STM32F723E-Discovery BSP User Manual
|
stm32f723e_discovery_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f723e_discovery_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00006 * mounted on STM32F723E-DISCOVERY board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 00038 /* File Info : ----------------------------------------------------------------- 00039 User NOTES 00040 1. How To use this driver: 00041 -------------------------- 00042 - This driver is used to drive indirectly an LCD TFT. 00043 - This driver supports the ST7789H2 LCD. 00044 - The ST7789H2 component driver MUST be included with this driver. 00045 00046 2. Driver description: 00047 --------------------- 00048 + Initialization steps: 00049 o Initialize the LCD using the BSP_LCD_Init() function. 00050 00051 + Display on LCD 00052 o Clear the hole LCD using BSP_LCD_Clear() function or only one specified string 00053 line using the BSP_LCD_ClearStringLine() function. 00054 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00055 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00056 o Display a string line on the specified position (x,y in pixel) and align mode 00057 using the BSP_LCD_DisplayStringAtLine() function. 00058 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00059 on LCD using the available set of functions. 00060 00061 ------------------------------------------------------------------------------*/ 00062 00063 /* Dependencies 00064 - stm32f723e_discovery.c 00065 - stm32f7xx_hal_gpio.c 00066 - stm32f7xx_hal_sram.c 00067 - stm32f7xx_hal_rcc_ex.h 00068 - st7789h2.c 00069 - fonts.h 00070 - font24.c 00071 - font20.c 00072 - font16.c 00073 - font12.c 00074 - font8.c" 00075 EndDependencies */ 00076 00077 /* Includes ------------------------------------------------------------------*/ 00078 #include "stm32f723e_discovery_lcd.h" 00079 #include "../../../Utilities/Fonts/fonts.h" 00080 #include "../../../Utilities/Fonts/font24.c" 00081 #include "../../../Utilities/Fonts/font20.c" 00082 #include "../../../Utilities/Fonts/font16.c" 00083 #include "../../../Utilities/Fonts/font12.c" 00084 #include "../../../Utilities/Fonts/font8.c" 00085 00086 /** @addtogroup BSP 00087 * @{ 00088 */ 00089 00090 /** @addtogroup STM32F723E_DISCOVERY 00091 * @{ 00092 */ 00093 00094 /** @defgroup STM32F723E_DISCOVERY_LCD STM32F723E-DISCOVERY LCD 00095 * @{ 00096 */ 00097 00098 /** @defgroup STM32F723E_DISCOVERY_LCD_Private_TypesDefinitions STM32F723E Discovery Lcd Private TypesDef 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32F723E_DISCOVERY_LCD_Private_Defines STM32F723E Discovery Lcd Private Defines 00106 * @{ 00107 */ 00108 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00109 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32F723E_DISCOVERY_LCD_Private_Macros STM32F723E Discovery Lcd Private Macros 00115 * @{ 00116 */ 00117 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32F723E_DISCOVERY_LCD_Private_Variables STM32F723E Discovery Lcd Private Variables 00123 * @{ 00124 */ 00125 LCD_DrawPropTypeDef DrawProp; 00126 static LCD_DrvTypeDef *LcdDrv; 00127 00128 /** 00129 * @} 00130 */ 00131 00132 /** @defgroup STM32F723E_DISCOVERY_LCD_Private_FunctionPrototypes STM32F723E Discovery Lcd Private Prototypes 00133 * @{ 00134 */ 00135 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00136 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00137 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00138 /** 00139 * @} 00140 */ 00141 00142 /** @defgroup STM32F723E_DISCOVERY_LCD_Private_Functions STM32F723E Discovery Lcd Private Functions 00143 * @{ 00144 */ 00145 /** 00146 * @brief Initializes the LCD. 00147 * @retval LCD state 00148 */ 00149 uint8_t BSP_LCD_Init(void) 00150 { 00151 return (BSP_LCD_InitEx(LCD_ORIENTATION_LANDSCAPE)); 00152 } 00153 /** 00154 * @brief Initializes the LCD with a given orientation. 00155 * @param orientation: LCD_ORIENTATION_PORTRAIT or LCD_ORIENTATION_LANDSCAPE 00156 * @retval LCD state 00157 */ 00158 uint8_t BSP_LCD_InitEx(uint32_t orientation) 00159 { 00160 uint8_t ret = LCD_ERROR; 00161 00162 /* Default value for draw propriety */ 00163 DrawProp.BackColor = 0xFFFF; 00164 DrawProp.pFont = &Font24; 00165 DrawProp.TextColor = 0x0000; 00166 00167 /* Initialize LCD special pins GPIOs */ 00168 BSP_LCD_MspInit(); 00169 00170 /* Backlight control signal assertion */ 00171 HAL_GPIO_WritePin(LCD_BL_CTRL_GPIO_PORT, LCD_BL_CTRL_PIN, GPIO_PIN_SET); 00172 00173 /* Apply hardware reset according to procedure indicated in FRD154BP2901 documentation */ 00174 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_RESET); 00175 HAL_Delay(5); /* Reset signal asserted during 5ms */ 00176 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_SET); 00177 HAL_Delay(10); /* Reset signal released during 10ms */ 00178 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_RESET); 00179 HAL_Delay(20); /* Reset signal asserted during 20ms */ 00180 HAL_GPIO_WritePin(LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_SET); 00181 HAL_Delay(10); /* Reset signal released during 10ms */ 00182 00183 if(ST7789H2_drv.ReadID() == ST7789H2_ID) 00184 { 00185 LcdDrv = &ST7789H2_drv; 00186 00187 /* LCD Init */ 00188 LcdDrv->Init(); 00189 00190 if(orientation == LCD_ORIENTATION_PORTRAIT) 00191 { 00192 ST7789H2_SetOrientation(LCD_ORIENTATION_PORTRAIT); 00193 } 00194 else if(orientation == LCD_ORIENTATION_LANDSCAPE_ROT180) 00195 { 00196 ST7789H2_SetOrientation(LCD_ORIENTATION_LANDSCAPE_ROT180); 00197 } 00198 else 00199 { 00200 /* Default landscape orientation is selected */ 00201 } 00202 /* Initialize the font */ 00203 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00204 00205 ret = LCD_OK; 00206 } 00207 00208 return ret; 00209 } 00210 00211 /** 00212 * @brief DeInitializes the LCD. 00213 * @retval LCD state 00214 */ 00215 uint8_t BSP_LCD_DeInit(void) 00216 { 00217 /* Actually LcdDrv does not provide a DeInit function */ 00218 return LCD_OK; 00219 } 00220 00221 /** 00222 * @brief Gets the LCD X size. 00223 * @retval Used LCD X size 00224 */ 00225 uint32_t BSP_LCD_GetXSize(void) 00226 { 00227 return(LcdDrv->GetLcdPixelWidth()); 00228 } 00229 00230 /** 00231 * @brief Gets the LCD Y size. 00232 * @retval Used LCD Y size 00233 */ 00234 uint32_t BSP_LCD_GetYSize(void) 00235 { 00236 return(LcdDrv->GetLcdPixelHeight()); 00237 } 00238 00239 /** 00240 * @brief Gets the LCD text color. 00241 * @retval Used text color. 00242 */ 00243 uint16_t BSP_LCD_GetTextColor(void) 00244 { 00245 return DrawProp.TextColor; 00246 } 00247 00248 /** 00249 * @brief Gets the LCD background color. 00250 * @retval Used background color 00251 */ 00252 uint16_t BSP_LCD_GetBackColor(void) 00253 { 00254 return DrawProp.BackColor; 00255 } 00256 00257 /** 00258 * @brief Sets the LCD text color. 00259 * @param Color: Text color code RGB(5-6-5) 00260 * @retval None 00261 */ 00262 void BSP_LCD_SetTextColor(uint16_t Color) 00263 { 00264 DrawProp.TextColor = Color; 00265 } 00266 00267 /** 00268 * @brief Sets the LCD background color. 00269 * @param Color: Background color code RGB(5-6-5) 00270 * @retval None 00271 */ 00272 void BSP_LCD_SetBackColor(uint16_t Color) 00273 { 00274 DrawProp.BackColor = Color; 00275 } 00276 00277 /** 00278 * @brief Sets the LCD text font. 00279 * @param fonts: Font to be used 00280 * @retval None 00281 */ 00282 void BSP_LCD_SetFont(sFONT *fonts) 00283 { 00284 DrawProp.pFont = fonts; 00285 } 00286 00287 /** 00288 * @brief Gets the LCD text font. 00289 * @retval Used font 00290 */ 00291 sFONT *BSP_LCD_GetFont(void) 00292 { 00293 return DrawProp.pFont; 00294 } 00295 00296 /** 00297 * @brief Clears the hole LCD. 00298 * @param Color: Color of the background 00299 * @retval None 00300 */ 00301 void BSP_LCD_Clear(uint16_t Color) 00302 { 00303 uint32_t counter = 0; 00304 uint32_t y_size = 0; 00305 uint32_t color_backup = DrawProp.TextColor; 00306 00307 DrawProp.TextColor = Color; 00308 y_size = BSP_LCD_GetYSize(); 00309 00310 for(counter = 0; counter < y_size; counter++) 00311 { 00312 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00313 } 00314 DrawProp.TextColor = color_backup; 00315 BSP_LCD_SetTextColor(DrawProp.TextColor); 00316 } 00317 00318 /** 00319 * @brief Clears the selected line. 00320 * @param Line: Line to be cleared 00321 * This parameter can be one of the following values: 00322 * @arg 0..9: if the Current fonts is Font16x24 00323 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00324 * @arg 0..29: if the Current fonts is Font8x8 00325 * @retval None 00326 */ 00327 void BSP_LCD_ClearStringLine(uint16_t Line) 00328 { 00329 uint32_t color_backup = DrawProp.TextColor; 00330 00331 DrawProp.TextColor = DrawProp.BackColor; 00332 00333 /* Draw a rectangle with background color */ 00334 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00335 00336 DrawProp.TextColor = color_backup; 00337 BSP_LCD_SetTextColor(DrawProp.TextColor); 00338 } 00339 00340 /** 00341 * @brief Displays one character. 00342 * @param Xpos: Start column address 00343 * @param Ypos: Line where to display the character shape. 00344 * @param Ascii: Character ascii code 00345 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00346 * @retval None 00347 */ 00348 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00349 { 00350 DrawChar(Xpos, Ypos, &DrawProp.pFont->table[(Ascii-' ') *\ 00351 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00352 } 00353 00354 /** 00355 * @brief Displays characters on the LCD. 00356 * @param Xpos: X position (in pixel) 00357 * @param Ypos: Y position (in pixel) 00358 * @param Text: Pointer to string to display on LCD 00359 * @param Mode: Display mode 00360 * This parameter can be one of the following values: 00361 * @arg CENTER_MODE 00362 * @arg RIGHT_MODE 00363 * @arg LEFT_MODE 00364 * @retval None 00365 */ 00366 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode) 00367 { 00368 uint16_t refcolumn = 1, i = 0; 00369 uint32_t size = 0, xsize = 0; 00370 uint8_t *ptr = Text; 00371 00372 /* Get the text size */ 00373 while (*ptr++) size ++ ; 00374 00375 /* Characters number per line */ 00376 xsize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00377 00378 switch (Mode) 00379 { 00380 case CENTER_MODE: 00381 { 00382 refcolumn = Xpos + ((xsize - size)* DrawProp.pFont->Width) / 2; 00383 break; 00384 } 00385 case LEFT_MODE: 00386 { 00387 refcolumn = Xpos; 00388 break; 00389 } 00390 case RIGHT_MODE: 00391 { 00392 refcolumn = - Xpos + ((xsize - size)*DrawProp.pFont->Width); 00393 break; 00394 } 00395 default: 00396 { 00397 refcolumn = Xpos; 00398 break; 00399 } 00400 } 00401 00402 /* Check that the Start column is located in the screen */ 00403 if ((refcolumn < 1) || (refcolumn >= 0x8000)) 00404 { 00405 refcolumn = 1; 00406 } 00407 00408 /* Send the string character by character on lCD */ 00409 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00410 { 00411 /* Display one character on LCD */ 00412 BSP_LCD_DisplayChar(refcolumn, Ypos, *Text); 00413 /* Decrement the column position by 16 */ 00414 refcolumn += DrawProp.pFont->Width; 00415 /* Point on the next character */ 00416 Text++; 00417 i++; 00418 } 00419 } 00420 00421 /** 00422 * @brief Displays a character on the LCD. 00423 * @param Line: Line where to display the character shape 00424 * This parameter can be one of the following values: 00425 * @arg 0..9: if the Current fonts is Font16x24 00426 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00427 * @arg 0..29: if the Current fonts is Font8x8 00428 * @param ptr: Pointer to string to display on LCD 00429 * @retval None 00430 */ 00431 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00432 { 00433 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00434 } 00435 00436 /** 00437 * @brief Reads an LCD pixel. 00438 * @param Xpos: X position 00439 * @param Ypos: Y position 00440 * @retval RGB pixel color 00441 */ 00442 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00443 { 00444 uint16_t ret = 0; 00445 00446 if(LcdDrv->ReadPixel != NULL) 00447 { 00448 ret = LcdDrv->ReadPixel(Xpos, Ypos); 00449 } 00450 00451 return ret; 00452 } 00453 00454 /** 00455 * @brief Draws a pixel on LCD. 00456 * @param Xpos: X position 00457 * @param Ypos: Y position 00458 * @param RGB_Code: Pixel color in RGB mode (5-6-5) 00459 * @retval None 00460 */ 00461 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code) 00462 { 00463 if(LcdDrv->WritePixel != NULL) 00464 { 00465 LcdDrv->WritePixel(Xpos, Ypos, RGB_Code); 00466 } 00467 } 00468 00469 /** 00470 * @brief Draws an horizontal line. 00471 * @param Xpos: X position 00472 * @param Ypos: Y position 00473 * @param Length: Line length 00474 * @retval None 00475 */ 00476 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00477 { 00478 uint32_t index = 0; 00479 00480 if(LcdDrv->DrawHLine != NULL) 00481 { 00482 LcdDrv->DrawHLine(DrawProp.TextColor, Xpos, Ypos, Length); 00483 } 00484 else 00485 { 00486 for(index = 0; index < Length; index++) 00487 { 00488 BSP_LCD_DrawPixel((Xpos + index), Ypos, DrawProp.TextColor); 00489 } 00490 } 00491 } 00492 00493 /** 00494 * @brief Draws a vertical line. 00495 * @param Xpos: X position 00496 * @param Ypos: Y position 00497 * @param Length: Line length 00498 * @retval None 00499 */ 00500 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00501 { 00502 uint32_t index = 0; 00503 00504 if(LcdDrv->DrawVLine != NULL) 00505 { 00506 LcdDrv->DrawVLine(DrawProp.TextColor, Xpos, Ypos, Length); 00507 } 00508 else 00509 { 00510 for(index = 0; index < Length; index++) 00511 { 00512 BSP_LCD_DrawPixel(Xpos, Ypos + index, DrawProp.TextColor); 00513 } 00514 } 00515 } 00516 00517 /** 00518 * @brief Draws an uni-line (between two points). 00519 * @param x1: Point 1 X position 00520 * @param y1: Point 1 Y position 00521 * @param x2: Point 2 X position 00522 * @param y2: Point 2 Y position 00523 * @retval None 00524 */ 00525 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00526 { 00527 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00528 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00529 curpixel = 0; 00530 00531 deltax = ABS(x2 - x1); /* The difference between the x's */ 00532 deltay = ABS(y2 - y1); /* The difference between the y's */ 00533 x = x1; /* Start x off at the first pixel */ 00534 y = y1; /* Start y off at the first pixel */ 00535 00536 if (x2 >= x1) /* The x-values are increasing */ 00537 { 00538 xinc1 = 1; 00539 xinc2 = 1; 00540 } 00541 else /* The x-values are decreasing */ 00542 { 00543 xinc1 = -1; 00544 xinc2 = -1; 00545 } 00546 00547 if (y2 >= y1) /* The y-values are increasing */ 00548 { 00549 yinc1 = 1; 00550 yinc2 = 1; 00551 } 00552 else /* The y-values are decreasing */ 00553 { 00554 yinc1 = -1; 00555 yinc2 = -1; 00556 } 00557 00558 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00559 { 00560 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00561 yinc2 = 0; /* Don't change the y for every iteration */ 00562 den = deltax; 00563 num = deltax / 2; 00564 numadd = deltay; 00565 numpixels = deltax; /* There are more x-values than y-values */ 00566 } 00567 else /* There is at least one y-value for every x-value */ 00568 { 00569 xinc2 = 0; /* Don't change the x for every iteration */ 00570 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00571 den = deltay; 00572 num = deltay / 2; 00573 numadd = deltax; 00574 numpixels = deltay; /* There are more y-values than x-values */ 00575 } 00576 00577 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00578 { 00579 BSP_LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00580 num += numadd; /* Increase the numerator by the top of the fraction */ 00581 if (num >= den) /* Check if numerator >= denominator */ 00582 { 00583 num -= den; /* Calculate the new numerator value */ 00584 x += xinc1; /* Change the x as appropriate */ 00585 y += yinc1; /* Change the y as appropriate */ 00586 } 00587 x += xinc2; /* Change the x as appropriate */ 00588 y += yinc2; /* Change the y as appropriate */ 00589 } 00590 } 00591 00592 /** 00593 * @brief Draws a rectangle. 00594 * @param Xpos: X position 00595 * @param Ypos: Y position 00596 * @param Width: Rectangle width 00597 * @param Height: Rectangle height 00598 * @retval None 00599 */ 00600 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00601 { 00602 /* Draw horizontal lines */ 00603 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00604 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00605 00606 /* Draw vertical lines */ 00607 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00608 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00609 } 00610 00611 /** 00612 * @brief Draws a circle. 00613 * @param Xpos: X position 00614 * @param Ypos: Y position 00615 * @param Radius: Circle radius 00616 * @retval None 00617 */ 00618 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00619 { 00620 int32_t decision; /* Decision Variable */ 00621 uint32_t current_x; /* Current X Value */ 00622 uint32_t current_y; /* Current Y Value */ 00623 00624 decision = 3 - (Radius << 1); 00625 current_x = 0; 00626 current_y = Radius; 00627 00628 while (current_x <= current_y) 00629 { 00630 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp.TextColor); 00631 00632 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp.TextColor); 00633 00634 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp.TextColor); 00635 00636 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp.TextColor); 00637 00638 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp.TextColor); 00639 00640 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp.TextColor); 00641 00642 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp.TextColor); 00643 00644 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp.TextColor); 00645 00646 /* Initialize the font */ 00647 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00648 00649 if (decision < 0) 00650 { 00651 decision += (current_x << 2) + 6; 00652 } 00653 else 00654 { 00655 decision += ((current_x - current_y) << 2) + 10; 00656 current_y--; 00657 } 00658 current_x++; 00659 } 00660 } 00661 00662 /** 00663 * @brief Draws an poly-line (between many points). 00664 * @param Points: Pointer to the points array 00665 * @param PointCount: Number of points 00666 * @retval None 00667 */ 00668 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00669 { 00670 int16_t x = 0, y = 0; 00671 00672 if(PointCount < 2) 00673 { 00674 return; 00675 } 00676 00677 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00678 00679 while(--PointCount) 00680 { 00681 x = Points->X; 00682 y = Points->Y; 00683 Points++; 00684 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00685 } 00686 } 00687 00688 /** 00689 * @brief Draws an ellipse on LCD. 00690 * @param Xpos: X position 00691 * @param Ypos: Y position 00692 * @param XRadius: Ellipse X radius 00693 * @param YRadius: Ellipse Y radius 00694 * @retval None 00695 */ 00696 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00697 { 00698 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00699 float k = 0, rad1 = 0, rad2 = 0; 00700 00701 rad1 = XRadius; 00702 rad2 = YRadius; 00703 00704 k = (float)(rad2/rad1); 00705 00706 do { 00707 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00708 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00709 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00710 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00711 00712 e2 = err; 00713 if (e2 <= x) { 00714 err += ++x*2+1; 00715 if (-y == x && e2 <= y) e2 = 0; 00716 } 00717 if (e2 > y) err += ++y*2+1; 00718 } 00719 while (y <= 0); 00720 } 00721 00722 /** 00723 * @brief Draws a bitmap picture (16 bpp). 00724 * @param Xpos: Bmp X position in the LCD 00725 * @param Ypos: Bmp Y position in the LCD 00726 * @param pbmp: Pointer to Bmp picture address. 00727 * @retval None 00728 */ 00729 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00730 { 00731 uint32_t height = 0; 00732 uint32_t width = 0; 00733 00734 00735 /* Read bitmap width */ 00736 width = *(uint16_t *) (pbmp + 18); 00737 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00738 00739 /* Read bitmap height */ 00740 height = *(uint16_t *) (pbmp + 22); 00741 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00742 00743 SetDisplayWindow(Xpos, Ypos, width, height); 00744 00745 if(LcdDrv->DrawBitmap != NULL) 00746 { 00747 LcdDrv->DrawBitmap(Xpos, Ypos, pbmp); 00748 } 00749 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00750 } 00751 00752 /** 00753 * @brief Draws RGB Image (16 bpp). 00754 * @param Xpos: X position in the LCD 00755 * @param Ypos: Y position in the LCD 00756 * @param Xsize: X size in the LCD 00757 * @param Ysize: Y size in the LCD 00758 * @param pdata: Pointer to the RGB Image address. 00759 * @retval None 00760 */ 00761 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00762 { 00763 00764 SetDisplayWindow(Xpos, Ypos, Xsize, Ysize); 00765 00766 if(LcdDrv->DrawRGBImage != NULL) 00767 { 00768 LcdDrv->DrawRGBImage(Xpos, Ypos, Xsize, Ysize, pdata); 00769 } 00770 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00771 } 00772 00773 /** 00774 * @brief Draws a full rectangle. 00775 * @param Xpos: X position 00776 * @param Ypos: Y position 00777 * @param Width: Rectangle width 00778 * @param Height: Rectangle height 00779 * @retval None 00780 */ 00781 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00782 { 00783 BSP_LCD_SetTextColor(DrawProp.TextColor); 00784 do 00785 { 00786 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00787 } 00788 while(Height--); 00789 } 00790 00791 /** 00792 * @brief Draws a full circle. 00793 * @param Xpos: X position 00794 * @param Ypos: Y position 00795 * @param Radius: Circle radius 00796 * @retval None 00797 */ 00798 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00799 { 00800 int32_t decision; /* Decision Variable */ 00801 uint32_t current_x; /* Current X Value */ 00802 uint32_t current_y; /* Current Y Value */ 00803 00804 decision = 3 - (Radius << 1); 00805 00806 current_x = 0; 00807 current_y = Radius; 00808 00809 BSP_LCD_SetTextColor(DrawProp.TextColor); 00810 00811 while (current_x <= current_y) 00812 { 00813 if(current_y > 0) 00814 { 00815 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 00816 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 00817 } 00818 00819 if(current_x > 0) 00820 { 00821 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 00822 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 00823 } 00824 if (decision < 0) 00825 { 00826 decision += (current_x << 2) + 6; 00827 } 00828 else 00829 { 00830 decision += ((current_x - current_y) << 2) + 10; 00831 current_y--; 00832 } 00833 current_x++; 00834 } 00835 00836 BSP_LCD_SetTextColor(DrawProp.TextColor); 00837 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00838 } 00839 00840 /** 00841 * @brief Draws a full poly-line (between many points). 00842 * @param Points: Pointer to the points array 00843 * @param PointCount: Number of points 00844 * @retval None 00845 */ 00846 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 00847 { 00848 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 00849 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 00850 00851 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 00852 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 00853 00854 for(counter = 1; counter < PointCount; counter++) 00855 { 00856 pixelX = POLY_X(counter); 00857 if(pixelX < IMAGE_LEFT) 00858 { 00859 IMAGE_LEFT = pixelX; 00860 } 00861 if(pixelX > IMAGE_RIGHT) 00862 { 00863 IMAGE_RIGHT = pixelX; 00864 } 00865 00866 pixelY = POLY_Y(counter); 00867 if(pixelY < IMAGE_TOP) 00868 { 00869 IMAGE_TOP = pixelY; 00870 } 00871 if(pixelY > IMAGE_BOTTOM) 00872 { 00873 IMAGE_BOTTOM = pixelY; 00874 } 00875 } 00876 00877 if(PointCount < 2) 00878 { 00879 return; 00880 } 00881 00882 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 00883 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 00884 00885 X_first = Points->X; 00886 Y_first = Points->Y; 00887 00888 while(--PointCount) 00889 { 00890 X = Points->X; 00891 Y = Points->Y; 00892 Points++; 00893 X2 = Points->X; 00894 Y2 = Points->Y; 00895 00896 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 00897 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 00898 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 00899 } 00900 00901 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 00902 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 00903 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 00904 } 00905 00906 /** 00907 * @brief Draws a full ellipse. 00908 * @param Xpos: X position 00909 * @param Ypos: Y position 00910 * @param XRadius: Ellipse X radius 00911 * @param YRadius: Ellipse Y radius 00912 * @retval None 00913 */ 00914 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00915 { 00916 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00917 float k = 0, rad1 = 0, rad2 = 0; 00918 00919 rad1 = XRadius; 00920 rad2 = YRadius; 00921 00922 k = (float)(rad2/rad1); 00923 00924 do 00925 { 00926 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 00927 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 00928 00929 e2 = err; 00930 if (e2 <= x) 00931 { 00932 err += ++x*2+1; 00933 if (-y == x && e2 <= y) e2 = 0; 00934 } 00935 if (e2 > y) err += ++y*2+1; 00936 } 00937 while (y <= 0); 00938 } 00939 00940 /** 00941 * @brief Enables the display. 00942 * @retval None 00943 */ 00944 void BSP_LCD_DisplayOn(void) 00945 { 00946 LcdDrv->DisplayOn(); 00947 } 00948 00949 /** 00950 * @brief Disables the display. 00951 * @retval None 00952 */ 00953 void BSP_LCD_DisplayOff(void) 00954 { 00955 LcdDrv->DisplayOff(); 00956 } 00957 00958 00959 /** 00960 * @brief Initializes the LCD GPIO special pins MSP. 00961 * @retval None 00962 */ 00963 __weak void BSP_LCD_MspInit(void) 00964 { 00965 GPIO_InitTypeDef gpio_init_structure; 00966 00967 /* Enable GPIOs clock */ 00968 LCD_RESET_GPIO_CLK_ENABLE(); 00969 LCD_TE_GPIO_CLK_ENABLE(); 00970 LCD_BL_CTRL_GPIO_CLK_ENABLE(); 00971 00972 /* LCD_RESET GPIO configuration */ 00973 gpio_init_structure.Pin = LCD_RESET_PIN; /* LCD_RESET pin has to be manually controlled */ 00974 gpio_init_structure.Pull = GPIO_NOPULL; 00975 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00976 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00977 HAL_GPIO_Init(LCD_RESET_GPIO_PORT, &gpio_init_structure); 00978 HAL_GPIO_WritePin( LCD_RESET_GPIO_PORT, LCD_RESET_PIN, GPIO_PIN_RESET); 00979 00980 /* LCD_TE GPIO configuration */ 00981 gpio_init_structure.Pin = LCD_TE_PIN; /* LCD_TE pin has to be manually managed */ 00982 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00983 HAL_GPIO_Init(LCD_TE_GPIO_PORT, &gpio_init_structure); 00984 00985 /* LCD_BL_CTRL GPIO configuration */ 00986 gpio_init_structure.Pin = LCD_BL_CTRL_PIN; /* LCD_BL_CTRL pin has to be manually controlled */ 00987 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00988 gpio_init_structure.Speed = GPIO_SPEED_FREQ_LOW; 00989 HAL_GPIO_Init(LCD_BL_CTRL_GPIO_PORT, &gpio_init_structure); 00990 } 00991 00992 /** 00993 * @brief DeInitializes LCD GPIO special pins MSP. 00994 * @retval None 00995 */ 00996 __weak void BSP_LCD_MspDeInit(void) 00997 { 00998 GPIO_InitTypeDef gpio_init_structure; 00999 01000 /* LCD_RESET GPIO deactivation */ 01001 gpio_init_structure.Pin = LCD_RESET_PIN; 01002 HAL_GPIO_DeInit(LCD_RESET_GPIO_PORT, gpio_init_structure.Pin); 01003 01004 /* LCD_TE GPIO deactivation */ 01005 gpio_init_structure.Pin = LCD_TE_PIN; 01006 HAL_GPIO_DeInit(LCD_TE_GPIO_PORT, gpio_init_structure.Pin); 01007 01008 /* LCD_BL_CTRL GPIO deactivation */ 01009 gpio_init_structure.Pin = LCD_BL_CTRL_PIN; 01010 HAL_GPIO_DeInit(LCD_BL_CTRL_GPIO_PORT, gpio_init_structure.Pin); 01011 01012 /* GPIO pins clock can be shut down in the application 01013 by surcharging this __weak function */ 01014 } 01015 01016 /****************************************************************************** 01017 Static Functions 01018 *******************************************************************************/ 01019 01020 /** 01021 * @brief Draws a character on LCD. 01022 * @param Xpos: Line where to display the character shape 01023 * @param Ypos: Start column address 01024 * @param c: Pointer to the character data 01025 * @retval None 01026 */ 01027 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01028 { 01029 uint32_t i = 0, j = 0; 01030 uint16_t height, width; 01031 uint8_t offset; 01032 uint8_t *pchar; 01033 uint32_t line; 01034 01035 height = DrawProp.pFont->Height; 01036 width = DrawProp.pFont->Width; 01037 01038 offset = 8 *((width + 7)/8) - width ; 01039 01040 for(i = 0; i < height; i++) 01041 { 01042 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01043 01044 switch(((width + 7)/8)) 01045 { 01046 case 1: 01047 line = pchar[0]; 01048 break; 01049 01050 case 2: 01051 line = (pchar[0]<< 8) | pchar[1]; 01052 break; 01053 01054 case 3: 01055 default: 01056 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01057 break; 01058 } 01059 01060 for (j = 0; j < width; j++) 01061 { 01062 if(line & (1 << (width- j + offset- 1))) 01063 { 01064 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.TextColor); 01065 } 01066 else 01067 { 01068 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.BackColor); 01069 } 01070 } 01071 Ypos++; 01072 } 01073 } 01074 01075 /** 01076 * @brief Sets display window. 01077 * @param Xpos: LCD X position 01078 * @param Ypos: LCD Y position 01079 * @param Width: LCD window width 01080 * @param Height: LCD window height 01081 * @retval None 01082 */ 01083 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01084 { 01085 if(LcdDrv->SetDisplayWindow != NULL) 01086 { 01087 LcdDrv->SetDisplayWindow(Xpos, Ypos, Width, Height); 01088 } 01089 } 01090 01091 /** 01092 * @brief Fills a triangle (between 3 points). 01093 * @param x1: Point 1 X position 01094 * @param y1: Point 1 Y position 01095 * @param x2: Point 2 X position 01096 * @param y2: Point 2 Y position 01097 * @param x3: Point 3 X position 01098 * @param y3: Point 3 Y position 01099 * @retval None 01100 */ 01101 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01102 { 01103 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01104 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01105 curpixel = 0; 01106 01107 deltax = ABS(x2 - x1); /* The difference between the x's */ 01108 deltay = ABS(y2 - y1); /* The difference between the y's */ 01109 x = x1; /* Start x off at the first pixel */ 01110 y = y1; /* Start y off at the first pixel */ 01111 01112 if (x2 >= x1) /* The x-values are increasing */ 01113 { 01114 xinc1 = 1; 01115 xinc2 = 1; 01116 } 01117 else /* The x-values are decreasing */ 01118 { 01119 xinc1 = -1; 01120 xinc2 = -1; 01121 } 01122 01123 if (y2 >= y1) /* The y-values are increasing */ 01124 { 01125 yinc1 = 1; 01126 yinc2 = 1; 01127 } 01128 else /* The y-values are decreasing */ 01129 { 01130 yinc1 = -1; 01131 yinc2 = -1; 01132 } 01133 01134 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01135 { 01136 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01137 yinc2 = 0; /* Don't change the y for every iteration */ 01138 den = deltax; 01139 num = deltax / 2; 01140 numadd = deltay; 01141 numpixels = deltax; /* There are more x-values than y-values */ 01142 } 01143 else /* There is at least one y-value for every x-value */ 01144 { 01145 xinc2 = 0; /* Don't change the x for every iteration */ 01146 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01147 den = deltay; 01148 num = deltay / 2; 01149 numadd = deltax; 01150 numpixels = deltay; /* There are more y-values than x-values */ 01151 } 01152 01153 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01154 { 01155 BSP_LCD_DrawLine(x, y, x3, y3); 01156 01157 num += numadd; /* Increase the numerator by the top of the fraction */ 01158 if (num >= den) /* Check if numerator >= denominator */ 01159 { 01160 num -= den; /* Calculate the new numerator value */ 01161 x += xinc1; /* Change the x as appropriate */ 01162 y += yinc1; /* Change the y as appropriate */ 01163 } 01164 x += xinc2; /* Change the x as appropriate */ 01165 y += yinc2; /* Change the y as appropriate */ 01166 } 01167 } 01168 01169 /** 01170 * @} 01171 */ 01172 01173 /** 01174 * @} 01175 */ 01176 01177 /** 01178 * @} 01179 */ 01180 01181 /** 01182 * @} 01183 */ 01184 01185 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue May 30 2017 13:59:12 for STM32F723E-Discovery BSP User Manual by
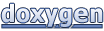