STM32F723E-Discovery BSP User Manual
|
stm32f723e_discovery_psram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f723e_discovery_psram.c 00004 * @author MCD Application Team 00005 * @brief This file includes the PSRAM driver for the IS61WV51216BLL-10MLI memory 00006 * device mounted on STM32F723E-DISCOVERY boards. 00007 @verbatim 00008 How To use this driver: 00009 ----------------------- 00010 - This driver is used to drive the IS61WV51216BLL-10M PSRAM external memory mounted 00011 on STM32F723E discovery board. 00012 - This driver does not need a specific component driver for the PSRAM device 00013 to be included with. 00014 00015 Driver description: 00016 ------------------ 00017 + Initialization steps: 00018 o Initialize the PSRAM external memory using the BSP_PSRAM_Init() function. This 00019 function includes the MSP layer hardware resources initialization and the 00020 FMC controller configuration to interface with the external PSRAM memory. 00021 00022 + PSRAM read/write operations 00023 o PSRAM external memory can be accessed with read/write operations once it is 00024 initialized. 00025 Read/write operation can be performed with AHB access using the functions 00026 BSP_PSRAM_ReadData()/BSP_PSRAM_WriteData(), or by DMA transfer using the functions 00027 BSP_PSRAM_ReadData_DMA()/BSP_PSRAM_WriteData_DMA(). 00028 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00029 configuration is fixed at single (no burst) halfword transfer 00030 (see the PSRAM_MspInit() static function). 00031 o User can implement his own functions for read/write access with his desired 00032 configurations. 00033 o If interrupt mode is used for DMA transfer, the function BSP_PSRAM_DMA_IRQHandler() 00034 is called in IRQ handler file, to serve the generated interrupt once the DMA 00035 transfer is complete. 00036 @endverbatim 00037 ****************************************************************************** 00038 * @attention 00039 * 00040 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00041 * 00042 * Redistribution and use in source and binary forms, with or without modification, 00043 * are permitted provided that the following conditions are met: 00044 * 1. Redistributions of source code must retain the above copyright notice, 00045 * this list of conditions and the following disclaimer. 00046 * 2. Redistributions in binary form must reproduce the above copyright notice, 00047 * this list of conditions and the following disclaimer in the documentation 00048 * and/or other materials provided with the distribution. 00049 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00050 * may be used to endorse or promote products derived from this software 00051 * without specific prior written permission. 00052 * 00053 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00054 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00055 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00056 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00057 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00058 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00059 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00060 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00061 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00062 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00063 * 00064 ****************************************************************************** 00065 */ 00066 00067 /* Dependencies 00068 - stm32f7xx_hal_sram.c 00069 - stm32f7xx_hal_gpio.c 00070 - stm32f7xx_hal_dma.c 00071 - stm32f7xx_hal_cortex.c 00072 - stm32f7xx_hal_rcc_ex.h 00073 EndDependencies */ 00074 00075 /* Includes ------------------------------------------------------------------*/ 00076 #include "stm32f723e_discovery_psram.h" 00077 00078 /** @addtogroup BSP 00079 * @{ 00080 */ 00081 00082 /** @addtogroup STM32F723E_DISCOVERY 00083 * @{ 00084 */ 00085 00086 /** @defgroup STM32F723E_DISCOVERY_PSRAM STM32F723E-DISCOVERY PSRAM 00087 * @{ 00088 */ 00089 00090 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Types_Definitions PSRAM Private Types Definitions 00091 * @{ 00092 */ 00093 /** 00094 * @} 00095 */ 00096 00097 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Defines PSRAM Private Defines 00098 * @{ 00099 */ 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Macros PSRAM Private Macros 00105 * @{ 00106 */ 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Variables PSRAM Private Variables 00112 * @{ 00113 */ 00114 SRAM_HandleTypeDef psramHandle; 00115 00116 /** 00117 * @} 00118 */ 00119 00120 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Function_Prototypes PSRAM Private Function Prototypes 00121 * @{ 00122 */ 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup STM32F723E_DISCOVERY_PSRAM_Private_Functions PSRAM Private Functions 00128 * @{ 00129 */ 00130 00131 /** 00132 * @brief Initializes the PSRAM device. 00133 * @retval PSRAM status 00134 */ 00135 uint8_t BSP_PSRAM_Init(void) 00136 { 00137 static FMC_NORSRAM_TimingTypeDef Timing; 00138 static uint8_t psram_status = PSRAM_ERROR; 00139 00140 /* PSRAM device configuration */ 00141 psramHandle.Instance = FMC_NORSRAM_DEVICE; 00142 psramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00143 00144 /* PSRAM device configuration */ 00145 /* Timing configuration derived from system clock (up to 216Mhz) 00146 for 108Mhz as PSRAM clock frequency */ 00147 Timing.AddressSetupTime = 9; 00148 Timing.AddressHoldTime = 2; 00149 Timing.DataSetupTime = 6; 00150 Timing.BusTurnAroundDuration = 1; 00151 Timing.CLKDivision = 2; 00152 Timing.DataLatency = 2; 00153 Timing.AccessMode = FMC_ACCESS_MODE_A; 00154 00155 psramHandle.Init.NSBank = FMC_NORSRAM_BANK1; 00156 psramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00157 psramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00158 psramHandle.Init.MemoryDataWidth = PSRAM_MEMORY_WIDTH; 00159 psramHandle.Init.BurstAccessMode = PSRAM_BURSTACCESS; 00160 psramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00161 psramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00162 psramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00163 psramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00164 psramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00165 psramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00166 psramHandle.Init.WriteBurst = PSRAM_WRITEBURST; 00167 psramHandle.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00168 psramHandle.Init.PageSize = FMC_PAGE_SIZE_NONE; 00169 psramHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00170 00171 /* PSRAM controller initialization */ 00172 BSP_PSRAM_MspInit(&psramHandle, NULL); /* __weak function can be rewritten by the application */ 00173 if(HAL_SRAM_Init(&psramHandle, &Timing, &Timing) != HAL_OK) 00174 { 00175 psram_status = PSRAM_ERROR; 00176 } 00177 else 00178 { 00179 psram_status = PSRAM_OK; 00180 } 00181 return psram_status; 00182 } 00183 00184 /** 00185 * @brief DeInitializes the PSRAM device. 00186 * @retval PSRAM status 00187 */ 00188 uint8_t BSP_PSRAM_DeInit(void) 00189 { 00190 static uint8_t psram_status = PSRAM_ERROR; 00191 /* PSRAM device de-initialization */ 00192 psramHandle.Instance = FMC_NORSRAM_DEVICE; 00193 psramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00194 00195 if(HAL_SRAM_DeInit(&psramHandle) != HAL_OK) 00196 { 00197 psram_status = PSRAM_ERROR; 00198 } 00199 else 00200 { 00201 psram_status = PSRAM_OK; 00202 } 00203 00204 /* PSRAM controller de-initialization */ 00205 BSP_PSRAM_MspDeInit(&psramHandle, NULL); 00206 00207 return psram_status; 00208 } 00209 00210 /** 00211 * @brief Reads an amount of data from the PSRAM device in polling mode. 00212 * @param uwStartAddress: Read start address 00213 * @param pData: Pointer to data to be read 00214 * @param uwDataSize: Size of read data from the memory 00215 * @retval PSRAM status 00216 */ 00217 uint8_t BSP_PSRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00218 { 00219 if(HAL_SRAM_Read_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00220 { 00221 return PSRAM_ERROR; 00222 } 00223 else 00224 { 00225 return PSRAM_OK; 00226 } 00227 } 00228 00229 /** 00230 * @brief Reads an amount of data from the PSRAM device in DMA mode. 00231 * @param uwStartAddress: Read start address 00232 * @param pData: Pointer to data to be read 00233 * @param uwDataSize: Size of read data from the memory 00234 * @retval PSRAM status 00235 */ 00236 uint8_t BSP_PSRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00237 { 00238 if(HAL_SRAM_Read_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00239 { 00240 return PSRAM_ERROR; 00241 } 00242 else 00243 { 00244 return PSRAM_OK; 00245 } 00246 } 00247 00248 /** 00249 * @brief Writes an amount of data from the PSRAM device in polling mode. 00250 * @param uwStartAddress: Write start address 00251 * @param pData: Pointer to data to be written 00252 * @param uwDataSize: Size of written data from the memory 00253 * @retval PSRAM status 00254 */ 00255 uint8_t BSP_PSRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00256 { 00257 if(HAL_SRAM_Write_16b(&psramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00258 { 00259 return PSRAM_ERROR; 00260 } 00261 else 00262 { 00263 return PSRAM_OK; 00264 } 00265 } 00266 00267 /** 00268 * @brief Writes an amount of data from the PSRAM device in DMA mode. 00269 * @param uwStartAddress: Write start address 00270 * @param pData: Pointer to data to be written 00271 * @param uwDataSize: Size of written data from the memory 00272 * @retval PSRAM status 00273 */ 00274 uint8_t BSP_PSRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00275 { 00276 if(HAL_SRAM_Write_DMA(&psramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00277 { 00278 return PSRAM_ERROR; 00279 } 00280 else 00281 { 00282 return PSRAM_OK; 00283 } 00284 } 00285 00286 /** 00287 * @brief Initializes PSRAM MSP. 00288 * @param hsram: PSRAM handle 00289 * @param Params 00290 * @retval None 00291 */ 00292 __weak void BSP_PSRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params) 00293 { 00294 static DMA_HandleTypeDef dma_handle; 00295 GPIO_InitTypeDef gpio_init_structure; 00296 00297 /* Enable FMC clock */ 00298 __HAL_RCC_FMC_CLK_ENABLE(); 00299 00300 /* Enable chosen DMAx clock */ 00301 __PSRAM_DMAx_CLK_ENABLE(); 00302 00303 /* Enable GPIOs clock */ 00304 __HAL_RCC_GPIOF_CLK_ENABLE(); 00305 __HAL_RCC_GPIOG_CLK_ENABLE(); 00306 __HAL_RCC_GPIOD_CLK_ENABLE(); 00307 __HAL_RCC_GPIOE_CLK_ENABLE(); 00308 00309 /* Common GPIO configuration */ 00310 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00311 gpio_init_structure.Pull = GPIO_PULLUP; 00312 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00313 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00314 00315 /* GPIOD configuration: GPIO_PIN_7 is FMC_NE1 , GPIO_PIN_11 ans GPIO_PIN_12 are PSRAM_A16 and PSRAM_A17 */ 00316 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00317 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15; 00318 00319 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00320 00321 /* GPIOE configuration */ 00322 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 |\ 00323 GPIO_PIN_12 |GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00324 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00325 00326 /* GPIOF configuration */ 00327 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00328 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00329 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00330 00331 /* GPIOG configuration */ 00332 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00333 GPIO_PIN_5; 00334 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00335 00336 /* Configure common DMA parameters */ 00337 dma_handle.Init.Channel = PSRAM_DMAx_CHANNEL; 00338 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00339 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00340 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00341 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00342 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00343 dma_handle.Init.Mode = DMA_NORMAL; 00344 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00345 dma_handle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00346 dma_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00347 dma_handle.Init.MemBurst = DMA_MBURST_SINGLE; 00348 dma_handle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00349 00350 dma_handle.Instance = PSRAM_DMAx_STREAM; 00351 00352 /* Associate the DMA handle */ 00353 __HAL_LINKDMA(hsram, hdma, dma_handle); 00354 00355 /* Deinitialize the Stream for new transfer */ 00356 HAL_DMA_DeInit(&dma_handle); 00357 00358 /* Configure the DMA Stream */ 00359 HAL_DMA_Init(&dma_handle); 00360 00361 /* NVIC configuration for DMA transfer complete interrupt */ 00362 HAL_NVIC_SetPriority(PSRAM_DMAx_IRQn, 0x0F, 0); 00363 HAL_NVIC_EnableIRQ(PSRAM_DMAx_IRQn); 00364 } 00365 00366 00367 /** 00368 * @brief DeInitializes SRAM MSP. 00369 * @param hsram: SRAM handle 00370 * @param Params 00371 * @retval None 00372 */ 00373 __weak void BSP_PSRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params) 00374 { 00375 static DMA_HandleTypeDef dma_handle; 00376 00377 /* Disable NVIC configuration for DMA interrupt */ 00378 HAL_NVIC_DisableIRQ(PSRAM_DMAx_IRQn); 00379 00380 /* Deinitialize the stream for new transfer */ 00381 dma_handle.Instance = PSRAM_DMAx_STREAM; 00382 HAL_DMA_DeInit(&dma_handle); 00383 00384 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the applications 00385 by surcharging this __weak function */ 00386 } 00387 /** 00388 * @} 00389 */ 00390 00391 /** 00392 * @} 00393 */ 00394 00395 /** 00396 * @} 00397 */ 00398 00399 /** 00400 * @} 00401 */ 00402 00403 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue May 30 2017 13:59:12 for STM32F723E-Discovery BSP User Manual by
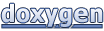