STM32F3xx_Nucleo_32 BSP User Manual
|
stm32f3xx_nucleo_32.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f3xx_nucleo_32.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage: 00006 * - LED available on STM32F3XX-Nucleo Kit 00007 * from STMicroelectronics. 00008 * - Gravitech 7segment shield available separatly. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32f3xx_nucleo_32.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32F3XX_NUCLEO_32 00047 * @brief This file provides set of firmware functions to manage Leds and push-button 00048 * available on STM32F3XX-NUCLEO_32 Kit from STMicroelectronics. 00049 * @{ 00050 */ 00051 /** @addtogroup STM32F3XX_NUCLEO_32_Common 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32F3XX_NUCLEO_32_Private_Constants 00056 * @{ 00057 */ 00058 00059 /** 00060 * @brief STM32F3XX NUCLEO BSP Driver version number V1.0.4 00061 */ 00062 #define __STM32F3XX_NUCLEO_32_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00063 #define __STM32F3XX_NUCLEO_32_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00064 #define __STM32F3XX_NUCLEO_32_BSP_VERSION_SUB2 (0x04) /*!< [15:8] sub2 version */ 00065 #define __STM32F3XX_NUCLEO_32_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00066 #define __STM32F3XX_NUCLEO_32_BSP_VERSION ((__STM32F3XX_NUCLEO_32_BSP_VERSION_MAIN << 24)\ 00067 |(__STM32F3XX_NUCLEO_32_BSP_VERSION_SUB1 << 16)\ 00068 |(__STM32F3XX_NUCLEO_32_BSP_VERSION_SUB2 << 8 )\ 00069 |(__STM32F3XX_NUCLEO_32_BSP_VERSION_RC)) 00070 00071 /** 00072 * @} 00073 */ 00074 00075 /** @addtogroup STM32F3XX_NUCLEO_32_Private_Variables 00076 * @{ 00077 */ 00078 /** 00079 * @brief LED variables 00080 */ 00081 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT}; 00082 const uint16_t LED_PIN[LEDn] = {LED3_PIN}; 00083 00084 00085 /** 00086 * @brief BUS variables 00087 */ 00088 #if defined(HAL_I2C_MODULE_ENABLED) 00089 uint32_t I2c1Timeout = BSP_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00090 I2C_HandleTypeDef nucleo32_I2c1; 00091 #endif /* HAL_I2C_MODULE_ENABLED */ 00092 00093 /** 00094 * @} 00095 */ 00096 00097 /** @defgroup STM32F3XX_NUCLEO_32_BUS Bus Operation functions 00098 * @{ 00099 */ 00100 00101 #if defined(HAL_I2C_MODULE_ENABLED) 00102 /* I2C1 bus function */ 00103 /* Link function for I2C peripherals */ 00104 void I2C1_Init(void); 00105 void I2C1_Error (void); 00106 void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00107 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00108 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg); 00109 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00110 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00111 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00112 #endif /* HAL_I2C_MODULE_ENABLED */ 00113 00114 /** 00115 * @} 00116 */ 00117 00118 /** @addtogroup STM32F3XX_NUCLEO_32_Exported_Functions 00119 * @{ 00120 */ 00121 00122 /** 00123 * @brief This method returns the STM32F3XX NUCLEO BSP Driver revision 00124 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00125 */ 00126 00127 uint32_t BSP_GetVersion(void) 00128 { 00129 return __STM32F3XX_NUCLEO_32_BSP_VERSION; 00130 } 00131 00132 /** 00133 * @brief Configures LED GPIO. 00134 * @param Led Specifies the Led to be configured. 00135 * This parameter can be one of following parameters: 00136 * @arg LED3 00137 * @retval None 00138 */ 00139 void BSP_LED_Init(Led_TypeDef Led) 00140 { 00141 GPIO_InitTypeDef GPIO_InitStruct; 00142 00143 /* Enable the GPIO_LED Clock */ 00144 LEDx_GPIO_CLK_ENABLE(Led); 00145 00146 /* Configure the GPIO_LED pin */ 00147 GPIO_InitStruct.Pin = LED_PIN[Led]; 00148 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00149 GPIO_InitStruct.Pull = GPIO_NOPULL; 00150 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00151 00152 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00153 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00154 } 00155 00156 /** 00157 * @brief Turns selected LED On. 00158 * @param Led Specifies the Led to be set on. 00159 * This parameter can be one of following parameters: 00160 * @arg LED3 00161 * @retval None 00162 */ 00163 void BSP_LED_On(Led_TypeDef Led) 00164 { 00165 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00166 } 00167 00168 /** 00169 * @brief Turns selected LED Off. 00170 * @param Led Specifies the Led to be set off. 00171 * This parameter can be one of following parameters: 00172 * @arg LED3 00173 * @retval None 00174 */ 00175 void BSP_LED_Off(Led_TypeDef Led) 00176 { 00177 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00178 } 00179 00180 /** 00181 * @brief Toggles the selected LED. 00182 * @param Led Specifies the Led to be toggled. 00183 * This parameter can be one of following parameters: 00184 * @arg LED3 00185 * @retval None 00186 */ 00187 void BSP_LED_Toggle(Led_TypeDef Led) 00188 { 00189 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00190 } 00191 00192 /** 00193 * @} 00194 */ 00195 00196 /** @addtogroup STM32F3XX_NUCLEO_32_BUS 00197 * @{ 00198 */ 00199 /****************************************************************************** 00200 BUS OPERATIONS 00201 *******************************************************************************/ 00202 #if defined(HAL_I2C_MODULE_ENABLED) 00203 /******************************* I2C Routines *********************************/ 00204 00205 /** 00206 * @brief I2C Bus initialization 00207 * @retval None 00208 */ 00209 void I2C1_Init(void) 00210 { 00211 if(HAL_I2C_GetState(&nucleo32_I2c1) == HAL_I2C_STATE_RESET) 00212 { 00213 nucleo32_I2c1.Instance = BSP_I2C1; 00214 nucleo32_I2c1.Init.Timing = I2C1_TIMING; 00215 nucleo32_I2c1.Init.OwnAddress1 = 0; 00216 nucleo32_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00217 nucleo32_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00218 nucleo32_I2c1.Init.OwnAddress2 = 0; 00219 nucleo32_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00220 nucleo32_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00221 nucleo32_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00222 00223 /* Init the I2C */ 00224 I2C1_MspInit(&nucleo32_I2c1); 00225 HAL_I2C_Init(&nucleo32_I2c1); 00226 } 00227 } 00228 00229 /** 00230 * @brief Writes a single data. 00231 * @param Addr I2C address 00232 * @param Reg Register address 00233 * @param Value Data to be written 00234 * @retval None 00235 */ 00236 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00237 { 00238 HAL_StatusTypeDef status = HAL_OK; 00239 00240 status = HAL_I2C_Mem_Write(&nucleo32_I2c1, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00241 00242 /* Check the communication status */ 00243 if(status != HAL_OK) 00244 { 00245 /* Execute user timeout callback */ 00246 I2C1_Error(); 00247 } 00248 } 00249 00250 /** 00251 * @brief Reads a single data. 00252 * @param Addr I2C address 00253 * @param Reg Register address 00254 * @retval Read data 00255 */ 00256 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg) 00257 { 00258 HAL_StatusTypeDef status = HAL_OK; 00259 uint8_t Value = 0; 00260 00261 status = HAL_I2C_Mem_Read(&nucleo32_I2c1, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00262 00263 /* Check the communication status */ 00264 if(status != HAL_OK) 00265 { 00266 /* Execute user timeout callback */ 00267 I2C1_Error(); 00268 } 00269 return Value; 00270 } 00271 00272 /** 00273 * @brief Reads multiple data on the BUS. 00274 * @param Addr I2C Address 00275 * @param Reg Reg Address 00276 * @param RegSize The target register size (can be 8BIT or 16BIT) 00277 * @param pBuffer pointer to read data buffer 00278 * @param Length length of the data 00279 * @retval 0 if no problems to read multiple data 00280 */ 00281 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00282 { 00283 HAL_StatusTypeDef status = HAL_OK; 00284 00285 status = HAL_I2C_Mem_Read(&nucleo32_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00286 00287 /* Check the communication status */ 00288 if(status != HAL_OK) 00289 { 00290 /* Re-Initiaize the BUS */ 00291 I2C1_Error(); 00292 } 00293 return status; 00294 } 00295 00296 /** 00297 * @brief Checks if target device is ready for communication. 00298 * @note This function is used with Memory devices 00299 * @param DevAddress Target device address 00300 * @param Trials Number of trials 00301 * @retval HAL status 00302 */ 00303 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00304 { 00305 return (HAL_I2C_IsDeviceReady(&nucleo32_I2c1, DevAddress, Trials, I2c1Timeout)); 00306 } 00307 00308 /** 00309 * @brief Write a value in a register of the device through BUS. 00310 * @param Addr Device address on BUS Bus. 00311 * @param Reg The target register address to write 00312 * @param RegSize The target register size (can be 8BIT or 16BIT) 00313 * @param pBuffer The target register value to be written 00314 * @param Length buffer size to be written 00315 * @retval None 00316 */ 00317 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00318 { 00319 HAL_StatusTypeDef status = HAL_OK; 00320 00321 status = HAL_I2C_Mem_Write(&nucleo32_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00322 00323 /* Check the communication status */ 00324 if(status != HAL_OK) 00325 { 00326 /* Re-Initiaize the BUS */ 00327 I2C1_Error(); 00328 } 00329 return status; 00330 } 00331 00332 /** 00333 * @brief Manages error callback by re-initializing I2C. 00334 * @retval None 00335 */ 00336 void I2C1_Error(void) 00337 { 00338 /* De-initialize the I2C communication BUS */ 00339 HAL_I2C_DeInit(&nucleo32_I2c1); 00340 00341 /* Re-Initiaize the I2C communication BUS */ 00342 I2C1_Init(); 00343 } 00344 00345 /** 00346 * @brief I2C MSP Initialization 00347 * @param hi2c I2C handle 00348 * @retval None 00349 */ 00350 void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00351 { 00352 GPIO_InitTypeDef GPIO_InitStruct; 00353 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00354 00355 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00356 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00357 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00358 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00359 00360 /*##-2- Configure the GPIOs ################################################*/ 00361 00362 /* Enable GPIO clock */ 00363 BSP_I2C1_GPIO_CLK_ENABLE(); 00364 00365 /* Configure I2C SCL & SDA as alternate function */ 00366 GPIO_InitStruct.Pin = (BSP_I2C1_SCL_PIN| BSP_I2C1_SDA_PIN); 00367 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00368 GPIO_InitStruct.Pull = GPIO_PULLUP; 00369 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00370 GPIO_InitStruct.Alternate = BSP_I2C1_SCL_SDA_AF; 00371 HAL_GPIO_Init(BSP_I2C1_GPIO_PORT, &GPIO_InitStruct); 00372 00373 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00374 /* Enable I2C clock */ 00375 BSP_I2C1_CLK_ENABLE(); 00376 00377 /* Force the I2C peripheral clock reset */ 00378 BSP_I2C1_FORCE_RESET(); 00379 00380 /* Release the I2C peripheral clock reset */ 00381 BSP_I2C1_RELEASE_RESET(); 00382 } 00383 00384 #endif /*HAL_I2C_MODULE_ENABLED*/ 00385 00386 /** 00387 * @} 00388 */ 00389 00390 /** 00391 * @} 00392 */ 00393 00394 /** @addtogroup STM32F3XX_NUCLEO_32_GRAVITECH_4DIGITS 00395 * @{ 00396 */ 00397 00398 /** @addtogroup STM32_GRAVITECH_4DIGITS_Exported_Functions 00399 * @{ 00400 */ 00401 00402 #if defined(HAL_I2C_MODULE_ENABLED) 00403 00404 /** 00405 * @brief BSP of the 4 digits 7 Segment Display shield for Arduino Nano - Gravitech. 00406 init 00407 * @retval HAL_StatusTypeDef 00408 */ 00409 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Init(void) 00410 { 00411 uint8_t control[1] = {0x47}; 00412 00413 /* Init I2C */ 00414 I2C1_Init(); 00415 00416 /* Configure the SAA1064 component */ 00417 return I2C1_WriteBuffer(0x70, 0, 1, control, sizeof(control)); 00418 } 00419 00420 /** 00421 * @brief BSP of the 4 digits 7 Segment Display shield for Arduino Nano - Gravitech. 00422 Display the value if value belong to [0-9999] 00423 * @param Value A number between 0 and 9999 will be displayed on the screen. 00424 * DIGIT4_SEG7_RESET will reset the screen (any value above 9999 will reset the screen also) 00425 * @retval HAL_StatusTypeDef 00426 */ 00427 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Display(uint32_t Value) 00428 { 00429 const uint8_t lookup[10] = {0x3F,0x06,0x5B,0x4F,0x66, 00430 0x6D,0x7D,0x07,0x7F,0x6F}; 00431 00432 uint32_t thousands, hundreds, tens, base; 00433 HAL_StatusTypeDef status = HAL_ERROR; 00434 uint8_t d1d2d3d4[4]; 00435 00436 if (Value < 10000) 00437 { 00438 thousands = Value / 1000; 00439 hundreds = (Value - (thousands * 1000)) / 100; 00440 tens = (Value - ((thousands * 1000) + (hundreds * 100))) / 10; 00441 base = Value - ((thousands * 1000) + (hundreds * 100) + (tens * 10)); 00442 00443 d1d2d3d4[3] = lookup[thousands]; 00444 d1d2d3d4[2] = lookup[hundreds]; 00445 d1d2d3d4[1] = lookup[tens]; 00446 d1d2d3d4[0] = lookup[base]; 00447 00448 } 00449 else 00450 { 00451 d1d2d3d4[3] = 0; 00452 d1d2d3d4[2] = 0; 00453 d1d2d3d4[1] = 0; 00454 d1d2d3d4[0] = 0; 00455 } 00456 00457 /* Send the four digits to the SAA1064 component */ 00458 status = I2C1_WriteBuffer(0x70, 1, 1, d1d2d3d4, sizeof(d1d2d3d4)); 00459 00460 return status; 00461 } 00462 00463 #endif /*HAL_I2C_MODULE_ENABLED*/ 00464 00465 /** 00466 * @} 00467 */ 00468 00469 /** 00470 * @} 00471 */ 00472 00473 /** 00474 * @} 00475 */ 00476 00477 /** 00478 * @} 00479 */ 00480 00481 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 11:09:08 for STM32F3xx_Nucleo_32 BSP User Manual by
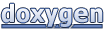