STM32F723E-Discovery BSP User Manual
|
stm32f723e_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f723e_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage LEDs, 00006 * push-buttons, external PSRAM, external QSPI Flash, TS available on 00007 * STM32F723E-Discovery board (MB1260) from STMicroelectronics. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Dependencies 00039 - stm32f7xx_hal_cortex.c 00040 - stm32f7xx_hal_gpio.c 00041 - stm32f7xx_hal_uart.c 00042 - stm32f7xx_hal_i2c.c 00043 - stm32f7xx_hal_sram.c 00044 - stm32f7xx_hal_rcc_ex.c 00045 EndDependencies */ 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f723e_discovery.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F723E_DISCOVERY 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL STM32F723E-DISCOVERY LOW LEVEL 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_TypesDefinitions STM32F723E Discovery Low Level Private Typedef 00063 * @{ 00064 */ 00065 typedef struct 00066 { 00067 __IO uint16_t REG; 00068 __IO uint16_t RAM; 00069 }LCD_CONTROLLER_TypeDef; 00070 /** 00071 * @} 00072 */ 00073 00074 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Defines LOW_LEVEL Private Defines 00075 * @{ 00076 */ 00077 /** 00078 * @brief STM32F723E Discovery BSP Driver version number V1.0.1 00079 */ 00080 #define __STM32F723E_DISCOVERY_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00081 #define __STM32F723E_DISCOVERY_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00082 #define __STM32F723E_DISCOVERY_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00083 #define __STM32F723E_DISCOVERY_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00084 #define __STM32F723E_DISCOVERY_BSP_VERSION ((__STM32F723E_DISCOVERY_BSP_VERSION_MAIN << 24)\ 00085 |(__STM32F723E_DISCOVERY_BSP_VERSION_SUB1 << 16)\ 00086 |(__STM32F723E_DISCOVERY_BSP_VERSION_SUB2 << 8 )\ 00087 |(__STM32F723E_DISCOVERY_BSP_VERSION_RC)) 00088 00089 /* We use BANK2 as we use FMC_NE2 signal */ 00090 #define FMC_BANK2_BASE ((uint32_t)(0x60000000 | 0x04000000)) 00091 #define FMC_BANK2 ((LCD_CONTROLLER_TypeDef *) FMC_BANK2_BASE) 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Macros LOW_LEVEL Private Macros 00097 * @{ 00098 */ 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Variables LOW_LEVEL Private Variables 00104 * @{ 00105 */ 00106 uint32_t GPIO_PIN[LEDn] = {LED5_PIN, 00107 LED6_PIN}; 00108 00109 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED5_GPIO_PORT, 00110 LED6_GPIO_PORT}; 00111 00112 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT }; 00113 00114 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN }; 00115 00116 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn }; 00117 00118 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00119 00120 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00121 00122 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00123 00124 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00125 00126 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00127 00128 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00129 00130 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00131 00132 static I2C_HandleTypeDef hI2cAudioHandler = {0}; 00133 static I2C_HandleTypeDef hI2cTsHandler = {0}; 00134 00135 /** 00136 * @} 00137 */ 00138 00139 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_FunctionPrototypes LOW_LEVEL Private FunctionPrototypes 00140 * @{ 00141 */ 00142 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00143 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00144 00145 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00146 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00147 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00148 00149 static void FMC_BANK2_WriteData(uint16_t Data); 00150 static void FMC_BANK2_WriteReg(uint8_t Reg); 00151 static uint16_t FMC_BANK2_ReadData(void); 00152 static void FMC_BANK2_Init(void); 00153 static void FMC_BANK2_MspInit(void); 00154 00155 /* LCD IO functions */ 00156 void LCD_IO_Init(void); 00157 void LCD_IO_WriteData(uint16_t RegValue); 00158 void LCD_IO_WriteReg(uint8_t Reg); 00159 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size); 00160 uint16_t LCD_IO_ReadData(void); 00161 void LCD_IO_Delay(uint32_t Delay); 00162 00163 /* AUDIO IO functions */ 00164 void AUDIO_IO_Init(void); 00165 void AUDIO_IO_DeInit(void); 00166 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00167 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00168 void AUDIO_IO_Delay(uint32_t Delay); 00169 00170 /* TouchScreen (TS) IO functions */ 00171 void TS_IO_Init(void); 00172 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00173 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00174 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00175 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00176 void TS_IO_Delay(uint32_t Delay); 00177 00178 /** 00179 * @} 00180 */ 00181 00182 /** @defgroup STM32F723E_DISCOVERY_BSP_Public_Functions BSP Public Functions 00183 * @{ 00184 */ 00185 00186 /** 00187 * @brief This method returns the STM32F723E Discovery BSP Driver revision 00188 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00189 */ 00190 uint32_t BSP_GetVersion(void) 00191 { 00192 return __STM32F723E_DISCOVERY_BSP_VERSION; 00193 } 00194 00195 /** 00196 * @brief Configures LED GPIO. 00197 * @param Led: LED to be configured. 00198 * This parameter can be one of the following values: 00199 * @arg LED5 00200 * @arg LED6 00201 * @retval None 00202 */ 00203 void BSP_LED_Init(Led_TypeDef Led) 00204 { 00205 GPIO_InitTypeDef gpio_init_structure; 00206 00207 LEDx_GPIO_CLK_ENABLE(Led); 00208 /* Configure the GPIO_LED pin */ 00209 gpio_init_structure.Pin = GPIO_PIN[Led]; 00210 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00211 gpio_init_structure.Pull = GPIO_PULLUP; 00212 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00213 00214 HAL_GPIO_Init(GPIO_PORT[Led], &gpio_init_structure); 00215 00216 } 00217 00218 00219 /** 00220 * @brief DeInit LEDs. 00221 * @param Led: LED to be configured. 00222 * This parameter can be one of the following values: 00223 * @arg LED5 00224 * @arg LED6 00225 * @note Led DeInit does not disable the GPIO clock 00226 * @retval None 00227 */ 00228 void BSP_LED_DeInit(Led_TypeDef Led) 00229 { 00230 GPIO_InitTypeDef gpio_init_structure; 00231 00232 /* DeInit the GPIO_LED pin */ 00233 gpio_init_structure.Pin = GPIO_PIN[Led]; 00234 00235 /* Turn off LED */ 00236 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00237 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00238 } 00239 00240 /** 00241 * @brief Turns selected LED On. 00242 * @param Led: LED to be set on 00243 * This parameter can be one of the following values: 00244 * @arg LED5 00245 * @arg LED6 00246 * @retval None 00247 */ 00248 void BSP_LED_On(Led_TypeDef Led) 00249 { 00250 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00251 } 00252 00253 /** 00254 * @brief Turns selected LED Off. 00255 * @param Led: LED to be set off 00256 * This parameter can be one of the following values: 00257 * @arg LED5 00258 * @arg LED6 00259 * @retval None 00260 */ 00261 void BSP_LED_Off(Led_TypeDef Led) 00262 { 00263 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00264 } 00265 00266 /** 00267 * @brief Toggles the selected LED. 00268 * @param Led: LED to be toggled 00269 * This parameter can be one of the following values: 00270 * @arg LED5 00271 * @arg LED6 00272 * @retval None 00273 */ 00274 void BSP_LED_Toggle(Led_TypeDef Led) 00275 { 00276 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00277 } 00278 00279 /** 00280 * @brief Configures button GPIO and EXTI Line. 00281 * @param Button: Button to be configured 00282 * This parameter can be one of the following values: 00283 * @arg BUTTON_WAKEUP: Wakeup Push Button 00284 * @arg BUTTON_USER: User Push Button 00285 * @param Button_Mode: Button mode 00286 * This parameter can be one of the following values: 00287 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00288 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00289 * with interrupt generation capability 00290 * @retval None 00291 */ 00292 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00293 { 00294 GPIO_InitTypeDef gpio_init_structure; 00295 00296 /* Enable the BUTTON clock */ 00297 BUTTON_GPIO_CLK_ENABLE(); 00298 00299 if(Button_Mode == BUTTON_MODE_GPIO) 00300 { 00301 /* Configure Button pin as input */ 00302 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00303 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00304 gpio_init_structure.Pull = GPIO_NOPULL; 00305 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00306 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00307 } 00308 00309 if(Button_Mode == BUTTON_MODE_EXTI) 00310 { 00311 /* Configure Button pin as input with External interrupt */ 00312 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00313 gpio_init_structure.Pull = GPIO_NOPULL; 00314 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00315 00316 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00317 00318 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00319 00320 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00321 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00322 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00323 } 00324 } 00325 00326 /** 00327 * @brief Push Button DeInit. 00328 * @param Button: Button to be configured 00329 * This parameter can be one of the following values: 00330 * @arg BUTTON_WAKEUP: Wakeup Push Button 00331 * @arg BUTTON_USER: User Push Button 00332 * @note PB DeInit does not disable the GPIO clock 00333 * @retval None 00334 */ 00335 void BSP_PB_DeInit(Button_TypeDef Button) 00336 { 00337 GPIO_InitTypeDef gpio_init_structure; 00338 00339 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00340 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00341 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00342 } 00343 00344 00345 /** 00346 * @brief Returns the selected button state. 00347 * @param Button: Button to be checked 00348 * This parameter can be one of the following values: 00349 * @arg BUTTON_WAKEUP: Wakeup Push Button 00350 * @arg BUTTON_USER: User Push Button 00351 * @retval The Button GPIO pin value 00352 */ 00353 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00354 { 00355 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00356 } 00357 00358 /** 00359 * @} 00360 */ 00361 00362 /** @defgroup STM32F723E_DISCOVERY_LOW_LEVEL_Private_Functions STM32F723E_DISCOVERY_LOW_LEVEL Private Functions 00363 * @{ 00364 */ 00365 /** 00366 * @brief Configures COM port. 00367 * @param COM: COM port to be configured. 00368 * This parameter can be one of the following values: 00369 * @arg COM1 00370 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00371 * configuration information for the specified USART peripheral. 00372 * @retval None 00373 */ 00374 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00375 { 00376 GPIO_InitTypeDef gpio_init_structure; 00377 00378 /* Enable GPIO clock */ 00379 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00380 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00381 00382 /* Enable USART clock */ 00383 DISCOVERY_COMx_CLK_ENABLE(COM); 00384 00385 /* Configure USART Tx as alternate function */ 00386 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00387 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00388 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00389 gpio_init_structure.Pull = GPIO_PULLUP; 00390 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00391 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00392 00393 /* Configure USART Rx as alternate function */ 00394 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00395 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00396 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00397 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00398 00399 /* USART configuration */ 00400 huart->Instance = COM_USART[COM]; 00401 HAL_UART_Init(huart); 00402 } 00403 00404 /** 00405 * @brief DeInit COM port. 00406 * @param COM: COM port to be configured. 00407 * This parameter can be one of the following values: 00408 * @arg COM1 00409 * @arg COM2 00410 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00411 * configuration information for the specified USART peripheral. 00412 * @retval None 00413 */ 00414 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00415 { 00416 /* USART configuration */ 00417 huart->Instance = COM_USART[COM]; 00418 HAL_UART_DeInit(huart); 00419 00420 /* Enable USART clock */ 00421 DISCOVERY_COMx_CLK_DISABLE(COM); 00422 00423 /* DeInit GPIO pins can be done in the application 00424 (by surcharging this __weak function) */ 00425 00426 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00427 by surcharging this __weak function */ 00428 } 00429 00430 /******************************************************************************* 00431 BUS OPERATIONS 00432 *******************************************************************************/ 00433 00434 /******************************* I2C Routines *********************************/ 00435 /** 00436 * @brief Initializes I2C MSP. 00437 * @param i2c_handler : I2C handler 00438 * @retval None 00439 */ 00440 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00441 { 00442 GPIO_InitTypeDef gpio_init_structure; 00443 00444 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00445 { 00446 /*** Configure the GPIOs ***/ 00447 /* Enable GPIO clock */ 00448 DISCOVERY_AUDIO_I2Cx_SCL_GPIO_CLK_ENABLE(); 00449 DISCOVERY_AUDIO_I2Cx_SDA_GPIO_CLK_ENABLE(); 00450 /* Configure I2C Tx as alternate function */ 00451 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SCL_PIN; 00452 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00453 gpio_init_structure.Pull = GPIO_NOPULL; 00454 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00455 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SCL_AF; 00456 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SCL_GPIO_PORT, &gpio_init_structure); 00457 00458 /* Configure I2C Rx as alternate function */ 00459 gpio_init_structure.Pin = DISCOVERY_AUDIO_I2Cx_SDA_PIN; 00460 gpio_init_structure.Alternate = DISCOVERY_AUDIO_I2Cx_SDA_AF; 00461 HAL_GPIO_Init(DISCOVERY_AUDIO_I2Cx_SDA_GPIO_PORT, &gpio_init_structure); 00462 00463 /*** Configure the I2C peripheral ***/ 00464 /* Enable I2C clock */ 00465 DISCOVERY_AUDIO_I2Cx_CLK_ENABLE(); 00466 00467 /* Force the I2C peripheral clock reset */ 00468 DISCOVERY_AUDIO_I2Cx_FORCE_RESET(); 00469 00470 /* Release the I2C peripheral clock reset */ 00471 DISCOVERY_AUDIO_I2Cx_RELEASE_RESET(); 00472 00473 /* Enable and set I2C1 Interrupt to a lower priority */ 00474 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_EV_IRQn, 0x0F, 0); 00475 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_EV_IRQn); 00476 00477 /* Enable and set I2C1 Interrupt to a lower priority */ 00478 HAL_NVIC_SetPriority(DISCOVERY_AUDIO_I2Cx_ER_IRQn, 0x0F, 0); 00479 HAL_NVIC_EnableIRQ(DISCOVERY_AUDIO_I2Cx_ER_IRQn); 00480 00481 } 00482 else if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cTsHandler)) 00483 { 00484 /*** Configure the GPIOs ***/ 00485 /* Enable GPIO clock */ 00486 TS_I2Cx_SCL_GPIO_CLK_ENABLE(); 00487 TS_I2Cx_SDA_GPIO_CLK_ENABLE(); 00488 /* Configure I2C SCL as alternate function */ 00489 gpio_init_structure.Pin = TS_I2Cx_SCL_PIN; 00490 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00491 gpio_init_structure.Pull = GPIO_NOPULL; 00492 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00493 gpio_init_structure.Alternate = TS_I2Cx_SCL_AF; 00494 HAL_GPIO_Init(TS_I2Cx_SCL_GPIO_PORT, &gpio_init_structure); 00495 00496 /* Configure I2C SDA as alternate function */ 00497 gpio_init_structure.Pin = TS_I2Cx_SDA_PIN; 00498 gpio_init_structure.Alternate = TS_I2Cx_SDA_AF; 00499 HAL_GPIO_Init(TS_I2Cx_SDA_GPIO_PORT, &gpio_init_structure); 00500 00501 /*** Configure the I2C peripheral ***/ 00502 /* Enable I2C clock */ 00503 TS_I2Cx_CLK_ENABLE(); 00504 00505 /* Force the I2C peripheral clock reset */ 00506 TS_I2Cx_FORCE_RESET(); 00507 00508 /* Release the I2C peripheral clock reset */ 00509 TS_I2Cx_RELEASE_RESET(); 00510 00511 /* Enable and set I2C Interrupt to a lower priority */ 00512 HAL_NVIC_SetPriority(TS_I2Cx_EV_IRQn, 0x0F, 0); 00513 HAL_NVIC_EnableIRQ(TS_I2Cx_EV_IRQn); 00514 00515 /* Enable and set I2C Interrupt to a lower priority */ 00516 HAL_NVIC_SetPriority(TS_I2Cx_ER_IRQn, 0x0F, 0); 00517 HAL_NVIC_EnableIRQ(TS_I2Cx_ER_IRQn); 00518 00519 } 00520 else 00521 { 00522 /*** Configure the GPIOs ***/ 00523 /* Enable GPIO clock */ 00524 DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00525 00526 /* Configure I2C Tx as alternate function */ 00527 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SCL_PIN; 00528 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00529 gpio_init_structure.Pull = GPIO_NOPULL; 00530 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00531 gpio_init_structure.Alternate = DISCOVERY_EXT_I2Cx_SCL_SDA_AF; 00532 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00533 00534 /* Configure I2C Rx as alternate function */ 00535 gpio_init_structure.Pin = DISCOVERY_EXT_I2Cx_SDA_PIN; 00536 HAL_GPIO_Init(DISCOVERY_EXT_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00537 00538 /*** Configure the I2C peripheral ***/ 00539 /* Enable I2C clock */ 00540 DISCOVERY_EXT_I2Cx_CLK_ENABLE(); 00541 00542 /* Force the I2C peripheral clock reset */ 00543 DISCOVERY_EXT_I2Cx_FORCE_RESET(); 00544 00545 /* Release the I2C peripheral clock reset */ 00546 DISCOVERY_EXT_I2Cx_RELEASE_RESET(); 00547 00548 /* Enable and set I2C1 Interrupt to a lower priority */ 00549 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_EV_IRQn, 0x0F, 0); 00550 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_EV_IRQn); 00551 00552 /* Enable and set I2C1 Interrupt to a lower priority */ 00553 HAL_NVIC_SetPriority(DISCOVERY_EXT_I2Cx_ER_IRQn, 0x0F, 0); 00554 HAL_NVIC_EnableIRQ(DISCOVERY_EXT_I2Cx_ER_IRQn); 00555 } 00556 } 00557 00558 /** 00559 * @brief Initializes I2C HAL. 00560 * @param i2c_handler : I2C handler 00561 * @retval None 00562 */ 00563 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00564 { 00565 if(HAL_I2C_GetState(i2c_handler) == HAL_I2C_STATE_RESET) 00566 { 00567 if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cAudioHandler)) 00568 { 00569 /* Audio and LCD I2C configuration */ 00570 i2c_handler->Instance = DISCOVERY_AUDIO_I2Cx; 00571 } 00572 else if (i2c_handler == (I2C_HandleTypeDef*)(&hI2cTsHandler)) 00573 { 00574 /* TouchScreen I2C configuration */ 00575 i2c_handler->Instance = TS_I2Cx; 00576 } 00577 else 00578 { 00579 /* External, camera and Arduino connector I2C configuration */ 00580 i2c_handler->Instance = DISCOVERY_EXT_I2Cx; 00581 } 00582 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00583 i2c_handler->Init.OwnAddress1 = 0; 00584 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00585 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00586 i2c_handler->Init.OwnAddress2 = 0; 00587 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00588 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00589 00590 /* Init the I2C */ 00591 I2Cx_MspInit(i2c_handler); 00592 HAL_I2C_Init(i2c_handler); 00593 } 00594 } 00595 00596 /** 00597 * @brief Reads multiple data. 00598 * @param i2c_handler : I2C handler 00599 * @param Addr: I2C address 00600 * @param Reg: Reg address 00601 * @param MemAddress: memory address 00602 * @param Buffer: Pointer to data buffer 00603 * @param Length: Length of the data 00604 * @retval HAL status 00605 */ 00606 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00607 { 00608 HAL_StatusTypeDef status = HAL_OK; 00609 00610 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00611 00612 /* Check the communication status */ 00613 if(status != HAL_OK) 00614 { 00615 /* I2C error occured */ 00616 I2Cx_Error(i2c_handler, Addr); 00617 } 00618 return status; 00619 } 00620 00621 00622 /** 00623 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00624 * @param i2c_handler : I2C handler 00625 * @param Addr: Device address on BUS Bus. 00626 * @param Reg: The target register address to write 00627 * @param MemAddress: memory address 00628 * @param Buffer: The target register value to be written 00629 * @param Length: buffer size to be written 00630 * @retval HAL status 00631 */ 00632 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00633 { 00634 HAL_StatusTypeDef status = HAL_OK; 00635 00636 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00637 00638 /* Check the communication status */ 00639 if(status != HAL_OK) 00640 { 00641 /* Re-Initiaize the I2C Bus */ 00642 I2Cx_Error(i2c_handler, Addr); 00643 } 00644 return status; 00645 } 00646 00647 00648 /** 00649 * @brief Manages error callback by re-initializing I2C. 00650 * @param i2c_handler : I2C handler 00651 * @param Addr: I2C Address 00652 * @retval None 00653 */ 00654 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00655 { 00656 /* De-initialize the I2C communication bus */ 00657 HAL_I2C_DeInit(i2c_handler); 00658 00659 /* Re-Initialize the I2C communication bus */ 00660 I2Cx_Init(i2c_handler); 00661 } 00662 00663 /** 00664 * @} 00665 */ 00666 00667 /******************************************************************************* 00668 LINK OPERATIONS 00669 *******************************************************************************/ 00670 /*************************** FMC Routines *************************************/ 00671 /** 00672 * @brief Initializes FMC_BANK2 MSP. 00673 * @retval None 00674 */ 00675 static void FMC_BANK2_MspInit(void) 00676 { 00677 GPIO_InitTypeDef gpio_init_structure; 00678 00679 /* Enable FMC clock */ 00680 __HAL_RCC_FMC_CLK_ENABLE(); 00681 00682 /* Enable FSMC clock */ 00683 __HAL_RCC_FMC_CLK_ENABLE(); 00684 __HAL_RCC_FMC_FORCE_RESET(); 00685 __HAL_RCC_FMC_RELEASE_RESET(); 00686 00687 /* Enable GPIOs clock */ 00688 __HAL_RCC_GPIOD_CLK_ENABLE(); 00689 __HAL_RCC_GPIOE_CLK_ENABLE(); 00690 __HAL_RCC_GPIOF_CLK_ENABLE(); 00691 __HAL_RCC_GPIOG_CLK_ENABLE(); 00692 00693 /* Common GPIO configuration */ 00694 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00695 gpio_init_structure.Pull = GPIO_PULLUP; 00696 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00697 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00698 00699 /* GPIOD configuration */ 00700 /* LCD_PSRAM_D2, LCD_PSRAM_D3, LCD_PSRAM_NOE, LCD_PSRAM_NWE, PSRAM_NE1, LCD_PSRAM_D13, 00701 LCD_PSRAM_D14, LCD_PSRAM_D15, PSRAM_A16, PSRAM_A17, LCD_PSRAM_D0, LCD_PSRAM_D1 */ 00702 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00703 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15; 00704 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00705 00706 /* GPIOE configuration */ 00707 /* PSRAM_NBL0, PSRAM_NBL1, LCD_PSRAM_D4, LCD_PSRAM_D5, LCD_PSRAM_D6, LCD_PSRAM_D7, 00708 LCD_PSRAM_D8, LCD_PSRAM_D9, LCD_PSRAM_D10, LCD_PSRAM_D11, LCD_PSRAM_D12 */ 00709 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 |GPIO_PIN_10 |\ 00710 GPIO_PIN_11 | GPIO_PIN_12 |GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00711 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00712 00713 /* GPIOF configuration */ 00714 /* PSRAM_A0, PSRAM_A1, PSRAM_A2, PSRAM_A3, PSRAM_A4, PSRAM_A5, 00715 PSRAM_A6, PSRAM_A7, PSRAM_A8, PSRAM_A9 */ 00716 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 |\ 00717 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00718 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00719 00720 /* GPIOG configuration */ 00721 /* PSRAM_A10, PSRAM_A11, PSRAM_A12, PSRAM_A13, PSRAM_A14, PSRAM_A15, LCD_NE */ 00722 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 |\ 00723 GPIO_PIN_9 ; 00724 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00725 } 00726 00727 00728 /** 00729 * @brief Initializes LCD IO. 00730 * @retval None 00731 */ 00732 static void FMC_BANK2_Init(void) 00733 { 00734 SRAM_HandleTypeDef hsram; 00735 FMC_NORSRAM_TimingTypeDef sram_timing; 00736 00737 /* GPIO configuration for FMC signals (LCD) */ 00738 FMC_BANK2_MspInit(); 00739 00740 /* PSRAM device configuration */ 00741 hsram.Instance = FMC_NORSRAM_DEVICE; 00742 hsram.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00743 00744 /* Set parameters for LCD access (FMC_NORSRAM_BANK2) */ 00745 hsram.Init.NSBank = FMC_NORSRAM_BANK2; 00746 hsram.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00747 hsram.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00748 hsram.Init.MemoryDataWidth = FMC_NORSRAM_MEM_BUS_WIDTH_16; 00749 hsram.Init.BurstAccessMode = FMC_BURST_ACCESS_MODE_DISABLE; 00750 hsram.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00751 hsram.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00752 hsram.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00753 hsram.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00754 hsram.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00755 hsram.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00756 hsram.Init.WriteBurst = FMC_WRITE_BURST_DISABLE; 00757 hsram.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00758 hsram.Init.PageSize = FMC_PAGE_SIZE_NONE; 00759 hsram.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00760 00761 /* PSRAM device configuration */ 00762 /* Timing configuration derived from system clock (up to 216Mhz) 00763 for 108Mhz as PSRAM clock frequency */ 00764 sram_timing.AddressSetupTime = 9; 00765 sram_timing.AddressHoldTime = 2; 00766 sram_timing.DataSetupTime = 6; 00767 sram_timing.BusTurnAroundDuration = 1; 00768 sram_timing.CLKDivision = 2; 00769 sram_timing.DataLatency = 2; 00770 sram_timing.AccessMode = FMC_ACCESS_MODE_A; 00771 00772 /* Initialize the FMC controller for LCD (FMC_NORSRAM_BANK2) */ 00773 HAL_SRAM_Init(&hsram, &sram_timing, &sram_timing); 00774 } 00775 00776 /** 00777 * @brief Writes register value. 00778 * @param Data: Data to be written 00779 * @retval None 00780 */ 00781 static void FMC_BANK2_WriteData(uint16_t Data) 00782 { 00783 /* Write 16-bit Reg */ 00784 FMC_BANK2->RAM = Data; 00785 __DSB(); 00786 } 00787 00788 /** 00789 * @brief Writes register address. 00790 * @param Reg: Register to be written 00791 * @retval None 00792 */ 00793 static void FMC_BANK2_WriteReg(uint8_t Reg) 00794 { 00795 /* Write 16-bit Index, then write register */ 00796 FMC_BANK2->REG = Reg; 00797 __DSB(); 00798 } 00799 00800 /** 00801 * @brief Reads register value. 00802 * @retval Read value 00803 */ 00804 static uint16_t FMC_BANK2_ReadData(void) 00805 { 00806 return FMC_BANK2->RAM; 00807 } 00808 00809 /******************************************************************************* 00810 LINK OPERATIONS 00811 *******************************************************************************/ 00812 00813 /********************************* LINK LCD ***********************************/ 00814 00815 /** 00816 * @brief Initializes LCD low level. 00817 * @retval None 00818 */ 00819 void LCD_IO_Init(void) 00820 { 00821 FMC_BANK2_Init(); 00822 } 00823 00824 /** 00825 * @brief Writes data on LCD data register. 00826 * @param RegValue: Register value to be written 00827 * @retval None 00828 */ 00829 void LCD_IO_WriteData(uint16_t RegValue) 00830 { 00831 /* Write 16-bit Reg */ 00832 FMC_BANK2_WriteData(RegValue); 00833 __DSB(); 00834 } 00835 00836 /** 00837 * @brief Writes several data on LCD data register. 00838 * @param pData: pointer on data to be written 00839 * @param Size: data amount in 16bits short unit 00840 * @retval None 00841 */ 00842 void LCD_IO_WriteMultipleData(uint16_t *pData, uint32_t Size) 00843 { 00844 uint32_t i; 00845 00846 for (i = 0; i < Size; i++) 00847 { 00848 FMC_BANK2_WriteData(pData[i]); 00849 __DSB(); 00850 } 00851 } 00852 00853 /** 00854 * @brief Writes register on LCD register. 00855 * @param Reg: Register to be written 00856 * @retval None 00857 */ 00858 void LCD_IO_WriteReg(uint8_t Reg) 00859 { 00860 /* Write 16-bit Index, then Write Reg */ 00861 FMC_BANK2_WriteReg(Reg); 00862 __DSB(); 00863 } 00864 00865 /** 00866 * @brief Reads data from LCD data register. 00867 * @retval Read data. 00868 */ 00869 uint16_t LCD_IO_ReadData(void) 00870 { 00871 return FMC_BANK2_ReadData(); 00872 } 00873 00874 /** 00875 * @brief LCD delay 00876 * @param Delay: Delay in ms 00877 * @retval None 00878 */ 00879 void LCD_IO_Delay(uint32_t Delay) 00880 { 00881 HAL_Delay(Delay); 00882 } 00883 00884 /********************************* LINK AUDIO *********************************/ 00885 00886 /** 00887 * @brief Initializes Audio low level. 00888 */ 00889 void AUDIO_IO_Init(void) 00890 { 00891 I2Cx_Init(&hI2cAudioHandler); 00892 } 00893 00894 /** 00895 * @brief DeInitializes Audio low level. 00896 */ 00897 void AUDIO_IO_DeInit(void) 00898 { 00899 00900 } 00901 00902 /** 00903 * @brief Writes a single data. 00904 * @param Addr: I2C address 00905 * @param Reg: Reg address 00906 * @param Value: Data to be written 00907 * @retval None 00908 */ 00909 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00910 { 00911 uint16_t tmp = Value; 00912 00913 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00914 00915 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00916 00917 I2Cx_WriteMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00918 } 00919 00920 /** 00921 * @brief Reads a single data. 00922 * @param Addr: I2C address 00923 * @param Reg: Reg address 00924 * @retval Data to be read 00925 */ 00926 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00927 { 00928 uint16_t read_value = 0, tmp = 0; 00929 00930 I2Cx_ReadMultiple(&hI2cAudioHandler, Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 00931 00932 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 00933 00934 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 00935 00936 read_value = tmp; 00937 00938 return read_value; 00939 } 00940 00941 /** 00942 * @brief AUDIO Codec delay 00943 * @param Delay: Delay in ms 00944 */ 00945 void AUDIO_IO_Delay(uint32_t Delay) 00946 { 00947 HAL_Delay(Delay); 00948 } 00949 00950 /******************************** LINK TS (TouchScreen) ***********************/ 00951 00952 /** 00953 * @brief Initializes Touchscreen low level. 00954 * @retval None 00955 */ 00956 void TS_IO_Init(void) 00957 { 00958 TS_RESET_GPIO_CLK_ENABLE(); 00959 GPIO_InitTypeDef gpio_init_structure; 00960 /* Configure Button pin as input */ 00961 gpio_init_structure.Pin = TS_RESET_PIN; 00962 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00963 gpio_init_structure.Pull = GPIO_NOPULL; 00964 gpio_init_structure.Speed = GPIO_SPEED_FREQ_LOW; 00965 HAL_GPIO_Init(TS_RESET_GPIO_PORT, &gpio_init_structure); 00966 HAL_GPIO_WritePin(TS_RESET_GPIO_PORT, TS_RESET_PIN, GPIO_PIN_SET); 00967 I2Cx_Init(&hI2cTsHandler); 00968 } 00969 00970 /** 00971 * @brief Writes a single data. 00972 * @param Addr: I2C address 00973 * @param Reg: Reg address 00974 * @param Value: Data to be written 00975 * @retval None 00976 */ 00977 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00978 { 00979 I2Cx_WriteMultiple(&hI2cTsHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00980 } 00981 00982 /** 00983 * @brief Reads a single data. 00984 * @param Addr: I2C address 00985 * @param Reg: Reg address 00986 * @retval Data to be read 00987 */ 00988 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 00989 { 00990 uint8_t read_value = 0; 00991 00992 I2Cx_ReadMultiple(&hI2cTsHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00993 00994 return read_value; 00995 } 00996 00997 /** 00998 * @brief Reads multiple data with I2C communication 00999 * channel from TouchScreen. 01000 * @param Addr: I2C address 01001 * @param Reg: Register address 01002 * @param Buffer: Pointer to data buffer 01003 * @param Length: Length of the data 01004 * @retval Number of read data 01005 */ 01006 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01007 { 01008 return I2Cx_ReadMultiple(&hI2cTsHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01009 } 01010 01011 /** 01012 * @brief Writes multiple data with I2C communication 01013 * channel from MCU to TouchScreen. 01014 * @param Addr: I2C address 01015 * @param Reg: Register address 01016 * @param Buffer: Pointer to data buffer 01017 * @param Length: Length of the data 01018 * @retval None 01019 */ 01020 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01021 { 01022 I2Cx_WriteMultiple(&hI2cTsHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01023 } 01024 01025 /** 01026 * @brief Delay function used in TouchScreen low level driver. 01027 * @param Delay: Delay in ms 01028 * @retval None 01029 */ 01030 void TS_IO_Delay(uint32_t Delay) 01031 { 01032 HAL_Delay(Delay); 01033 } 01034 01035 /** 01036 * @} 01037 */ 01038 01039 /** 01040 * @} 01041 */ 01042 01043 /** 01044 * @} 01045 */ 01046 01047 /** 01048 * @} 01049 */ 01050 01051 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue May 30 2017 13:59:12 for STM32F723E-Discovery BSP User Manual by
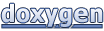