STM32L152C-Discovery BSP User Manual
|
stm32l152c_discovery_glass_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152c_discovery_glass_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the LCD Glass driver for LCD Module of 00006 * STM32L152C-Discovery board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l152c_discovery_glass_lcd.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32L152C_DISCOVERY 00045 * @{ 00046 */ 00047 00048 /** @defgroup STM32L152C_Discovery_GLASS_LCD Glass LCD 00049 * @brief This file includes the LCD Glass driver for LCD Module of 00050 * STM32L152C-DISCOVERY board. 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L152C-Discovery_GLASS_LCD_Private_Defines Private Defines 00055 * @{ 00056 */ 00057 #define ASCII_CHAR_0 0x30 /* 0 */ 00058 #define ASCII_CHAR_AT_SYMBOL 0x40 /* @ */ 00059 #define ASCII_CHAR_LEFT_OPEN_BRACKET 0x5B /* [ */ 00060 #define ASCII_CHAR_APOSTROPHE 0x60 /* ` */ 00061 #define ASCII_CHAR_LEFT_OPEN_BRACE 0x7B /* ( */ 00062 /** 00063 * @} 00064 */ 00065 00066 00067 /** @defgroup STM32L152C-Discovery_GLASS_LCD_Private_Variables Private Variables 00068 * @{ 00069 */ 00070 00071 /* this variable can be used for accelerate the scrolling exit when push user button */ 00072 __IO uint8_t bLCDGlass_KeyPressed = 0; 00073 00074 /** 00075 @verbatim 00076 ================================================================================ 00077 GLASS LCD MAPPING 00078 ================================================================================ 00079 LCD allows to display informations on six 14-segment digits and 4 bars: 00080 00081 1 2 3 4 5 6 00082 ----- ----- ----- ----- ----- ----- 00083 |\|/| o |\|/| o |\|/| o |\|/| o |\|/| |\|/| BAR3 00084 -- -- -- -- -- -- -- -- -- -- -- -- BAR2 00085 |/|\| o |/|\| o |/|\| o |/|\| o |/|\| |/|\| BAR1 00086 ----- * ----- * ----- * ----- * ----- ----- BAR0 00087 00088 LCD segment mapping: 00089 -------------------- 00090 -----A----- _ 00091 |\ | /| COL |_| 00092 F H J K B 00093 | \ | / | _ 00094 --G-- --M-- COL |_| 00095 | / | \ | 00096 E Q P N C 00097 |/ | \| _ 00098 -----D----- DP |_| 00099 00100 An LCD character coding is based on the following matrix: 00101 COM 0 1 2 3 00102 SEG(n) { E , D , P , N } 00103 SEG(n+1) { M , C , COL , DP } 00104 SEG(23-n-1) { B , A , K , J } 00105 SEG(23-n) { G , F , Q , H } 00106 with n positif odd number. 00107 00108 The character 'A' for example is: 00109 ------------------------------- 00110 LSB { 1 , 0 , 0 , 0 } 00111 { 1 , 1 , 0 , 0 } 00112 { 1 , 1 , 0 , 0 } 00113 MSB { 1 , 1 , 0 , 0 } 00114 ------------------- 00115 'A' = F E 0 0 hexa 00116 00117 @endverbatim 00118 */ 00119 00120 LCD_HandleTypeDef LCDHandle; 00121 00122 /* Constant table for cap characters 'A' --> 'Z' */ 00123 const uint16_t CapLetterMap[26]= 00124 { 00125 /* A B C D E F G H I */ 00126 0xFE00, 0x6714, 0x1D00, 0x4714, 0x9D00, 0x9C00, 0x3F00, 0xFA00, 0x0014, 00127 /* J K L M N O P Q R */ 00128 0x5300, 0x9841, 0x1900, 0x5A48, 0x5A09, 0x5F00, 0xFC00, 0x5F01, 0xFC01, 00129 /* S T U V W X Y Z */ 00130 0xAF00, 0x0414, 0x5b00, 0x18C0, 0x5A81, 0x00C9, 0x0058, 0x05C0 00131 }; 00132 00133 /* Constant table for number '0' --> '9' */ 00134 const uint16_t NumberMap[10]= 00135 { 00136 /* 0 1 2 3 4 5 6 7 8 9 */ 00137 0x5F00,0x4200,0xF500,0x6700,0xEa00,0xAF00,0xBF00,0x04600,0xFF00,0xEF00 00138 }; 00139 00140 uint32_t Digit[4]; /* Digit frame buffer */ 00141 00142 /* LCD BAR status: To save the bar setting after writing in LCD RAM memory */ 00143 uint8_t LCDBar = BATTERYLEVEL_FULL; 00144 00145 /** 00146 * @} 00147 */ 00148 00149 /** @defgroup STM32L152C-Discovery_LCD_Private_Functions Private Functions 00150 * @{ 00151 */ 00152 static void Convert(uint8_t* Char, Point_Typedef Point, DoublePoint_Typedef DoublePoint); 00153 static void LCD_MspInit(LCD_HandleTypeDef *hlcd); 00154 static void LCD_MspDeInit(LCD_HandleTypeDef *hlcd); 00155 00156 /** 00157 * @} 00158 */ 00159 00160 /** @defgroup STM32L152C-Discovery_LCD_Exported_Functions Exported Functions 00161 * @{ 00162 */ 00163 00164 /** 00165 * @brief Configures the LCD GLASS relative GPIO port IOs and LCD peripheral. 00166 * @retval None 00167 */ 00168 void BSP_LCD_GLASS_Init(void) 00169 { 00170 LCDHandle.Instance = LCD; 00171 LCDHandle.Init.Prescaler = LCD_PRESCALER_1; 00172 LCDHandle.Init.Divider = LCD_DIVIDER_31; 00173 LCDHandle.Init.Duty = LCD_DUTY_1_4; 00174 LCDHandle.Init.Bias = LCD_BIAS_1_3; 00175 LCDHandle.Init.VoltageSource = LCD_VOLTAGESOURCE_INTERNAL; 00176 LCDHandle.Init.Contrast = LCD_CONTRASTLEVEL_5; 00177 LCDHandle.Init.DeadTime = LCD_DEADTIME_0; 00178 LCDHandle.Init.PulseOnDuration = LCD_PULSEONDURATION_4; 00179 LCDHandle.Init.BlinkMode = LCD_BLINKMODE_OFF; 00180 LCDHandle.Init.BlinkFrequency = LCD_BLINKFREQUENCY_DIV32; 00181 LCDHandle.Init.MuxSegment = LCD_MUXSEGMENT_ENABLE; 00182 /* Initialize the LCD */ 00183 LCD_MspInit(&LCDHandle); 00184 HAL_LCD_Init(&LCDHandle); 00185 00186 BSP_LCD_GLASS_Clear(); 00187 } 00188 00189 /** 00190 * @brief Unconfigures the LCD GLASS relative GPIO port IOs and LCD peripheral. 00191 * @retval None 00192 */ 00193 void BSP_LCD_GLASS_DeInit(void) 00194 { 00195 /* De-Initialize the LCD */ 00196 LCD_MspDeInit(&LCDHandle); 00197 HAL_LCD_DeInit(&LCDHandle); 00198 } 00199 00200 /** 00201 * @brief Configures the LCD Blink mode and Blink frequency. 00202 * @param BlinkMode: specifies the LCD blink mode. 00203 * This parameter can be one of the following values: 00204 * @arg LCD_BLINKMODE_OFF: Blink disabled 00205 * @arg LCD_BLINKMODE_SEG0_COM0: Blink enabled on SEG[0], COM[0] (1 pixel) 00206 * @arg LCD_BLINKMODE_SEG0_ALLCOM: Blink enabled on SEG[0], all COM (up to 8 00207 * pixels according to the programmed duty) 00208 * @arg LCD_BLINKMODE_ALLSEG_ALLCOM: Blink enabled on all SEG and all COM 00209 * (all pixels) 00210 * @param BlinkFrequency: specifies the LCD blink frequency. 00211 * @arg LCD_BLINKFREQUENCY_DIV8: The Blink frequency = fLcd/8 00212 * @arg LCD_BLINKFREQUENCY_DIV16: The Blink frequency = fLcd/16 00213 * @arg LCD_BLINKFREQUENCY_DIV32: The Blink frequency = fLcd/32 00214 * @arg LCD_BLINKFREQUENCY_DIV64: The Blink frequency = fLcd/64 00215 * @arg LCD_BLINKFREQUENCY_DIV128: The Blink frequency = fLcd/128 00216 * @arg LCD_BLINKFREQUENCY_DIV256: The Blink frequency = fLcd/256 00217 * @arg LCD_BLINKFREQUENCY_DIV512: The Blink frequency = fLcd/512 00218 * @arg LCD_BLINKFREQUENCY_DIV1024: The Blink frequency = fLcd/1024 00219 * @retval None 00220 */ 00221 void BSP_LCD_GLASS_BlinkConfig(uint32_t BlinkMode, uint32_t BlinkFrequency) 00222 { 00223 __HAL_LCD_BLINK_CONFIG(&LCDHandle, BlinkMode, BlinkFrequency); 00224 } 00225 00226 /** 00227 * @brief LCD contrast setting 00228 * @param Contrast: specifies the LCD Contrast. 00229 * This parameter can be one of the following values: 00230 * @arg LCD_CONTRASTLEVEL_0: Maximum Voltage = 2.60V 00231 * @arg LCD_CONTRASTLEVEL_1: Maximum Voltage = 2.73V 00232 * @arg LCD_CONTRASTLEVEL_2: Maximum Voltage = 2.86V 00233 * @arg LCD_CONTRASTLEVEL_3: Maximum Voltage = 2.99V 00234 * @arg LCD_CONTRASTLEVEL_4: Maximum Voltage = 3.12V 00235 * @arg LCD_CONTRASTLEVEL_5: Maximum Voltage = 3.25V 00236 * @arg LCD_CONTRASTLEVEL_6: Maximum Voltage = 3.38V 00237 * @arg LCD_CONTRASTLEVEL_7: Maximum Voltage = 3.51V 00238 * @retval None 00239 */ 00240 void BSP_LCD_GLASS_Contrast(uint32_t Contrast) 00241 { 00242 __HAL_LCD_CONTRAST_CONFIG(&LCDHandle, Contrast); 00243 } 00244 00245 /** 00246 * @brief Display one or several bar on LCD frame buffer 00247 * @param BarId: specifies the LCD GLASS Bar to display 00248 * This parameter can be one of the following values: 00249 * @arg BAR0: LCD GLASS Bar 0 00250 * @arg BAR0: LCD GLASS Bar 1 00251 * @arg BAR0: LCD GLASS Bar 2 00252 * @arg BAR0: LCD GLASS Bar 3 00253 * @retval None 00254 */ 00255 void BSP_LCD_GLASS_DisplayBar(uint32_t BarId) 00256 { 00257 uint32_t position = 0; 00258 00259 /* Check which bar is selected */ 00260 while ((BarId) >> position) 00261 { 00262 /* Check if current bar is selected */ 00263 switch(BarId & (1 << position)) 00264 { 00265 /* Bar 0 */ 00266 case LCD_BAR_0: 00267 /* Set BAR0 */ 00268 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG), LCD_BAR0_SEG); 00269 break; 00270 00271 /* Bar 1 */ 00272 case LCD_BAR_1: 00273 /* Set BAR1 */ 00274 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG), LCD_BAR1_SEG); 00275 break; 00276 00277 /* Bar 2 */ 00278 case LCD_BAR_2: 00279 /* Set BAR2 */ 00280 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR2_SEG), LCD_BAR2_SEG); 00281 break; 00282 00283 /* Bar 3 */ 00284 case LCD_BAR_3: 00285 /* Set BAR3 */ 00286 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR3_SEG), LCD_BAR3_SEG); 00287 break; 00288 00289 default: 00290 break; 00291 } 00292 position++; 00293 } 00294 00295 /* Update the LCD display */ 00296 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00297 } 00298 00299 /** 00300 * @brief Clear one or several bar on LCD frame buffer 00301 * @param BarId: specifies the LCD GLASS Bar to display 00302 * This parameter can be combination of one of the following values: 00303 * @arg LCD_BAR_0: LCD GLASS Bar 0 00304 * @arg LCD_BAR_1: LCD GLASS Bar 1 00305 * @arg LCD_BAR_2: LCD GLASS Bar 2 00306 * @arg LCD_BAR_3: LCD GLASS Bar 3 00307 * @retval None 00308 */ 00309 void BSP_LCD_GLASS_ClearBar(uint32_t BarId) 00310 { 00311 uint32_t position = 0; 00312 00313 /* Check which bar is selected */ 00314 while ((BarId) >> position) 00315 { 00316 /* Check if current bar is selected */ 00317 switch(BarId & (1 << position)) 00318 { 00319 /* Bar 0 */ 00320 case LCD_BAR_0: 00321 /* Set BAR0 */ 00322 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG) , 0); 00323 break; 00324 00325 /* Bar 1 */ 00326 case LCD_BAR_1: 00327 /* Set BAR1 */ 00328 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG), 0); 00329 break; 00330 00331 /* Bar 2 */ 00332 case LCD_BAR_2: 00333 /* Set BAR2 */ 00334 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR2_SEG), 0); 00335 break; 00336 00337 /* Bar 3 */ 00338 case LCD_BAR_3: 00339 /* Set BAR3 */ 00340 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR3_SEG), 0); 00341 break; 00342 00343 default: 00344 break; 00345 } 00346 position++; 00347 } 00348 00349 /* Update the LCD display */ 00350 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00351 } 00352 00353 /** 00354 * @brief Setting bar on LCD, writes bar value in LCD frame buffer 00355 * @param BarLevel: specifies the LCD GLASS Batery Level. 00356 * This parameter can be one of the following values: 00357 * @arg BATTERYLEVEL_OFF: LCD GLASS Batery Empty 00358 * @arg BATTERYLEVEL_1_4: LCD GLASS Batery 1/4 Full 00359 * @arg BATTERYLEVEL_1_2: LCD GLASS Batery 1/2 Full 00360 * @arg BATTERYLEVEL_3_4: LCD GLASS Batery 3/4 Full 00361 * @arg BATTERYLEVEL_FULL: LCD GLASS Batery Full 00362 * @retval None 00363 */ 00364 void BSP_LCD_GLASS_BarLevelConfig(uint8_t BarLevel) 00365 { 00366 switch (BarLevel) 00367 { 00368 /* BATTERYLEVEL_OFF */ 00369 case BATTERYLEVEL_OFF: 00370 /* Set BAR0 & BAR2 off */ 00371 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), 0); 00372 /* Set BAR1 & BAR3 off */ 00373 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), 0); 00374 LCDBar = BATTERYLEVEL_OFF; 00375 break; 00376 00377 /* BARLEVEL 1/4 */ 00378 case BATTERYLEVEL_1_4: 00379 /* Set BAR0 on & BAR2 off */ 00380 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), LCD_BAR0_SEG); 00381 /* Set BAR1 & BAR3 off */ 00382 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), 0); 00383 LCDBar = BATTERYLEVEL_1_4; 00384 break; 00385 00386 /* BARLEVEL 1/2 */ 00387 case BATTERYLEVEL_1_2: 00388 /* Set BAR0 on & BAR2 off */ 00389 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), LCD_BAR0_SEG); 00390 /* Set BAR1 on & BAR3 off */ 00391 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), LCD_BAR1_SEG); 00392 LCDBar = BATTERYLEVEL_1_2; 00393 break; 00394 00395 /* Battery Level 3/4 */ 00396 case BATTERYLEVEL_3_4: 00397 /* Set BAR0 & BAR2 on */ 00398 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), (LCD_BAR0_SEG | LCD_BAR2_SEG)); 00399 /* Set BAR1 on & BAR3 off */ 00400 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), LCD_BAR1_SEG); 00401 LCDBar = BATTERYLEVEL_3_4; 00402 break; 00403 00404 /* BATTERYLEVEL_FULL */ 00405 case BATTERYLEVEL_FULL: 00406 /* Set BAR0 & BAR2 on */ 00407 HAL_LCD_Write(&LCDHandle, LCD_BAR0_2_COM, ~(LCD_BAR0_SEG | LCD_BAR2_SEG), (LCD_BAR0_SEG | LCD_BAR2_SEG)); 00408 /* Set BAR1 on & BAR3 on */ 00409 HAL_LCD_Write(&LCDHandle, LCD_BAR1_3_COM, ~(LCD_BAR1_SEG | LCD_BAR3_SEG), (LCD_BAR1_SEG | LCD_BAR3_SEG)); 00410 LCDBar = BATTERYLEVEL_FULL; 00411 break; 00412 00413 default: 00414 break; 00415 } 00416 00417 /* Update the LCD display */ 00418 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00419 } 00420 00421 /** 00422 * @brief This function writes a char in the LCD frame buffer and update BAR LEVEL. 00423 * @param ch: the character to display. 00424 * @param Point: a point to add in front of char 00425 * This parameter can be: POINT_OFF or POINT_ON 00426 * @param Column: flag indicating if a column has to be add in front 00427 * of displayed character. 00428 * This parameter can be: DOUBLEPOINT_OFF or DOUBLEPOINT_ON. 00429 * @param Position: position in the LCD of the character to write (DigitPosition_Typedef) 00430 * @retval None 00431 * @note Required preconditions: The LCD should be cleared before to start the 00432 * write operation. 00433 */ 00434 void BSP_LCD_GLASS_WriteChar(uint8_t* ch, uint8_t Point, uint8_t Column, uint8_t Position) 00435 { 00436 BSP_LCD_GLASS_DisplayChar(ch, (Point_Typedef)Point, (DoublePoint_Typedef)Column, (DigitPosition_Typedef)Position); 00437 00438 /* Refresh LCD bar */ 00439 BSP_LCD_GLASS_BarLevelConfig(LCDBar); 00440 } 00441 00442 /** 00443 * @brief This function writes a char in the LCD RAM and do not update BAR level. 00444 * @param ch: The character to display. 00445 * @param Point: A point to add in front of char. 00446 * This parameter can be one of the following values: 00447 * @arg POINT_OFF: No point to add in front of char. 00448 * @arg POINT_ON: Add a point in front of char. 00449 * @param Column: Flag indicating if a apostrophe has to be add in front 00450 * of displayed character. 00451 * This parameter can be one of the following values: 00452 * @arg DOUBLEPOINT_OFF: No colon to add in back of char. 00453 * @arg DOUBLEPOINT_ON: Add an colon in back of char. 00454 * @param Position: Position in the LCD of the caracter to write. 00455 * This parameter can be any value in DigitPosition_Typedef. 00456 * @retval None 00457 */ 00458 void BSP_LCD_GLASS_DisplayChar(uint8_t* ch, Point_Typedef Point, DoublePoint_Typedef Column, DigitPosition_Typedef Position) 00459 { 00460 uint32_t data =0x00; 00461 /* To convert displayed character in segment in array digit */ 00462 Convert(ch, (Point_Typedef)Point, (DoublePoint_Typedef)Column); 00463 00464 switch (Position) 00465 { 00466 /* Position 1 on LCD (Digit1)*/ 00467 case LCD_DIGIT_POSITION_1: 00468 data = ((Digit[0] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00469 | (((Digit[0] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00470 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM0, LCD_DIGIT1_COM0_SEG_MASK, data); /* 1G 1B 1M 1E */ 00471 00472 data = ((Digit[1] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00473 | (((Digit[1] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00474 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM1, LCD_DIGIT1_COM1_SEG_MASK, data) ; /* 1F 1A 1C 1D */ 00475 00476 data = ((Digit[2] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00477 | (((Digit[2] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00478 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM2, LCD_DIGIT1_COM2_SEG_MASK, data) ; /* 1Q 1K 1Col 1P */ 00479 00480 data = ((Digit[3] & 0x1) << LCD_SEG0_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG1_SHIFT) 00481 | (((Digit[3] & 0x4) >> 2) << LCD_SEG22_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG23_SHIFT); 00482 HAL_LCD_Write(&LCDHandle, LCD_DIGIT1_COM3, LCD_DIGIT1_COM3_SEG_MASK, data) ; /* 1H 1J 1DP 1N */ 00483 break; 00484 00485 /* Position 2 on LCD (Digit2)*/ 00486 case LCD_DIGIT_POSITION_2: 00487 data = ((Digit[0] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00488 | (((Digit[0] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00489 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM0, LCD_DIGIT2_COM0_SEG_MASK, data); /* 2G 2B 2M 2E */ 00490 00491 data = ((Digit[1] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00492 | (((Digit[1] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00493 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM1, LCD_DIGIT2_COM1_SEG_MASK, data) ; /* 2F 2A 2C 2D */ 00494 00495 data = ((Digit[2] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00496 | (((Digit[2] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00497 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM2, LCD_DIGIT2_COM2_SEG_MASK, data) ; /* 2Q 2K 2Col 2P */ 00498 00499 data = ((Digit[3] & 0x1) << LCD_SEG2_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG3_SHIFT) 00500 | (((Digit[3] & 0x4) >> 2) << LCD_SEG20_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG21_SHIFT); 00501 HAL_LCD_Write(&LCDHandle, LCD_DIGIT2_COM3, LCD_DIGIT2_COM3_SEG_MASK, data) ; /* 2H 2J 2DP 2N */ 00502 break; 00503 00504 /* Position 3 on LCD (Digit3)*/ 00505 case LCD_DIGIT_POSITION_3: 00506 data = ((Digit[0] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00507 | (((Digit[0] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00508 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM0, LCD_DIGIT3_COM0_SEG_MASK, data); /* 3G 3B 3M 3E */ 00509 00510 data = ((Digit[1] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00511 | (((Digit[1] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00512 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM1, LCD_DIGIT3_COM1_SEG_MASK, data) ; /* 3F 3A 3C 3D */ 00513 00514 data = ((Digit[2] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00515 | (((Digit[2] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00516 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM2, LCD_DIGIT3_COM2_SEG_MASK, data) ; /* 3Q 3K 3Col 3P */ 00517 00518 data = ((Digit[3] & 0x1) << LCD_SEG4_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG5_SHIFT) 00519 | (((Digit[3] & 0x4) >> 2) << LCD_SEG18_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG19_SHIFT); 00520 HAL_LCD_Write(&LCDHandle, LCD_DIGIT3_COM3, LCD_DIGIT3_COM3_SEG_MASK, data) ; /* 3H 3J 3DP 3N */ 00521 break; 00522 00523 /* Position 4 on LCD (Digit4)*/ 00524 case LCD_DIGIT_POSITION_4: 00525 data = ((Digit[0] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00526 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM0, LCD_DIGIT4_COM0_SEG_MASK, data); /* 4G 4B 4M 4E */ 00527 00528 data = (((Digit[0] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[0] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00529 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM0_1, LCD_DIGIT4_COM0_1_SEG_MASK, data); /* 4G 4B 4M 4E */ 00530 00531 data = ((Digit[1] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00532 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM1, LCD_DIGIT4_COM1_SEG_MASK, data) ; /* 4F 4A 4C 4D */ 00533 00534 data = (((Digit[1] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[1] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00535 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM1_1, LCD_DIGIT4_COM1_1_SEG_MASK, data) ; /* 4F 4A 4C 4D */ 00536 00537 data = ((Digit[2] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00538 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM2, LCD_DIGIT4_COM2_SEG_MASK, data) ; /* 4Q 4K 4Col 4P */ 00539 00540 data = (((Digit[2] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[2] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00541 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM2_1, LCD_DIGIT4_COM2_1_SEG_MASK, data) ; /* 4Q 4K 4Col 4P */ 00542 00543 data = ((Digit[3] & 0x1) << LCD_SEG6_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG17_SHIFT); 00544 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM3, LCD_DIGIT4_COM3_SEG_MASK, data) ; /* 4H 4J 4DP 4N */ 00545 00546 data = (((Digit[3] & 0x2) >> 1) << LCD_SEG7_SHIFT) | (((Digit[3] & 0x4) >> 2) << LCD_SEG16_SHIFT); 00547 HAL_LCD_Write(&LCDHandle, LCD_DIGIT4_COM3_1, LCD_DIGIT4_COM3_1_SEG_MASK, data) ; /* 4H 4J 4DP 4N */ 00548 break; 00549 00550 /* Position 5 on LCD (Digit5)*/ 00551 case LCD_DIGIT_POSITION_5: 00552 data = (((Digit[0] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[0] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00553 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM0, LCD_DIGIT5_COM0_SEG_MASK, data); /* 5G 5B 5M 5E */ 00554 00555 data = ((Digit[0] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00556 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM0_1, LCD_DIGIT5_COM0_1_SEG_MASK, data); /* 5G 5B 5M 5E */ 00557 00558 data = (((Digit[1] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[1] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00559 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM1, LCD_DIGIT5_COM1_SEG_MASK, data) ; /* 5F 5A 5C 5D */ 00560 00561 data = ((Digit[1] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00562 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM1_1, LCD_DIGIT5_COM1_1_SEG_MASK, data) ; /* 5F 5A 5C 5D */ 00563 00564 data = (((Digit[2] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[2] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00565 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM2, LCD_DIGIT5_COM2_SEG_MASK, data) ; /* 5Q 5K 5P */ 00566 00567 data = ((Digit[2] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00568 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM2_1, LCD_DIGIT5_COM2_1_SEG_MASK, data) ; /* 5Q 5K 5P */ 00569 00570 data = (((Digit[3] & 0x2) >> 1) << LCD_SEG9_SHIFT) | (((Digit[3] & 0x4) >> 2) << LCD_SEG14_SHIFT); 00571 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM3, LCD_DIGIT5_COM3_SEG_MASK, data) ; /* 5H 5J 5N */ 00572 00573 data = ((Digit[3] & 0x1) << LCD_SEG8_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG15_SHIFT); 00574 HAL_LCD_Write(&LCDHandle, LCD_DIGIT5_COM3_1, LCD_DIGIT5_COM3_1_SEG_MASK, data) ; /* 5H 5J 5N */ 00575 break; 00576 00577 /* Position 6 on LCD (Digit6)*/ 00578 case LCD_DIGIT_POSITION_6: 00579 data = ((Digit[0] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[0] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00580 | (((Digit[0] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[0] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00581 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM0, LCD_DIGIT6_COM0_SEG_MASK, data); /* 6G 6B 6M 6E */ 00582 00583 data = ((Digit[1] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[1] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00584 | (((Digit[1] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[1] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00585 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM1, LCD_DIGIT6_COM1_SEG_MASK, data) ; /* 6G 6B 6M 6E */ 00586 00587 data = ((Digit[2] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[2] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00588 | (((Digit[2] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[2] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00589 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM2, LCD_DIGIT6_COM2_SEG_MASK, data) ; /* 6Q 6K 6P */ 00590 00591 data = ((Digit[3] & 0x1) << LCD_SEG10_SHIFT) | (((Digit[3] & 0x2) >> 1) << LCD_SEG11_SHIFT) 00592 | (((Digit[3] & 0x4) >> 2) << LCD_SEG12_SHIFT) | (((Digit[3] & 0x8) >> 3) << LCD_SEG13_SHIFT); 00593 HAL_LCD_Write(&LCDHandle, LCD_DIGIT6_COM3, LCD_DIGIT6_COM3_SEG_MASK, data) ; /* 6Q 6K 6P */ 00594 break; 00595 00596 default: 00597 break; 00598 } 00599 00600 /* Update the LCD display */ 00601 HAL_LCD_UpdateDisplayRequest(&LCDHandle); 00602 } 00603 00604 /** 00605 * @brief This function writes a char in the LCD RAM. 00606 * @param ptr: Pointer to string to display on the LCD Glass. 00607 * @retval None 00608 */ 00609 void BSP_LCD_GLASS_DisplayString(uint8_t* ptr) 00610 { 00611 DigitPosition_Typedef position = LCD_DIGIT_POSITION_1; 00612 00613 /* Send the string character by character on lCD */ 00614 while ((*ptr != 0) & (position <= LCD_DIGIT_POSITION_6)) 00615 { 00616 /* Display one character on LCD */ 00617 BSP_LCD_GLASS_WriteChar(ptr, POINT_OFF, DOUBLEPOINT_OFF, position); 00618 00619 /* Point on the next character */ 00620 ptr++; 00621 00622 /* Increment the character counter */ 00623 position++; 00624 } 00625 } 00626 00627 /** 00628 * @brief This function writes a char in the LCD RAM. 00629 * @param ptr: Pointer to string to display on the LCD Glass. 00630 * @retval None 00631 * @note Required preconditions: Char is ASCCI value "Ored" with decimal point or Column flag 00632 */ 00633 void BSP_LCD_GLASS_DisplayStrDeci(uint16_t* ptr) 00634 { 00635 DigitPosition_Typedef index = LCD_DIGIT_POSITION_1; 00636 uint8_t tmpchar = 0; 00637 00638 /* Send the string character by character on lCD */ 00639 while((*ptr != 0) & (index <= LCD_DIGIT_POSITION_6)) 00640 { 00641 tmpchar = (*ptr) & 0x00FF; 00642 00643 switch((*ptr) & 0xF000) 00644 { 00645 case DOT: 00646 /* Display one character on LCD with decimal point */ 00647 BSP_LCD_GLASS_WriteChar(&tmpchar, POINT_ON, DOUBLEPOINT_OFF, index); 00648 break; 00649 case DOUBLE_DOT: 00650 /* Display one character on LCD with decimal point */ 00651 BSP_LCD_GLASS_WriteChar(&tmpchar, POINT_OFF, DOUBLEPOINT_ON, index); 00652 break; 00653 default: 00654 BSP_LCD_GLASS_WriteChar(&tmpchar, POINT_OFF, DOUBLEPOINT_OFF, index); 00655 break; 00656 }/* Point on the next character */ 00657 ptr++; 00658 00659 /* Increment the character counter */ 00660 index++; 00661 } 00662 } 00663 00664 /** 00665 * @brief This function Clear the whole LCD RAM. 00666 * @retval None 00667 */ 00668 void BSP_LCD_GLASS_Clear(void) 00669 { 00670 HAL_LCD_Clear(&LCDHandle); 00671 } 00672 00673 /** 00674 * @brief Display a string in scrolling mode 00675 * @param ptr: Pointer to string to display on the LCD Glass. 00676 * @param nScroll: Specifies how many time the message will be scrolled 00677 * @param ScrollSpeed : Specifies the speed of the scroll, low value gives 00678 * higher speed 00679 * @retval None 00680 * @note Required preconditions: The LCD should be cleared before to start the 00681 * write operation. 00682 */ 00683 void BSP_LCD_GLASS_ScrollSentence(uint8_t* ptr, uint16_t nScroll, uint16_t ScrollSpeed) 00684 { 00685 uint8_t repetition = 0, nbrchar = 0, sizestr = 0; 00686 uint8_t* ptr1; 00687 uint8_t str[6] = ""; 00688 00689 if(ptr == 0) 00690 { 00691 return; 00692 } 00693 00694 /* To calculate end of string */ 00695 for(ptr1 = ptr, sizestr = 0; *ptr1 != 0; sizestr++, ptr1++); 00696 00697 ptr1 = ptr; 00698 00699 BSP_LCD_GLASS_DisplayString(str); 00700 HAL_Delay(ScrollSpeed); 00701 00702 /* To shift the string for scrolling display*/ 00703 for (repetition = 0; repetition < nScroll; repetition++) 00704 { 00705 for(nbrchar = 0; nbrchar < sizestr; nbrchar++) 00706 { 00707 *(str) =* (ptr1+((nbrchar+1)%sizestr)); 00708 *(str+1) =* (ptr1+((nbrchar+2)%sizestr)); 00709 *(str+2) =* (ptr1+((nbrchar+3)%sizestr)); 00710 *(str+3) =* (ptr1+((nbrchar+4)%sizestr)); 00711 *(str+4) =* (ptr1+((nbrchar+5)%sizestr)); 00712 *(str+5) =* (ptr1+((nbrchar+6)%sizestr)); 00713 BSP_LCD_GLASS_Clear(); 00714 BSP_LCD_GLASS_DisplayString(str); 00715 00716 /* user button pressed stop the scrolling sentence */ 00717 if(bLCDGlass_KeyPressed) 00718 { 00719 bLCDGlass_KeyPressed = 0; 00720 return; 00721 } 00722 HAL_Delay(ScrollSpeed); 00723 } 00724 } 00725 } 00726 00727 /** 00728 * @} 00729 */ 00730 00731 /** @addtogroup STM32L152C-Discovery_LCD_Private_Functions 00732 * @{ 00733 */ 00734 00735 /** 00736 * @brief LCD MSP Init. 00737 * @param hlcd: LCD handle 00738 * @retval None 00739 */ 00740 static void LCD_MspInit(LCD_HandleTypeDef *hlcd) 00741 { 00742 GPIO_InitTypeDef gpioinitstruct = {0}; 00743 RCC_OscInitTypeDef oscinitstruct = {0}; 00744 RCC_PeriphCLKInitTypeDef periphclkstruct = {0}; 00745 00746 /*##-1- Enable PWR peripheral Clock #######################################*/ 00747 __HAL_RCC_PWR_CLK_ENABLE(); 00748 00749 /*##-2- Configue LSE as RTC clock soucre ###################################*/ 00750 oscinitstruct.OscillatorType = RCC_OSCILLATORTYPE_LSE; 00751 oscinitstruct.PLL.PLLState = RCC_PLL_NONE; 00752 oscinitstruct.LSEState = RCC_LSE_ON; 00753 if(HAL_RCC_OscConfig(&oscinitstruct) != HAL_OK) 00754 { 00755 while(1); 00756 } 00757 00758 /*##-3- select LSE as RTC clock source.##########################*/ 00759 /* Backup domain management is done in RCC function */ 00760 periphclkstruct.PeriphClockSelection = RCC_PERIPHCLK_RTC; 00761 periphclkstruct.RTCClockSelection = RCC_RTCCLKSOURCE_LSE; 00762 HAL_RCCEx_PeriphCLKConfig(&periphclkstruct); 00763 00764 /*##-4- Enable LCD GPIO Clocks #############################################*/ 00765 __HAL_RCC_GPIOA_CLK_ENABLE(); 00766 __HAL_RCC_GPIOB_CLK_ENABLE(); 00767 __HAL_RCC_GPIOC_CLK_ENABLE(); 00768 00769 /*##-5- Configure peripheral GPIO ##########################################*/ 00770 /* Configure Output for LCD */ 00771 /* Port A */ 00772 gpioinitstruct.Pin = LCD_GPIO_BANKA_PINS; 00773 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00774 gpioinitstruct.Pull = GPIO_NOPULL; 00775 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00776 gpioinitstruct.Alternate = GPIO_AF11_LCD; 00777 HAL_GPIO_Init(GPIOA, &gpioinitstruct); 00778 00779 /* Port B */ 00780 gpioinitstruct.Pin = LCD_GPIO_BANKB_PINS; 00781 HAL_GPIO_Init(GPIOB, &gpioinitstruct); 00782 00783 /* Port C*/ 00784 gpioinitstruct.Pin = LCD_GPIO_BANKC_PINS; 00785 HAL_GPIO_Init(GPIOC, &gpioinitstruct); 00786 00787 /* Wait for the external capacitor Cext which is connected to the VLCD pin is charged 00788 (approximately 2ms for Cext=1uF) */ 00789 HAL_Delay(2); 00790 00791 /*##-6- Enable LCD peripheral Clock ########################################*/ 00792 __HAL_RCC_LCD_CLK_ENABLE(); 00793 } 00794 00795 /** 00796 * @brief LCD MSP DeInit. 00797 * @param hlcd: LCD handle 00798 * @retval None 00799 */ 00800 static void LCD_MspDeInit(LCD_HandleTypeDef *hlcd) 00801 { 00802 uint32_t gpiopin = 0; 00803 00804 /*##-1- Enable LCD GPIO Clocks #############################################*/ 00805 __HAL_RCC_GPIOA_CLK_ENABLE(); 00806 __HAL_RCC_GPIOB_CLK_ENABLE(); 00807 __HAL_RCC_GPIOC_CLK_ENABLE(); 00808 00809 /*##-1- Configure peripheral GPIO ##########################################*/ 00810 /* Configure Output for LCD */ 00811 /* Port A */ 00812 gpiopin = LCD_GPIO_BANKA_PINS; 00813 HAL_GPIO_DeInit(GPIOA, gpiopin); 00814 00815 /* Port B */ 00816 gpiopin = LCD_GPIO_BANKB_PINS; 00817 HAL_GPIO_DeInit(GPIOB, gpiopin); 00818 00819 /* Port C*/ 00820 gpiopin = LCD_GPIO_BANKC_PINS; 00821 HAL_GPIO_DeInit(GPIOC, gpiopin); 00822 00823 /*##-5- Enable LCD peripheral Clock ########################################*/ 00824 __HAL_RCC_LCD_CLK_DISABLE(); 00825 } 00826 00827 /** 00828 * @brief Converts an ascii char to the a LCD digit. 00829 * @param Char: a char to display. 00830 * @param Point: a point to add in front of char 00831 * This parameter can be: POINT_OFF or POINT_ON 00832 * @param DoublePoint : flag indicating if a column has to be add in front 00833 * of displayed character. 00834 * This parameter can be: DOUBLEPOINT_OFF or DOUBLEPOINT_ON. 00835 * @retval None 00836 */ 00837 static void Convert(uint8_t* Char, Point_Typedef Point, DoublePoint_Typedef DoublePoint) 00838 { 00839 uint16_t ch = 0 ; 00840 uint8_t loop = 0, index = 0; 00841 00842 switch (*Char) 00843 { 00844 case ' ' : 00845 ch = 0x00; 00846 break; 00847 00848 case '*': 00849 ch = C_STAR; 00850 break; 00851 00852 case '(' : 00853 ch = C_OPENPARMAP; 00854 break; 00855 00856 case ')' : 00857 ch = C_CLOSEPARMAP; 00858 break; 00859 00860 case 'm' : 00861 ch = C_MMAP; 00862 break; 00863 00864 case 'n' : 00865 ch = C_NMAP; 00866 break; 00867 00868 case '�' : 00869 ch = C_UMAP; 00870 break; 00871 00872 case '-' : 00873 ch = C_MINUS; 00874 break; 00875 00876 case '/' : 00877 ch = C_SLATCH; 00878 break; 00879 00880 case '�' : 00881 ch = C_PERCENT_1; 00882 break; 00883 case '%' : 00884 ch = C_PERCENT_2; 00885 break; 00886 case 255 : 00887 ch = C_FULL; 00888 break ; 00889 00890 case '0': 00891 case '1': 00892 case '2': 00893 case '3': 00894 case '4': 00895 case '5': 00896 case '6': 00897 case '7': 00898 case '8': 00899 case '9': 00900 ch = NumberMap[*Char - ASCII_CHAR_0]; 00901 break; 00902 00903 default: 00904 /* The character Char is one letter in upper case*/ 00905 if ( (*Char < ASCII_CHAR_LEFT_OPEN_BRACKET) && (*Char > ASCII_CHAR_AT_SYMBOL) ) 00906 { 00907 ch = CapLetterMap[*Char - 'A']; 00908 } 00909 /* The character Char is one letter in lower case*/ 00910 if ( (*Char < ASCII_CHAR_LEFT_OPEN_BRACE) && ( *Char > ASCII_CHAR_APOSTROPHE) ) 00911 { 00912 ch = CapLetterMap[*Char - 'a']; 00913 } 00914 break; 00915 } 00916 00917 /* Set the digital point can be displayed if the point is on */ 00918 if (Point == POINT_ON) 00919 { 00920 ch |= 0x0002; 00921 } 00922 00923 /* Set the "COL" segment in the character that can be displayed if the column is on */ 00924 if (DoublePoint == DOUBLEPOINT_ON) 00925 { 00926 ch |= 0x0020; 00927 } 00928 00929 for (loop = 12,index=0 ;index < 4; loop -= 4,index++) 00930 { 00931 Digit[index] = (ch >> loop) & 0x0f; /*To isolate the less signifiant dibit */ 00932 } 00933 } 00934 00935 /** 00936 * @} 00937 */ 00938 00939 /** 00940 * @} 00941 */ 00942 00943 /** 00944 * @} 00945 */ 00946 00947 /** 00948 * @} 00949 */ 00950 00951 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00952
Generated on Thu Aug 24 2017 17:55:24 for STM32L152C-Discovery BSP User Manual by
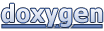