STM32L0xx_Nucleo BSP User Manual
|
stm32l0xx_nucleo.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l0xx_nucleo.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage: 00006 * - LEDs and push-button available on STM32L0XX-Nucleo Kit 00007 * from STMicroelectronics 00008 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00009 * shield (reference ID 802) 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32l0xx_nucleo.h" 00042 00043 /** @addtogroup BSP 00044 * @{ 00045 */ 00046 00047 /** @addtogroup STM32L0XX_NUCLEO 00048 * @{ 00049 */ 00050 00051 /** @addtogroup STM32L0XX_NUCLEO_LOW_LEVEL 00052 * @brief This file provides set of firmware functions to manage Leds and push-button 00053 * available on STM32L0XX-Nucleo Kit from STMicroelectronics. 00054 * @{ 00055 */ 00056 00057 /** @defgroup STM32L0XX_NUCLEO_LOW_LEVEL_Private_TypesDefinitions Private Types Definitions 00058 * @{ 00059 */ 00060 /** 00061 * @} 00062 */ 00063 00064 00065 /** @defgroup STM32L0XX_NUCLEO_LOW_LEVEL_Private_Defines Private Defines 00066 * @{ 00067 */ 00068 00069 /** 00070 * @brief STM32L0XX NUCLEO BSP Driver version number 00071 */ 00072 #define __STM32L0XX_NUCLEO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00073 #define __STM32L0XX_NUCLEO_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00074 #define __STM32L0XX_NUCLEO_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00075 #define __STM32L0XX_NUCLEO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00076 #define __STM32L0XX_NUCLEO_BSP_VERSION ((__STM32L0XX_NUCLEO_BSP_VERSION_MAIN << 24)\ 00077 |(__STM32L0XX_NUCLEO_BSP_VERSION_SUB1 << 16)\ 00078 |(__STM32L0XX_NUCLEO_BSP_VERSION_SUB2 << 8 )\ 00079 |(__STM32L0XX_NUCLEO_BSP_VERSION_RC)) 00080 00081 /** 00082 * @brief LINK SD Card 00083 */ 00084 #define SD_DUMMY_BYTE 0xFF 00085 #define SD_NO_RESPONSE_EXPECTED 0x80 00086 00087 /** 00088 * @} 00089 */ 00090 00091 00092 /** @defgroup STM32L0XX_NUCLEO_LOW_LEVEL_Private_Variables Private Variables 00093 * @{ 00094 */ 00095 GPIO_TypeDef* LED_PORT[LEDn] = {LED2_GPIO_PORT}; 00096 00097 const uint16_t LED_PIN[LEDn] = {LED2_PIN}; 00098 00099 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT }; 00100 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN }; 00101 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn }; 00102 00103 /** 00104 * @brief BUS variables 00105 */ 00106 00107 #ifdef HAL_SPI_MODULE_ENABLED 00108 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00109 static SPI_HandleTypeDef hnucleo_Spi; 00110 #endif /* HAL_SPI_MODULE_ENABLED */ 00111 00112 #ifdef HAL_ADC_MODULE_ENABLED 00113 static ADC_HandleTypeDef hnucleo_Adc; 00114 /* ADC channel configuration structure declaration */ 00115 static ADC_ChannelConfTypeDef sConfig; 00116 #endif /* HAL_ADC_MODULE_ENABLED */ 00117 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32L0XX_NUCLEO_LOW_LEVEL_Private_FunctionPrototypes Private Function Prototypes 00123 * @{ 00124 */ 00125 #ifdef HAL_SPI_MODULE_ENABLED 00126 static void SPIx_Init(void); 00127 static void SPIx_Write(uint8_t Value); 00128 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength); 00129 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth); 00130 static void SPIx_Error (void); 00131 static void SPIx_MspInit(void); 00132 00133 /* SD IO functions */ 00134 void SD_IO_Init(void); 00135 void SD_IO_CSState(uint8_t state); 00136 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00137 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength); 00138 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength); 00139 uint8_t SD_IO_WriteByte(uint8_t Data); 00140 uint8_t SD_IO_ReadByte(void); 00141 00142 /* LCD IO functions */ 00143 void LCD_IO_Init(void); 00144 void LCD_IO_WriteData(uint8_t Data); 00145 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00146 void LCD_IO_WriteReg(uint8_t LCDReg); 00147 void LCD_Delay(uint32_t delay); 00148 #endif /* HAL_SPI_MODULE_ENABLED */ 00149 00150 #ifdef HAL_ADC_MODULE_ENABLED 00151 static HAL_StatusTypeDef ADCx_Init(void); 00152 static void ADCx_DeInit(void); 00153 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00154 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc); 00155 #endif /* HAL_ADC_MODULE_ENABLED */ 00156 /** 00157 * @} 00158 */ 00159 00160 /** @defgroup STM32L0XX_NUCLEO_LOW_LEVEL_Private_Functions Private Functions 00161 * @{ 00162 */ 00163 00164 /** 00165 * @brief This method returns the STM32L0XX NUCLEO BSP Driver revision 00166 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00167 */ 00168 uint32_t BSP_GetVersion(void) 00169 { 00170 return __STM32L0XX_NUCLEO_BSP_VERSION; 00171 } 00172 00173 /** 00174 * @brief Configures LED GPIO. 00175 * @param Led: Led to be configured. 00176 * This parameter can be one of the following values: 00177 * @arg LED2 00178 * @retval None 00179 */ 00180 void BSP_LED_Init(Led_TypeDef Led) 00181 { 00182 GPIO_InitTypeDef gpioinitstruct; 00183 00184 /* Enable the GPIO_LED Clock */ 00185 LEDx_GPIO_CLK_ENABLE(Led); 00186 00187 /* Configure the GPIO_LED pin */ 00188 gpioinitstruct.Pin = LED_PIN[Led]; 00189 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00190 gpioinitstruct.Pull = GPIO_NOPULL; 00191 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00192 00193 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00194 00195 /* Reset PIN to switch off the LED */ 00196 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00197 } 00198 00199 /** 00200 * @brief DeInit LEDs. 00201 * @param Led: LED to be de-init. 00202 * This parameter can be one of the following values: 00203 * @arg LED2 00204 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00205 * @retval None 00206 */ 00207 void BSP_LED_DeInit(Led_TypeDef Led) 00208 { 00209 GPIO_InitTypeDef gpio_init_structure; 00210 00211 /* Turn off LED */ 00212 HAL_GPIO_WritePin(LED_PORT[Led],LED_PIN[Led], GPIO_PIN_RESET); 00213 /* DeInit the GPIO_LED pin */ 00214 gpio_init_structure.Pin = LED_PIN[Led]; 00215 HAL_GPIO_DeInit(LED_PORT[Led], gpio_init_structure.Pin); 00216 } 00217 00218 /** 00219 * @brief Turns selected LED On. 00220 * @param Led: Specifies the Led to be set on. 00221 * This parameter can be one of following parameters: 00222 * @arg LED2 00223 * @retval None 00224 */ 00225 void BSP_LED_On(Led_TypeDef Led) 00226 { 00227 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00228 } 00229 00230 /** 00231 * @brief Turns selected LED Off. 00232 * @param Led: Specifies the Led to be set off. 00233 * This parameter can be one of following parameters: 00234 * @arg LED2 00235 * @retval None 00236 */ 00237 void BSP_LED_Off(Led_TypeDef Led) 00238 { 00239 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00240 } 00241 00242 /** 00243 * @brief Toggles the selected LED. 00244 * @param Led: Specifies the Led to be toggled. 00245 * This parameter can be one of following parameters: 00246 * @arg LED2 00247 * @retval None 00248 */ 00249 void BSP_LED_Toggle(Led_TypeDef Led) 00250 { 00251 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00252 } 00253 00254 /** 00255 * @brief Configures Button GPIO and EXTI Line. 00256 * @param Button: Specifies the Button to be configured. 00257 * This parameter should be: BUTTON_USER 00258 * @param ButtonMode: Specifies Button mode. 00259 * This parameter can be one of following parameters: 00260 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00261 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00262 * generation capability 00263 * @retval None 00264 */ 00265 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00266 { 00267 GPIO_InitTypeDef gpioinitstruct; 00268 00269 /* Enable the BUTTON Clock */ 00270 BUTTONx_GPIO_CLK_ENABLE(Button); 00271 00272 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00273 gpioinitstruct.Pull = GPIO_NOPULL; 00274 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00275 00276 if(ButtonMode == BUTTON_MODE_GPIO) 00277 { 00278 /* Configure Button pin as input */ 00279 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00280 00281 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00282 } 00283 00284 if(ButtonMode == BUTTON_MODE_EXTI) 00285 { 00286 /* Configure Button pin as input with External interrupt */ 00287 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00288 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00289 00290 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00291 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00292 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00293 } 00294 } 00295 00296 /** 00297 * @brief Push Button DeInit. 00298 * @param Button: Button to be configured 00299 * This parameter should be: BUTTON_USER 00300 * @note PB DeInit does not disable the GPIO clock 00301 * @retval None 00302 */ 00303 void BSP_PB_DeInit(Button_TypeDef Button) 00304 { 00305 GPIO_InitTypeDef gpio_init_structure; 00306 00307 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00308 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00309 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00310 } 00311 00312 /** 00313 * @brief Returns the selected Button state. 00314 * @param Button: Specifies the Button to be checked. 00315 * This parameter should be: BUTTON_USER 00316 * @retval Button state. 00317 */ 00318 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00319 { 00320 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00321 } 00322 00323 00324 #ifdef HAL_SPI_MODULE_ENABLED 00325 /****************************************************************************** 00326 BUS OPERATIONS 00327 *******************************************************************************/ 00328 /** 00329 * @brief Initialize SPI MSP. 00330 * @retval None 00331 */ 00332 static void SPIx_MspInit(void) 00333 { 00334 GPIO_InitTypeDef gpioinitstruct = {0}; 00335 00336 /*** Configure the GPIOs ***/ 00337 /* Enable GPIO clock */ 00338 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00339 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00340 00341 /* Configure SPI SCK */ 00342 gpioinitstruct.Pin = NUCLEO_SPIx_SCK_PIN; 00343 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00344 gpioinitstruct.Pull = GPIO_PULLUP; 00345 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00346 gpioinitstruct.Alternate = NUCLEO_SPIx_SCK_AF; 00347 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00348 00349 /* Configure SPI MISO and MOSI */ 00350 gpioinitstruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00351 gpioinitstruct.Alternate = NUCLEO_SPIx_MISO_MOSI_AF; 00352 gpioinitstruct.Pull = GPIO_PULLDOWN; 00353 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00354 00355 gpioinitstruct.Pin = NUCLEO_SPIx_MISO_PIN; 00356 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00357 00358 /*** Configure the SPI peripheral ***/ 00359 /* Enable SPI clock */ 00360 NUCLEO_SPIx_CLK_ENABLE(); 00361 } 00362 00363 /** 00364 * @brief Initialize SPI HAL. 00365 * @retval None 00366 */ 00367 static void SPIx_Init(void) 00368 { 00369 if(HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00370 { 00371 /* SPI Config */ 00372 hnucleo_Spi.Instance = NUCLEO_SPIx; 00373 /* SPI baudrate is set to 8 MHz maximum (PCLK2/SPI_BaudRatePrescaler = 32/4 = 8 MHz) 00374 to verify these constraints: 00375 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00376 Since the provided driver doesn't use read capability from LCD, only constraint 00377 on write baudrate is considered. 00378 - SD card SPI interface max baudrate is 25MHz for write/read 00379 - PCLK2 max frequency is 32 MHz 00380 */ 00381 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_4; 00382 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00383 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_1EDGE; 00384 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_LOW; 00385 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00386 hnucleo_Spi.Init.CRCPolynomial = 7; 00387 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00388 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00389 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00390 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00391 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00392 00393 SPIx_MspInit(); 00394 HAL_SPI_Init(&hnucleo_Spi); 00395 } 00396 } 00397 00398 /** 00399 * @brief SPI Write a byte to device 00400 * @param DataIn: value to be written 00401 * @param DataOut: read value 00402 * @param DataLength: number of bytes to write 00403 * @retval None 00404 */ 00405 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00406 { 00407 HAL_StatusTypeDef status = HAL_OK; 00408 00409 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) DataIn, DataOut, DataLength, SpixTimeout); 00410 00411 /* Check the communication status */ 00412 if(status != HAL_OK) 00413 { 00414 /* Execute user timeout callback */ 00415 SPIx_Error(); 00416 } 00417 } 00418 00419 /** 00420 * @brief SPI Write an amount of data to device 00421 * @param DataIn: value to be written 00422 * @param DataLength: number of bytes to write 00423 * @retval None 00424 */ 00425 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength) 00426 { 00427 HAL_StatusTypeDef status = HAL_OK; 00428 00429 status = HAL_SPI_Transmit(&hnucleo_Spi, DataIn, DataLength, SpixTimeout); 00430 00431 /* Check the communication status */ 00432 if(status != HAL_OK) 00433 { 00434 /* Execute user timeout callback */ 00435 SPIx_Error(); 00436 } 00437 } 00438 00439 /** 00440 * @brief SPI Write a byte to device 00441 * @param Value: value to be written 00442 * @retval None 00443 */ 00444 static void SPIx_Write(uint8_t Value) 00445 { 00446 HAL_StatusTypeDef status = HAL_OK; 00447 uint8_t data; 00448 00449 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00450 00451 /* Check the communication status */ 00452 if(status != HAL_OK) 00453 { 00454 /* Execute user timeout callback */ 00455 SPIx_Error(); 00456 } 00457 } 00458 00459 /** 00460 * @brief SPI error treatment function 00461 * @retval None 00462 */ 00463 static void SPIx_Error (void) 00464 { 00465 /* De-initialize the SPI communication BUS */ 00466 HAL_SPI_DeInit(&hnucleo_Spi); 00467 00468 /* Re-Initiaize the SPI communication BUS */ 00469 SPIx_Init(); 00470 } 00471 00472 /****************************************************************************** 00473 LINK OPERATIONS 00474 *******************************************************************************/ 00475 00476 /********************************* LINK SD ************************************/ 00477 /** 00478 * @brief Initialize the SD Card and put it into StandBy State (Ready for 00479 * data transfer). 00480 * @retval None 00481 */ 00482 void SD_IO_Init(void) 00483 { 00484 GPIO_InitTypeDef gpioinitstruct = {0}; 00485 uint8_t counter = 0; 00486 00487 /* SD_CS_GPIO Periph clock enable */ 00488 SD_CS_GPIO_CLK_ENABLE(); 00489 00490 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00491 gpioinitstruct.Pin = SD_CS_PIN; 00492 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00493 gpioinitstruct.Pull = GPIO_PULLUP; 00494 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00495 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00496 00497 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00498 gpioinitstruct.Pin = LCD_CS_PIN; 00499 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00500 gpioinitstruct.Pull = GPIO_NOPULL; 00501 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00502 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00503 LCD_CS_HIGH(); 00504 /*------------Put SD in SPI mode--------------*/ 00505 /* SD SPI Config */ 00506 SPIx_Init(); 00507 00508 /* SD chip select high */ 00509 SD_CS_HIGH(); 00510 00511 /* Send dummy byte 0xFF, 10 times with CS high */ 00512 /* Rise CS and MOSI for 80 clocks cycles */ 00513 for (counter = 0; counter <= 9; counter++) 00514 { 00515 /* Send dummy byte 0xFF */ 00516 SD_IO_WriteByte(SD_DUMMY_BYTE); 00517 } 00518 } 00519 00520 /** 00521 * @brief Set the SD_CS pin. 00522 * @param val: pin value. 00523 * @retval None 00524 */ 00525 void SD_IO_CSState(uint8_t val) 00526 { 00527 if(val == 1) 00528 { 00529 SD_CS_HIGH(); 00530 } 00531 else 00532 { 00533 SD_CS_LOW(); 00534 } 00535 } 00536 00537 /** 00538 * @brief Write byte(s) on the SD 00539 * @param DataIn: Pointer to data buffer to write 00540 * @param DataOut: Pointer to data buffer for read data 00541 * @param DataLength: number of bytes to write 00542 * @retval None 00543 */ 00544 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00545 { 00546 /* Send the byte */ 00547 SPIx_WriteReadData(DataIn, DataOut, DataLength); 00548 } 00549 00550 /** 00551 * @brief Write a byte on the SD. 00552 * @param Data: byte to send. 00553 * @retval Data written 00554 */ 00555 uint8_t SD_IO_WriteByte(uint8_t Data) 00556 { 00557 uint8_t tmp; 00558 00559 /* Send the byte */ 00560 SPIx_WriteReadData(&Data,&tmp,1); 00561 return tmp; 00562 } 00563 00564 /** 00565 * @brief Write an amount of data on the SD. 00566 * @param DataOut: byte to send. 00567 * @param DataLength: number of bytes to write 00568 * @retval none 00569 */ 00570 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength) 00571 { 00572 /* Send the byte */ 00573 SD_IO_WriteReadData(DataOut, DataOut, DataLength); 00574 } 00575 00576 /** 00577 * @brief Write an amount of data on the SD. 00578 * @param Data: byte to send. 00579 * @param DataLength: number of bytes to write 00580 * @retval none 00581 */ 00582 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength) 00583 { 00584 /* Send the byte */ 00585 SPIx_WriteData((uint8_t *)Data, DataLength); 00586 } 00587 00588 /********************************* LINK LCD ***********************************/ 00589 /** 00590 * @brief Initialize the LCD 00591 * @retval None 00592 */ 00593 void LCD_IO_Init(void) 00594 { 00595 GPIO_InitTypeDef gpioinitstruct = {0}; 00596 00597 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00598 LCD_CS_GPIO_CLK_ENABLE(); 00599 LCD_DC_GPIO_CLK_ENABLE(); 00600 00601 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00602 gpioinitstruct.Pin = LCD_CS_PIN; 00603 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00604 gpioinitstruct.Pull = GPIO_NOPULL; 00605 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00606 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00607 00608 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00609 gpioinitstruct.Pin = LCD_DC_PIN; 00610 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &gpioinitstruct); 00611 00612 /* LCD chip select high */ 00613 LCD_CS_HIGH(); 00614 00615 /* LCD SPI Config */ 00616 SPIx_Init(); 00617 } 00618 00619 /** 00620 * @brief Write command to select the LCD register. 00621 * @param LCDReg: Address of the selected register. 00622 * @retval None 00623 */ 00624 void LCD_IO_WriteReg(uint8_t LCDReg) 00625 { 00626 /* Reset LCD control line CS */ 00627 LCD_CS_LOW(); 00628 00629 /* Set LCD data/command line DC to Low */ 00630 LCD_DC_LOW(); 00631 00632 /* Send Command */ 00633 SPIx_Write(LCDReg); 00634 00635 /* Deselect : Chip Select high */ 00636 LCD_CS_HIGH(); 00637 } 00638 00639 /** 00640 * @brief Writes data to select the LCD register. 00641 * This function must be used after st7735_WriteReg() function 00642 * @param Data: data to write to the selected register. 00643 * @retval None 00644 */ 00645 void LCD_IO_WriteData(uint8_t Data) 00646 { 00647 /* Reset LCD control line CS */ 00648 LCD_CS_LOW(); 00649 00650 /* Set LCD data/command line DC to High */ 00651 LCD_DC_HIGH(); 00652 00653 /* Send Data */ 00654 SPIx_Write(Data); 00655 00656 /* Deselect : Chip Select high */ 00657 LCD_CS_HIGH(); 00658 } 00659 00660 /** 00661 * @brief Write register value. 00662 * @param pData Pointer on the register value 00663 * @param Size Size of byte to transmit to the register 00664 * @retval None 00665 */ 00666 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00667 { 00668 uint32_t counter = 0; 00669 __IO uint32_t data = 0; 00670 00671 /* Reset LCD control line CS */ 00672 LCD_CS_LOW(); 00673 00674 /* Set LCD data/command line DC to High */ 00675 LCD_DC_HIGH(); 00676 00677 if (Size == 1) 00678 { 00679 /* Only 1 byte to be sent to LCD - general interface can be used */ 00680 /* Send Data */ 00681 SPIx_Write(*pData); 00682 } 00683 else 00684 { 00685 /* Several data should be sent in a raw */ 00686 /* Direct SPI accesses for optimization */ 00687 for (counter = Size; counter != 0; counter--) 00688 { 00689 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00690 { 00691 } 00692 /* Need to invert bytes for LCD*/ 00693 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *(pData+1); 00694 00695 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00696 { 00697 } 00698 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *pData; 00699 counter--; 00700 pData += 2; 00701 } 00702 00703 /* Wait until the bus is ready before releasing Chip select */ 00704 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 00705 { 00706 } 00707 } 00708 00709 /* Empty the Rx fifo */ 00710 data = *(&hnucleo_Spi.Instance->DR); 00711 UNUSED(data); /* Remove GNU warning */ 00712 00713 /* Deselect : Chip Select high */ 00714 LCD_CS_HIGH(); 00715 } 00716 00717 /** 00718 * @brief Wait for loop in ms. 00719 * @param Delay in ms. 00720 * @retval None 00721 */ 00722 void LCD_Delay(uint32_t Delay) 00723 { 00724 HAL_Delay(Delay); 00725 } 00726 #endif /* HAL_SPI_MODULE_ENABLED */ 00727 00728 /******************************* LINK JOYSTICK ********************************/ 00729 #ifdef HAL_ADC_MODULE_ENABLED 00730 /** 00731 * @brief Initialize ADC MSP. 00732 * @retval None 00733 */ 00734 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 00735 { 00736 GPIO_InitTypeDef gpioinitstruct = {0}; 00737 00738 /*** Configure the GPIOs ***/ 00739 /* Enable GPIO clock */ 00740 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 00741 00742 /* Configure ADC1 Channel8 as analog input */ 00743 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00744 gpioinitstruct.Mode = GPIO_MODE_ANALOG; 00745 gpioinitstruct.Pull = GPIO_NOPULL; 00746 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &gpioinitstruct); 00747 00748 /*** Configure the ADC peripheral ***/ 00749 /* Enable ADC clock */ 00750 NUCLEO_ADCx_CLK_ENABLE(); 00751 } 00752 00753 /** 00754 * @brief DeInitializes ADC MSP. 00755 * @param hadc: ADC peripheral 00756 * @note ADC DeInit does not disable the GPIO clock 00757 * @retval None 00758 */ 00759 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc) 00760 { 00761 GPIO_InitTypeDef gpioinitstruct; 00762 00763 /*** DeInit the ADC peripheral ***/ 00764 /* Disable ADC clock */ 00765 NUCLEO_ADCx_CLK_DISABLE(); 00766 00767 /* Configure the selected ADC Channel as analog input */ 00768 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00769 HAL_GPIO_DeInit(NUCLEO_ADCx_GPIO_PORT, gpioinitstruct.Pin); 00770 00771 /* Disable GPIO clock has to be done by the application*/ 00772 /* NUCLEO_ADCx_GPIO_CLK_DISABLE(); */ 00773 } 00774 00775 /** 00776 * @brief Initializes ADC HAL. 00777 * @retval None 00778 */ 00779 static HAL_StatusTypeDef ADCx_Init(void) 00780 { 00781 /* Set ADC instance */ 00782 hnucleo_Adc.Instance = NUCLEO_ADCx; 00783 00784 if(HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 00785 { 00786 /* ADC Config */ 00787 hnucleo_Adc.Instance = NUCLEO_ADCx; 00788 hnucleo_Adc.Init.OversamplingMode = DISABLE; 00789 hnucleo_Adc.Init.ClockPrescaler = ADC_CLOCK_SYNC_PCLK_DIV2; /* (must not exceed 16MHz) */ 00790 hnucleo_Adc.Init.LowPowerAutoPowerOff = DISABLE; 00791 hnucleo_Adc.Init.LowPowerFrequencyMode = ENABLE; 00792 hnucleo_Adc.Init.LowPowerAutoWait = ENABLE; 00793 hnucleo_Adc.Init.Resolution = ADC_RESOLUTION_12B; 00794 hnucleo_Adc.Init.SamplingTime = ADC_SAMPLETIME_1CYCLE_5; 00795 hnucleo_Adc.Init.ScanConvMode = ADC_SCAN_DIRECTION_FORWARD; 00796 hnucleo_Adc.Init.DataAlign = ADC_DATAALIGN_RIGHT; 00797 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; 00798 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; 00799 hnucleo_Adc.Init.ExternalTrigConv = ADC_SOFTWARE_START; /* Trig of conversion start done manually by software, without external event */ 00800 hnucleo_Adc.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because trig by software start */ 00801 hnucleo_Adc.Init.EOCSelection = ADC_EOC_SEQ_CONV; 00802 hnucleo_Adc.Init.DMAContinuousRequests = DISABLE; 00803 00804 /* Initialize MSP related to ADC */ 00805 ADCx_MspInit(&hnucleo_Adc); 00806 00807 /* Initialize ADC */ 00808 if (HAL_ADC_Init(&hnucleo_Adc) != HAL_OK) 00809 { 00810 return HAL_ERROR; 00811 } 00812 00813 if (HAL_ADCEx_Calibration_Start(&hnucleo_Adc,ADC_SINGLE_ENDED) != HAL_OK) 00814 { 00815 return HAL_ERROR; 00816 } 00817 } 00818 00819 return HAL_OK; 00820 } 00821 00822 /** 00823 * @brief Initializes ADC HAL. 00824 * @retval None 00825 */ 00826 static void ADCx_DeInit(void) 00827 { 00828 hnucleo_Adc.Instance = NUCLEO_ADCx; 00829 00830 HAL_ADC_DeInit(&hnucleo_Adc); 00831 ADCx_MspDeInit(&hnucleo_Adc); 00832 } 00833 00834 /******************************* LINK JOYSTICK ********************************/ 00835 00836 /** 00837 * @brief Configures joystick available on adafruit 1.8" TFT shield 00838 * managed through ADC to detect motion. 00839 * @retval Joystickstatus (0=> success, 1=> fail) 00840 */ 00841 uint8_t BSP_JOY_Init(void) 00842 { 00843 if (ADCx_Init() != HAL_OK) 00844 { 00845 return (uint8_t) HAL_ERROR; 00846 } 00847 00848 /* Select Channel 8 to be converted */ 00849 sConfig.Channel = ADC_CHANNEL_8; 00850 sConfig.Rank = ADC_RANK_CHANNEL_NUMBER; 00851 00852 /* Return Joystick initialization status */ 00853 return (uint8_t) HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00854 } 00855 00856 /** 00857 * @brief DeInit joystick GPIOs. 00858 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00859 * @retval None. 00860 */ 00861 void BSP_JOY_DeInit(void) 00862 { 00863 ADCx_DeInit(); 00864 } 00865 00866 /** 00867 * @brief Returns the Joystick key pressed. 00868 * @note To know which Joystick key is pressed we need to detect the voltage 00869 * level on each key output 00870 * - None : 3.3 V / 4095 00871 * - SEL : 1.055 V / 1308 00872 * - DOWN : 0.71 V / 88 00873 * - LEFT : 3.0 V / 3720 00874 * - RIGHT : 0.595 V / 737 00875 * - UP : 1.65 V / 2046 00876 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00877 */ 00878 JOYState_TypeDef BSP_JOY_GetState(void) 00879 { 00880 JOYState_TypeDef state = JOY_NONE; 00881 uint16_t keyconvertedvalue = 0; 00882 00883 /* Start the conversion process */ 00884 HAL_ADC_Start(&hnucleo_Adc); 00885 00886 /* Wait for the end of conversion */ 00887 if (HAL_ADC_PollForConversion(&hnucleo_Adc, 10) != HAL_TIMEOUT) 00888 { 00889 /* Get the converted value of regular channel */ 00890 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00891 } 00892 00893 if((keyconvertedvalue > 2010) && (keyconvertedvalue < 2090)) 00894 { 00895 state = JOY_UP; 00896 } 00897 else if((keyconvertedvalue > 680) && (keyconvertedvalue < 780)) 00898 { 00899 state = JOY_RIGHT; 00900 } 00901 else if((keyconvertedvalue > 1270) && (keyconvertedvalue < 1350)) 00902 { 00903 state = JOY_SEL; 00904 } 00905 else if((keyconvertedvalue > 50) && (keyconvertedvalue < 130)) 00906 { 00907 state = JOY_DOWN; 00908 } 00909 else if((keyconvertedvalue > 3570) && (keyconvertedvalue < 3800)) 00910 { 00911 state = JOY_LEFT; 00912 } 00913 else 00914 { 00915 state = JOY_NONE; 00916 } 00917 00918 /* Return the code of the Joystick key pressed */ 00919 return state; 00920 } 00921 #endif /* HAL_ADC_MODULE_ENABLED */ 00922 00923 /** 00924 * @} 00925 */ 00926 00927 /** 00928 * @} 00929 */ 00930 00931 /** 00932 * @} 00933 */ 00934 00935 /** 00936 * @} 00937 */ 00938 00939 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:45:03 for STM32L0xx_Nucleo BSP User Manual by
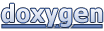