![]() |
|
GFXStateBlockData Class Reference
[GFX]
A state block description for rendering. More...
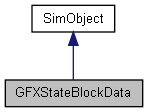
Public Attributes | |
Alpha Test | |
bool | alphaDefined |
Set to true if the alpha test state is not all defaults. | |
bool | alphaTestEnable |
Enables per-pixel alpha testing. The default is false. | |
GFXCmpFunc | alphaTestFunc |
The test function used to accept or reject a pixel based on its alpha value. The default is GFXCmpGreaterEqual. | |
int | alphaTestRef |
The reference alpha value against which pixels are tested. The default is zero. | |
Alpha Blending | |
bool | blendDefined |
Set to true if the alpha blend state is not all defaults. | |
GFXBlend | blendDest |
The destination blend state. The default is GFXBlendZero. | |
bool | blendEnable |
Enables alpha blending. The default is false. | |
GFXBlendOp | blendOp |
The arithmetic operation applied to alpha blending. The default is GFXBlendOpAdd. | |
GFXBlend | blendSrc |
The source blend state. The default is GFXBlendOne. | |
Color Write | |
bool | colorWriteAlpha |
Enables alpha channel writes. The default is true. | |
bool | colorWriteBlue |
Enables blue channel writes. The default is true. | |
bool | colorWriteDefined |
Set to true if the color write state is not all defaults. | |
bool | colorWriteGreen |
Enables green channel writes. The default is true. | |
bool | colorWriteRed |
Enables red channel writes. The default is true. | |
Culling | |
bool | cullDefined |
Set to true if the culling state is not all defaults. | |
GFXCullMode | cullMode |
Defines how back facing triangles are culled if at all. The default is GFXCullCCW. | |
Fixed Function | |
bool | ffLighting |
Enables fixed function lighting when rendering without a shader on geometry with vertex normals. The default is false. | |
bool | vertexColorEnable |
Enables fixed function vertex coloring when rendering without a shader. The default is false. | |
Sampler States | |
bool | samplersDefined |
Set to true if the sampler states are not all defaults. | |
GFXSamplerStateData | samplerStates [16] |
The array of texture sampler states. | |
ColorI | textureFactor |
The color used for multiple-texture blending with the GFXTATFactor texture-blending argument or the GFXTOPBlendFactorAlpha texture-blending operation. The default is opaque white (255, 255, 255, 255). | |
Separate Alpha Blending | |
bool | separateAlphaBlendDefined |
Set to true if the seperate alpha blend state is not all defaults. | |
GFXBlend | separateAlphaBlendDest |
The destination blend state. The default is GFXBlendZero. | |
bool | separateAlphaBlendEnable |
Enables the separate blend mode for the alpha channel. The default is false. | |
GFXBlendOp | separateAlphaBlendOp |
The arithmetic operation applied to separate alpha blending. The default is GFXBlendOpAdd. | |
GFXBlend | separateAlphaBlendSrc |
The source blend state. The default is GFXBlendOne. | |
Stencil | |
bool | stencilDefined |
Set to true if the stencil state is not all defaults. | |
bool | stencilEnable |
Enables stenciling. The default is false. | |
GFXStencilOp | stencilFailOp |
The stencil operation to perform if the stencil test fails. The default is GFXStencilOpKeep. | |
GFXCmpFunc | stencilFunc |
The comparison function to test the reference value to a stencil buffer entry. The default is GFXCmpNever. | |
int | stencilMask |
The mask applied to the reference value and each stencil buffer entry to determine the significant bits for the stencil test. The default is 0xFFFFFFFF. | |
GFXStencilOp | stencilPassOp |
The stencil operation to perform if both the stencil and the depth tests pass. The default is GFXStencilOpKeep. | |
int | stencilRef |
The reference value for the stencil test. The default is zero. | |
int | stencilWriteMask |
The write mask applied to values written into the stencil buffer. The default is 0xFFFFFFFF. | |
GFXStencilOp | stencilZFailOp |
The stencil operation to perform if the stencil test passes and the depth test fails. The default is GFXStencilOpKeep. | |
Depth | |
float | zBias |
A floating-point bias used when comparing depth values. The default is zero. | |
bool | zDefined |
Set to true if the depth state is not all defaults. | |
bool | zEnable |
Enables z-buffer reads. The default is true. | |
GFXCmpFunc | zFunc |
The depth comparision function which a pixel must pass to be written to the z-buffer. The default is GFXCmpLessEqual. | |
float | zSlopeBias |
An additional floating-point bias based on the maximum depth slop of the triangle being rendered. The default is zero. | |
bool | zWriteEnable |
Enables z-buffer writes. The default is true. |
Detailed Description
A state block description for rendering.
This object is used with ShaderData in CustomMaterial and PostEffect to define the render state.
- Example:
singleton GFXStateBlockData( PFX_DOFDownSampleStateBlock ) { zDefined = true; zEnable = false; zWriteEnable = false; samplersDefined = true; samplerStates[0] = SamplerClampLinear; samplerStates[1] = SamplerClampPoint; // Copy the clamped linear sampler, but change // the u coord to wrap for this special case. samplerStates[2] = new GFXSamplerStateData( : SamplerClampLinear ) { addressModeU = GFXAddressWrap; }; };
- Note:
- The 'xxxxDefined' fields are used to know what groups of fields are modified when combining multiple state blocks in material processing. You should take care to enable the right ones when setting values.
Member Data Documentation
Set to true if the alpha test state is not all defaults.
Enables per-pixel alpha testing. The default is false.
The test function used to accept or reject a pixel based on its alpha value. The default is GFXCmpGreaterEqual.
The reference alpha value against which pixels are tested. The default is zero.
Set to true if the alpha blend state is not all defaults.
The destination blend state. The default is GFXBlendZero.
Enables alpha blending. The default is false.
The arithmetic operation applied to alpha blending. The default is GFXBlendOpAdd.
The source blend state. The default is GFXBlendOne.
Enables alpha channel writes. The default is true.
Enables blue channel writes. The default is true.
Set to true if the color write state is not all defaults.
Enables green channel writes. The default is true.
Enables red channel writes. The default is true.
Set to true if the culling state is not all defaults.
Defines how back facing triangles are culled if at all. The default is GFXCullCCW.
Enables fixed function lighting when rendering without a shader on geometry with vertex normals. The default is false.
Set to true if the sampler states are not all defaults.
The array of texture sampler states.
- Note:
- Not all graphics devices support 16 samplers. In general all systems support 4 samplers with most modern cards doing 8.
Set to true if the seperate alpha blend state is not all defaults.
The destination blend state. The default is GFXBlendZero.
Enables the separate blend mode for the alpha channel. The default is false.
The arithmetic operation applied to separate alpha blending. The default is GFXBlendOpAdd.
The source blend state. The default is GFXBlendOne.
Set to true if the stencil state is not all defaults.
Enables stenciling. The default is false.
The stencil operation to perform if the stencil test fails. The default is GFXStencilOpKeep.
The comparison function to test the reference value to a stencil buffer entry. The default is GFXCmpNever.
The mask applied to the reference value and each stencil buffer entry to determine the significant bits for the stencil test. The default is 0xFFFFFFFF.
The stencil operation to perform if both the stencil and the depth tests pass. The default is GFXStencilOpKeep.
The reference value for the stencil test. The default is zero.
The write mask applied to values written into the stencil buffer. The default is 0xFFFFFFFF.
The stencil operation to perform if the stencil test passes and the depth test fails. The default is GFXStencilOpKeep.
The color used for multiple-texture blending with the GFXTATFactor texture-blending argument or the GFXTOPBlendFactorAlpha texture-blending operation. The default is opaque white (255, 255, 255, 255).
Enables fixed function vertex coloring when rendering without a shader. The default is false.
float GFXStateBlockData::zBias |
A floating-point bias used when comparing depth values. The default is zero.
Set to true if the depth state is not all defaults.
Enables z-buffer reads. The default is true.
The depth comparision function which a pixel must pass to be written to the z-buffer. The default is GFXCmpLessEqual.
An additional floating-point bias based on the maximum depth slop of the triangle being rendered. The default is zero.
Enables z-buffer writes. The default is true.