sl_mqtt_server
|
D:/Project/SimpleLink/mqtt/doxygen/server/sl_mqtt_server.h
Go to the documentation of this file.
00001 /******************************************************************************* 00002 Copyright (c) (2014) Texas Instruments Incorporated 00003 All rights reserved not granted herein. 00004 00005 Limited License. 00006 00007 Texas Instruments Incorporated grants a world-wide, royalty-free, non-exclusive 00008 license under copyrights and patents it now or hereafter owns or controls to make, 00009 have made, use, import, offer to sell and sell ("Utilize") this software subject 00010 to the terms herein. With respect to the foregoing patent license, such license 00011 is granted solely to the extent that any such patent is necessary to Utilize the 00012 software alone. The patent license shall not apply to any combinations which 00013 include this software, other than combinations with devices manufactured by or 00014 for TI (�TI Devices�). No hardware patent is licensed hereunder. 00015 00016 Redistributions must preserve existing copyright notices and reproduce this license 00017 (including the above copyright notice and the disclaimer and (if applicable) source 00018 code license limitations below) in the documentation and/or other materials provided 00019 with the distribution 00020 00021 Redistribution and use in binary form, without modification, are permitted provided 00022 that the following conditions are met: 00023 * No reverse engineering, decompilation, or disassembly of this software is 00024 permitted with respect to any software provided in binary form. 00025 * any redistribution and use are licensed by TI for use only with TI Devices. 00026 * Nothing shall obligate TI to provide you with source code for the software 00027 licensed and provided to you in object code. 00028 00029 If software source code is provided to you, modification and redistribution of the 00030 source code are permitted provided that the following conditions are met; 00031 * any redistribution and use of the source code, including any resulting derivative 00032 works, are licensed by TI for use only with TI Devices. 00033 * any redistribution and use of any object code compiled from the source code and 00034 any resulting derivative works, are licensed by TI for use only with TI Devices. 00035 00036 Neither the name of Texas Instruments Incorporated nor the names of its suppliers 00037 may be used to endorse or promote products derived from this software without 00038 specific prior written permission. 00039 00040 DISCLAIMER. 00041 00042 THIS SOFTWARE IS PROVIDED BY TI AND TI�S LICENSORS "AS IS" AND ANY EXPRESS OR IMPLIED 00043 WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY 00044 AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL TI AND TI�S 00045 LICENSORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00046 CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE 00047 GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00048 HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00049 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00050 THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00051 00052 ******************************************************************************/ 00053 00054 #include <stdio.h> 00055 #include <string.h> 00056 #include <stdbool.h> 00057 #include "simplelink.h" 00058 00059 #ifndef __SL_MQTT_SRVR_H__ 00060 #define __SL_MQTT_SRVR_H__ 00061 00062 #ifdef __cplusplus 00063 extern "C" 00064 { 00065 #endif 00066 00126 #define SL_MQTT_SRVR_EVT_PUBACK 0x11 00127 #define SL_MQTT_SRVR_EVT_NOCONN 0x12 /* End SL MQTT Server events */ 00129 00130 00141 typedef struct { 00142 00157 _u16 (*sl_ExtLib_MqttConn)(_const char *clientId_str, _i32 clientId_len, 00158 _const char *username_str, _i32 username_len, 00159 _const char *password_str, _i32 password_len, 00160 void **usr); 00161 00162 00177 void (*sl_ExtLib_MqttRecv)(_const char *topstr, _i32 toplen, 00178 _const void *payload, _i32 pay_len, 00179 bool dup, _u8 qos, 00180 bool retain); 00181 00182 // Note: Double check whether retain, dup, qos would be required for a server app (with no topic management) 00183 00192 void (*sl_ExtLib_MqttDisconn)(void *usr, bool due2err); 00193 00194 00204 void (*sl_ExtLib_MqttEvent)(void *usr, _i32 evt, _const void *buf, _u32 len); 00205 00206 } SlMqttServerCbs_t; 00207 00209 typedef struct { 00210 00211 #define SL_MQTT_SRVR_NETCONN_IP6 0x01 00212 #define SL_MQTT_SRVR_NETCONN_URL 0x02 00213 #define SL_MQTT_SRVR_NETCONN_SEC 0x04 00215 _u32 netconn_flags; 00216 _const char *server_addr; 00217 _u16 port_number; 00218 _u8 method; 00219 _u32 cipher; 00220 _u32 n_files; 00221 char * _const *secure_files; /*SL needs 4 files*/ 00222 00223 } SlMqttServerParams_t; 00224 00225 00227 typedef struct 00228 { 00229 SlMqttServerParams_t server_info; 00230 _u16 loopback_port; 00231 _u32 rx_tsk_priority; 00232 _u32 resp_time; 00233 bool aux_debug_en; 00234 _i32 (*dbg_print)(_const char *pcFormat, ...); 00235 } SlMqttServerCfg_t; 00236 00244 _i32 sl_ExtLib_MqttServerInit(_const SlMqttServerCfg_t *cfg, 00245 _const SlMqttServerCbs_t *cbs); 00246 00257 _i32 sl_ExtLib_MqttTopicEnroll(_const char *topic); 00258 00259 static inline _i32 sl_ExtLib_MqttTopicSub(_const char *topic) 00260 { 00261 return sl_ExtLib_MqttTopicEnroll(topic); 00262 } 00263 00272 _i32 sl_ExtLib_MqttTopicDisenroll(_const char *topic); 00273 00274 static inline _i32 sl_ExtLib_MqttTopicUnsub(_const char *topic) 00275 { 00276 return sl_ExtLib_MqttTopicDisenroll(topic); 00277 } 00278 00298 _i32 sl_ExtLib_MqttServerSend(_const char *topic, _const void *data, _i32 len, 00299 _u8 qos, bool retain, _u32 flags); 00300 /* End SL MQTT Server API */ 00302 00303 #ifdef __cplusplus 00304 } 00305 #endif 00306 00307 00308 00309 #endif // __SL_MQTT_SRVR_H__ 00310 00311
Generated on Thu Jan 15 2015 18:28:55 for sl_mqtt_server by
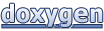