STM32756G_EVAL BSP User Manual
|
stm32756g_eval_camera.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval_camera.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 22-May-2015 00007 * @brief This file includes the driver for Camera modules mounted on 00008 * STM32756G-EVAL and STM32746G-EVAL evaluation boards. 00009 @verbatim 00010 How to use this driver: 00011 ----------------------- 00012 - This driver is used to drive the camera. 00013 - The S5K5CAG component driver MUST be included with this driver. 00014 00015 Driver description: 00016 ------------------ 00017 + Initialization steps: 00018 o Initialize the camera using the BSP_CAMERA_Init() function. 00019 o Start the camera capture/snapshot using the CAMERA_Start() function. 00020 o Suspend, resume or stop the camera capture using the following functions: 00021 - BSP_CAMERA_Suspend() 00022 - BSP_CAMERA_Resume() 00023 - BSP_CAMERA_Stop() 00024 00025 + Options 00026 o Increase or decrease on the fly the brightness and/or contrast 00027 using the following function: 00028 - BSP_CAMERA_ContrastBrightnessConfig 00029 o Add a special effect on the fly using the following functions: 00030 - BSP_CAMERA_BlackWhiteConfig() 00031 - BSP_CAMERA_ColorEffectConfig() 00032 @endverbatim 00033 ****************************************************************************** 00034 * @attention 00035 * 00036 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00037 * 00038 * Redistribution and use in source and binary forms, with or without modification, 00039 * are permitted provided that the following conditions are met: 00040 * 1. Redistributions of source code must retain the above copyright notice, 00041 * this list of conditions and the following disclaimer. 00042 * 2. Redistributions in binary form must reproduce the above copyright notice, 00043 * this list of conditions and the following disclaimer in the documentation 00044 * and/or other materials provided with the distribution. 00045 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00046 * may be used to endorse or promote products derived from this software 00047 * without specific prior written permission. 00048 * 00049 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00050 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00051 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00052 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00053 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00054 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00055 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00056 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00057 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00058 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00059 * 00060 ****************************************************************************** 00061 */ 00062 00063 /* Includes ------------------------------------------------------------------*/ 00064 #include "stm32756g_eval_camera.h" 00065 00066 /** @addtogroup BSP 00067 * @{ 00068 */ 00069 00070 /** @addtogroup STM32756G_EVAL 00071 * @{ 00072 */ 00073 00074 /** @addtogroup STM32756G_EVAL_CAMERA 00075 * @{ 00076 */ 00077 00078 /** @defgroup STM32756G_EVAL_CAMERA_Private_TypesDefinitions 00079 * @{ 00080 */ 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32756G_EVAL_CAMERA_Private_Defines 00086 * @{ 00087 */ 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM32756G_EVAL_CAMERA_Private_Macros 00093 * @{ 00094 */ 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM32756G_EVAL_CAMERA_Private_Variables 00100 * @{ 00101 */ 00102 static DCMI_HandleTypeDef hDcmiEval; 00103 CAMERA_DrvTypeDef *camera_drv; 00104 /* Camera current resolution naming (QQVGA, VGA, ...) */ 00105 static uint32_t CameraCurrentResolution; 00106 00107 /* Camera module I2C HW address */ 00108 static uint32_t CameraHwAddress; 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup STM32756G_EVAL_CAMERA_Private_FunctionPrototypes 00114 * @{ 00115 */ 00116 static uint32_t GetSize(uint32_t resolution); 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM32756G_EVAL_CAMERA_Private_Functions 00122 * @{ 00123 */ 00124 00125 /** 00126 * @brief Initializes the camera. 00127 * @param uint32_t Resolution : camera sensor requested resolution (x, y) : standard resolution 00128 * naming QQVGA, QVGA, VGA ... 00129 * 00130 * @retval Camera status 00131 */ 00132 uint8_t BSP_CAMERA_Init(uint32_t Resolution) 00133 { 00134 DCMI_HandleTypeDef *phdcmi; 00135 uint8_t status = CAMERA_ERROR; 00136 00137 /* Get the DCMI handle structure */ 00138 phdcmi = &hDcmiEval; 00139 00140 /*** Configures the DCMI to interface with the camera module ***/ 00141 /* DCMI configuration */ 00142 phdcmi->Init.CaptureRate = DCMI_CR_ALL_FRAME; 00143 phdcmi->Init.HSPolarity = DCMI_HSPOLARITY_HIGH; 00144 phdcmi->Init.SynchroMode = DCMI_SYNCHRO_HARDWARE; 00145 phdcmi->Init.VSPolarity = DCMI_VSPOLARITY_HIGH; 00146 phdcmi->Init.ExtendedDataMode = DCMI_EXTEND_DATA_8B; 00147 phdcmi->Init.PCKPolarity = DCMI_PCKPOLARITY_RISING; 00148 phdcmi->Instance = DCMI; 00149 00150 /* Configure IO functionalities for CAMERA detect pin */ 00151 BSP_IO_Init(); 00152 00153 /* Apply Camera Module hardware reset */ 00154 BSP_CAMERA_HwReset(); 00155 00156 /* Check if the CAMERA Module is plugged on board */ 00157 if(BSP_IO_ReadPin(CAM_PLUG_PIN) == BSP_IO_PIN_SET) 00158 { 00159 status = CAMERA_NOT_DETECTED; 00160 return status; /* Exit with error */ 00161 } 00162 00163 /* Read ID of Camera module via I2C */ 00164 if (s5k5cag_ReadID(CAMERA_I2C_ADDRESS) == S5K5CAG_ID) 00165 { 00166 /* Initialize the camera driver structure */ 00167 camera_drv = &s5k5cag_drv; 00168 CameraHwAddress = CAMERA_I2C_ADDRESS; 00169 00170 /* DCMI Initialization */ 00171 BSP_CAMERA_MspInit(&hDcmiEval, NULL); 00172 HAL_DCMI_Init(phdcmi); 00173 00174 /* Camera Module Initialization via I2C to the wanted 'Resolution' */ 00175 camera_drv->Init(CameraHwAddress, Resolution); 00176 00177 CameraCurrentResolution = Resolution; 00178 00179 /* Return CAMERA_OK status */ 00180 status = CAMERA_OK; 00181 } 00182 else 00183 { 00184 /* Return CAMERA_NOT_SUPPORTED status */ 00185 status = CAMERA_NOT_SUPPORTED; 00186 } 00187 00188 return status; 00189 } 00190 00191 /** 00192 * @brief DeInitializes the camera. 00193 * @param Camera: Pointer to the camera configuration structure 00194 * @retval Camera status 00195 */ 00196 uint8_t BSP_CAMERA_DeInit(void) 00197 { 00198 hDcmiEval.Instance = DCMI; 00199 00200 HAL_DCMI_DeInit(&hDcmiEval); 00201 BSP_CAMERA_MspDeInit(&hDcmiEval, NULL); 00202 return CAMERA_OK; 00203 } 00204 00205 /** 00206 * @brief Starts the camera capture in continuous mode. 00207 * @param buff: pointer to the camera output buffer 00208 * @retval None 00209 */ 00210 void BSP_CAMERA_ContinuousStart(uint8_t *buff) 00211 { 00212 /* Start the camera capture */ 00213 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_CONTINUOUS, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00214 } 00215 00216 /** 00217 * @brief Starts the camera capture in snapshot mode. 00218 * @param buff: pointer to the camera output buffer 00219 * @retval None 00220 */ 00221 void BSP_CAMERA_SnapshotStart(uint8_t *buff) 00222 { 00223 /* Start the camera capture */ 00224 HAL_DCMI_Start_DMA(&hDcmiEval, DCMI_MODE_SNAPSHOT, (uint32_t)buff, GetSize(CameraCurrentResolution)); 00225 } 00226 00227 /** 00228 * @brief Suspend the CAMERA capture 00229 00230 * @retval None 00231 */ 00232 void BSP_CAMERA_Suspend(void) 00233 { 00234 /* Disable the DMA */ 00235 __HAL_DMA_DISABLE(hDcmiEval.DMA_Handle); 00236 /* Disable the DCMI */ 00237 __HAL_DCMI_DISABLE(&hDcmiEval); 00238 00239 } 00240 00241 /** 00242 * @brief Resume the CAMERA capture 00243 * @retval None 00244 */ 00245 void BSP_CAMERA_Resume(void) 00246 { 00247 /* Enable the DCMI */ 00248 __HAL_DCMI_ENABLE(&hDcmiEval); 00249 /* Enable the DMA */ 00250 __HAL_DMA_ENABLE(hDcmiEval.DMA_Handle); 00251 } 00252 00253 /** 00254 * @brief Stop the CAMERA capture 00255 * @retval Camera status 00256 */ 00257 uint8_t BSP_CAMERA_Stop(void) 00258 { 00259 uint8_t status = CAMERA_ERROR; 00260 00261 if(HAL_DCMI_Stop(&hDcmiEval) == HAL_OK) 00262 { 00263 status = CAMERA_OK; 00264 } 00265 00266 /* Set Camera in Power Down */ 00267 BSP_CAMERA_PwrDown(); 00268 00269 return status; 00270 } 00271 00272 /** 00273 * @brief CANERA hardware reset 00274 * @retval None 00275 */ 00276 void BSP_CAMERA_HwReset(void) 00277 { 00278 /* Camera sensor RESET sequence */ 00279 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00280 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00281 00282 /* Assert the camera STANDBY pin (active high) */ 00283 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_SET); 00284 00285 /* Assert the camera RSTI pin (active low) */ 00286 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00287 00288 HAL_Delay(100); /* RST and XSDN signals asserted during 100ms */ 00289 00290 /* De-assert the camera STANDBY pin (active high) */ 00291 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00292 00293 HAL_Delay(3); /* RST de-asserted and XSDN asserted during 3ms */ 00294 00295 /* De-assert the camera RSTI pin (active low) */ 00296 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_SET); 00297 00298 HAL_Delay(6); /* RST de-asserted during 3ms */ 00299 } 00300 00301 /** 00302 * @brief CAMERA power down 00303 * @retval None 00304 */ 00305 void BSP_CAMERA_PwrDown(void) 00306 { 00307 /* Camera power down sequence */ 00308 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00309 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00310 00311 /* De-assert the camera STANDBY pin (active high) */ 00312 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00313 00314 /* Assert the camera RSTI pin (active low) */ 00315 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00316 } 00317 00318 /** 00319 * @brief Configures the camera contrast and brightness. 00320 * @param contrast_level: Contrast level 00321 * This parameter can be one of the following values: 00322 * @arg CAMERA_CONTRAST_LEVEL4: for contrast +2 00323 * @arg CAMERA_CONTRAST_LEVEL3: for contrast +1 00324 * @arg CAMERA_CONTRAST_LEVEL2: for contrast 0 00325 * @arg CAMERA_CONTRAST_LEVEL1: for contrast -1 00326 * @arg CAMERA_CONTRAST_LEVEL0: for contrast -2 00327 * @param brightness_level: Contrast level 00328 * This parameter can be one of the following values: 00329 * @arg CAMERA_BRIGHTNESS_LEVEL4: for brightness +2 00330 * @arg CAMERA_BRIGHTNESS_LEVEL3: for brightness +1 00331 * @arg CAMERA_BRIGHTNESS_LEVEL2: for brightness 0 00332 * @arg CAMERA_BRIGHTNESS_LEVEL1: for brightness -1 00333 * @arg CAMERA_BRIGHTNESS_LEVEL0: for brightness -2 00334 * @retval None 00335 */ 00336 void BSP_CAMERA_ContrastBrightnessConfig(uint32_t contrast_level, uint32_t brightness_level) 00337 { 00338 if(camera_drv->Config != NULL) 00339 { 00340 camera_drv->Config(CameraHwAddress, CAMERA_CONTRAST_BRIGHTNESS, contrast_level, brightness_level); 00341 } 00342 } 00343 00344 /** 00345 * @brief Configures the camera white balance. 00346 * @param Mode: black_white mode 00347 * This parameter can be one of the following values: 00348 * @arg CAMERA_BLACK_WHITE_BW 00349 * @arg CAMERA_BLACK_WHITE_NEGATIVE 00350 * @arg CAMERA_BLACK_WHITE_BW_NEGATIVE 00351 * @arg CAMERA_BLACK_WHITE_NORMAL 00352 * @retval None 00353 */ 00354 void BSP_CAMERA_BlackWhiteConfig(uint32_t Mode) 00355 { 00356 if(camera_drv->Config != NULL) 00357 { 00358 camera_drv->Config(CameraHwAddress, CAMERA_BLACK_WHITE, Mode, 0); 00359 } 00360 } 00361 00362 /** 00363 * @brief Configures the camera color effect. 00364 * @param Effect: Color effect 00365 * This parameter can be one of the following values: 00366 * @arg CAMERA_COLOR_EFFECT_ANTIQUE 00367 * @arg CAMERA_COLOR_EFFECT_BLUE 00368 * @arg CAMERA_COLOR_EFFECT_GREEN 00369 * @arg CAMERA_COLOR_EFFECT_RED 00370 * @retval None 00371 */ 00372 void BSP_CAMERA_ColorEffectConfig(uint32_t Effect) 00373 { 00374 if(camera_drv->Config != NULL) 00375 { 00376 camera_drv->Config(CameraHwAddress, CAMERA_COLOR_EFFECT, Effect, 0); 00377 } 00378 } 00379 00380 /** 00381 * @brief Handles DCMI interrupt request. 00382 * @retval None 00383 */ 00384 void BSP_CAMERA_IRQHandler(void) 00385 { 00386 HAL_DCMI_IRQHandler(&hDcmiEval); 00387 } 00388 00389 /** 00390 * @brief Handles DMA interrupt request. 00391 * @retval None 00392 */ 00393 void BSP_CAMERA_DMA_IRQHandler(void) 00394 { 00395 HAL_DMA_IRQHandler(hDcmiEval.DMA_Handle); 00396 } 00397 00398 /** 00399 * @brief Get the capture size in pixels unit. 00400 * @param resolution: the current resolution. 00401 * @retval capture size in pixels unit. 00402 */ 00403 static uint32_t GetSize(uint32_t resolution) 00404 { 00405 uint32_t size = 0; 00406 00407 /* Get capture size */ 00408 switch (resolution) 00409 { 00410 case CAMERA_R160x120: 00411 { 00412 size = 0x2580; 00413 } 00414 break; 00415 case CAMERA_R320x240: 00416 { 00417 size = 0x9600; 00418 } 00419 break; 00420 case CAMERA_R480x272: 00421 { 00422 size = 0xFF00; 00423 } 00424 break; 00425 case CAMERA_R640x480: 00426 { 00427 size = 0x25800; 00428 } 00429 break; 00430 default: 00431 { 00432 break; 00433 } 00434 } 00435 00436 return size; 00437 } 00438 00439 /** 00440 * @brief Initializes the DCMI MSP. 00441 * @param hdcmi: HDMI handle 00442 * @retval None 00443 */ 00444 __weak void BSP_CAMERA_MspInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00445 { 00446 static DMA_HandleTypeDef hdma_eval; 00447 GPIO_InitTypeDef gpio_init_structure; 00448 00449 /*** Enable peripherals and GPIO clocks ***/ 00450 /* Enable DCMI clock */ 00451 __HAL_RCC_DCMI_CLK_ENABLE(); 00452 00453 /* Enable DMA2 clock */ 00454 __HAL_RCC_DMA2_CLK_ENABLE(); 00455 00456 /* Enable GPIO clocks */ 00457 __HAL_RCC_GPIOA_CLK_ENABLE(); 00458 __HAL_RCC_GPIOB_CLK_ENABLE(); 00459 __HAL_RCC_GPIOC_CLK_ENABLE(); 00460 __HAL_RCC_GPIOD_CLK_ENABLE(); 00461 __HAL_RCC_GPIOE_CLK_ENABLE(); 00462 00463 /*** Configure the GPIO ***/ 00464 /* Configure DCMI GPIO as alternate function */ 00465 /* On STM32756G-EVAL RevB, to use camera, ensure that JP23 is in position 1-2, 00466 * LED3 is then no more usable */ 00467 gpio_init_structure.Pin = GPIO_PIN_4 | GPIO_PIN_6; 00468 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00469 gpio_init_structure.Pull = GPIO_PULLUP; 00470 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00471 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00472 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 00473 00474 gpio_init_structure.Pin = GPIO_PIN_7; 00475 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00476 gpio_init_structure.Pull = GPIO_PULLUP; 00477 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00478 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00479 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00480 00481 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_8 |\ 00482 GPIO_PIN_9 | GPIO_PIN_11; 00483 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00484 gpio_init_structure.Pull = GPIO_PULLUP; 00485 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00486 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00487 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00488 00489 gpio_init_structure.Pin = GPIO_PIN_3 | GPIO_PIN_6; 00490 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00491 gpio_init_structure.Pull = GPIO_PULLUP; 00492 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00493 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00494 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00495 00496 gpio_init_structure.Pin = GPIO_PIN_5 | GPIO_PIN_6; 00497 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00498 gpio_init_structure.Pull = GPIO_PULLUP; 00499 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00500 gpio_init_structure.Alternate = GPIO_AF13_DCMI; 00501 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00502 00503 /*** Configure the DMA ***/ 00504 /* Set the parameters to be configured */ 00505 hdma_eval.Init.Channel = DMA_CHANNEL_1; 00506 hdma_eval.Init.Direction = DMA_PERIPH_TO_MEMORY; 00507 hdma_eval.Init.PeriphInc = DMA_PINC_DISABLE; 00508 hdma_eval.Init.MemInc = DMA_MINC_ENABLE; 00509 hdma_eval.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00510 hdma_eval.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00511 hdma_eval.Init.Mode = DMA_CIRCULAR; 00512 hdma_eval.Init.Priority = DMA_PRIORITY_HIGH; 00513 hdma_eval.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00514 hdma_eval.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00515 hdma_eval.Init.MemBurst = DMA_MBURST_SINGLE; 00516 hdma_eval.Init.PeriphBurst = DMA_PBURST_SINGLE; 00517 00518 hdma_eval.Instance = DMA2_Stream1; 00519 00520 /* Associate the initialized DMA handle to the DCMI handle */ 00521 __HAL_LINKDMA(hdcmi, DMA_Handle, hdma_eval); 00522 00523 /*** Configure the NVIC for DCMI and DMA ***/ 00524 /* NVIC configuration for DCMI transfer complete interrupt */ 00525 HAL_NVIC_SetPriority(DCMI_IRQn, 5, 0); 00526 HAL_NVIC_EnableIRQ(DCMI_IRQn); 00527 00528 /* NVIC configuration for DMA2D transfer complete interrupt */ 00529 HAL_NVIC_SetPriority(DMA2_Stream1_IRQn, 5, 0); 00530 HAL_NVIC_EnableIRQ(DMA2_Stream1_IRQn); 00531 00532 /* Configure the DMA stream */ 00533 HAL_DMA_Init(hdcmi->DMA_Handle); 00534 } 00535 00536 00537 /** 00538 * @brief DeInitializes the DCMI MSP. 00539 * @param hdcmi: HDMI handle 00540 * @retval None 00541 */ 00542 __weak void BSP_CAMERA_MspDeInit(DCMI_HandleTypeDef *hdcmi, void *Params) 00543 { 00544 /* Disable NVIC for DCMI transfer complete interrupt */ 00545 HAL_NVIC_DisableIRQ(DCMI_IRQn); 00546 00547 /* Disable NVIC for DMA2 transfer complete interrupt */ 00548 HAL_NVIC_DisableIRQ(DMA2_Stream1_IRQn); 00549 00550 /* Configure the DMA stream */ 00551 HAL_DMA_DeInit(hdcmi->DMA_Handle); 00552 00553 /* Disable DCMI clock */ 00554 __HAL_RCC_DCMI_CLK_DISABLE(); 00555 00556 /* GPIO pins clock and DMA clock can be shut down in the application 00557 by surcharging this __weak function */ 00558 } 00559 00560 /** 00561 * @brief Line event callback 00562 * @param hdcmi: pointer to the DCMI handle 00563 * @retval None 00564 */ 00565 void HAL_DCMI_LineEventCallback(DCMI_HandleTypeDef *hdcmi) 00566 { 00567 BSP_CAMERA_LineEventCallback(); 00568 } 00569 00570 /** 00571 * @brief Line Event callback. 00572 * @retval None 00573 */ 00574 __weak void BSP_CAMERA_LineEventCallback(void) 00575 { 00576 /* NOTE : This function Should not be modified, when the callback is needed, 00577 the HAL_DCMI_LineEventCallback could be implemented in the user file 00578 */ 00579 } 00580 00581 /** 00582 * @brief VSYNC event callback 00583 * @param hdcmi: pointer to the DCMI handle 00584 * @retval None 00585 */ 00586 void HAL_DCMI_VsyncEventCallback(DCMI_HandleTypeDef *hdcmi) 00587 { 00588 BSP_CAMERA_VsyncEventCallback(); 00589 } 00590 00591 /** 00592 * @brief VSYNC Event callback. 00593 * @retval None 00594 */ 00595 __weak void BSP_CAMERA_VsyncEventCallback(void) 00596 { 00597 /* NOTE : This function Should not be modified, when the callback is needed, 00598 the HAL_DCMI_VsyncEventCallback could be implemented in the user file 00599 */ 00600 } 00601 00602 /** 00603 * @brief Frame event callback 00604 * @param hdcmi: pointer to the DCMI handle 00605 * @retval None 00606 */ 00607 void HAL_DCMI_FrameEventCallback(DCMI_HandleTypeDef *hdcmi) 00608 { 00609 BSP_CAMERA_FrameEventCallback(); 00610 } 00611 00612 /** 00613 * @brief Frame Event callback. 00614 * @retval None 00615 */ 00616 __weak void BSP_CAMERA_FrameEventCallback(void) 00617 { 00618 /* NOTE : This function Should not be modified, when the callback is needed, 00619 the HAL_DCMI_FrameEventCallback could be implemented in the user file 00620 */ 00621 } 00622 00623 /** 00624 * @brief Error callback 00625 * @param hdcmi: pointer to the DCMI handle 00626 * @retval None 00627 */ 00628 void HAL_DCMI_ErrorCallback(DCMI_HandleTypeDef *hdcmi) 00629 { 00630 BSP_CAMERA_ErrorCallback(); 00631 } 00632 00633 /** 00634 * @brief Error callback. 00635 * @retval None 00636 */ 00637 __weak void BSP_CAMERA_ErrorCallback(void) 00638 { 00639 /* NOTE : This function Should not be modified, when the callback is needed, 00640 the HAL_DCMI_ErrorCallback could be implemented in the user file 00641 */ 00642 } 00643 00644 /** 00645 * @} 00646 */ 00647 00648 /** 00649 * @} 00650 */ 00651 00652 /** 00653 * @} 00654 */ 00655 00656 /** 00657 * @} 00658 */ 00659 00660 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri May 22 2015 13:59:20 for STM32756G_EVAL BSP User Manual by
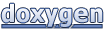