![]() |
ATEsystem.PIRIS-driver
1.0.1
ATEsystem.PIRIS-driver
|
ATEsystem.PIRIS.h File Reference
#include <string>
#include <map>
#include <vector>
#include <list>
#include "pylon/PylonIncludes.h"
#include "serial.h"
Go to the source code of this file.
Classes | |
class | ATEsystem_PIRIS::Utils |
class | ATEsystem_PIRIS::PirisDevice |
class | ATEsystem_PIRIS::ErrorCluster |
class | ATEsystem_PIRIS::Version |
class | ATEsystem_PIRIS::FocusZoomIris< T > |
class | ATEsystem_PIRIS::StatusEx |
class | ATEsystem_PIRIS::DataID |
class | ATEsystem_PIRIS::DataPosition |
class | ATEsystem_PIRIS::DataParams |
class | ATEsystem_PIRIS::DataState |
class | ATEsystem_PIRIS::CPirisMain |
class | ATEsystem_PIRIS::SmartPointer< T > |
class | ATEsystem_PIRIS::Factory |
Namespaces | |
ATEsystem_PIRIS | |
Macros | |
#define | OK "OK" |
#define | ERR0 "Command was not recognized." |
#define | ERR1 "Wrong input parameter." |
#define | ERR2 "Device is not initialized." |
#define | ERR3 "Internal driver circuit is in error state (overheat, undervoltage)." |
#define | ERR4 "Wrong lens type." |
#define | ERR5 "Damaged or missing rear sensor." |
#define | ERR6 "Step generator timer overflow." |
#define | ERR7 "Device is busy." |
#define | ERR_UN "Unknown error." |
#define | ERR_PA "Parse answer failed." |
#define | TXT_SUCCESS "Success" |
#define | TXT_GENERAL_ERROR "General Error" |
#define | TXT_OPEN_FAILED "Open failed" |
#define | TXT_CLOSE_FAILED "CLose failed" |
#define | TXT_ALREADY_OPEN "Already open" |
#define | TXT_DEVICE_IS_BUSY "Device is busy" |
#define | TXT_DEVICE_IS_CLOSED "Device is closed" |
#define | TXT_INVALID_DEVICE "Invalid device" |
#define | TXT_WRITE_ERROR "Write error" |
#define | TXT_READ_ERROR "Read error" |
#define | TXT_SCAN_FAILED "Scan failed" |
#define | TXT_INTERNAL_ERROR "Internal error" |
#define | TXT_RX_TIMEOUT "Receive timeout" |
#define | TXT_DEV_SET_FAILED "Device set failed" |
#define | TXT_WRONG_MODE "Wrong mode" |
#define | TXT_DEVICE_NOT_EXIST "Device does not exist" |
#define | TXT_PARSE_ANSWER_FAIL "Parse answer failed" |
#define | TXT_ETHERNET_DEVICE "Ethernet device" |
#define | TXT_ETHERNET_PYLON "Ethernet pylon (camera overiden)" |
#define | TXT_ETHERNET_CAMERA "Ethernet camera (camera overiden)" |
#define | TXT_SERIAL "Serial" |
#define | TXT_SUCCESS2 "success" |
#define | TXT_FAILED "failed" |
#define | TXT_NAME "name" |
#define | TXT_VERSION "version" |
#define | TXT_FOCUS "focus" |
#define | TXT_ZOOM "zoom" |
#define | TXT_IRIS "iris" |
#define | TXT_LENS "lens" |
#define | TXT_IR_FILTER "ir_filter" |
#define | TXT_IR_PRESENT "ir_present" |
#define | TXT_SENS_PRESENT "sens_present" |
#define | TXT_BUSY "busy" |
#define | TXT_TYPE "type" |
#define | CMD_READ_ID "IDN" |
#define | CMD_READ_POS "GP" |
#define | CMD_READ_TYPE "GT" |
#define | CMD_READ_STATE "GS" |
#define | CMD_RESET "RST" |
#define | CMD_HOMING "INI" |
#define | CMD_SET_ABS "SETA:" |
#define | CMD_SET_REL "SETR:" |
#define | CMD_SUFFIX "\r\n" |
#define | RESP_FOCUS "F%hu" |
#define | RESP_ZOOM "Z%hu" |
#define | RESP_IRIS "P%hu" |
#define | TRUE_VAL '1' |
#define | DELIM ";" |
#define | LEN_CMD_READ_ID 3 |
#define | LEN_CMD_READ_POS 5 |
#define | LEN_CMD_READ_TYPE 7 |
#define | LEN_CMD_READ_STATE1 2 |
#define | LEN_CMD_READ_STATE2 3 |
#define | LEN_CMD_RESET 0 |
#define | LEN_CMD_HOMING 1 |
#define | LEN_CMD_SET_ABS 1 |
#define | LEN_CMD_SET_REL 1 |
#define | FW_MIN_STAT_POLL 1, 7, 2 |
#define | RX_TIMEOUT_OLD_FW 20000 |
#define | RX_TIMEOUT_NEW_FW 1000 |
#define | MSG(x) x CMD_SUFFIX |
#define | STR_MAX_SIZE 200 |
#define | GET_VAR_NAME(Variable) (#Variable) |
Typedefs | |
typedef int16_t | ATEsystem_PIRIS::Status_t |
typedef int16_t | ATEsystem_PIRIS::DevID_t |
typedef SmartPointer< IDevice > | ATEsystem_PIRIS::IPiris |
typedef IDevice *(* | ATEsystem_PIRIS::CreateDeviceFn) (void) |
Functions | |
std::ostream & | ATEsystem_PIRIS::operator<< (std::ostream &os, const Status stat) |
std::ostream & | ATEsystem_PIRIS::operator<< (std::ostream &os, const PirisDeviceType type) |
virtual Status | ATEsystem_PIRIS::Open (const PirisDevice &dev, VerboseLevel verbose=VerboseLevel::NONE)=0 |
virtual Status | ATEsystem_PIRIS::Close ()=0 |
virtual std::tuple< StatusEx, DataID > | ATEsystem_PIRIS::ReadID ()=0 |
virtual std::tuple< StatusEx, DataPosition > | ATEsystem_PIRIS::ReadPosition ()=0 |
virtual std::tuple< StatusEx, DataParams > | ATEsystem_PIRIS::ReadParams ()=0 |
virtual std::tuple< StatusEx, DataState > | ATEsystem_PIRIS::ReadState ()=0 |
virtual StatusEx | ATEsystem_PIRIS::DevReset ()=0 |
virtual StatusEx | ATEsystem_PIRIS::DevHoming ()=0 |
virtual StatusEx | ATEsystem_PIRIS::SetAbsolute (uint16_t focus=0, uint16_t zoom=0, uint16_t iris=0, bool ir_filter=false)=0 |
virtual StatusEx | ATEsystem_PIRIS::SetAbsolute (const FocusZoomIris< uint16_t > &values, bool ir_filter=false)=0 |
virtual StatusEx | ATEsystem_PIRIS::SetRelative (int16_t focus=0, int16_t zoom=0, int16_t iris=0)=0 |
virtual StatusEx | ATEsystem_PIRIS::SetRelative (const FocusZoomIris< int16_t > &values)=0 |
virtual YesNoNA | ATEsystem_PIRIS::GetFwPollSupport ()=0 |
virtual VerboseLevel | ATEsystem_PIRIS::GetVerboseLevel ()=0 |
virtual void | ATEsystem_PIRIS::SetVerboseLevel (VerboseLevel level)=0 |
virtual | ATEsystem_PIRIS::~IDevice ()=0 |
virtual Status | ATEsystem_PIRIS::close (bool ignore_err=false)=0 |
virtual Status | ATEsystem_PIRIS::write (const std::string data)=0 |
virtual Status | ATEsystem_PIRIS::read (std::string &data)=0 |
virtual Status | ATEsystem_PIRIS::flush (bool ignore_err=false)=0 |
virtual void | ATEsystem_PIRIS::remove ()=0 |
virtual | ATEsystem_PIRIS::~IComm ()=0 |
Variables | |
Interface | ATEsystem_PIRIS::IDevice |
Interface | ATEsystem_PIRIS::IComm |
Macro Definition Documentation
◆ CMD_HOMING
#define CMD_HOMING "INI" |
◆ CMD_READ_ID
#define CMD_READ_ID "IDN" |
◆ CMD_READ_POS
#define CMD_READ_POS "GP" |
◆ CMD_READ_STATE
#define CMD_READ_STATE "GS" |
◆ CMD_READ_TYPE
#define CMD_READ_TYPE "GT" |
◆ CMD_RESET
#define CMD_RESET "RST" |
◆ CMD_SET_ABS
#define CMD_SET_ABS "SETA:" |
◆ CMD_SET_REL
#define CMD_SET_REL "SETR:" |
◆ CMD_SUFFIX
#define CMD_SUFFIX "\r\n" |
◆ DELIM
#define DELIM ";" |
◆ ERR0
#define ERR0 "Command was not recognized." |
◆ ERR1
#define ERR1 "Wrong input parameter." |
◆ ERR2
#define ERR2 "Device is not initialized." |
◆ ERR3
#define ERR3 "Internal driver circuit is in error state (overheat, undervoltage)." |
◆ ERR4
#define ERR4 "Wrong lens type." |
◆ ERR5
#define ERR5 "Damaged or missing rear sensor." |
◆ ERR6
#define ERR6 "Step generator timer overflow." |
◆ ERR7
#define ERR7 "Device is busy." |
◆ ERR_PA
#define ERR_PA "Parse answer failed." |
◆ ERR_UN
#define ERR_UN "Unknown error." |
◆ FW_MIN_STAT_POLL
#define FW_MIN_STAT_POLL 1, 7, 2 |
◆ GET_VAR_NAME
#define GET_VAR_NAME | ( | Variable | ) | (#Variable) |
◆ LEN_CMD_HOMING
#define LEN_CMD_HOMING 1 |
◆ LEN_CMD_READ_ID
#define LEN_CMD_READ_ID 3 |
◆ LEN_CMD_READ_POS
#define LEN_CMD_READ_POS 5 |
◆ LEN_CMD_READ_STATE1
#define LEN_CMD_READ_STATE1 2 |
◆ LEN_CMD_READ_STATE2
#define LEN_CMD_READ_STATE2 3 |
◆ LEN_CMD_READ_TYPE
#define LEN_CMD_READ_TYPE 7 |
◆ LEN_CMD_RESET
#define LEN_CMD_RESET 0 |
◆ LEN_CMD_SET_ABS
#define LEN_CMD_SET_ABS 1 |
◆ LEN_CMD_SET_REL
#define LEN_CMD_SET_REL 1 |
◆ MSG
#define MSG | ( | x | ) | x CMD_SUFFIX |
◆ OK
#define OK "OK" |
◆ RESP_FOCUS
#define RESP_FOCUS "F%hu" |
◆ RESP_IRIS
#define RESP_IRIS "P%hu" |
◆ RESP_ZOOM
#define RESP_ZOOM "Z%hu" |
◆ RX_TIMEOUT_NEW_FW
#define RX_TIMEOUT_NEW_FW 1000 |
◆ RX_TIMEOUT_OLD_FW
#define RX_TIMEOUT_OLD_FW 20000 |
◆ STR_MAX_SIZE
#define STR_MAX_SIZE 200 |
◆ TRUE_VAL
#define TRUE_VAL '1' |
◆ TXT_ALREADY_OPEN
#define TXT_ALREADY_OPEN "Already open" |
◆ TXT_BUSY
#define TXT_BUSY "busy" |
◆ TXT_CLOSE_FAILED
#define TXT_CLOSE_FAILED "CLose failed" |
◆ TXT_DEV_SET_FAILED
#define TXT_DEV_SET_FAILED "Device set failed" |
◆ TXT_DEVICE_IS_BUSY
#define TXT_DEVICE_IS_BUSY "Device is busy" |
◆ TXT_DEVICE_IS_CLOSED
#define TXT_DEVICE_IS_CLOSED "Device is closed" |
◆ TXT_DEVICE_NOT_EXIST
#define TXT_DEVICE_NOT_EXIST "Device does not exist" |
◆ TXT_ETHERNET_CAMERA
#define TXT_ETHERNET_CAMERA "Ethernet camera (camera overiden)" |
◆ TXT_ETHERNET_DEVICE
#define TXT_ETHERNET_DEVICE "Ethernet device" |
◆ TXT_ETHERNET_PYLON
#define TXT_ETHERNET_PYLON "Ethernet pylon (camera overiden)" |
◆ TXT_FAILED
#define TXT_FAILED "failed" |
◆ TXT_FOCUS
#define TXT_FOCUS "focus" |
◆ TXT_GENERAL_ERROR
#define TXT_GENERAL_ERROR "General Error" |
◆ TXT_INTERNAL_ERROR
#define TXT_INTERNAL_ERROR "Internal error" |
◆ TXT_INVALID_DEVICE
#define TXT_INVALID_DEVICE "Invalid device" |
◆ TXT_IR_FILTER
#define TXT_IR_FILTER "ir_filter" |
◆ TXT_IR_PRESENT
#define TXT_IR_PRESENT "ir_present" |
◆ TXT_IRIS
#define TXT_IRIS "iris" |
◆ TXT_LENS
#define TXT_LENS "lens" |
◆ TXT_NAME
#define TXT_NAME "name" |
◆ TXT_OPEN_FAILED
#define TXT_OPEN_FAILED "Open failed" |
◆ TXT_PARSE_ANSWER_FAIL
#define TXT_PARSE_ANSWER_FAIL "Parse answer failed" |
◆ TXT_READ_ERROR
#define TXT_READ_ERROR "Read error" |
◆ TXT_RX_TIMEOUT
#define TXT_RX_TIMEOUT "Receive timeout" |
◆ TXT_SCAN_FAILED
#define TXT_SCAN_FAILED "Scan failed" |
◆ TXT_SENS_PRESENT
#define TXT_SENS_PRESENT "sens_present" |
◆ TXT_SERIAL
#define TXT_SERIAL "Serial" |
◆ TXT_SUCCESS
#define TXT_SUCCESS "Success" |
◆ TXT_SUCCESS2
#define TXT_SUCCESS2 "success" |
◆ TXT_TYPE
#define TXT_TYPE "type" |
◆ TXT_VERSION
#define TXT_VERSION "version" |
◆ TXT_WRITE_ERROR
#define TXT_WRITE_ERROR "Write error" |
◆ TXT_WRONG_MODE
#define TXT_WRONG_MODE "Wrong mode" |
◆ TXT_ZOOM
#define TXT_ZOOM "zoom" |
Generated by
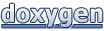