STM32L0538-Discovery BSP User Manual
|
stm32l0538_discovery_epd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l0538_discovery_epd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the EPD driver for Display Module of 00006 * STM32L0538-DISCO kit (MB1143). 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l0xx_hal.h" 00039 #include "stm32l0538_discovery_epd.h" 00040 #include "stm32l0538_discovery.h" 00041 #include "font20epd.c" 00042 #include "font16epd.c" 00043 #include "font12epd.c" 00044 #include "font8epd.c" 00045 00046 /** @addtogroup BSP 00047 * @{ 00048 */ 00049 00050 /** @addtogroup STM32L0538_DISCOVERY 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L0538_DISCOVERY_EPD 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32L0538_DISCOVERY_EPD_Private_TypesDefinitions 00059 * @{ 00060 */ 00061 /** 00062 * @} 00063 */ 00064 00065 /** @defgroup STM32L0538_DISCOVERY_EPD_Private_Defines 00066 * @{ 00067 */ 00068 /** 00069 * @} 00070 */ 00071 00072 /** @defgroup STM32L0538_DISCOVERY_EPD_Private_Variables 00073 * @{ 00074 */ 00075 static sFONT *pFont; 00076 static EPD_DrvTypeDef *epd_drv; 00077 /** 00078 * @} 00079 */ 00080 00081 /** @defgroup STM32L0538_DISCOVERY_EPD_Private_FunctionPrototypes 00082 * @{ 00083 */ 00084 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00085 /** 00086 * @} 00087 */ 00088 00089 /** @defgroup STM32L0538_DISCOVERY_EPD_Private_Functions 00090 * @{ 00091 */ 00092 00093 /** 00094 * @brief Initializes the EPD. 00095 * @param None 00096 * @retval EPD state 00097 */ 00098 uint8_t BSP_EPD_Init(void) 00099 { 00100 uint8_t ret = EPD_ERROR; 00101 00102 /* Default value for the Font */ 00103 pFont = &Font16; 00104 00105 epd_drv = &gde021a1_drv; 00106 00107 /* EPD Init */ 00108 epd_drv->Init(); 00109 00110 /* Clear the EPD screen */ 00111 BSP_EPD_Clear(EPD_COLOR_WHITE); 00112 00113 /* Initialize the font */ 00114 BSP_EPD_SetFont(&EPD_DEFAULT_FONT); 00115 00116 ret = EPD_OK; 00117 00118 return ret; 00119 } 00120 00121 /** 00122 * @brief Gets the EPD X size. 00123 * @param None 00124 * @retval EPD X size 00125 */ 00126 uint32_t BSP_EPD_GetXSize(void) 00127 { 00128 return(epd_drv->GetEpdPixelWidth()); 00129 } 00130 00131 /** 00132 * @brief Gets the EPD Y size. 00133 * @param None 00134 * @retval EPD Y size 00135 */ 00136 uint32_t BSP_EPD_GetYSize(void) 00137 { 00138 return(epd_drv->GetEpdPixelHeight()); 00139 } 00140 00141 /** 00142 * @brief Sets the Text Font. 00143 * @param pFonts: specifies the layer font to be used. 00144 * @retval None 00145 */ 00146 void BSP_EPD_SetFont(sFONT *pFonts) 00147 { 00148 pFont = pFonts; 00149 } 00150 00151 /** 00152 * @brief Gets the Text Font. 00153 * @param None. 00154 * @retval the used layer font. 00155 */ 00156 sFONT *BSP_EPD_GetFont(void) 00157 { 00158 return pFont; 00159 } 00160 00161 /** 00162 * @brief Clears the hole EPD. 00163 * @param Color: Color of the background 00164 * @retval None 00165 */ 00166 void BSP_EPD_Clear(uint16_t Color) 00167 { 00168 uint32_t index = 0; 00169 00170 epd_drv->SetDisplayWindow(0, 0, 171, 17); 00171 00172 for(index = 0; index < 3096; index++) 00173 { 00174 epd_drv->WritePixel(Color); 00175 } 00176 } 00177 00178 /** 00179 * @brief Displays one character. 00180 * @param Xpos: start column address. 00181 * @param Ypos: the Line where to display the character shape. 00182 * @param Ascii: character ascii code, must be between 0x20 and 0x7E. 00183 * @retval None 00184 */ 00185 void BSP_EPD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00186 { 00187 Ascii -= 32; 00188 00189 DrawChar(Xpos, Ypos, &pFont->table[Ascii * ((pFont->Height) * (pFont->Width))]); 00190 } 00191 00192 /** 00193 * @brief Displays characters on the EPD. 00194 * @param Xpos: X position 00195 * @param Ypos: Y position 00196 * @param Text: Pointer to string to display on EPD 00197 * @param Mode: Display mode 00198 * This parameter can be one of the following values: 00199 * @arg CENTER_MODE 00200 * @arg RIGHT_MODE 00201 * @arg LEFT_MODE 00202 * @retval None 00203 */ 00204 void BSP_EPD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00205 { 00206 uint16_t refcolumn = 1, i = 0; 00207 uint32_t size = 0, xsize = 0; 00208 uint8_t *ptr = Text; 00209 00210 /* Get the text size */ 00211 while (*ptr++) size ++ ; 00212 00213 /* Characters number per line */ 00214 xsize = (BSP_EPD_GetXSize()/pFont->Width); 00215 00216 switch (Mode) 00217 { 00218 case CENTER_MODE: 00219 { 00220 refcolumn = Xpos + ((xsize - size)* pFont->Width) / 2; 00221 break; 00222 } 00223 case LEFT_MODE: 00224 { 00225 refcolumn = Xpos; 00226 break; 00227 } 00228 case RIGHT_MODE: 00229 { 00230 refcolumn = - Xpos + ((xsize - size)*pFont->Width); 00231 break; 00232 } 00233 default: 00234 { 00235 refcolumn = Xpos; 00236 break; 00237 } 00238 } 00239 00240 /* Send the string character by character on EPD */ 00241 while ((*Text != 0) & (((BSP_EPD_GetXSize() - (i*pFont->Width)) & 0xFFFF) >= pFont->Width)) 00242 { 00243 /* Display one character on EPD */ 00244 BSP_EPD_DisplayChar(refcolumn, Ypos, *Text); 00245 /* Decrement the column position by 16 */ 00246 refcolumn += pFont->Width; 00247 /* Point on the next character */ 00248 Text++; 00249 i++; 00250 } 00251 } 00252 00253 /** 00254 * @brief Displays a character on the EPD. 00255 * @param Line: Line where to display the character shape 00256 * This parameter can be one of the following values: 00257 * @arg 0..8: if the Current fonts is Font8 00258 * @arg 0..5: if the Current fonts is Font12 00259 * @arg 0..3: if the Current fonts is Font16 00260 * @arg 0..2: if the Current fonts is Font20 00261 * @param ptr: Pointer to string to display on EPD 00262 * @retval None 00263 */ 00264 void BSP_EPD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00265 { 00266 BSP_EPD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00267 } 00268 00269 /** 00270 * @brief Draws an horizontal line. 00271 * @param Xpos: X position 00272 * @param Ypos: Y position 00273 * @param Length: line length 00274 * @retval None 00275 */ 00276 void BSP_EPD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00277 { 00278 uint32_t index = 0; 00279 00280 epd_drv->SetDisplayWindow(Xpos, Ypos, Xpos + Length, Ypos); 00281 00282 for(index = 0; index < Length; index++) 00283 { 00284 /* Prepare the register to write data on the RAM */ 00285 epd_drv->WritePixel(0x3F); 00286 } 00287 } 00288 00289 /** 00290 * @brief Draws a vertical line. 00291 * @param Xpos: X position 00292 * @param Ypos: Y position 00293 * @param Length: line length. 00294 * @retval None 00295 */ 00296 void BSP_EPD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00297 { 00298 uint32_t index = 0; 00299 00300 epd_drv->SetDisplayWindow(Xpos, Ypos, Xpos, Ypos + Length); 00301 00302 for(index = 0; index < Length; index++) 00303 { 00304 /* Prepare the register to write data on the RAM */ 00305 epd_drv->WritePixel(0x00); 00306 } 00307 } 00308 00309 /** 00310 * @brief Draws a rectangle. 00311 * @param Xpos: X position 00312 * @param Ypos: Y position 00313 * @param Height: rectangle height 00314 * @param Width: rectangle width 00315 * @retval None 00316 */ 00317 void BSP_EPD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00318 { 00319 /* Draw horizontal lines */ 00320 BSP_EPD_DrawHLine(Xpos, Ypos, Width); 00321 BSP_EPD_DrawHLine(Xpos, (Ypos + Height), (Width + 1)); 00322 00323 /* Draw vertical lines */ 00324 BSP_EPD_DrawVLine(Xpos, Ypos, Height); 00325 BSP_EPD_DrawVLine((Xpos + Width), Ypos , Height); 00326 } 00327 00328 /** 00329 * @brief Displays a full rectangle. 00330 * @param Xpos: X position. 00331 * @param Ypos: Y position. 00332 * @param Height: display rectangle height. 00333 * @param Width: display rectangle width. 00334 * @retval None 00335 */ 00336 void BSP_EPD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00337 { 00338 uint16_t index = 0; 00339 00340 /* Set the rectangle */ 00341 epd_drv->SetDisplayWindow(Xpos, Ypos, (Xpos + Width), (Ypos + Height)); 00342 00343 for(index = 0; index < 3096; index++) 00344 { 00345 epd_drv->WritePixel(0xFF); 00346 } 00347 } 00348 00349 /** 00350 * @brief Draws an Image. 00351 * @param Xpos: X position in the EPD 00352 * @param Ypos: Y position in the EPD 00353 * @param Xsize: X size in the EPD 00354 * @param Ysize: Y size in the EPD 00355 * @param pdata: Pointer to the Image address 00356 * @retval None 00357 */ 00358 void BSP_EPD_DrawImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00359 { 00360 /* Set display window */ 00361 epd_drv->SetDisplayWindow(Xpos, Ypos, (Xpos+Ysize-1), (Ypos+(Xsize/4)-1)); 00362 00363 if(epd_drv->DrawImage != NULL) 00364 { 00365 epd_drv->DrawImage(Xpos, Ypos, Xsize, Ysize, pdata); 00366 } 00367 epd_drv->SetDisplayWindow(0, 0, BSP_EPD_GetXSize(), BSP_EPD_GetYSize()); 00368 } 00369 00370 /** 00371 * @brief Disables the clock and the charge pump. 00372 * @param None 00373 * @retval None 00374 */ 00375 void BSP_EPD_CloseChargePump(void) 00376 { 00377 /* Close charge pump */ 00378 epd_drv->CloseChargePump(); 00379 00380 /* Add a 400 ms delay */ 00381 EPD_Delay(400); 00382 } 00383 00384 /** 00385 * @brief Updates the display from the data located into the RAM. 00386 * @param None 00387 * @retval None 00388 */ 00389 void BSP_EPD_RefreshDisplay(void) 00390 { 00391 /* Refresh display sequence */ 00392 epd_drv->RefreshDisplay(); 00393 00394 /* Poll on the BUSY signal and wait for the EPD to be ready */ 00395 while (HAL_GPIO_ReadPin(EPD_BUSY_GPIO_PORT, EPD_BUSY_PIN) != (uint16_t)RESET); 00396 00397 /* EPD reset pin mamagement */ 00398 EPD_RESET_HIGH(); 00399 00400 /* Add a 10 ms Delay after EPD pin Reset */ 00401 EPD_Delay(10); 00402 } 00403 00404 /******************************************************************************* 00405 Static Functions 00406 *******************************************************************************/ 00407 00408 /** 00409 * @brief Draws a character on EPD. 00410 * @param Xpos: specifies the X position, can be a value from 0 to 171 00411 * @param Ypos: specifies the Y position, can be a value from 0 to 17 00412 * @param c: pointer to the character data 00413 * @retval None 00414 */ 00415 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 00416 { 00417 uint32_t index = 0; 00418 uint32_t data_length = 0; 00419 uint16_t height = 0; 00420 uint16_t width = 0; 00421 00422 width = pFont->Width; 00423 height = pFont->Height; 00424 00425 /* Set the Character display window */ 00426 epd_drv->SetDisplayWindow(Xpos, Ypos, (Xpos + width - 1), (Ypos + height - 1)); 00427 00428 data_length = (height * width); 00429 00430 for(index = 0; index < data_length; index++) 00431 { 00432 epd_drv->WritePixel(c[index]); 00433 } 00434 } 00435 00436 /** 00437 * @} 00438 */ 00439 00440 /** 00441 * @} 00442 */ 00443 00444 /** 00445 * @} 00446 */ 00447 00448 /** 00449 * @} 00450 */ 00451 00452 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:51:54 for STM32L0538-Discovery BSP User Manual by
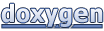