SYSTIMER
|
Usage
This example demonstrates software timer callback registration and generation of software timer callback event at every one second time interval using SYSTIMER APP for XMC45 target device. Software timer callback event is indicated by LED toggling at every one second time interval.
Requirements
Boards Required: XMC4500 CPU Board.
Instantiate the required APPs
Drag an instance of SYSTIMER and DIGITAL_IO APPs. Update the fields in the GUI of these APPs with the following configuration.
Configure the APPs
SYSTIMER APP:
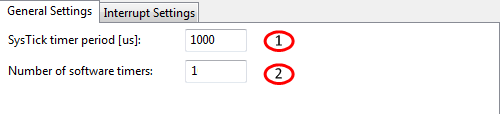
Step1: Configure SysTick timer period value
Configure SysTick timer period [us] = 1000
Note: SysTick is reference to all software timers. SysTick events are generated for every one millisecond time interval.
Step2: Configure required number of software timers
Configure Number of software timers = 1
Note: In main.c, need to call SYSTIMER_CreateTimer() API to configure the required time interval and user call back function. SYSTIMER_StartTimer() API to start the software timer.
DIGITAL_IO APP:
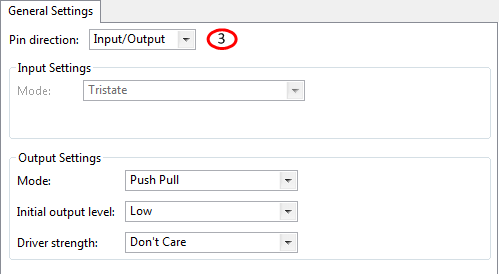
Step3: Pin Direction setting
Set Pin direction = Input/Output
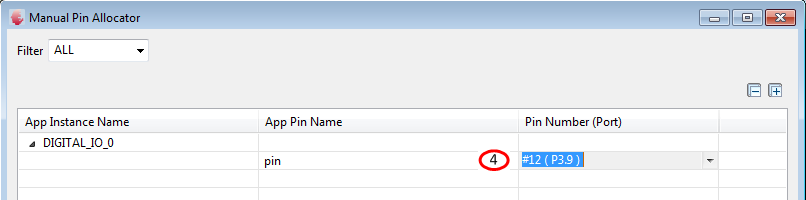
Step4: Pin Allocation
Allocate P3.9 for DIGITAL_IO
Generate code
Files are generated here: `<project_name>/Dave/Generated/' (`project_name' is the name chosen by the user during project creation). APP instance definitions and APIs are generated only after code generation.
Note: Code must be explicitly generated for every change in the GUI configuration.
Important: Any manual modification to APP specific files will be overwritten by a subsequent code generation operation.
Sample Application (main.c)
#include <DAVE.h> #define ONESEC 1000000U void LED_Toggle_EverySec(void) { // LED Toggle for every second DIGITAL_IO_ToggleOutput(&DIGITAL_IO_0); } int main(void) { uint32_t TimerId,status; // ... Initializes APPs configuration ... DAVE_Init(); // SYSTIMER APP Initialized during DAVE Initialization // Create Software Timer with one second time interval in order to generate software timer callback event at // every second TimerId = SYSTIMER_CreateTimer(ONESEC,SYSTIMER_MODE_PERIODIC,(void*)LED_Toggle_EverySec,NULL); if(TimerId != 0U) { //Timer is created successfully // Start/Run Software Timer status = SYSTIMER_StartTimer(TimerId); if(status == SYSTIMER_STATUS_SUCCESS) { // Timer is running } else { // Error during software timer start operation } } else { // Timer ID Can not be zero } while(1) { } return (1); }
Build and Run the Project
Observation
- The LED connected to P3.9 of XMC45 device toggles at every second.