Parameters:
-
addr address of breakpoint to monitor. proc javascript function that will be called each time breakpoint at address addr is hit.
- Returns:
- must return true to continue execution, otherwise it must return false;
- Todo:
- add BPGOTO suport.
Installs a callback function that will be called each time breakpoint at address addr is hit.
void BPHWC | ( | ulong | addr | ) |
void BPHWS | ( | ulong | addr, |
char * | mode = "x" , |
||
int | size = 1 |
||
) |
void BPL | ( | ulong | addr, |
char * | expr | ||
) |
see ODBGScript documentation for details.
BPL(0x401163, "dword ptr [esp+8]");
void BPLCND | ( | ulong | addr, |
char * | cond, | ||
char * | expr | ||
) |
see ODBGScript documentation for details.
BPLCND(0x401000, "eax > 1", "eax");
void BPMC | ( | ) |
see ODBGScript documentation for details.
BPMC();
void BPRM | ( | ulong | addr, |
int | size | ||
) |
see ODBGScript documentation for details.
BPRM(0x401000, 4);
void BPWM | ( | ulong | addr, |
int | size | ||
) |
see ODBGScript documentation for details.
BPWM(0x405750, 0x200);
void BPX | ( | char * | procname, |
DWORD | addr = 0 |
||
) |
set bp on every caller of label procname
- Parameters:
-
procname procedure name. addr address belonging to a memory page where you want to search for all intermodular call (imc) in. this will help you to find imc in memory not belonging to anu module. if not given, memory at ACPUDUMP is presumed.
- Returns:
- nothing
set bp on every caller of label procname.
ulong BUF | ( | var | ) |
ulong CLOSE | ( | window | ) |
void CMT | ( | char * | comment | ) |
see ODBGScript documentation for details.
CMT(0x401000, "CMT test");
void COB | ( | ) |
deprecated.
- See also:
- EOB
void COE | ( | ) |
deprecated.
- See also:
- EOE
ulong DB | ( | ulong | addr | ) |
ulong DBH | ( | void | ) |
ulong DBS | ( | void | ) |
ulong DD | ( | ulong | addr | ) |
ulong DM | ( | ulong | addr, |
int | size, | ||
file | |||
) |
ulong DMA | ( | ulong | addr, |
int | size, | ||
file | |||
) |
ulong DPE | ( | char * | filename, |
ulong | ep | ||
) |
ulong DW | ( | ulong | addr | ) |
ulong ENDE | ( | void | ) |
void ENDSEARCH | ( | void | ) |
void EOB | ( | FUNC * | proc | ) |
installs a callbacks function that will be called every time a breakpoint is hit.
- Parameters:
-
proc the callback function.
- Returns:
- false to disable calling the callback function ( in place of cob();), otherwise it must return true.
installs a callbacks function that will be called every time a breakpoint is hit.
function breakpoints_handler() { if(eip.v == 0x40118F) return false; // COB() equivalent as COB() is now deprecated LOG("this message is logged from eob"); return true; // keep taking control of breakpoints handling } function exceptions_handler() { if(eip.v == 0x415C00) return false; // COE() equivalent as COE() is now deprecated LOG("this message is logged from eoe"); return true; // keep taking control of exceptions handling } function jsmain() { BP(0x401172); BP(0x40115E); BP(0x401181); BP(0x40118F); EOB(breakpoints_handler); EOE(exceptions_handler); ESTO(); LOG("this message is logged after eob finshed"); LOG("this message is logged after eoe finshed"); BC(); }
void EOE | ( | FUNC * | proc | ) |
installs a callbacks function that will be called every time an exception occures.
- Parameters:
-
proc the callback function.
- Returns:
- false to disable calling the callback function ( in place of coe();), otherwise it must return true.
installs a callbacks function that will be called every time an exception occures.
function breakpoints_handler() { if(eip.v == 0x40118F) return false; // COB() equivalent as COB() is now deprecated LOG("this message is logged from eob"); return true; // keep taking control of breakpoints handling } function exceptions_handler() { if(eip.v == 0x415C00) return false; // COE() equivalent as COE() is now deprecated LOG("this message is logged from eoe"); return true; // keep taking control of exceptions handling } function jsmain() { BP(0x401172); BP(0x40115E); BP(0x401181); BP(0x40118F); EOB(breakpoints_handler); EOE(exceptions_handler); ESTO(); LOG("this message is logged after eob finshed"); LOG("this message is logged after eoe finshed"); BC(); }
void ERUN | ( | void | ) |
void ESTEP | ( | void | ) |
see ODBGScript documentation for details.
void ESTI | ( | void | ) |
void EVAL | ( | void | ) |
void EXEC | ( | void | ) |
ulong FILL | ( | ulong | addr, |
int | len, | ||
char * | value | ||
) |
see ODBGScript documentation for details.
FILL(0x40115C, 30, 0x89);
ulong FIND | ( | ulong | addr, |
char * | what | ||
) |
search for a pattern of bytes starting from address addr
- Parameters:
-
addr begining of the search what either a numerique, a string bytes representation or an array of bytes;
- Returns:
- first address of matching result starting from addr;
search for a pattern of bytes starting from address addr
ulong FINDO | ( | ulong | addr, |
char * | what, | ||
int | blocklen, | ||
func | callback, | ||
... | |||
) |
stands for find-do, it allows you to call a callback function eatch time the pattern is found.
- Parameters:
-
addr address to begin search from. what javascript array of bytes, hex string representation of pattern to search for or a dword. blocklen length of block of search starting from address addr. set to zero to search from addr to the end of memory page. callback javascript function to call eatch time the pattern what is found. This callback must return true to increment counter of your matched conditions, else it must return false. ... arguments to be passed to callback in addition to the address of next found pattern.
- Returns:
- number of matched conditions over all found pattern/value/array.
this is a super-repl function. It allows you to call a callback function eatch time the pattern is found, thus, you can do testing/address manipulation/more checking before replacing the pattern (or doing what ever you want). It's also a good replacment to calling find() in loops, calling findo can be reusable and the same callback can be called from different places of the script. Please, have in mind that any failure of any function called from the callback will cause the fail of the callback.
function jsmain() { var min = GMEMI(eip.v, MEMORYBASE); var max = min + GMEMI(eip.v, MEMORYSIZE) - 1; LOG(FINDO(eip.v, "E8??????00", 0, call_callback)); LOG(FINDO(eip.v, "E9??????60", 0, jump_callback, min, max)); } function call_callback(addr) { var tmpdest = addr; tmpdest = GCI(tmpdest, FINALDEST); if (tmpdest > 0x60000000) { LBL(addr, "this is intermodular call"); // set label at address addr return true; // return true to count this } return false; // else return false } function jump_callback(addr, minaddr, maxaddr) { var tmpdest = addr; tmpdest = GCI(tmpdest, FINALDEST); if (tmpdest > maxaddr || tmpdest < minaddr) { LBL(addr, "this is intermodular jump"); // set label at address addr return true; } return false; }
void FREE | ( | ulong | addr | ) |
ulong GAPI | ( | ulong | addr | ) |
void GBPM | ( | ) |
void GBPR | ( | ) |
ulong GCI | ( | ulong | addr, |
int | info | ||
) |
stands for get command information
- Parameters:
-
addr pointer to command info information to get
- Returns:
- what was requested
- See also:
- e_cmdinfo
stands for get command information. info is a member of e_cmdinfo
ulong GFO | ( | ulong | addr | ) |
ulong GMA | ( | char * | name, |
int | info | ||
) |
returns information about module named name
- Parameters:
-
name name of module info information to get
- Returns:
- See also:
- t_moduleinfo
returns information about module named name
LOG(GMA("kernel32", MODULEBASE)); LOG(GMA("user32", MODULEBASE));
ulong GMEMI | ( | ulong | addr, |
int | info | ||
) |
see ODBGScript documentation for details. see e_memoryinfo
LOG(GMEMI(eip.v, MEMORYBASE));
ulong GMEXP | ( | ulong | moduleaddr, |
int | info | ||
) |
char* GN | ( | ulong | addr | ) |
void GO | ( | ulong | addr | ) |
ulong GPA | ( | char * | proc, |
char * | lib, | ||
bool | bKeepInMem | ||
) |
ulong GPI | ( | info | ) |
see ODBGScript documentation for details.
- See also:
- e_processinfo
LOG("mainbase:", GPI(MAINBASE)); LOG("processname:", GPI(PROCESSNAME)); LOG("exefilename:", GPI(EXEFILENAME));
ulong GREF | ( | ) |
ulong GRO | ( | ulong | addr | ) |
ulong GSL | ( | ) |
char* GSTR | ( | ulong | addr[, int len] | ) |
return string of length len from memory addr
- Parameters:
-
addr pointer to memory of string len size of characters to copy
- Returns:
- the string
return string of length len from memory addr. If called with len, it copies zeros also<> it sets only $RESULT with the string and $RESULT_1 with its length.
// Example 1 var str = GSTR(0x405000, 0xE6); var my_array = str.split('\0'); for(i = 0; i < my_array.length; i++) /* you could also do: * LOG("The length of string:'", my_array[i], "' is:", my_array[i].length); */ LOG("The length of string:'", my_array[i], "' is:", LEN(my_array[i])); // Example 2 var str = GSTR(0x405000); LOG("Using LEN(), the length of string:'", str, "' is:", LEN(str)); LOG("Using $RESULT_1, the length of string:'", str, "' is:", $RESULT_1); LOG("Using .length, the length of string:'", str, "' is:", str.length);
uchar* GSTRW | ( | ulong | addr[, int len] | ) |
return unicode string of length len from memory addr
- Parameters:
-
addr pointer to memory of string len size of unicode characters to copy
- Returns:
- the unicode string
return unicode string of length len from memory addr. If called with len, it copies zeros also it sets only $RESULT with the unicode string and $RESULT_1 with its length.
// Example 1 var str = GSTRU(0x405000, 0xE6); var my_array = str.split('\0'); for(i = 0; i < my_array.length; i++) /* you could also do: * LOG("The length of string:'", my_array[i], "' is:", my_array[i].length); */ LOG("The length of string:'", my_array[i], "' is:", LEN(my_array[i])); // Example 2 var str = GSTRU(0x405000); LOG("Using LEN(), the length of string:'", str, "' is:", LEN(str)); LOG("Using $RESULT_1, the length of string:'", str, "' is:", $RESULT_1); LOG("Using .length, the length of string:'", str, "' is:", str.length);
void KEY | ( | int | vkcode, |
bool | shift = false , |
||
bool | ctrl = false , |
||
int | origin = PM_DISASM |
||
) |
void LBL | ( | ulong | addr, |
char * | text | ||
) |
see ODBGScript documentation for details.
LBL(ecx.v, "this is lbl test");
void LC | ( | ) |
see ODBGScript documentation for details.
LC();
ulong LEN | ( | char * | str | ) |
see ODBGScript documentation for details. Note that either len and LEN are reserved names in ODBJScript.
ulong LM | ( | ulong | addr, |
int | size, | ||
char * | filename | ||
) |
ulong LOADLIB | ( | char * | dllname | ) |
void LOG | ( | ... | ) |
logs any data to log window.
- Parameters:
-
... arguments you wanna log.
logs any data to log window. can be DWORD, doubles, floats, strings, arrays, function names ...
LOG("data at: ", eax.v, " must be: ", [0x68, 0x40, 0x90], ", or equal to: ", ebx);
void LOGBUF | ( | ulong | ip, |
size_t | size, | ||
int | nepl = 32 , |
||
char * | separator = "" |
||
) |
logs size bytes of memory beginning from address ip.
- Parameters:
-
ip pbuffer start address of dump size size of dumped bytes to be logged nepl elemperline number of elements per line separator separator between bytes
- Returns:
- void
logs size bytes of memory beginning from address ip with options: nepl element per line and separating element by separator.
LOGBUF(0x401000, 45, 100, ':');
ulong MEMCPY | ( | ulong | dest, |
ulong | src, | ||
int | size | ||
) |
ulong MOV | ( | ulong | addr, |
char * | what, | ||
int | n = lenght_of_what |
||
) |
writes n or all element of what to address addr
- Parameters:
-
addr pointer to address to write to. what a string representation of binary data, or a js array of char or a DWORD n in case you want to write just a part from what, n is the needed number of elements.
- Returns:
- number of bytes wrote.
writes n or all element of what to address addr. Doesn't set $RESULT
void MOVB | ( | object * | objaddr, |
object * | odjdata | ||
) |
batch mov
- Parameters:
-
objaddr javascript array containning address odjdata javascript array of binary data (DWORD/array/string representation of binary data) to be written. Eatch element is written to corresponding address from objaddr.
movb stands for batch mov, i.e. you can write to a debuggee memory page tons of data at once. As access of debuggee memory is a time consuming operation, this function can accelerate the process handreds of times as it reads the whole page, modifies it and then rewrite it back one time.
- See also:
- ASMB
function jsmain() { var my_array = [[], []]; // array compsed by 2 arrays // populate address object my_array[0].push(0x401000); my_array[0].push(0x401004); my_array[0].push(0x401008); my_array[0].push(0x40100C); my_array[0].push(0x401010); my_array[0].push(0x401014); my_array[0].push(0x401018); my_array[0].push(0x40101C); my_array[0].push(0x401020); my_array[0].push(0x401024); // populate data object my_array[1].push(0); my_array[1].push(1); my_array[1].push(2); my_array[1].push(3); my_array[1].push(4); my_array[1].push(5); my_array[1].push(6); my_array[1].push(7); my_array[1].push(8); my_array[1].push(9); tc = TICK(); for (var i = 0; i < my_array[0].length; i++) MOV(my_array[0][i], my_array[1][i]); LOG("calling mov in loop took:", TICK() - tc, " ms"); tc = TICK(); MOVB(my_array[0], my_array[1]); LOG("calling movb took:", TICK() - tc, " ms"); }
ulong MOVS | ( | ulong | addr, |
char * | str, | ||
int | n = lenght_of_what |
||
) |
writes n or all characters of string str to address addr
- Parameters:
-
addr pointer to address to write to. what a string. n in case you want to write just a part from what, n is the needed number of characters.
- Returns:
- number of characters wrote.
writes n or all characters of string str to address addr. Doesn't set $RESULT
void MSG | ( | void | ) |
see ODBGScript documentation for details.
MSG("No pattern found? Will abort script!");
void MSGYN | ( | void | ) |
ulong NAMES | ( | ulong | addr | ) |
ulong OLLY | ( | int | info | ) |
ulong OPCODE | ( | ulong | addr | ) |
void OPENDUMP | ( | ulong | addr, |
ulong | base, | ||
ulong | size, | ||
int | type = Hex/ASCII |
||
) |
- Todo:
- Fix OPENDUMP API close after open.
- See also:
- e_dumptype
OPENDUMP(0x40115C); // or OPENDUMP(0x40115C, 0x401000, 0x200, DU_HEXTEXT);
void OPENTRACE | ( | ) |
see ODBGScript documentation for details.
OPENTRACE();
void PAUSE | ( | void | ) |
ulong POP | ( | ) |
void POPA | ( | void | ) |
ulong PREOP | ( | ulong | addr | ) |
Get asm command line address just before specified address.
- Parameters:
-
addr current position
- Returns:
- address of command 1 step back from address addr. if addr == PREOP(addr), function returns 0 to facilitate testing.
Get asm command line address just before specified address.
/* preop(addr); * Normally ollydbg returns the same address if addr is the first address of a * memory page. But to keep things simple, I changed that to let you test the * function without saving the return address in a var. see this example: */ while (PREOP(jmpdest)) { if (DW($RESULT) != 0) break; jmpdest = $RESULT; }
void PUSHA | ( | void | ) |
char* READSTR | ( | char * | str, |
int | len | ||
) |
see ODBGScript documentation for details.
void REFRESH | ( | bool | onoff | ) |
sets on/off refreshing ollydbg MDI windows.
- Parameters:
-
onoff if true, plugin will call Broadcast function to let you see visual changes like breakpoint, memory changes ... but this slows downs excution al little bit.
- Returns:
- nothing
sets on/off refreshing ollydbg MDI windows. thus, if you want to give the script a hand, call it with false in the beginning of your script.
REFRESH(true);
ulong REPL | ( | ulong | addr, |
char * | find, | ||
char * | repl, | ||
int | len | ||
) |
ulong ROL | ( | ulong | op, |
ulong ulong | count | ||
) |
ulong ROR | ( | ulong | op, |
ulong | count | ||
) |
void RTR | ( | void | ) |
void RTU | ( | void | ) |
void RUN | ( | void | ) |
ulong SETOPTION | ( | DWORD | mask | ) |
sets/unsets exception to be ignored by ollydbg.
- Parameters:
-
mask for exceptions to set/unset
- See also:
- e_exceptmask
sets/unsets exception to be ignored by ollydbg. can do it manually or internally.
SETOPTION(IGNORE_ALL); // or SETOPTION(IGNORE_ACCESS_KER32|IGNORE_INT3); // or SETOPTION(IGNORE_MANUALLY);
void STI | ( | void | ) |
void STO | ( | void | ) |
void STR | ( | var | ) |
void TC | ( | ) |
see ODBGScript documentation for details.
TC();
void TI | ( | void | ) |
ulong TICK | ( | void | ) |
returns value of GetTickCount()
returns value of GetTickCount() witch retrieves the number of milliseconds that have elapsed since Windows was started.
void TICND | ( | char * | cond, |
ulong | in0, | ||
ulong | in1, | ||
ulong | out0, | ||
ulong | out1 | ||
) |
Traces into calls until cond is true and/or on EIP in range [in0-in1] and/or out of range [out0-out1].
- Parameters:
-
cond condition to stop execution if met in0,in1 - 'in range' request. Run trace will pause if EIP is in this range (in1 not included). out0,out1 - 'out of range' request. Run trace will pause if EIP is outside this range or equals to out1.
- Returns:
- nothing
this javascript version of function accepts either 1, 3 or 5 arguments. in case of one argument, it will be treated as string condition. In case of 3, they will be treated as string cond,in0,int1, else it will be the full version of function.
void TO | ( | void | ) |
void TOCND | ( | char * | cond | ) |
Traces over calls until cond is true and/or on EIP in range [in0-in1] and/or out of range [out0-out1].
- Parameters:
-
cond condition to stop execution if met in0,in1 - 'in range' request. Run trace will pause if EIP is in this range (in1 not included). out0,out1 - 'out of range' request. Run trace will pause if EIP is outside this range or equals to out1.
- Returns:
- nothing
this javascript version of function accepts either 1, 3 or 5 arguments. in case of one argument, it will be treated as string condition. In case of 3, they will be treated as string cond,in0,int1, else it will be the full version of function.
ulong WRT | ( | char * | file, |
char * | data | ||
) |
see ODBGScript documentation for details.
var my_array = new Array(); my_array[0] = 0x90; my_array[1] = 0x10; my_array[2] = 0x11; my_array[3] = 0x12; my_array[4] = 0x13; my_array[5] = 0x14; my_array[6] = 0x15; my_array[7] = 0x16; my_array[8] = 0x17; my_array[9] = 0x18; WRT("stoleb.bytes", "; This is wrta/wrt example"); WRTA("stoleb.bytes", my_array, ""); WRTA("stoleb.bytes", 0x68909090);
ulong WRTA | ( | char * | file, |
char * | data[, char *separator] | ||
) |
see ODBGScript documentation for details.
var my_array = new Array(); my_array[0] = 0x90; my_array[1] = 0x10; my_array[2] = 0x11; my_array[3] = 0x12; my_array[4] = 0x13; my_array[5] = 0x14; my_array[6] = 0x15; my_array[7] = 0x16; my_array[8] = 0x17; my_array[9] = 0x18; WRT("stoleb.bytes", "; This is wrta/wrt example"); WRTA("stoleb.bytes", my_array, ""); WRTA("stoleb.bytes", 0x68909090);
ulong XCHG | ( | object * | regdest, |
object * | regsrc | ||
) |
Generated on Fri Jan 27 2012 17:54:31 for ODBJscript by
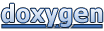