STM32469I-Discovery BSP User Manual
|
stm32469i_discovery_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32469i_discovery_sd.c 00004 * @author MCD Application Team 00005 * @version V2.0.0 00006 * @date 31-January-2017 00007 * @brief This file includes the uSD card driver mounted on STM32469I-Discovery 00008 * board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the micro SD external card mounted on STM32469I-Discovery 00044 board. 00045 - This driver does not need a specific component driver for the micro SD device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the micro SD card using the BSP_SD_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 SDIO interface configuration to interface with the external micro SD. It 00054 also includes the micro SD initialization sequence. 00055 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00056 returns the detection status 00057 o If SD presence detection interrupt mode is desired, you must configure the 00058 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00059 is generated as an external interrupt whenever the micro SD card is 00060 plugged/unplugged in/from the board. 00061 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00062 which is stored in the structure "HAL_SD_CardInfoTypeDef". 00063 00064 + Micro SD card operations 00065 o The micro SD card can be accessed with read/write block(s) operations once 00066 it is ready for access. The access can be performed whether using the polling 00067 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00068 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00069 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00070 is complete, the SD interrupt is handled using the function BSP_SD_IRQHandler(), 00071 the DMA Tx/Rx transfer complete are handled using the functions 00072 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00073 are implemented by the user at application level. 00074 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00075 the number of blocks to erase. 00076 o The SD runtime status is returned when calling the function BSP_SD_GetCardState(). 00077 00078 ------------------------------------------------------------------------------*/ 00079 00080 /* Includes ------------------------------------------------------------------*/ 00081 #include "stm32469i_discovery_sd.h" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM32469I_Discovery 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM32469I-Discovery_SD STM32469I Discovery SD 00092 * @{ 00093 */ 00094 00095 00096 /** @defgroup STM32469I-Discovery_SD_Private_TypesDefinitions STM32469I Discovery SD Private TypesDef 00097 * @{ 00098 */ 00099 /** 00100 * @} 00101 */ 00102 00103 /** @defgroup STM32469I-Discovery_SD_Private_Defines STM32469I Discovery SD Private Defines 00104 * @{ 00105 */ 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32469I-Discovery_SD_Private_Macros STM32469I Discovery SD Private Macro 00111 * @{ 00112 */ 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM32469I-Discovery_SD_Private_Variables STM32469I Discovery SD Private Variables 00118 * @{ 00119 */ 00120 SD_HandleTypeDef uSdHandle; 00121 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM32469I-Discovery_SD_Private_FunctionPrototypes STM32469I Discovery SD Private Prototypes 00127 * @{ 00128 */ 00129 /** 00130 * @} 00131 */ 00132 00133 /** @defgroup STM32469I-Discovery_SD_Private_Functions STM32469I Discovery SD Private Functions 00134 * @{ 00135 */ 00136 00137 /** 00138 * @brief Initializes the SD card device. 00139 * @retval SD status 00140 */ 00141 uint8_t BSP_SD_Init(void) 00142 { 00143 uint8_t sd_state = MSD_OK; 00144 00145 /* PLLSAI is dedicated to LCD periph. Do not use it to get 48MHz*/ 00146 00147 /* uSD device interface configuration */ 00148 uSdHandle.Instance = SDIO; 00149 00150 uSdHandle.Init.ClockEdge = SDIO_CLOCK_EDGE_RISING; 00151 uSdHandle.Init.ClockBypass = SDIO_CLOCK_BYPASS_DISABLE; 00152 uSdHandle.Init.ClockPowerSave = SDIO_CLOCK_POWER_SAVE_DISABLE; 00153 uSdHandle.Init.BusWide = SDIO_BUS_WIDE_1B; 00154 uSdHandle.Init.HardwareFlowControl = SDIO_HARDWARE_FLOW_CONTROL_ENABLE; 00155 uSdHandle.Init.ClockDiv = SDIO_TRANSFER_CLK_DIV; 00156 00157 /* Msp SD Detect pin initialization */ 00158 BSP_SD_Detect_MspInit(&uSdHandle, NULL); 00159 if(BSP_SD_IsDetected() != SD_PRESENT) /* Check if SD card is present */ 00160 { 00161 return MSD_ERROR_SD_NOT_PRESENT; 00162 } 00163 00164 /* Msp SD initialization */ 00165 BSP_SD_MspInit(&uSdHandle, NULL); 00166 00167 /* HAL SD initialization */ 00168 if(HAL_SD_Init(&uSdHandle) != HAL_OK) 00169 { 00170 sd_state = MSD_ERROR; 00171 } 00172 00173 /* Configure SD Bus width */ 00174 if(sd_state == MSD_OK) 00175 { 00176 /* Enable wide operation */ 00177 if(HAL_SD_ConfigWideBusOperation(&uSdHandle, SDIO_BUS_WIDE_4B) != HAL_OK) 00178 { 00179 sd_state = MSD_ERROR; 00180 } 00181 else 00182 { 00183 sd_state = MSD_OK; 00184 } 00185 } 00186 return sd_state; 00187 } 00188 00189 /** 00190 * @brief DeInitializes the SD card device. 00191 * @retval SD status 00192 */ 00193 uint8_t BSP_SD_DeInit(void) 00194 { 00195 uint8_t sd_state = MSD_OK; 00196 00197 uSdHandle.Instance = SDIO; 00198 00199 /* HAL SD deinitialization */ 00200 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00201 { 00202 sd_state = MSD_ERROR; 00203 } 00204 00205 /* Msp SD deinitialization */ 00206 uSdHandle.Instance = SDIO; 00207 BSP_SD_MspDeInit(&uSdHandle, NULL); 00208 00209 return sd_state; 00210 } 00211 00212 /** 00213 * @brief Configures Interrupt mode for SD detection pin. 00214 * @retval Returns 0 00215 */ 00216 uint8_t BSP_SD_ITConfig(void) 00217 { 00218 GPIO_InitTypeDef gpio_init_structure; 00219 00220 /* Configure Interrupt mode for SD detection pin */ 00221 gpio_init_structure.Pin = SD_DETECT_PIN; 00222 gpio_init_structure.Pull = GPIO_PULLUP; 00223 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00224 gpio_init_structure.Mode = GPIO_MODE_IT_RISING_FALLING; 00225 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00226 00227 /* Enable and set SD detect EXTI Interrupt to the lowest priority */ 00228 HAL_NVIC_SetPriority((IRQn_Type)(SD_DETECT_EXTI_IRQn), 0x0F, 0x00); 00229 HAL_NVIC_EnableIRQ((IRQn_Type)(SD_DETECT_EXTI_IRQn)); 00230 00231 return MSD_OK; 00232 } 00233 00234 /** 00235 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00236 * @retval Returns if SD is detected or not 00237 */ 00238 uint8_t BSP_SD_IsDetected(void) 00239 { 00240 __IO uint8_t status = SD_PRESENT; 00241 00242 /* Check SD card detect pin */ 00243 if (HAL_GPIO_ReadPin(SD_DETECT_GPIO_PORT, SD_DETECT_PIN) == GPIO_PIN_SET) 00244 { 00245 status = SD_NOT_PRESENT; 00246 } 00247 00248 return status; 00249 } 00250 00251 /** 00252 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00253 * @param pData: Pointer to the buffer that will contain the data to transmit 00254 * @param ReadAddr: Address from where data is to be read 00255 * @param NumOfBlocks: Number of SD blocks to read 00256 * @param Timeout: Timeout for read operation 00257 * @retval SD status 00258 */ 00259 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00260 { 00261 if(HAL_SD_ReadBlocks(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout) != HAL_OK) 00262 { 00263 return MSD_ERROR; 00264 } 00265 else 00266 { 00267 return MSD_OK; 00268 } 00269 } 00270 00271 /** 00272 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00273 * @param pData: Pointer to the buffer that will contain the data to transmit 00274 * @param WriteAddr: Address from where data is to be written 00275 * @param NumOfBlocks: Number of SD blocks to write 00276 * @param Timeout: Timeout for write operation 00277 * @retval SD status 00278 */ 00279 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00280 { 00281 if(HAL_SD_WriteBlocks(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout) != HAL_OK) 00282 { 00283 return MSD_ERROR; 00284 } 00285 else 00286 { 00287 return MSD_OK; 00288 } 00289 } 00290 00291 /** 00292 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00293 * @param pData: Pointer to the buffer that will contain the data to transmit 00294 * @param ReadAddr: Address from where data is to be read 00295 * @param NumOfBlocks: Number of SD blocks to read 00296 * @retval SD status 00297 */ 00298 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00299 { 00300 /* Read block(s) in DMA transfer mode */ 00301 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks) != HAL_OK) 00302 { 00303 return MSD_ERROR; 00304 } 00305 else 00306 { 00307 return MSD_OK; 00308 } 00309 } 00310 00311 /** 00312 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00313 * @param pData: Pointer to the buffer that will contain the data to transmit 00314 * @param WriteAddr: Address from where data is to be written 00315 * @param NumOfBlocks: Number of SD blocks to write 00316 * @retval SD status 00317 */ 00318 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00319 { 00320 /* Write block(s) in DMA transfer mode */ 00321 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks) != HAL_OK) 00322 { 00323 return MSD_ERROR; 00324 } 00325 else 00326 { 00327 return MSD_OK; 00328 } 00329 } 00330 00331 /** 00332 * @brief Erases the specified memory area of the given SD card. 00333 * @param StartAddr: Start byte address 00334 * @param EndAddr: End byte address 00335 * @retval SD status 00336 */ 00337 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr) 00338 { 00339 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != HAL_OK) 00340 { 00341 return MSD_ERROR; 00342 } 00343 else 00344 { 00345 return MSD_OK; 00346 } 00347 } 00348 00349 /** 00350 * @brief Initializes the SD MSP. 00351 * @param hsd: SD handle 00352 * @param Params : pointer on additional configuration parameters, can be NULL. 00353 */ 00354 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00355 { 00356 static DMA_HandleTypeDef dma_rx_handle; 00357 static DMA_HandleTypeDef dma_tx_handle; 00358 GPIO_InitTypeDef gpio_init_structure; 00359 00360 /* Enable SDIO clock */ 00361 __HAL_RCC_SDIO_CLK_ENABLE(); 00362 00363 /* Enable DMA2 clocks */ 00364 __DMAx_TxRx_CLK_ENABLE(); 00365 00366 /* Enable GPIOs clock */ 00367 __HAL_RCC_GPIOC_CLK_ENABLE(); 00368 __HAL_RCC_GPIOD_CLK_ENABLE(); 00369 00370 /* Common GPIO configuration */ 00371 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00372 gpio_init_structure.Pull = GPIO_PULLUP; 00373 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00374 gpio_init_structure.Alternate = GPIO_AF12_SDIO; 00375 00376 /* GPIOC configuration */ 00377 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00378 00379 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00380 00381 /* GPIOD configuration */ 00382 gpio_init_structure.Pin = GPIO_PIN_2; 00383 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00384 00385 /* NVIC configuration for SDIO interrupts */ 00386 HAL_NVIC_SetPriority(SDIO_IRQn, 0x0E, 0); 00387 HAL_NVIC_EnableIRQ(SDIO_IRQn); 00388 00389 /* Configure DMA Rx parameters */ 00390 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00391 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00392 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00393 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00394 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00395 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00396 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00397 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00398 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00399 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00400 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00401 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00402 00403 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00404 00405 /* Associate the DMA handle */ 00406 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00407 00408 /* Deinitialize the stream for new transfer */ 00409 HAL_DMA_DeInit(&dma_rx_handle); 00410 00411 /* Configure the DMA stream */ 00412 HAL_DMA_Init(&dma_rx_handle); 00413 00414 /* Configure DMA Tx parameters */ 00415 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00416 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00417 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00418 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00419 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00420 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00421 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00422 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00423 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00424 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00425 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00426 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00427 00428 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00429 00430 /* Associate the DMA handle */ 00431 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00432 00433 /* Deinitialize the stream for new transfer */ 00434 HAL_DMA_DeInit(&dma_tx_handle); 00435 00436 /* Configure the DMA stream */ 00437 HAL_DMA_Init(&dma_tx_handle); 00438 00439 /* NVIC configuration for DMA transfer complete interrupt */ 00440 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 0x0F, 0); 00441 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00442 00443 /* NVIC configuration for DMA transfer complete interrupt */ 00444 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 0x0F, 0); 00445 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00446 } 00447 00448 /** 00449 * @brief Initializes the SD Detect pin MSP. 00450 * @param hsd: SD handle 00451 * @param Params : pointer on additional configuration parameters, can be NULL. 00452 */ 00453 __weak void BSP_SD_Detect_MspInit(SD_HandleTypeDef *hsd, void *Params) 00454 { 00455 GPIO_InitTypeDef gpio_init_structure; 00456 00457 SD_DETECT_GPIO_CLK_ENABLE(); 00458 00459 /* GPIO configuration in input for uSD_Detect signal */ 00460 gpio_init_structure.Pin = SD_DETECT_PIN; 00461 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00462 gpio_init_structure.Pull = GPIO_PULLUP; 00463 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00464 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00465 } 00466 00467 /** 00468 * @brief DeInitializes the SD MSP. 00469 * @param hsd: SD handle 00470 * @param Params : pointer on additional configuration parameters, can be NULL. 00471 */ 00472 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00473 { 00474 static DMA_HandleTypeDef dma_rx_handle; 00475 static DMA_HandleTypeDef dma_tx_handle; 00476 00477 /* Disable NVIC for DMA transfer complete interrupts */ 00478 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00479 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00480 00481 /* Deinitialize the stream for new transfer */ 00482 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00483 HAL_DMA_DeInit(&dma_rx_handle); 00484 00485 /* Deinitialize the stream for new transfer */ 00486 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00487 HAL_DMA_DeInit(&dma_tx_handle); 00488 00489 /* Disable NVIC for SDIO interrupts */ 00490 HAL_NVIC_DisableIRQ(SDIO_IRQn); 00491 00492 /* DeInit GPIO pins can be done in the application 00493 (by surcharging this __weak function) */ 00494 00495 /* Disable SDIO clock */ 00496 __HAL_RCC_SDIO_CLK_DISABLE(); 00497 00498 /* GPOI pins clock and DMA cloks can be shut down in the applic 00499 by surcgarging this __weak function */ 00500 } 00501 00502 /** 00503 * @brief Gets the current SD card data status. 00504 * @retval Data transfer state. 00505 * This value can be one of the following values: 00506 * @arg SD_TRANSFER_OK: No data transfer is acting 00507 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00508 */ 00509 uint8_t BSP_SD_GetCardState(void) 00510 { 00511 return((HAL_SD_GetCardState(&uSdHandle) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00512 } 00513 00514 00515 /** 00516 * @brief Get SD information about specific SD card. 00517 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00518 * @retval None 00519 */ 00520 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypeDef *CardInfo) 00521 { 00522 /* Get SD card Information */ 00523 HAL_SD_GetCardInfo(&uSdHandle, CardInfo); 00524 } 00525 00526 /** 00527 * @brief SD Abort callbacks 00528 * @param hsd: SD handle 00529 * @retval None 00530 */ 00531 void HAL_SD_AbortCallback(SD_HandleTypeDef *hsd) 00532 { 00533 BSP_SD_AbortCallback(); 00534 } 00535 00536 /** 00537 * @brief Tx Transfer completed callbacks 00538 * @param hsd: SD handle 00539 * @retval None 00540 */ 00541 void HAL_SD_TxCpltCallback(SD_HandleTypeDef *hsd) 00542 { 00543 BSP_SD_WriteCpltCallback(); 00544 } 00545 00546 /** 00547 * @brief Rx Transfer completed callbacks 00548 * @param hsd: SD handle 00549 * @retval None 00550 */ 00551 void HAL_SD_RxCpltCallback(SD_HandleTypeDef *hsd) 00552 { 00553 BSP_SD_ReadCpltCallback(); 00554 } 00555 00556 /** 00557 * @brief BSP SD Abort callbacks 00558 * @retval None 00559 */ 00560 __weak void BSP_SD_AbortCallback(void) 00561 { 00562 00563 } 00564 00565 /** 00566 * @brief BSP Tx Transfer completed callbacks 00567 * @retval None 00568 */ 00569 __weak void BSP_SD_WriteCpltCallback(void) 00570 { 00571 00572 } 00573 00574 /** 00575 * @brief BSP Rx Transfer completed callbacks 00576 * @retval None 00577 */ 00578 __weak void BSP_SD_ReadCpltCallback(void) 00579 { 00580 00581 } 00582 00583 /** 00584 * @} 00585 */ 00586 00587 /** 00588 * @} 00589 */ 00590 00591 /** 00592 * @} 00593 */ 00594 00595 /** 00596 * @} 00597 */ 00598 00599 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 13 2017 11:00:15 for STM32469I-Discovery BSP User Manual by
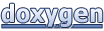