Alpha Engine
|
Header file for the graphics library. More...
Go to the source code of this file.
Classes | |
struct | AEGfxVertexList |
struct | AEGfxTexture |
Typedefs | |
typedef struct AEGfxVertexBuffer | AEGfxVertexBuffer |
typedef struct AEGfxVertexList | AEGfxVertexList |
typedef struct AEGfxSurface | AEGfxSurface |
typedef struct AEGfxTexture | AEGfxTexture |
Enumerations | |
enum | AEGfxRenderMode { AE_GFX_RM_NONE, AE_GFX_RM_COLOR, AE_GFX_RM_TEXTURE, AE_GFX_RM_NUM } |
enum | AEGfxBlendMode { AE_GFX_BM_NONE = 0, AE_GFX_BM_BLEND, AE_GFX_BM_ADD, AE_GFX_BM_NUM } |
enum | AEGfxTextureMode { AE_GFX_TM_PRECISE = 0, AE_GFX_TM_AVERAGE } |
enum | AEGfxMeshDrawMode { AE_GFX_MDM_POINTS = 0, AE_GFX_MDM_LINES, AE_GFX_MDM_LINES_STRIP, AE_GFX_MDM_TRIANGLES, AE_GFX_MDM_NUM } |
Functions | |
s32 | AEGfxInit (s32 Width, s32 Height) |
Initialize AEGraphics. More... | |
DECLARE_FUNCTION_FOR_ANDROID_2_INT (AEGfxInit, s32 Width, s32 Height) | |
void | AEGfxReset () |
Reset AEGraphics. More... | |
void | AEGfxExit () |
Free AEGraphics. More... | |
void | AEGfxStart () |
Tell AEGraphics that a new frame is starting. More... | |
DECLARE_FUNCTION_FOR_ANDROID (AEGfxStart) | |
void | AEGfxEnd () |
Tell AEGraphics that the current frame is ending. More... | |
DECLARE_FUNCTION_FOR_ANDROID (AEGfxEnd) | |
void | AEGfxSetBackgroundColor (f32 Red, f32 Green, f32 Blue) |
Set the background colour to selected RGB values. More... | |
void | AEGfxSetRenderMode (AEGfxRenderMode RenderMode) |
Set the render mode. More... | |
void | AEGfxSetBlendMode (AEGfxBlendMode BlendMode) |
Set the blend mode. More... | |
f32 | AEGfxGetWinMinX (void) |
Get the minimum X world coordinate. More... | |
f32 | AEGfxGetWinMaxX (void) |
Get the maximum X world coordinate. More... | |
f32 | AEGfxGetWinMinY (void) |
Get the minimum Y world coordinate. More... | |
f32 | AEGfxGetWinMaxY (void) |
Get the maximum Y world coordinate. More... | |
void | AEGfxSetCamPosition (f32 X, f32 Y) |
Set the camera's X and Y position. More... | |
void | AEGfxGetCamPosition (f32 *pX, f32 *pY) |
Get the camera's X and Y position. More... | |
void | AEGfxSetPosition (f32 X, f32 Y) |
Set the position (translation) to render from. More... | |
void | AEGfxSetTransform (f32 pTransform[3][3]) |
Set the new global transformation matrix. More... | |
void | AEGfxSetTransform3D (f32 pTransform[4][4]) |
Set the new global transformation matrix. More... | |
void | AEGfxSetTransparency (f32 Alpha) |
Set the new global transparency value. More... | |
void | AEGfxSetBlendColor (f32 Red, f32 Green, f32 Blue, f32 Alpha) |
Sets a color (RGBA) that will be used to blend with the original material. More... | |
void | AEGfxSetTintColor (float Red, float Green, float Blue, float Alpha) |
Sets a color (RGBA) that will be used to tint the original material. More... | |
void | AEGfxMeshStart () |
Instruct AEGraphics to start creating a new mesh. More... | |
void | AEGfxTriAdd (f32 x0, f32 y0, u32 c0, f32 tu0, f32 tv0, f32 x1, f32 y1, u32 c1, f32 tu1, f32 tv1, f32 x2, f32 y2, u32 c2, f32 tu2, f32 tv2) |
Add a new triangle to the mesh. More... | |
void | AEGfxVertexAdd (f32 x0, f32 y0, u32 c0, f32 tu0, f32 tv0) |
Add a new point to the mesh. More... | |
AEGfxVertexList * | AEGfxMeshEnd () |
Instruct AEGraphics to end creating of new mesh. More... | |
void | AEGfxMeshDraw (AEGfxVertexList *pVertexList, AEGfxMeshDrawMode MeshDrawMode) |
Draw the mesh on screen. More... | |
void | AEGfxMeshFree (AEGfxVertexList *pVertexList) |
Free the mesh. More... | |
AEGfxTexture * | AEGfxTextureLoad (const s8 *pFileName) |
Load a texture file into memory. More... | |
void | AEGfxTextureSet (AEGfxTexture *pTexture, f32 offset_x, f32 offset_y) |
Set a texture to be used for rendering. More... | |
void | AEGfxTextureUnload (AEGfxTexture *pTexture) |
Unload a texture file from memory. More... | |
AEGfxTexture * | AEGfxTextureLoadFromMemory (u8 *pColors, u32 Width, u32 Height) |
Load a texture from data in memory. More... | |
void | AEGfxSaveTextureToFile (AEGfxTexture *pTexture, s8 *pFileName) |
Save a texture from memory to file. More... | |
void | AEGfxSetTextureMode (AEGfxTextureMode TextureMode) |
Set the texture mode. More... | |
void | AEGfxPoint (f32 x0, f32 y0, f32 z0, u32 c0) |
Draw a point on screen. More... | |
void | AEGfxLine (f32 x0, f32 y0, f32 z0, f32 r0, f32 g0, f32 b0, f32 a0, f32 x1, f32 y1, f32 z1, f32 r1, f32 g1, f32 b1, f32 a1) |
Draw a line on screen. More... | |
void | AEGfxTri (f32 x0, f32 y0, f32 z0, u32 c0, f32 x1, f32 y1, f32 z1, u32 c1, f32 x2, f32 y2, f32 z2, u32 c2) |
Draw a triangle on screen. More... | |
void | AEGfxQuad (f32 x0, f32 y0, f32 z0, u32 c0, f32 x1, f32 y1, f32 z1, u32 c1, f32 x2, f32 y2, f32 z2, u32 c2, f32 x3, f32 y3, f32 z3, u32 c3) |
Draw a rectangle on screen. More... | |
void | AEGfxBox (f32 x0, f32 y0, f32 z0, f32 sizeX, f32 sizeY, f32 sizeZ, u32 c0, u32 c1) |
Draw a 3D box on screen. More... | |
void | AEGfxSphere (f32 x0, f32 y0, f32 z0, f32 radius, u32 c0, u32 c1, u32 division) |
Draw a 3D sphere on screen. More... | |
void | AEGfxCone (f32 x0, f32 y0, f32 z0, f32 x1, f32 y1, f32 z1, f32 radius, u32 c0, u32 c1, u32 division) |
Draw a 3D cone on screen. More... | |
void | AEGfxAxis (f32 scale) |
Draw a 3D axis on screen. More... | |
u32 | AEGfxColInterp (u32 c0, u32 c1, f32 t) |
Get the interpolation between two colours (c0 and c1) at time interval t. More... | |
void | AEGfxPrint (s32 x, s32 y, u32 color, s8 *pStr) |
Render text onto the screen. More... | |
Detailed Description
Header file for the graphics library.
- Project: Alpha Engine
- Author
- Sun Tjen Fam
- Date
- January 30, 2008
- Copyright
- Copyright (C) 2013 DigiPen Institute of Technology. Reproduction or disclosure of this file or its contents without the prior written consent of DigiPen Institute of Technology is prohibited.
Definition in file AEGraphics.h.
Typedef Documentation
typedef struct AEGfxSurface AEGfxSurface |
Definition at line 80 of file AEGraphics.h.
typedef struct AEGfxTexture AEGfxTexture |
typedef struct AEGfxVertexBuffer AEGfxVertexBuffer |
Definition at line 70 of file AEGraphics.h.
typedef struct AEGfxVertexList AEGfxVertexList |
Enumeration Type Documentation
enum AEGfxBlendMode |
AEEngine blend option
Enumerator | |
---|---|
AE_GFX_BM_NONE |
No blending. |
AE_GFX_BM_BLEND |
Color blending. |
AE_GFX_BM_ADD |
Additive blending. |
AE_GFX_BM_NUM |
Definition at line 37 of file AEGraphics.h.
enum AEGfxMeshDrawMode |
Enumerator | |
---|---|
AE_GFX_MDM_POINTS | |
AE_GFX_MDM_LINES | |
AE_GFX_MDM_LINES_STRIP | |
AE_GFX_MDM_TRIANGLES | |
AE_GFX_MDM_NUM |
Definition at line 56 of file AEGraphics.h.
enum AEGfxRenderMode |
AEEngine render option
Enumerator | |
---|---|
AE_GFX_RM_NONE |
No render. |
AE_GFX_RM_COLOR |
Color rendering. |
AE_GFX_RM_TEXTURE |
Texture rendering. |
AE_GFX_RM_NUM |
Definition at line 24 of file AEGraphics.h.
enum AEGfxTextureMode |
Enumerator | |
---|---|
AE_GFX_TM_PRECISE | |
AE_GFX_TM_AVERAGE |
Definition at line 48 of file AEGraphics.h.
Function Documentation
void AEGfxAxis | ( | f32 | scale | ) |
Draw a 3D axis on screen.
The axis is rendered on the center of the screen with size scale.
- Warning
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] scale Size of the axis
- Return values
-
void No return.
Draw a 3D box on screen.
The box is centered around the point (x0, y0, z0) and sizeX, sizeY and sizeZ are the width, height and depth. c0 and c1 are the box color. Default values are white and grey.
- Warning
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] x0 X coordinate of the center of the box [in] y0 Y coordinate of the center of the box [in] z0 Z coordinate of the center of the box [in] sizeX Width of the box [in] sizeY Height of the box [in] sizeZ Depth of the box [in] c0 First color of the box. [in] c1 Second color of the box.
- Return values
-
void No return.
Get the interpolation between two colours (c0 and c1) at time interval t.
- Warning
- t should be between 0.0f to 1.0f.
- Parameters
-
[in] c0 The first colour. [in] c1 The second colour. [in] t The time interval to calculate the interpolation at. At t = 0.0f, the result will be c0. At t = 1.0f, the result will be c1.
- Return values
-
void No return.
void AEGfxCone | ( | f32 | x0, |
f32 | y0, | ||
f32 | z0, | ||
f32 | x1, | ||
f32 | y1, | ||
f32 | z1, | ||
f32 | radius, | ||
u32 | c0, | ||
u32 | c1, | ||
u32 | division | ||
) |
Draw a 3D cone on screen.
The base of the cone is centered around the point (x0, y0, z0) with size radius. The tip of the cone is at point(x1, y1, z1). c0 and c1 are the sphere color. Default colors are white and grey. Division is the resolution of the cone, or how many parts the cone consists of. Default value is 8.
- Warning
- This function is not implemented.
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] x0 X coordinate of the center of the base of cone [in] y0 Y coordinate of the center of the base of cone [in] z0 Z coordinate of the tip of cone [in] x1 X coordinate of the tip of cone [in] y1 Y coordinate of the tip of cone [in] z1 Z coordinate of the tip of cone [in] radius Radius of the cone [in] c0 First color of the cone. [in] c1 Second color of the cone. [in] division Number of parts to divide the cone by.
- Return values
-
void No return.
void AEGfxEnd | ( | ) |
Tell AEGraphics that the current frame is ending.
- Warning
- This function is already called in AESysFrameEnd.
- Return values
-
void No return.
void AEGfxExit | ( | ) |
Free AEGraphics.
- Warning
- This function is already called in AESysExit.
- Return values
-
void No return.
Get the camera's X and Y position.
- Parameters
-
[out] pX Pointer to f32 to store the X position in. [out] pY Pointer to f32 to store the Y position in.
- Return values
-
void No return.
f32 AEGfxGetWinMaxX | ( | void | ) |
Get the maximum X world coordinate.
- Return values
-
f32 Returns the maximum X world coordinate.
f32 AEGfxGetWinMaxY | ( | void | ) |
Get the maximum Y world coordinate.
- Return values
-
f32 Returns the maximum Y world coordinate.
f32 AEGfxGetWinMinX | ( | void | ) |
Get the minimum X world coordinate.
- Return values
-
f32 Returns the minimum X world coordinate.
f32 AEGfxGetWinMinY | ( | void | ) |
Get the minimum Y world coordinate.
- Return values
-
f32 Returns the minimum Y world coordinate.
Initialize AEGraphics.
- Warning
- This function is already called in AESysInit.
- Parameters
-
[in] Width Set the width of the window. [in] Height Set the height of the window.
- Return values
-
s32 Return 1 if initialization is successful. Else return 0.
void AEGfxLine | ( | f32 | x0, |
f32 | y0, | ||
f32 | z0, | ||
f32 | r0, | ||
f32 | g0, | ||
f32 | b0, | ||
f32 | a0, | ||
f32 | x1, | ||
f32 | y1, | ||
f32 | z1, | ||
f32 | r1, | ||
f32 | g1, | ||
f32 | b1, | ||
f32 | a1 | ||
) |
Draw a line on screen.
The line is defined by 2 points: pt0 and pt1.
- Warning
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] x0 X coordinate of pt0 [in] y0 Y coordinate of pt0 [in] z0 Z coordinate of pt0 [in] r0 Percentage of red of pt0. Range from 0.0f to 1.0f. [in] g0 Percentage of green of pt0. Range from 0.0f to 1.0f. [in] b0 Percentage of blue of pt0. Range from 0.0f to 1.0f. [in] a0 Percentage of alpha (transparency) of pt0. Range from 0.0f (clear) to 1.0f (opaque). [in] x1 X coordinate of pt1 [in] y1 Y coordinate of pt1 [in] z1 Z coordinate of pt1 [in] r1 Percentage of red of pt1. Range from 0.0f to 1.0f. [in] g1 Percentage of green of pt1. Range from 0.0f to 1.0f. [in] b1 Percentage of blue of pt1. Range from 0.0f to 1.0f. [in] a1 Percentage of alpha (transparency) of pt1. Range from 0.0f (clear) to 1.0f (opaque).
- Return values
-
void No return.
void AEGfxMeshDraw | ( | AEGfxVertexList * | pVertexList, |
AEGfxMeshDrawMode | MeshDrawMode | ||
) |
Draw the mesh on screen.
Render the mesh onto the screen using the MeshDrawMode stated.
- Parameters
-
[in] pVertexList Pointer to the AEGfxVertexList to be rendered. [in] MeshDrawMode The AEGfxMeshDrawMode to use for rendering the mesh.
- Return values
-
void No return.
AEGfxVertexList * AEGfxMeshEnd | ( | ) |
Instruct AEGraphics to end creating of new mesh.
Call this function once after all the points have been added to the mesh.
- Return values
-
AEGfxVertexList* Returns a AEGfxVertexList pointer to the new mesh.
void AEGfxMeshFree | ( | AEGfxVertexList * | pVertexList | ) |
Free the mesh.
Release the mesh from AEGraphics memory. The mesh is destroyed and can no longer be used.
- Parameters
-
[in] pVertexList Pointer to the AEGfxVertexList to be free.
- Return values
-
void No return.
void AEGfxMeshStart | ( | ) |
Instruct AEGraphics to start creating a new mesh.
Call this function once before adding any points to the mesh.
- Return values
-
void No return.
Draw a point on screen.
The point is defined by x0, y0, z0 with color c0.
- Warning
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] x0 X coordinate of point [in] y0 Y coordinate of point [in] z0 Z coordinate of point [in] c0 Color value of point
- Return values
-
void No return.
Render text onto the screen.
Render a null-terminated string of text (pStr) onto the screen starting at the point(x, y) using the color set.
- Parameters
-
[in] x X coordinate to start rendering the text from. This is given in terms of actual windows coordinates. [in] y X coordinate to start rendering the text from. This is given in terms of actual windows coordinates. [in] color The color to render the text with. [in] pStr Pointer to a NULL-terminated string containing the text to be rendered onto the screen.
- Return values
-
void No return.
void AEGfxQuad | ( | f32 | x0, |
f32 | y0, | ||
f32 | z0, | ||
u32 | c0, | ||
f32 | x1, | ||
f32 | y1, | ||
f32 | z1, | ||
u32 | c1, | ||
f32 | x2, | ||
f32 | y2, | ||
f32 | z2, | ||
u32 | c2, | ||
f32 | x3, | ||
f32 | y3, | ||
f32 | z3, | ||
u32 | c3 | ||
) |
Draw a rectangle on screen.
The rectangle is defined by 4 points: pt0, pt1, pt2 and pt3. The points are listed in a counter-clockwise order.
- Warning
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] x0 X coordinate of pt0 [in] y0 Y coordinate of pt0 [in] z0 Z coordinate of pt0 [in] c0 Color value of pt0 [in] x1 X coordinate of pt1 [in] y1 Y coordinate of pt1 [in] z1 Z coordinate of pt1 [in] c1 Color value of pt1 [in] x2 X coordinate of pt2 [in] y2 Y coordinate of pt2 [in] z2 Z coordinate of pt2 [in] c2 Color value of pt2 [in] x3 X coordinate of pt3 [in] y3 Y coordinate of pt3 [in] z3 Z coordinate of pt3 [in] c3 Color value of pt3
- Return values
-
void No return.
void AEGfxReset | ( | ) |
Reset AEGraphics.
- Warning
- This function is already called in AESysReset.
- Return values
-
void No return.
void AEGfxSaveTextureToFile | ( | AEGfxTexture * | pTexture, |
s8 * | pFileName | ||
) |
Save a texture from memory to file.
- Parameters
-
[in] pTexture Pointer to AEGfxTexture to be saved. [in] pFileName Pointer to a null-terminated string containing the relative path of the file.
- Return values
-
void No return.
Set the background colour to selected RGB values.
- Parameters
-
[in] Red Percentage of red. Range from 0.0f to 1.0f. [in] Green Percentage of green. Range from 0.0f to 1.0f. [in] Blue Percentage of blue. Range from 0.0f to 1.0f.
- Return values
-
void No return.
Sets a color (RGBA) that will be used to blend with the original material.
- Parameters
-
[in] Red Percentage of red. Range from 0.0f to 1.0f. [in] Green Percentage of green. Range from 0.0f to 1.0f. [in] Blue Percentage of blue. Range from 0.0f to 1.0f. [in] Alpha Percentage of alpha (transparency). Range from 0.0f (clear) to 1.0f (opaque).
- Return values
-
void No return.
void AEGfxSetBlendMode | ( | AEGfxBlendMode | BlendMode | ) |
Set the blend mode.
- Parameters
-
[in] BlendMode The AEGfxBlendMode to set.
- Return values
-
void No return.
Set the camera's X and Y position.
- Parameters
-
[in] X X position to set camera to. [in] Y Y position to set camera to.
- Return values
-
void No return.
Set the position (translation) to render from.
- Parameters
-
[in] X X position to render from. [out] Y Y position to render from.
- Return values
-
void No return.
void AEGfxSetRenderMode | ( | AEGfxRenderMode | RenderMode | ) |
Set the render mode.
- Warning
- This function should be called at least once per frame, before any rendering is done.
- Parameters
-
[in] RenderMode The AEGfxRenderMode to set.
- Return values
-
void No return.
void AEGfxSetTextureMode | ( | AEGfxTextureMode | TextureMode | ) |
Set the texture mode.
- Parameters
-
[in] TextureMode The AEGfxTextureMode to set.
- Return values
-
void No return.
void AEGfxSetTintColor | ( | float | Red, |
float | Green, | ||
float | Blue, | ||
float | Alpha | ||
) |
Sets a color (RGBA) that will be used to tint the original material.
- Parameters
-
[in] Red Percentage of red. Range from 0.0f to 1.0f. [in] Green Percentage of green. Range from 0.0f to 1.0f. [in] Blue Percentage of blue. Range from 0.0f to 1.0f. [in] Alpha Percentage of alpha (transparency). Range from 0.0f (clear) to 1.0f (opaque).
- Return values
-
void No return.
void AEGfxSetTransform | ( | f32 | pTransform[3][3] | ) |
Set the new global transformation matrix.
The new matrix will be applied to all object drawn after this function was called.
- Parameters
-
[in] pTransform Pointer to a 3x3 matrix to set with. User may pass in a AEMtx33.m.
- Return values
-
void No return.
void AEGfxSetTransform3D | ( | f32 | pTransform[4][4] | ) |
Set the new global transformation matrix.
The new matrix will be applied to all object drawn after this function was called.
- Parameters
-
[in] pTransform Pointer to a 4x4 matrix to set with.
- Return values
-
void No return.
void AEGfxSetTransparency | ( | f32 | Alpha | ) |
Set the new global transparency value.
The new transparency value will be applied to all object drawn after this function was called.
- Parameters
-
[in] Alpha Percentage of alpha (transparency). Range from 0.0f (clear) to 1.0f (opaque).
- Return values
-
void No return.
Draw a 3D sphere on screen.
The sphere is centered around the point (x0, y0, z0) with size radius. c0 and c1 are the sphere color. Default colors are white and grey. Division is the resolution, or how many parts the sphere consists of. Default value is 8.
- Warning
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] x0 X coordinate of the center of the sphere [in] y0 Y coordinate of the center of the sphere [in] z0 Z coordinate of the center of the sphere [in] radius Radius of the sphere [in] c0 First color of the sphere. [in] c1 Second color of the sphere. [in] division Number of parts to divide the sphere by.
- Return values
-
void No return.
void AEGfxStart | ( | ) |
Tell AEGraphics that a new frame is starting.
- Warning
- This function is already called in AESysFrameStart.
- Return values
-
void No return.
AEGfxTexture * AEGfxTextureLoad | ( | const s8 * | pFileName | ) |
Load a texture file into memory.
- Parameters
-
[in] pFileName Pointer to a null-terminated string containing the relative path of the file to be loaded.
- Return values
-
AEGfxTexture* Returns a AEGfxTexture pointer to the loaded texture.
AEGfxTexture * AEGfxTextureLoadFromMemory | ( | u8 * | pColors, |
u32 | Width, | ||
u32 | Height | ||
) |
Load a texture from data in memory.
- Parameters
-
[in] pColors Pointer to an array containing the data. [in] Width The width of the texture. [in] Height The height of the texture.
- Return values
-
AEGfxTexture* Returns a AEGfxTexture pointer to the loaded texture.
void AEGfxTextureSet | ( | AEGfxTexture * | pTexture, |
f32 | offset_x, | ||
f32 | offset_y | ||
) |
Set a texture to be used for rendering.
- Parameters
-
[in] pTexture Pointer to the AEGfxTexture to be used. Set to null if not using texture. [in] offset_x X offset for the texture. Range from 0.0f to 1.0f. [in] offset_y Y offset for the texture. Range from 0.0f to 1.0f.
- Return values
-
void No return.
void AEGfxTextureUnload | ( | AEGfxTexture * | pTexture | ) |
Unload a texture file from memory.
- Parameters
-
[in] pTexture Pointer to AEGfxTexture to be unloaded.
- Return values
-
void No return.
void AEGfxTri | ( | f32 | x0, |
f32 | y0, | ||
f32 | z0, | ||
u32 | c0, | ||
f32 | x1, | ||
f32 | y1, | ||
f32 | z1, | ||
u32 | c1, | ||
f32 | x2, | ||
f32 | y2, | ||
f32 | z2, | ||
u32 | c2 | ||
) |
Draw a triangle on screen.
The triangle is defined by 3 points: pt0, pt1 and pt2. The points are listed in a counter-clockwise order.
- Warning
- Do not call this function when AEGraphics is building mesh.
- Function may be slow. Use with caution.
- Parameters
-
[in] x0 X coordinate of pt0 [in] y0 Y coordinate of pt0 [in] z0 Z coordinate of pt0 [in] c0 Color value of pt0 [in] x1 X coordinate of pt1 [in] y1 Y coordinate of pt1 [in] z1 Z coordinate of pt1 [in] c1 Color value of pt1 [in] x2 X coordinate of pt2 [in] y2 Y coordinate of pt2 [in] z2 Z coordinate of pt2 [in] c2 Color value of pt2
- Return values
-
void No return.
void AEGfxTriAdd | ( | f32 | x0, |
f32 | y0, | ||
u32 | c0, | ||
f32 | tu0, | ||
f32 | tv0, | ||
f32 | x1, | ||
f32 | y1, | ||
u32 | c1, | ||
f32 | tu1, | ||
f32 | tv1, | ||
f32 | x2, | ||
f32 | y2, | ||
u32 | c2, | ||
f32 | tu2, | ||
f32 | tv2 | ||
) |
Add a new triangle to the mesh.
The triangle is defined by 3 points: pt0, pt1 and pt2. The points are listed in a counter-clockwise order. The Z-value of the points are set to default 0.
- Parameters
-
[in] x0 X coordinate of pt0. [in] y0 Y coordinate of pt0. [in] c0 Color value of pt0. [in] tu0 Texture translation of pt0. [in] tv0 Texture translation of pt0. [in] x1 X coordinate of pt1. [in] y1 Y coordinate of pt1. [in] c1 Color value of pt1. [in] tu1 Texture translation of pt1. [in] tv1 Texture translation of pt1. [in] x2 X coordinate of pt2. [in] y2 Y coordinate of pt2. [in] c2 Color value of pt2. [in] tu2 Texture translation of pt2. [in] tv2 Texture translation of pt2.
- Return values
-
void No return.
Add a new point to the mesh.
The Z-value of the point are set to default 0.
- Parameters
-
[in] x0 X coordinate of the point. [in] y0 Y coordinate of the point. [in] c0 Color value of the point. [in] tu0 Texture translation of the point. [in] tv0 Texture translation of the point.
- Return values
-
void No return.
DECLARE_FUNCTION_FOR_ANDROID | ( | AEGfxStart | ) |
DECLARE_FUNCTION_FOR_ANDROID | ( | AEGfxEnd | ) |
Generated on Sat Jan 4 2014 02:06:22 for Alpha Engine by
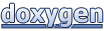