STM32756G_EVAL BSP User Manual
|
stm32756g_eval_eeprom.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval_eeprom.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 22-May-2015 00007 * @brief This file provides a set of functions needed to manage an I2C M24LR64 00008 * EEPROM memory. 00009 @verbatim 00010 To be able to use this driver, the switch EE_M24LR64 must be defined 00011 in your toolchain compiler preprocessor 00012 00013 =================================================================== 00014 Notes: 00015 - The I2C EEPROM memory (M24LR64) is available on separate daughter 00016 board ANT7-M24LR-A, which is not provided with the STM32756G_EVAL 00017 and STM32746G_EVAL boards. 00018 To use this driver you have to connect the ANT7-M24LR-A to CN2 00019 connector of STM32756G_EVAL board. 00020 =================================================================== 00021 00022 It implements a high level communication layer for read and write 00023 from/to this memory. The needed STM32F7xx hardware resources (I2C and 00024 GPIO) are defined in stm32756g_eval.h file, and the initialization is 00025 performed in EEPROM_IO_Init() function declared in stm32756g_eval.c 00026 file. 00027 You can easily tailor this driver to any other development board, 00028 by just adapting the defines for hardware resources and 00029 EEPROM_IO_Init() function. 00030 00031 @note In this driver, basic read and write functions (BSP_EEPROM_ReadBuffer() 00032 and BSP_EEPROM_WritePage()) use DMA mode to perform the data 00033 transfer to/from EEPROM memory. 00034 00035 @note Regarding BSP_EEPROM_WritePage(), it is an optimized function to perform 00036 small write (less than 1 page) BUT the number of bytes (combined to write start address) must not 00037 cross the EEPROM page boundary. This function can only writes into 00038 the boundaries of an EEPROM page. 00039 This function doesn't check on boundaries condition (in this driver 00040 the function BSP_EEPROM_WriteBuffer() which calls BSP_EEPROM_WritePage() is 00041 responsible of checking on Page boundaries). 00042 00043 00044 +-----------------------------------------------------------------+ 00045 | Pin assignment for M24LR64 EEPROM | 00046 +---------------------------------------+-----------+-------------+ 00047 | STM32F7xx I2C Pins | EEPROM | Pin | 00048 +---------------------------------------+-----------+-------------+ 00049 | . | E0(GND) | 1 (0V) | 00050 | . | AC0 | 2 | 00051 | . | AC1 | 3 | 00052 | . | VSS | 4 (0V) | 00053 | SDA | SDA | 5 | 00054 | SCL | SCL | 6 | 00055 | . | E1(GND) | 7 (0V) | 00056 | . | VDD | 8 (3.3V) | 00057 +---------------------------------------+-----------+-------------+ 00058 @endverbatim 00059 ****************************************************************************** 00060 * @attention 00061 * 00062 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00063 * 00064 * Redistribution and use in source and binary forms, with or without modification, 00065 * are permitted provided that the following conditions are met: 00066 * 1. Redistributions of source code must retain the above copyright notice, 00067 * this list of conditions and the following disclaimer. 00068 * 2. Redistributions in binary form must reproduce the above copyright notice, 00069 * this list of conditions and the following disclaimer in the documentation 00070 * and/or other materials provided with the distribution. 00071 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00072 * may be used to endorse or promote products derived from this software 00073 * without specific prior written permission. 00074 * 00075 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00076 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00077 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00078 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00079 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00080 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00081 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00082 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00083 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00084 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00085 * 00086 ****************************************************************************** 00087 */ 00088 /* Includes ------------------------------------------------------------------*/ 00089 #include "stm32756g_eval_eeprom.h" 00090 00091 /** @addtogroup BSP 00092 * @{ 00093 */ 00094 00095 /** @addtogroup STM32756G_EVAL 00096 * @{ 00097 */ 00098 00099 /** @addtogroup STM32756G_EVAL_EEPROM 00100 * @brief This file includes the I2C EEPROM driver of STM32756G-EVAL evaluation board. 00101 * @{ 00102 */ 00103 00104 /** @defgroup STM32756G_EVAL_EEPROM_Private_Types 00105 * @{ 00106 */ 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32756G_EVAL_EEPROM_Private_Defines 00112 * @{ 00113 */ 00114 /** 00115 * @} 00116 */ 00117 00118 /** @defgroup STM32756G_EVAL_EEPROM_Private_Macros 00119 * @{ 00120 */ 00121 /** 00122 * @} 00123 */ 00124 00125 /** @defgroup STM32756G_EVAL_EEPROM_Private_Variables 00126 * @{ 00127 */ 00128 __IO uint16_t EEPROMAddress = 0; 00129 __IO uint16_t EEPROMDataRead; 00130 __IO uint8_t EEPROMDataWrite; 00131 /** 00132 * @} 00133 */ 00134 00135 /** @defgroup STM32756G_EVAL_EEPROM_Private_Function_Prototypes 00136 * @{ 00137 */ 00138 /** 00139 * @} 00140 */ 00141 00142 /** @defgroup STM32756G_EVAL_EEPROM_Private_Functions 00143 * @{ 00144 */ 00145 00146 /** 00147 * @brief Initializes peripherals used by the I2C EEPROM driver. 00148 * @note There are 2 different versions of M24LR64 (A01 & A02). 00149 * Then try to connect on 1st one (EEPROM_I2C_ADDRESS_A01) 00150 * and if problem, check the 2nd one (EEPROM_I2C_ADDRESS_A02) 00151 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00152 * different from EEPROM_OK (0) 00153 */ 00154 uint32_t BSP_EEPROM_Init(void) 00155 { 00156 /* I2C Initialization */ 00157 EEPROM_IO_Init(); 00158 00159 /* Select the EEPROM address for A01 and check if OK */ 00160 EEPROMAddress = EEPROM_I2C_ADDRESS_A01; 00161 if(EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00162 { 00163 /* Select the EEPROM address for A02 and check if OK */ 00164 EEPROMAddress = EEPROM_I2C_ADDRESS_A02; 00165 if(EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00166 { 00167 return EEPROM_FAIL; 00168 } 00169 } 00170 return EEPROM_OK; 00171 } 00172 00173 /** 00174 * @brief DeInitializes the EEPROM. 00175 * @retval EEPROM state 00176 */ 00177 uint8_t BSP_EEPROM_DeInit(void) 00178 { 00179 /* I2C won't be disabled because common to other functionalities */ 00180 return EEPROM_OK; 00181 } 00182 00183 /** 00184 * @brief Reads a block of data from the EEPROM. 00185 * @param pBuffer: pointer to the buffer that receives the data read from 00186 * the EEPROM. 00187 * @param ReadAddr: EEPROM's internal address to start reading from. 00188 * @param NumByteToRead: pointer to the variable holding number of bytes to 00189 * be read from the EEPROM. 00190 * 00191 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00192 * data are read from the EEPROM. Application should monitor this 00193 * variable in order know when the transfer is complete. 00194 * 00195 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00196 * different from EEPROM_OK (0) or the timeout user callback. 00197 */ 00198 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint16_t* NumByteToRead) 00199 { 00200 uint32_t buffersize = *NumByteToRead; 00201 00202 /* Set the pointer to the Number of data to be read. This pointer will be used 00203 by the DMA Transfer Completer interrupt Handler in order to reset the 00204 variable to 0. User should check on this variable in order to know if the 00205 DMA transfer has been complete or not. */ 00206 EEPROMDataRead = *NumByteToRead; 00207 00208 if(EEPROM_IO_ReadData(EEPROMAddress, ReadAddr, pBuffer, buffersize) != HAL_OK) 00209 { 00210 BSP_EEPROM_TIMEOUT_UserCallback(); 00211 return EEPROM_FAIL; 00212 } 00213 00214 /* If all operations OK, return EEPROM_OK (0) */ 00215 return EEPROM_OK; 00216 } 00217 00218 /** 00219 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00220 * 00221 * @note The number of bytes (combined to write start address) must not 00222 * cross the EEPROM page boundary. This function can only write into 00223 * the boundaries of an EEPROM page. 00224 * This function doesn't check on boundaries condition (in this driver 00225 * the function BSP_EEPROM_WriteBuffer() which calls BSP_EEPROM_WritePage() is 00226 * responsible of checking on Page boundaries). 00227 * 00228 * @param pBuffer: pointer to the buffer containing the data to be written to 00229 * the EEPROM. 00230 * @param WriteAddr: EEPROM's internal address to write to. 00231 * @param NumByteToWrite: pointer to the variable holding number of bytes to 00232 * be written into the EEPROM. 00233 * 00234 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00235 * data are written to the EEPROM. Application should monitor this 00236 * variable in order know when the transfer is complete. 00237 * 00238 * @note This function just configure the communication and enable the DMA 00239 * channel to transfer data. Meanwhile, the user application may perform 00240 * other tasks in parallel. 00241 * 00242 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00243 * different from EEPROM_OK (0) or the timeout user callback. 00244 */ 00245 uint32_t BSP_EEPROM_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint8_t* NumByteToWrite) 00246 { 00247 uint32_t buffersize = *NumByteToWrite; 00248 uint32_t status = EEPROM_OK; 00249 00250 /* Set the pointer to the Number of data to be written. This pointer will be used 00251 by the DMA Transfer Completer interrupt Handler in order to reset the 00252 variable to 0. User should check on this variable in order to know if the 00253 DMA transfer has been complete or not. */ 00254 EEPROMDataWrite = *NumByteToWrite; 00255 00256 if(EEPROM_IO_WriteData(EEPROMAddress, WriteAddr, pBuffer, buffersize) != HAL_OK) 00257 { 00258 BSP_EEPROM_TIMEOUT_UserCallback(); 00259 status = EEPROM_FAIL; 00260 } 00261 00262 if(BSP_EEPROM_WaitEepromStandbyState() != EEPROM_OK) 00263 { 00264 return EEPROM_FAIL; 00265 } 00266 00267 /* If all operations OK, return EEPROM_OK (0) */ 00268 return status; 00269 } 00270 00271 /** 00272 * @brief Writes buffer of data to the I2C EEPROM. 00273 * @param pBuffer: pointer to the buffer containing the data to be written 00274 * to the EEPROM. 00275 * @param WriteAddr: EEPROM's internal address to write to. 00276 * @param NumByteToWrite: number of bytes to write to the EEPROM. 00277 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00278 * different from EEPROM_OK (0) or the timeout user callback. 00279 */ 00280 uint32_t BSP_EEPROM_WriteBuffer(uint8_t *pBuffer, uint16_t WriteAddr, uint16_t NumByteToWrite) 00281 { 00282 uint16_t numofpage = 0, numofsingle = 0, count = 0; 00283 uint16_t addr = 0; 00284 uint8_t dataindex = 0; 00285 uint32_t status = EEPROM_OK; 00286 00287 addr = WriteAddr % EEPROM_PAGESIZE; 00288 count = EEPROM_PAGESIZE - addr; 00289 numofpage = NumByteToWrite / EEPROM_PAGESIZE; 00290 numofsingle = NumByteToWrite % EEPROM_PAGESIZE; 00291 00292 /* If WriteAddr is EEPROM_PAGESIZE aligned */ 00293 if(addr == 0) 00294 { 00295 /* If NumByteToWrite < EEPROM_PAGESIZE */ 00296 if(numofpage == 0) 00297 { 00298 /* Store the number of data to be written */ 00299 dataindex = numofsingle; 00300 /* Start writing data */ 00301 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00302 if(status != EEPROM_OK) 00303 { 00304 return status; 00305 } 00306 } 00307 /* If NumByteToWrite > EEPROM_PAGESIZE */ 00308 else 00309 { 00310 while(numofpage--) 00311 { 00312 /* Store the number of data to be written */ 00313 dataindex = EEPROM_PAGESIZE; 00314 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00315 if(status != EEPROM_OK) 00316 { 00317 return status; 00318 } 00319 00320 WriteAddr += EEPROM_PAGESIZE; 00321 pBuffer += EEPROM_PAGESIZE; 00322 } 00323 00324 if(numofsingle!=0) 00325 { 00326 /* Store the number of data to be written */ 00327 dataindex = numofsingle; 00328 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00329 if(status != EEPROM_OK) 00330 { 00331 return status; 00332 } 00333 } 00334 } 00335 } 00336 /* If WriteAddr is not EEPROM_PAGESIZE aligned */ 00337 else 00338 { 00339 /* If NumByteToWrite < EEPROM_PAGESIZE */ 00340 if(numofpage== 0) 00341 { 00342 /* If the number of data to be written is more than the remaining space 00343 in the current page: */ 00344 if(NumByteToWrite > count) 00345 { 00346 /* Store the number of data to be written */ 00347 dataindex = count; 00348 /* Write the data contained in same page */ 00349 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00350 if(status != EEPROM_OK) 00351 { 00352 return status; 00353 } 00354 00355 /* Store the number of data to be written */ 00356 dataindex = (NumByteToWrite - count); 00357 /* Write the remaining data in the following page */ 00358 status = BSP_EEPROM_WritePage((uint8_t*)(pBuffer + count), (WriteAddr + count), (uint8_t*)(&dataindex)); 00359 if(status != EEPROM_OK) 00360 { 00361 return status; 00362 } 00363 } 00364 else 00365 { 00366 /* Store the number of data to be written */ 00367 dataindex = numofsingle; 00368 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00369 if(status != EEPROM_OK) 00370 { 00371 return status; 00372 } 00373 } 00374 } 00375 /* If NumByteToWrite > EEPROM_PAGESIZE */ 00376 else 00377 { 00378 NumByteToWrite -= count; 00379 numofpage = NumByteToWrite / EEPROM_PAGESIZE; 00380 numofsingle = NumByteToWrite % EEPROM_PAGESIZE; 00381 00382 if(count != 0) 00383 { 00384 /* Store the number of data to be written */ 00385 dataindex = count; 00386 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00387 if(status != EEPROM_OK) 00388 { 00389 return status; 00390 } 00391 WriteAddr += count; 00392 pBuffer += count; 00393 } 00394 00395 while(numofpage--) 00396 { 00397 /* Store the number of data to be written */ 00398 dataindex = EEPROM_PAGESIZE; 00399 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00400 if(status != EEPROM_OK) 00401 { 00402 return status; 00403 } 00404 WriteAddr += EEPROM_PAGESIZE; 00405 pBuffer += EEPROM_PAGESIZE; 00406 } 00407 if(numofsingle != 0) 00408 { 00409 /* Store the number of data to be written */ 00410 dataindex = numofsingle; 00411 status = BSP_EEPROM_WritePage(pBuffer, WriteAddr, (uint8_t*)(&dataindex)); 00412 if(status != EEPROM_OK) 00413 { 00414 return status; 00415 } 00416 } 00417 } 00418 } 00419 00420 /* If all operations OK, return EEPROM_OK (0) */ 00421 return EEPROM_OK; 00422 } 00423 00424 /** 00425 * @brief Wait for EEPROM Standby state. 00426 * 00427 * @note This function allows to wait and check that EEPROM has finished the 00428 * last operation. It is mostly used after Write operation: after receiving 00429 * the buffer to be written, the EEPROM may need additional time to actually 00430 * perform the write operation. During this time, it doesn't answer to 00431 * I2C packets addressed to it. Once the write operation is complete 00432 * the EEPROM responds to its address. 00433 * 00434 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00435 * different from EEPROM_OK (0) or the timeout user callback. 00436 */ 00437 uint32_t BSP_EEPROM_WaitEepromStandbyState(void) 00438 { 00439 /* Check if the maximum allowed number of trials has bee reached */ 00440 if(EEPROM_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00441 { 00442 /* If the maximum number of trials has been reached, exit the function */ 00443 BSP_EEPROM_TIMEOUT_UserCallback(); 00444 return EEPROM_TIMEOUT; 00445 } 00446 return EEPROM_OK; 00447 } 00448 00449 /** 00450 * @brief Basic management of the timeout situation. 00451 * @retval None 00452 */ 00453 __weak void BSP_EEPROM_TIMEOUT_UserCallback(void) 00454 { 00455 } 00456 00457 /** 00458 * @} 00459 */ 00460 00461 /** 00462 * @} 00463 */ 00464 00465 /** 00466 * @} 00467 */ 00468 00469 /** 00470 * @} 00471 */ 00472 00473 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri May 22 2015 13:59:20 for STM32756G_EVAL BSP User Manual by
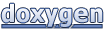