STM32F723E-Discovery BSP User Manual
|
stm32f723e_discovery_qspi.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f723e_discovery_qspi.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32f723e_discovery_qspi.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /** @addtogroup BSP 00038 * @{ 00039 */ 00040 00041 /** @addtogroup STM32F723E_DISCOVERY 00042 * @{ 00043 */ 00044 00045 /* Define to prevent recursive inclusion -------------------------------------*/ 00046 #ifndef __STM32F723E_DISCOVERY_QSPI_H 00047 #define __STM32F723E_DISCOVERY_QSPI_H 00048 00049 #ifdef __cplusplus 00050 extern "C" { 00051 #endif 00052 00053 /* Includes ------------------------------------------------------------------*/ 00054 #include "stm32f7xx_hal.h" 00055 #include "../Components/mx25l512/mx25l512.h" 00056 00057 /** @addtogroup STM32F723E_DISCOVERY_QSPI 00058 * @{ 00059 */ 00060 00061 00062 /* Exported constants --------------------------------------------------------*/ 00063 /** @defgroup STM32F723E_DISCOVERY_QSPI_Exported_Constants STM32F723E_DISCOVERY_QSPI Exported Constants 00064 * @{ 00065 */ 00066 /* QSPI Error codes */ 00067 #define QSPI_OK ((uint8_t)0x00) 00068 #define QSPI_ERROR ((uint8_t)0x01) 00069 #define QSPI_BUSY ((uint8_t)0x02) 00070 #define QSPI_NOT_SUPPORTED ((uint8_t)0x04) 00071 #define QSPI_SUSPENDED ((uint8_t)0x08) 00072 00073 00074 /* Definition for QSPI clock resources */ 00075 #define QSPI_CLK_ENABLE() __HAL_RCC_QSPI_CLK_ENABLE() 00076 #define QSPI_CLK_DISABLE() __HAL_RCC_QSPI_CLK_DISABLE() 00077 #define QSPI_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00078 #define QSPI_CLK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00079 #define QSPI_D0_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00080 #define QSPI_D1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00081 #define QSPI_D2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOE_CLK_ENABLE() 00082 #define QSPI_D3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00083 00084 #define QSPI_FORCE_RESET() __HAL_RCC_QSPI_FORCE_RESET() 00085 #define QSPI_RELEASE_RESET() __HAL_RCC_QSPI_RELEASE_RESET() 00086 00087 /* Definition for QSPI Pins */ 00088 /* QSPI_CS */ 00089 #define QSPI_CS_PIN GPIO_PIN_6 00090 #define QSPI_CS_GPIO_PORT GPIOB 00091 #define QSPI_CS_PIN_AF GPIO_AF10_QUADSPI 00092 /* QSPI_CLK */ 00093 #define QSPI_CLK_PIN GPIO_PIN_2 00094 #define QSPI_CLK_GPIO_PORT GPIOB 00095 #define QSPI_CLK_PIN_AF GPIO_AF9_QUADSPI 00096 /* QSPI_D0 */ 00097 #define QSPI_D0_PIN GPIO_PIN_9 00098 #define QSPI_D0_GPIO_PORT GPIOC 00099 #define QSPI_D0_PIN_AF GPIO_AF9_QUADSPI 00100 /* QSPI_D1 */ 00101 #define QSPI_D1_PIN GPIO_PIN_10 00102 #define QSPI_D1_GPIO_PORT GPIOC 00103 #define QSPI_D1_PIN_AF GPIO_AF9_QUADSPI 00104 /* QSPI_D2 */ 00105 #define QSPI_D2_PIN GPIO_PIN_2 00106 #define QSPI_D2_GPIO_PORT GPIOE 00107 #define QSPI_D2_PIN_AF GPIO_AF9_QUADSPI 00108 /* QSPI_D3 */ 00109 #define QSPI_D3_PIN GPIO_PIN_13 00110 #define QSPI_D3_GPIO_PORT GPIOD 00111 #define QSPI_D3_PIN_AF GPIO_AF9_QUADSPI 00112 00113 /** 00114 * @} 00115 */ 00116 00117 /* Exported types ------------------------------------------------------------*/ 00118 /** @defgroup STM32F723E_DISCOVERY_QSPI_Exported_Types STM32F723E_DISCOVERY_QSPI Exported Types 00119 * @{ 00120 */ 00121 /* QSPI Info */ 00122 typedef struct { 00123 uint32_t FlashSize; /*!< Size of the flash */ 00124 uint32_t EraseSectorSize; /*!< Size of sectors for the erase operation */ 00125 uint32_t EraseSectorsNumber; /*!< Number of sectors for the erase operation */ 00126 uint32_t ProgPageSize; /*!< Size of pages for the program operation */ 00127 uint32_t ProgPagesNumber; /*!< Number of pages for the program operation */ 00128 } QSPI_Info; 00129 00130 /** 00131 * @} 00132 */ 00133 00134 00135 /* Exported functions --------------------------------------------------------*/ 00136 /** @addtogroup STM32F723E_DISCOVERY_QSPI_Exported_Functions 00137 * @{ 00138 */ 00139 uint8_t BSP_QSPI_Init (void); 00140 uint8_t BSP_QSPI_DeInit (void); 00141 uint8_t BSP_QSPI_Read (uint8_t* pData, uint32_t ReadAddr, uint32_t Size); 00142 uint8_t BSP_QSPI_Write (uint8_t* pData, uint32_t WriteAddr, uint32_t Size); 00143 uint8_t BSP_QSPI_Erase_Block(uint32_t BlockAddress); 00144 uint8_t BSP_QSPI_Erase_Chip (void); 00145 uint8_t BSP_QSPI_GetStatus (void); 00146 uint8_t BSP_QSPI_GetInfo (QSPI_Info* pInfo); 00147 uint8_t BSP_QSPI_EnableMemoryMappedMode(void); 00148 00149 /* These functions can be modified in case the current settings 00150 need to be changed for specific application needs */ 00151 void BSP_QSPI_MspInit(QSPI_HandleTypeDef *hqspi, void *Params); 00152 void BSP_QSPI_MspDeInit(QSPI_HandleTypeDef *hqspi, void *Params); 00153 00154 /** 00155 * @} 00156 */ 00157 00158 /** 00159 * @} 00160 */ 00161 00162 #ifdef __cplusplus 00163 } 00164 #endif 00165 00166 #endif /* __STM32F723E_DISCOVERY_QSPI_H */ 00167 /** 00168 * @} 00169 */ 00170 00171 /** 00172 * @} 00173 */ 00174 00175 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue May 30 2017 13:59:12 for STM32F723E-Discovery BSP User Manual by
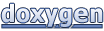