_BSP_User_Manual
|
stm32f1xx_nucleo.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f1xx_nucleo.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file contains definitions for: 00008 * - LEDs and push-button available on STM32F1XX-Nucleo Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 #ifndef __STM32F1XX_NUCLEO_H 00044 #define __STM32F1XX_NUCLEO_H 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F1XX_NUCLEO 00055 * @{ 00056 */ 00057 00058 /* Includes ------------------------------------------------------------------*/ 00059 #include "stm32f1xx_hal.h" 00060 00061 00062 /** @defgroup STM32F1XX_NUCLEO_Exported_Types Exported Types 00063 * @{ 00064 */ 00065 typedef enum 00066 { 00067 LED2 = 0, 00068 00069 LED_GREEN = LED2, 00070 00071 } Led_TypeDef; 00072 00073 typedef enum 00074 { 00075 BUTTON_USER = 0, 00076 } Button_TypeDef; 00077 00078 typedef enum 00079 { 00080 BUTTON_MODE_GPIO = 0, 00081 BUTTON_MODE_EXTI = 1 00082 } ButtonMode_TypeDef; 00083 00084 typedef enum 00085 { 00086 JOY_NONE = 0, 00087 JOY_SEL = 1, 00088 JOY_DOWN = 2, 00089 JOY_LEFT = 3, 00090 JOY_RIGHT = 4, 00091 JOY_UP = 5 00092 } JOYState_TypeDef; 00093 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32F1XX_NUCLEO_Exported_Constants Exported Constants 00099 * @{ 00100 */ 00101 00102 /** 00103 * @brief Define for STM32F1xx_NUCLEO board 00104 */ 00105 #if !defined (USE_STM32F1xx_NUCLEO) 00106 #define USE_STM32F1xx_NUCLEO 00107 #endif 00108 00109 /** @defgroup STM32F1XX_NUCLEO_LED LED Constants 00110 * @{ 00111 */ 00112 #define LEDn 1 00113 00114 #define LED2_PIN GPIO_PIN_5 00115 #define LED2_GPIO_PORT GPIOA 00116 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00117 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00118 00119 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do {LED2_GPIO_CLK_ENABLE(); } while(0) 00120 00121 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) LED2_GPIO_CLK_DISABLE()) 00122 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup STM32F1XX_NUCLEO_BUTTON BUTTON Constants 00128 * @{ 00129 */ 00130 #define BUTTONn 1 00131 00132 /** 00133 * @brief Key push-button 00134 */ 00135 #define USER_BUTTON_PIN GPIO_PIN_13 00136 #define USER_BUTTON_GPIO_PORT GPIOC 00137 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00138 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00139 #define USER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00140 00141 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do {USER_BUTTON_GPIO_CLK_ENABLE(); } while(0) 00142 00143 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) (USER_BUTTON_GPIO_CLK_DISABLE()) 00144 /** 00145 * @} 00146 */ 00147 00148 /** @addtogroup STM32F1XX_NUCLEO_BUS BUS Constants 00149 * @{ 00150 */ 00151 /*###################### SPI1 ###################################*/ 00152 #define NUCLEO_SPIx SPI1 00153 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00154 00155 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00156 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_5 00157 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00158 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00159 00160 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOA 00161 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00162 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00163 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_6 00164 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_7 00165 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00166 on accurate values, they just guarantee that the application will not remain 00167 stuck if the SPI communication is corrupted. 00168 You may modify these timeout values depending on CPU frequency and application 00169 conditions (interrupts routines ...). */ 00170 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00171 00172 00173 /** 00174 * @brief SD Control Lines management 00175 */ 00176 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00177 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00178 00179 /** 00180 * @brief LCD Control Lines management 00181 */ 00182 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00183 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00184 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00185 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00186 00187 /** 00188 * @brief SD Control Interface pins 00189 */ 00190 #define SD_CS_PIN GPIO_PIN_5 00191 #define SD_CS_GPIO_PORT GPIOB 00192 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00193 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00194 00195 /** 00196 * @brief LCD Control Interface pins 00197 */ 00198 #define LCD_CS_PIN GPIO_PIN_6 00199 #define LCD_CS_GPIO_PORT GPIOB 00200 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00201 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00202 00203 /** 00204 * @brief LCD Data/Command Interface pins 00205 */ 00206 #define LCD_DC_PIN GPIO_PIN_9 00207 #define LCD_DC_GPIO_PORT GPIOA 00208 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00209 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00210 00211 /*##################### ADC1 ###################################*/ 00212 /** 00213 * @brief ADC Interface pins 00214 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00215 */ 00216 #define NUCLEO_ADCx ADC1 00217 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC1_CLK_ENABLE() 00218 00219 #define NUCLEO_ADCx_GPIO_PORT GPIOB 00220 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_0 00221 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00222 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00223 00224 /** 00225 * @} 00226 */ 00227 00228 00229 /** 00230 * @} 00231 */ 00232 00233 00234 /** @addtogroup STM32F1XX_NUCLEO_Exported_Functions 00235 * @{ 00236 */ 00237 uint32_t BSP_GetVersion(void); 00238 /** @addtogroup STM32F1XX_NUCLEO_LED_Functions 00239 * @{ 00240 */ 00241 00242 void BSP_LED_Init(Led_TypeDef Led); 00243 void BSP_LED_On(Led_TypeDef Led); 00244 void BSP_LED_Off(Led_TypeDef Led); 00245 void BSP_LED_Toggle(Led_TypeDef Led); 00246 00247 /** 00248 * @} 00249 */ 00250 00251 /** @addtogroup STM32F1XX_NUCLEO_BUTTON_Functions 00252 * @{ 00253 */ 00254 00255 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00256 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00257 00258 #ifdef HAL_ADC_MODULE_ENABLED 00259 uint8_t BSP_JOY_Init(void); 00260 JOYState_TypeDef BSP_JOY_GetState(void); 00261 #endif /* HAL_ADC_MODULE_ENABLED */ 00262 00263 00264 /** 00265 * @} 00266 */ 00267 00268 /** 00269 * @} 00270 */ 00271 00272 /** 00273 * @} 00274 */ 00275 00276 /** 00277 * @} 00278 */ 00279 00280 #ifdef __cplusplus 00281 } 00282 #endif 00283 00284 #endif /* __STM32F1XX_NUCLEO_H */ 00285 00286 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 16:58:14 for _BSP_User_Manual by
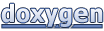