![]() |
NFC ZigBee 3.0 Modules and Libraries
NFC Commissioning using ZigBee Installation Codes
|
NCI driver for reading and writing data (interface) More...
#include <jendefs.h>
Go to the source code of this file.
Typedefs | |
typedef void(* | tprNciCbEvent) (teNciEvent eNciEvent, uint32 u32Address, uint32 u32Length, uint8 *pu8Data) |
Enumerations | |
enum | teNciEvent { E_NCI_EVENT_ABSENT, E_NCI_EVENT_PRESENT, E_NCI_EVENT_READ_FAIL, E_NCI_EVENT_READ_OK, E_NCI_EVENT_WRITE_FAIL, E_NCI_EVENT_WRITE_OK } |
Functions | |
PUBLIC void | NCI_vInitialise (uint8 u8Address, bool_t bLocation, uint32 u32FrequencyHz, uint8 u8InputVen, uint8 u8InputIrq) |
Initialises NCI data and hardware. More... | |
PUBLIC void | NCI_vRegCbEvent (tprNciCbEvent prRegCbEvent) |
Registers callback for NCI events. More... | |
PUBLIC void | NCI_vTick (uint32 u32TickMs) |
Timer function to drive NCI processing. More... | |
PUBLIC bool_t | NCI_bRead (uint32 u32ReadAddress, uint32 u32ReadLength, uint8 *pu8ReadData) |
Requests read of data from NTAG in reader's field. More... | |
PUBLIC bool_t | NCI_bReadVersion (uint32 u32ReadLength, uint8 *pu8ReadData) |
Requests read of version data from NTAG in reader's field. More... | |
PUBLIC bool_t | NCI_bWrite (uint32 u32WriteAddress, uint32 u32WriteLength, uint8 *pu8WriteData) |
Requests write of data to NTAG in reader's field. More... | |
PUBLIC bool_t | NCI_bEnd (void) |
Ends NCI processing. More... | |
PUBLIC uint32 | NCI_u32Nci (void) |
Returns the model of the NTAG in the reader's field. More... | |
PUBLIC uint32 | NCI_u32Config (void) |
Returns the byte address of the configuration registers of the NTAG in the reader's field. More... | |
PUBLIC uint32 | NCI_u32Session (void) |
Returns the byte address of the session registers of the NTAG in the reader's field. More... | |
PUBLIC uint32 | NCI_u32Sram (void) |
Returns the byte address of the SRAM data of the NTAG in the reader's field. More... | |
PUBLIC uint8 * | NCI_pu8Header (void) |
Returns the 16 header bytes of the memory of the NTAG in the reader's field. More... | |
PUBLIC uint8 * | NCI_pu8Version (void) |
Returns the 8 version information bytes for the NTAG in the reader's field. More... | |
Detailed Description
NCI driver for reading and writing data (interface)
nci.h contains low level APIs for using an NFC reader to reading and writing raw data to the NTAGs.
The typical set up sequence is as follows:
- NCI_vInitialise() is called to initialise the hardware.
- NCI_vRegCbEvent() is called to register the event callback function in the application the library should call to inform the application of NCI events. APP_NciCbEvent() is used in these examples.
- NCI_vTick() should be called regularly (recommended every 5ms) to provide processing time to the library.
- APP_NciCbEvent() will be called by the library to pass events to the application layer. The E_NCI_EVENT_ABSENT and E_NCI_EVENT_PRESENT events will be raised when a NTAG is removed from and placed into an NFC reader's field.
A typical sequence to read data is shown below. Data can only be read when a NTAG is in the reader's field in response (indicated by the E_NCI_EVENT_PRESENT event). When the callback function is registered at initialisation the E_NCI_EVENT_ABSENT will be raised if a NTAG is not in the field.
- NCI_vTick() is called by the application
- APP_NciCbEvent(E_NCI_EVENT_PRESENT) is called in the application
- NCI_bRead() is called by the application, the byte address and length of data to be read from the NTAG is provided along with a buffer to store the read data in. This call will return TRUE if the request is accepted.
- NCI_vTick() continues to be called regularly by the application.
- APP_NciCbEvent(E_NCI_EVENT_READ_OK) is called in the application if the read is successful. The data read from the NTAG will be in the buffer provided by the call to NCI_bRead(), this data pointer is also provided as a parameter to APP_NciCbEvent()
- APP_NciCbEvent(E_NCI_EVENT_READ_FAIL) is called in the application if the read is unsuccessful.
- NCI_bEnd() should be called if the application has finished interacting with the NTAG.
A typical sequence to write data is shown below. Data can only be written when a NTAG is in the reader's field (indicated by the E_NCI_EVENT_PRESENT event). Data may also be written following a successful read as shown below:
- NCI_vTick() is called by the application
- APP_NciCbEvent(E_NCI_EVENT_READ_OK) is called in the application following a successful read.
- NCI_bWrite() is called by the application, the byte address and length of data to be written to the NTAG is provided along with a buffer containing the data to be written. This call will return TRUE if the request is accepted.
- NCI_vTick() continues to be called regularly by the application.
- APP_NciCbEvent(E_NCI_EVENT_WRITE_OK) is called in the application if the write is successful.
- APP_NciCbEvent(E_NCI_EVENT_WRITE_FAIL) is called in the application if the write is unsuccessful.
- NCI_bEnd() should be called if the application has finished interacting with the NTAG.
The message sequence chart below shows a sequence of function calls for initialisation, a data read and a data write:
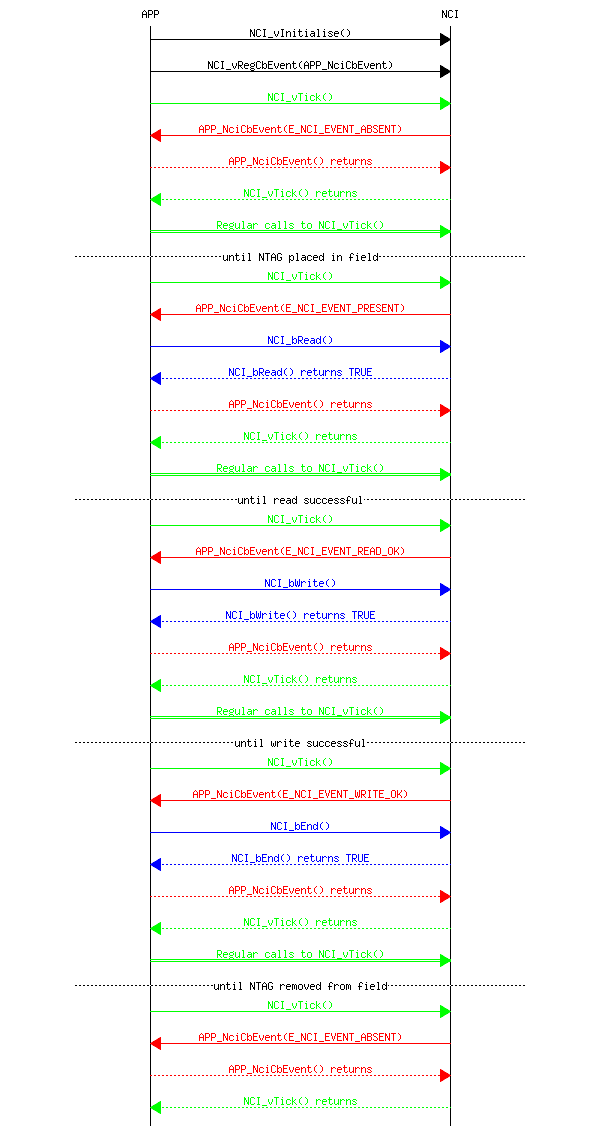
Typedef Documentation
◆ tprNciCbEvent
typedef void(* tprNciCbEvent) ( teNciEvent eNciEvent, uint32 u32Address, uint32 u32Length, uint8 *pu8Data) |
NCI Event Callback function
Enumeration Type Documentation
◆ teNciEvent
enum teNciEvent |
NTAG Events - Passed to application by library calling the registerd callback function
Function Documentation
◆ NCI_vInitialise()
PUBLIC void NCI_vInitialise | ( | uint8 | u8Address, |
bool_t | bLocation, | ||
uint32 | u32FrequencyHz, | ||
uint8 | u8InputVen, | ||
uint8 | u8InputIrq | ||
) |
Initialises NCI data and hardware.
- Parameters
-
u8Address Reader I2C address (0xFF for automatic detection of NPC100 or PN7120) bLocation Use alternative JN516x I2C pins u32FrequencyHz Prescale value for I2C (63 recommended) u8InputVen Output DIO for VEN u8InputIrq Input DIO for IRQ
◆ NCI_vRegCbEvent()
PUBLIC void NCI_vRegCbEvent | ( | tprNciCbEvent | prRegCbEvent | ) |
Registers callback for NCI events.
- Parameters
-
prRegCbEvent Pointer to event callback function
◆ NCI_vTick()
PUBLIC void NCI_vTick | ( | uint32 | u32TickMs | ) |
Timer function to drive NCI processing.
Should be called regularly, every 5ms is recommended.
- Parameters
-
u32TickMs Time in ms since previous call
◆ NCI_bRead()
PUBLIC bool_t NCI_bRead | ( | uint32 | u32ReadAddress, |
uint32 | u32ReadLength, | ||
uint8 * | pu8ReadData | ||
) |
Requests read of data from NTAG in reader's field.
If the request is successful the final outcome of the read request is indicated by a call to the NCI event callback function, along with the data if successful.
- Return values
-
TRUE Request accepted FALSE Request failed
- Parameters
-
u32ReadAddress Byte address of data to read u32ReadLength Number of bytes to read pu8ReadData Buffer to read data into
◆ NCI_bReadVersion()
PUBLIC bool_t NCI_bReadVersion | ( | uint32 | u32ReadLength, |
uint8 * | pu8ReadData | ||
) |
Requests read of version data from NTAG in reader's field.
If the request is successful the final outcome of the read request is indicated by a call to the NCI event callback function, along with the data if successful.
The version information is always 8 bytes in size the buffer and length needs to be set appropriately.
- Return values
-
TRUE Request accepted FALSE Request failed
- Parameters
-
u32ReadLength Number of bytes to read (minimum 8) pu8ReadData Buffer to read data into
◆ NCI_bWrite()
PUBLIC bool_t NCI_bWrite | ( | uint32 | u32WriteAddress, |
uint32 | u32WriteLength, | ||
uint8 * | pu8WriteData | ||
) |
Requests write of data to NTAG in reader's field.
If the request is successful the final outcome of the write request is indicated by a call to the NCI event callback function, along with the data if successful.
- Return values
-
TRUE Request accepted FALSE Request failed
- Parameters
-
u32WriteAddress Byte address of write u32WriteLength Number of bytes to write pu8WriteData Buffer to write data from
◆ NCI_bEnd()
PUBLIC bool_t NCI_bEnd | ( | void | ) |
Ends NCI processing.
This function should be called when the reader has finished processing data in the NTAG in the reader's field.
- Return values
-
TRUE Request accepted FALSE Request failed
◆ NCI_u32Nci()
PUBLIC uint32 NCI_u32Nci | ( | void | ) |
Returns the model of the NTAG in the reader's field.
The NCI_bReadVersion() function must have been called previously in order to detect the NTAG model.
- Return values
-
NFC_NTAG_UNKNOWN Unknown NTAG model NFC_NTAG_NT3H1101 NT3H1101 NTAG model NFC_NTAG_NT3H1201 NT3H1201 NTAG model NFC_NTAG_NT3H2111 NT3H2111 NTAG model NFC_NTAG_NT3H2211 NT3H2211 NTAG model
◆ NCI_u32Config()
PUBLIC uint32 NCI_u32Config | ( | void | ) |
Returns the byte address of the configuration registers of the NTAG in the reader's field.
The NCI_bReadVersion() function must have been called previously in order to determine the address.
- Returns
- Byte address of configuration registers
◆ NCI_u32Session()
PUBLIC uint32 NCI_u32Session | ( | void | ) |
Returns the byte address of the session registers of the NTAG in the reader's field.
The NCI_bReadVersion() function must have been called previously in order to determine the address.
- Returns
- Byte address of session registers
◆ NCI_u32Sram()
PUBLIC uint32 NCI_u32Sram | ( | void | ) |
Returns the byte address of the SRAM data of the NTAG in the reader's field.
The NCI_bReadVersion() function must have been called previously in order to determine the address.
- Returns
- Byte address of the SRAM data
◆ NCI_pu8Header()
PUBLIC uint8* NCI_pu8Header | ( | void | ) |
Returns the 16 header bytes of the memory of the NTAG in the reader's field.
The NCI_bRead() function must have been called previously with a read of these first 16 bytes from address 0.
- Returns
- Pointer to header data
◆ NCI_pu8Version()
PUBLIC uint8* NCI_pu8Version | ( | void | ) |
Returns the 8 version information bytes for the NTAG in the reader's field.
The NCI_bReadVersion() function must have been called previously.
- Returns
- Pointer to version data
Generated by
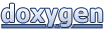