![]() |
NFC ZigBee 3.0 Modules and Libraries
NFC Commissioning using ZigBee Installation Codes
|
ntag.h
Go to the documentation of this file.
11 * $HeadURL: https://www.collabnet.nxp.com/svn/lprf_sware/Projects/Components/NFC/Tags/+v1000/Include/ntag.h $
PUBLIC uint8 * NTAG_pu8Header(void)
Returns the 16 header bytes of the NTAG's memory.
Definition: ntag.h:149
PUBLIC uint32 NTAG_u32Session(void)
Returns the byte address of the NTAG's session registers.
void(* tprNtagCbEvent)(teNtagEvent eNtagEvent, uint32 u32Address, uint32 u32Length, uint8 *pu8Data)
Definition: ntag.h:161
PUBLIC bool_t NTAG_bWriteReg(uint32 u32WriteAddress, uint32 u32WriteLength, uint8 *pu8WriteData)
Requests write of NTAG register data.
Definition: ntag.h:155
Definition: ntag.h:157
Definition: ntag.h:154
PUBLIC bool_t NTAG_bReadVersion(uint32 u32ReadLength, uint8 *pu8ReadData)
Requests read of NTAG version data.
Definition: ntag.h:150
PUBLIC bool_t NTAG_bRead(uint32 u32ReadAddress, uint32 u32ReadLength, uint8 *pu8ReadData)
Requests read of NTAG data.
Definition: ntag.h:152
Definition: ntag.h:153
Definition: ntag.h:148
PUBLIC void NTAG_vRegCbEvent(tprNtagCbEvent prRegCbEvent)
Registers callback for NTAG events.
PUBLIC uint8 * NTAG_pu8Version(void)
Returns the 8 version information bytes for the NTAG.
PUBLIC bool_t NTAG_bReadReg(uint32 u32ReadAddress, uint32 u32ReadLength, uint8 *pu8ReadData)
Requests read of NTAG register data.
Definition: ntag.h:151
PUBLIC uint32 NTAG_u32Config(void)
Returns the byte address of the NTAG's configuration registers.
Definition: ntag.h:156
PUBLIC bool_t NTAG_bWrite(uint32 u32WriteAddress, uint32 u32WriteLength, uint8 *pu8WriteData)
Requests write of NTAG data.
PUBLIC void NTAG_vInitialise(uint8 u8Address, bool_t bLocation, uint32 u32FrequencyHz, uint8 u8InputFd)
Initialises NTAG data and hardware.
Generated by
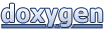