STM32F3348-Discovery BSP User Manual
|
stm32f3348_discovery.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f3348_discovery.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage Leds and 00006 * push-button available on STM32F3348-DISCO Kit from STMicroelectronics. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32f3348_discovery.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup STM32F3348_DISCOVERY 00045 * @brief This file provides set of firmware functions to manage Leds and push-button 00046 * available on STM32F3348-Discovery Kit from STMicroelectronics. 00047 * @{ 00048 */ 00049 /** @addtogroup STM32F3348_DISCOVERY_Common 00050 * @{ 00051 */ 00052 00053 /** @addtogroup STM32F3348_DISCOVERY_Private_Constants 00054 * @{ 00055 */ 00056 00057 /** 00058 * @brief STM32F3348 DISCO BSP Driver version number V2.0.4 00059 */ 00060 #define __STM32F3348_DISCO_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00061 #define __STM32F3348_DISCO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00062 #define __STM32F3348_DISCO_BSP_VERSION_SUB2 (0x04) /*!< [15:8] sub2 version */ 00063 #define __STM32F3348_DISCO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00064 #define __STM32F3348_DISCO_BSP_VERSION ((__STM32F3348_DISCO_BSP_VERSION_MAIN << 24)\ 00065 |(__STM32F3348_DISCO_BSP_VERSION_SUB1 << 16)\ 00066 |(__STM32F3348_DISCO_BSP_VERSION_SUB2 << 8 )\ 00067 |(__STM32F3348_DISCO_BSP_VERSION_RC)) 00068 /** 00069 * @} 00070 */ 00071 00072 /** @addtogroup STM32F3348_DISCOVERY_Private_Variables 00073 * @{ 00074 */ 00075 /** 00076 * @brief LED variables 00077 */ 00078 00079 00080 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT, 00081 LED4_GPIO_PORT, 00082 LED5_GPIO_PORT, 00083 LED6_GPIO_PORT}; 00084 00085 const uint16_t LED_PIN[LEDn] = {LED3_PIN, 00086 LED4_PIN, 00087 LED5_PIN, 00088 LED6_PIN}; 00089 /** 00090 * @brief BUTTON variables 00091 */ 00092 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00093 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00094 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00095 00096 /** 00097 * @} 00098 */ 00099 00100 /** @addtogroup STM32F3348_DISCOVERY_Exported_Functions 00101 * @{ 00102 */ 00103 00104 /** 00105 * @brief This method returns the STM32F3348-DISCOVERY BSP Driver revision 00106 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00107 */ 00108 uint32_t BSP_GetVersion(void) 00109 { 00110 return __STM32F3348_DISCO_BSP_VERSION; 00111 } 00112 00113 /** 00114 * @brief Configures LED GPIO. 00115 * @param Led Specifies the Led to be configured. 00116 * This parameter can be one of following parameters: 00117 * @arg LED_RED 00118 * @arg LED_BLUE 00119 * @arg LED_ORANGE 00120 * @arg LED_GREEN 00121 * @retval None 00122 */ 00123 void BSP_LED_Init(Led_TypeDef Led) 00124 { 00125 GPIO_InitTypeDef GPIO_InitStruct; 00126 00127 /* Enable the GPIO_LED Clock */ 00128 LEDx_GPIO_CLK_ENABLE(Led); 00129 00130 /* Configure the GPIO_LED pin */ 00131 GPIO_InitStruct.Pin = LED_PIN[Led]; 00132 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00133 GPIO_InitStruct.Pull = GPIO_PULLUP; 00134 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00135 00136 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00137 00138 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00139 } 00140 00141 /** 00142 * @brief Turns selected LED On. 00143 * @param Led Specifies the Led to be set on. 00144 * This parameter can be one of following parameters: 00145 * @arg LED_RED 00146 * @arg LED_BLUE 00147 * @arg LED_ORANGE 00148 * @arg LED_GREEN 00149 * @retval None 00150 */ 00151 void BSP_LED_On(Led_TypeDef Led) 00152 { 00153 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00154 } 00155 00156 /** 00157 * @brief Turns selected LED Off. 00158 * @param Led Specifies the Led to be set off. 00159 * This parameter can be one of following parameters: 00160 * @arg LED_RED 00161 * @arg LED_BLUE 00162 * @arg LED_ORANGE 00163 * @arg LED_GREEN 00164 * @retval None 00165 */ 00166 void BSP_LED_Off(Led_TypeDef Led) 00167 { 00168 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00169 } 00170 00171 /** 00172 * @brief Toggles the selected LED. 00173 * @param Led Specifies the Led to be toggled. 00174 * This parameter can be one of following parameters: 00175 * @arg LED_RED 00176 * @arg LED_BLUE 00177 * @arg LED_ORANGE 00178 * @arg LED_GREEN 00179 * @retval None 00180 */ 00181 void BSP_LED_Toggle(Led_TypeDef Led) 00182 { 00183 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00184 } 00185 00186 00187 /** 00188 * @brief Configures Button GPIO and EXTI Line. 00189 * @param Button Specifies the Button to be configured. 00190 * This parameter should be: BUTTON_USER 00191 * @param ButtonMode Specifies Button mode. 00192 * This parameter can be one of following parameters: 00193 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00194 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00195 * generation capability 00196 * @retval None 00197 */ 00198 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00199 { 00200 GPIO_InitTypeDef GPIO_InitStruct; 00201 00202 /* Enable the BUTTON Clock */ 00203 BUTTONx_GPIO_CLK_ENABLE(Button); 00204 __HAL_RCC_SYSCFG_CLK_ENABLE(); 00205 00206 if (ButtonMode == BUTTON_MODE_GPIO) 00207 { 00208 /* Configure Button pin as input */ 00209 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00210 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00211 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 00212 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00213 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00214 } 00215 00216 if (ButtonMode == BUTTON_MODE_EXTI) 00217 { 00218 /* Configure Button pin as input with External interrupt */ 00219 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00220 GPIO_InitStruct.Pull = GPIO_NOPULL; 00221 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00222 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00223 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00224 00225 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00226 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00227 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00228 } 00229 } 00230 00231 /** 00232 * @brief Returns the selected Push Button state. 00233 * @param Button Specifies the Button to be checked. 00234 * This parameter should be: BUTTON_USER 00235 * @retval The Button GPIO pin value. 00236 */ 00237 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00238 { 00239 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00240 } 00241 00242 /** 00243 * @} 00244 */ 00245 00246 /** 00247 * @} 00248 */ 00249 00250 /** 00251 * @} 00252 */ 00253 00254 /** 00255 * @} 00256 */ 00257 00258 /******************* (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:51:50 for STM32F3348-Discovery BSP User Manual by
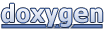