STM32F0xx-Nucleo BSP User Manual
|
stm32f0xx_nucleo.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f0xx_nucleo.h 00004 * @author MCD Application Team 00005 * @brief This file contains definitions for: 00006 * - LEDs and push-button available on STM32F0XX-Nucleo Kit 00007 * from STMicroelectronics 00008 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00009 * shield (reference ID 802) 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Define to prevent recursive inclusion -------------------------------------*/ 00041 #ifndef __STM32F0XX_NUCLEO_H 00042 #define __STM32F0XX_NUCLEO_H 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32F0XX_NUCLEO STM32F0XX-NUCLEO 00053 * @{ 00054 */ 00055 00056 /* Includes ------------------------------------------------------------------*/ 00057 #include "stm32f0xx_hal.h" 00058 00059 00060 /** @defgroup STM32F0XX_NUCLEO_Exported_Types Exported Types 00061 * @{ 00062 */ 00063 typedef enum 00064 { 00065 LED2 = 0, 00066 LED_GREEN = LED2 00067 } Led_TypeDef; 00068 00069 typedef enum 00070 { 00071 BUTTON_USER = 0, 00072 /* Alias */ 00073 BUTTON_KEY = BUTTON_USER 00074 } Button_TypeDef; 00075 00076 typedef enum 00077 { 00078 BUTTON_MODE_GPIO = 0, 00079 BUTTON_MODE_EXTI = 1 00080 } ButtonMode_TypeDef; 00081 00082 typedef enum 00083 { 00084 JOY_NONE = 0, 00085 JOY_SEL = 1, 00086 JOY_DOWN = 2, 00087 JOY_LEFT = 3, 00088 JOY_RIGHT = 4, 00089 JOY_UP = 5 00090 } JOYState_TypeDef; 00091 00092 /** 00093 * @} 00094 */ 00095 00096 /** @defgroup STM32F0XX_NUCLEO_Exported_Constants Exported Constants 00097 * @{ 00098 */ 00099 00100 /** 00101 * @brief Define for STM32F0XX_NUCLEO board 00102 */ 00103 #if !defined (USE_STM32F0XX_NUCLEO) 00104 #define USE_STM32F0XX_NUCLEO 00105 #endif 00106 00107 /** @defgroup STM32F0XX_NUCLEO_LED LED Constants 00108 * @{ 00109 */ 00110 #define LEDn 1 00111 00112 #define LED2_PIN GPIO_PIN_5 00113 #define LED2_GPIO_PORT GPIOA 00114 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00115 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00116 00117 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) LED2_GPIO_CLK_ENABLE();} while(0) 00118 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? LED2_GPIO_CLK_DISABLE() : 0) 00119 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM32F0XX_NUCLEO_BUTTON BUTTON Constants 00125 * @{ 00126 */ 00127 #define BUTTONn 1 00128 00129 /** 00130 * @brief User push-button 00131 */ 00132 #define USER_BUTTON_PIN GPIO_PIN_13 00133 #define USER_BUTTON_GPIO_PORT GPIOC 00134 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00135 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00136 #define USER_BUTTON_EXTI_LINE GPIO_PIN_13 00137 #define USER_BUTTON_EXTI_IRQn EXTI4_15_IRQn 00138 /* Aliases */ 00139 #define KEY_BUTTON_PIN USER_BUTTON_PIN 00140 #define KEY_BUTTON_GPIO_PORT USER_BUTTON_GPIO_PORT 00141 #define KEY_BUTTON_GPIO_CLK_ENABLE() USER_BUTTON_GPIO_CLK_ENABLE() 00142 #define KEY_BUTTON_GPIO_CLK_DISABLE() USER_BUTTON_GPIO_CLK_DISABLE() 00143 #define KEY_BUTTON_EXTI_LINE USER_BUTTON_EXTI_LINE 00144 #define KEY_BUTTON_EXTI_IRQn USER_BUTTON_EXTI_IRQn 00145 00146 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == 0) USER_BUTTON_GPIO_CLK_ENABLE();} while(0) 00147 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == 0) ? USER_BUTTON_GPIO_CLK_DISABLE() : 0) 00148 /** 00149 * @} 00150 */ 00151 00152 /** @defgroup STM32F0XX_NUCLEO_BUS BUS Constants 00153 * @{ 00154 */ 00155 /*###################### SPI1 ###################################*/ 00156 #define NUCLEO_SPIx SPI1 00157 #define NUCLEO_SPIx_CLK_ENABLE() __HAL_RCC_SPI1_CLK_ENABLE() 00158 00159 #define NUCLEO_SPIx_SCK_AF GPIO_AF0_SPI1 00160 #define NUCLEO_SPIx_SCK_GPIO_PORT GPIOA 00161 #define NUCLEO_SPIx_SCK_PIN GPIO_PIN_5 00162 #define NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00163 #define NUCLEO_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00164 00165 #define NUCLEO_SPIx_MISO_MOSI_AF GPIO_AF0_SPI1 00166 #define NUCLEO_SPIx_MISO_MOSI_GPIO_PORT GPIOA 00167 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00168 #define NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00169 #define NUCLEO_SPIx_MISO_PIN GPIO_PIN_6 00170 #define NUCLEO_SPIx_MOSI_PIN GPIO_PIN_7 00171 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00172 on accurate values, they just guarantee that the application will not remain 00173 stuck if the SPI communication is corrupted. 00174 You may modify these timeout values depending on CPU frequency and application 00175 conditions (interrupts routines ...). */ 00176 #define NUCLEO_SPIx_TIMEOUT_MAX 1000 00177 00178 00179 /** 00180 * @brief SD Control Lines management 00181 */ 00182 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00183 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00184 00185 /** 00186 * @brief LCD Control Lines management 00187 */ 00188 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_RESET) 00189 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_CS_GPIO_PORT, LCD_CS_PIN, GPIO_PIN_SET) 00190 #define LCD_DC_LOW() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_RESET) 00191 #define LCD_DC_HIGH() HAL_GPIO_WritePin(LCD_DC_GPIO_PORT, LCD_DC_PIN, GPIO_PIN_SET) 00192 00193 /** 00194 * @brief SD Control Interface pins (shield D4) 00195 */ 00196 #define SD_CS_PIN GPIO_PIN_5 00197 #define SD_CS_GPIO_PORT GPIOB 00198 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00199 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00200 00201 /** 00202 * @brief LCD Control Interface pins (shield D10) 00203 */ 00204 #define LCD_CS_PIN GPIO_PIN_6 00205 #define LCD_CS_GPIO_PORT GPIOB 00206 #define LCD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00207 #define LCD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00208 00209 /** 00210 * @brief LCD Data/Command Interface pins 00211 */ 00212 #define LCD_DC_PIN GPIO_PIN_9 00213 #define LCD_DC_GPIO_PORT GPIOA 00214 #define LCD_DC_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00215 #define LCD_DC_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00216 00217 /*##################### ADC1 ###################################*/ 00218 /** 00219 * @brief ADC Interface pins 00220 * used to detect motion of Joystick available on Adafruit 1.8" TFT shield 00221 */ 00222 #define NUCLEO_ADCx ADC1 00223 #define NUCLEO_ADCx_CLK_ENABLE() __HAL_RCC_ADC1_CLK_ENABLE() 00224 #define NUCLEO_ADCx_CLK_DISABLE() __HAL_RCC_ADC1_CLK_DISABLE() 00225 00226 #define NUCLEO_ADCx_GPIO_PORT GPIOB 00227 #define NUCLEO_ADCx_GPIO_PIN GPIO_PIN_0 00228 #define NUCLEO_ADCx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00229 #define NUCLEO_ADCx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00230 00231 /** 00232 * @} 00233 */ 00234 00235 /** 00236 * @} 00237 */ 00238 00239 /** @defgroup STM32F0XX_NUCLEO_Exported_Functions Exported Functions 00240 * @{ 00241 */ 00242 uint32_t BSP_GetVersion(void); 00243 /** @defgroup STM32F0XX_NUCLEO_LED_Functions LED Functions 00244 * @{ 00245 */ 00246 void BSP_LED_Init(Led_TypeDef Led); 00247 void BSP_LED_DeInit(Led_TypeDef Led); 00248 void BSP_LED_On(Led_TypeDef Led); 00249 void BSP_LED_Off(Led_TypeDef Led); 00250 void BSP_LED_Toggle(Led_TypeDef Led); 00251 /** 00252 * @} 00253 */ 00254 00255 /** @addtogroup STM32F0XX_NUCLEO_BUTTON_Functions 00256 * @{ 00257 */ 00258 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00259 void BSP_PB_DeInit(Button_TypeDef Button); 00260 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00261 #if defined(HAL_ADC_MODULE_ENABLED) 00262 uint8_t BSP_JOY_Init(void); 00263 JOYState_TypeDef BSP_JOY_GetState(void); 00264 void BSP_JOY_DeInit(void); 00265 #endif /* HAL_ADC_MODULE_ENABLED */ 00266 00267 00268 /** 00269 * @} 00270 */ 00271 00272 /** 00273 * @} 00274 */ 00275 00276 /** 00277 * @} 00278 */ 00279 00280 /** 00281 * @} 00282 */ 00283 00284 #ifdef __cplusplus 00285 } 00286 #endif 00287 00288 #endif /* __STM32F0XX_NUCLEO_H */ 00289 00290 00291 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00292
Generated on Wed Jul 5 2017 09:45:49 for STM32F0xx-Nucleo BSP User Manual by
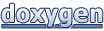