STM32756G_EVAL BSP User Manual
|
stm32756g_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32756g_eval.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 22-May-2015 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32756G-EVAL and STM32746G-EVAL 00009 * evaluation board(MB1167) from STMicroelectronics. 00010 * 00011 @verbatim 00012 This driver requires the stm32756g_eval_io.c/.h files to manage the 00013 IO module resources mapped on the MFX IO expander. 00014 These resources are mainly LEDs, Joystick push buttons, SD detect pin, 00015 USB OTG power switch/over current drive pins, Camera plug pin, Audio 00016 INT pin 00017 The use of the above eval resources is conditioned by the "USE_IOEXPANDER" 00018 preprocessor define which is enabled by default for the STM327x6G-EVAL 00019 boards Rev A. However for Rev B boards these resources are disabled by default 00020 (except LED1 and LED2) and to be able to use them, user must add "USE_IOEXPANDER" 00021 define in the compiler preprocessor configuration (or any header file that 00022 is processed before stm32756g_eval.h). 00023 On the STM327x6G-EVAL RevB LED1 and LED2 are directly mapped on GPIO pins, 00024 to avoid the unnecessary overhead of code brought by the use of MFX IO 00025 expander when no further evaluation board resources are needed by the 00026 application/example. 00027 For precise details on the use of the MFX IO expander, you can refer to 00028 the description provided within the stm32756g_eval_io.c file header 00029 @endverbatim 00030 ****************************************************************************** 00031 * @attention 00032 * 00033 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00034 * 00035 * Redistribution and use in source and binary forms, with or without modification, 00036 * are permitted provided that the following conditions are met: 00037 * 1. Redistributions of source code must retain the above copyright notice, 00038 * this list of conditions and the following disclaimer. 00039 * 2. Redistributions in binary form must reproduce the above copyright notice, 00040 * this list of conditions and the following disclaimer in the documentation 00041 * and/or other materials provided with the distribution. 00042 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00043 * may be used to endorse or promote products derived from this software 00044 * without specific prior written permission. 00045 * 00046 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00047 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00048 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00049 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00050 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00051 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00052 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00053 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00054 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00055 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00056 * 00057 ****************************************************************************** 00058 */ 00059 00060 /* Includes ------------------------------------------------------------------*/ 00061 #include "stm32756g_eval.h" 00062 #if defined(USE_IOEXPANDER) 00063 #include "stm32756g_eval_io.h" 00064 #endif /* USE_IOEXPANDER */ 00065 00066 /** @addtogroup BSP 00067 * @{ 00068 */ 00069 00070 /** @addtogroup STM32756G_EVAL 00071 * @{ 00072 */ 00073 00074 /** @defgroup STM32756G_EVAL_LOW_LEVEL STM32756G-EVAL LOW LEVEL 00075 * @{ 00076 */ 00077 00078 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_TypesDefinitions STM32756G-EVAL LOW LEVEL Private Types Definitions 00079 * @{ 00080 */ 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Defines STM32756G-EVAL LOW LEVEL Private Defines 00086 * @{ 00087 */ 00088 /** 00089 * @brief STM32756G EVAL BSP Driver version number V1.0.0 00090 */ 00091 #define __STM32756G_EVAL_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00092 #define __STM32756G_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00093 #define __STM32756G_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00094 #define __STM32756G_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00095 #define __STM32756G_EVAL_BSP_VERSION ((__STM32756G_EVAL_BSP_VERSION_MAIN << 24)\ 00096 |(__STM32756G_EVAL_BSP_VERSION_SUB1 << 16)\ 00097 |(__STM32756G_EVAL_BSP_VERSION_SUB2 << 8 )\ 00098 |(__STM32756G_EVAL_BSP_VERSION_RC)) 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Macros STM32756G-EVAL LOW LEVEL Private Macros 00104 * @{ 00105 */ 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Variables STM32756G-EVAL LOW LEVEL Private Variables 00111 * @{ 00112 */ 00113 00114 #if defined(USE_IOEXPANDER) 00115 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00116 LED2_PIN, 00117 LED3_PIN, 00118 LED4_PIN}; 00119 #else 00120 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00121 LED3_PIN}; 00122 #endif /* USE_IOEXPANDER */ 00123 00124 00125 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00126 TAMPER_BUTTON_GPIO_PORT, 00127 KEY_BUTTON_GPIO_PORT}; 00128 00129 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00130 TAMPER_BUTTON_PIN, 00131 KEY_BUTTON_PIN}; 00132 00133 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00134 TAMPER_BUTTON_EXTI_IRQn, 00135 KEY_BUTTON_EXTI_IRQn}; 00136 00137 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00138 00139 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00140 00141 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00142 00143 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00144 00145 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00146 00147 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00148 00149 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00150 00151 static I2C_HandleTypeDef hEvalI2c; 00152 00153 /** 00154 * @} 00155 */ 00156 00157 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM32756G_EVAL LOW LEVEL Private Function Prototypes 00158 * @{ 00159 */ 00160 static void I2Cx_MspInit(void); 00161 static void I2Cx_Init(void); 00162 #if defined(USE_IOEXPANDER) 00163 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00164 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00165 #endif /* USE_IOEXPANDER */ 00166 00167 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00168 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00169 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00170 static void I2Cx_Error(uint8_t Addr); 00171 00172 #if defined(USE_IOEXPANDER) 00173 /* IOExpander IO functions */ 00174 void IOE_Init(void); 00175 void IOE_ITConfig(void); 00176 void IOE_Delay(uint32_t Delay); 00177 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00178 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00179 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00180 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00181 00182 /* MFX IO functions */ 00183 void MFX_IO_Init(void); 00184 void MFX_IO_DeInit(void); 00185 void MFX_IO_ITConfig(void); 00186 void MFX_IO_Delay(uint32_t Delay); 00187 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00188 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00189 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00190 void MFX_IO_Wakeup(void); 00191 void MFX_IO_EnableWakeupPin(void); 00192 #endif /* USE_IOEXPANDER */ 00193 00194 /* AUDIO IO functions */ 00195 void AUDIO_IO_Init(void); 00196 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00197 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00198 void AUDIO_IO_Delay(uint32_t Delay); 00199 00200 /* CAMERA IO functions */ 00201 void CAMERA_IO_Init(void); 00202 void CAMERA_Delay(uint32_t Delay); 00203 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00204 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg); 00205 00206 /* I2C EEPROM IO function */ 00207 void EEPROM_IO_Init(void); 00208 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00209 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00210 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00211 /** 00212 * @} 00213 */ 00214 00215 /** @defgroup STM32756G_EVAL_LOW_LEVEL_Private_Functions STM32756G_EVAL LOW LEVEL Private Functions 00216 * @{ 00217 */ 00218 00219 /** 00220 * @brief This method returns the STM32756G EVAL BSP Driver revision 00221 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00222 */ 00223 uint32_t BSP_GetVersion(void) 00224 { 00225 return __STM32756G_EVAL_BSP_VERSION; 00226 } 00227 00228 /** 00229 * @brief Configures LED on GPIO and/or on MFX. 00230 * @param Led: LED to be configured. 00231 * This parameter can be one of the following values: 00232 * @arg LED1 00233 * @arg LED2 00234 * @arg LED3 00235 * @arg LED4 00236 * @retval None 00237 */ 00238 void BSP_LED_Init(Led_TypeDef Led) 00239 { 00240 #if !defined(USE_STM32756G_EVAL_REVA) 00241 /* On RevB and above evaluation boards, LED1 and LED3 are connected to GPIOs */ 00242 /* To use LED1 on RevB board, ensure that JP24 is in position 2-3, potentiometer is then no more usable */ 00243 /* To use LED3 on RevB board, ensure that JP23 is in position 2-3, camera is then no more usable */ 00244 GPIO_InitTypeDef gpio_init_structure; 00245 GPIO_TypeDef* gpio_led; 00246 00247 if ((Led == LED1) || (Led == LED3)) 00248 { 00249 if (Led == LED1) 00250 { 00251 gpio_led = LED1_GPIO_PORT; 00252 /* Enable the GPIO_LED clock */ 00253 LED1_GPIO_CLK_ENABLE(); 00254 } 00255 else 00256 { 00257 gpio_led = LED3_GPIO_PORT; 00258 /* Enable the GPIO_LED clock */ 00259 LED3_GPIO_CLK_ENABLE(); 00260 } 00261 00262 /* Configure the GPIO_LED pin */ 00263 gpio_init_structure.Pin = GPIO_PIN[Led]; 00264 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00265 gpio_init_structure.Pull = GPIO_PULLUP; 00266 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00267 00268 HAL_GPIO_Init(gpio_led, &gpio_init_structure); 00269 00270 /* By default, turn off LED */ 00271 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00272 } 00273 else 00274 { 00275 #endif /* !USE_STM32756G_EVAL_REVA */ 00276 00277 #if defined(USE_IOEXPANDER) 00278 /* On RevA eval board, all LEDs are connected to MFX */ 00279 /* On RevB and above eval board, LED2 and LED4 are connected to MFX */ 00280 BSP_IO_Init(); /* Initialize MFX */ 00281 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OUTPUT_PP_PU); 00282 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00283 #endif /* USE_IOEXPANDER */ 00284 00285 #if !defined(USE_STM32756G_EVAL_REVA) 00286 } 00287 #endif /* !USE_STM32756G_EVAL_REVA */ 00288 } 00289 00290 00291 /** 00292 * @brief DeInit LEDs. 00293 * @param Led: LED to be configured. 00294 * This parameter can be one of the following values: 00295 * @arg LED1 00296 * @arg LED2 00297 * @arg LED3 00298 * @arg LED4 00299 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00300 * @retval None 00301 */ 00302 void BSP_LED_DeInit(Led_TypeDef Led) 00303 { 00304 #if !defined(USE_STM32756G_EVAL_REVA) 00305 GPIO_InitTypeDef gpio_init_structure; 00306 GPIO_TypeDef* gpio_led; 00307 00308 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00309 if ((Led == LED1) || (Led == LED3)) 00310 { 00311 if (Led == LED1) 00312 { 00313 gpio_led = LED1_GPIO_PORT; 00314 } 00315 else 00316 { 00317 gpio_led = LED3_GPIO_PORT; 00318 } 00319 /* Turn off LED */ 00320 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00321 /* Configure the GPIO_LED pin */ 00322 gpio_init_structure.Pin = GPIO_PIN[Led]; 00323 HAL_GPIO_DeInit(gpio_led, gpio_init_structure.Pin); 00324 } 00325 else 00326 { 00327 #endif /* !USE_STM32756G_EVAL_REVA */ 00328 00329 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00330 /* GPIO_PIN[Led] depends on the board revision: */ 00331 /* - in case of RevA all leds are deinit */ 00332 /* - in case of RevB just led 2 and led4 are deinit */ 00333 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OFF); 00334 #endif /* USE_IOEXPANDER */ 00335 00336 #if !defined(USE_STM32756G_EVAL_REVA) 00337 } 00338 #endif /* !USE_STM32756G_EVAL_REVA */ 00339 } 00340 00341 /** 00342 * @brief Turns selected LED On. 00343 * @param Led: LED to be set on 00344 * This parameter can be one of the following values: 00345 * @arg LED1 00346 * @arg LED2 00347 * @arg LED3 00348 * @arg LED4 00349 * @retval None 00350 */ 00351 void BSP_LED_On(Led_TypeDef Led) 00352 { 00353 #if !defined(USE_STM32756G_EVAL_REVA) 00354 GPIO_TypeDef* gpio_led; 00355 00356 if ((Led == LED1) || (Led == LED3)) /* Switch On LED connected to GPIO */ 00357 { 00358 if (Led == LED1) 00359 { 00360 gpio_led = LED1_GPIO_PORT; 00361 } 00362 else 00363 { 00364 gpio_led = LED3_GPIO_PORT; 00365 } 00366 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00367 } 00368 else 00369 { 00370 #endif /* !USE_STM32756G_EVAL_REVA */ 00371 00372 #if defined(USE_IOEXPANDER) /* Switch On LED connected to MFX */ 00373 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_RESET); 00374 #endif /* USE_IOEXPANDER */ 00375 00376 #if !defined(USE_STM32756G_EVAL_REVA) 00377 } 00378 #endif /* !USE_STM32756G_EVAL_REVA */ 00379 } 00380 00381 /** 00382 * @brief Turns selected LED Off. 00383 * @param Led: LED to be set off 00384 * This parameter can be one of the following values: 00385 * @arg LED1 00386 * @arg LED2 00387 * @arg LED3 00388 * @arg LED4 00389 * @retval None 00390 */ 00391 void BSP_LED_Off(Led_TypeDef Led) 00392 { 00393 #if !defined(USE_STM32756G_EVAL_REVA) 00394 GPIO_TypeDef* gpio_led; 00395 00396 if ((Led == LED1) || (Led == LED3)) /* Switch Off LED connected to GPIO */ 00397 { 00398 if (Led == LED1) 00399 { 00400 gpio_led = LED1_GPIO_PORT; 00401 } 00402 else 00403 { 00404 gpio_led = LED3_GPIO_PORT; 00405 } 00406 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00407 } 00408 else 00409 { 00410 #endif /* !USE_STM32756G_EVAL_REVA */ 00411 00412 #if defined(USE_IOEXPANDER) /* Switch Off LED connected to MFX */ 00413 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00414 #endif /* USE_IOEXPANDER */ 00415 00416 #if !defined(USE_STM32756G_EVAL_REVA) 00417 } 00418 #endif /* !USE_STM32756G_EVAL_REVA */ 00419 00420 } 00421 00422 /** 00423 * @brief Toggles the selected LED. 00424 * @param Led: LED to be toggled 00425 * This parameter can be one of the following values: 00426 * @arg LED1 00427 * @arg LED2 00428 * @arg LED3 00429 * @arg LED4 00430 * @retval None 00431 */ 00432 void BSP_LED_Toggle(Led_TypeDef Led) 00433 { 00434 #if !defined(USE_STM32756G_EVAL_REVA) 00435 GPIO_TypeDef* gpio_led; 00436 00437 if ((Led == LED1) || (Led == LED3)) /* Toggle LED connected to GPIO */ 00438 { 00439 if (Led == LED1) 00440 { 00441 gpio_led = LED1_GPIO_PORT; 00442 } 00443 else 00444 { 00445 gpio_led = LED3_GPIO_PORT; 00446 } 00447 HAL_GPIO_TogglePin(gpio_led, GPIO_PIN[Led]); 00448 } 00449 else 00450 { 00451 #endif /* !USE_STM32756G_EVAL_REVA */ 00452 00453 #if defined(USE_IOEXPANDER) /* Toggle LED connected to MFX */ 00454 BSP_IO_TogglePin(GPIO_PIN[Led]); 00455 #endif /* USE_IOEXPANDER */ 00456 00457 #if !defined(USE_STM32756G_EVAL_REVA) 00458 } 00459 #endif /* !USE_STM32756G_EVAL_REVA */ 00460 } 00461 00462 /** 00463 * @brief Configures button GPIO and EXTI Line. 00464 * @param Button: Button to be configured 00465 * This parameter can be one of the following values: 00466 * @arg BUTTON_WAKEUP: Wakeup Push Button 00467 * @arg BUTTON_TAMPER: Tamper Push Button 00468 * @arg BUTTON_KEY: Key Push Button 00469 * @param Button_Mode: Button mode 00470 * This parameter can be one of the following values: 00471 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00472 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00473 * with interrupt generation capability 00474 * @note On STM32756G-EVAL evaluation board, the three buttons (Wakeup, Tamper 00475 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00476 * on the board serigraphy. 00477 * @retval None 00478 */ 00479 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00480 { 00481 GPIO_InitTypeDef gpio_init_structure; 00482 00483 /* Enable the BUTTON clock */ 00484 BUTTONx_GPIO_CLK_ENABLE(Button); 00485 00486 if(ButtonMode == BUTTON_MODE_GPIO) 00487 { 00488 /* Configure Button pin as input */ 00489 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00490 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00491 gpio_init_structure.Pull = GPIO_NOPULL; 00492 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00493 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00494 } 00495 00496 if(ButtonMode == BUTTON_MODE_EXTI) 00497 { 00498 /* Configure Button pin as input with External interrupt */ 00499 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00500 gpio_init_structure.Pull = GPIO_NOPULL; 00501 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00502 00503 if(Button != BUTTON_WAKEUP) 00504 { 00505 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00506 } 00507 else 00508 { 00509 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00510 } 00511 00512 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00513 00514 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00515 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00516 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00517 } 00518 } 00519 00520 /** 00521 * @brief Push Button DeInit. 00522 * @param Button: Button to be configured 00523 * This parameter can be one of the following values: 00524 * @arg BUTTON_WAKEUP: Wakeup Push Button 00525 * @arg BUTTON_TAMPER: Tamper Push Button 00526 * @arg BUTTON_KEY: Key Push Button 00527 * @note On STM32756G-EVAL evaluation board, the three buttons (Wakeup, Tamper 00528 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00529 * on the board serigraphy. 00530 * @note PB DeInit does not disable the GPIO clock 00531 * @retval None 00532 */ 00533 void BSP_PB_DeInit(Button_TypeDef Button) 00534 { 00535 GPIO_InitTypeDef gpio_init_structure; 00536 00537 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00538 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00539 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00540 } 00541 00542 00543 /** 00544 * @brief Returns the selected button state. 00545 * @param Button: Button to be checked 00546 * This parameter can be one of the following values: 00547 * @arg BUTTON_WAKEUP: Wakeup Push Button 00548 * @arg BUTTON_TAMPER: Tamper Push Button 00549 * @arg BUTTON_KEY: Key Push Button 00550 * @note On STM32756G-EVAL evaluation board, the three buttons (Wakeup, Tamper 00551 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00552 * on the board serigraphy. 00553 * @retval The Button GPIO pin value 00554 */ 00555 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00556 { 00557 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00558 } 00559 00560 /** 00561 * @brief Configures COM port. 00562 * @param COM: COM port to be configured. 00563 * This parameter can be one of the following values: 00564 * @arg COM1 00565 * @arg COM2 00566 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00567 * configuration information for the specified USART peripheral. 00568 * @retval None 00569 */ 00570 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00571 { 00572 GPIO_InitTypeDef gpio_init_structure; 00573 00574 /* Enable GPIO clock */ 00575 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00576 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00577 00578 /* Enable USART clock */ 00579 EVAL_COMx_CLK_ENABLE(COM); 00580 00581 /* Configure USART Tx as alternate function */ 00582 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00583 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00584 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00585 gpio_init_structure.Pull = GPIO_PULLUP; 00586 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00587 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00588 00589 /* Configure USART Rx as alternate function */ 00590 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00591 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00592 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00593 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00594 00595 /* USART configuration */ 00596 huart->Instance = COM_USART[COM]; 00597 HAL_UART_Init(huart); 00598 } 00599 00600 /** 00601 * @brief DeInit COM port. 00602 * @param COM: COM port to be configured. 00603 * This parameter can be one of the following values: 00604 * @arg COM1 00605 * @arg COM2 00606 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00607 * configuration information for the specified USART peripheral. 00608 * @retval None 00609 */ 00610 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00611 { 00612 /* USART configuration */ 00613 huart->Instance = COM_USART[COM]; 00614 HAL_UART_DeInit(huart); 00615 00616 /* Enable USART clock */ 00617 EVAL_COMx_CLK_DISABLE(COM); 00618 00619 /* DeInit GPIO pins can be done in the application 00620 (by surcharging this __weak function) */ 00621 00622 /* GPIO pins clock, DMA clock can be shut down in the application 00623 by surcharging this __weak function */ 00624 } 00625 00626 #if defined(USE_IOEXPANDER) 00627 /** 00628 * @brief Configures joystick GPIO and EXTI modes. 00629 * @param JoyMode: Button mode. 00630 * This parameter can be one of the following values: 00631 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00632 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00633 * with interrupt generation capability 00634 * @retval IO_OK: if all initializations are OK. Other value if error. 00635 */ 00636 uint8_t BSP_JOY_Init(JOYMode_TypeDef JoyMode) 00637 { 00638 uint8_t ret = 0; 00639 00640 /* Initialize the IO functionalities */ 00641 ret = BSP_IO_Init(); 00642 00643 /* Configure joystick pins in IT mode */ 00644 if(JoyMode == JOY_MODE_EXTI) 00645 { 00646 /* Configure IO interrupt acquisition mode */ 00647 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00648 } 00649 else 00650 { 00651 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00652 } 00653 00654 return ret; 00655 } 00656 00657 00658 /** 00659 * @brief DeInit joystick GPIOs. 00660 * @note JOY DeInit does not disable the MFX, just set the MFX pins in Off mode 00661 * @retval None. 00662 */ 00663 void BSP_JOY_DeInit() 00664 { 00665 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00666 } 00667 00668 /** 00669 * @brief Returns the current joystick status. 00670 * @retval Code of the joystick key pressed 00671 * This code can be one of the following values: 00672 * @arg JOY_NONE 00673 * @arg JOY_SEL 00674 * @arg JOY_DOWN 00675 * @arg JOY_LEFT 00676 * @arg JOY_RIGHT 00677 * @arg JOY_UP 00678 */ 00679 JOYState_TypeDef BSP_JOY_GetState(void) 00680 { 00681 uint16_t pin_status = 0; 00682 00683 /* Read the status joystick pins */ 00684 pin_status = BSP_IO_ReadPin(JOY_ALL_PINS); 00685 00686 /* Check the pressed keys */ 00687 if((pin_status & JOY_NONE_PIN) == JOY_NONE) 00688 { 00689 return(JOYState_TypeDef) JOY_NONE; 00690 } 00691 else if(!(pin_status & JOY_SEL_PIN)) 00692 { 00693 return(JOYState_TypeDef) JOY_SEL; 00694 } 00695 else if(!(pin_status & JOY_DOWN_PIN)) 00696 { 00697 return(JOYState_TypeDef) JOY_DOWN; 00698 } 00699 else if(!(pin_status & JOY_LEFT_PIN)) 00700 { 00701 return(JOYState_TypeDef) JOY_LEFT; 00702 } 00703 else if(!(pin_status & JOY_RIGHT_PIN)) 00704 { 00705 return(JOYState_TypeDef) JOY_RIGHT; 00706 } 00707 else if(!(pin_status & JOY_UP_PIN)) 00708 { 00709 return(JOYState_TypeDef) JOY_UP; 00710 } 00711 else 00712 { 00713 return(JOYState_TypeDef) JOY_NONE; 00714 } 00715 } 00716 00717 /** 00718 * @brief Check TS3510 touch screen presence 00719 * @retval Return 0 if TS3510 is detected, return 1 if not detected 00720 */ 00721 uint8_t BSP_TS3510_IsDetected(void) 00722 { 00723 HAL_StatusTypeDef status = HAL_OK; 00724 uint32_t error = 0; 00725 uint8_t a_buffer; 00726 00727 uint8_t tmp_buffer[2] = {0x81, 0x08}; 00728 00729 /* Prepare for LCD read data */ 00730 IOE_WriteMultiple(TS3510_I2C_ADDRESS, 0x8A, tmp_buffer, 2); 00731 00732 status = HAL_I2C_Mem_Read(&hEvalI2c, TS3510_I2C_ADDRESS, 0x8A, I2C_MEMADD_SIZE_8BIT, &a_buffer, 1, 1000); 00733 00734 /* Check the communication status */ 00735 if(status != HAL_OK) 00736 { 00737 error = (uint32_t)HAL_I2C_GetError(&hEvalI2c); 00738 00739 /* I2C error occurred */ 00740 I2Cx_Error(TS3510_I2C_ADDRESS); 00741 00742 if(error == HAL_I2C_ERROR_AF) 00743 { 00744 return 1; 00745 } 00746 } 00747 return 0; 00748 } 00749 #endif /* USE_IOEXPANDER */ 00750 00751 /******************************************************************************* 00752 BUS OPERATIONS 00753 *******************************************************************************/ 00754 00755 /******************************* I2C Routines *********************************/ 00756 /** 00757 * @brief Initializes I2C MSP. 00758 * @retval None 00759 */ 00760 static void I2Cx_MspInit(void) 00761 { 00762 GPIO_InitTypeDef gpio_init_structure; 00763 00764 /*** Configure the GPIOs ***/ 00765 /* Enable GPIO clock */ 00766 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00767 00768 /* Configure I2C Tx as alternate function */ 00769 gpio_init_structure.Pin = EVAL_I2Cx_SCL_PIN; 00770 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00771 gpio_init_structure.Pull = GPIO_NOPULL; 00772 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00773 gpio_init_structure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00774 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00775 00776 /* Configure I2C Rx as alternate function */ 00777 gpio_init_structure.Pin = EVAL_I2Cx_SDA_PIN; 00778 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00779 00780 /*** Configure the I2C peripheral ***/ 00781 /* Enable I2C clock */ 00782 EVAL_I2Cx_CLK_ENABLE(); 00783 00784 /* Force the I2C peripheral clock reset */ 00785 EVAL_I2Cx_FORCE_RESET(); 00786 00787 /* Release the I2C peripheral clock reset */ 00788 EVAL_I2Cx_RELEASE_RESET(); 00789 00790 /* Enable and set I2Cx Interrupt to a lower priority */ 00791 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x05, 0); 00792 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00793 00794 /* Enable and set I2Cx Interrupt to a lower priority */ 00795 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x05, 0); 00796 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00797 } 00798 00799 /** 00800 * @brief Initializes I2C HAL. 00801 * @retval None 00802 */ 00803 static void I2Cx_Init(void) 00804 { 00805 if(HAL_I2C_GetState(&hEvalI2c) == HAL_I2C_STATE_RESET) 00806 { 00807 hEvalI2c.Instance = EVAL_I2Cx; 00808 hEvalI2c.Init.Timing = EVAL_I2Cx_TIMING; 00809 hEvalI2c.Init.OwnAddress1 = 0; 00810 hEvalI2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00811 hEvalI2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00812 hEvalI2c.Init.OwnAddress2 = 0; 00813 hEvalI2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00814 hEvalI2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00815 00816 /* Init the I2C */ 00817 I2Cx_MspInit(); 00818 HAL_I2C_Init(&hEvalI2c); 00819 } 00820 } 00821 00822 00823 #if defined(USE_IOEXPANDER) 00824 /** 00825 * @brief Writes a single data. 00826 * @param Addr: I2C address 00827 * @param Reg: Register address 00828 * @param Value: Data to be written 00829 * @retval None 00830 */ 00831 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00832 { 00833 HAL_StatusTypeDef status = HAL_OK; 00834 00835 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00836 00837 /* Check the communication status */ 00838 if(status != HAL_OK) 00839 { 00840 /* Execute user timeout callback */ 00841 I2Cx_Error(Addr); 00842 } 00843 } 00844 00845 /** 00846 * @brief Reads a single data. 00847 * @param Addr: I2C address 00848 * @param Reg: Register address 00849 * @retval Read data 00850 */ 00851 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00852 { 00853 HAL_StatusTypeDef status = HAL_OK; 00854 uint8_t Value = 0; 00855 00856 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00857 00858 /* Check the communication status */ 00859 if(status != HAL_OK) 00860 { 00861 /* Execute user timeout callback */ 00862 I2Cx_Error(Addr); 00863 } 00864 return Value; 00865 } 00866 #endif /* USE_IOEXPANDER */ 00867 00868 /** 00869 * @brief Reads multiple data. 00870 * @param Addr: I2C address 00871 * @param Reg: Reg address 00872 * @param Buffer: Pointer to data buffer 00873 * @param Length: Length of the data 00874 * @retval Number of read data 00875 */ 00876 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00877 { 00878 HAL_StatusTypeDef status = HAL_OK; 00879 00880 if(Addr == EXC7200_I2C_ADDRESS) 00881 { 00882 status = HAL_I2C_Master_Receive(&hEvalI2c, Addr, Buffer, Length, 1000); 00883 } 00884 else 00885 { 00886 status = HAL_I2C_Mem_Read(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00887 } 00888 00889 /* Check the communication status */ 00890 if(status != HAL_OK) 00891 { 00892 /* I2C error occurred */ 00893 I2Cx_Error(Addr); 00894 } 00895 return status; 00896 } 00897 00898 /** 00899 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00900 * @param Addr: Device address on BUS Bus. 00901 * @param Reg: The target register address to write 00902 * @param pBuffer: The target register value to be written 00903 * @param Length: buffer size to be written 00904 * @retval HAL status 00905 */ 00906 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00907 { 00908 HAL_StatusTypeDef status = HAL_OK; 00909 00910 status = HAL_I2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00911 00912 /* Check the communication status */ 00913 if(status != HAL_OK) 00914 { 00915 /* Re-Initiaize the I2C Bus */ 00916 I2Cx_Error(Addr); 00917 } 00918 return status; 00919 } 00920 00921 /** 00922 * @brief Checks if target device is ready for communication. 00923 * @note This function is used with Memory devices 00924 * @param DevAddress: Target device address 00925 * @param Trials: Number of trials 00926 * @retval HAL status 00927 */ 00928 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00929 { 00930 return (HAL_I2C_IsDeviceReady(&hEvalI2c, DevAddress, Trials, 1000)); 00931 } 00932 00933 /** 00934 * @brief Manages error callback by re-initializing I2C. 00935 * @param Addr: I2C Address 00936 * @retval None 00937 */ 00938 static void I2Cx_Error(uint8_t Addr) 00939 { 00940 /* De-initialize the I2C communication bus */ 00941 HAL_I2C_DeInit(&hEvalI2c); 00942 00943 /* Re-Initialize the I2C communication bus */ 00944 I2Cx_Init(); 00945 } 00946 00947 /******************************************************************************* 00948 LINK OPERATIONS 00949 *******************************************************************************/ 00950 00951 /********************************* LINK IOE ***********************************/ 00952 #if defined(USE_IOEXPANDER) 00953 /** 00954 * @brief Initializes IOE low level. 00955 * @retval None 00956 */ 00957 void IOE_Init(void) 00958 { 00959 I2Cx_Init(); 00960 } 00961 00962 /** 00963 * @brief Configures IOE low level interrupt. 00964 * @retval None 00965 */ 00966 void IOE_ITConfig(void) 00967 { 00968 /* STMPE811 IO expander IT config done by BSP_TS_ITConfig function */ 00969 } 00970 00971 /** 00972 * @brief IOE writes single data. 00973 * @param Addr: I2C address 00974 * @param Reg: Register address 00975 * @param Value: Data to be written 00976 * @retval None 00977 */ 00978 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00979 { 00980 I2Cx_Write(Addr, Reg, Value); 00981 } 00982 00983 /** 00984 * @brief IOE reads single data. 00985 * @param Addr: I2C address 00986 * @param Reg: Register address 00987 * @retval Read data 00988 */ 00989 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00990 { 00991 return I2Cx_Read(Addr, Reg); 00992 } 00993 00994 /** 00995 * @brief IOE reads multiple data. 00996 * @param Addr: I2C address 00997 * @param Reg: Register address 00998 * @param Buffer: Pointer to data buffer 00999 * @param Length: Length of the data 01000 * @retval Number of read data 01001 */ 01002 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01003 { 01004 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01005 } 01006 01007 /** 01008 * @brief IOE writes multiple data. 01009 * @param Addr: I2C address 01010 * @param Reg: Register address 01011 * @param Buffer: Pointer to data buffer 01012 * @param Length: Length of the data 01013 * @retval None 01014 */ 01015 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01016 { 01017 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01018 } 01019 01020 /** 01021 * @brief IOE delay 01022 * @param Delay: Delay in ms 01023 * @retval None 01024 */ 01025 void IOE_Delay(uint32_t Delay) 01026 { 01027 HAL_Delay(Delay); 01028 } 01029 #endif /* USE_IOEXPANDER */ 01030 01031 /********************************* LINK MFX ***********************************/ 01032 01033 #if defined(USE_IOEXPANDER) 01034 /** 01035 * @brief Initializes MFX low level. 01036 * @retval None 01037 */ 01038 void MFX_IO_Init(void) 01039 { 01040 I2Cx_Init(); 01041 } 01042 01043 /** 01044 * @brief DeInitializes MFX low level. 01045 * @retval None 01046 */ 01047 void MFX_IO_DeInit(void) 01048 { 01049 } 01050 01051 /** 01052 * @brief Configures MFX low level interrupt. 01053 * @retval None 01054 */ 01055 void MFX_IO_ITConfig(void) 01056 { 01057 static uint8_t mfx_io_it_enabled = 0; 01058 GPIO_InitTypeDef gpio_init_structure; 01059 01060 if(mfx_io_it_enabled == 0) 01061 { 01062 mfx_io_it_enabled = 1; 01063 /* Enable the GPIO EXTI clock */ 01064 __HAL_RCC_GPIOI_CLK_ENABLE(); 01065 __HAL_RCC_SYSCFG_CLK_ENABLE(); 01066 01067 gpio_init_structure.Pin = GPIO_PIN_8; 01068 gpio_init_structure.Pull = GPIO_NOPULL; 01069 gpio_init_structure.Speed = GPIO_SPEED_LOW; 01070 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 01071 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 01072 01073 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 01074 HAL_NVIC_SetPriority((IRQn_Type)(EXTI9_5_IRQn), 0x0F, 0x0F); 01075 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI9_5_IRQn)); 01076 } 01077 } 01078 01079 /** 01080 * @brief MFX writes single data. 01081 * @param Addr: I2C address 01082 * @param Reg: Register address 01083 * @param Value: Data to be written 01084 * @retval None 01085 */ 01086 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01087 { 01088 I2Cx_Write((uint8_t) Addr, Reg, Value); 01089 } 01090 01091 /** 01092 * @brief MFX reads single data. 01093 * @param Addr: I2C address 01094 * @param Reg: Register address 01095 * @retval Read data 01096 */ 01097 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01098 { 01099 return I2Cx_Read((uint8_t) Addr, Reg); 01100 } 01101 01102 /** 01103 * @brief MFX reads multiple data. 01104 * @param Addr: I2C address 01105 * @param Reg: Register address 01106 * @param Buffer: Pointer to data buffer 01107 * @param Length: Length of the data 01108 * @retval Number of read data 01109 */ 01110 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01111 { 01112 return I2Cx_ReadMultiple((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01113 } 01114 01115 /** 01116 * @brief MFX delay 01117 * @param Delay: Delay in ms 01118 * @retval None 01119 */ 01120 void MFX_IO_Delay(uint32_t Delay) 01121 { 01122 HAL_Delay(Delay); 01123 } 01124 01125 /** 01126 * @brief Used by Lx family but requested for MFX component compatibility. 01127 * @retval None 01128 */ 01129 void MFX_IO_Wakeup(void) 01130 { 01131 } 01132 01133 /** 01134 * @brief Used by Lx family but requested for MXF component compatibility. 01135 * @retval None 01136 */ 01137 void MFX_IO_EnableWakeupPin(void) 01138 { 01139 } 01140 01141 #endif /* USE_IOEXPANDER */ 01142 01143 /********************************* LINK AUDIO *********************************/ 01144 01145 /** 01146 * @brief Initializes Audio low level. 01147 * @retval None 01148 */ 01149 void AUDIO_IO_Init(void) 01150 { 01151 I2Cx_Init(); 01152 } 01153 01154 /** 01155 * @brief Writes a single data. 01156 * @param Addr: I2C address 01157 * @param Reg: Reg address 01158 * @param Value: Data to be written 01159 * @retval None 01160 */ 01161 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01162 { 01163 uint16_t tmp = Value; 01164 01165 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01166 01167 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01168 01169 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01170 } 01171 01172 /** 01173 * @brief Reads a single data. 01174 * @param Addr: I2C address 01175 * @param Reg: Reg address 01176 * @retval Data to be read 01177 */ 01178 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01179 { 01180 uint16_t read_value = 0, tmp = 0; 01181 01182 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01183 01184 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01185 01186 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01187 01188 read_value = tmp; 01189 01190 return read_value; 01191 } 01192 01193 /** 01194 * @brief AUDIO Codec delay 01195 * @param Delay: Delay in ms 01196 * @retval None 01197 */ 01198 void AUDIO_IO_Delay(uint32_t Delay) 01199 { 01200 HAL_Delay(Delay); 01201 } 01202 01203 /********************************* LINK CAMERA ********************************/ 01204 01205 /** 01206 * @brief Initializes Camera low level. 01207 * @retval None 01208 */ 01209 void CAMERA_IO_Init(void) 01210 { 01211 I2Cx_Init(); 01212 } 01213 01214 /** 01215 * @brief Camera writes single data. 01216 * @param Addr: I2C address 01217 * @param Reg: Register address 01218 * @param Value: Data to be written 01219 * @retval None 01220 */ 01221 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01222 { 01223 uint16_t tmp = Value; 01224 /* For S5K5CAG sensor, 16 bits accesses are used */ 01225 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01226 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01227 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01228 } 01229 01230 /** 01231 * @brief Camera reads single data. 01232 * @param Addr: I2C address 01233 * @param Reg: Register address 01234 * @retval Read data 01235 */ 01236 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg) 01237 { 01238 uint16_t read_value = 0, tmp = 0; 01239 /* For S5K5CAG sensor, 16 bits accesses are used */ 01240 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01241 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01242 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01243 read_value = tmp; 01244 return read_value; 01245 } 01246 01247 /** 01248 * @brief Camera delay 01249 * @param Delay: Delay in ms 01250 * @retval None 01251 */ 01252 void CAMERA_Delay(uint32_t Delay) 01253 { 01254 HAL_Delay(Delay); 01255 } 01256 01257 /******************************** LINK I2C EEPROM *****************************/ 01258 01259 /** 01260 * @brief Initializes peripherals used by the I2C EEPROM driver. 01261 * @retval None 01262 */ 01263 void EEPROM_IO_Init(void) 01264 { 01265 I2Cx_Init(); 01266 } 01267 01268 /** 01269 * @brief Write data to I2C EEPROM driver in using DMA channel. 01270 * @param DevAddress: Target device address 01271 * @param MemAddress: Internal memory address 01272 * @param pBuffer: Pointer to data buffer 01273 * @param BufferSize: Amount of data to be sent 01274 * @retval HAL status 01275 */ 01276 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01277 { 01278 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01279 } 01280 01281 /** 01282 * @brief Read data from I2C EEPROM driver in using DMA channel. 01283 * @param DevAddress: Target device address 01284 * @param MemAddress: Internal memory address 01285 * @param pBuffer: Pointer to data buffer 01286 * @param BufferSize: Amount of data to be read 01287 * @retval HAL status 01288 */ 01289 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01290 { 01291 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01292 } 01293 01294 /** 01295 * @brief Checks if target device is ready for communication. 01296 * @note This function is used with Memory devices 01297 * @param DevAddress: Target device address 01298 * @param Trials: Number of trials 01299 * @retval HAL status 01300 */ 01301 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01302 { 01303 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01304 } 01305 01306 /** 01307 * @} 01308 */ 01309 01310 /** 01311 * @} 01312 */ 01313 01314 /** 01315 * @} 01316 */ 01317 01318 /** 01319 * @} 01320 */ 01321 01322 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri May 22 2015 13:59:20 for STM32756G_EVAL BSP User Manual by
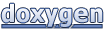