source/vcap_osd.h
Go to the documentation of this file.
00001 /** @file vcap_osd.h 00002 * 00003 * @brief This file provides the capture OSD and Mask related function calls or IOCTLs 00004 * 00005 * @author Francis Huang 00006 * @version 0.1.0 00007 * @date 2010/09/28 00008 * 00009 */ 00010 00011 /** @mainpage GM8126/GM812X Capture IOCTL and API Reference Documentation 00012 * 00013 * @n vcap_osd.h describe the capture OSD and Mask related function calls or IOCTLs 00014 */ 00015 00016 /** 00017 * @example cap_osd_string.c 00018 * @example cap_osd_mask.c 00019 */ 00020 00021 #ifndef _VCAP_OSD_H_ 00022 #define _VCAP_OSD_H_ 00023 #include <linux/ioctl.h> 00024 #include <linux/videodev.h> 00025 00026 #ifndef __KERNEL__ 00027 #include <stdint.h> 00028 #endif 00029 00030 #define MAX_TARGET 10000 00031 00032 #define X2OFFSET(base_w, x) (MAX_TARGET * x / base_w) 00033 #define Y2OFFSET(base_h, y) (MAX_TARGET * y / base_h) 00034 #define W2OFFSET(base_w, w) (MAX_TARGET * w / base_w) 00035 #define H2OFFSET(base_h, h) (MAX_TARGET * h / base_h) 00036 00037 #define OFFSET2X(base_w, off_x) (off_x * base_w / MAX_TARGET) 00038 #define OFFSET2Y(base_h, off_y) (off_y * base_h / MAX_TARGET) 00039 #define OFFSET2W(base_w, off_w) (off_w * base_w / MAX_TARGET) 00040 #define OFFSET2H(base_h, off_h) (off_h * base_h / MAX_TARGET) 00041 00042 /** 00043 * @brief This structure defines for setting OSD window postion and size. 00044 */ 00045 typedef struct fiosd_win { 00046 uint32_t x:16; ///< left position of OSD window 00047 uint32_t y:16; ///< top position of OSD window 00048 uint32_t hdim:16; ///< the dimension width of OSD window 00049 uint32_t vdim:16; ///< the dimension height of OSD window 00050 uint8_t windex; ///< the OSD window index 00051 } fiosd_win_t; 00052 00053 /** 00054 * @brief This structure defines for setting OSD font feature. 00055 * @n 00056 * @n If width and height smaller than fonts in OSD ram, the display font will be cut. 00057 * @n For example: 00058 * @n If the fonts size is 12x16 in sram, we set the fiosd_font_info as 6x8. 00059 * @n The OSD will display the quarter font from top-left. 00060 */ 00061 typedef struct fiosd_font_info { 00062 uint32_t width:8; ///< the font width that will be display 00063 uint32_t height:8; ///< the font height that will be display 00064 uint32_t row_space:8; ///< the font row space 00065 uint32_t col_space:8; ///< the font column space 00066 uint8_t windex; ///< the OSD window index 00067 } fiosd_font_info_t; 00068 00069 /** 00070 * @brief This structure defines for setting palette color. 00071 */ 00072 typedef struct fiosd_palette { 00073 uint8_t index; ///< the palette color index. The value is from 0 to 6 00074 uint8_t y; ///< the color of Y 00075 uint8_t cb; ///< the color of Cb 00076 uint8_t cr; ///< the color of Cr 00077 } fiosd_palette_t; 00078 00079 /** 00080 * @brief This structure defines for add or replace a character in OSD ram. 00081 * @n 00082 * @n The index 0 is special for FIOSDS_RMCHAR. 00083 */ 00084 typedef struct fiosd_char { 00085 char font; ///< the font index that you want to setting or replacing 00086 uint8_t fbitmap[36]; ///< the font bitmap 00087 } fiosd_char_t; 00088 00089 /** 00090 * @brief This structure defines for setting display string. 00091 * @n 00092 * @n The OSD will display string from fiosd_string->start to fiosd_string->start + 00093 * @n strlen(fiosd_string->string) in OSD display array. The string[128] is string 00094 * @n index that depend on char bit map. While using multiple OSD windows at the same 00095 * @n time, user has to manager string length in the common string buffer. 00096 */ 00097 typedef struct fiosd_string { 00098 uint8_t windex; ///< the OSD window index 00099 uint8_t start; ///< the string start index in OSD display array. 0-255 00100 uint8_t fg_color; ///< the forward color index that assign by FIOSDS_PALTCOLOR 00101 uint8_t bg_color; ///< the backward color index that assign by FIOSDS_PALTCOLOR 00102 char string[128]; ///< the string that will be displayed 00103 } fiosd_string_t; 00104 00105 /** 00106 * @brief This structure defines for setting transparent level. 00107 */ 00108 typedef struct fiosd_transparent { 00109 uint8_t windex; ///< the OSD window index 00110 uint8_t level; ///< the transparent level 0, 50, 75, 100 percent 00111 #define FOSD_TRANSPARENT_0PERCENT 0 00112 #define FOSD_TRANSPARENT_50PERCENT 1 00113 #define FOSD_TRANSPARENT_75PERCENT 2 00114 #define FOSD_TRANSPARENT_100PERCENT 3 00115 } fiosd_transparent_t; 00116 00117 /** 00118 * @brief This structure defines for getting the bit map of characters. 00119 */ 00120 typedef struct fiosd_charmap { 00121 uint32_t map[8]; ///< the bitmap of fonts that store in OSD ram 00122 } fiosd_charmap_t; 00123 00124 /** 00125 * @brief This structure defines for setting mask window postion and size 00126 */ 00127 typedef struct fiosdmask_win { 00128 uint32_t x:16; ///< left position of mask window 00129 uint32_t y:16; ///< top position of mask window 00130 uint32_t width:16; ///< the dimension width of mask window 00131 uint32_t height:16; ///< the dimension height of mask window 00132 uint8_t windex; ///< the mask window index 00133 uint8_t color; ///< the mask color index that assign by FIOSDS_PALTCOLOR 00134 } fiosdmask_win_t; 00135 00136 /** 00137 * @brief This structure defines for getting OSD hardware information. 00138 */ 00139 typedef struct fiosd_hw_info { 00140 int MaxFontNum; ///< max OSD font number 00141 int MaxDispNum; ///< max OSD display number 00142 } fiosd_hw_info_t; 00143 00144 /* Use 'f' as magic number */ 00145 #define VCAP_IOC_MAGIC 'f' 00146 00147 /* 00148 * IOCTL 00149 */ 00150 00151 /** 00152 * @brief Use to enable OSD window 00153 * @n 00154 * @n @b ioctl(osd_fd, FIOSDS_ON, &windex) 00155 * @arg parameter : 00156 * @n @b @e osd_fd : OSD device handler 00157 * @n @b @e windex : specify to enable which OSD window, from 0~3 00158 */ 00159 #define FIOSDS_ON _IOW(VCAP_IOC_MAGIC, 1, int) 00160 00161 /** 00162 * @brief Use to disable OSD window 00163 * @n 00164 * @n @b ioctl(osd_fd, FIOSDS_OFF, &windex) 00165 * @arg parameter : 00166 * @n @b @e osd_fd : OSD device handler 00167 * @n @b @e windex : specify to disable which OSD window, from 0~3 00168 */ 00169 #define FIOSDS_OFF _IOW(VCAP_IOC_MAGIC, 2, int) 00170 00171 /** 00172 * @brief Use to setup OSD window size and position 00173 * @n 00174 * @n @b ioctl(osd_fd, FIOSDS_WIN, &win) 00175 * @arg parameter : 00176 * @n @b @e osd_fd : OSD device handler 00177 * @n @b @e win : reference to fiosd_win_t structure 00178 */ 00179 #define FIOSDS_WIN _IOW(VCAP_IOC_MAGIC, 3, fiosd_win_t) 00180 00181 /** 00182 * @brief Use to setup OSD font feature 00183 * @n 00184 * @n @b ioctl(osd_fd, FIOSDS_FONTSETTING, &font_info) 00185 * @arg parameter : 00186 * @n @b @e osd_fd : OSD device handler 00187 * @n @b @e font_info : reference to fiosd_font_info_t structure 00188 */ 00189 #define FIOSDS_FONTSETTING _IOW(VCAP_IOC_MAGIC, 4, fiosd_font_info_t) 00190 00191 /** 00192 * @brief Use to setup OSD font transparent level 00193 * @n 00194 * @n @b ioctl(osd_fd, FIOSDS_TRANSPARENT, &font_tran) 00195 * @arg parameter : 00196 * @n @b @e osd_fd : OSD device handler 00197 * @n @b @e font_tran : reference to fiosd_transparent_t structure 00198 */ 00199 #define FIOSDS_TRANSPARENT _IOW(VCAP_IOC_MAGIC, 5, fiosd_transparent_t) 00200 00201 /** 00202 * @brief Use to add or replace a character in OSD ram 00203 * @n 00204 * @n @b ioctl(osd_fd, FIOSDS_CHAR, &font_char) 00205 * @arg parameter : 00206 * @n @b @e osd_fd : OSD device handler 00207 * @n @b @e font_char : reference to fiosd_char_t structure 00208 */ 00209 #define FIOSDS_CHAR _IOW(VCAP_IOC_MAGIC, 6, fiosd_char_t) 00210 00211 /** 00212 * @brief Use to setup OSD display string 00213 * @n 00214 * @n @b ioctl(osd_fd, FIOSDS_STRING, &font_string) 00215 * @arg parameter : 00216 * @n @b @e osd_fd : OSD device handler 00217 * @n @b @e font_string : reference to fiosd_string_t structure 00218 */ 00219 #define FIOSDS_STRING _IOW(VCAP_IOC_MAGIC, 7, fiosd_string_t) 00220 00221 /** 00222 * @brief Use to setup OSD palette color 00223 * @n 00224 * @n @b ioctl(osd_fd, FIOSDS_PALTCOLOR, &palette) 00225 * @arg parameter : 00226 * @n @b @e osd_fd : OSD device handler 00227 * @n @b @e palette : reference to fiosd_palette_t structure 00228 */ 00229 #define FIOSDS_PALTCOLOR _IOW(VCAP_IOC_MAGIC, 8, fiosd_palette_t) 00230 00231 /** 00232 * @brief Use to remove a character from OSD ram 00233 * @n 00234 * @n @b ioctl(osd_fd, FIOSDS_RMCHAR, &font) 00235 * @arg parameter : 00236 * @n @b @e osd_fd : OSD device handler 00237 * @n @b @e font : character index 00238 */ 00239 #define FIOSDS_RMCHAR _IOW(VCAP_IOC_MAGIC, 9, char) 00240 00241 /** 00242 * @brief Use to get the bit map of characters 00243 * @n 00244 * @n @b ioctl(osd_fd, FIOSDG_CHARMAP, &charmap) 00245 * @arg parameter : 00246 * @n @b @e osd_fd : OSD device handler 00247 * @n @b @e charmap : reference to fiosd_charmap_t structure 00248 */ 00249 #define FIOSDG_CHARMAP _IOR(VCAP_IOC_MAGIC, 10, fiosd_charmap_t) 00250 00251 /** 00252 * @brief Use to get OSD hardware information 00253 * @n 00254 * @n @b ioctl(osd_fd, FIOSDG_HWINFO, &hwinfo) 00255 * @arg parameter : 00256 * @n @b @e osd_fd : OSD device handler 00257 * @n @b @e hwinfo : reference to fiosd_hw_info_t structure 00258 */ 00259 #define FIOSDG_HWINFO _IOR(VCAP_IOC_MAGIC, 11, fiosd_hw_info_t) 00260 00261 /** 00262 * @brief Use relative OSD window postion and dimension setup control 00263 * @n 00264 * @n @b ioctl(osd_fd, FIOSDS_BWINOFFSET, &enable) 00265 * @arg parameter : 00266 * @n @b @e osd_fd : OSD device handler 00267 * @n @b @e enable : 0: disable, 1: enable 00268 */ 00269 #define FIOSDS_BWINOFFSET _IOR(VCAP_IOC_MAGIC, 12, int) 00270 00271 /** 00272 * @brief Use to enable/disable OSD force frame type 00273 * @n 00274 * @n @b ioctl(osd_fd, FIOSDS_FRAMEMODE, &enable) 00275 * @arg parameter : 00276 * @n @b @e osd_fd : OSD device handler 00277 * @n @b @e enable : 0: disable, 1: enable 00278 */ 00279 #define FIOSDS_FRAMEMODE _IOR(VCAP_IOC_MAGIC, 13, int) 00280 00281 /** 00282 * @brief Use to enable OSD mask window 00283 * @n 00284 * @n @b ioctl(osd_fd, FIOSDMASKS_ON, &windex) 00285 * @arg parameter : 00286 * @n @b @e osd_fd : OSD device handler 00287 * @n @b @e windex : specify to enable which OSD mask window, from 0~7 00288 */ 00289 #define FIOSDMASKS_ON _IOW(VCAP_IOC_MAGIC, 30, int) 00290 00291 /** 00292 * @brief Use to disable OSD mask window 00293 * @n 00294 * @n @b ioctl(osd_fd, FIOSDMASKS_OFF, &windex) 00295 * @arg parameter : 00296 * @n @b @e osd_fd : OSD device handler 00297 * @n @b @e windex : specify to disable which OSD mask window, from 0~7 00298 */ 00299 #define FIOSDMASKS_OFF _IOW(VCAP_IOC_MAGIC, 31, int) 00300 00301 /** 00302 * @brief Use to setup OSD mask transparent level 00303 * @n 00304 * @n @b ioctl(osd_fd, FIOSDMASKS_TRANSPARENT, &mask_tran) 00305 * @arg parameter : 00306 * @n @b @e osd_fd : OSD device handler 00307 * @n @b @e mask_tran : reference to fiosd_transparent_t structure 00308 */ 00309 #define FIOSDMASKS_TRANSPARENT _IOW(VCAP_IOC_MAGIC, 32, fiosd_transparent_t) 00310 00311 /** 00312 * @brief Use to setup OSD mask window size and position 00313 * @n 00314 * @n @b ioctl(osd_fd, FIOSDMASKS_WIN, &win) 00315 * @arg parameter : 00316 * @n @b @e osd_fd : OSD device handler 00317 * @n @b @e win : reference to fiosdmask_win_t structure 00318 */ 00319 #define FIOSDMASKS_WIN _IOW(VCAP_IOC_MAGIC, 33, fiosdmask_win_t) 00320 00321 /** 00322 * @brief Use relative OSD mask window postion and dimension setup control 00323 * @n 00324 * @n @b ioctl(osd_fd, FIOSDMASKS_BWINOFFSET, &enable) 00325 * @arg parameter : 00326 * @n @b @e osd_fd : OSD device handler 00327 * @n @b @e enable : 0: disable, 1: enable 00328 */ 00329 #define FIOSDMASKS_BWINOFFSET _IOW(VCAP_IOC_MAGIC, 34, int) 00330 #endif /* _VCAP_OSD_H_ */
Generated on Thu Nov 25 2010 17:50:15 for GM8126/GM812X Capture IOCTL and API Reference by
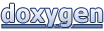