CONIO
2.1
|
A conio implementation for Mingw/Dev-C++. More...
#include <conio.h>
Data Structures | |
struct | text_info |
Structure holding information about screen. More... | |
struct | char_info |
Structure used by gettext/puttext. More... | |
Macros | |
#define | gettext _conio_gettext |
Define alias for _conio_gettext. | |
#define | cgets _cgets |
This defines enables you to use all MinGW conio.h functions without underscore. | |
Cursor types | |
#define | _NOCURSOR 0 |
no cursor | |
#define | _SOLIDCURSOR 100 |
cursor filling whole cell | |
#define | _NORMALCURSOR 20 |
cursor filling 20 percent of cell height | |
Enumerations | |
enum | COLORS { BLACK, BLUE, GREEN, CYAN, RED, MAGENTA, BROWN, LIGHTGRAY, DARKGRAY, LIGHTBLUE, LIGHTGREEN, LIGHTCYAN, LIGHTRED, LIGHTMAGENTA, YELLOW, WHITE } |
Colors which you can use in your application. More... | |
Functions | |
void | gettextinfo (struct text_info *info) |
Returns information of the screen. | |
void | inittextinfo (void) |
Call this if you need real value of normattr attribute in the text_info structure. | |
void | clreol (void) |
Clears rest of the line from cursor position to the end of line without moving the cursor. | |
void | clrscr (void) |
Clears whole screen. | |
void | delline (void) |
Delete the current line (line on which is cursor) and then moves all lines below one line up. | |
void | insline (void) |
Insert blank line at the cursor position. | |
void | _conio_gettext (int left, int top, int right, int bottom, struct char_info *buf) |
Gets text from the screen. | |
void | puttext (int left, int top, int right, int bottom, struct char_info *buf) |
Puts text back to the screen. | |
void | movetext (int left, int top, int right, int bottom, int destleft, int desttop) |
Copies text. | |
void | gotoxy (int x, int y) |
Moves cursor to the specified position. | |
void | cputsxy (int x, int y, char *str) |
Puts string at the specified position. | |
void | putchxy (int x, int y, char ch) |
Puts char at the specified position. | |
void | _setcursortype (int type) |
Sets the cursor type. | |
void | textattr (int _attr) |
Sets attribute of text. | |
void | normvideo (void) |
Sets text attribute back to value it had after program start. | |
void | textbackground (int color) |
Sets text background color. | |
void | textcolor (int color) |
Sets text foreground color. | |
int | wherex (void) |
Reads the cursor X position. | |
int | wherey (void) |
Reads the cursor Y position. | |
char * | getpass (const char *prompt, char *str) |
Reads password. | |
void | highvideo (void) |
Makes foreground colors light. | |
void | lowvideo (void) |
Makes foreground colors dark. | |
void | delay (int ms) |
Pauses program execution for a given time. | |
void | switchbackground (int color) |
Replaces background color in the whole window. | |
void | flashbackground (int color, int ms) |
Changes background color for a given time and then it restores it back. | |
void | clearkeybuf (void) |
Clears the keyboard buffer. | |
Detailed Description
A conio implementation for Mingw/Dev-C++.
Written by: Hongli Lai hongl.nosp@m.i@te.nosp@m.lekab.nosp@m.el.n.nosp@m.l tkorrovi tkorr.nosp@m.ovi@.nosp@m.altav.nosp@m.ista.nosp@m..net on 2002/02/26. Andrew Westcott ajwes.nosp@m.tco@.nosp@m.users.nosp@m..sou.nosp@m.rcefo.nosp@m.rge..nosp@m.net Michal Molhanec micha.nosp@m.l@mo.nosp@m.lhane.nosp@m.c.ne.nosp@m.t
Offered for use in the public domain without any warranty.
Macro Definition Documentation
#define gettext _conio_gettext |
Define alias for _conio_gettext.
If you want to use gettext function from some other library (e.g. GNU gettext) you have to define _CONIO_NO_GETTEXT_ so you won't get name conflict.
Enumeration Type Documentation
enum COLORS |
Colors which you can use in your application.
- Enumerator:
Function Documentation
void _conio_gettext | ( | int | left, |
int | top, | ||
int | right, | ||
int | bottom, | ||
struct char_info * | buf | ||
) |
Gets text from the screen.
If you haven't defined _CONIO_NO_GETTEXT_
prior to including conio2.h
you can use this function also under the gettext
name.
- Parameters
-
left Left coordinate of the rectangle, inclusive, starting from 1. top Top coordinate of the rectangle, inclusive, starting from 1. right Right coordinate of the rectangle, inclusive, starting from 1. bottom Bottom coordinate of the rectangle, inclusive, starting from 1. buf You have to pass buffer of size (right - left + 1) * (bottom - top + 1) * sizeof(char_info)
.
void _setcursortype | ( | int | type | ) |
Sets the cursor type.
- See Also
- cursortypes
- Parameters
-
type cursor type, under Win32 it is height of the cursor in percents
void clearkeybuf | ( | void | ) |
Clears the keyboard buffer.
To see it in effect run conio_test
and try to press a key during the 'Flashing...' phase.
void cputsxy | ( | int | x, |
int | y, | ||
char * | str | ||
) |
Puts string at the specified position.
- Parameters
-
x horizontal position y vertical position str string
void delay | ( | int | ms | ) |
void delline | ( | void | ) |
Delete the current line (line on which is cursor) and then moves all lines below one line up.
Lines below the line are moved one line up.
void flashbackground | ( | int | color, |
int | ms | ||
) |
Changes background color for a given time and then it restores it back.
You can use it for visual bell. Does not modify textbackground().
- See Also
- switchbackground
- delay
- Parameters
-
color background color ms miliseconds
char* getpass | ( | const char * | prompt, |
char * | str | ||
) |
Reads password.
This function behaves like cgets.
- See Also
- cgets
- Parameters
-
prompt prompt which will be displayed to user str string for the password. str[0]
have to contain length of thestr
- 3
- Returns
&str[2]
, the password will be stored instr
beginning atstr[2]
, instr[1]
will be length of the string without\0
, atstr[2 + str[1]]
will be \0.
void gotoxy | ( | int | x, |
int | y | ||
) |
Moves cursor to the specified position.
- Parameters
-
x horizontal position y vertical position
void highvideo | ( | void | ) |
void inittextinfo | ( | void | ) |
void insline | ( | void | ) |
Insert blank line at the cursor position.
Original content of the line and content of lines below moves one line down. The last line is deleted.
void lowvideo | ( | void | ) |
void movetext | ( | int | left, |
int | top, | ||
int | right, | ||
int | bottom, | ||
int | destleft, | ||
int | desttop | ||
) |
Copies text.
- Parameters
-
left Left coordinate of the rectangle, inclusive, starting from 1. top Top coordinate of the rectangle, inclusive, starting from 1. right Right coordinate of the rectangle, inclusive, starting from 1. bottom Bottom coordinate of the rectangle, inclusive, starting from 1. destleft Left coordinate of the destination rectangle. desttop Top coordinate of the destination rectangle.
void normvideo | ( | void | ) |
void putchxy | ( | int | x, |
int | y, | ||
char | ch | ||
) |
Puts char at the specified position.
- Parameters
-
x horizontal position y vertical position ch char
void puttext | ( | int | left, |
int | top, | ||
int | right, | ||
int | bottom, | ||
struct char_info * | buf | ||
) |
Puts text back to the screen.
- See Also
- char_info
- _conio_gettext
- Parameters
-
left Left coordinate of the rectangle, inclusive, starting from 1. top Top coordinate of the rectangle, inclusive, starting from 1. right Right coordinate of the rectangle, inclusive, starting from 1. bottom Bottom coordinate of the rectangle, inclusive, starting from 1. buf You have to pass buffer of size (right - left + 1) * (bottom - top + 1) * sizeof(char_info)
.
void switchbackground | ( | int | color | ) |
Replaces background color in the whole window.
The text however is left intact. Does not modify textbackground().
- See Also
- flashbackground
- Parameters
-
color background color
void textattr | ( | int | _attr | ) |
Sets attribute of text.
- Parameters
-
_attr new text attribute
void textbackground | ( | int | color | ) |
void textcolor | ( | int | color | ) |
int wherex | ( | void | ) |
Reads the cursor X position.
- Returns
- cursor X position
int wherey | ( | void | ) |
Reads the cursor Y position.
- Returns
- cursor Y position
Generated on Fri Dec 6 2013 20:09:46 for CONIO by
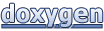