STM32L152C-Discovery BSP User Manual
|
stm32l152c_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152c_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides 00006 * - set of firmware functions to manage Led and push-button 00007 * available on STM32L152C-Discovery board from STMicroelectronics. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l152c_discovery.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @defgroup STM32L152C_DISCOVERY STM32L152C-Discovery 00045 * @brief This file provides firmware functions to manage Leds and push-buttons 00046 * available on STM32L152C discovery board from STMicroelectronics. 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32L152C_Discovery_Common Common 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L152C_DISCOVERY_Private_Defines Private Defines 00055 * @{ 00056 */ 00057 00058 /** 00059 * @brief STM32L152C-Discovery BSP Driver version number 00060 */ 00061 #define __STM32L152C_DISCO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00062 #define __STM32L152C_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00063 #define __STM32L152C_DISCO_BSP_VERSION_SUB2 (0x04) /*!< [15:8] sub2 version */ 00064 #define __STM32L152C_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00065 #define __STM32L152C_DISCO_BSP_VERSION ((__STM32L152C_DISCO_BSP_VERSION_MAIN << 24)\ 00066 |(__STM32L152C_DISCO_BSP_VERSION_SUB1 << 16)\ 00067 |(__STM32L152C_DISCO_BSP_VERSION_SUB2 << 8 )\ 00068 |(__STM32L152C_DISCO_BSP_VERSION_RC)) 00069 /** 00070 * @} 00071 */ 00072 00073 00074 /** @defgroup STM32L152C_DISCOVERY_Private_Variables Private Variables 00075 * @{ 00076 */ 00077 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED3_GPIO_PORT, 00078 LED4_GPIO_PORT}; 00079 00080 const uint16_t GPIO_PIN[LEDn] = {LED3_PIN, 00081 LED4_PIN}; 00082 00083 00084 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00085 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00086 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00087 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM32L152C_DISCOVERY_Exported_Functions Exported Functions 00093 * @{ 00094 */ 00095 00096 /** 00097 * @brief This method returns the STM32L152C-Discovery BSP Driver revision 00098 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00099 */ 00100 uint32_t BSP_GetVersion(void) 00101 { 00102 return __STM32L152C_DISCO_BSP_VERSION; 00103 } 00104 00105 /** @defgroup STM32152C_DISCOVERY_LED_Functions LED Functions 00106 * @{ 00107 */ 00108 00109 /** 00110 * @brief Configures LED GPIO. 00111 * @param Led: Specifies the Led to be configured. 00112 * This parameter can be one of following parameters: 00113 * @arg LED3 00114 * @arg LED4 00115 * @retval None 00116 */ 00117 void BSP_LED_Init(Led_TypeDef Led) 00118 { 00119 GPIO_InitTypeDef gpioinitstruct = {0}; 00120 00121 /* Enable the GPIO_LED Clock */ 00122 LEDx_GPIO_CLK_ENABLE(Led); 00123 00124 /* Configure the GPIO_LED pin */ 00125 gpioinitstruct.Pin = GPIO_PIN[Led]; 00126 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00127 gpioinitstruct.Pull = GPIO_NOPULL; 00128 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00129 HAL_GPIO_Init(GPIO_PORT[Led], &gpioinitstruct); 00130 00131 /* Reset PIN to switch off the LED */ 00132 HAL_GPIO_WritePin(GPIO_PORT[Led],GPIO_PIN[Led], GPIO_PIN_RESET); 00133 } 00134 00135 /** 00136 * @brief Turns selected LED On. 00137 * @param Led: Specifies the Led to be set on. 00138 * This parameter can be one of following parameters: 00139 * @arg LED3 00140 * @arg LED4 00141 * @retval None 00142 */ 00143 void BSP_LED_On(Led_TypeDef Led) 00144 { 00145 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00146 } 00147 00148 /** 00149 * @brief Turns selected LED Off. 00150 * @param Led: Specifies the Led to be set off. 00151 * This parameter can be one of following parameters: 00152 * @arg LED3 00153 * @arg LED4 00154 * @retval None 00155 */ 00156 void BSP_LED_Off(Led_TypeDef Led) 00157 { 00158 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00159 } 00160 00161 /** 00162 * @brief Toggles the selected LED. 00163 * @param Led: Specifies the Led to be toggled. 00164 * This parameter can be one of following parameters: 00165 * @arg LED3 00166 * @arg LED4 00167 * @retval None 00168 */ 00169 void BSP_LED_Toggle(Led_TypeDef Led) 00170 { 00171 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00172 } 00173 00174 /** 00175 * @} 00176 */ 00177 00178 /** @defgroup STM32152C_DISCOVERY_BUTTON_Functions BUTTON Functions 00179 * @{ 00180 */ 00181 00182 /** 00183 * @brief Configures Button GPIO and EXTI Line. 00184 * @param Button: Specifies the Button to be configured. 00185 * This parameter should be: BUTTON_USER 00186 * @param Mode: Specifies Button mode. 00187 * This parameter can be one of following parameters: 00188 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00189 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00190 * generation capability 00191 * @retval None 00192 */ 00193 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Mode) 00194 { 00195 GPIO_InitTypeDef gpioinitstruct = {0}; 00196 00197 /* Enable the BUTTON Clock */ 00198 BUTTONx_GPIO_CLK_ENABLE(Button); 00199 00200 if (Mode == BUTTON_MODE_GPIO) 00201 { 00202 /* Configure Button pin as input */ 00203 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00204 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00205 gpioinitstruct.Pull = GPIO_NOPULL; 00206 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00207 00208 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00209 } 00210 00211 if (Mode == BUTTON_MODE_EXTI) 00212 { 00213 /* Configure Button pin as input with External interrupt */ 00214 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00215 gpioinitstruct.Pull = GPIO_NOPULL; 00216 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00217 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00218 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00219 00220 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00221 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00222 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00223 } 00224 } 00225 00226 /** 00227 * @brief Returns the selected Button state. 00228 * @param Button: Specifies the Button to be checked. 00229 * This parameter should be: BUTTON_USER 00230 * @retval Button state. 00231 */ 00232 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00233 { 00234 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00235 } 00236 00237 /** 00238 * @} 00239 */ 00240 00241 /** 00242 * @} 00243 */ 00244 00245 /** 00246 * @} 00247 */ 00248 00249 /** 00250 * @} 00251 */ 00252 00253 /** 00254 * @} 00255 */ 00256 00257 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:55:24 for STM32L152C-Discovery BSP User Manual by
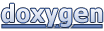