STM32756G_EVAL BSP User Manual
|
stm32756g_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 22-May-2015 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32756G-EVAL and STM32746G-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive directly an LCD TFT using the LTDC controller. 00013 - This driver selects dynamically the mounted LCD, AMPIRE 640x480 LCD mounted 00014 on MB1063 or AMPIRE 480x272 LCD mounted on MB1046 daughter board, 00015 and uses the adequate timing and setting for the specified LCD using 00016 device ID of the STMPE811 mounted on MB1046 daughter board. 00017 00018 Driver description: 00019 ------------------ 00020 + Initialization steps: 00021 o Initialize the LCD using the BSP_LCD_Init() function. 00022 o Apply the Layer configuration using the BSP_LCD_LayerDefaultInit() function. 00023 o Select the LCD layer to be used using the BSP_LCD_SelectLayer() function. 00024 o Enable the LCD display using the BSP_LCD_DisplayOn() function. 00025 00026 + Options 00027 o Configure and enable the colour keying functionality using the 00028 BSP_LCD_SetColorKeying() function. 00029 o Modify in the fly the transparency and/or the frame buffer address 00030 using the following functions: 00031 - BSP_LCD_SetTransparency() 00032 - BSP_LCD_SetLayerAddress() 00033 00034 + Display on LCD 00035 o Clear the whole LCD using BSP_LCD_Clear() function or only one specified string 00036 line using the BSP_LCD_ClearStringLine() function. 00037 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00038 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00039 o Display a string line on the specified position (x,y in pixel) and align mode 00040 using the BSP_LCD_DisplayStringAtLine() function. 00041 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00042 on LCD using the available set of functions. 00043 @endverbatim 00044 ****************************************************************************** 00045 * @attention 00046 * 00047 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00048 * 00049 * Redistribution and use in source and binary forms, with or without modification, 00050 * are permitted provided that the following conditions are met: 00051 * 1. Redistributions of source code must retain the above copyright notice, 00052 * this list of conditions and the following disclaimer. 00053 * 2. Redistributions in binary form must reproduce the above copyright notice, 00054 * this list of conditions and the following disclaimer in the documentation 00055 * and/or other materials provided with the distribution. 00056 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00057 * may be used to endorse or promote products derived from this software 00058 * without specific prior written permission. 00059 * 00060 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00061 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00062 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00063 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00064 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00065 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00066 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00067 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00068 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00069 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00070 * 00071 ****************************************************************************** 00072 */ 00073 00074 /* Includes ------------------------------------------------------------------*/ 00075 #include "stm32756g_eval_lcd.h" 00076 #include "../../../Utilities/Fonts/fonts.h" 00077 #include "../../../Utilities/Fonts/font24.c" 00078 #include "../../../Utilities/Fonts/font20.c" 00079 #include "../../../Utilities/Fonts/font16.c" 00080 #include "../../../Utilities/Fonts/font12.c" 00081 #include "../../../Utilities/Fonts/font8.c" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32756G_EVAL 00088 * @{ 00089 */ 00090 00091 /** @addtogroup STM32756G_EVAL_LCD 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM32756G_EVAL_LCD_Private_TypesDefinitions 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32756G_EVAL_LCD_Private_Defines 00103 * @{ 00104 */ 00105 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00106 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32756G_EVAL_LCD_Private_Macros 00112 * @{ 00113 */ 00114 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00115 /** 00116 * @} 00117 */ 00118 00119 /** @defgroup STM32756G_EVAL_LCD_Private_Variables 00120 * @{ 00121 */ 00122 static LTDC_HandleTypeDef hLtdcEval; 00123 static DMA2D_HandleTypeDef hDma2dEval; 00124 00125 /* Default LCD configuration with LCD Layer 1 */ 00126 static uint32_t ActiveLayer = 0; 00127 static LCD_DrawPropTypeDef DrawProp[MAX_LAYER_NUMBER]; 00128 /** 00129 * @} 00130 */ 00131 00132 /** @defgroup STM32756G_EVAL_LCD_Private_FunctionPrototypes 00133 * @{ 00134 */ 00135 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00136 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00137 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00138 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00139 /** 00140 * @} 00141 */ 00142 00143 /** @defgroup STM32756G_EVAL_LCD_Private_Functions 00144 * @{ 00145 */ 00146 00147 /** 00148 * @brief Initializes the LCD. 00149 * @retval LCD state 00150 */ 00151 uint8_t BSP_LCD_Init(void) 00152 { 00153 /* Select the used LCD */ 00154 /* The AMPIRE 480x272 does not contain an ID register then we check the availability 00155 of AMPIRE 480x640 LCD using device ID of the STMPE811 mounted on MB1046 daughter board */ 00156 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00157 { 00158 /* The AMPIRE LCD 480x272 is selected */ 00159 /* Timing Configuration */ 00160 hLtdcEval.Init.HorizontalSync = (AMPIRE480272_HSYNC - 1); 00161 hLtdcEval.Init.VerticalSync = (AMPIRE480272_VSYNC - 1); 00162 hLtdcEval.Init.AccumulatedHBP = (AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00163 hLtdcEval.Init.AccumulatedVBP = (AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00164 hLtdcEval.Init.AccumulatedActiveH = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00165 hLtdcEval.Init.AccumulatedActiveW = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00166 hLtdcEval.Init.TotalHeigh = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP + AMPIRE480272_VFP - 1); 00167 hLtdcEval.Init.TotalWidth = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP + AMPIRE480272_HFP - 1); 00168 00169 /* LCD clock configuration */ 00170 BSP_LCD_ClockConfig(&hLtdcEval, NULL); 00171 00172 /* Initialize the LCD pixel width and pixel height */ 00173 hLtdcEval.LayerCfg->ImageWidth = AMPIRE480272_WIDTH; 00174 hLtdcEval.LayerCfg->ImageHeight = AMPIRE480272_HEIGHT; 00175 } 00176 else 00177 { 00178 /* The LCD AMPIRE 640x480 is selected */ 00179 /* Timing configuration */ 00180 hLtdcEval.Init.HorizontalSync = (AMPIRE640480_HSYNC - 1); 00181 hLtdcEval.Init.VerticalSync = (AMPIRE640480_VSYNC - 1); 00182 hLtdcEval.Init.AccumulatedHBP = (AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00183 hLtdcEval.Init.AccumulatedVBP = (AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00184 hLtdcEval.Init.AccumulatedActiveH = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00185 hLtdcEval.Init.AccumulatedActiveW = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00186 hLtdcEval.Init.TotalHeigh = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP + AMPIRE640480_VFP - 1); 00187 hLtdcEval.Init.TotalWidth = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP + AMPIRE640480_HFP - 1); 00188 00189 /* LCD clock configuration */ 00190 BSP_LCD_ClockConfig(&hLtdcEval, NULL); 00191 00192 /* Initialize the LCD pixel width and pixel height */ 00193 hLtdcEval.LayerCfg->ImageWidth = AMPIRE640480_WIDTH; 00194 hLtdcEval.LayerCfg->ImageHeight = AMPIRE640480_HEIGHT; 00195 } 00196 00197 /* Background value */ 00198 hLtdcEval.Init.Backcolor.Blue = 0; 00199 hLtdcEval.Init.Backcolor.Green = 0; 00200 hLtdcEval.Init.Backcolor.Red = 0; 00201 00202 /* Polarity */ 00203 hLtdcEval.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00204 hLtdcEval.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00205 hLtdcEval.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00206 hLtdcEval.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00207 hLtdcEval.Instance = LTDC; 00208 00209 if(HAL_LTDC_GetState(&hLtdcEval) == HAL_LTDC_STATE_RESET) 00210 { 00211 /* Initialize the LCD Msp: this __weak function can be rewritten by the application */ 00212 BSP_LCD_MspInit(&hLtdcEval, NULL); 00213 } 00214 HAL_LTDC_Init(&hLtdcEval); 00215 00216 #if !defined(DATA_IN_ExtSDRAM) 00217 /* When DATA_IN_ExtSDRAM define is enabled, the SDRAM will be configured in SystemInit() 00218 function (from system_stm32f7xx.c) before branch to main() routine. In such case, there 00219 is no need to reconfigure the SDRAM within the LCD driver, since it's already initialized. 00220 Otherwise the SDRAM must be configured. */ 00221 BSP_SDRAM_Init(); 00222 #endif 00223 00224 /* Initialize the font */ 00225 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00226 00227 return LCD_OK; 00228 } 00229 00230 /** 00231 * @brief DeInitializes the LCD. 00232 * @retval LCD state 00233 */ 00234 uint8_t BSP_LCD_DeInit(void) 00235 { 00236 /* Initialize the hltdc_eval Instance parameter */ 00237 hLtdcEval.Instance = LTDC; 00238 00239 /* Disable LTDC block */ 00240 __HAL_LTDC_DISABLE(&hLtdcEval); 00241 00242 /* DeInit the LTDC */ 00243 HAL_LTDC_DeInit(&hLtdcEval); 00244 00245 /* DeInit the LTDC MSP : this __weak function can be rewritten by the application */ 00246 BSP_LCD_MspDeInit(&hLtdcEval, NULL); 00247 00248 return LCD_OK; 00249 } 00250 00251 /** 00252 * @brief Gets the LCD X size. 00253 * @retval Used LCD X size 00254 */ 00255 uint32_t BSP_LCD_GetXSize(void) 00256 { 00257 return hLtdcEval.LayerCfg[ActiveLayer].ImageWidth; 00258 } 00259 00260 /** 00261 * @brief Gets the LCD Y size. 00262 * @retval Used LCD Y size 00263 */ 00264 uint32_t BSP_LCD_GetYSize(void) 00265 { 00266 return hLtdcEval.LayerCfg[ActiveLayer].ImageHeight; 00267 } 00268 00269 /** 00270 * @brief Set the LCD X size. 00271 * @param imageWidthPixels : image width in pixels unit 00272 * @retval None 00273 */ 00274 void BSP_LCD_SetXSize(uint32_t imageWidthPixels) 00275 { 00276 hLtdcEval.LayerCfg[ActiveLayer].ImageWidth = imageWidthPixels; 00277 } 00278 00279 /** 00280 * @brief Set the LCD Y size. 00281 * @param imageHeightPixels : image height in lines unit 00282 * @retval None 00283 */ 00284 void BSP_LCD_SetYSize(uint32_t imageHeightPixels) 00285 { 00286 hLtdcEval.LayerCfg[ActiveLayer].ImageHeight = imageHeightPixels; 00287 } 00288 00289 /** 00290 * @brief Initializes the LCD layers. 00291 * @param LayerIndex: Layer foreground or background 00292 * @param FB_Address: Layer frame buffer 00293 * @retval None 00294 */ 00295 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00296 { 00297 LCD_LayerCfgTypeDef layer_cfg; 00298 00299 /* Layer Init */ 00300 layer_cfg.WindowX0 = 0; 00301 layer_cfg.WindowX1 = BSP_LCD_GetXSize(); 00302 layer_cfg.WindowY0 = 0; 00303 layer_cfg.WindowY1 = BSP_LCD_GetYSize(); 00304 layer_cfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00305 layer_cfg.FBStartAdress = FB_Address; 00306 layer_cfg.Alpha = 255; 00307 layer_cfg.Alpha0 = 0; 00308 layer_cfg.Backcolor.Blue = 0; 00309 layer_cfg.Backcolor.Green = 0; 00310 layer_cfg.Backcolor.Red = 0; 00311 layer_cfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00312 layer_cfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00313 layer_cfg.ImageWidth = BSP_LCD_GetXSize(); 00314 layer_cfg.ImageHeight = BSP_LCD_GetYSize(); 00315 00316 HAL_LTDC_ConfigLayer(&hLtdcEval, &layer_cfg, LayerIndex); 00317 00318 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00319 DrawProp[LayerIndex].pFont = &Font24; 00320 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00321 } 00322 00323 /** 00324 * @brief Selects the LCD Layer. 00325 * @param LayerIndex: Layer foreground or background 00326 * @retval None 00327 */ 00328 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00329 { 00330 ActiveLayer = LayerIndex; 00331 } 00332 00333 /** 00334 * @brief Sets an LCD Layer visible 00335 * @param LayerIndex: Visible Layer 00336 * @param State: New state of the specified layer 00337 * This parameter can be one of the following values: 00338 * @arg ENABLE 00339 * @arg DISABLE 00340 * @retval None 00341 */ 00342 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00343 { 00344 if(State == ENABLE) 00345 { 00346 __HAL_LTDC_LAYER_ENABLE(&hLtdcEval, LayerIndex); 00347 } 00348 else 00349 { 00350 __HAL_LTDC_LAYER_DISABLE(&hLtdcEval, LayerIndex); 00351 } 00352 __HAL_LTDC_RELOAD_CONFIG(&hLtdcEval); 00353 } 00354 00355 /** 00356 * @brief Configures the transparency. 00357 * @param LayerIndex: Layer foreground or background. 00358 * @param Transparency: Transparency 00359 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00360 * @retval None 00361 */ 00362 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00363 { 00364 HAL_LTDC_SetAlpha(&hLtdcEval, Transparency, LayerIndex); 00365 } 00366 00367 /** 00368 * @brief Sets an LCD layer frame buffer address. 00369 * @param LayerIndex: Layer foreground or background 00370 * @param Address: New LCD frame buffer value 00371 * @retval None 00372 */ 00373 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00374 { 00375 HAL_LTDC_SetAddress(&hLtdcEval, Address, LayerIndex); 00376 } 00377 00378 /** 00379 * @brief Sets display window. 00380 * @param LayerIndex: Layer index 00381 * @param Xpos: LCD X position 00382 * @param Ypos: LCD Y position 00383 * @param Width: LCD window width 00384 * @param Height: LCD window height 00385 * @retval None 00386 */ 00387 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00388 { 00389 /* Reconfigure the layer size */ 00390 HAL_LTDC_SetWindowSize(&hLtdcEval, Width, Height, LayerIndex); 00391 00392 /* Reconfigure the layer position */ 00393 HAL_LTDC_SetWindowPosition(&hLtdcEval, Xpos, Ypos, LayerIndex); 00394 } 00395 00396 /** 00397 * @brief Configures and sets the color keying. 00398 * @param LayerIndex: Layer foreground or background 00399 * @param RGBValue: Color reference 00400 * @retval None 00401 */ 00402 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00403 { 00404 /* Configure and Enable the color Keying for LCD Layer */ 00405 HAL_LTDC_ConfigColorKeying(&hLtdcEval, RGBValue, LayerIndex); 00406 HAL_LTDC_EnableColorKeying(&hLtdcEval, LayerIndex); 00407 } 00408 00409 /** 00410 * @brief Disables the color keying. 00411 * @param LayerIndex: Layer foreground or background 00412 * @retval None 00413 */ 00414 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00415 { 00416 /* Disable the color Keying for LCD Layer */ 00417 HAL_LTDC_DisableColorKeying(&hLtdcEval, LayerIndex); 00418 } 00419 00420 /** 00421 * @brief Sets the LCD text color. 00422 * @param Color: Text color code ARGB(8-8-8-8) 00423 * @retval None 00424 */ 00425 void BSP_LCD_SetTextColor(uint32_t Color) 00426 { 00427 DrawProp[ActiveLayer].TextColor = Color; 00428 } 00429 00430 /** 00431 * @brief Gets the LCD text color. 00432 * @retval Used text color. 00433 */ 00434 uint32_t BSP_LCD_GetTextColor(void) 00435 { 00436 return DrawProp[ActiveLayer].TextColor; 00437 } 00438 00439 /** 00440 * @brief Sets the LCD background color. 00441 * @param Color: Layer background color code ARGB(8-8-8-8) 00442 * @retval None 00443 */ 00444 void BSP_LCD_SetBackColor(uint32_t Color) 00445 { 00446 DrawProp[ActiveLayer].BackColor = Color; 00447 } 00448 00449 /** 00450 * @brief Gets the LCD background color. 00451 * @retval Used background color 00452 */ 00453 uint32_t BSP_LCD_GetBackColor(void) 00454 { 00455 return DrawProp[ActiveLayer].BackColor; 00456 } 00457 00458 /** 00459 * @brief Sets the LCD text font. 00460 * @param fonts: Layer font to be used 00461 * @retval None 00462 */ 00463 void BSP_LCD_SetFont(sFONT *fonts) 00464 { 00465 DrawProp[ActiveLayer].pFont = fonts; 00466 } 00467 00468 /** 00469 * @brief Gets the LCD text font. 00470 * @retval Used layer font 00471 */ 00472 sFONT *BSP_LCD_GetFont(void) 00473 { 00474 return DrawProp[ActiveLayer].pFont; 00475 } 00476 00477 /** 00478 * @brief Reads an LCD pixel. 00479 * @param Xpos: X position 00480 * @param Ypos: Y position 00481 * @retval RGB pixel color 00482 */ 00483 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00484 { 00485 uint32_t ret = 0; 00486 00487 if(hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00488 { 00489 /* Read data value from SDRAM memory */ 00490 ret = *(__IO uint32_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00491 } 00492 else if(hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00493 { 00494 /* Read data value from SDRAM memory */ 00495 ret = (*(__IO uint32_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00496 } 00497 else if((hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00498 (hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00499 (hLtdcEval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00500 { 00501 /* Read data value from SDRAM memory */ 00502 ret = *(__IO uint16_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00503 } 00504 else 00505 { 00506 /* Read data value from SDRAM memory */ 00507 ret = *(__IO uint8_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00508 } 00509 00510 return ret; 00511 } 00512 00513 /** 00514 * @brief Clears the hole LCD. 00515 * @param Color: Color of the background 00516 * @retval None 00517 */ 00518 void BSP_LCD_Clear(uint32_t Color) 00519 { 00520 /* Clear the LCD */ 00521 LL_FillBuffer(ActiveLayer, (uint32_t *)(hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00522 } 00523 00524 /** 00525 * @brief Clears the selected line. 00526 * @param Line: Line to be cleared 00527 * @retval None 00528 */ 00529 void BSP_LCD_ClearStringLine(uint32_t Line) 00530 { 00531 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00532 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00533 00534 /* Draw rectangle with background color */ 00535 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00536 00537 DrawProp[ActiveLayer].TextColor = color_backup; 00538 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00539 } 00540 00541 /** 00542 * @brief Displays one character. 00543 * @param Xpos: Start column address 00544 * @param Ypos: Line where to display the character shape. 00545 * @param Ascii: Character ascii code 00546 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00547 * @retval None 00548 */ 00549 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00550 { 00551 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00552 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00553 } 00554 00555 /** 00556 * @brief Displays characters on the LCD. 00557 * @param Xpos: X position (in pixel) 00558 * @param Ypos: Y position (in pixel) 00559 * @param Text: Pointer to string to display on LCD 00560 * @param Mode: Display mode 00561 * This parameter can be one of the following values: 00562 * @arg CENTER_MODE 00563 * @arg RIGHT_MODE 00564 * @arg LEFT_MODE 00565 * @retval None 00566 */ 00567 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00568 { 00569 uint16_t ref_column = 1, i = 0; 00570 uint32_t size = 0, xsize = 0; 00571 uint8_t *ptr = Text; 00572 00573 /* Get the text size */ 00574 while (*ptr++) size ++ ; 00575 00576 /* Characters number per line */ 00577 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00578 00579 switch (Mode) 00580 { 00581 case CENTER_MODE: 00582 { 00583 ref_column = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00584 break; 00585 } 00586 case LEFT_MODE: 00587 { 00588 ref_column = Xpos; 00589 break; 00590 } 00591 case RIGHT_MODE: 00592 { 00593 ref_column = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00594 break; 00595 } 00596 default: 00597 { 00598 ref_column = Xpos; 00599 break; 00600 } 00601 } 00602 00603 /* Check that the Start column is located in the screen */ 00604 if ((ref_column < 1) || (ref_column >= 0x8000)) 00605 { 00606 ref_column = 1; 00607 } 00608 00609 /* Send the string character by character on LCD */ 00610 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00611 { 00612 /* Display one character on LCD */ 00613 BSP_LCD_DisplayChar(ref_column, Ypos, *Text); 00614 /* Decrement the column position by 16 */ 00615 ref_column += DrawProp[ActiveLayer].pFont->Width; 00616 /* Point on the next character */ 00617 Text++; 00618 i++; 00619 } 00620 } 00621 00622 /** 00623 * @brief Displays a maximum of 60 characters on the LCD. 00624 * @param Line: Line where to display the character shape 00625 * @param ptr: Pointer to string to display on LCD 00626 * @retval None 00627 */ 00628 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00629 { 00630 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00631 } 00632 00633 /** 00634 * @brief Draws an horizontal line. 00635 * @param Xpos: X position 00636 * @param Ypos: Y position 00637 * @param Length: Line length 00638 * @retval None 00639 */ 00640 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00641 { 00642 uint32_t Xaddress = 0; 00643 00644 /* Get the line address */ 00645 Xaddress = (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00646 00647 /* Write line */ 00648 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00649 } 00650 00651 /** 00652 * @brief Draws a vertical line. 00653 * @param Xpos: X position 00654 * @param Ypos: Y position 00655 * @param Length: Line length 00656 * @retval None 00657 */ 00658 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00659 { 00660 uint32_t Xaddress = 0; 00661 00662 /* Get the line address */ 00663 Xaddress = (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00664 00665 /* Write line */ 00666 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00667 } 00668 00669 /** 00670 * @brief Draws an uni-line (between two points). 00671 * @param x1: Point 1 X position 00672 * @param y1: Point 1 Y position 00673 * @param x2: Point 2 X position 00674 * @param y2: Point 2 Y position 00675 * @retval None 00676 */ 00677 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00678 { 00679 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00680 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 00681 curpixel = 0; 00682 00683 deltax = ABS(x2 - x1); /* The difference between the x's */ 00684 deltay = ABS(y2 - y1); /* The difference between the y's */ 00685 x = x1; /* Start x off at the first pixel */ 00686 y = y1; /* Start y off at the first pixel */ 00687 00688 if (x2 >= x1) /* The x-values are increasing */ 00689 { 00690 xinc1 = 1; 00691 xinc2 = 1; 00692 } 00693 else /* The x-values are decreasing */ 00694 { 00695 xinc1 = -1; 00696 xinc2 = -1; 00697 } 00698 00699 if (y2 >= y1) /* The y-values are increasing */ 00700 { 00701 yinc1 = 1; 00702 yinc2 = 1; 00703 } 00704 else /* The y-values are decreasing */ 00705 { 00706 yinc1 = -1; 00707 yinc2 = -1; 00708 } 00709 00710 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00711 { 00712 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00713 yinc2 = 0; /* Don't change the y for every iteration */ 00714 den = deltax; 00715 num = deltax / 2; 00716 num_add = deltay; 00717 num_pixels = deltax; /* There are more x-values than y-values */ 00718 } 00719 else /* There is at least one y-value for every x-value */ 00720 { 00721 xinc2 = 0; /* Don't change the x for every iteration */ 00722 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00723 den = deltay; 00724 num = deltay / 2; 00725 num_add = deltax; 00726 num_pixels = deltay; /* There are more y-values than x-values */ 00727 } 00728 00729 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 00730 { 00731 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00732 num += num_add; /* Increase the numerator by the top of the fraction */ 00733 if (num >= den) /* Check if numerator >= denominator */ 00734 { 00735 num -= den; /* Calculate the new numerator value */ 00736 x += xinc1; /* Change the x as appropriate */ 00737 y += yinc1; /* Change the y as appropriate */ 00738 } 00739 x += xinc2; /* Change the x as appropriate */ 00740 y += yinc2; /* Change the y as appropriate */ 00741 } 00742 } 00743 00744 /** 00745 * @brief Draws a rectangle. 00746 * @param Xpos: X position 00747 * @param Ypos: Y position 00748 * @param Width: Rectangle width 00749 * @param Height: Rectangle height 00750 * @retval None 00751 */ 00752 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00753 { 00754 /* Draw horizontal lines */ 00755 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00756 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00757 00758 /* Draw vertical lines */ 00759 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00760 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00761 } 00762 00763 /** 00764 * @brief Draws a circle. 00765 * @param Xpos: X position 00766 * @param Ypos: Y position 00767 * @param Radius: Circle radius 00768 * @retval None 00769 */ 00770 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00771 { 00772 int32_t decision; /* Decision Variable */ 00773 uint32_t current_x; /* Current X Value */ 00774 uint32_t current_y; /* Current Y Value */ 00775 00776 decision = 3 - (Radius << 1); 00777 current_x = 0; 00778 current_y = Radius; 00779 00780 while (current_x <= current_y) 00781 { 00782 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00783 00784 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp[ActiveLayer].TextColor); 00785 00786 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00787 00788 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp[ActiveLayer].TextColor); 00789 00790 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00791 00792 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp[ActiveLayer].TextColor); 00793 00794 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00795 00796 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp[ActiveLayer].TextColor); 00797 00798 if (decision < 0) 00799 { 00800 decision += (current_x << 2) + 6; 00801 } 00802 else 00803 { 00804 decision += ((current_x - current_y) << 2) + 10; 00805 current_y--; 00806 } 00807 current_x++; 00808 } 00809 } 00810 00811 /** 00812 * @brief Draws an poly-line (between many points). 00813 * @param Points: Pointer to the points array 00814 * @param PointCount: Number of points 00815 * @retval None 00816 */ 00817 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00818 { 00819 int16_t x = 0, y = 0; 00820 00821 if(PointCount < 2) 00822 { 00823 return; 00824 } 00825 00826 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00827 00828 while(--PointCount) 00829 { 00830 x = Points->X; 00831 y = Points->Y; 00832 Points++; 00833 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00834 } 00835 } 00836 00837 /** 00838 * @brief Draws an ellipse on LCD. 00839 * @param Xpos: X position 00840 * @param Ypos: Y position 00841 * @param XRadius: Ellipse X radius 00842 * @param YRadius: Ellipse Y radius 00843 * @retval None 00844 */ 00845 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00846 { 00847 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00848 float k = 0, rad1 = 0, rad2 = 0; 00849 00850 rad1 = XRadius; 00851 rad2 = YRadius; 00852 00853 k = (float)(rad2/rad1); 00854 00855 do { 00856 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00857 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00858 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00859 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00860 00861 e2 = err; 00862 if (e2 <= x) { 00863 err += ++x*2+1; 00864 if (-y == x && e2 <= y) e2 = 0; 00865 } 00866 if (e2 > y) err += ++y*2+1; 00867 } 00868 while (y <= 0); 00869 } 00870 00871 /** 00872 * @brief Draws a pixel on LCD. 00873 * @param Xpos: X position 00874 * @param Ypos: Y position 00875 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 00876 * @retval None 00877 */ 00878 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 00879 { 00880 /* Write data value to all SDRAM memory */ 00881 *(__IO uint32_t*) (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 00882 } 00883 00884 /** 00885 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00886 * @param Xpos: Bmp X position in the LCD 00887 * @param Ypos: Bmp Y position in the LCD 00888 * @param pbmp: Pointer to Bmp picture address in the internal Flash 00889 * @retval None 00890 */ 00891 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 00892 { 00893 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 00894 uint32_t address; 00895 uint32_t input_color_mode = 0; 00896 00897 /* Get bitmap data address offset */ 00898 index = *(__IO uint16_t *) (pbmp + 10); 00899 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 00900 00901 /* Read bitmap width */ 00902 width = *(uint16_t *) (pbmp + 18); 00903 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00904 00905 /* Read bitmap height */ 00906 height = *(uint16_t *) (pbmp + 22); 00907 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00908 00909 /* Read bit/pixel */ 00910 bit_pixel = *(uint16_t *) (pbmp + 28); 00911 00912 /* Set the address */ 00913 address = hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Ypos) + Xpos)*(4)); 00914 00915 /* Get the layer pixel format */ 00916 if ((bit_pixel/8) == 4) 00917 { 00918 input_color_mode = CM_ARGB8888; 00919 } 00920 else if ((bit_pixel/8) == 2) 00921 { 00922 input_color_mode = CM_RGB565; 00923 } 00924 else 00925 { 00926 input_color_mode = CM_RGB888; 00927 } 00928 00929 /* Bypass the bitmap header */ 00930 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 00931 00932 /* Convert picture to ARGB8888 pixel format */ 00933 for(index=0; index < height; index++) 00934 { 00935 /* Pixel format conversion */ 00936 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)address, width, input_color_mode); 00937 00938 /* Increment the source and destination buffers */ 00939 address+= (BSP_LCD_GetXSize()*4); 00940 pbmp -= width*(bit_pixel/8); 00941 } 00942 } 00943 00944 /** 00945 * @brief Draws a full rectangle. 00946 * @param Xpos: X position 00947 * @param Ypos: Y position 00948 * @param Width: Rectangle width 00949 * @param Height: Rectangle height 00950 * @retval None 00951 */ 00952 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00953 { 00954 uint32_t x_address = 0; 00955 00956 /* Set the text color */ 00957 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00958 00959 /* Get the rectangle start address */ 00960 x_address = (hLtdcEval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00961 00962 /* Fill the rectangle */ 00963 LL_FillBuffer(ActiveLayer, (uint32_t *)x_address, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 00964 } 00965 00966 /** 00967 * @brief Draws a full circle. 00968 * @param Xpos: X position 00969 * @param Ypos: Y position 00970 * @param Radius: Circle radius 00971 * @retval None 00972 */ 00973 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00974 { 00975 int32_t decision; /* Decision Variable */ 00976 uint32_t current_x; /* Current X Value */ 00977 uint32_t current_y; /* Current Y Value */ 00978 00979 decision = 3 - (Radius << 1); 00980 00981 current_x = 0; 00982 current_y = Radius; 00983 00984 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00985 00986 while (current_x <= current_y) 00987 { 00988 if(current_y > 0) 00989 { 00990 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 00991 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 00992 } 00993 00994 if(current_x > 0) 00995 { 00996 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 00997 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 00998 } 00999 if (decision < 0) 01000 { 01001 decision += (current_x << 2) + 6; 01002 } 01003 else 01004 { 01005 decision += ((current_x - current_y) << 2) + 10; 01006 current_y--; 01007 } 01008 current_x++; 01009 } 01010 01011 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 01012 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 01013 } 01014 01015 /** 01016 * @brief Draws a full poly-line (between many points). 01017 * @param Points: Pointer to the points array 01018 * @param PointCount: Number of points 01019 * @retval None 01020 */ 01021 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01022 { 01023 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 01024 uint16_t image_left = 0, image_right = 0, image_top = 0, image_bottom = 0; 01025 01026 image_left = image_right = Points->X; 01027 image_top= image_bottom = Points->Y; 01028 01029 for(counter = 1; counter < PointCount; counter++) 01030 { 01031 pixelX = POLY_X(counter); 01032 if(pixelX < image_left) 01033 { 01034 image_left = pixelX; 01035 } 01036 if(pixelX > image_right) 01037 { 01038 image_right = pixelX; 01039 } 01040 01041 pixelY = POLY_Y(counter); 01042 if(pixelY < image_top) 01043 { 01044 image_top = pixelY; 01045 } 01046 if(pixelY > image_bottom) 01047 { 01048 image_bottom = pixelY; 01049 } 01050 } 01051 01052 if(PointCount < 2) 01053 { 01054 return; 01055 } 01056 01057 X_center = (image_left + image_right)/2; 01058 Y_center = (image_bottom + image_top)/2; 01059 01060 X_first = Points->X; 01061 Y_first = Points->Y; 01062 01063 while(--PointCount) 01064 { 01065 X = Points->X; 01066 Y = Points->Y; 01067 Points++; 01068 X2 = Points->X; 01069 Y2 = Points->Y; 01070 01071 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 01072 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 01073 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 01074 } 01075 01076 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 01077 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 01078 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 01079 } 01080 01081 /** 01082 * @brief Draws a full ellipse. 01083 * @param Xpos: X position 01084 * @param Ypos: Y position 01085 * @param XRadius: Ellipse X radius 01086 * @param YRadius: Ellipse Y radius 01087 * @retval None 01088 */ 01089 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01090 { 01091 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01092 float k = 0, rad1 = 0, rad2 = 0; 01093 01094 rad1 = XRadius; 01095 rad2 = YRadius; 01096 01097 k = (float)(rad2/rad1); 01098 01099 do 01100 { 01101 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 01102 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 01103 01104 e2 = err; 01105 if (e2 <= x) 01106 { 01107 err += ++x*2+1; 01108 if (-y == x && e2 <= y) e2 = 0; 01109 } 01110 if (e2 > y) err += ++y*2+1; 01111 } 01112 while (y <= 0); 01113 } 01114 01115 /** 01116 * @brief Enables the display. 01117 * @retval None 01118 */ 01119 void BSP_LCD_DisplayOn(void) 01120 { 01121 /* Display On */ 01122 __HAL_LTDC_ENABLE(&hLtdcEval); 01123 } 01124 01125 /** 01126 * @brief Disables the display. 01127 * @retval None 01128 */ 01129 void BSP_LCD_DisplayOff(void) 01130 { 01131 /* Display Off */ 01132 __HAL_LTDC_DISABLE(&hLtdcEval); 01133 } 01134 01135 /** 01136 * @brief Initializes the LTDC MSP. 01137 * @param hltdc: LTDC handle 01138 * @retval None 01139 */ 01140 __weak void BSP_LCD_MspInit(LTDC_HandleTypeDef *hltdc, void *Params) 01141 { 01142 GPIO_InitTypeDef gpio_init_structure; 01143 01144 /* Enable the LTDC and DMA2D clocks */ 01145 __HAL_RCC_LTDC_CLK_ENABLE(); 01146 __HAL_RCC_DMA2D_CLK_ENABLE(); 01147 01148 /* Enable GPIOs clock */ 01149 __HAL_RCC_GPIOI_CLK_ENABLE(); 01150 __HAL_RCC_GPIOJ_CLK_ENABLE(); 01151 __HAL_RCC_GPIOK_CLK_ENABLE(); 01152 01153 /*** LTDC Pins configuration ***/ 01154 /* GPIOI configuration */ 01155 gpio_init_structure.Pin = GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01156 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01157 gpio_init_structure.Pull = GPIO_NOPULL; 01158 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01159 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01160 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 01161 01162 /* GPIOJ configuration */ 01163 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01164 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01165 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01166 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01167 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01168 gpio_init_structure.Pull = GPIO_NOPULL; 01169 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01170 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01171 HAL_GPIO_Init(GPIOJ, &gpio_init_structure); 01172 01173 /* GPIOK configuration */ 01174 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01175 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01176 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 01177 gpio_init_structure.Pull = GPIO_NOPULL; 01178 gpio_init_structure.Speed = GPIO_SPEED_FAST; 01179 gpio_init_structure.Alternate = GPIO_AF14_LTDC; 01180 HAL_GPIO_Init(GPIOK, &gpio_init_structure); 01181 } 01182 01183 /** 01184 * @brief DeInitializes BSP_LCD MSP. 01185 * @param hltdc: LTDC handle 01186 * @retval None 01187 */ 01188 __weak void BSP_LCD_MspDeInit(LTDC_HandleTypeDef *hltdc, void *Params) 01189 { 01190 GPIO_InitTypeDef gpio_init_structure; 01191 01192 /* Disable LTDC block */ 01193 __HAL_LTDC_DISABLE(hltdc); 01194 01195 /* LTDC Pins deactivation */ 01196 /* GPIOI deactivation */ 01197 gpio_init_structure.Pin = GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01198 HAL_GPIO_DeInit(GPIOI, gpio_init_structure.Pin); 01199 /* GPIOJ deactivation */ 01200 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01201 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01202 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01203 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01204 HAL_GPIO_DeInit(GPIOJ, gpio_init_structure.Pin); 01205 /* GPIOK deactivation */ 01206 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01207 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01208 HAL_GPIO_DeInit(GPIOK, gpio_init_structure.Pin); 01209 01210 /* Disable LTDC clock */ 01211 __HAL_RCC_LTDC_CLK_DISABLE(); 01212 01213 /* GPIO pins clock can be shut down in the application 01214 by surcharging this __weak function */ 01215 } 01216 01217 /** 01218 * @brief Clock Config. 01219 * @param hltdc: LTDC handle 01220 * @note This API is called by BSP_LCD_Init() 01221 * Being __weak it can be overwritten by the application 01222 * @retval None 01223 */ 01224 __weak void BSP_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params) 01225 { 01226 static RCC_PeriphCLKInitTypeDef periph_clk_init_struct; 01227 01228 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 01229 { 01230 /* AMPIRE480272 LCD clock configuration */ 01231 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01232 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 192 Mhz */ 01233 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 192/5 = 38.4 Mhz */ 01234 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_4 = 38.4/4 = 9.6Mhz */ 01235 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01236 periph_clk_init_struct.PLLSAI.PLLSAIN = 192; 01237 periph_clk_init_struct.PLLSAI.PLLSAIR = AMPIRE480272_FREQUENCY_DIVIDER; 01238 periph_clk_init_struct.PLLSAIDivR = RCC_PLLSAIDIVR_4; 01239 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01240 } 01241 else 01242 { 01243 /* AMPIRE640480 LCD clock configuration */ 01244 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01245 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 151 Mhz */ 01246 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 151/3 = 50.3 Mhz */ 01247 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_2 = 50.3/2 = 25.1 Mhz */ 01248 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01249 periph_clk_init_struct.PLLSAI.PLLSAIN = 151; 01250 periph_clk_init_struct.PLLSAI.PLLSAIR = AMPIRE640480_FREQUENCY_DIVIDER; 01251 periph_clk_init_struct.PLLSAIDivR = RCC_PLLSAIDIVR_2; 01252 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01253 } 01254 } 01255 01256 01257 /******************************************************************************* 01258 Static Functions 01259 *******************************************************************************/ 01260 01261 /** 01262 * @brief Draws a character on LCD. 01263 * @param Xpos: Line where to display the character shape 01264 * @param Ypos: Start column address 01265 * @param c: Pointer to the character data 01266 * @retval None 01267 */ 01268 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01269 { 01270 uint32_t i = 0, j = 0; 01271 uint16_t height, width; 01272 uint8_t offset; 01273 uint8_t *pchar; 01274 uint32_t line; 01275 01276 height = DrawProp[ActiveLayer].pFont->Height; 01277 width = DrawProp[ActiveLayer].pFont->Width; 01278 01279 offset = 8 *((width + 7)/8) - width ; 01280 01281 for(i = 0; i < height; i++) 01282 { 01283 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01284 01285 switch(((width + 7)/8)) 01286 { 01287 01288 case 1: 01289 line = pchar[0]; 01290 break; 01291 01292 case 2: 01293 line = (pchar[0]<< 8) | pchar[1]; 01294 break; 01295 01296 case 3: 01297 default: 01298 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01299 break; 01300 } 01301 01302 for (j = 0; j < width; j++) 01303 { 01304 if(line & (1 << (width- j + offset- 1))) 01305 { 01306 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01307 } 01308 else 01309 { 01310 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01311 } 01312 } 01313 Ypos++; 01314 } 01315 } 01316 01317 /** 01318 * @brief Fills a triangle (between 3 points). 01319 * @param Points: Pointer to the points array 01320 * @param x1: Point 1 X position 01321 * @param y1: Point 1 Y position 01322 * @param x2: Point 2 X position 01323 * @param y2: Point 2 Y position 01324 * @param x3: Point 3 X position 01325 * @param y3: Point 3 Y position 01326 * @retval None 01327 */ 01328 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01329 { 01330 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01331 yinc1 = 0, yinc2 = 0, den = 0, num = 0, num_add = 0, num_pixels = 0, 01332 curpixel = 0; 01333 01334 deltax = ABS(x2 - x1); /* The difference between the x's */ 01335 deltay = ABS(y2 - y1); /* The difference between the y's */ 01336 x = x1; /* Start x off at the first pixel */ 01337 y = y1; /* Start y off at the first pixel */ 01338 01339 if (x2 >= x1) /* The x-values are increasing */ 01340 { 01341 xinc1 = 1; 01342 xinc2 = 1; 01343 } 01344 else /* The x-values are decreasing */ 01345 { 01346 xinc1 = -1; 01347 xinc2 = -1; 01348 } 01349 01350 if (y2 >= y1) /* The y-values are increasing */ 01351 { 01352 yinc1 = 1; 01353 yinc2 = 1; 01354 } 01355 else /* The y-values are decreasing */ 01356 { 01357 yinc1 = -1; 01358 yinc2 = -1; 01359 } 01360 01361 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01362 { 01363 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01364 yinc2 = 0; /* Don't change the y for every iteration */ 01365 den = deltax; 01366 num = deltax / 2; 01367 num_add = deltay; 01368 num_pixels = deltax; /* There are more x-values than y-values */ 01369 } 01370 else /* There is at least one y-value for every x-value */ 01371 { 01372 xinc2 = 0; /* Don't change the x for every iteration */ 01373 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01374 den = deltay; 01375 num = deltay / 2; 01376 num_add = deltax; 01377 num_pixels = deltay; /* There are more y-values than x-values */ 01378 } 01379 01380 for (curpixel = 0; curpixel <= num_pixels; curpixel++) 01381 { 01382 BSP_LCD_DrawLine(x, y, x3, y3); 01383 01384 num += num_add; /* Increase the numerator by the top of the fraction */ 01385 if (num >= den) /* Check if numerator >= denominator */ 01386 { 01387 num -= den; /* Calculate the new numerator value */ 01388 x += xinc1; /* Change the x as appropriate */ 01389 y += yinc1; /* Change the y as appropriate */ 01390 } 01391 x += xinc2; /* Change the x as appropriate */ 01392 y += yinc2; /* Change the y as appropriate */ 01393 } 01394 } 01395 01396 /** 01397 * @brief Fills a buffer. 01398 * @param LayerIndex: Layer index 01399 * @param pDst: Pointer to destination buffer 01400 * @param xSize: Buffer width 01401 * @param ySize: Buffer height 01402 * @param OffLine: Offset 01403 * @param ColorIndex: Color index 01404 * @retval None 01405 */ 01406 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01407 { 01408 /* Register to memory mode with ARGB8888 as color Mode */ 01409 hDma2dEval.Init.Mode = DMA2D_R2M; 01410 hDma2dEval.Init.ColorMode = DMA2D_ARGB8888; 01411 hDma2dEval.Init.OutputOffset = OffLine; 01412 01413 hDma2dEval.Instance = DMA2D; 01414 01415 /* DMA2D Initialization */ 01416 if(HAL_DMA2D_Init(&hDma2dEval) == HAL_OK) 01417 { 01418 if(HAL_DMA2D_ConfigLayer(&hDma2dEval, LayerIndex) == HAL_OK) 01419 { 01420 if (HAL_DMA2D_Start(&hDma2dEval, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01421 { 01422 /* Polling For DMA transfer */ 01423 HAL_DMA2D_PollForTransfer(&hDma2dEval, 10); 01424 } 01425 } 01426 } 01427 } 01428 01429 /** 01430 * @brief Converts a line to an ARGB8888 pixel format. 01431 * @param pSrc: Pointer to source buffer 01432 * @param pDst: Output color 01433 * @param xSize: Buffer width 01434 * @param ColorMode: Input color mode 01435 * @retval None 01436 */ 01437 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01438 { 01439 /* Configure the DMA2D Mode, Color Mode and output offset */ 01440 hDma2dEval.Init.Mode = DMA2D_M2M_PFC; 01441 hDma2dEval.Init.ColorMode = DMA2D_ARGB8888; 01442 hDma2dEval.Init.OutputOffset = 0; 01443 01444 /* Foreground Configuration */ 01445 hDma2dEval.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01446 hDma2dEval.LayerCfg[1].InputAlpha = 0xFF; 01447 hDma2dEval.LayerCfg[1].InputColorMode = ColorMode; 01448 hDma2dEval.LayerCfg[1].InputOffset = 0; 01449 01450 hDma2dEval.Instance = DMA2D; 01451 01452 /* DMA2D Initialization */ 01453 if(HAL_DMA2D_Init(&hDma2dEval) == HAL_OK) 01454 { 01455 if(HAL_DMA2D_ConfigLayer(&hDma2dEval, 1) == HAL_OK) 01456 { 01457 if (HAL_DMA2D_Start(&hDma2dEval, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01458 { 01459 /* Polling For DMA transfer */ 01460 HAL_DMA2D_PollForTransfer(&hDma2dEval, 10); 01461 } 01462 } 01463 } 01464 } 01465 01466 /** 01467 * @} 01468 */ 01469 01470 /** 01471 * @} 01472 */ 01473 01474 /** 01475 * @} 01476 */ 01477 01478 /** 01479 * @} 01480 */ 01481 01482 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri May 22 2015 13:59:20 for STM32756G_EVAL BSP User Manual by
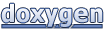