xrefutil.h
Go to the documentation of this file.00001 // Copyright (C) 2000 by Autodesk, Inc. 00002 // 00003 // Permission to use, copy, modify, and distribute this software in 00004 // object code form for any purpose and without fee is hereby granted, 00005 // provided that the above copyright notice appears in all copies and 00006 // that both that copyright notice and the limited warranty and 00007 // restricted rights notice below appear in all supporting 00008 // documentation. 00009 // 00010 // AUTODESK PROVIDES THIS PROGRAM "AS IS" AND WITH ALL FAULTS. 00011 // AUTODESK SPECIFICALLY DISCLAIMS ANY IMPLIED WARRANTY OF 00012 // MERCHANTABILITY OR FITNESS FOR A PARTICULAR USE. AUTODESK, INC. 00013 // DOES NOT WARRANT THAT THE OPERATION OF THE PROGRAM WILL BE 00014 // UNINTERRUPTED OR ERROR FREE. 00015 // 00016 // Use, duplication, or disclosure by the U.S. Government is subject to 00017 // restrictions set forth in FAR 52.227-19 (Commercial Computer 00018 // Software - Restricted Rights) and DFAR 252.227-7013(c)(1)(ii) 00019 // (Rights in Technical Data and Computer Software), as applicable. 00021 // XREFUTIL.H 00022 // 00023 // DESCR: 00024 // 00025 // 00026 // CHANGE LOG: 00027 // 03/2000 : DY : Created 00028 // 00030 00031 #ifndef __XREFUTIL__H 00032 #define __XREFUTIL__H 00033 00035 // includes 00037 00038 #include "Max.h" 00039 #include "resource.h" 00040 #include "istdplug.h" 00041 #include "iparamb2.h" 00042 #include "iparamm2.h" 00043 00044 #include "utilapi.h" 00045 00046 extern TCHAR *GetString(int id); 00047 00048 extern HINSTANCE hInstance; 00049 00050 // TODO: Change ClassID if re-used 00051 #define XREFUTIL_CLASS_ID Class_ID(0x1b3e9d52, 0x582bf699) 00052 00053 00055 // Xrefutil class declaration -- our main utility plugin class 00057 00058 class Xrefutil : public UtilityObj 00059 { 00060 00061 public: 00062 00063 IUtil * m_pUtil; 00064 Interface * m_pInterface; 00065 HWND m_hPanel; // Windows handle of our UI 00066 00067 // some "cache" member vars for the xref obj dialog 00068 Tab<TSTR *> m_objnamesholder; 00069 TSTR m_picknameholder; 00070 bool m_proxyholder; 00071 bool m_ignoreanimholder; 00072 00073 00074 // Constructor/Destructor 00075 Xrefutil(); 00076 ~Xrefutil(); 00077 00078 // from UtilityObj 00079 void BeginEditParams(Interface *ip,IUtil *iu); 00080 void EndEditParams(Interface *ip,IUtil *iu); 00081 void DeleteThis(); 00082 00083 // internal (public) utility methods 00084 void Init(HWND hWnd); 00085 void Destroy(HWND hWnd); 00086 00087 00088 bool DoOpenSaveDialog(TSTR &fileName, bool bOpen = false); 00089 bool DoPickObjDialog(); 00090 00091 // xref utility methods 00092 00093 // (xref scene methods) 00094 00095 void AddNewXrefScene(HWND hWnd); 00096 void ConvertSelectedToXrefScene(HWND hWnd); 00097 void RefreshAllXrefScenes(HWND hWnd); 00098 void MergeAllXrefScenes(HWND hWnd); 00099 00100 // (xref object methods) 00101 00102 void NodeToXref(INode * pNode, TSTR &filename, bool bProxy, bool bIgnoreAnim = false); 00103 void DeleteAllAnimation(ReferenceTarget *ref); 00104 void AddNewXrefObject(HWND hWnd); 00105 void ConvertSelectedToXrefObject(HWND hWnd); 00106 void ExportXrefObjects(HWND hWnd); 00107 00108 }; 00109 00110 static Xrefutil theXrefutil; 00111 00112 00114 // Handy enumerator base classes 00116 00117 class NodeEnumerator 00118 { 00119 protected: 00120 bool m_continue; 00121 00122 public: 00123 00124 NodeEnumerator() : m_continue(true) {}; 00125 virtual ~NodeEnumerator() {}; 00126 00127 void reset() { m_continue = true; }; 00128 00129 virtual bool Proc(INode * pNode) = 0; // return true if should continue processing 00130 00131 virtual void Enumerate(INode * pNode, bool procfirst = false) 00132 { 00133 if (procfirst) { 00134 m_continue = Proc(pNode); 00135 } 00136 for (int c = 0; c < pNode->NumberOfChildren(); c++) { 00137 if (m_continue) Enumerate(pNode->GetChildNode(c)); 00138 } 00139 if (!procfirst && m_continue) { 00140 m_continue = Proc(pNode); 00141 } 00142 }; 00143 }; 00144 00145 00151 00152 class NodeFlagger : public NodeEnumerator 00153 { 00154 protected: 00155 int m_flag; 00156 bool m_clear; 00157 00158 public: 00159 00160 NodeFlagger() : m_flag(0), m_clear(false) {}; 00161 00162 00169 NodeFlagger(int flag) { m_flag = flag; m_clear = false; }; 00170 00171 virtual void set_flag(int flag) { m_flag = flag; }; 00172 virtual void set_clear(bool clear) { m_clear = clear; }; 00173 00174 virtual bool Proc(INode * pNode) 00175 { m_clear ? pNode->ClearAFlag(m_flag) : pNode->SetAFlag(m_flag); return true; }; 00176 00177 }; 00178 00179 00180 00181 00182 #endif // __XREFUTIL__H
Generated at Mon Nov 6 14:11:58 2000 by
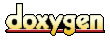